### 單一職責原則(SRP) **一個元件應該只有一個改變的理由,這意味著它應該只有一項工作。** #### 範例:使用者設定檔元件 **應該這樣:** - 將職責分解為更小的功能元件。 ``` // UserProfile.js const UserProfile = ({ user }) => { return ( <div> <UserAvatar user={user} /> <UserInfo user={user} /> </div> ); }; // UserAvatar.js const UserAvatar = ({ user }) => { return <img src={user.avatarUrl} alt={`${user.name}'s avatar`} />; }; // UserInfo.js const UserInfo = ({ user }) => { return ( <div> <h1>{user.name}</h1> <p>{user.bio}</p> </div> ); }; ``` **不要這樣:** - 將顯示、資料取得和業務邏輯組合在一個元件中。 ``` // IncorrectUserProfile.js const IncorrectUserProfile = ({ user }) => { // Fetching data, handling business logic and displaying all in one const handleEdit = () => { console.log("Edit user"); }; return ( <div> <img src={user.avatarUrl} alt={`${user.name}'s avatar`} /> <h1>{user.name}</h1> <p>{user.bio}</p> <button onClick={handleEdit}>Edit User</button> </div> ); }; ``` ### 開閉原理 (OCP) **軟體實體應該對擴充開放,但對修改關閉。** #### 範例:主題按鈕 **應該這樣:** - 使用 props 來擴充元件功能,而無需修改原始元件。 ``` // Button.js const Button = ({ onClick, children, style }) => { return ( <button onClick={onClick} style={style}> {children} </button> ); }; // Usage const PrimaryButton = (props) => { const primaryStyle = { backgroundColor: 'blue', color: 'white' }; return <Button {...props} style={primaryStyle} />; }; ``` **不要這樣:** - 修改原有元件程式碼,直接加入新的樣式或行為。 ``` // IncorrectButton.js // Modifying the original Button component directly for a specific style const Button = ({ onClick, children, primary }) => { const style = primary ? { backgroundColor: 'blue', color: 'white' } : null; return ( <button onClick={onClick} style={style}> {children} </button> ); }; ``` ### 里氏替換原理 (LSP) **超類別的物件可以用其子類別的物件替換,而不會破壞應用程式。** #### 範例:基本按鈕和圖示按鈕 **應該這樣:** - 確保子類元件可以無縫替換超類元件。 ``` // BasicButton.js const BasicButton = ({ onClick, children }) => { return <button onClick={onClick}>{children}</button>; }; // IconButton.js const IconButton = ({ onClick, icon, children }) => { return ( <button onClick={onClick}> <img src={icon} alt="icon" /> {children} </button> ); }; ``` **不要這樣:** - 引入特定於子類別的屬性,這些屬性在替換時會破壞功能。 ``` // IncorrectIconButton.js // This button expects an icon and does not handle the absence of one, breaking when used as a BasicButton const IncorrectIconButton = ({ onClick, icon }) => { if (!icon) { throw new Error("Icon is required"); } return ( <button onClick={onClick}> <img src={icon} alt="icon" /> </button> ); }; ``` ### 介面隔離原則(ISP) **任何客戶端都不應該被迫依賴它不使用的方法。** #### 範例:文字元件 **應該這樣:** - 針對不同的用途提供特定的介面。 ``` // Text.js const Text = ({ type, children }) => { switch (type) { case 'header': return <h1>{children}</h1>; case 'title': return <h2>{children}</h2>; default: return <p>{children}</p>; } }; ``` **不要這樣:** - 用不必要的屬性使元件變得混亂。 ``` // IncorrectText.js // This component expects multiple unrelated props, cluttering the interface const IncorrectText = ({ type, children, onClick, isLoggedIn }) => { if (isLoggedIn && onClick) { return <a href="#" onClick={onClick}>{children}</a>; } return type === 'header' ? <h1>{children}</h1> : <p>{children}</p>; }; ``` ### 依賴倒置原則(DIP) **高層模組不應該依賴低層模組。兩者都應該依賴抽象。** #### 範例:資料獲取 **應該這樣:** - 使用鉤子或類似的模式來抽象資料獲取 和狀態管理。 ``` // useUserData.js (Abstraction) const useUserData = (userId) => { const [user, setUser] = useState(null); useEffect(() => { fetchData(userId).then(setUser); }, [userId]); return user; }; // UserProfile.js const UserProfile = ({ userId }) => { const user = useUserData(userId); if (!user) return <p>Loading...</p>; return <div><h1>{user.name}</h1></div>; }; ``` **不要這樣:** - 在元件內部硬編碼資料取得。 ``` // IncorrectUserProfile.js const IncorrectUserProfile = ({ userId }) => { const [user, setUser] = useState(null); useEffect(() => { // Fetching data directly inside the component fetch(`https://api.example.com/users/${userId}`) .then(response => response.json()) .then(setUser); }, [userId]); if (!user) return <p>Loading...</p>; return <div><h1>{user.name}</h1></div>; }; ``` --- 原文出處:https://dev.to/drruvari/mastering-solid-principles-in-react-easy-examples-and-best-practices-142b
**長話短說** -------- 在本文中,您將了解如何建立由 AI 驅動的前端 UI 元件產生器,該產生器使您能夠透過實作教學產生 Next.js Tailwind CSS UI 元件。 我們將介紹如何: - 使用 Next.js、TypeScript 和 Tailwind CSS 建立 UI 元件產生器 Web 應用程式。 - 使用 CopilotKit 將 AI 功能整合到 UI 元件產生器中。 - 整合嵌入式程式碼編輯器以變更產生的程式碼。 先決條件 ---- 要完全理解本教程,您需要對 React 或 Next.js 有基本的了解。 以下是建立 AI 支援的 UI 元件產生器所需的工具: - [Ace 程式碼編輯器](https://ace.c9.io/)- 用 JvaScript 編寫的嵌入式程式碼編輯器,與本機編輯器的功能和效能相符。 - [Langchain](https://www.langchain.com/) - 提供了一個框架,使人工智慧代理能夠搜尋網路並研究任何主題。 - [OpenAI API](https://platform.openai.com/api-keys) - 提供 API 金鑰,讓您能夠使用 ChatGPT 模型執行各種任務。 - [Tavily AI](https://tavily.com/) - 一個搜尋引擎,使人工智慧代理能夠在應用程式中進行研究並存取即時知識。 - [CopilotKit](https://github.com/CopilotKit) - 一個開源副駕駛框架,用於建立自訂 AI 聊天機器人、應用程式內 AI 代理程式和文字區域。 專案設定和套件安裝 --------- 首先,透過在終端機中執行以下程式碼片段來建立 Next.js 應用程式: ``` npx create-next-app@latest aiuigenerator ``` 選擇您首選的配置設定。在本教學中,我們將使用 TypeScript 和 Next.js App Router。 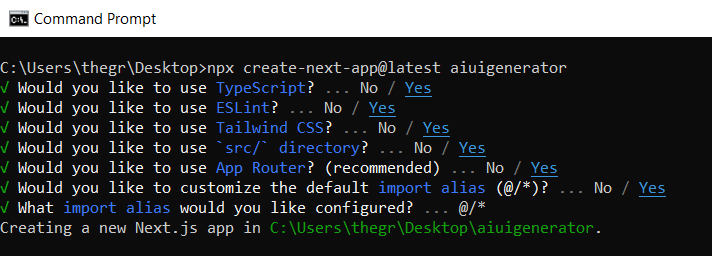 接下來,安裝 Ace 程式碼編輯器和 Langchain 軟體套件及其相依性。 ``` npm install react-ace @langchain/langgraph ``` 最後,安裝 CopilotKit 軟體套件。這些套件使我們能夠從 React 狀態檢索資料並將 AI copilot 新增至應用程式。 ``` npm install @copilotkit/react-ui @copilotkit/react-textarea @copilotkit/react-core @copilotkit/backend ``` 恭喜!您現在已準備好建立由人工智慧驅動的部落格。 **建構 UI 元件產生器前端** ----------------- 在本節中,我將引導您完成使用靜態內容建立 UI 元件產生器前端的過程,以定義生成器的使用者介面。 首先,請在程式碼編輯器中前往`/[root]/src/app`並建立一個名為`components`的資料夾。在 Components 資料夾中,建立兩個名為`Header.tsx`和`CodeTutorial.tsx`的檔案。 在`Header.tsx`檔案中,新增以下程式碼,定義一個名為`Header`的功能元件,該元件將呈現生成器的導覽列。 ``` "use client"; import Link from "next/link"; export default function Header() { return ( <> <header className="flex flex-wrap sm:justify-start sm:flex-nowrap z-50 w-full bg-gray-800 border-b border-gray-200 text-sm py-3 sm:py-0 "> <nav className="relative max-w-7xl w-full mx-auto px-4 sm:flex sm:items-center sm:justify-between sm:px-6 lg:px-8" aria-label="Global"> <div className="flex items-center justify-between"> <Link className="w-full flex-none text-xl text-white font-semibold p-6" href="/" aria-label="Brand"> AI-UI-Components-Generator </Link> </div> </nav> </header> </> ); } ``` 在`CodeTutorial.tsx`檔案中,加入以下程式碼,定義一個名為`CodeTutorial`的功能元件,該元件呈現 UI 元件產生器主頁,該首頁將顯示產生的 UI 元件、嵌入式程式碼編輯器和產生的實作教學。 ``` "use client"; import Markdown from "react-markdown"; import { useState } from "react"; import AceEditor from "react-ace"; import React from "react"; export default function CodeTutorial() { const [code, setCode] = useState<string[]>([ `<h1 class="text-red-500">Hello World</h1>`, ]); const [codeToDisplay, setCodeToDisplay] = useState<string>(code[0] || ""); const [codeTutorial, setCodeTutorial] = useState(``); function onChange(newCode: any) { setCodeToDisplay(newCode); } return ( <> <main className=" min-h-screen px-4"> <div className="w-full h-full min-h-[70vh] flex justify-between gap-x-1 "> <div className="w-2/3 min-h-[60vh] rounded-lg bg-white shadow-lg p-2 border mt-8 overflow-auto"> <div className="w-full min-h-[60vh] rounded-lg" dangerouslySetInnerHTML={{ __html: codeToDisplay }} /> </div> <AceEditor placeholder="Placeholder Text" mode="html" theme="monokai" name="blah2" className="w-[50%] min-h-[60vh] p-2 mt-8 rounded-lg" onChange={onChange} fontSize={14} lineHeight={19} showPrintMargin={true} showGutter={true} highlightActiveLine={true} value={codeToDisplay} setOptions={{ enableBasicAutocompletion: true, enableLiveAutocompletion: true, enableSnippets: false, showLineNumbers: true, tabSize: 2, }} /> </div> <div className="w-10/12 mx-auto"> <div className="mt-8"> <h1 className="text-white text-center text-xl font-semibold p-6"> Code Tutorial </h1> {codeTutorial ? ( <Markdown className="text-white">{codeTutorial}</Markdown> ) : ( <div className="text-white"> The Code Tutorial Will Appear Here </div> )} </div> </div> </main> </> ); } ``` 接下來,前往`/[root]/src/page.tsx`文件,新增以下程式碼,導入`CodeTutorial`和`Header`元件,並定義名為`Home`的功能元件。 ``` import React from "react"; import Header from "./components/Header"; import CodeTutorial from "./components/CodeTutorial"; export default function Home() { return ( <> <Header /> <CodeTutorial /> </> ); } ``` 接下來,刪除 globals.css 檔案中的 CSS 程式碼並新增以下 CSS 程式碼。 ``` @tailwind base; @tailwind components; @tailwind utilities; @tailwind base; @tailwind components; @tailwind utilities; body { height: 100vh; background-color: rgb(16, 23, 42); } pre { margin: 1rem; padding: 1rem; border-radius: 10px; background-color: black; overflow: auto; } h2, p { padding-bottom: 1rem; padding-top: 1rem; } code { margin-bottom: 2rem; } ``` 最後,在命令列上執行命令`npm run dev` ,然後導航到 http://localhost:3000/。 現在您應該在瀏覽器上查看 UI 元件產生器前端,如下所示。 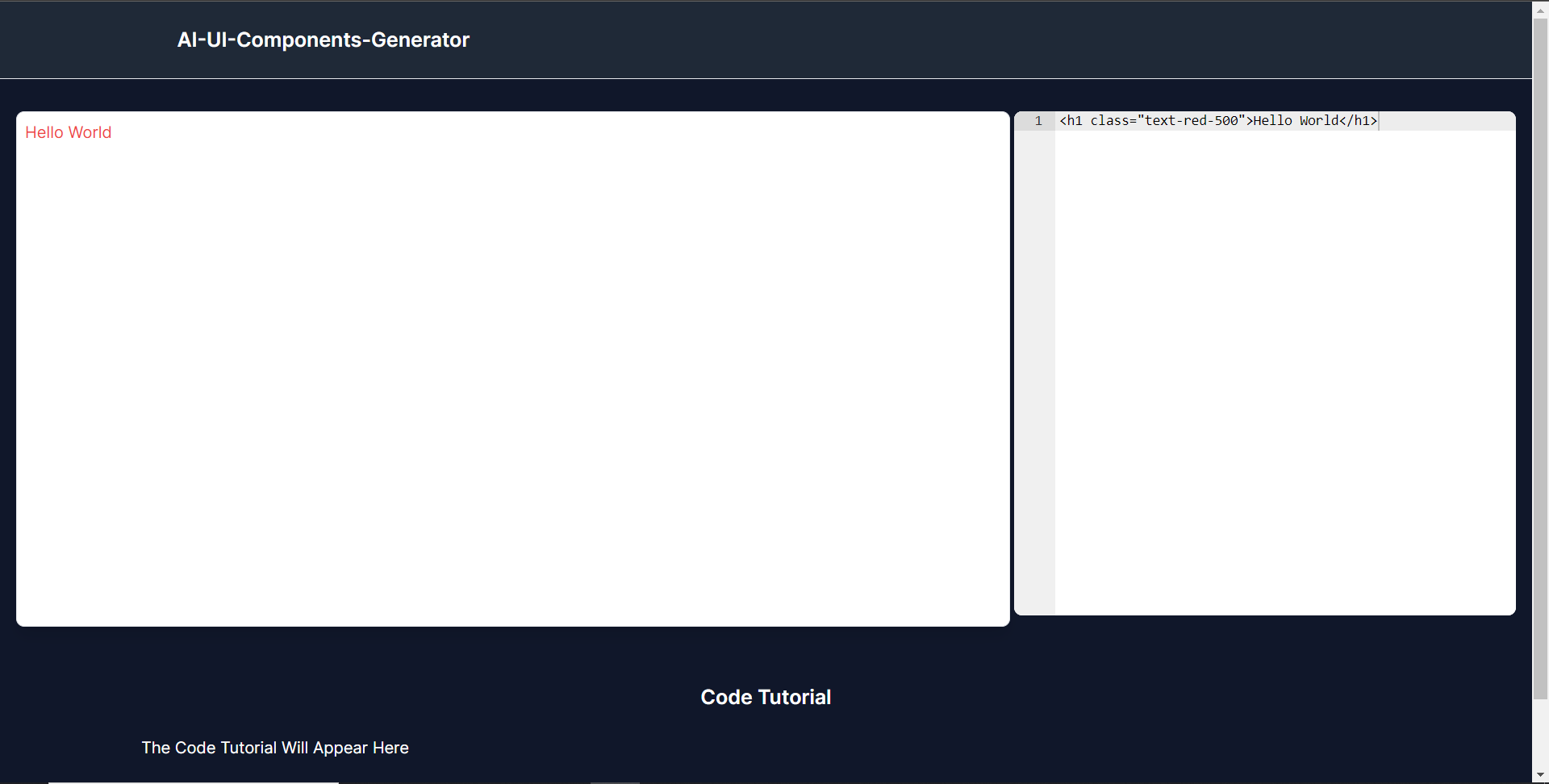 **使用 CopilotKit 將 AI 功能整合到元件產生器** --------------------------------- 在本節中,您將學習如何為 UI 元件產生器新增 AI 副駕駛以產生 UI 元件程式碼以及使用 CopilotKit 的實作教學。 CopilotKit 提供前端和[後端](https://docs.copilotkit.ai/getting-started/quickstart-backend)套件。它們使您能夠插入 React 狀態並使用 AI 代理在後端處理應用程式資料。 首先,讓我們將 CopilotKit React 元件加入到部落格前端。 ### **將 CopilotKit 新增至部落格前端** 在這裡,我將引導您完成將 UI 元件產生器與 CopilotKit 前端整合的過程,以方便產生 UI 元件程式碼和實作教學。 首先,使用下面的程式碼片段導入`/[root]/src/app/components/CodeTutorial.tsx`檔案頂部的自訂掛鉤`useMakeCopilotReadable`和`useCopilotAction` 。 ``` import { useCopilotAction, useMakeCopilotReadable, } from "@copilotkit/react-core"; ``` 在`CodeTutorial`函數內的狀態變數下方,加入以下程式碼,該程式碼使用`useMakeCopilotReadable`掛鉤來新增將作為應用程式內聊天機器人的上下文產生的程式碼。該鉤子使副駕駛可以讀取程式碼。 ``` useMakeCopilotReadable(codeToDisplay); ``` 在上面的程式碼下方,新增以下程式碼,該程式碼使用`useCopilotAction`掛鉤來設定名為`generateCodeAndImplementationTutorial`的操作,該操作將啟用 UI 元件程式碼和實作教學課程的產生。 這個操作接受兩個參數,稱為`code`和`tutorial` ,這兩個參數可以產生 UI 元件程式碼和實作教程。 該操作包含一個處理函數,該函數根據給定的提示產生 UI 元件程式碼和實作教程。 在處理函數內部, `codeToDisplay`狀態會使用新產生的程式碼進行更新,而`codeTutorial`狀態會使用新產生的教學課程進行更新,如下所示。 ``` useCopilotAction( { name: "generateCodeAndImplementationTutorial", description: "Create Code Snippet with React.js(Next.js), tailwindcss and an implementation tutorial of the code generated.", parameters: [ { name: "code", type: "string", description: "Code to be generated", required: true, }, { name: "tutorial", type: "string", description: "Markdown of step by step guide tutorial on how to use the generated code accompanied with the code. Include introduction, prerequisites and what happens at every step accompanied with code generated earlier. Don't forget to add how to render the code on browser.", required: true, }, ], handler: async ({ code, tutorial }) => { setCode((prev) => [...prev, code]); setCodeToDisplay(code); setCodeTutorial(tutorial); }, }, [codeToDisplay, codeTutorial] ); ``` 之後,請前往`/[root]/src/app/page.tsx`檔案並使用下面的程式碼匯入頂部的 CopilotKit 前端套件和樣式。 ``` import { CopilotKit } from "@copilotkit/react-core"; import { CopilotSidebar } from "@copilotkit/react-ui"; import "@copilotkit/react-ui/styles.css"; ``` 然後使用`CopilotKit`包裝`CopilotSidebar`和`CodeTutorial`元件,如下所示。 `CopilotKit`元件指定 CopilotKit 後端端點 ( `/api/copilotkit/` ) 的 URL,而`CopilotSidebar`呈現應用程式內聊天機器人,您可以提示產生 UI 元件程式碼和實作教學。 ``` export default function Home() { return ( <> <Header /> <CopilotKit url="/api/copilotkit"> <CopilotSidebar instructions="Help the user generate code. Ask the user if to generate its tutorial." defaultOpen={true} labels={{ title: "Code & Tutorial Generator", initial: "Hi! 👋 I can help you generate code and its tutorial.", }}> <CodeTutorial /> </CopilotSidebar> </CopilotKit> </> ); } ``` 之後,執行開發伺服器並導航到 http://localhost:3000。您應該會看到應用程式內聊天機器人已整合到 UI 元件產生器中。 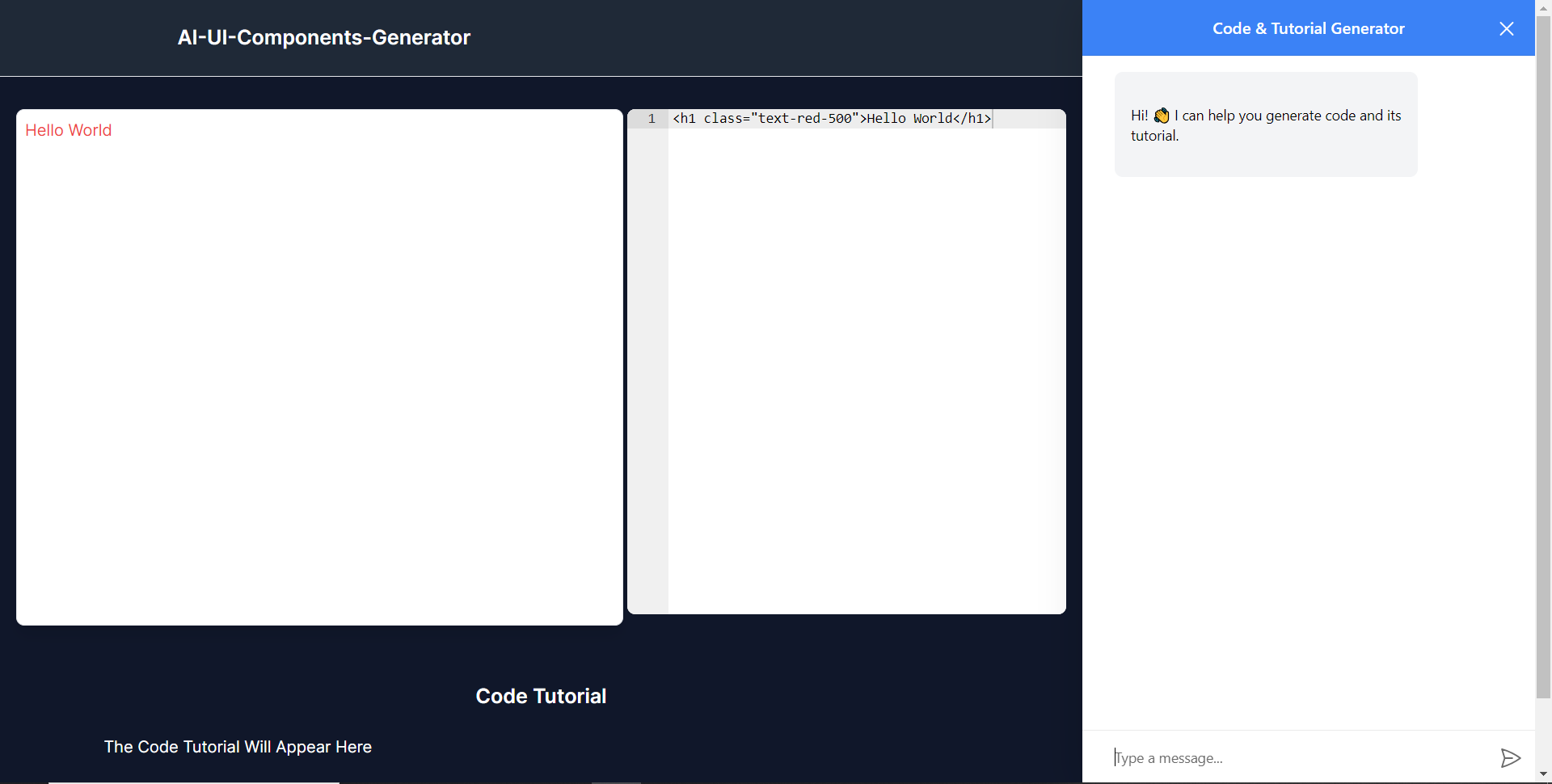 ### **將 CopilotKit 後端加入博客** 在這裡,我將引導您完成將 UI 元件產生器與 CopilotKit 後端整合的過程,該後端處理來自前端的請求,並提供函數呼叫和各種 LLM 後端(例如 GPT)。 此外,我們將整合一個名為 Tavily 的人工智慧代理,它可以研究網路上的任何主題。 首先,在根目錄中建立一個名為`.env.local`的檔案。然後在保存`ChatGPT`和`Tavily` Search API 金鑰的檔案中加入下面的環境變數。 ``` OPENAI_API_KEY="Your ChatGPT API key" TAVILY_API_KEY="Your Tavily Search API key" ``` 若要取得 ChatGPT API 金鑰,請導覽至 https://platform.openai.com/api-keys。 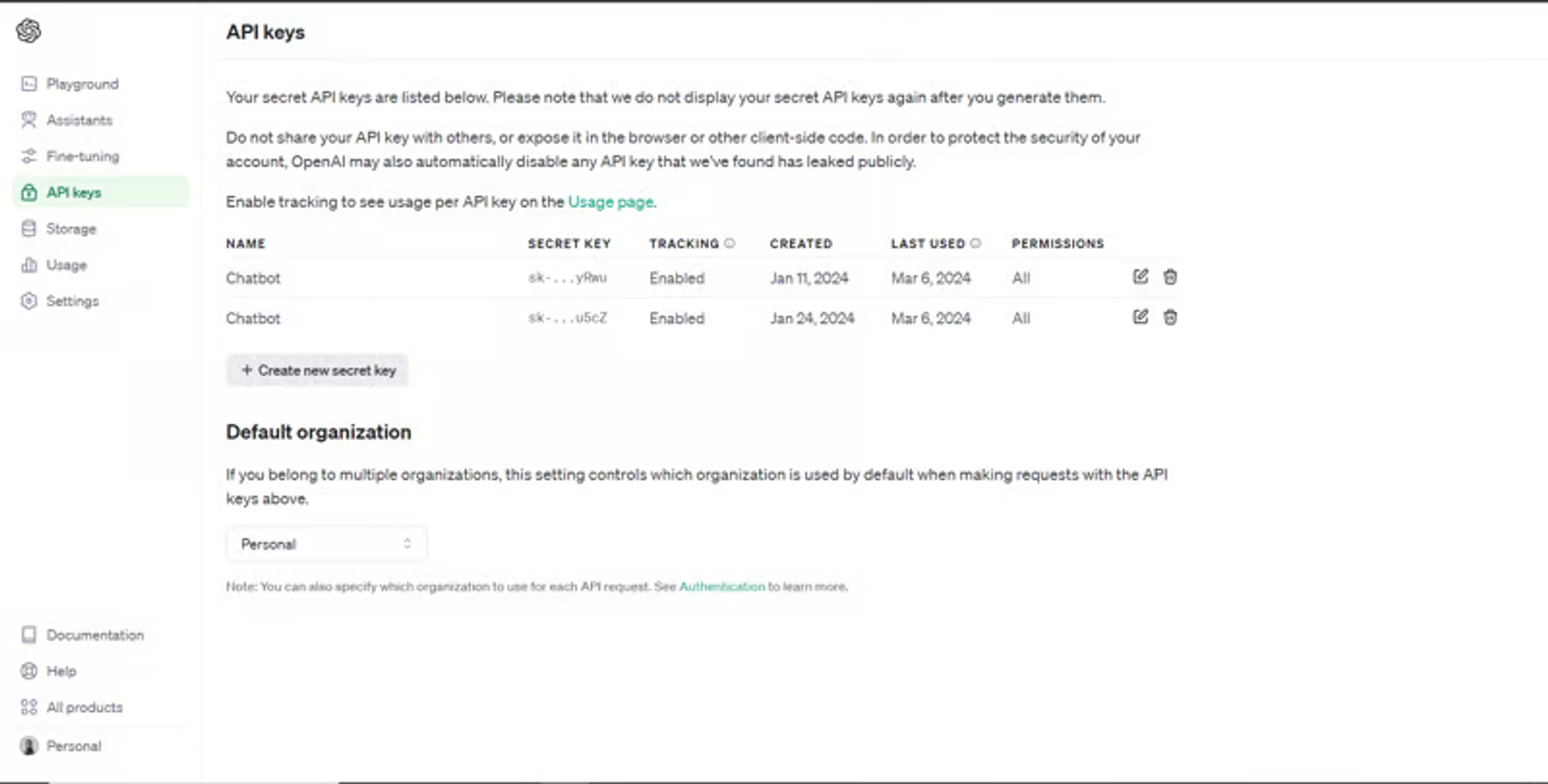 若要取得 Tavilly Search API 金鑰,請導覽至 https://app.tavily.com/home 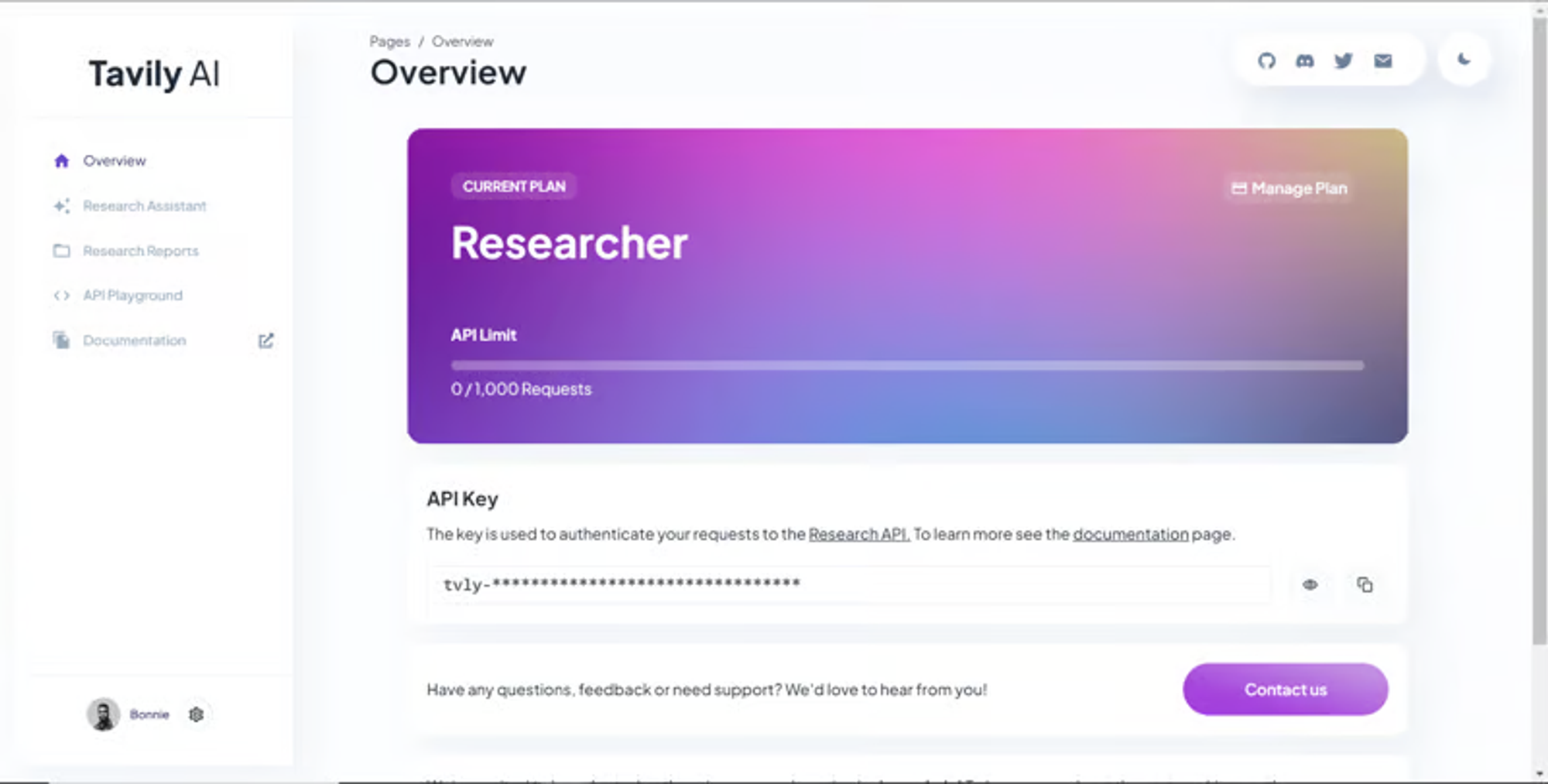 之後,轉到`/[root]/src/app`並建立一個名為`api`的資料夾。在`api`資料夾中,建立一個名為`copilotkit`的資料夾。 在`copilotkit`資料夾中,建立一個名為`research.ts`的檔案。然後導航到[該 Research.ts gist 文件](https://gist.github.com/TheGreatBonnie/58dc21ebbeeb8cbb08df665db762738c),複製程式碼,並將其新增至**`research.ts`**檔案中 接下來,在`/[root]/src/app/api/copilotkit`資料夾中建立一個名為`route.ts`的檔案。該文件將包含設定後端功能來處理 POST 請求的程式碼。它有條件地包括對給定主題進行研究的“研究”操作。 現在在文件頂部導入以下模組。 ``` import { CopilotBackend, OpenAIAdapter } from "@copilotkit/backend"; // For backend functionality with CopilotKit. import { researchWithLangGraph } from "./research"; // Import a custom function for conducting research. import { AnnotatedFunction } from "@copilotkit/shared"; // For annotating functions with metadata. ``` 在上面的程式碼下面,定義一個執行時間環境變數和一個名為`researchAction`的函數,該函數使用下面的程式碼研究某個主題。 ``` // Define a runtime environment variable, indicating the environment where the code is expected to run. export const runtime = "edge"; // Define an annotated function for research. This object includes metadata and an implementation for the function. const researchAction: AnnotatedFunction<any> = { name: "research", // Function name. description: "Call this function to conduct research on a certain topic. Respect other notes about when to call this function", // Function description. argumentAnnotations: [ // Annotations for arguments that the function accepts. { name: "topic", // Argument name. type: "string", // Argument type. description: "The topic to research. 5 characters or longer.", // Argument description. required: true, // Indicates that the argument is required. }, ], implementation: async (topic) => { // The actual function implementation. console.log("Researching topic: ", topic); // Log the research topic. return await researchWithLangGraph(topic); // Call the research function and return its result. }, }; ``` 然後在上面的程式碼下加入下面的程式碼來定義處理POST請求的非同步函數。 ``` // Define an asynchronous function that handles POST requests. export async function POST(req: Request): Promise<Response> { const actions: AnnotatedFunction<any>[] = []; // Initialize an array to hold actions. // Check if a specific environment variable is set, indicating access to certain functionality. if (process.env.TAVILY_API_KEY) { actions.push(researchAction); // Add the research action to the actions array if the condition is true. } // Instantiate CopilotBackend with the actions defined above. const copilotKit = new CopilotBackend({ actions: actions, }); // Use the CopilotBackend instance to generate a response for the incoming request using an OpenAIAdapter. return copilotKit.response(req, new OpenAIAdapter()); } ``` 如何產生 UI 元件 ---------- 現在轉到您之前整合的應用程式內聊天機器人,並給它一個提示,例如「產生聯絡表單」。 生成完成後,您應該會看到生成的聯絡表單元件及其實作教程,如下所示。您也可以使用嵌入式程式碼編輯器修改產生的程式碼。 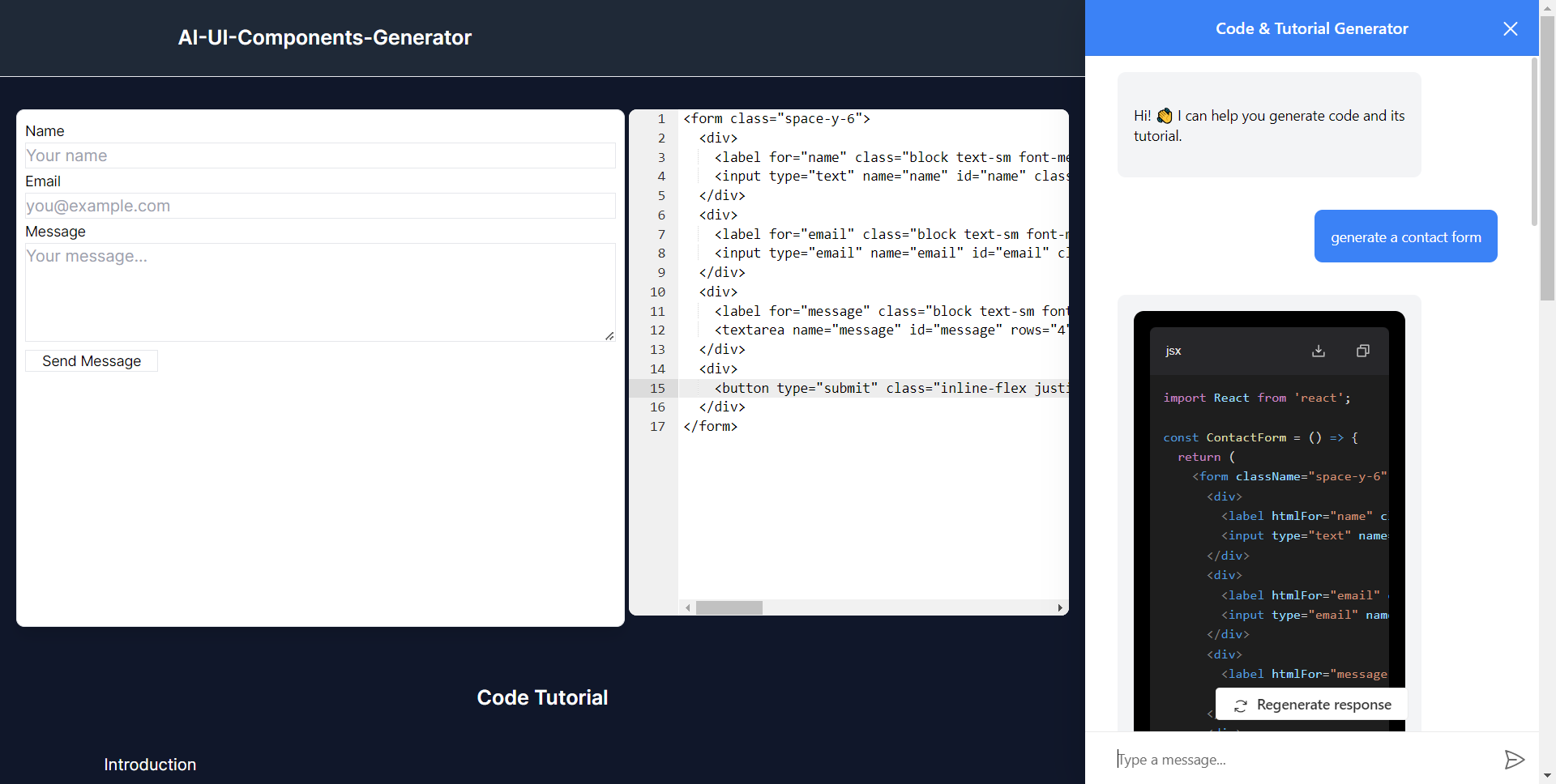 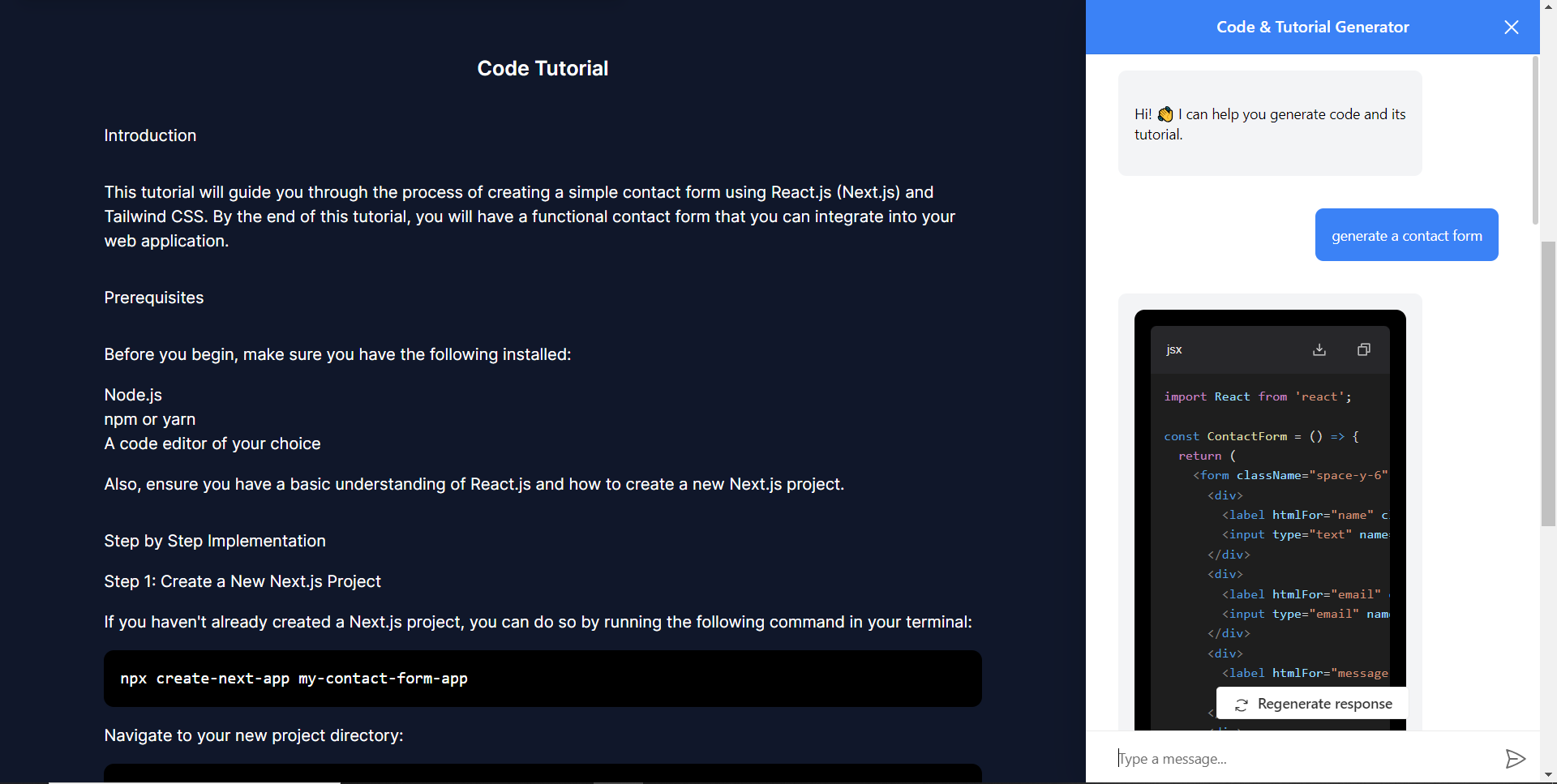 恭喜!您已完成本教學的專案。 結論 -- [CopilotKit](https://copilotkit.ai/)是一款令人難以置信的工具,可讓您在幾分鐘內將 AI Copilot 加入到您的產品中。無論您是對人工智慧聊天機器人和助理感興趣,還是對複雜任務的自動化感興趣,CopilotKit 都能讓您輕鬆實現。 如果您需要建立 AI 產品或將 AI 工具整合到您的軟體應用程式中,您應該考慮 CopilotKit。 您可以在 GitHub 上找到本教學的源程式碼: <https://github.com/TheGreatBonnie/AIPoweredUIComponentsGenerator> --- 原文出處:https://dev.to/tcms/ai-powered-frontend-ui-components-generator-nextjs-gpt4-langchain-copilotkit-1hac
**長話短說** -------- 在本文中,您將了解如何建立由 AI 驅動的前端 UI 元件產生器,該產生器使您能夠透過實作教學產生 Next.js Tailwind CSS UI 元件。 我們將介紹如何: - 使用 Next.js、TypeScript 和 Tailwind CSS 建立 UI 元件產生器 Web 應用程式。 - 使用 CopilotKit 將 AI 功能整合到 UI 元件產生器中。 - 整合嵌入式程式碼編輯器以變更產生的程式碼。 先決條件 ---- 要完全理解本教程,您需要對 React 或 Next.js 有基本的了解。 以下是建立 AI 支援的 UI 元件產生器所需的工具: - [Ace 程式碼編輯器](https://ace.c9.io/)- 用 JvaScript 編寫的嵌入式程式碼編輯器,與本機編輯器的功能和效能相符。 - [Langchain](https://www.langchain.com/) - 提供了一個框架,使人工智慧代理能夠搜尋網路並研究任何主題。 - [OpenAI API](https://platform.openai.com/api-keys) - 提供 API 金鑰,讓您能夠使用 ChatGPT 模型執行各種任務。 - [Tavily AI](https://tavily.com/) - 一個搜尋引擎,使人工智慧代理能夠在應用程式中進行研究並存取即時知識。 - [CopilotKit](https://github.com/CopilotKit) - 一個開源副駕駛框架,用於建立自訂 AI 聊天機器人、應用程式內 AI 代理程式和文字區域。 專案設定和套件安裝 --------- 首先,透過在終端機中執行以下程式碼片段來建立 Next.js 應用程式: ``` npx create-next-app@latest aiuigenerator ``` 選擇您首選的配置設定。在本教學中,我們將使用 TypeScript 和 Next.js App Router。 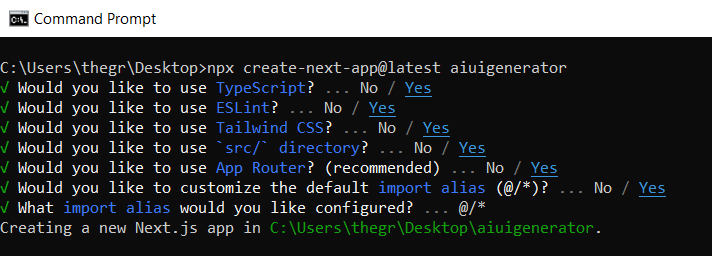 接下來,安裝 Ace 程式碼編輯器和 Langchain 軟體套件及其相依性。 ``` npm install react-ace @langchain/langgraph ``` 最後,安裝 CopilotKit 軟體套件。這些套件使我們能夠從 React 狀態檢索資料並將 AI copilot 新增至應用程式。 ``` npm install @copilotkit/react-ui @copilotkit/react-textarea @copilotkit/react-core @copilotkit/backend ``` 恭喜!您現在已準備好建立由人工智慧驅動的部落格。 **建構 UI 元件產生器前端** ----------------- 在本節中,我將引導您完成使用靜態內容建立 UI 元件產生器前端的過程,以定義生成器的使用者介面。 首先,請在程式碼編輯器中前往`/[root]/src/app`並建立一個名為`components`的資料夾。在 Components 資料夾中,建立兩個名為`Header.tsx`和`CodeTutorial.tsx`的檔案。 在`Header.tsx`檔案中,新增以下程式碼,定義一個名為`Header`的功能元件,該元件將呈現生成器的導覽列。 ``` "use client"; import Link from "next/link"; export default function Header() { return ( <> <header className="flex flex-wrap sm:justify-start sm:flex-nowrap z-50 w-full bg-gray-800 border-b border-gray-200 text-sm py-3 sm:py-0 "> <nav className="relative max-w-7xl w-full mx-auto px-4 sm:flex sm:items-center sm:justify-between sm:px-6 lg:px-8" aria-label="Global"> <div className="flex items-center justify-between"> <Link className="w-full flex-none text-xl text-white font-semibold p-6" href="/" aria-label="Brand"> AI-UI-Components-Generator </Link> </div> </nav> </header> </> ); } ``` 在`CodeTutorial.tsx`檔案中,加入以下程式碼,定義一個名為`CodeTutorial`的功能元件,該元件呈現 UI 元件產生器主頁,該首頁將顯示產生的 UI 元件、嵌入式程式碼編輯器和產生的實作教學。 ``` "use client"; import Markdown from "react-markdown"; import { useState } from "react"; import AceEditor from "react-ace"; import React from "react"; export default function CodeTutorial() { const [code, setCode] = useState<string[]>([ `<h1 class="text-red-500">Hello World</h1>`, ]); const [codeToDisplay, setCodeToDisplay] = useState<string>(code[0] || ""); const [codeTutorial, setCodeTutorial] = useState(``); function onChange(newCode: any) { setCodeToDisplay(newCode); } return ( <> <main className=" min-h-screen px-4"> <div className="w-full h-full min-h-[70vh] flex justify-between gap-x-1 "> <div className="w-2/3 min-h-[60vh] rounded-lg bg-white shadow-lg p-2 border mt-8 overflow-auto"> <div className="w-full min-h-[60vh] rounded-lg" dangerouslySetInnerHTML={{ __html: codeToDisplay }} /> </div> <AceEditor placeholder="Placeholder Text" mode="html" theme="monokai" name="blah2" className="w-[50%] min-h-[60vh] p-2 mt-8 rounded-lg" onChange={onChange} fontSize={14} lineHeight={19} showPrintMargin={true} showGutter={true} highlightActiveLine={true} value={codeToDisplay} setOptions={{ enableBasicAutocompletion: true, enableLiveAutocompletion: true, enableSnippets: false, showLineNumbers: true, tabSize: 2, }} /> </div> <div className="w-10/12 mx-auto"> <div className="mt-8"> <h1 className="text-white text-center text-xl font-semibold p-6"> Code Tutorial </h1> {codeTutorial ? ( <Markdown className="text-white">{codeTutorial}</Markdown> ) : ( <div className="text-white"> The Code Tutorial Will Appear Here </div> )} </div> </div> </main> </> ); } ``` 接下來,前往`/[root]/src/page.tsx`文件,新增以下程式碼,導入`CodeTutorial`和`Header`元件,並定義名為`Home`的功能元件。 ``` import React from "react"; import Header from "./components/Header"; import CodeTutorial from "./components/CodeTutorial"; export default function Home() { return ( <> <Header /> <CodeTutorial /> </> ); } ``` 接下來,刪除 globals.css 檔案中的 CSS 程式碼並新增以下 CSS 程式碼。 ``` @tailwind base; @tailwind components; @tailwind utilities; @tailwind base; @tailwind components; @tailwind utilities; body { height: 100vh; background-color: rgb(16, 23, 42); } pre { margin: 1rem; padding: 1rem; border-radius: 10px; background-color: black; overflow: auto; } h2, p { padding-bottom: 1rem; padding-top: 1rem; } code { margin-bottom: 2rem; } ``` 最後,在命令列上執行命令`npm run dev` ,然後導航到 http://localhost:3000/。 現在您應該在瀏覽器上查看 UI 元件產生器前端,如下所示。 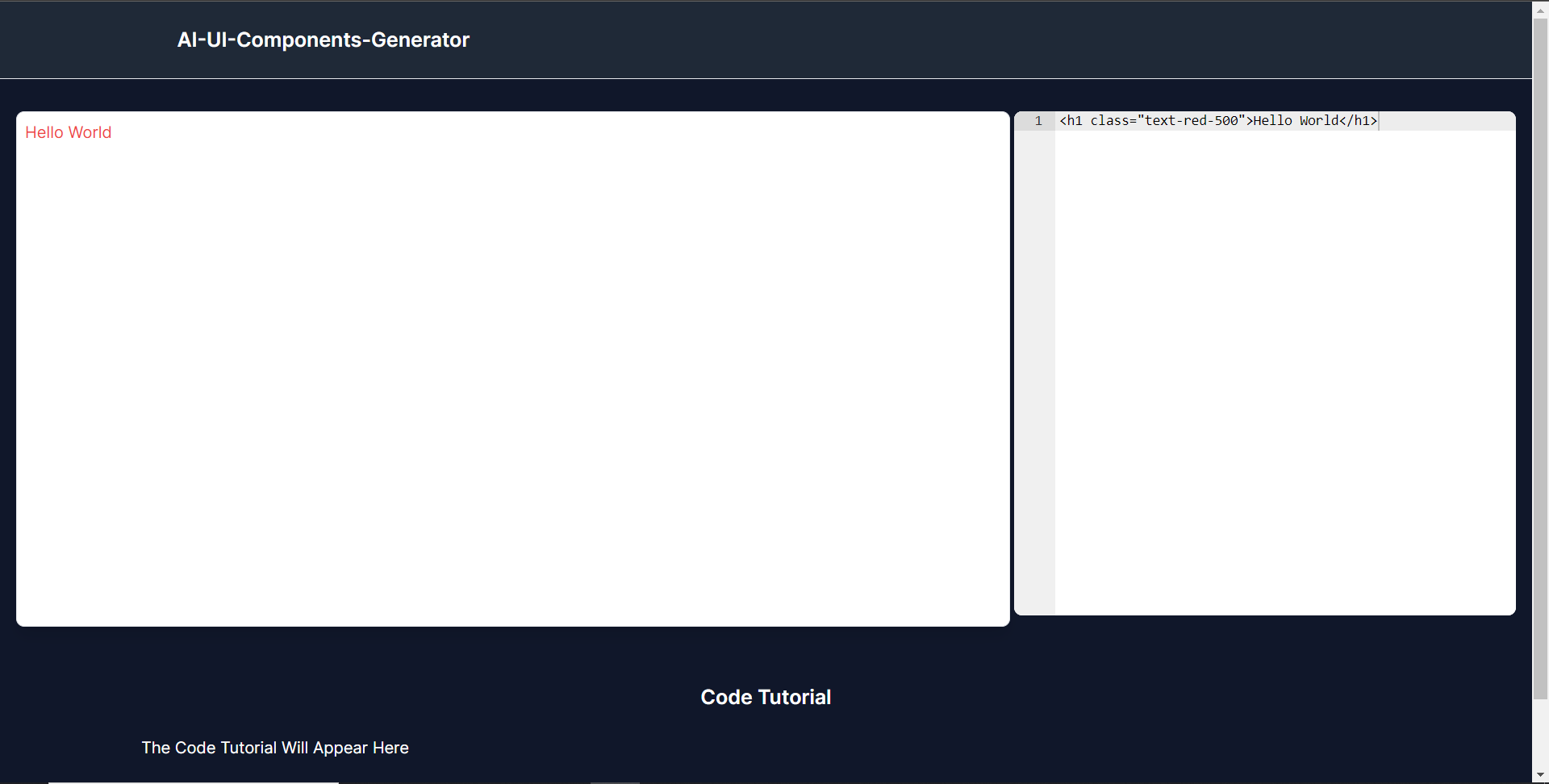 **使用 CopilotKit 將 AI 功能整合到元件產生器** --------------------------------- 在本節中,您將學習如何為 UI 元件產生器新增 AI 副駕駛以產生 UI 元件程式碼以及使用 CopilotKit 的實作教學。 CopilotKit 提供前端和[後端](https://docs.copilotkit.ai/getting-started/quickstart-backend)套件。它們使您能夠插入 React 狀態並使用 AI 代理在後端處理應用程式資料。 首先,我們將 CopilotKit React 元件加入到部落格前端。 ### **將 CopilotKit 新增至部落格前端** 在這裡,我將引導您完成將 UI 元件產生器與 CopilotKit 前端整合的過程,以方便產生 UI 元件程式碼和實作教學。 首先,使用下面的程式碼片段導入`/[root]/src/app/components/CodeTutorial.tsx`檔案頂部的自訂掛鉤`useMakeCopilotReadable`和`useCopilotAction` 。 ``` import { useCopilotAction, useMakeCopilotReadable, } from "@copilotkit/react-core"; ``` 在`CodeTutorial`函數內的狀態變數下方,加入以下程式碼,該程式碼使用`useMakeCopilotReadable`掛鉤來新增將作為應用程式內聊天機器人的上下文產生的程式碼。該鉤子使副駕駛可以讀取程式碼。 ``` useMakeCopilotReadable(codeToDisplay); ``` 在上面的程式碼下方,新增以下程式碼,該程式碼使用`useCopilotAction`掛鉤來設定名為`generateCodeAndImplementationTutorial`的操作,該操作將啟用 UI 元件程式碼和實作教學課程的產生。 這個操作接受兩個參數,稱為`code`和`tutorial` ,這兩個參數可以產生 UI 元件程式碼和實作教程。 該操作包含一個處理函數,該函數根據給定的提示產生 UI 元件程式碼和實作教程。 在處理函數內部, `codeToDisplay`狀態會使用新產生的程式碼進行更新,而`codeTutorial`狀態會使用新產生的教學課程進行更新,如下所示。 ``` useCopilotAction( { name: "generateCodeAndImplementationTutorial", description: "Create Code Snippet with React.js(Next.js), tailwindcss and an implementation tutorial of the code generated.", parameters: [ { name: "code", type: "string", description: "Code to be generated", required: true, }, { name: "tutorial", type: "string", description: "Markdown of step by step guide tutorial on how to use the generated code accompanied with the code. Include introduction, prerequisites and what happens at every step accompanied with code generated earlier. Don't forget to add how to render the code on browser.", required: true, }, ], handler: async ({ code, tutorial }) => { setCode((prev) => [...prev, code]); setCodeToDisplay(code); setCodeTutorial(tutorial); }, }, [codeToDisplay, codeTutorial] ); ``` 之後,請前往`/[root]/src/app/page.tsx`檔案並使用下面的程式碼匯入頂部的 CopilotKit 前端套件和樣式。 ``` import { CopilotKit } from "@copilotkit/react-core"; import { CopilotSidebar } from "@copilotkit/react-ui"; import "@copilotkit/react-ui/styles.css"; ``` 然後使用`CopilotKit`包裝`CopilotSidebar`和`CodeTutorial`元件,如下所示。 `CopilotKit`元件指定 CopilotKit 後端端點 ( `/api/copilotkit/` ) 的 URL,而`CopilotSidebar`呈現應用程式內聊天機器人,您可以提示產生 UI 元件程式碼和實作教學。 ``` export default function Home() { return ( <> <Header /> <CopilotKit url="/api/copilotkit"> <CopilotSidebar instructions="Help the user generate code. Ask the user if to generate its tutorial." defaultOpen={true} labels={{ title: "Code & Tutorial Generator", initial: "Hi! 👋 I can help you generate code and its tutorial.", }}> <CodeTutorial /> </CopilotSidebar> </CopilotKit> </> ); } ``` 之後,執行開發伺服器並導航到 http://localhost:3000。您應該會看到應用程式內聊天機器人已整合到 UI 元件產生器中。 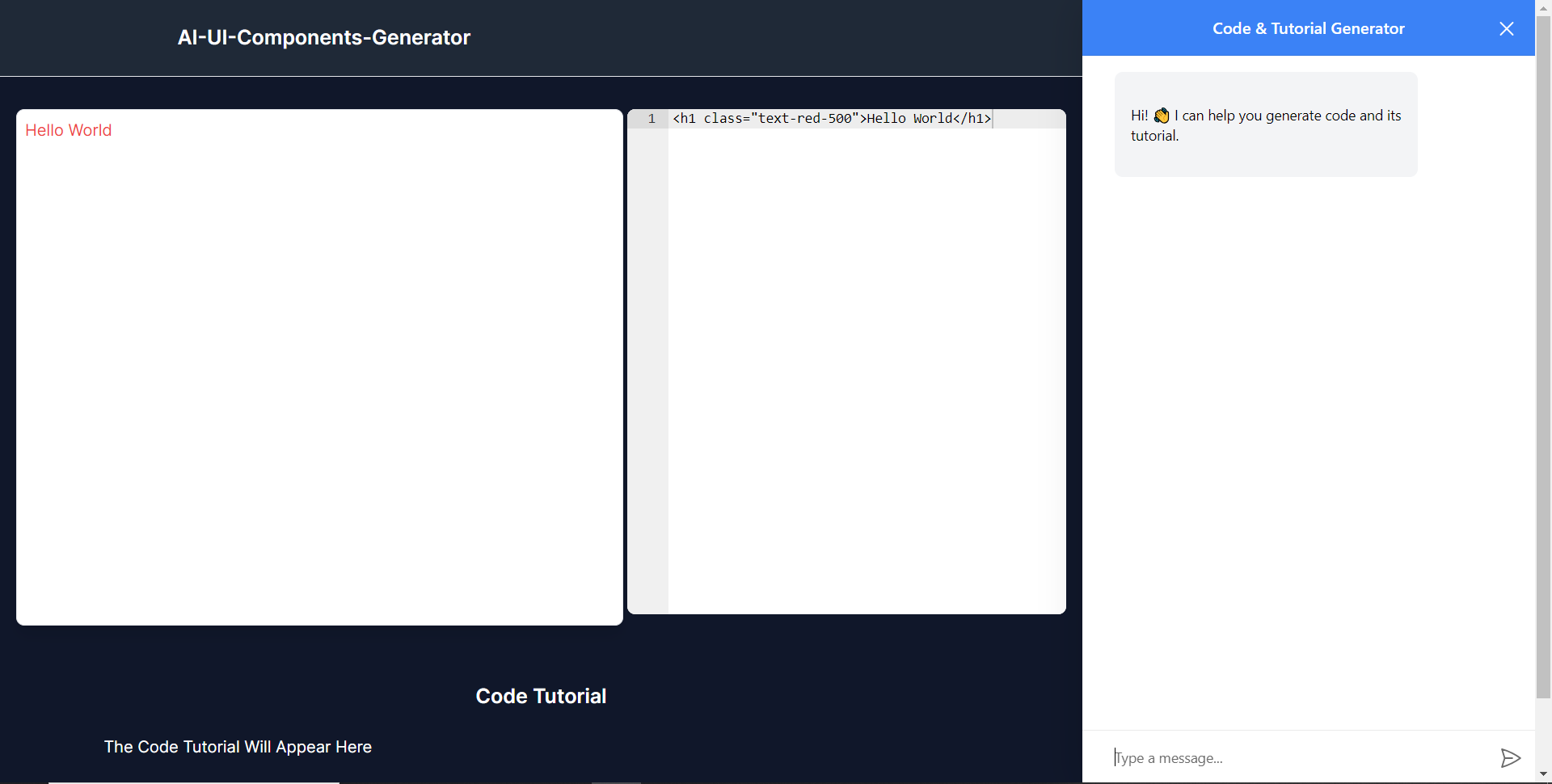 ### **將 CopilotKit 後端加入博客** 在這裡,我將引導您完成將 UI 元件產生器與 CopilotKit 後端整合的過程,該後端處理來自前端的請求,並提供函數呼叫和各種 LLM 後端(例如 GPT)。 此外,我們將整合一個名為 Tavily 的人工智慧代理,它可以研究網路上的任何主題。 首先,在根目錄中建立一個名為`.env.local`的檔案。然後在保存`ChatGPT`和`Tavily` Search API 金鑰的檔案中加入下面的環境變數。 ``` OPENAI_API_KEY="Your ChatGPT API key" TAVILY_API_KEY="Your Tavily Search API key" ``` 若要取得 ChatGPT API 金鑰,請導覽至 https://platform.openai.com/api-keys。 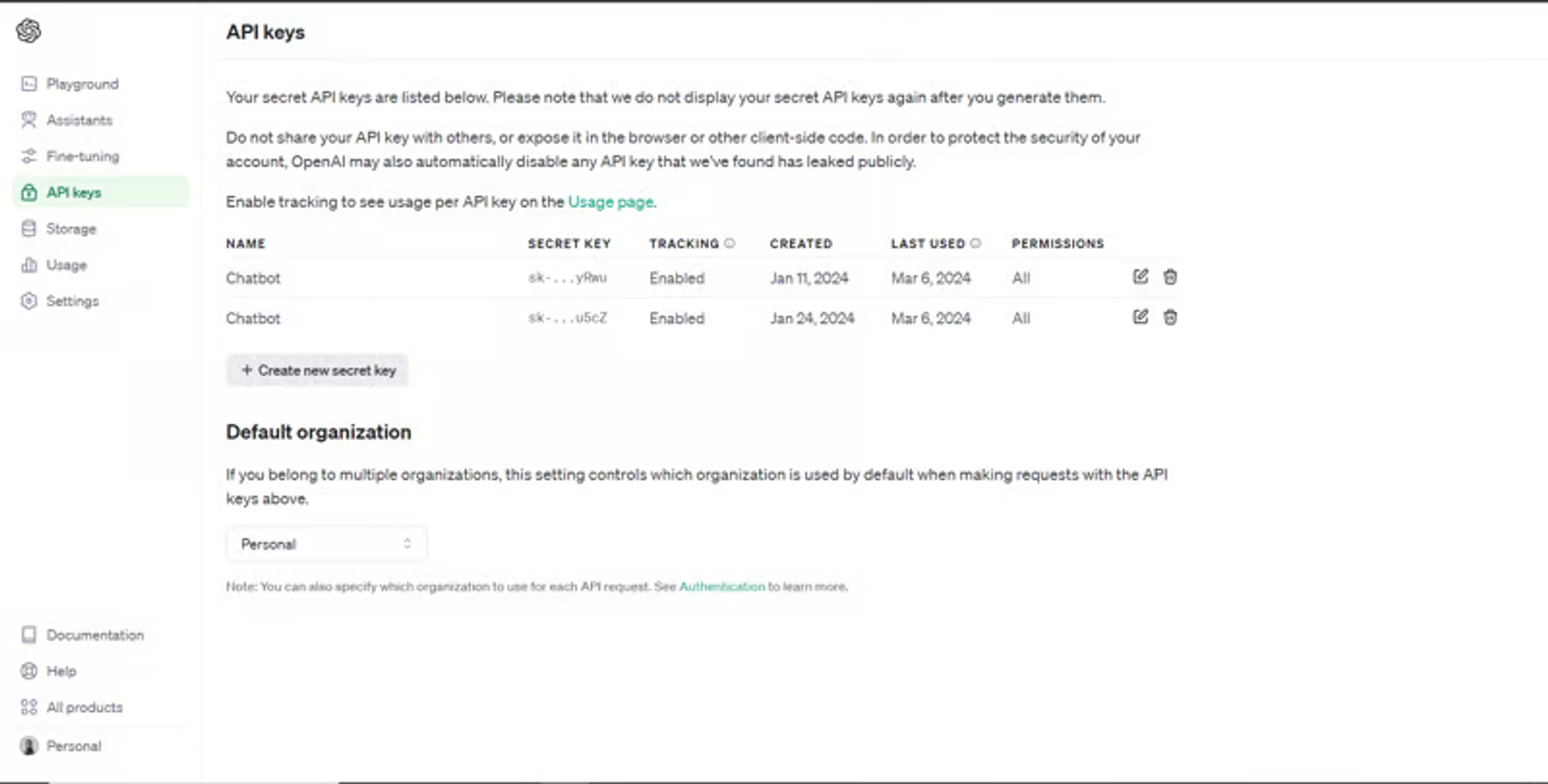 若要取得 Tavilly Search API 金鑰,請導覽至 https://app.tavily.com/home 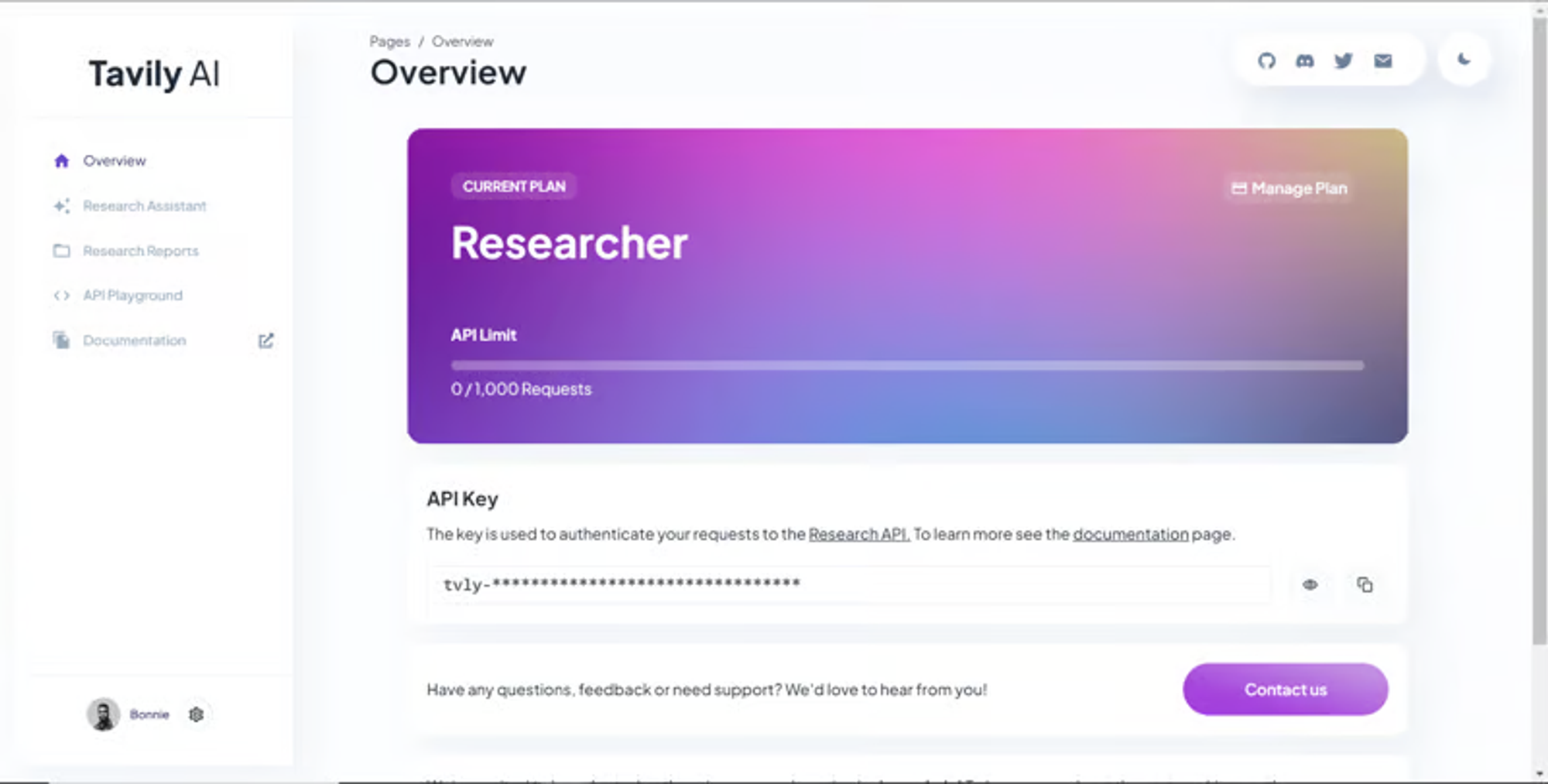 之後,轉到`/[root]/src/app`並建立一個名為`api`的資料夾。在`api`資料夾中,建立一個名為`copilotkit`的資料夾。 在`copilotkit`資料夾中,建立一個名為`research.ts`的檔案。然後導航到[該 Research.ts gist 文件](https://gist.github.com/TheGreatBonnie/58dc21ebbeeb8cbb08df665db762738c),複製程式碼,並將其新增至**`research.ts`**檔案中 接下來,在`/[root]/src/app/api/copilotkit`資料夾中建立一個名為`route.ts`的檔案。該文件將包含設定後端功能來處理 POST 請求的程式碼。它有條件地包括對給定主題進行研究的“研究”操作。 現在在文件頂部導入以下模組。 ``` import { CopilotBackend, OpenAIAdapter } from "@copilotkit/backend"; // For backend functionality with CopilotKit. import { researchWithLangGraph } from "./research"; // Import a custom function for conducting research. import { AnnotatedFunction } from "@copilotkit/shared"; // For annotating functions with metadata. ``` 在上面的程式碼下面,定義一個執行時間環境變數和一個名為`researchAction`的函數,該函數使用下面的程式碼研究某個主題。 ``` // Define a runtime environment variable, indicating the environment where the code is expected to run. export const runtime = "edge"; // Define an annotated function for research. This object includes metadata and an implementation for the function. const researchAction: AnnotatedFunction<any> = { name: "research", // Function name. description: "Call this function to conduct research on a certain topic. Respect other notes about when to call this function", // Function description. argumentAnnotations: [ // Annotations for arguments that the function accepts. { name: "topic", // Argument name. type: "string", // Argument type. description: "The topic to research. 5 characters or longer.", // Argument description. required: true, // Indicates that the argument is required. }, ], implementation: async (topic) => { // The actual function implementation. console.log("Researching topic: ", topic); // Log the research topic. return await researchWithLangGraph(topic); // Call the research function and return its result. }, }; ``` 然後在上面的程式碼下加入下面的程式碼來定義處理POST請求的非同步函數。 ``` // Define an asynchronous function that handles POST requests. export async function POST(req: Request): Promise<Response> { const actions: AnnotatedFunction<any>[] = []; // Initialize an array to hold actions. // Check if a specific environment variable is set, indicating access to certain functionality. if (process.env.TAVILY_API_KEY) { actions.push(researchAction); // Add the research action to the actions array if the condition is true. } // Instantiate CopilotBackend with the actions defined above. const copilotKit = new CopilotBackend({ actions: actions, }); // Use the CopilotBackend instance to generate a response for the incoming request using an OpenAIAdapter. return copilotKit.response(req, new OpenAIAdapter()); } ``` 如何產生 UI 元件 ---------- 現在轉到您之前整合的應用程式內聊天機器人,並給它一個提示,例如「產生聯絡表單」。 生成完成後,您應該會看到生成的聯絡表單元件及其實作教程,如下所示。您也可以使用嵌入式程式碼編輯器修改產生的程式碼。 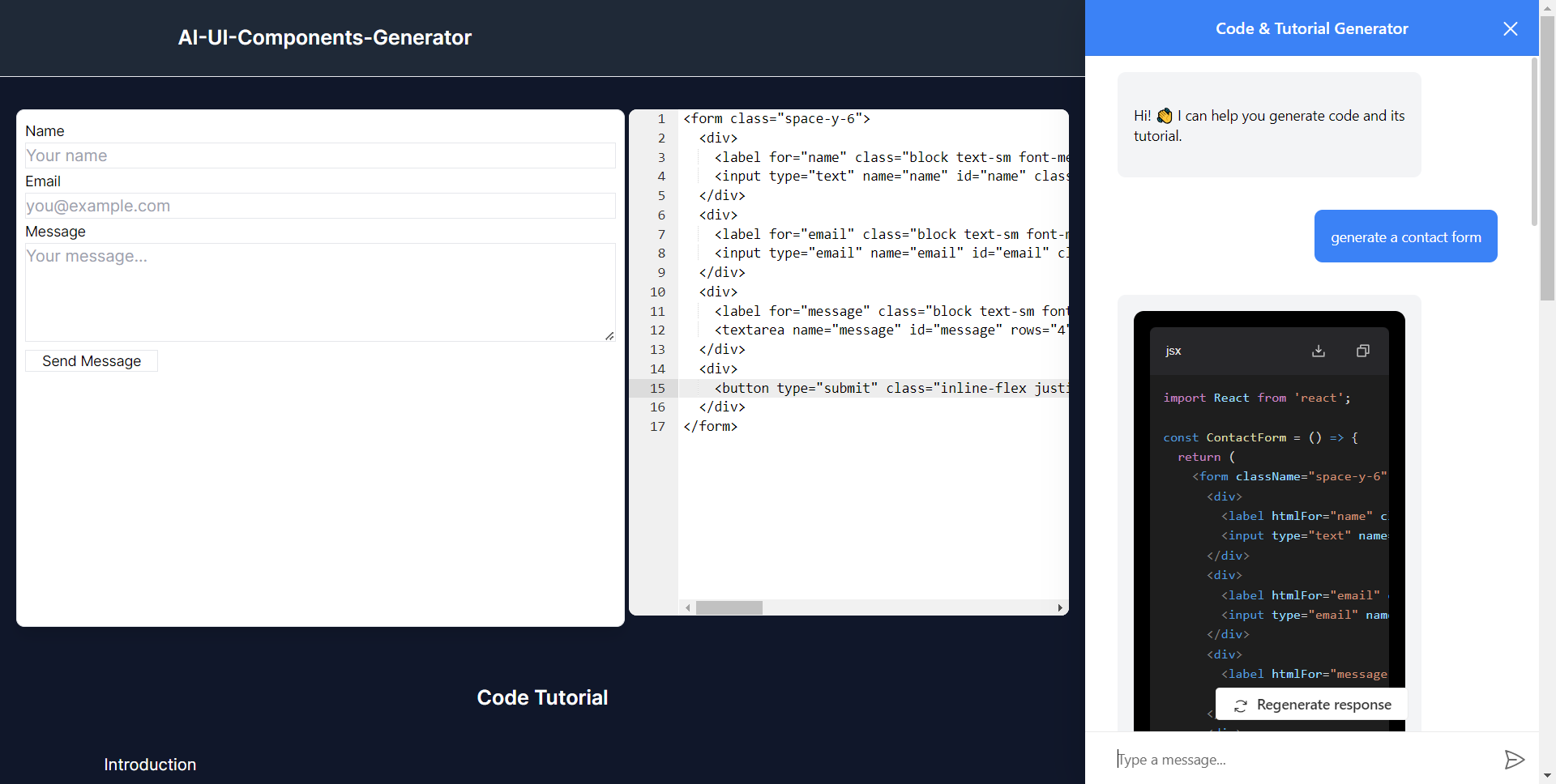 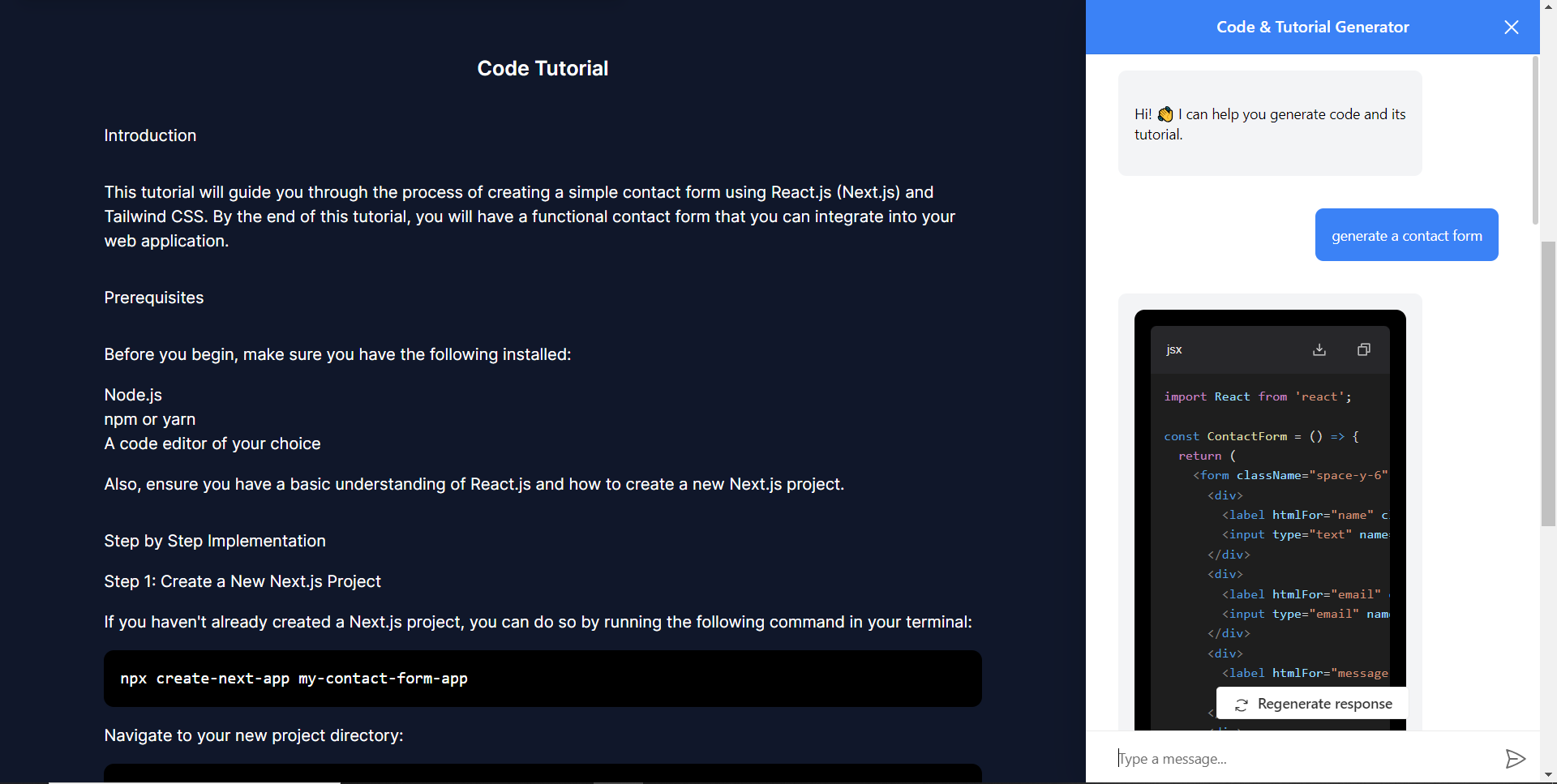 恭喜!您已完成本教學的專案。 結論 -- [CopilotKit](https://copilotkit.ai/)是一款令人難以置信的工具,可讓您在幾分鐘內將 AI Copilot 加入到您的產品中。無論您是對人工智慧聊天機器人和助理感興趣,還是對複雜任務的自動化感興趣,CopilotKit 都能讓您輕鬆實現。 如果您需要建立 AI 產品或將 AI 工具整合到您的軟體應用程式中,您應該考慮 CopilotKit。 您可以在 GitHub 上找到本教學的源程式碼: <https://github.com/TheGreatBonnie/AIPoweredUIComponentsGenerator> --- 原文出處:https://dev.to/the_greatbonnie/ai-powered-frontend-ui-components-generator-nextjs-gpt4-langchain-copilotkit-1hac
開發者生態系統已經發展了很多,並且有許多開發者不知道的框架。 我們「作為開發人員」有很多關於如何建立我們的應用程式的框架選項。這些選擇非常重要。 讓我們介紹 15 個框架,供您製作下一個專案。我將提供詳細的資源,以便您可以學習其中的每一個。 相信我!這份清單就是您所需要的。 讓我們開始吧。 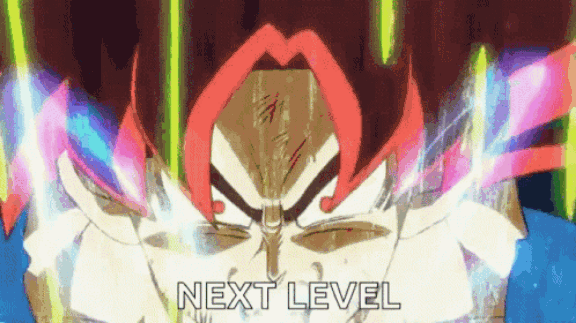 --- ### 庫與框架 在開始之前,讓我們先來了解一下框架與函式庫有何不同。開發人員可以互換使用它! 函式庫和框架都是由其他人編寫的可重複使用程式碼。 > 簡單來說: 將圖書館想像成IKEA之旅。您家裡有自己的空間,但您需要一些家具幫助。您不想從頭開始,所以您可以前往宜家,在那裡您可以挑選您需要的東西。你是做出決定的人。 現在,框架更像是建造一個樣品房。您已經有了一套計劃以及一些佈局和設計的選擇。但最終,藍圖和建造者處於控制之中。他們會讓你知道在哪裡可以加入你的意見,但他們正在掌控一切。 > 從技術角度來說。 透過庫,您可以指導應用程式的流程。您決定何時何地使用庫的功能。但有了框架,框架就控制了流程。它為您提供了一些插入程式碼的位置,但它是程式碼執行時發號施令的地方。 我使用了 Freecodecamp 的這篇文章“ [框架和庫之間的區別](https://www.freecodecamp.org/news/the-difference-between-a-framework-and-a-library-bd133054023f/)”,特別是因為它的解釋很簡單。完整閱讀一下! --- 1. [Wing](https://git.new/winlang-repo) - 一種雲端程式語言。 --------------------------------------------------- 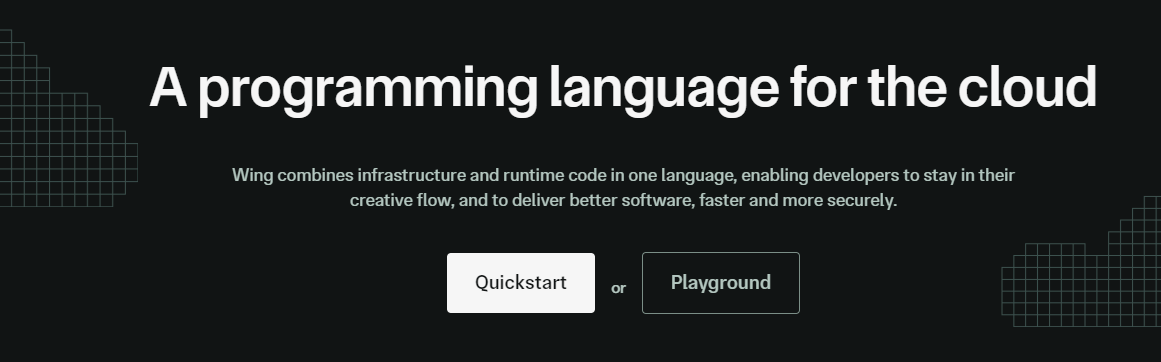 Wing是一個旨在開發雲端應用程式的框架。 它允許您在雲端中建立應用程式,並且具有相當簡單的語法。 核心概念是您可以直接在應用程式中指定資源。 您可以執行本機模擬並使用 Winglang 控制台視覺化每個步驟中發生的情況。 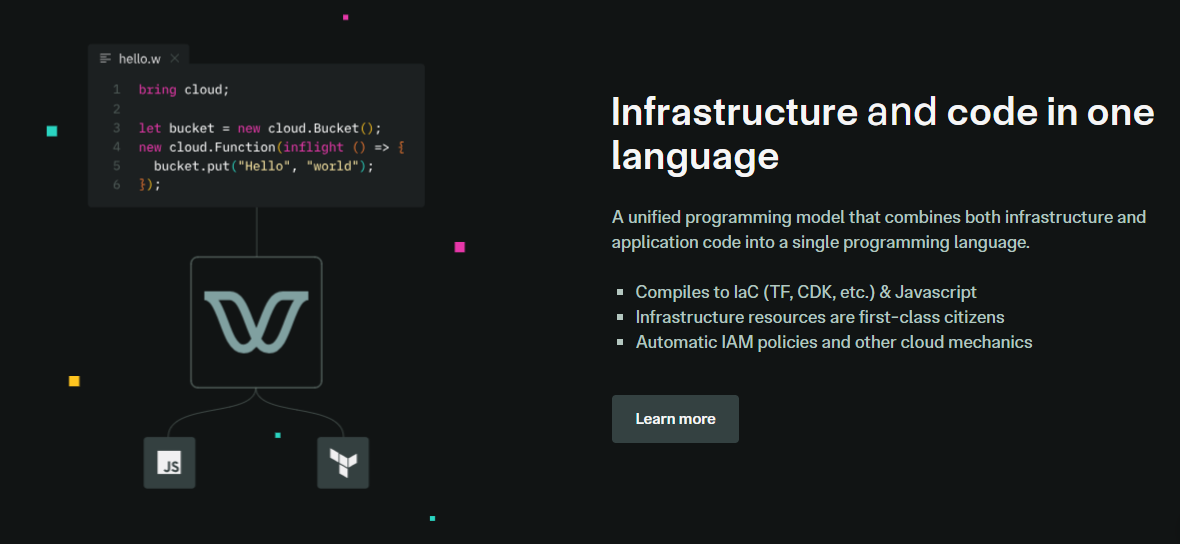 你**程式碼**。**本地測試**。**編譯**。**部署到雲端提供者**。 Wing 需要 Node `v20 or higher` 。 建立一個父目錄(我們使用的是`shared-counter` )並使用 Vite 使用新的 React 應用程式設定前端。您可以使用這個 npm 指令。 ``` npm create -y vite frontend --template react-ts // once installed, you can check if it's running properly. cd frontend npm install npm run dev ``` 您可以使用此 npm 命令安裝 Wing。 ``` npm install -g winglang ``` 您可以使用`wing -V`驗證安裝。 Wing 還提供官方[VSCode 擴充功能](https://marketplace.visualstudio.com/items?itemName=Monada.vscode-wing)和[IntelliJ](https://plugins.jetbrains.com/plugin/22353-wing) ,後者提供語法突出顯示、補全、轉到定義和嵌入式 Wing 控制台支援。您可以在建立應用程式之前安裝它! 您可以使用 Wing 作為雲端後端來建立任何全端應用程式。 建立後端目錄。 ``` mkdir ~/shared-counter/backend cd ~/shared-counter/backend ``` 建立一個新的空 Wing 專案。 ``` wing new empty // This will generate three files: package.json, package-lock.json and main.w file with a simple "hello world" program wing it // to run it in the Wing simulator // The Wing Simulator will be opened in your browser and will show a map of your app with a single function. //You can invoke the function from the interaction panel and check out the result. ``` 使用指令`wing new empty`後的結構如下。 ``` bring cloud; // define a queue, a bucket, and a counter let bucket = new cloud.Bucket(); let counter = new cloud.Counter(initial: 1); let queue = new cloud.Queue(); // When a message is received in the queue -> it should be consumed // by the following closure queue.setConsumer(inflight (message: str) => { // Increment the distributed counter, the index variable will // store the value before the increment let index = counter.inc(); // Once two messages are pushed to the queue, e.g. "Wing" and "Queue". // Two files will be created: // - wing-1.txt with "Hello Wing" // - wing-2.txt with "Hello Queue" bucket.put("wing-{index}.txt", "Hello, {message}"); log("file wing-{index}.txt created"); }); ``` 您可以安裝`@winglibs/vite`來啟動開發伺服器,而不是使用`npm run dev`來啟動本機 Web 伺服器。 ``` // in the backend directory npm i @winglibs/vite ``` 您可以使用`backend/main.w`中提供的 publicEnv 將資料傳送到前端。 讓我們來看一個小例子。 ``` // backend/main.w bring vite; new vite.Vite( root: "../frontend", publicEnv: { TITLE: "Wing + Vite + React" } ); // import it in frontend // frontend/src/App.tsx import "../.winglibs/wing-env.d.ts" //You can access that value like this. <h1>{window.wing.env.TITLE}</h1> ``` 你還可以做更多: - 讀取/更新 API 路線並使用 Wing Simulator 檢查它。 - 使用後端獲取值。 - 使用`@winglibs/websockets`來同步瀏覽器,在後端部署一個 WebSocket 伺服器,你可以連接這個 WebSocket 來接收即時通知。 可以節省大量時間的一些功能包括熱重載,以獲得即時回饋並順利產生必要的安全策略。 無需學習每個雲端提供者的語法。 您的程式碼可以編譯到 AWS、GCP、Azure 或任何自訂平台。太棒了:D 您可以閱讀完整的逐步指南,以了解[如何使用 React 作為前端和 Wing 作為後端建立簡單的 Web 應用程式](https://www.winglang.io/docs/guides/react-vite-websockets)。測試是使用 Wing Simulator 完成的,並使用 Terraform 部署到 AWS。 部署後的AWS架構是這樣的。 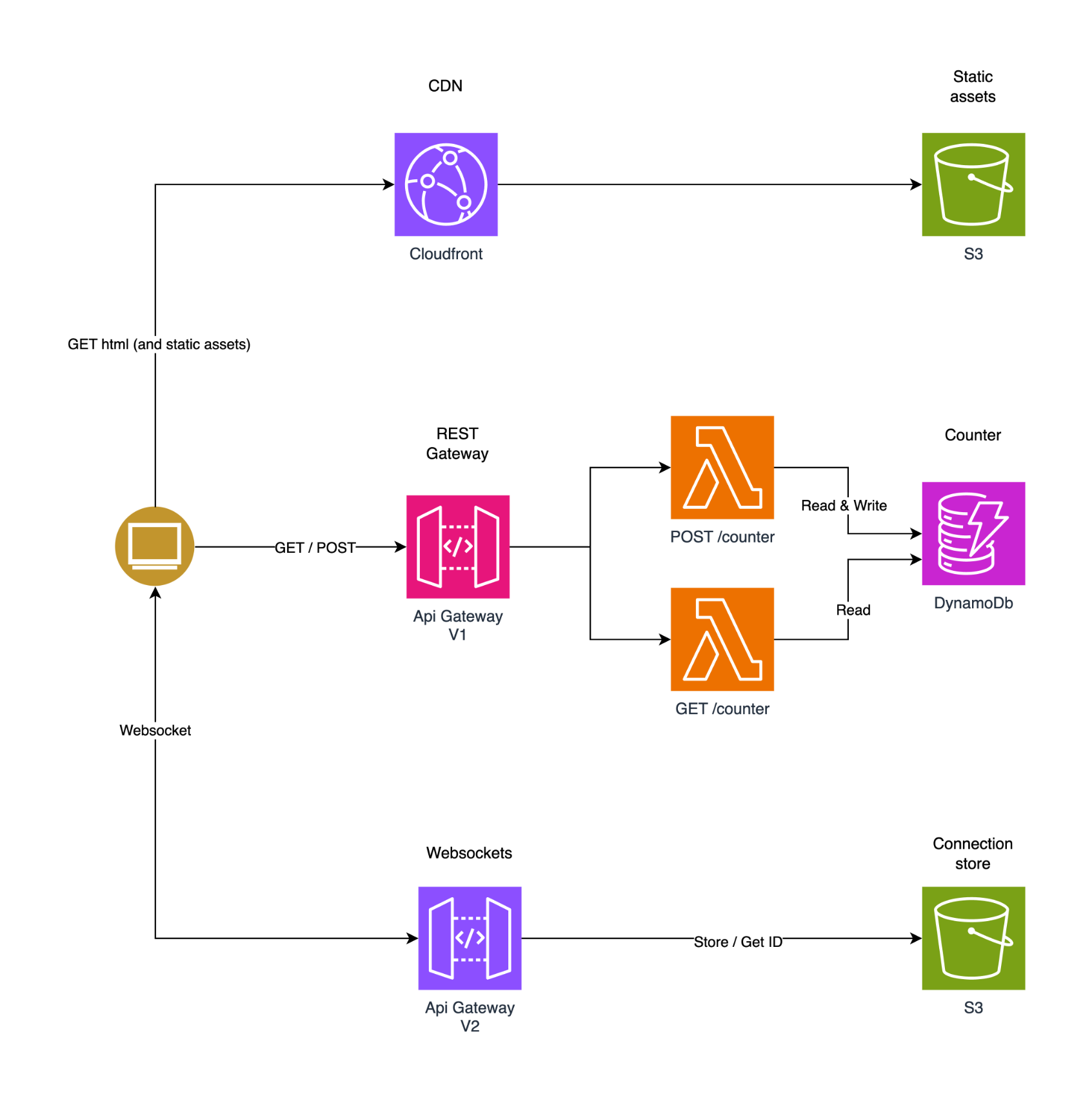 為了提供開發者選擇和更好的體驗,Wing 推出了對[TypeScript (Wing)](https://www.winglang.io/docs/typescript/)等其他語言的全面支援。唯一強制的事情是您必須安裝 Wing SDK。 這也將使控制台完全可用於本地偵錯和測試,而無需學習 Wing 語言。 該翼目前支援以下輸出: - AWS CDK 平台 - Terraform/AWS 平台 - Terraform/GCP 平台 - Terraform/Azure 平台 - 模擬器平台 - 客製化平台 Wing 甚至還有其他[指南](https://www.winglang.io/docs/category/guides),因此更容易遵循。 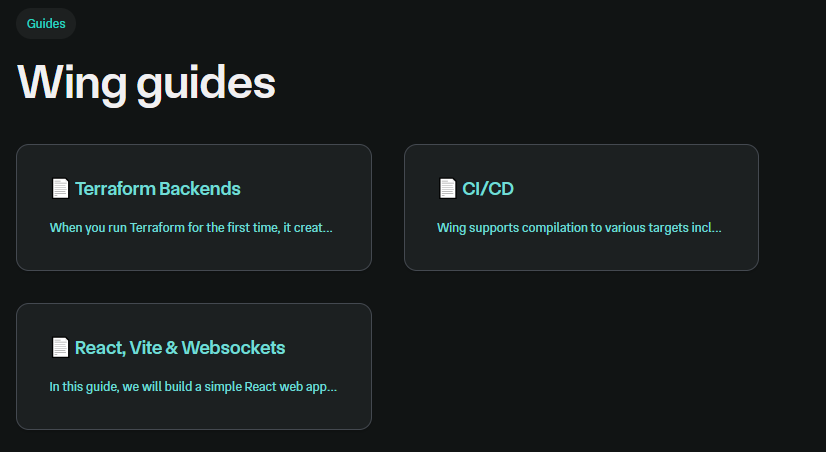 您可以閱讀[文件](https://www.winglang.io/docs)並查看[範例](https://www.winglang.io/docs/category/examples)。 您也可以在[Playground](https://www.winglang.io/play/)中使用 Wing 查看結構和範例。 如果你比較像輔導員。看這個! {% 嵌入 https://www.youtube.com/watch?v=wzqCXrsKWbo %} 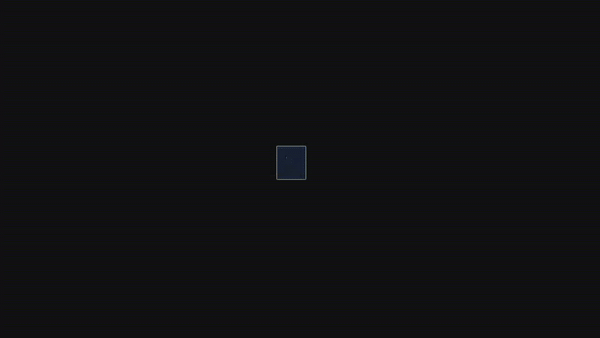 Wing 在 GitHub 上有 4500+ 顆星,1600+ 個版本,但仍未進入 v1 版本,這意味著意義重大。 {% cta https://git.new/winlang-repo %} 星翼 ⭐️ {% endcta %} --- 2. [Nest](https://github.com/nestjs/nest) - 高效且可擴展的伺服器端應用程式。 ------------------------------------------------------------ 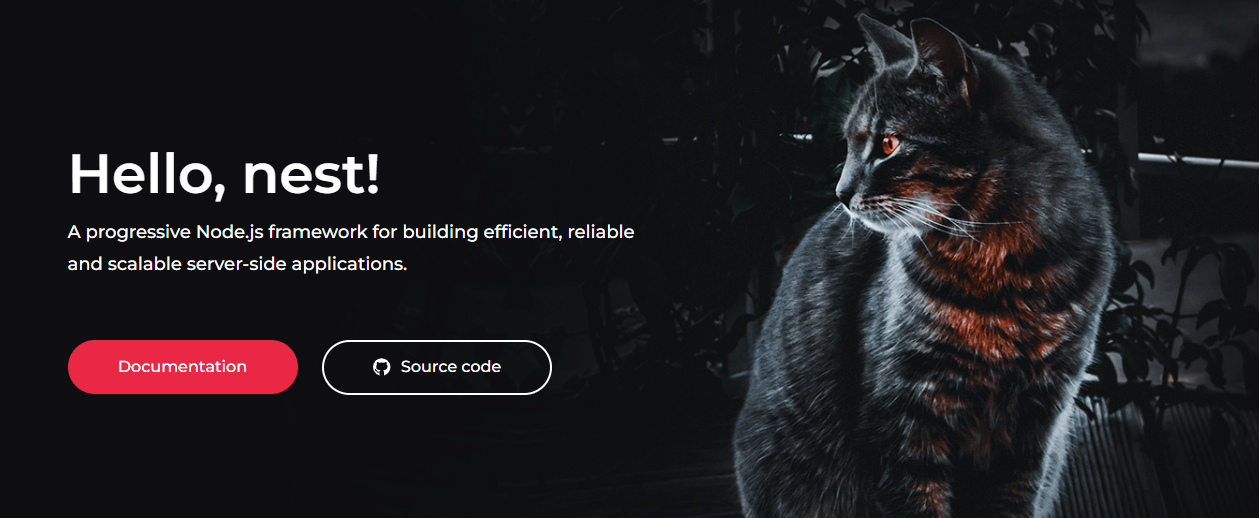 一個先進的 Node.js 框架,用於使用 TypeScript/JavaScript 建立高效且可擴展的伺服器端應用程式。 它使用現代 JavaScript,使用 TypeScript 建構(保留與純 JavaScript 的兼容性),並結合了 OOP(物件導向程式設計)、FP(函數式程式設計)和 FRP(函數式反應式程式設計)的元素。 在底層,Nest 使用 Express,但也提供與 Fastify 等各種其他庫的兼容性,從而可以輕鬆使用無數可用的第三方外掛程式。 Nest 提供了高於這些常見 Node.js 框架(Express/Fastify)的抽象級別,但也直接向開發人員公開其 API。這為開發人員提供了一定程度的自由。 在我們了解更多之前,請觀看 100 秒內的 Nestjs! {% 嵌入 https://www.youtube.com/watch?v=0M8AYU\_hPas&pp=ygUXZW1iZXIganMgaW4gMTAwIHNlY29uZHM%3D %} 考慮到它們提供的靈活性,您當然不必重新發明輪子。 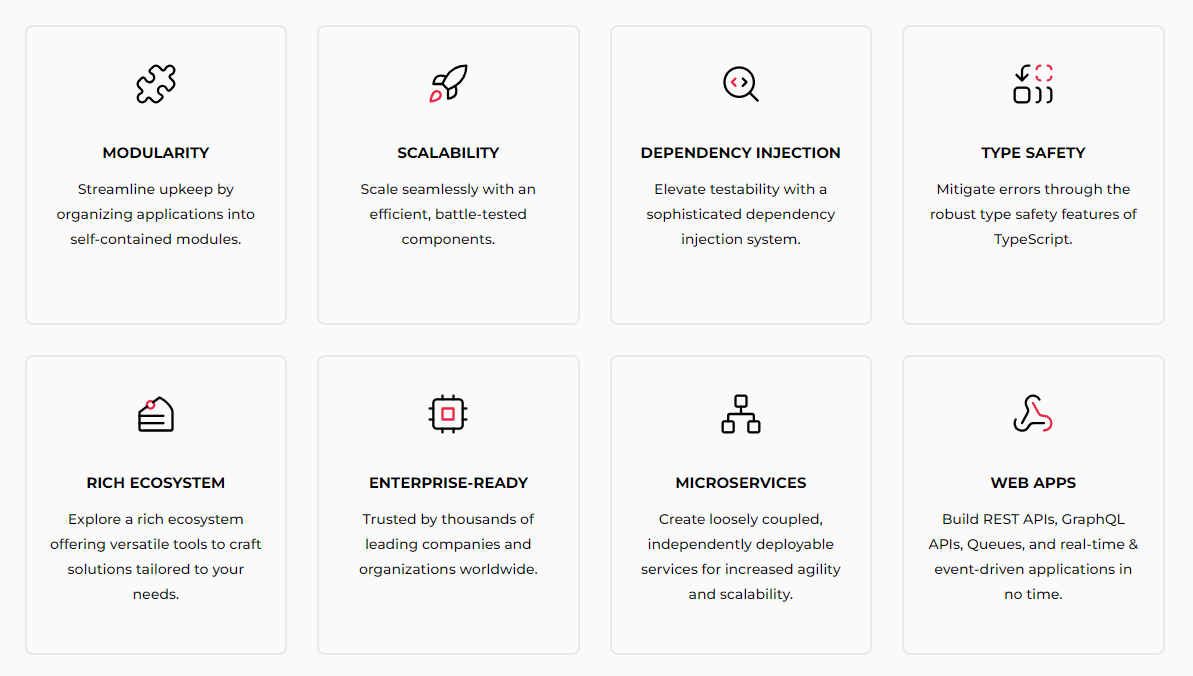 這是使用 Nest CLI 設定新專案的方法。 ``` npm i -g @nestjs/cli nest new project-name ``` 這將引導該應用程式。 ``` import { NestFactory } from '@nestjs/core'; import { AppModule } from './app.module'; async function bootstrap() { const app = await NestFactory.create(AppModule); await app.listen(3000); } bootstrap(); ``` 您可以閱讀[文件](docs.nestjs.com)。 他們還提供一套付費課程(我想知道為什麼)。如果您需要完整的路線圖並想成為 Nest 的使用專家,請隨時查看它們。 但我建議使用 Freecodecamp 提供的這些免費教學來學習。 - [NestJs 初學者課程 - 建立 REST API](https://www.youtube.com/watch?v=GHTA143_b-s) - 大約 3.42 小時,涵蓋許多[主題](https://www.freecodecamp.org/news/learn-nestjs-by-building-a-crud-api/)。 - [綜合 NestJS 課程](https://www.youtube.com/watch?v=sFnAHC9lLaw&t=1s)- 涵蓋 20 個模組,時長 14 小時。 {% 嵌入 https://www.youtube.com/watch?v=sFnAHC9lLaw&t=1s %} 如果您正在尋找入門專案,請學習[如何在 NestJS 中使用 Nodemailer 發送電子郵件](https://www.freecodecamp.org/news/how-to-use-nodemailer-in-nestjs/)。您可以使用它來獲得紮實的基礎知識。 Nest.js 擁有龐大的開發人員社區,並被許多公司使用。尋找已使用 Nest[的專案和公司的完整清單](https://docs.nestjs.com/discover/companies)。 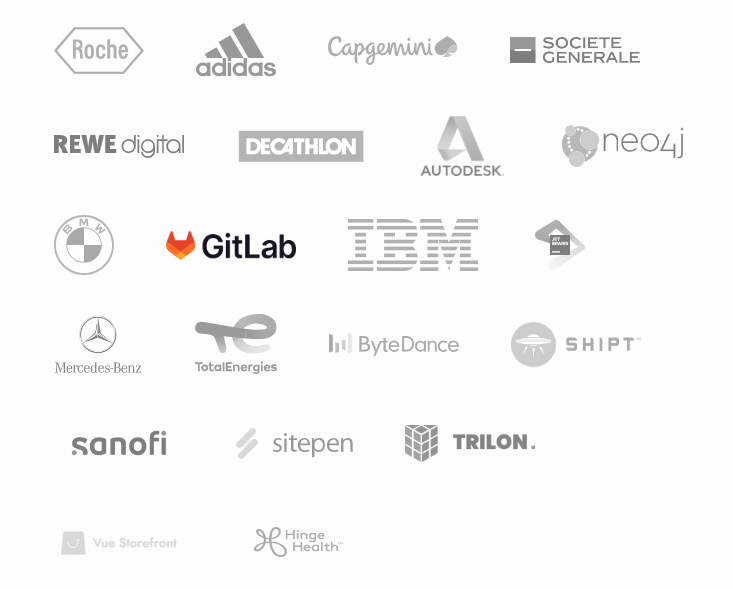 順便說一句,作為初學者,我最常擔心的是相似的名稱:Nextjs、Nuxtjs 和 Nestjs。我涵蓋了所有內容,這樣您就不必感到困惑。哈哈! Nest 在 GitHub 上擁有超過 64k 個 star,提交次數超過 15k,並且已發布`v10`版本。 {% cta https://github.com/nestjs/nest %} 星巢 ⭐️ {% endcta %} --- 3. [Gatsby](https://github.com/gatsbyjs/gatsby) - 最好的基於 React 的框架,具有內建的效能、可擴展性和安全性。 ----------------------------------------------------------------------------------- 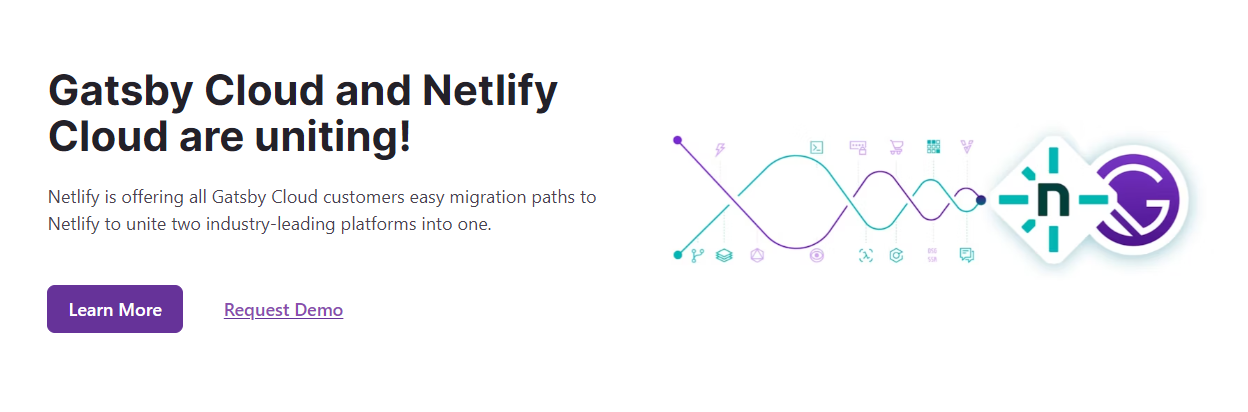 Gatsby 是一個基於 React 的免費開源框架,可協助開發人員建立速度極快的網站和應用程式。 它將動態呈現網站的控制和可擴展性與靜態網站生成的速度結合起來,創造了一個全新的可能性網絡。 Gatsby 從任何資料來源提取資料,無論是 Markdown 檔案、Contentful 或 WordPress 等無頭 CMS,還是 REST 或 GraphQL API。使用來源插件載入資料,然後使用 Gatsby 的統一 GraphQL 介面進行開發。 與 Next.js 不同,Gatsby 不執行伺服器端渲染。相反,它會在建置期間在客戶端產生 HTML 內容。 我見過一些使用 Gatsby 建立的優秀作品集。 開始使用以下 npm 指令。 ``` npm init gatsby ``` 它會要求提供網站標題和專案目錄的名稱。繼續按照提示選擇您的首選語言(JavaScript 或 TypeScript)、CMS、樣式工具和其他功能。 您可以這樣使用它。 ``` cd my-gatsby-site // to start the local dev server npm run develop ``` 您可以閱讀[文件](https://www.gatsbyjs.com/docs)。我個人很喜歡文件的流程。 您也可以按照[教學](https://www.gatsbyjs.com/docs/tutorial/getting-started/)開始,[操作指南](https://www.gatsbyjs.com/docs/how-to/)和[概念指南](https://www.gatsbyjs.com/docs/conceptual/)深入了解 Gatsby 概念以及網站架構。 Gatsby 提供了開箱即用的 PWA 和大量主題。使用 Gatsby 主題,您的所有預設配置(共享功能、資料來源、設計)都會從您的網站中抽象化出來,並放入可安裝的套件中。您可以閱讀有關[主題的](https://www.gatsbyjs.com/docs/themes/)更多資訊。 例如, `gatsby-theme-blog`是用於建立部落格的官方 Gatsby 主題。可能有可以透過`gatsby-config.js`配置的主題選項。 ``` npm install gatsby-theme-blog ``` 對於電子商務商店或廣泛的媒體網站等內容密集的企業級網站來說,Gatsby 並不是理想的解決方案。隨著內容大小的增加,建置時間將急劇增加。 尋找使用 Gatsby 建立的[606 個網站](https://www.gatsbyjs.com/showcase/)的清單。其中,53 個網站是開源的,因此這可以提供靈感,也是一個起點。 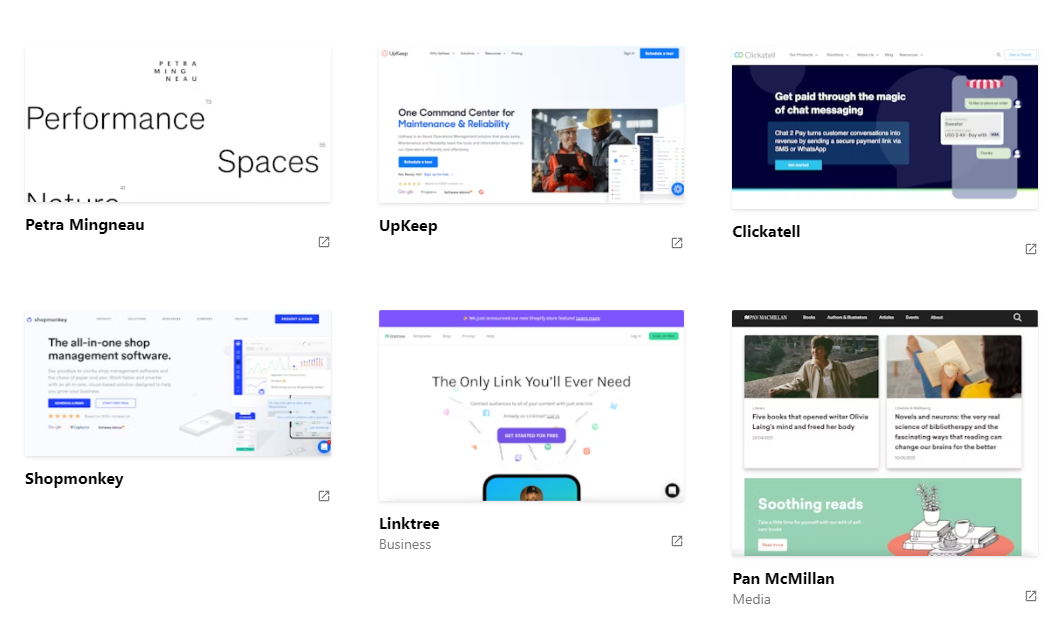 他們還提供了[大量按類別劃分的插件](https://www.gatsbyjs.com/plugins)以及每個插件中清晰的文件。其中一個範例是將 Google Analytics 新增至您的應用程式的插件。 ``` npm install gatsby-plugin-google-analytics ``` 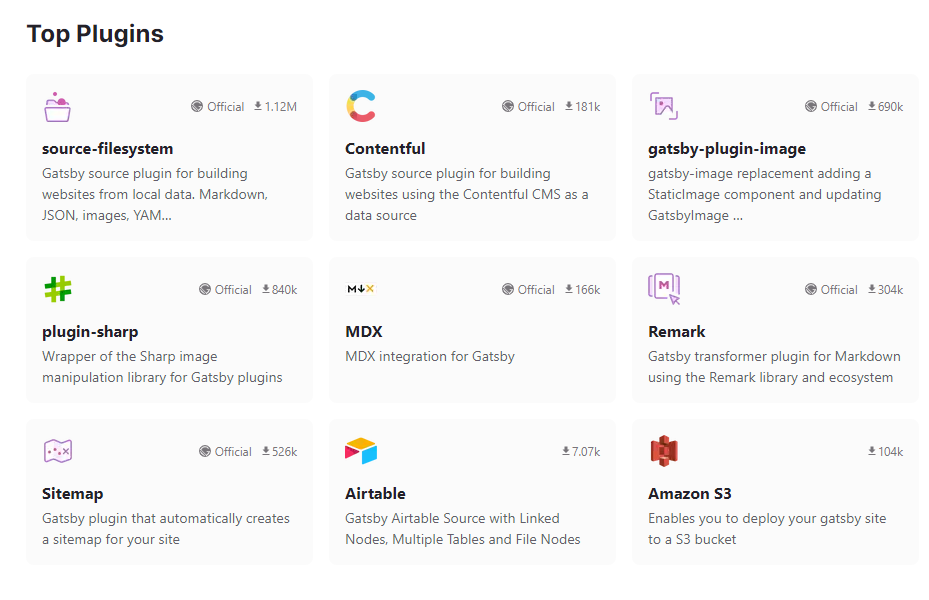 您也可以使用 Gatsby 的[Starter 庫](https://www.gatsbyjs.com/starters/)。使用 Gatsby 建立下一個應用程式還需要什麼? 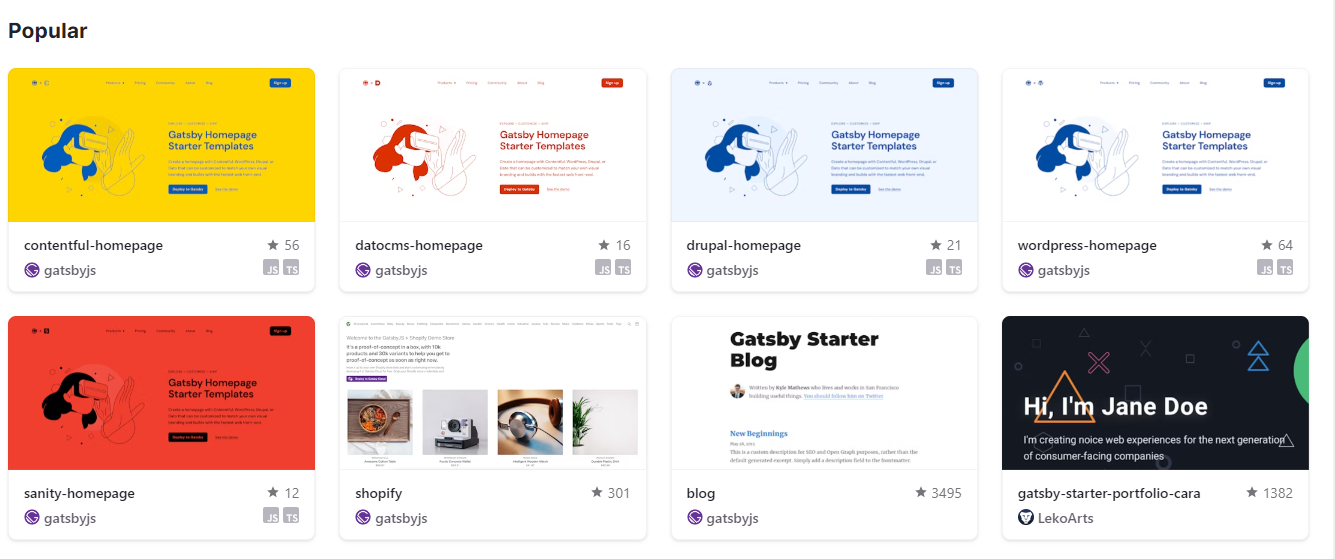 使用這些[參考指南](https://www.gatsbyjs.com/docs/reference/)來獲取有關 Gatsby API 的詳細資訊。 如果您喜歡完整的課程,我建議您觀看[Gatsby 靜態網站產生器教學](https://www.youtube.com/watch?v=RaTpreA0v7Q)- Freecodecamp 提供的 9 小時教學。 Gatsby 在 GitHub 上有 55,000 顆星,目前處於 v5 版本,並有超過 245,000 名開發人員使用。 {% cta https://github.com/gatsbyjs/gatsby %} 明星蓋茲比 ⭐️ {% endcta %} --- 4. [Nextjs](https://github.com/vercel/next.js) - Web 的 React 框架。 ---------------------------------------------------------------- 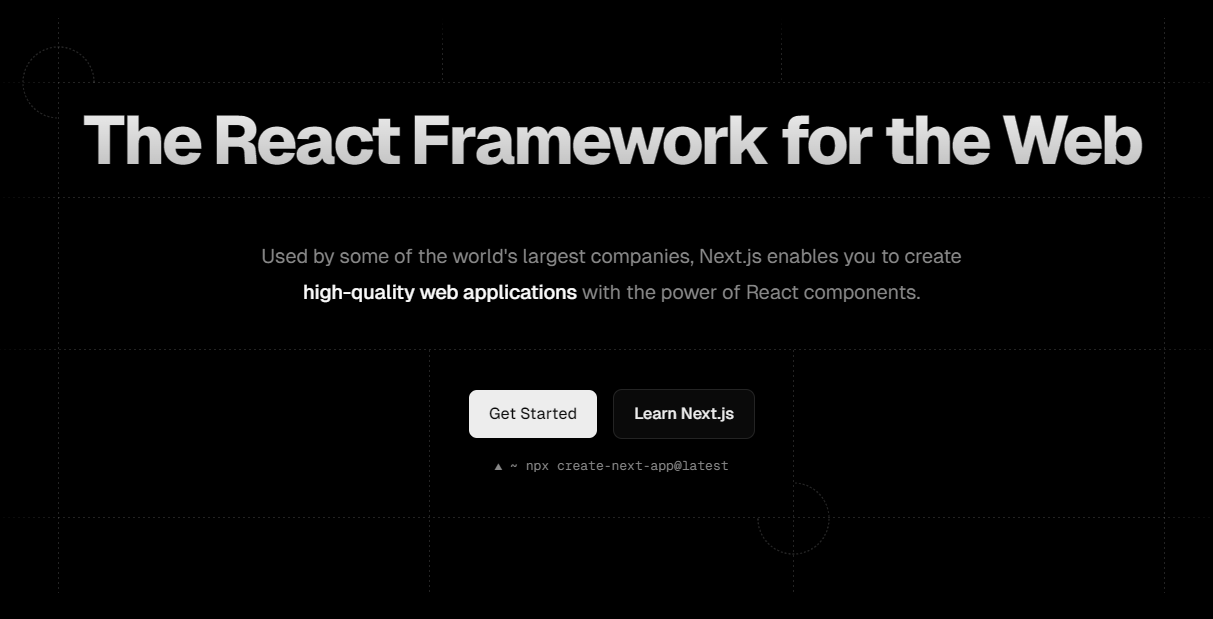 由於它提供的優化級別,它是我最喜歡的框架之一。 Next.js 使您能夠透過擴展最新的 React 功能並整合強大的基於 Rust 的 JavaScript 工具來建立全端 Web 應用程式,以實現最快的建置。 Next.js 由荷蘭公司 Vercel(以前稱為 ZEIT)於 2017 年建立。 Next.js 也像 Gatsby 一樣提供靜態產生器。 Next.js 的建置遵循`Build once, runs everywhere`的原則,因此您可以使用 Next.js 製作 Web 應用程式、行動應用程式、桌面應用程式和漸進式 Web 應用程式。 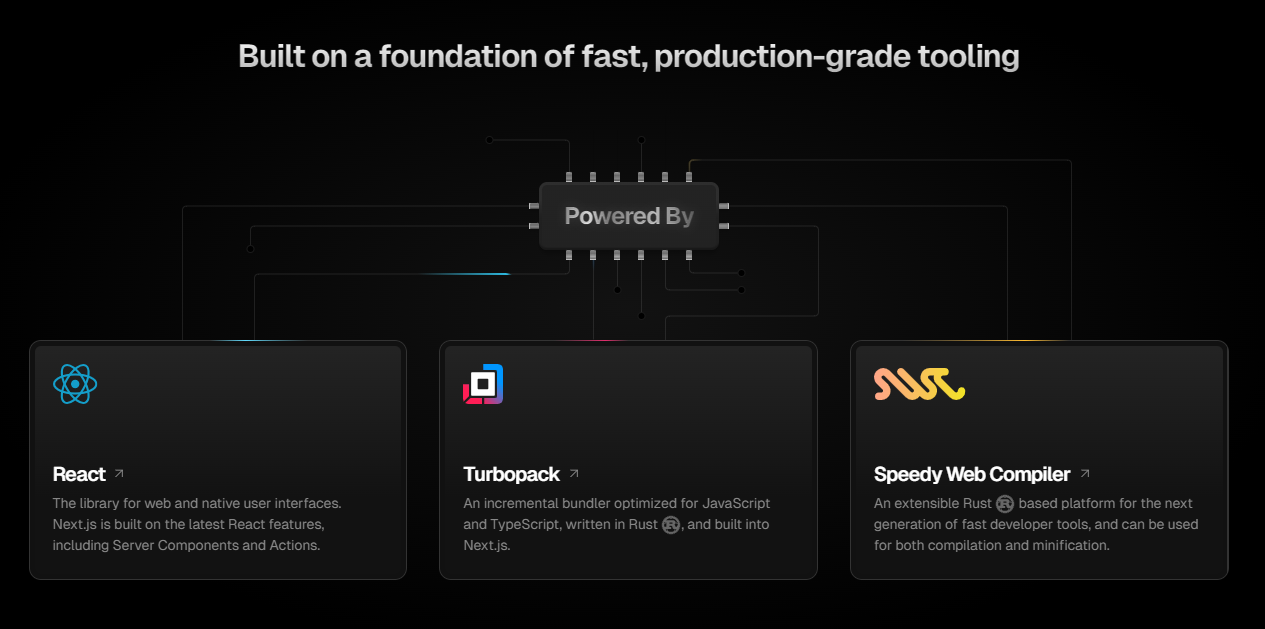 Nextjs 提供了許多功能,例如檔案路由、渲染技術(例如 ISR)以及深層的圖像和字體最佳化。你可以檢查任何 nextjs 網站的 SEO 統計資料,在大多數情況下它都是一流的。 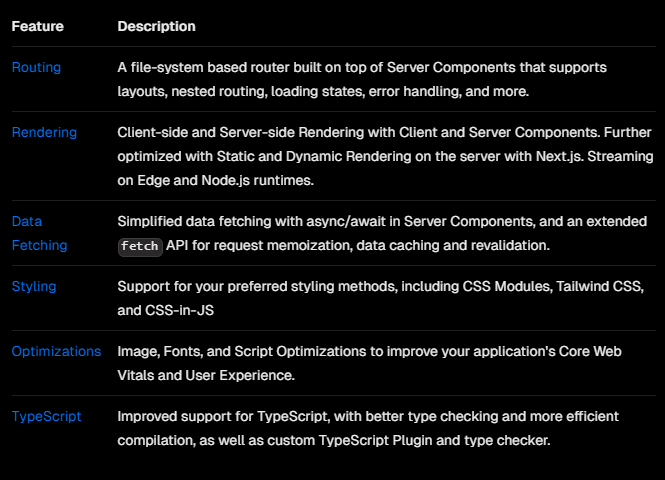 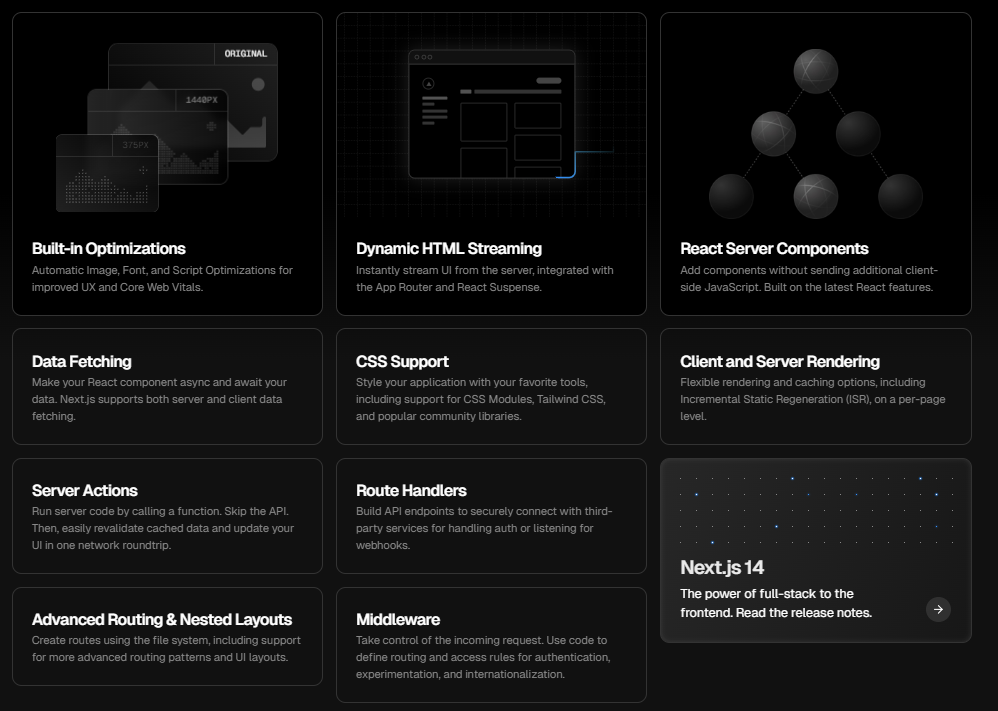 開始使用以下 npm 指令。 ``` npx create-next-app@latest ``` 您可以閱讀[文件](https://nextjs.org/docs)並按照[本指南](https://nextjs.org/docs/getting-started/installation)開始使用。 其中涉及很多概念,閱讀完整的文件需要幾個月的時間。我前段時間寫過一篇文章,你可以看看。它並沒有出名,但它是我憑藉多年的 Nextjs 經驗編寫的最好的文章之一。我還提到了 Nextjs 團隊提供的[官方課程](https://nextjs.org/learn/dashboard-app/getting-started)。 {% 嵌入 https://dev.to/anmolbaranwal/12-things-you-didnt-know-you-could-do-with-nextjs-386b %} 如果您想透過 YouTube 教學進行學習,我建議您觀看這些最近的教程,因為文件更新得非常頻繁,因此最好觀看最近的內容,而不是幾年前的內容。 - \[帶有 TypeScript 的 Nextjs 13(應用程式路由器)\](https://www.youtube.com/watch?v=ZVnjOPwW4ZA&pp=ygUTbmV4dGpzIGNyYXNoIGNvdXJzZQ%3D%3D ) - 1 小時教程。 - [Next.js 14 完整課程 2024](https://www.youtube.com/watch?v=wm5gMKuwSYk) - 3 小時教學。 您也可以觀看 Nextjs 100 秒。他們加入了一個基本教程,使其長達 11 分鐘。 {% 嵌入 https://www.youtube.com/watch?v=Sklc\_fQBmcs&t=4s&pp=ygUObmV4dGpzIGluIDEwMHM%3D %} 我自己使用文件學習了它,並使用它建置了超過 6 個專案,甚至是一個超過 20k 程式碼庫的 SAAS 應用程式。這就是為什麼我說,它是您可以選擇的最佳框架之一。 使用 Next.js 建立的一些熱門網站包括 Auth0、Coinbase、Docker、GitHub、Hulu、Netflix、Sesame、Starbucks、Trulia、Twitch 和 Uber。你可以看到所有使用Nextjs的[網站](https://nextjs.org/showcase)。 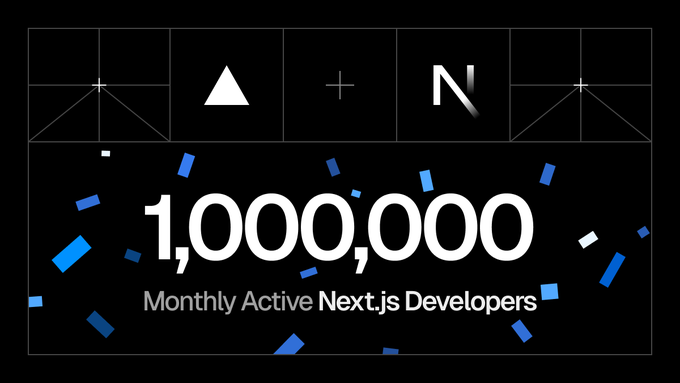 他們還提供了各種可以直接使用的[入門模板](https://vercel.com/templates/next.js)。 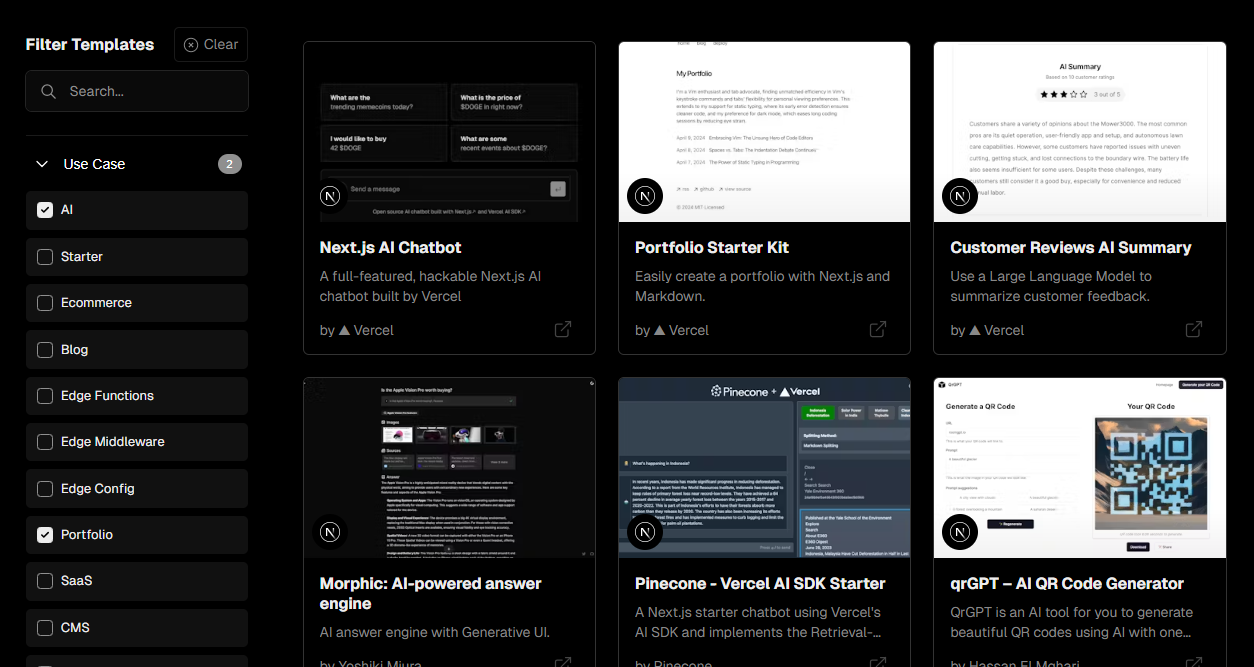 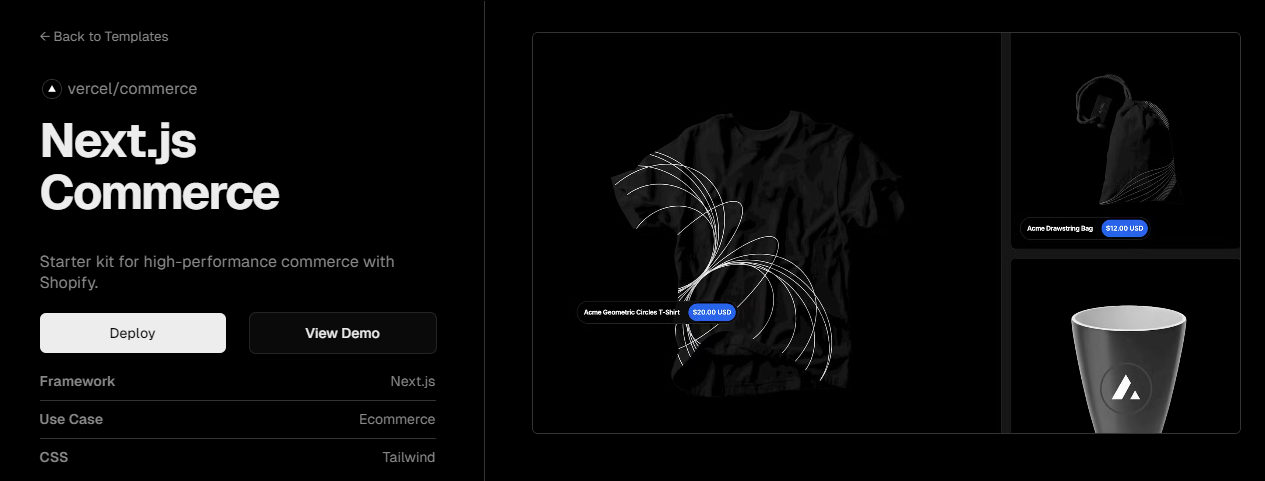 Next 在 GitHub 上有 12 萬顆星,發布`v14.2`版本,在 NPM 上每週下載量超過 600 萬次。如其儲存庫所示,有 260 萬開發人員使用。 {% cta https://github.com/vercel/next.js %} 明星 Nextjs ⭐️ {% endcta %} --- 5. [Preact](https://github.com/preactjs/preact) - 具有相同現代 API 的快速 3kB React 替代品。 ------------------------------------------------------------------------------- 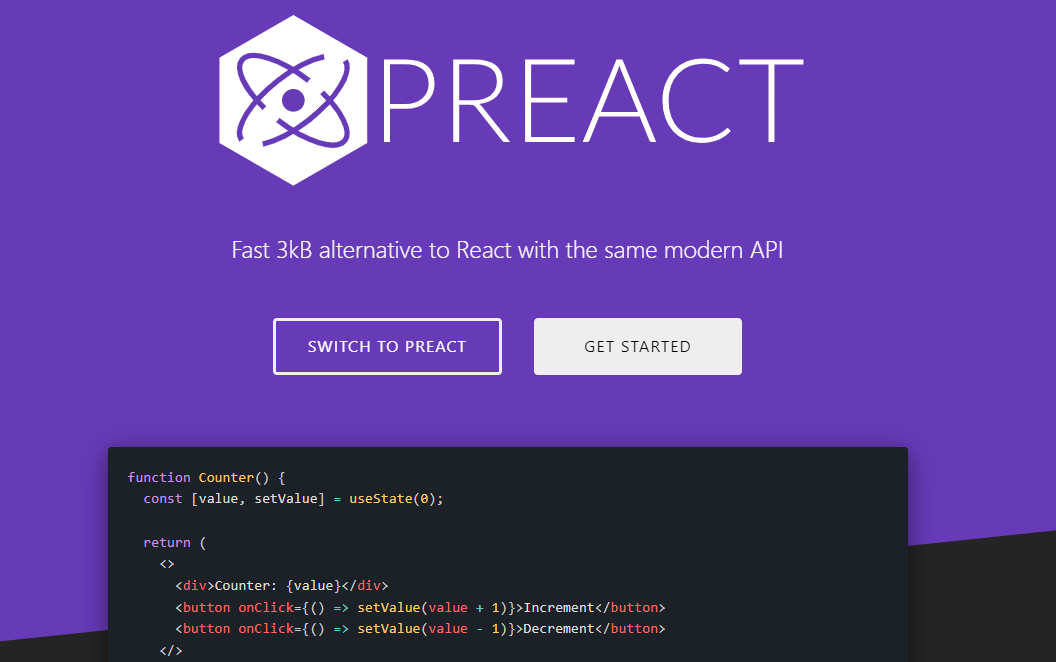 Preact 是一個輕量級、快速、高效能的函式庫,是 React 的替代品。 Preact 的大小僅為 3kb(經過壓縮和壓縮),但卻為您提供了 React 所需的所有功能,使其成為最好的 JavaScript 框架之一。 Preact 的建立者 Jason Miller 是 Google 的高級開發者計畫工程師。 Preact 基本上具有 Virtual DOM 元件的所有功能,而沒有諸如以下的開銷: - 熟悉 React API 和 ES6 類別、鉤子和功能元件模式。 - 透過簡單的 preact/compat 別名實現廣泛的 React 相容性。 - 您需要的一切,例如 JSX、VDOM、DevTools、HMR、SSR。 在生產過程中,您可以輕鬆地從現有專案中的 React 切換到 Preact,因為它們支援相同的 API。 程式碼範例結構如下所示。您也可以查看此範例[程式碼筆](https://codepen.io/developit/pen/LpNOdm),您可以查看它以了解 Preact 中程式碼庫的結構。 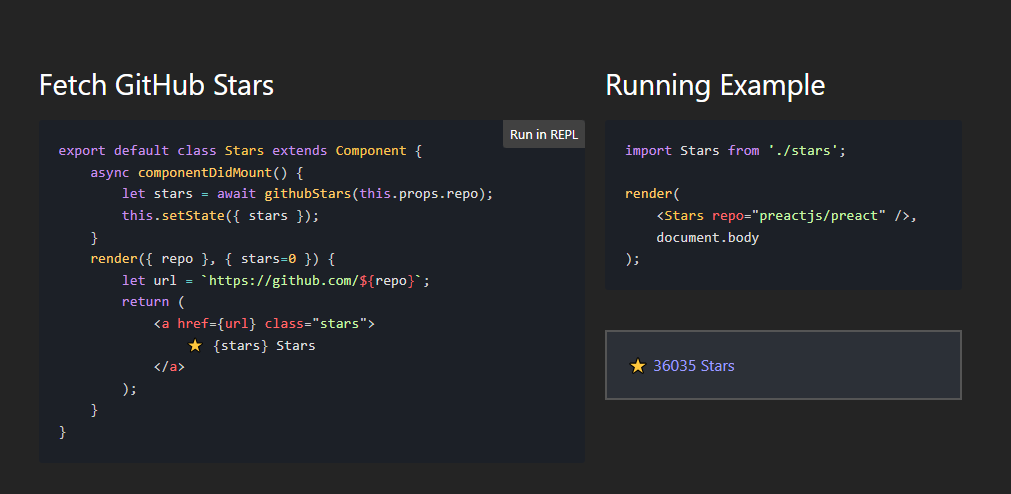 開始使用以下 npm 指令。 ``` npm init preact ``` 這是執行開發伺服器的方式。 ``` # Go into the generated project folder cd my-preact-app # Start a development server npm run dev ``` 您將必須配置一些東西,尤其是別名。請遵循[本指南](https://preactjs.com/guide/v10/getting-started)。 您可以閱讀[文件](https://preactjs.com/guide/v10/getting-started/)並查看詳細的[演示和範例](https://preactjs.com/about/demos-examples)清單。 他們還提供了基於 Web 的[教程](https://preactjs.com/tutorial/),您可以按照該教程來學習 Preact。 如果您需要範例應用程式、樣板檔案、元件、工具包等,請使用[Awesome Preact](https://github.com/preactjs/awesome-preact) 。 Preact 在 GitHub 上有 36,000 顆星,目前已發布`v10`版本。 {% cta https://github.com/preactjs/preact %} Star Preact ⭐️ {% endcta %} --- 6. [tRPC](https://github.com/trpc/trpc) - 端到端類型安全性 API 變得簡單。 ------------------------------------------------------------ 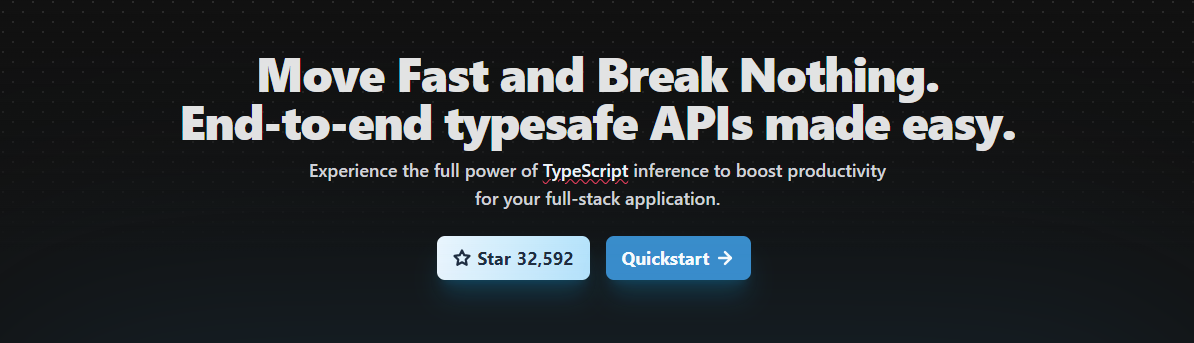 tRPC 可讓您輕鬆建立和使用完全類型安全的 API,而無需模式或程式碼產生。 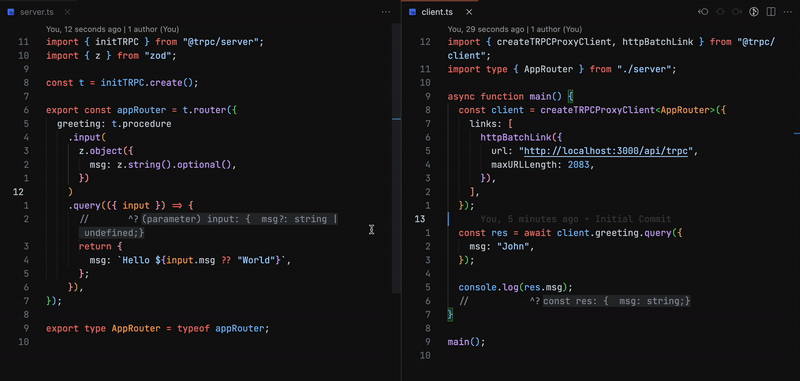 上面的客戶端沒有從伺服器導入任何程式碼,僅導入其類型聲明 如果我們要深入了解,那麼您絕對應該閱讀一些歷史。 {% 嵌入 https://dev.to/zenstack/a-brief-history-of-api-rpc-rest-graphql-trpc-fme %} 目前,GraphQL 是在 TypeScript 中實作型別安全 API 的主要方式(這太棒了!)。由於 GraphQL 被設計為用於實現 API 的與語言無關的規範,因此它沒有充分利用 TypeScript 這樣的語言的強大功能。 如果您的專案是使用全端 TypeScript 建置的,您可以直接在客戶端和伺服器之間共用類型,而無需依賴程式碼生成。 tRPC 適用於全端 TypeScript 開發人員。它使您可以輕鬆編寫可以在應用程式的前端和後端安全使用的端點。 API 合約的類型錯誤將在建置時被捕獲,從而減少應用程式在執行時出現錯誤的可能性。 這是為 Mono 儲存庫設計的,因為您需要從伺服器匯出/匯入類型定義。 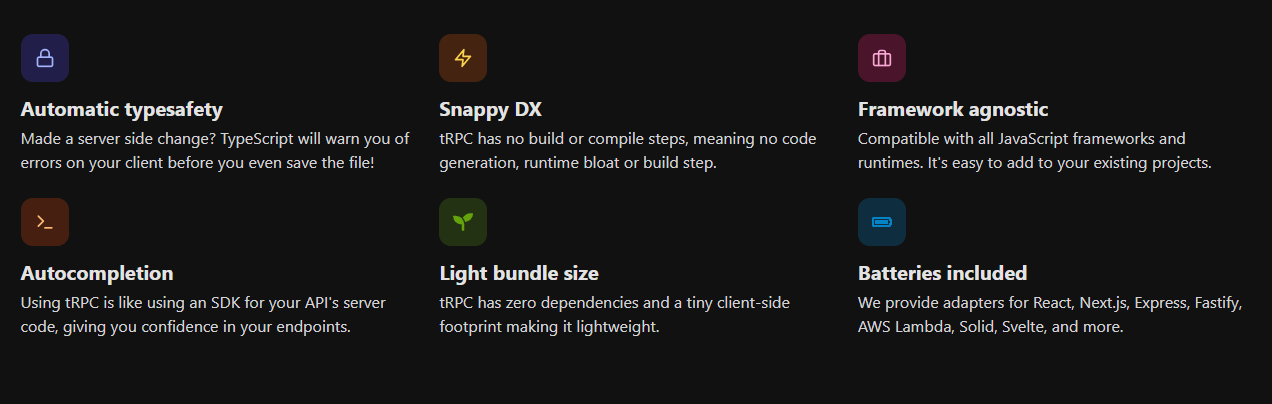 開始使用以下 npm 指令。 ``` npm install @trpc/server@next @trpc/client@next ``` 您必須使用實例定義後端路由器。閱讀[快速入門指南](https://trpc.io/docs/quickstart)以了解更多詳細資訊。 了解[trpc 中涉及的概念](https://trpc.io/docs/concepts)(例如 rpc 和使用的術語)非常重要。 您可以閱讀[文件](https://trpc.io/docs)。 如果您已經在一個混合語言的團隊中工作,或者擁有您無法控制的第三方消費者,那麼您應該建立一個與語言無關的 GraphQL-API。 如果您想測試一下,我建議使用此[模板](https://github.com/new?template_name=examples-minimal&template_owner=trpc),其中包含一個最小的範例。 您還可以觀看這個[45 分鐘的 YouTube 教學](https://www.youtube.com/watch?v=UfUbBWIFdJs&pp=ygUMd2hhdCBpcyB0cnBj)來了解有關 trpc 的更多資訊。 {% 嵌入 https://www.youtube.com/watch?v=UfUbBWIFdJs&pp=ygUMd2hhdCBpcyB0cnBj %} 它們在 GitHub 上擁有超過 32,000 顆星,目前處於`v11` beta 版本,並被 51,000 名開發人員使用。 {% cta https://github.com/trpc/trpc %} 啟動 tRPC ⭐️ {% endcta %} --- [7.Nuxtjs](https://github.com/nuxt/nuxt) - 直覺的 Vue 框架。 ------------------------------------------------------ 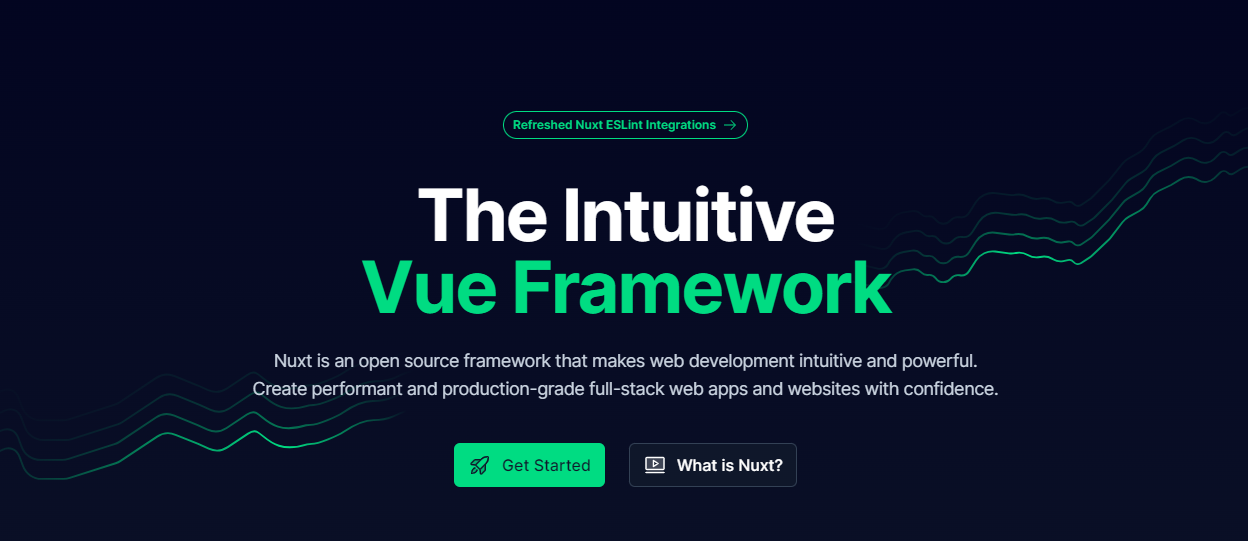 Nuxt 是一個基於 Vue.js 生態系統的漸進式開源框架,用於建立高效能 Web 應用程式,尤其是伺服器端渲染應用程式。 但請記住,Nuxt 並不是 Vue.js 的替代品,因為它無法單獨運作。而且它也不能被視為像 Express 這樣成熟的後端框架。 100 秒觀看 Nuxtjs,掌握整體概念。 {% 嵌入 https://www.youtube.com/watch?v=dCxSsr5xuL8 %} Nuxt 是建立這三種 Web 應用程式的最佳 JavaScript 框架之一 - 預先渲染靜態頁面、單頁 Web 應用程式 (SPA)、伺服器端渲染 Web 應用程式 (SSR) 甚至通用應用程式。 開發人員特別喜歡 Nuxt,因為它有豐富的函式庫和模組。 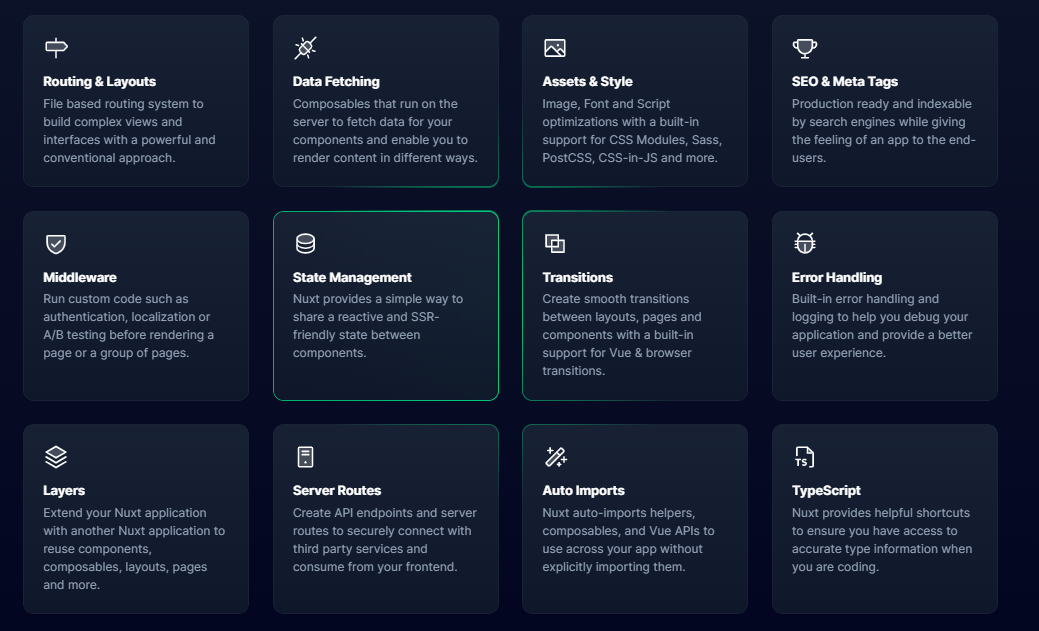 開始使用以下 npm 指令。 ``` npx nuxi@latest init <my-project> ``` 您可以閱讀[文件](https://nuxt.com/docs/getting-started/introduction)並檢查[codesandbox範例](https://codesandbox.io/s/github/nuxt/starter/tree/v3/)。 您可以按照本[指南](https://nuxt.com/docs/guide/concepts/auto-imports)了解更多關鍵概念。 有許多整合選項,因此您可以更輕鬆地繼續使用您喜歡的工具和服務。 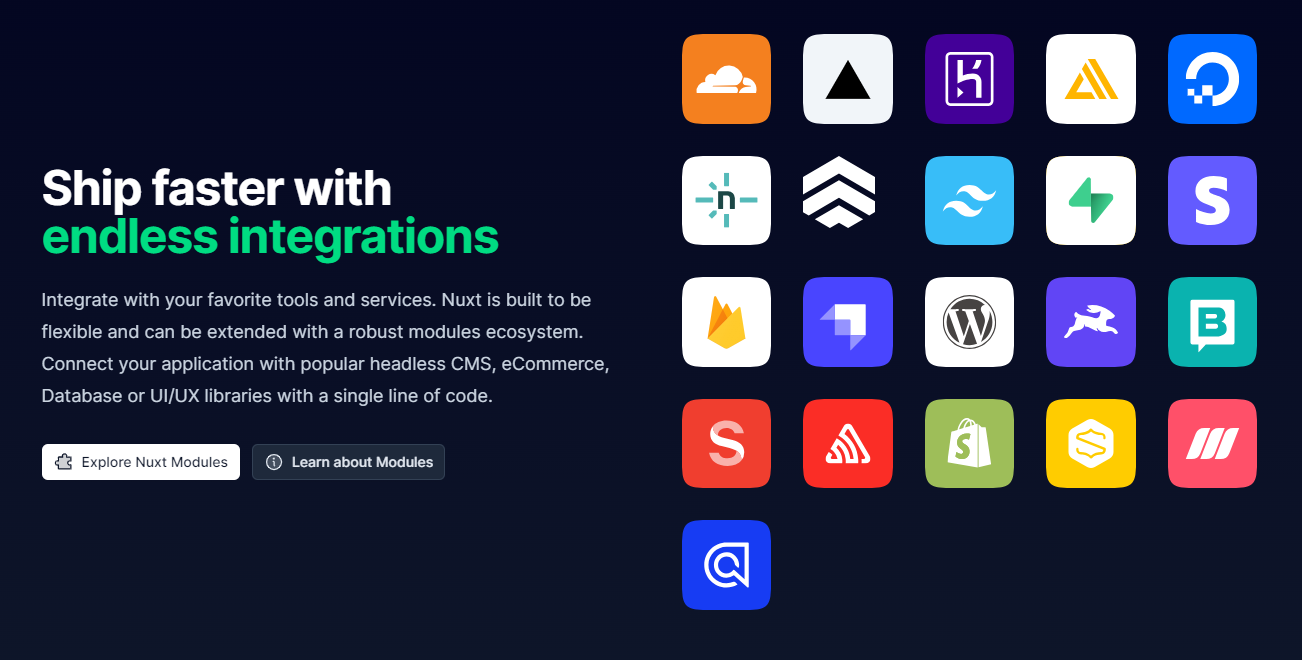 您可以查看[免費課程清單](https://nuxt.com/video-courses)來了解 Nuxt 生態系統。 如果您想要推薦的課程,請學習[Nuxt 3 — 初學者課程](https://www.youtube.com/watch?v=fTPCKnZZ2dk)— Freecodecamp 提供的 3 小時教學。 使用 Nuxt 建立的一些流行網站包括 Aircall、Amplitude、Backmarket、Bitpay、Bootstrap Vue、Fox News、Gitlab、Icons8、Instrument、MyScript、Nespresso、Note.com、Ozon.ru、Roland Garros、System76、Todoist、加油,Wappalyzer 。尋找不同類別下[展示網站的完整清單](https://nuxt.com/showcase)。 如果您想快速測試和建置,那麼我建議您查看[入門模板](https://nuxt.com/templates)。 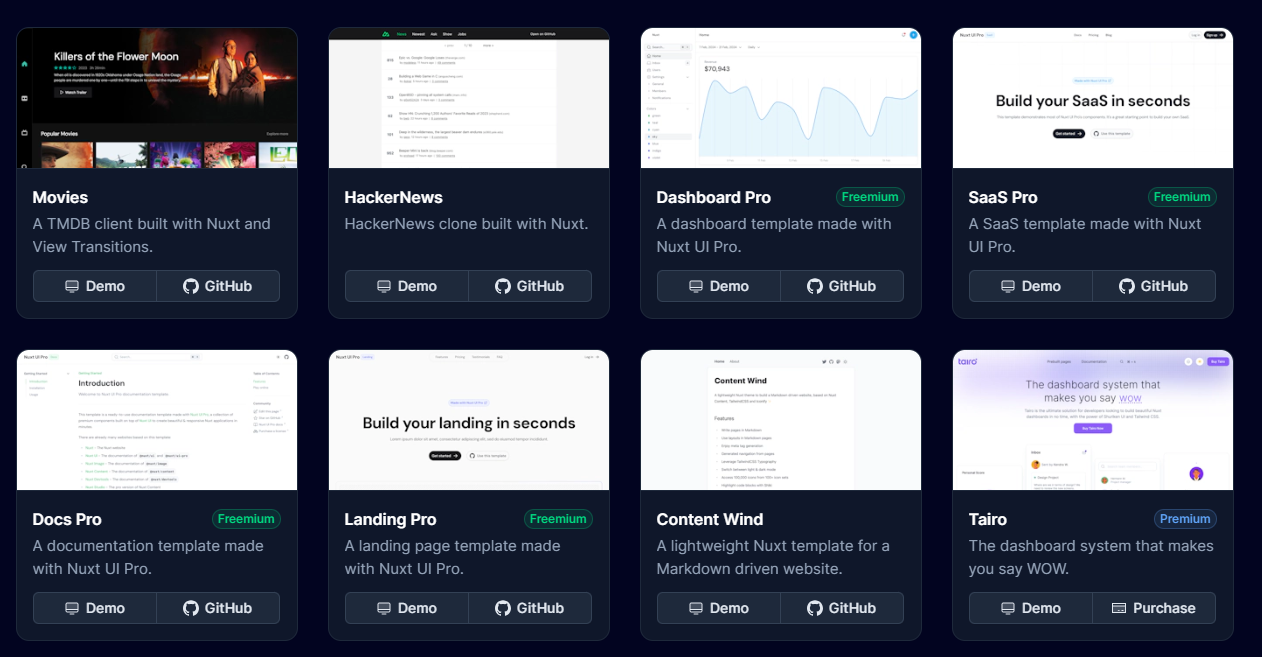 Nuxt 在 GitHub 上擁有超過 51,000 顆星,並被超過 318,000 名開發者使用。 {% cta https://github.com/nuxt/nuxt %} Star Nuxt ⭐️ {% endcta %} --- 8. [Ember.js](https://github.com/emberjs/ember.js) - 用於建立雄心勃勃的 Web 應用程式的 JavaScript 框架。 --------------------------------------------------------------------------------------- 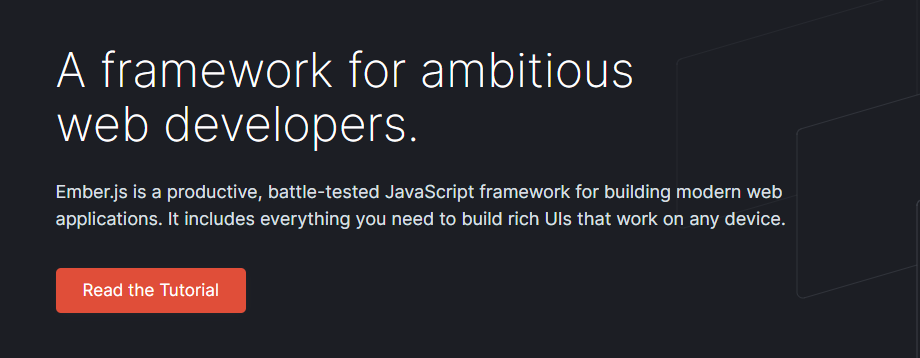 Ember.js 是一個 JavaScript 框架,用於為企業建立可擴展的單頁 Web 應用程式。與其他框架不同,模型-視圖-視圖模型 (MVVW) 架構是 Ember 的基礎。 Ember.js 最初是一個 SproutCore 2.0 框架,由其建立者 Yehuda Katz 更名為 Ember.js,Yehuda Katz 是一位出色的開發人員,被譽為 jQuery 的主要建立者之一。 他們還提供命令列介面工具。 Ember CLI 是建立、建置、測試和提供構成 Ember 應用程式或外掛程式的檔案的官方方式。 ``` npm install -g ember-cli ``` 儘管與 React、Vue 和 Svelte 相比,Ember.js 是一個較舊的前端 JavaScript 框架,但它仍然具有強大的功能,並且在 Microsoft、LinkedIn、Netflix 和 Twitch 等大公司中擁有龐大的用戶群。查看[完整清單](https://emberjs.com/ember-users/)。 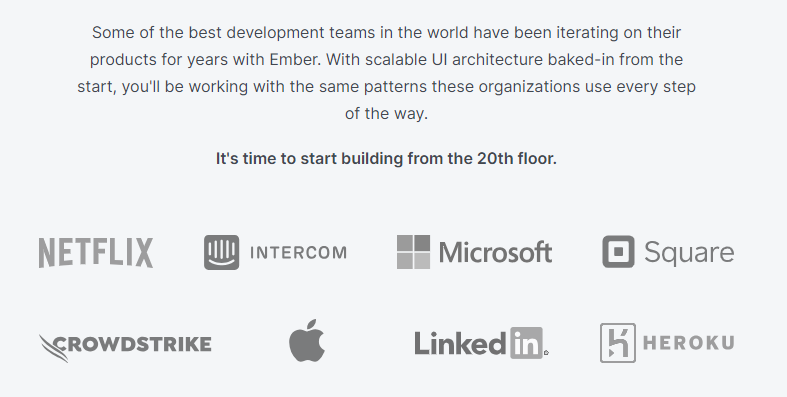 借助強大的預設設置,您可能永遠不需要在應用程式中配置任何內容,但如果您需要的話,選項就在那裡! 這意味著 Ember.js 遵循「CoC – 約定優於配置」方法,這可確保在大多數情況下不需要任何配置,以便您可以直接跳到編碼和建立 Web 應用程式。 它們還支援類似於 AngularJS 的 2 路資料綁定。 當我們深入研究時,了解 ember.js 是如何誕生的、其建立背後的先驅者以及製作開源軟體時做出的改變生活的決定非常重要。看這個! {% 嵌入 https://www.youtube.com/watch?v=Cvz-9ccflKQ %} 安裝 Ember CLI 後。 ``` npm install -g ember-cli ``` 您可以建立一個新應用程式,如圖所示。 ``` ember new ember-quickstart --lang en cd ember-quickstart npm start ``` 您可以閱讀[詳細的快速入門文件](https://guides.emberjs.com/release/getting-started/quick-start/)和[官方指南](https://guides.emberjs.com/release/)。 要學習 ember.js,您可以按照他們的官方團隊建立的[逐步教程](https://guides.emberjs.com/release/tutorial/part-1/)進行操作。您可以在[Ember API 文件](https://api.emberjs.com/ember/release)上閱讀有關 API 的更多資訊。 有數以千計的 JavaScript 庫可以在 Ember 中很好地工作。當 npm 套件提供一些 Ember 特定的功能時,他們稱之為`addon` 。外掛程式提供了一種編寫可重複使用程式碼、共用元件和樣式、擴充建置工具等的方法,所有這些都只需最少的配置。尋找[插件的完整清單](https://emberobserver.com/)。 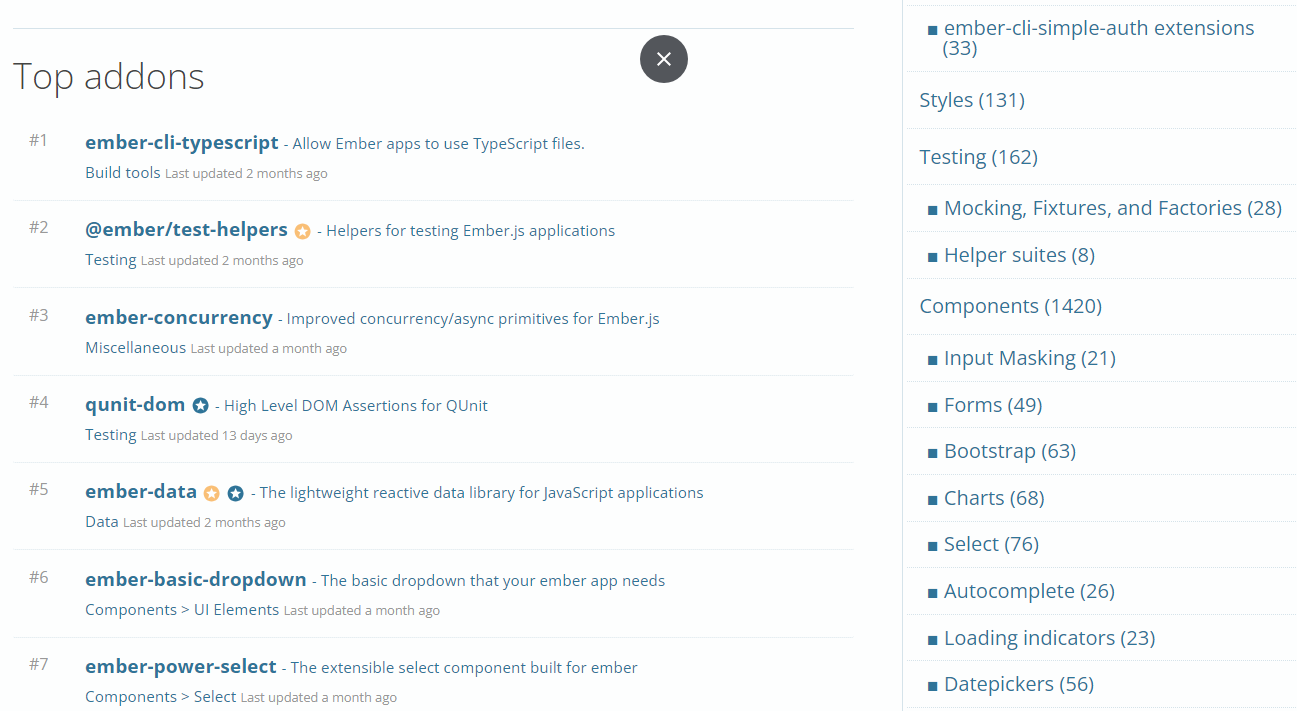 如果您正在尋找更多文章來學習 Ember.js,我推薦這些: - [Ember JS Essentials:Startech 提供的安裝及其功能的初學者指南](https://www.startechup.com/blog/ember-js/)。 - Toptal [建立您的第一個 Ember.js 應用程式的指南](https://www.toptal.com/javascript/a-step-by-step-guide-to-building-your-first-ember-js-app)。 這足以理解結構並決定 Ember 何時適合您的專案。 他們在 GitHub 上有 22k+ 顆星,而`v5.8`版本有 500 多個版本。 {% cta https://github.com/emberjs/ember.js %} 明星 Ember.js ⭐️ {% endcta %} --- 9. [Backbone.js](https://github.com/jashkenas/backbone) - 為您的 JS 應用程式提供一些帶有模型、視圖、集合和事件的 Backbone。 ------------------------------------------------------------------------------------------------- 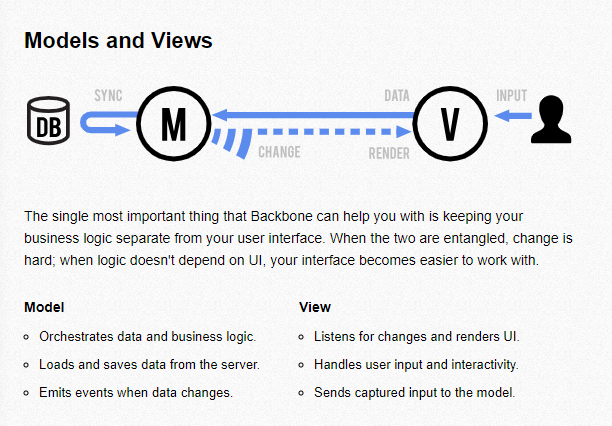 Backbone.js 是一個基於 JavaScript 的框架,透過 RESTful JSON 介面連接到 API。 Jeremy Ashkenas 因建立一些最好的 JavaScript 框架(例如 CoffeeScript 和 Underscore.js)而聞名,他於 2010 年 10 月推出了 Backbone.js。 它旨在建立單頁 Web 應用程式並維護不同 Web 應用程式元件(例如眾多客戶端和伺服器)之間的同步。 Backbone.js 以小而輕而聞名,因為它只需要 jQuery 和一個 JavaScript 函式庫 Underscore.js 即可使用整個函式庫。 Backbone.js 透過提供具有鍵值綁定和自訂事件的模型、具有豐富的可枚舉函數API 的集合、具有聲明性事件處理的視圖,為JavaScript 密集型應用程式提供結構,並透過RESTful JSON 接口將其全部連接到您現有的應用程式。 這是一個簡單的主幹視圖。 ``` var AppView = Backbone.View.extend({ // el - stands for element. Every view has an element associated with HTML // content will be rendered. el: '#container', // It's the first function called when this view is instantiated. initialize: function(){ this.render(); }, // $el - it's a cached jQuery object (el), in which you can use jQuery functions // to push content. Like the Hello World in this case. render: function(){ this.$el.html("Hello World"); } }); ``` 您可以閱讀[文件](https://backbonejs.org/)。 Backbone.js 被許多值得信賴的公司使用,例如 Walmart、Pinterest、SoundCloud 等。 您可以參考他們的[wiki](https://github.com/jashkenas/backbone/wiki/Tutorials%2C-blog-posts-and-example-sites) ,其中記錄了教程、部落格文章和範例網站。 您可以參考幾篇很棒的文章來了解更多: - [BackboneJS:入門](https://auth0.com/blog/backbonejs-getting-started/)- 推薦。 - [適合絕對初學者的 Backbone.js](https://adrianmejia.com/backbone-dot-js-for-absolute-beginners-getting-started/) - [BackboneJS 教學](https://www.tutorialspoint.com/backbonejs/index.htm)- 教學點。 根據儲存庫統計,它們在 GitHub 上擁有超過 28,000 顆星,並被超過 66,000 名開發人員使用。 {% cta https://github.com/jashkenas/backbone %} 明星 Backbone.js ⭐️ {% endcta %} --- 10. [Svelte](https://github.com/sveltejs/svelte) - 控制論增強的網路應用程式。 ---------------------------------------------------------------- 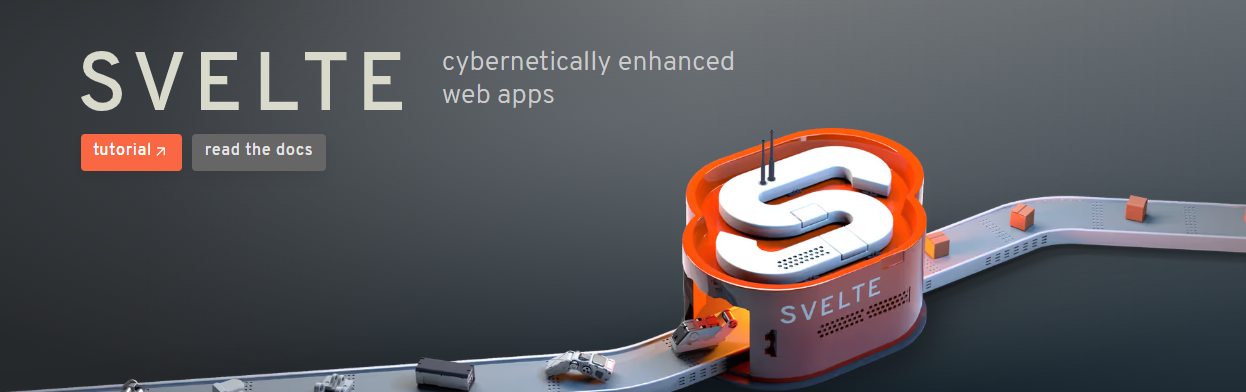 Svelte 是一種建立 Web 應用程式的新方法。 它是由 Rich Harris(著名前端開發人員)建立的。 Svelte 於 2016 年首次推出,人氣暴漲。 許多開發人員認為 Svelte 是一個真正改變遊戲規則的革命性想法,它從根本上改變了我們編碼 Web 應用程式的方式。 與 React 或 Vue.js 等其他 JavaScript 框架不同,Svelte 沒有虛擬 DOM。相反,您可以使用簡單的 HTML、CSS 和 JavaScript 程式碼來建立無樣板的元件。 然後,Svelte Compiler 在建置期間將此程式碼編譯成小型的無框架的普通 JavaScript 模組,並在狀態變更時精確地更新 DOM。 因此,與 React 或 Vue.js 等其他傳統框架不同,Svelte 不需要很高的瀏覽器處理能力。 Svelte 依靠反應式程式來徹底更新 DOM。因此,與幾乎任何其他框架相比,它可以實現最快的渲染,並且在大多數效能基準測試中名列前茅。 開始使用以下 npm 指令。 ``` npm create svelte@latest my-app ``` 您可以這樣使用它。 ``` cd my-app npm install npm run dev -- --open ``` 您可以閱讀[文件](https://svelte.dev/docs/introduction)。該團隊還提供了[官方的 VSCode 擴展](https://marketplace.visualstudio.com/items?itemName=svelte.svelte-vscode),它也可以與各種其他編輯器和工具整合。 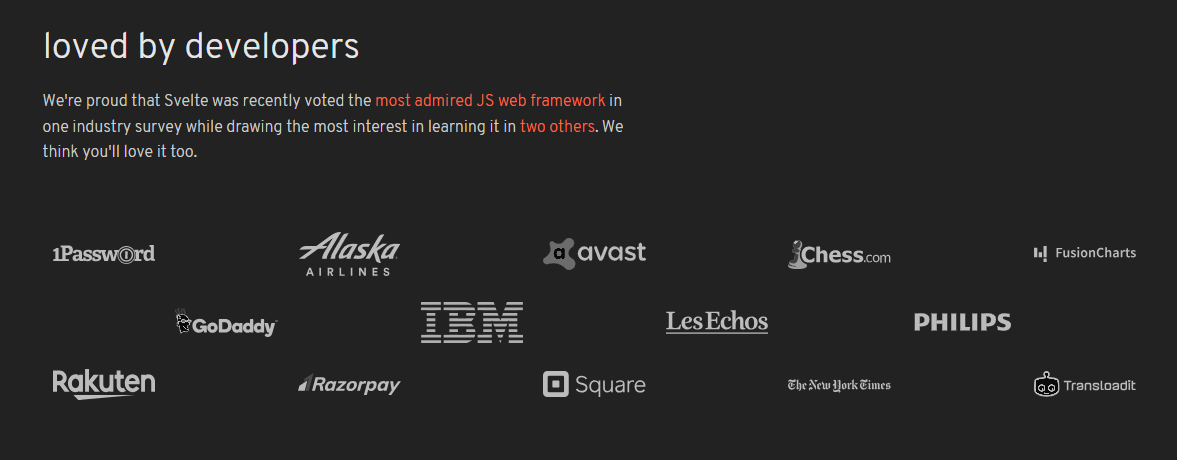 他們還提供了[詳細的基於網路的教程](https://svelte.dev/tutorial/basics)來學習 Svelte。 您可以查看所有[範例](https://svelte.dev/examples/nested-components)來了解關鍵概念和結構,包括 DOM 事件、生命週期、運動、過渡和處理 SVG。 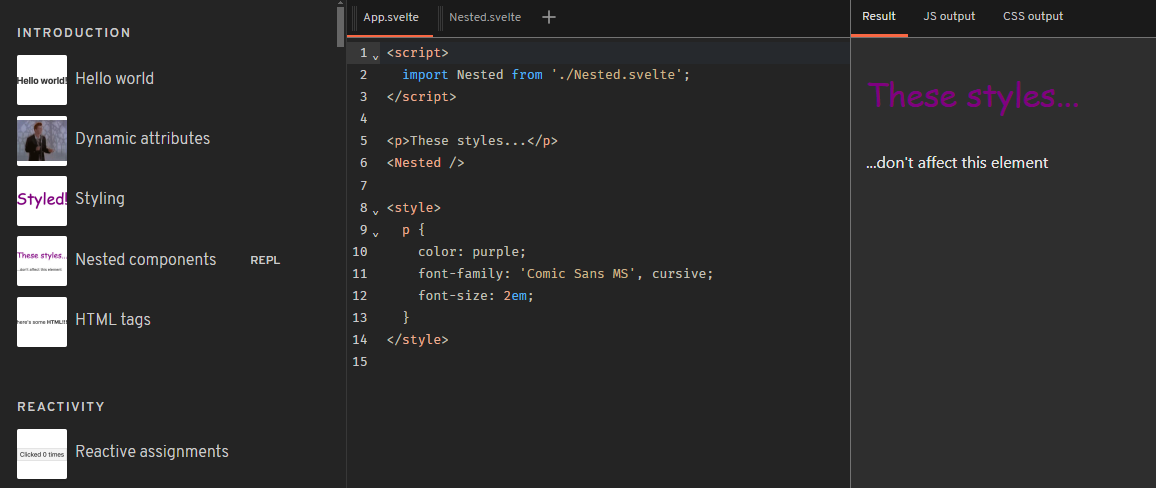 您可以觀看這些教學來了解有關 Svelte 的所有知識。 - [Learn Svelte – 初學者完整課程](https://www.youtube.com/watch?v=UGBJHYpHPvA)– Freecodecamp 的 23 小時教學。 - [Sveltekit & Tailwind](https://www.youtube.com/watch?v=vb7CgDcA_6U&t=2s) - Freecodecamp 的 2 小時教學。 非常感謝老師們免費提供如此詳細的教學! Svelte 在 GitHub 上擁有超過 76k 顆星,目前處於`v4.2`版本,有 282k 開發人員使用。 {% cta https://github.com/sveltejs/svelte %} Star Svelte ⭐️ {% endcta %} --- 11. [Remix](https://github.com/remix-run/remix) - 建立更好的網站。 ---------------------------------------------------------- 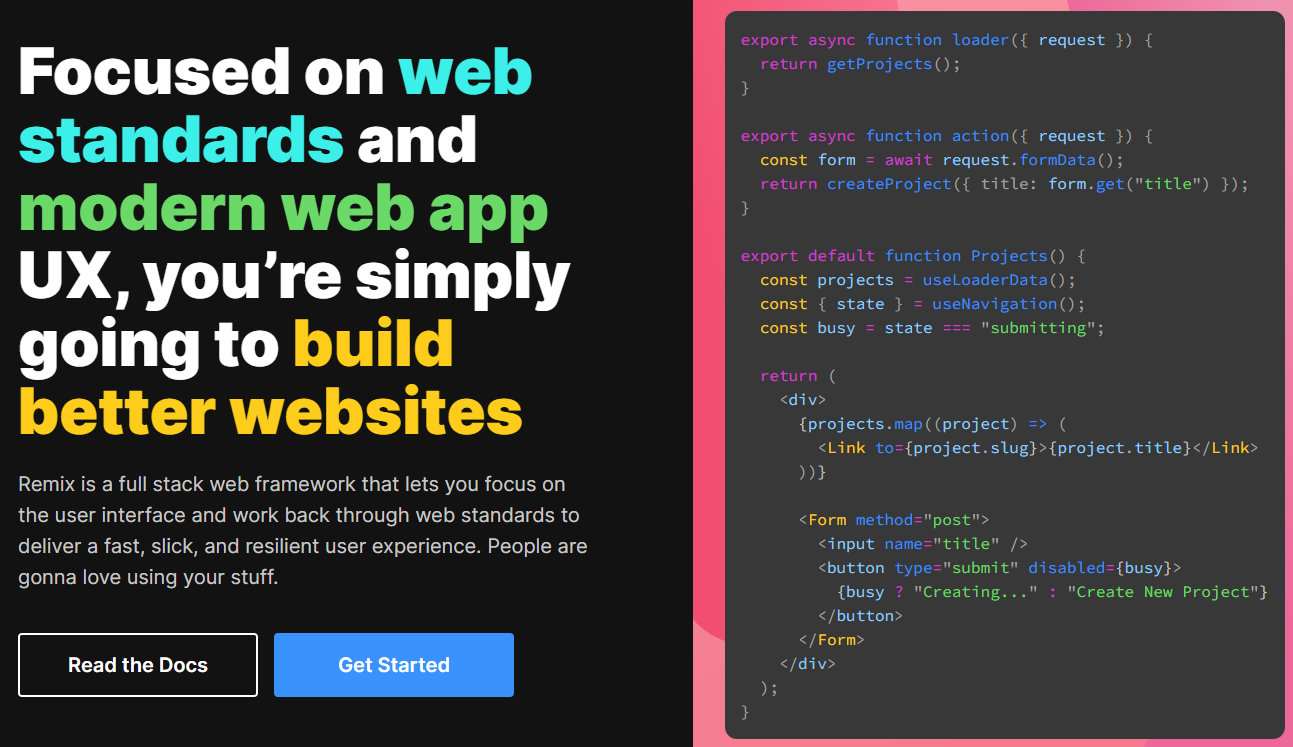 Remix 是一個全端Web 框架,可讓您專注於使用者介面並透過Web 基礎知識進行工作,以提供快速、流暢且有彈性的使用者體驗,可部署到任何Node.js 伺服器,甚至非Node. js 環境像 Cloudflare Workers 這樣的邊緣。 Remix 建構在 React Router 之上,有四個特點: - 一個編譯器 - 伺服器端 HTTP 處理程序 - 一個伺服器框架 - 一個瀏覽器框架 您可以觀看此內容以了解有關 Remix by Fireship 的更多資訊。 {% 嵌入 https://www.youtube.com/watch?v=r4B69HAOXnA&pp=ygUUcmVtaXggaW4gMTAwIHNlY29uZHM%3D %} 透過嵌套路由,Remix 可以消除幾乎所有載入狀態,如圖所示。 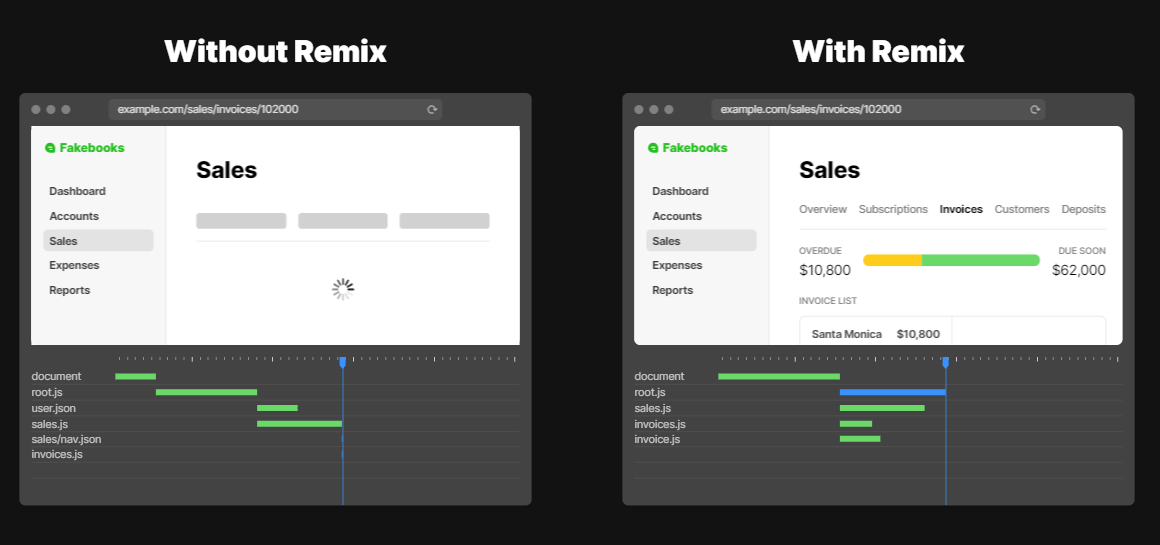 開始使用以下 npm 指令。 ``` npx create-remix@latest ``` 您可以這樣使用它。 ``` mkdir my-remix-app cd my-remix-app npm init -y # install runtime dependencies npm i @remix-run/node @remix-run/react @remix-run/serve isbot@4 react react-dom # install dev dependencies npm i -D @remix-run/dev vite ``` 如果您想包含您的伺服器,請閱讀此[快速入門指南](https://remix.run/docs/en/main/start/quickstart),並了解更多有關如何透過 Remix Vite 插件提供 Vite 配置的訊息,因為 Remix 使用 Vite。 您可以閱讀[文件](https://remix.run/docs/en/main)。他們根據你想做的事情來分發它,順便說一句,我很喜歡。 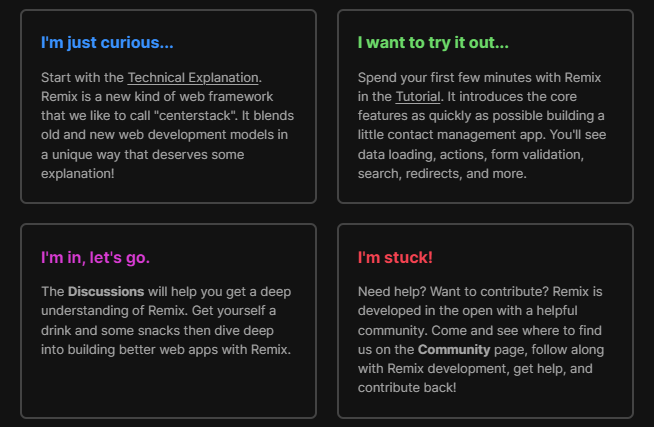 尋找使用 Remix 建立的[網站的完整清單](https://remix.run/showcase)。 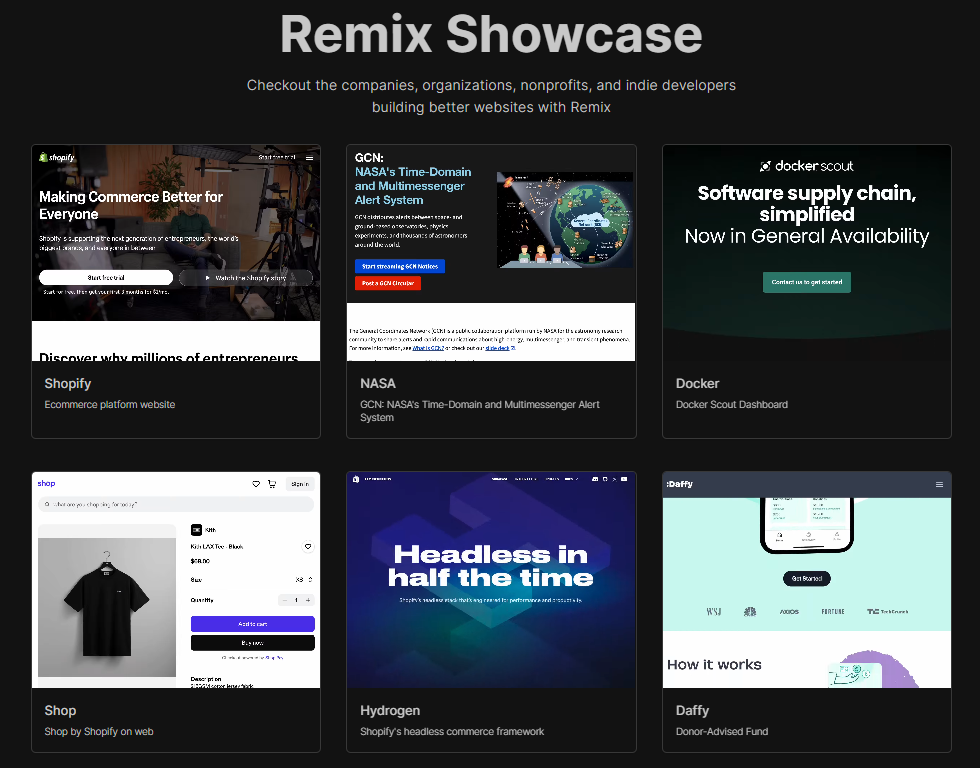 您還應該查看社區製作的[Remix 資源](https://remix.run/resources?category=all)。其中一些是有幫助的,可以改善整個生態系統。 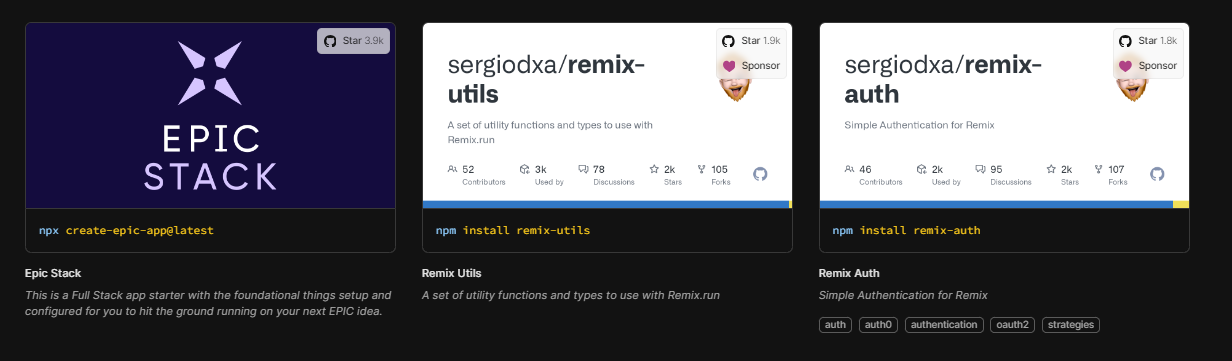 如果您是第一次接觸 Remix,我建議您閱讀官方團隊建立的[Remix 教學 -30min](https://remix.run/docs/en/main/start/tutorial) 。 他們在 GitHub 上擁有超過 27k 個 star,並且發布了`v2.8`版本。 {% cta https://github.com/remix-run/remix %} 明星混音 ⭐️ {% endcta %} --- 12. [AdonisJS](https://github.com/adonisjs/core) - TypeScript 優先的 Web 框架,用於建立 Web 應用程式和 API 伺服器。 ------------------------------------------------------------------------------------------------ 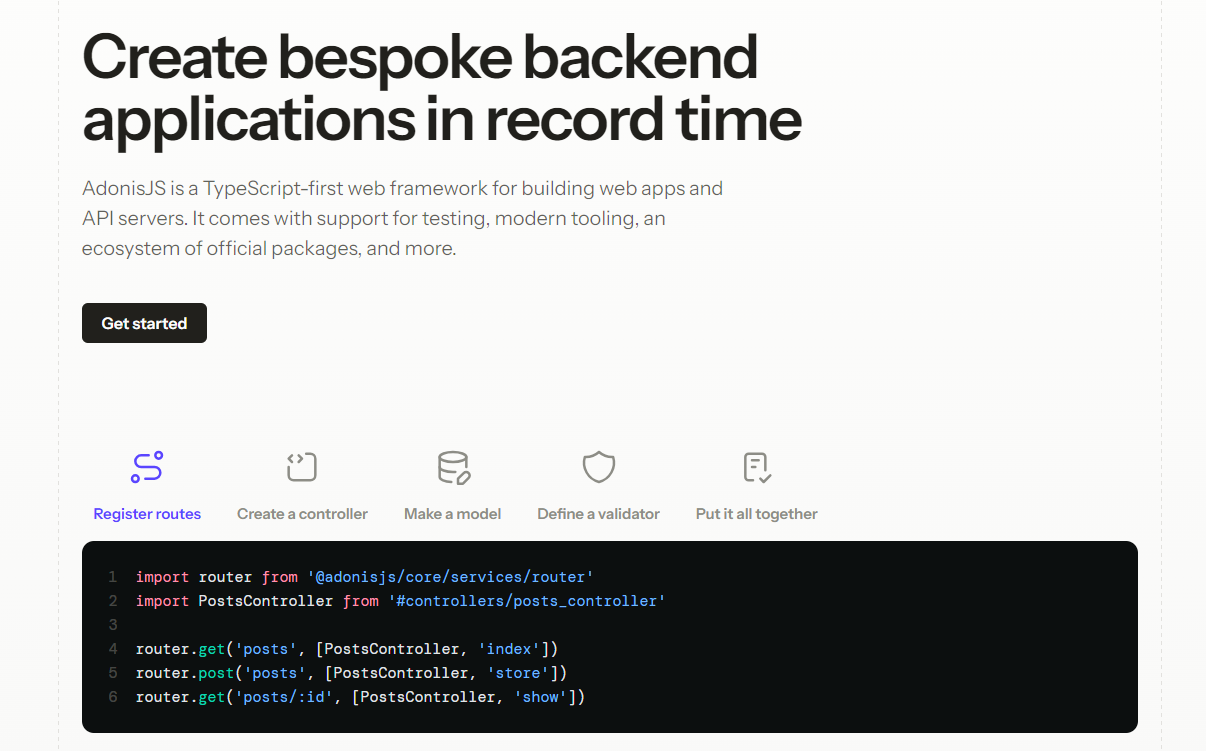 AdonisJS 是一個功能齊全的 Node.js 後端框架。該框架是從頭開始建立的,非常重視開發人員的人體工學和易用性。 AdonisJS 專注於後端,讓您選擇您選擇的前端堆疊,這意味著前端不可知。 它是 Node.js 社群中最稀有的框架之一,附帶一套第一方包,可幫助您建立和發布產品,而無需浪費數百小時組裝不同的 npm 包。 在基礎層面上,AdonisJS 為您的應用程式提供架構,配置無縫的 TypeScript 開發環境,為您的後端程式碼配置 HMR,並提供大量維護良好且記錄廣泛的軟體包。 他們強調了一點測試,這是非常好的。 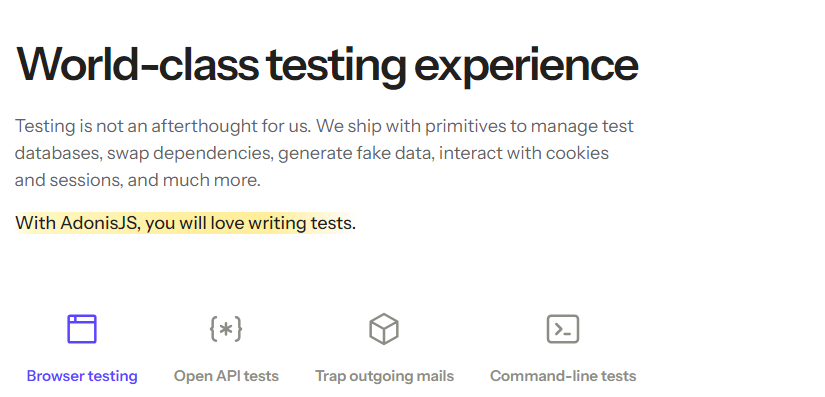 開始使用以下 npm 指令。 ``` npm init adonisjs@latest hello-world ``` AdonisJS 採用經典的 MVC 設計模式。首先,使用函數式 JavaScript API 定義路由,將控制器綁定到它們,並編寫邏輯來處理控制器內的 HTTP 請求。 ``` import router from '@adonisjs/core/services/router' import PostsController from '#controllers/posts_controller' router.get('posts', [PostsController, 'index']) ``` 控制器可以使用模型從資料庫中獲取資料並呈現視圖(也稱為模板)作為回應。 ``` import { HttpContext } from '@adonisjs/core/http' import Post from '#models/post' export default class PostsController { async index({ view }: HttpContext) { const posts = await Post.all() return view.render('pages/posts/list', { posts }) } } ``` 如果您正在建立 API 伺服器,則可以用 JSON 回應取代視圖層。但是,處理和回應 HTTP 請求的流程保持不變。 您可以閱讀[文件](https://docs.adonisjs.com/guides/introduction)。 您也可以參考[入門套件](https://docs.adonisjs.com/guides/installation#starter-kits)。 他們還提供了[VSCode 擴展,](https://marketplace.visualstudio.com/items?itemName=jripouteau.adonis-vscode-extension)如果您開始使用 Adonisjs,則應該使用該擴展。 您必須查看[Awesome Adonisjs](https://github.com/adonisjs-community/awesome-adonisjs) ,它提供了一系列很棒的書籤、軟體包、教程、影片、課程、擁有使用此內容的網站的公司以及來自 AdonisJS 生態系統的其他很酷的資源。 大多數時候,開始接觸一些非常新的東西是很困難的,因此團隊提供了[10 多個課程](https://adonismastery.com/)來了解 Adonisjs 生態系統。 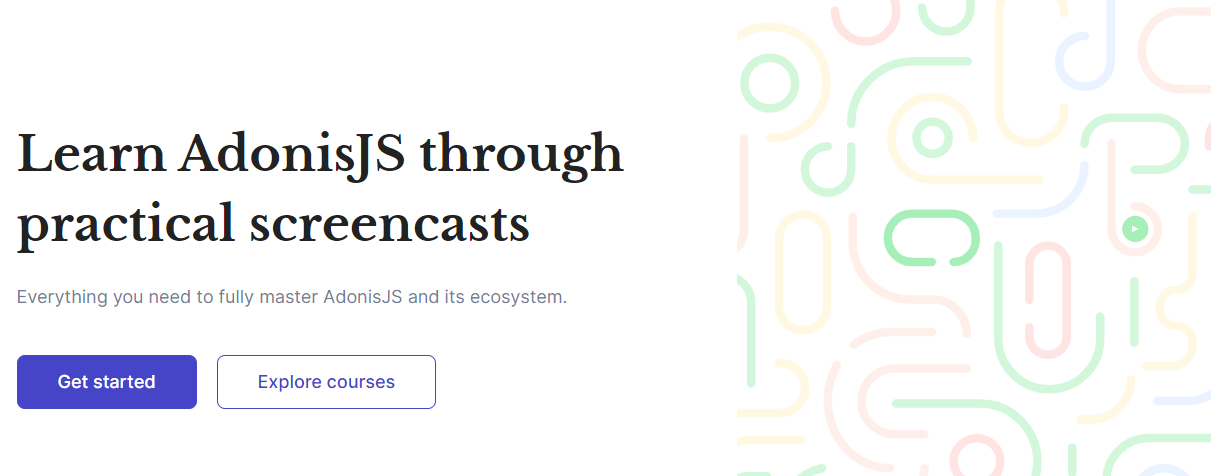 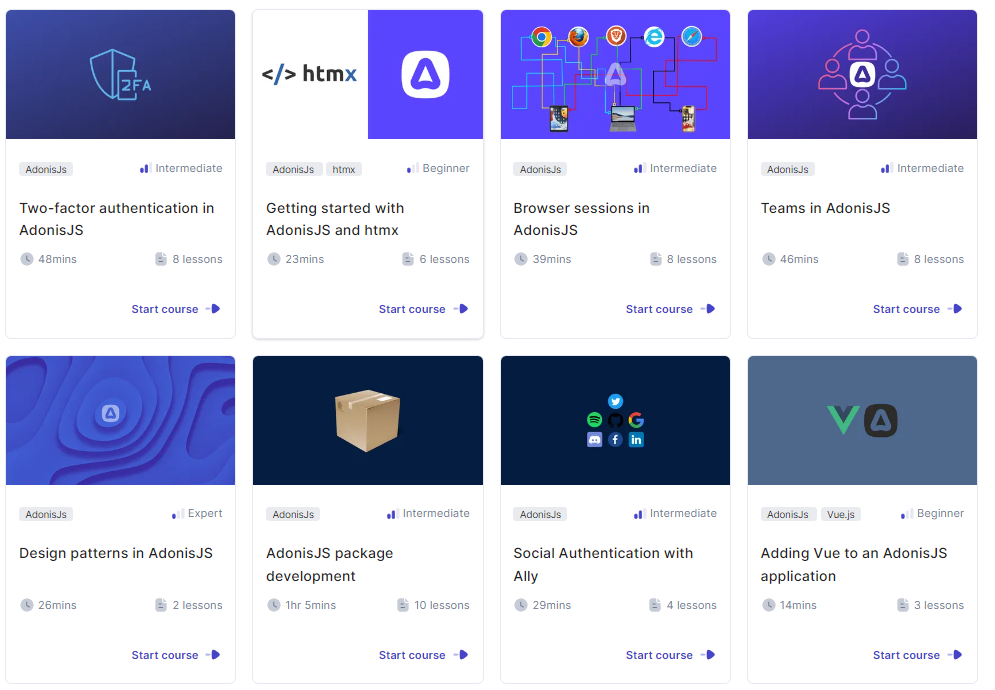 他們在 GitHub 上擁有超過 15k 個 star,並且發布了`v6.8`版本。 {% cta https://github.com/adonisjs/core %} 明星 AdonisJS ⭐️ {% endcta %} --- 13. [Astro](https://github.com/withastro/astro) - 內容驅動網站的網頁框架。 -------------------------------------------------------------- 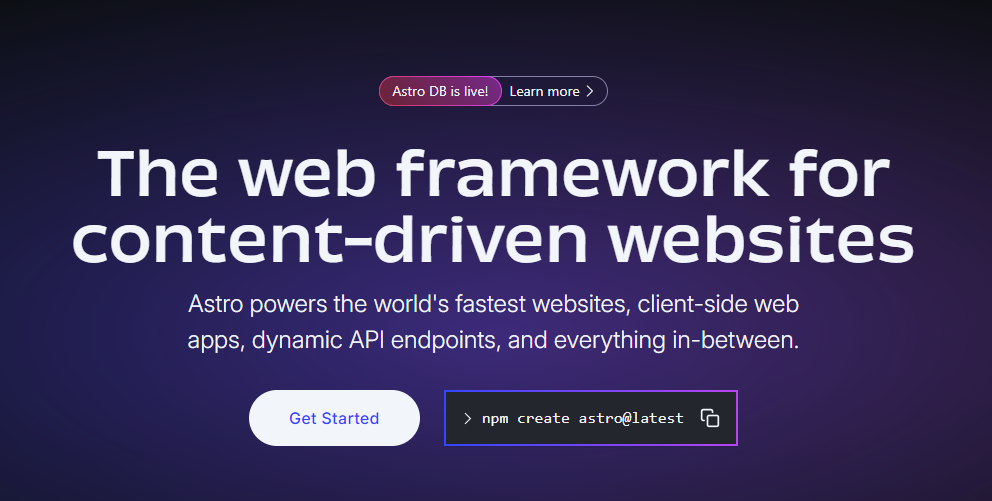 Astro 是一個開源、伺服器優先的 Web 框架,它結合了靜態網站產生 (SSG) 和伺服器端渲染 (SSR) 的優點,可建立快速、SEO 友善的網站。 Astro 專門為部落格和電子商務等內容豐富的網站提供支持,並擁有良好的開發生態系統。 開始使用以下 npm 指令。 ``` npm create astro@latest ``` 您可以閱讀使用 Astro 建立的[文件](https://docs.astro.build/en/getting-started/)和[展示的網站](https://astro.build/showcase/)。其中一些真的很棒並且視覺上令人驚嘆! Astro 支援 React、Preact、Svelte、Vue、Solid、Lit、HTMX、Web 元件等。閱讀所有[記錄的功能](https://docs.astro.build/en/concepts/why-astro/#features)。 您可以按照本教學[使用 Astro 建立您的第一個部落格](https://docs.astro.build/en/tutorial/0-introduction/)。或使用主題來快速啟動您的下一個專案。其中一些是免費的,而另一些則是付費的! 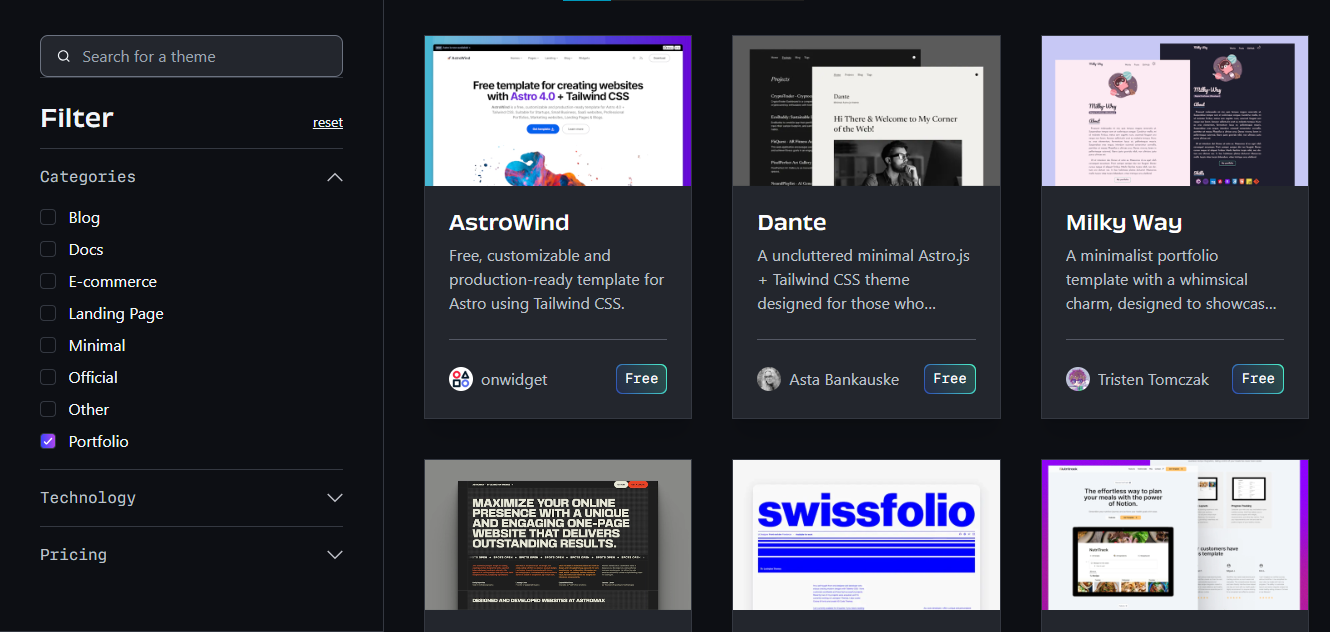 您可以看到如圖所示的加載性能,甚至我對此感到驚訝。 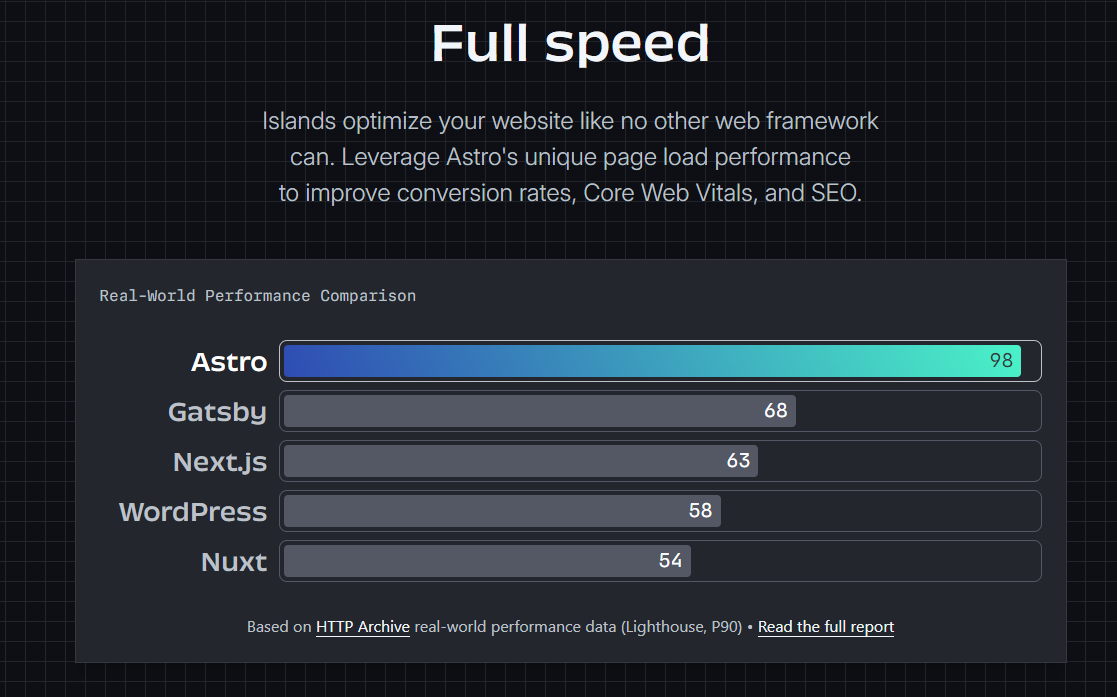 性能至關重要,尤其是在您從事商業活動時,因為高效的演算法將節省更多資金並減少麻煩。 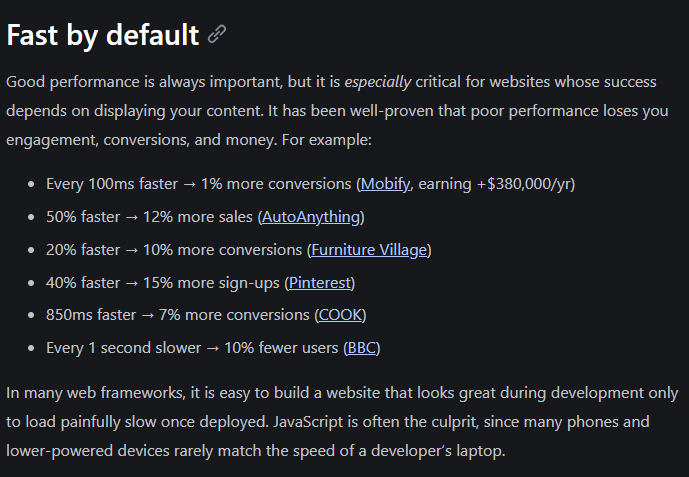 無論是在可存取性、圖標還是使用不同的庫方面,[整合選項](https://astro.build/integrations/)都是巨大的。 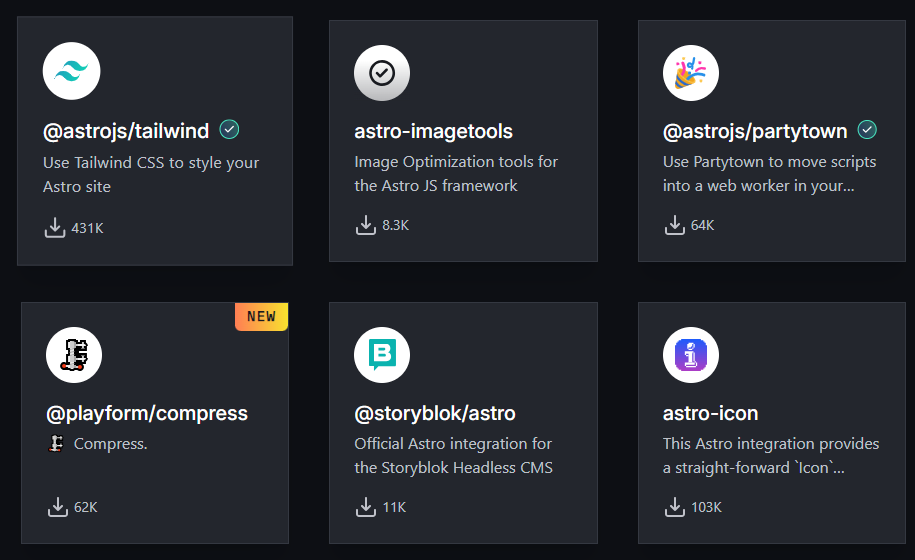 您可以觀看 Freecodecamp 提供的一小時[Astro Web 框架速成課程](https://www.youtube.com/watch?v=e-hTm5VmofI)。 {% 嵌入 https://www.youtube.com/watch?v=e-hTm5VmofI %} Astro 在 GitHub 上擁有超過 42k 顆星,處於`v4.6` (1800 多個版本),並由超過 112k 開發人員使用。 {% cta https://github.com/withastro/astro %} Star Astro ⭐️ {% endcta %} --- 14. [Fresh](https://github.com/denoland/fresh) - 下一代網路框架。 --------------------------------------------------------- 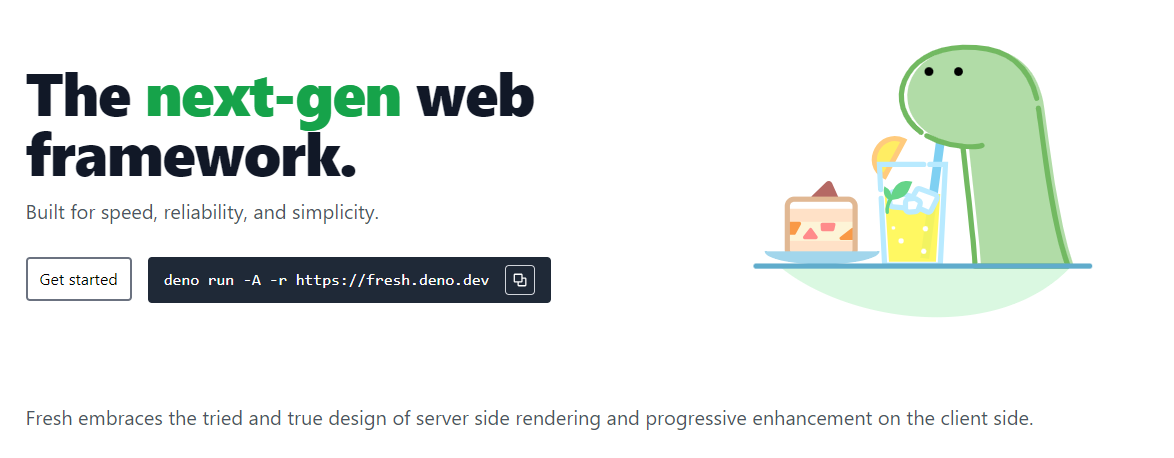 Fresh 是下一代 Web 框架,專為速度、可靠性和簡單性而建置。 一些突出的特點: - 島上的客戶水合作用可達到最大程度的互動。 - 零執行時開銷意味著預設不會將 JS 傳送到客戶端。 - 無需配置。 - 開箱即用的 TypeScript 支援。 該框架使用 Preact 和 JSX 進行渲染和模板化,處理伺服器和客戶端上的任務。 此外,Fresh 消除了建造步驟的需要。您編寫的程式碼直接在伺服器端和客戶端執行。 TypeScript 或 JSX 到純 JavaScript 的轉換是在需要時動態發生的。這有助於實現極其快速的迭代周期和快速部署。 從這個開始吧。 ``` deno run -A -r https://fresh.deno.dev ``` Fresh 採用的最重要的架構決策是其對[島嶼架構模式](https://www.patterns.dev/vanilla/islands-architecture)的使用。 這意味著 Fresh 應用程式預設將純 HTML 發送到客戶端。然後,伺服器渲染頁面的某些部分可以透過互動式小工具(島嶼)獨立重新水化。 客戶端只負責渲染頁面中互動性足以保證額外工作的部分。任何純靜態內容都沒有相關的客戶端 JavaScript,因此非常輕量級。 您可以閱讀[文件](https://fresh.deno.dev/docs/introduction)。 您可以找到所有使用此建立的[網站](https://fresh.deno.dev/showcase),例如[Max Schmidt](https://mooxl.dev/)的投資組合網站。 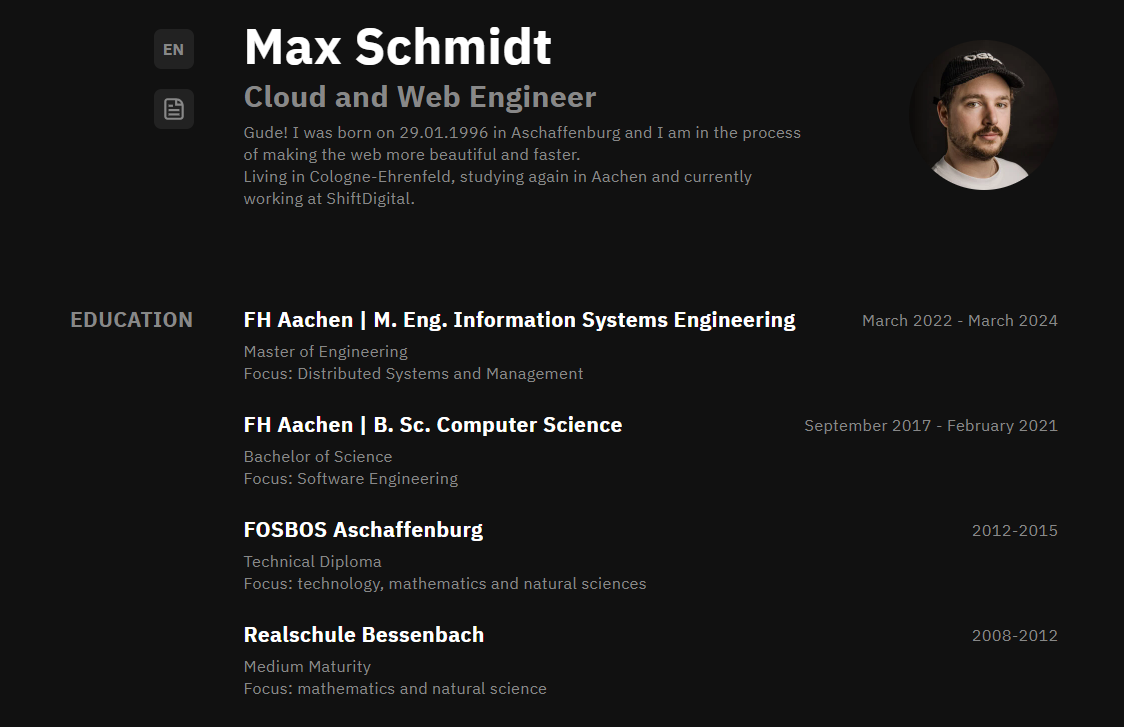 他們在 GitHub 上擁有超過 11k 個 star,並且發布了`v1.6`版本。 {% cta https://github.com/denoland/fresh %} 明星新鮮 ⭐️ {% endcta %} --- 15. [Vue.js](https://github.com/vuejs/core) - 用於在網路上建立 UI 的漸進式 JavaScript 框架。 ----------------------------------------------------------------------------- 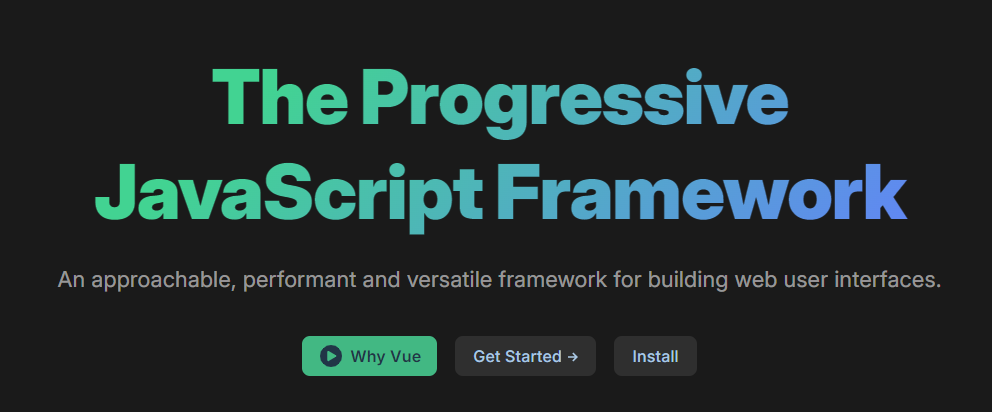 Vue.js 是一個漸進式框架,因為它能夠透過雙整合模式促進高階單頁 Web 應用程式的設計。閱讀[使用 Vue 的所有方法](https://vuejs.org/guide/extras/ways-of-using-vue.html),包括從嵌入 Web 元件到獨立腳本,甚至使用伺服器端渲染或靜態網站生成來建立複雜的應用程式。 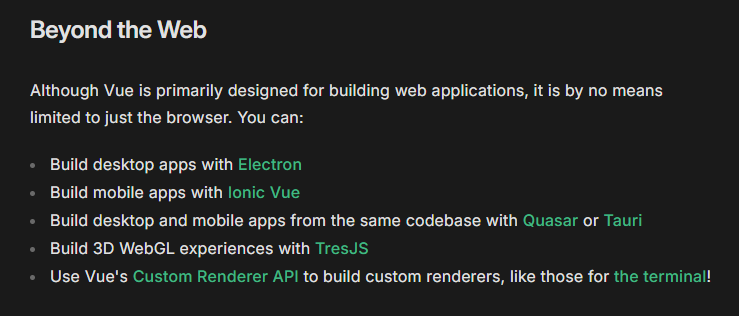 使用 MVVM(模型-視圖-視圖模型)架構,Vue.js 讓事情變得簡單、靈活且適合初學者。 Vue.js 於 2014 年由 Google 的開發人員 Evan You 首次推出,他從 AngularJS 中汲取靈感,提供了一種簡單、輕量級且高效的替代方案。 Vue.js 借用了 ReactJS 和 AngularJS 的一些功能,並對其進行了增強,以提供更流暢、更用戶友好的體驗。例如,Vue.js 將 AngularJS 的 2 路資料綁定與 React 的高效虛擬 DOM 結合。 與 React 不同,Vue 有一個內建的 MVC,可以快速輕鬆地進行設定。此外,Vue.js 的壓縮版本只有 18-20 kb,比其臃腫笨重的競爭對手(如 React 或 AngularJS)輕得多。 Vue.js 還包含一個方便的內建 CSS 過渡和動畫元件。 100 秒觀看 Vue.js 了解更多! {% 嵌入 https://www.youtube.com/watch?v=nhBVL41-\_Cw&pp=ygUXZW1iZXIganMgaW4gMTAwIHNlY29uZHM%3D %} 開始使用以下 npm 指令。 ``` npm create vue@latest ``` 該命令將安裝並執行 create-vue,官方的 Vue 專案腳手架工具。您將收到有關多個可選功能的提示,例如 TypeScript 和測試支援。 這是啟動開發伺服器的方法。 ``` cd <your-project-name> npm install npm run dev ``` 一個簡單的應用程式。 ``` import { createApp } from 'vue' createApp({ data() { return { count: 0 } } }).mount('#app') <div id="app"> <button @click="count++"> Count is: {{ count }} </button> </div> ``` 上面的例子展示了Vue的兩個核心特性: 1. **聲明式渲染**:Vue 使用模板語法擴充了標準 HTML,該模板語法基於 JavaScript 狀態以聲明方式描述 HTML 輸出。 2. **反應性**:Vue 會自動追蹤 JavaScript 狀態變化,並在變化發生時有效地更新 DOM。 您也可以使用 CDN 來使用它,CDN 將使用全域建置。閱讀[快速入門指南](https://vuejs.org/guide/quick-start)以了解更多資訊。 您可以閱讀[文件](https://vuejs.org/guide/introduction)並查看不同主題的[程式碼編輯器範例](https://vuejs.org/examples/#hello-world),甚至可以了解如何建立 Markdown 編輯器。 要體驗 Vue.js,您也可以直接在他們的[現場 Playground](https://play.vuejs.org/#eNp9kVFLwzAQx7/KeS9TmBuiT6MOVAbqg4oKvuSltLeuM01CcpmF0u/utaXVhzEISe7/vyS/yzV459ziEAlXmITMl47XylDtrGfIaZtGzdAoA5CnnJ5fDHsATxy9GSOAKhQrmD2S1ha+rNf52Wyw2m6RSUaynB6QgKlyOmWSCCDZXa2bprsF2jZZStSrpXGR4XBZ2Zz0rULxFYqVLKfTOEcOmTXbsljsgzVSRw+lMLOVKzX5V8elNUHhasRVmArnz3OvsY80H/VsR9n3EX0f6k5T+OYpkD+Qwsnj1BfEg735eKFa9pMp5FFL9gnznYLVsWMc0u6jyQX7X15P+1R1PSlN8Rk2NZMJY1EdaP/Jfb5CaebDidL/cK8XN2NzsP0F+HSp8w==)中嘗試。 我非常喜歡的一篇關於 Vue 的文章是 Michael 在 DEV 上發表的。必讀! {% 嵌入 https://dev.to/michaelthiessen/25-vue-tips-you-need-to-know-2h70 %} 如果您剛開始,您可以按照他們的團隊建立的[官方教程](https://vuejs.org/tutorial/#step-1)進行操作。 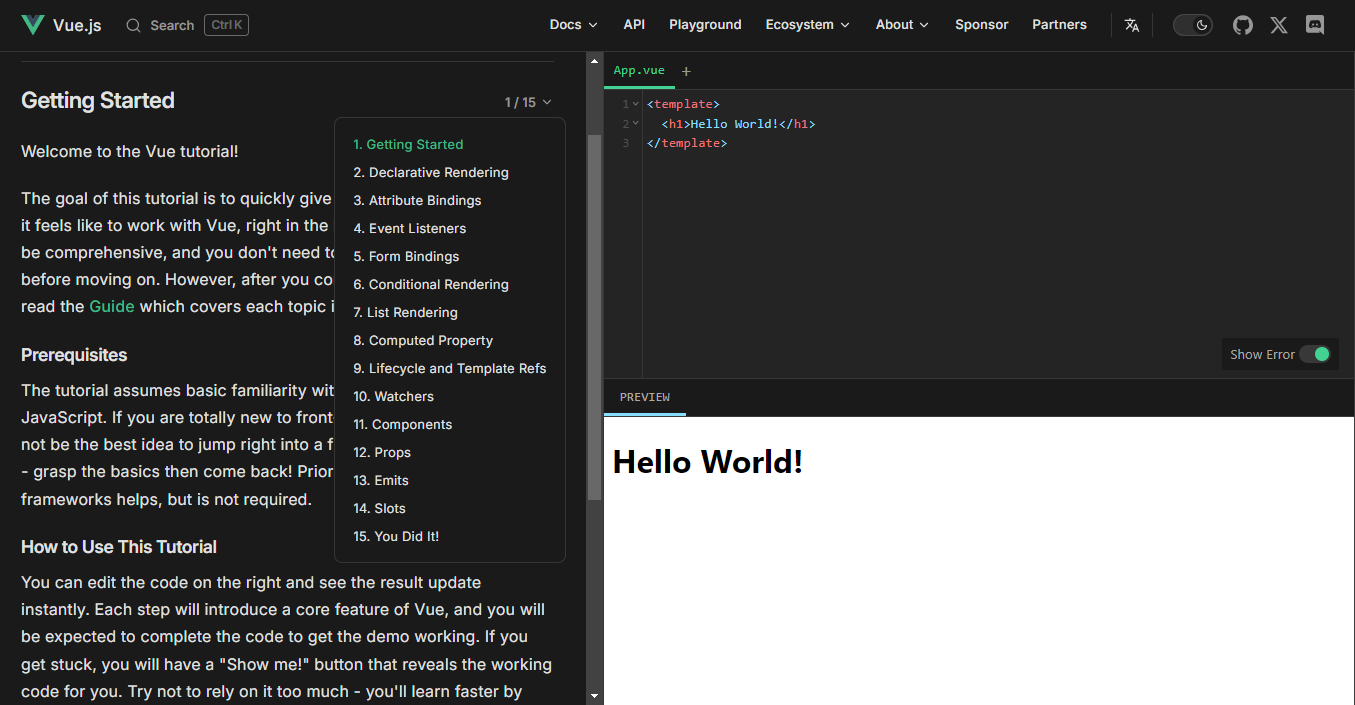 與 Astro 類似,他們也有[課程部分](https://www.vuemastery.com/courses/)和[Vue School](https://vueschool.io/) ,您可以在其中找到各種主題。 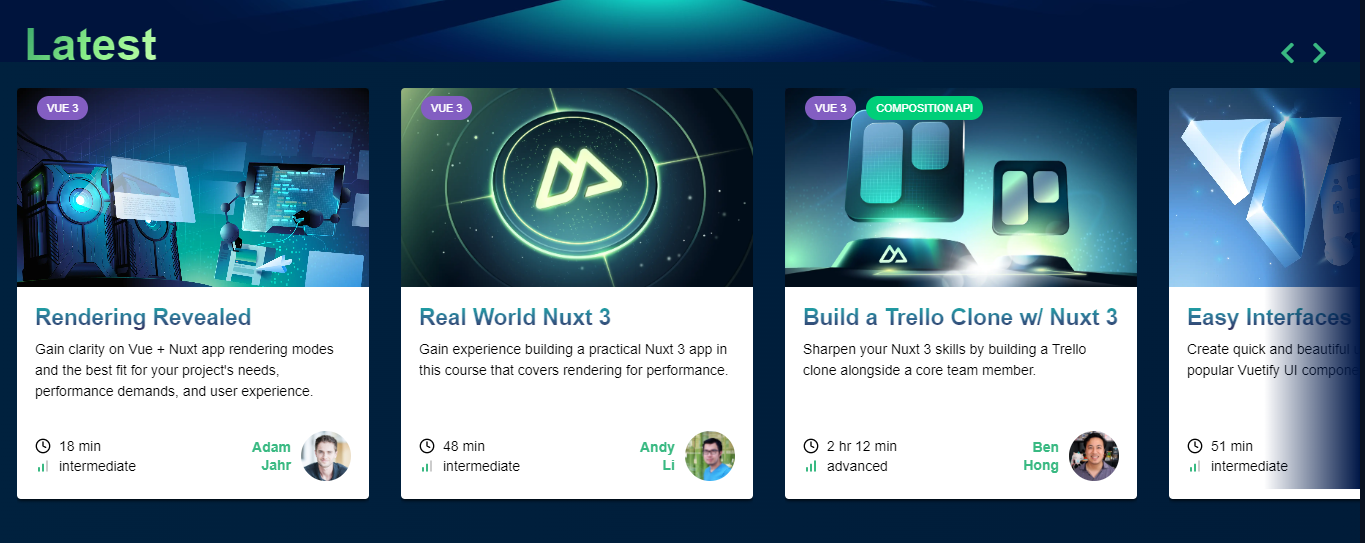 Vue.js 為許多知名網站提供支持,包括 Font Awesome、Upwork 和 Namecheap 等。 Freecodecamp 有一個[針對初學者的 3 小時 Vue](https://www.youtube.com/watch?v=4deVCNJq3qc)教程,但我不推薦它,因為它是 2019 年的,而且我們知道這些框架中的概念變化有多快。 他們在 GitHub 上擁有超過 44k 個 star,並且發布了`v3.4`版本。它是有史以來最受開發人員喜愛的框架之一。 {% cta https://github.com/vuejs/core %} Star Vuejs ⭐️ {% endcta %} --- 還有很多其他框架,您可以查看其中一些: [Aurelia.js](https://github.com/aurelia/framework) 、 [Mithril.js](https://github.com/MithrilJS/mithril.js) 、 [Stimulus.js](https://github.com/hotwired/stimulus) 、 [Meteor.js](https://github.com/meteor/meteor) 、 [Angular.js](https://github.com/angular/angular) 、 [React.js](https://github.com/facebook/react) 、 [Knockout.js](https://github.com/knockout/knockout)和[Alpine.js](https://github.com/alpinejs/alpine) 。 是的,我知道,我同時感到 😵 和興奮。哈哈! 我有一些影片推薦,可以讓本文更加深入。 {% 嵌入 https://www.youtube.com/watch?v=cuHDQhDhvPE&pp=ygUXZW1iZXIganMgaW4gMTAwIHNlY29uZHM%3D %} {% 嵌入 https://www.youtube.com/watch?v=WJRf7dh5Zws&pp=ygURZW1iZXIganMgdHV0b3JpYWw%3D %} --- 我特意製作了這一系列教程,以幫助您在一個地方找到所有內容。我希望你喜歡這個! 雖然我是 Next.js 的忠實粉絲,但探索 Wing 等其他出色的框架可能非常適合您的下一個專案。 讓我們知道您計劃使用哪些框架,或者您認為其他人是否應該了解其他內容。 祝你有美好的一天!直到下一次。 我建立技術內容是為了幫助其他人每天成長 1%,這樣您就可以在 Twitter 和 LinkedIn 上關注我以獲得每日見解。 |如果你喜歡這類東西, 請關注我以了解更多:) | [](https://twitter.com/Anmol_Codes) [](https://github.com/Anmol-Baranwal) [](https://www.linkedin.com/in/Anmol-Baranwal/) | |------------|----------| 關注Winglang以獲取更多此類內容。 {% 嵌入 https://dev.to/winglang %} --- 原文出處:https://dev.to/winglang/15-javascript-frameworks-for-your-next-project-1o7n
算法是計算的命脈。它們是電腦解決問題、分析資料和做出決策所遵循的逐步指令。就像食譜一樣,它們將複雜的任務分解為易於管理的程式。理解這些基本演算法是掌握電腦科學和程式設計的基石。 > [什麼是演算法](https://github.com/m-mdy-m/algorithms-data-structures/tree/main/1.IntroductionToAlgorithmsAndProblemSolving) 1. 搜尋演算法: --------- ### 什麼是搜尋? 搜尋是在資料集合中定位特定元素或專案的基本過程。此資料集合可以採用各種形式,例如陣列、列表、樹或其他結構化表示。搜尋的主要目標是確定資料中是否存在所需元素,如果存在,則辨識其精確位置或檢索它。它在各種計算任務和現實應用中發揮重要作用,包括資訊檢索、資料分析、決策過程等。 ### 介紹搜尋演算法 所有搜尋演算法都使用搜尋關鍵字來繼續執行該過程。搜尋演算法預計會返回成功或失敗狀態,通常以布林 true/false 表示。可以使用不同的搜尋演算法,其效能和效率取決於資料及其使用方式。 線性搜尋演算法被認為是所有搜尋演算法中最基本的。最好的也許是二分搜尋。還有其他搜尋演算法,例如深度優先搜尋演算法、廣度優先演算法等。搜尋演算法中使用的符號是 O(n),其中 n 是完成的比較次數。它給出了演算法在給定條件下所需執行時間的漸近上限的概念。 搜尋演算法中的搜尋案例可以分為最佳情況、平均情況和最壞情況。在某些演算法中,所有三種情況可能漸近相同,而在其他一些演算法中可能存在很大差異。搜尋演算法的平均行為有助於確定演算法的有用性。 > 摘要: 搜尋演算法是用於在資料集合中定位特定資料的逐步過程。它被認為是計算中的基本過程。在電腦科學中,搜尋資料時,快速應用程式和較慢應用程式之間的差異通常在於使用正確的搜尋演算法。 ### 術語: - **目標元素:**這就是你要尋找的寶藏!這是您想要在集合中尋找的特定資料。想像搜尋電話簿 - 目標元素是特定人的電話號碼。 - **搜尋空間:**將其視為可能隱藏針的大海撈針。它代表您正在搜尋的整個資料集合。這可以是數字陣列、名稱列表或更複雜的資料結構。 - **複雜度:**這是指搜尋演算法所需的工作量。這就像衡量圖書館員需要做多少工作才能找到你的書。複雜性通常以時間(找到目標需要多長時間)和空間(演算法需要多少額外記憶體)來衡量。 - **確定性與非確定性:**搜尋演算法可以像遵循食譜(確定性)或進行有根據的猜測(非確定性)。確定性演算法始終遵循相同的清晰步驟,例如二分搜尋,它將搜尋空間一分為二。非確定性演算法(例如線性搜尋)可能需要在最壞的情況下檢查整個集合。 ### 實際應用 - **資訊檢索:**想像在網路上搜尋特定的食譜。像Google這樣的搜尋引擎利用複雜的搜尋演算法來篩選大量資料集,為網站和內容建立索引。當您輸入查詢時,這些演算法會辨識與您的搜尋字詞最相關的網頁,並在幾分之一秒內提供您要尋找的資訊。 - **資料庫系統:**資料庫儲存大量訊息,從客戶記錄到金融交易。搜尋演算法是高效資料檢索的支柱。當您在資料庫管理系統中提交查詢時,搜尋演算法會快速找到符合您條件的特定記錄,從而節省您的時間和精力。 - **電子商務:**線上購物因高效搜尋而蓬勃發展。電子商務平台使用搜尋演算法來幫助您找到完美的產品。它們允許您根據價格、品牌或顏色等各種標準進行過濾和搜尋。在幕後,搜尋演算法為這些過濾器提供支持,精確定位滿足您偏好的產品,使您的購物體驗順暢高效。 - **網路:**網路是一個由互連設備組成的複雜網路。搜尋演算法在有效路由資料包方面發揮著至關重要的作用。它們可協助確定訊息在網路上傳輸的最佳路徑,確保您的視訊通話或線上遊戲順利運作。 - **人工智慧(AI):**人工智慧正在為許多領域帶來革命性的變化。搜尋演算法是人工智慧應用的基本工具。它們使人工智慧系統能夠解決問題、做出決策,甚至玩國際象棋等遊戲。透過有效地搜尋大量資料並辨識模式,搜尋演算法有助於人工智慧背後的智慧。 - **模式辨識:**模式辨識允許電腦辨識和理解資料中的模式,例如圖像、語音或手寫。搜尋演算法對於模式辨識很有幫助。它們可協助電腦將新資料與現有模式進行匹配,從而實現照片中的臉部辨識或虛擬助理的語音辨識等應用。 ### 演算法類型:  **1. `Linear Search` :** 想像一下,在一個雜亂無章的書架中尋找一本特定的書。線性搜尋模仿了這種方法。這是一種簡單的方法,可以**逐項**檢查集合中的每個專案,直到找到目標元素(您想要的書)。 **它的工作原理如下:** 1. 搜尋從集合中的第一項開始。 2. 此演算法將目標元素與目前專案進行比較。 ``` * If they match, the search is successful, and the algorithm returns the location (index) of the target element. ``` ``` * If they don't match, the algorithm moves on to the next item in the collection. ``` 3. 這種比較和移動的過程將持續下去,直到找到目標元素或耗盡整個集合。 **例子:** 讓我們在未排序清單中搜尋值「25」:\[10, 4, 12, 25, 18, 7\]。 - 搜尋從第一個元素 (10) 開始。由於 10 不是 25,我們繼續。 - 我們將目標 (25) 與下一個元素 (4) 進行比較。沒有匹配項,所以我們繼續。 - 重複此過程,直到到達索引 3 處的元素「25」。 **優勢:** - 易於理解和實施,使其成為初學者的好選擇。 - 適用於未排序的資料,在各種情況下提供靈活性。 **弱點:** - 對於大型資料集來說速度很慢。隨著集合大小的增加,搜尋時間也會成比例增加,導致海量資料集效率低。 > 怎麼運作的 : 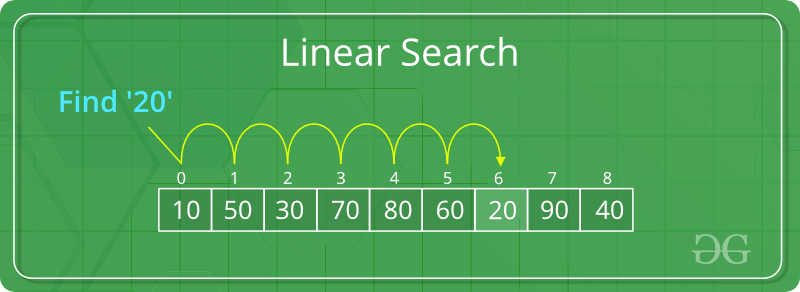 **2. `Binary Search` :** 二分搜尋在**排序資料**上蓬勃發展。想像一下在一本精心組織的字典中搜尋特定單字。在這種情況下,二分搜尋比線性搜尋快得多。它透過重複地將已排序的集合分成兩半來進行操作。策略如下: 1. 此演算法首先將目標元素與集合的中間元素進行比較。 2. 如果目標元素等於中間元素,則搜尋成功,演算法會傳回中間元素的索引。 3. 如果目標元素**小於**中間元素,則在剩餘集合的**左半部**(不包括中間元素)繼續搜尋。 4. 如果目標元素**大於**中間元素,則在剩餘集合的**右半部**繼續搜尋。 5. 在集合的縮小範圍的一半上重複步驟 1-4,直到找到目標元素或搜尋空間減少到單一元素(與目標不符)。 **例子:** 讓我們在排序清單中搜尋值「18」:\[4, 7, 10, 12, 18, 25\]。 - 中間的元素是 12。 - 現在,右半部的中間元素是18。目標元素位於索引 4 處。 **優勢:** - 對於大型排序資料集,比線性搜尋快得多。透過在每次比較時消除一半的剩餘元素,二分搜尋可以快速縮小搜尋空間。 **弱點:** - 要求預先對資料進行排序,如果資料尚未組織,則加入額外的步驟。 - 不適合未排序的資料。二分搜尋策略依賴資料的排序性質,以在每次迭代期間有效地消除一半的可能性。 > 怎麼運作的 : 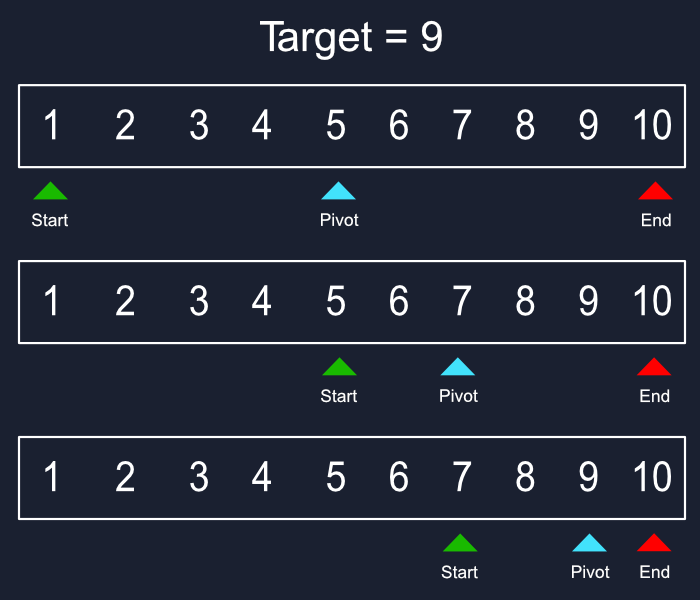 --- 2. 排序演算法: --------- ### 什麼是排序? 排序是指根據元素上的比較運算子對給定陣列或元素列表進行重新排列。比較運算子用於決定對應資料結構中元素的新順序。排序意味著將所有元素按升序或降序重新排序。 ### 介紹 在計算機科學中,排序演算法是將列表中的元素依序排列的演算法。最常用的順序是數字順序和字典順序,以及升序或降序。高效排序對於優化需要輸入資料位於排序清單中的其他演算法(例如搜尋和合併演算法)的效率非常重要。排序對於規範化資料和產生人類可讀的輸出通常也很有用。 形式上,任何排序演算法的輸出必須滿足兩個條件: 輸出是單調順序的(根據所需的順序,每個元素不小於/大於前一個元素)。 1. 輸出是輸入的排列(重新排序,但保留所有原始元素)。 2. 為了獲得最佳效率,輸入資料應儲存在允許隨機存取的資料結構中,而不是僅允許順序存取的資料結構中。 > 摘要:排序演算法是一組指令,它將陣列或列表作為輸入並將專案排列成特定的順序。排序最常見的是數字或字母(或字典)順序,並且可以按升序(AZ,0-9)或降序(ZA,9-0)。 ### 術語: - **就地分類:**想像一下重新整理抽屜裡的衣服而不把所有東西都拿出來。就地排序的工作原理類似。這些演算法透過修改現有清單本身內元素的順序來對資料進行排序,從而需要最少的額外空間。例如選擇排序、冒泡排序和插入排序。 - **內部排序:**這是指完全在電腦主記憶體 (RAM) 內執行的排序演算法。整個資料集可以一次載入到記憶體中,使其適合中小型資料集。堆排序、冒泡排序和合併排序等內部排序演算法通常用於記憶體資料操作。 - **外部排序:**當處理超出主存容量的海量資料集時,外部排序就發揮作用。這些演算法將大型資料集分解為較小的區塊,在磁碟(輔助儲存)上對它們進行排序,然後按特定順序將排序後的區塊合併在一起。合併排序是外部排序演算法的一個流行範例。 - **穩定排序:**想像一下按標題對書籍清單進行排序,但您也希望保留具有相同標題的書籍最初列出的順序。穩定的排序演算法在排序過程中保持具有相等鍵(值)的元素的相對順序。歸併排序和插入排序是穩定排序演算法的範例。 - **不穩定排序:**並非所有排序演算法都優先考慮順序保留。不穩定的排序演算法僅專注於實現所需的排序順序(例如,升序或降序),並且可能會打亂具有相同鍵的元素的相對位置。快速排序和堆排序是不穩定排序演算法的例子。 ### 排序演算法的特徵: - **時間複雜度:**這是指排序演算法完成其工作所需的時間(以步驟或比較而言)。我們通常在三種情況下分析時間複雜度: ``` * **Worst-case:** This represents the maximum amount of time the algorithm could take for a particular data size, considering the worst possible input scenario. ``` ``` * **Average-case:** This reflects the average time the algorithm takes across various random inputs of the same size. ``` ``` * **Best-case:** This represents the minimum amount of time the algorithm could take for a specific data size, considering the most favorable input scenario (e.g., data already partially sorted). ``` - **空間複雜度:**這是指排序演算法在原始資料所佔用的空間之外執行其操作所需的額外記憶體量。就地排序演算法透過在現有記憶體分配中操作資料來使用最少的額外空間,而某些演算法在排序過程中可能需要額外的臨時儲存。 - **穩定性:**此屬性決定排序演算法是否保留排序輸出中具有相等值的元素的原始順序。穩定的排序演算法維護這些元素的相對位置,這在特定應用中可能很重要。例如,如果您按姓名對客戶記錄清單進行排序,並且兩個客戶具有相同的姓名,您可能需要穩定的排序來維護它們最初列出的順序(例如,按帳戶建立日期)。 - **就地排序:**如前所述,就地排序演算法非常節省內存,因為它們透過修改原始列表本身來對資料進行排序,從而需要最少的額外空間。當處理大型資料集或記憶體有限時,這可能是有利的。 - **適應性:**一些排序演算法可以適應它們正在排序的資料的特徵。自適應排序演算法可以利用資料中預先存在的順序來提高其效能。例如,如果資料已經部分排序,自適應演算法可能會調整其方法以利用該部分順序並實現更快的排序。 ### 排序演算法的應用: - **搜尋演算法:**想像在電話簿中搜尋特定聯絡人。排序演算法是二分搜尋等高效搜尋演算法的無聲夥伴。透過確保電話簿條目按字母順序組織(排序),二分搜尋可以比透過未排序清單的線性搜尋更快找到您的聯絡人。 - **資料管理:**資料是現代計算的命脈,但有效管理資料需要組織。排序演算法在資料管理中起著至關重要的作用。當您按名稱、日期或大小對文件清單進行排序時,您可以更輕鬆地找到所需的特定文件。此外,排序的資料有助於更快地檢索和分析,從而節省您的時間和精力。 - **資料庫最佳化:**資料庫儲存大量訊息,從客戶記錄到金融交易。排序演算法顯著提高了資料庫查詢的效能。當您在電子商務資料庫中搜尋特定產品時,資料庫可能會根據您的搜尋條件(例如價格)對產品清單進行排序,以快速提供最相關的結果。 - **機器學習:**機器學習演算法從資料中學習以進行預測或分類。然而,需要準備好資料才能進行有效的學習。排序演算法通常用於資料預處理步驟,以在將資料輸入機器學習模型之前組織和建構資料。這可以顯著提高學習過程的準確性和效率。 - **資料分析:**資料分析就是從資訊中提取見解。排序演算法在這個過程中起著至關重要的作用。透過按各種屬性(例如日期、位置、客戶人口統計)對資料進行排序,您可以辨識在未排序的資料集中可能不易明顯的模式、趨勢和異常值。這使得資料分析師能夠更深入地了解資料,為金融、行銷和科學研究等各個領域的更好決策提供資訊。 - **作業系統:**作業系統管理電腦的資源。排序演算法適用於各種作業系統任務。例如,排序演算法可用於確定 CPU 任務的優先權、有效管理記憶體分配或以目錄結構組織文件,從而確保電腦系統的平穩運作。 > 這些只是排序演算法如何滲透到我們日常生活中的幾個例子。 ### 簡單解釋: 想像一下,你有一個雜亂的書架,裡面擺滿了各種主題的書。您想要按類型來組織它們(對它們進行排序)。以下是應用於此場景的排序演算法背後的主要概念和邏輯: 1. **比較:**您一次拿兩本書,並根據它們的類型進行比較(就像比較清單中的兩個元素)。 2. **交換:**如果類型不符合所需的順序(例如,歷史書放在小說之前),則交換它們在書架上的位置(就像重新排列清單中的元素一樣)。 3. **重複:**您繼續一次比較和交換兩本書,直到所有書籍按類型按所需順序排列(清單中的所有元素都根據所選標準排序)。 ### 排序演算法:: **1. 冒泡排序** **概念:**冒泡排序是一種簡單的排序演算法,它迭代列表,反覆比較相鄰元素,如果順序錯誤則交換它們。想像氣泡上升到液體表面 - 具有較大值的元素每次通過都會「冒泡」到清單中。這個過程一直持續到不需要交換為止,這表示清單已排序。 **解釋:** 想像一下將一堆亂七八糟的玩具進行分類。冒泡排序的工作原理如下: 1. **進行多次遍歷:**您多次瀏覽玩具清單。 2. **比較鄰居:**在每一遍中,您將每個玩具與其鄰居進行比較。如果第一個玩具更大(或排序術語中的“更高”),則交換它們的位置。 3. **泡泡上升:**每次交換時,較大的玩具(如泡泡)往往會移向清單的末端。 4. **繼續直到沒有交換:**您在整個清單中重複這些比較和交換,直到完成一次完整的傳遞而無需交換。這表示列表已排序。 **時間複雜度:**不幸的是,在最壞情況和平均情況下,冒泡排序的時間複雜度都是 O(n^2)。這意味著排序時間隨著元素數量 (n) 的二次方增加。對於大型資料集,冒泡排序變得非常低效。 **2.選擇排序** **概念:**選擇排序也遍歷列表,但它不是直接比較相鄰元素,而是專注於尋找未排序部分中的最小(或降序最大)元素。然後,該元素與未排序部分中的第一個元素交換,有效地將其放置在正確的排序位置。重複此過程,在每次通過時逐漸將未排序部分減少一個位置。 **解釋:** 想像一下根據身高安排學生拍照。選擇排序的工作原理如下: 1. **尋找最矮的(或最高的):**在每次遍歷中,您搜尋該行的整個未排序部分以尋找最矮的學生(或按降序排列最高的學生)。 2. **與第一個學生交換:**一旦找到最矮的學生,就可以與未排序部分中的第一個學生交換他們的位置,有效地將他們放在行開頭的正確排序位置(最矮的在前面) 。 3. **重複並減少未排序部分:**繼續此過程,將交換的元素視為已排序部分的開頭,並在剩餘的未排序部分中搜尋最小元素。 **時間複雜度:**與冒泡排序類似,選擇排序在平均情況和最壞情況下的時間複雜度均為 O(n^2)。這意味著排序時間隨元素數量呈二次方增長,從而導致大型資料集效率低下。 **3.插入排序** **概念:**插入排序的工作原理是維護一個已排序的子列表,並迭代地將未排序部分中的元素插入到子列表中的正確位置。想像一下用塊建造一座塔,但您只能將它們一一加入,並且希望保持塔按高度排序。插入排序就像策略性地將每個新區塊放置在不斷增長的排序塔中的正確位置。 **解釋:** 想像一下按高度對書架上的書籍進行排序。插入排序的工作原理如下: 1. **從單一排序元素開始:**從一個空的排序子清單開始(就像書架上只有一本書)。 2. **從未排序的部分中取出一個元素:**您從未排序的堆中挑選一本書。 3. **移動與插入:**您可以將新書與已排序子清單中的每本書進行比較,從右端開始。如果新書較短(或按降序排列較高),則可以移動現有書籍以騰出空間,並將新書插入到正確的位置以保持排序順序。 4. **重複並增長已排序的子清單:**繼續此過程,從未排序的堆中獲取元素,將它們與已排序的子列表進行比較,並將它們插入到正確的已排序位置。這會逐漸增長排序的子列表,直到合併所有元素。 **時間複雜度:**插入排序適用於部分排序的資料。在一般情況下,對於已經排序的資料,它的時間複雜度為 O(n),因此非常有效率。然而,對於完全隨機的資料(最壞情況),它可以回落到 O(n^2),類似於冒泡排序和選擇排序。 **4. 歸併排序** **概念:**合併排序採用巧妙的「分而治之」策略來有效地對清單進行排序。它將問題分解為更小、更易於管理的子問題,然後按排序順序將解決方案重新組合在一起。 **解釋:** 想像一下,你有一支龐大的軍隊,需要按照身高來組織。歸併排序的工作原理如下: 1. **劃分:**將軍隊(列表)分成越來越小的組(子列表),直到每組只有一名士兵(元素)。這就像將一個大問題分解成更小、更容易解決的部分。 2. **征服:**由於現在每個子清單只有一名士兵(元素),因此它已被視為「已排序」。這是分而治之方法的基本情況。 3. **合併:**現在到了合併部分。您可以策略性地將已排序的子清單重新組合在一起,但以特定的方式。您比較每個子清單中的第一個士兵(元素),並將較短的士兵(較小的元素)放入最終的排序清單中。您不斷重複這種比較和放置,直到兩個子清單中的所有士兵(元素)都合併到最終的排序清單中。 4. **重複:**您繼續遞歸地應用這種分而治之的策略,直到整個原始軍隊(列表)從最短到最高(最小到最大)排序。 **時間複雜度:**合併排序的美妙之處在於它的時間複雜度。在平均情況和最壞情況下,其複雜度為 O(n log n)。這意味著對清單進行排序所需的時間隨著元素數量(n) 呈對數增長,這比冒泡排序、選擇排序或插入排序(其複雜度為O(n^2))要快得多。 **5. 快速排序** **概念:**快速排序是另一種分而治之的排序演算法,但採用不同的方法。它依賴一個被稱為“樞軸”的策略性選擇元素來對列表進行分區並解決排序問題。 **解釋:** 想像一下,你的書架上堆滿了雜亂的書。快速排序的工作原理如下: 1. **選擇樞軸:**您從書架中選擇一本書(樞軸)。可以透過不同的方式選擇該主元,但通常它是清單中的第一個或最後一個元素。 2. **分區:**依樞軸重新排列書架上的書。類型按字母順序排列在樞軸類型之前的書籍位於一側,類型按字母順序排列在樞軸類型之後的書籍位於另一側。樞軸本身尚未放置。這種劃分有效地將較大的排序問題劃分為兩個較小的子問題。 3. **遞歸地征服:**現在,您將兩個子清單(成堆的書)中的每一個都視為單獨的排序問題。您可以遞歸地將快速排序策略套用到這些子列表,為每個子列表選擇一個新的主元並相應地對它們進行分割。 4. **合併:**兩個子清單排序後,將原始樞軸元素放置在兩個子清單之間正確的排序位置。現在,整個書架(清單)按字母順序排序。 **時間複雜度:**平均而言,快速排序的時間複雜度為 O(n log n),這使得它對大型資料集非常有效率。然而,其性能可能會根據所選樞軸元件的不同而有所不同。選擇不當的主元(例如,總是排序或部分排序清單中的第一個或最後一個元素)可能會導致O(n^2) 的最壞情況,類似於冒泡排序、選擇排序和插入排序。 --- 3.樹的遍歷演算法: ---------- ### 什麼是樹遍歷? 樹遍歷是指探索樹資料結構的系統方法。這就像有一個路線圖可以精確地存取鄰裡(樹)中的每個房屋(節點)一次,確保您不會迷路或重新存取同一棟房屋。與可以直接按位置存取元素的簡單資料結構不同,樹需要特定的演算法來導航節點之間的連接。這些遍歷演算法定義了存取每個節點的順序,可讓您對它們包含的資料執行操作,例如搜尋特定值、新增節點或刪除現有節點。遍歷技術有多種,每種技術都有其優點和應用,這使得樹遍歷成為電腦科學中的基本概念。 ### 介紹 樹遍歷,也稱為樹搜尋,是在僅包含樹邊的圖上執行的演算法,該演算法僅存取每個節點一次。此類別中的演算法僅在存取每個節點的順序上有所不同。遍歷樹的兩種經典方法是廣度優先搜尋(bfs),即在進入下一層之前存取同一級別或距根部距離的節點;深度優先搜尋,其中分支中的所有節點或從根到葉的一組路徑在傳遞到下一個分支之前都會被存取。還有其他方法,它們使用啟發式或隨機採樣在樹中移動來加速該過程。 #### 概括: - **目的:**系統探索樹資料結構,確保每個節點僅被存取一次。 - **優點:**支援搜尋特定資料、插入新節點或刪除現有節點等操作。 - **主要區別:**演算法分為兩種主要方法: ``` * **Breadth-First Search (BFS):** Visits nodes level by level, starting from the root and progressing outward. ``` ``` * **Depth-First Search (DFS):** Explores one branch (path) as far as possible before backtracking and exploring another branch. Further variations of DFS exist for specific applications. ``` - **其他技術:**其他方法利用啟發式或隨機取樣來加快遍歷速度。 ### 術語: **1. 樹:**一種分層資料結構,模擬顛倒的樹,其節點(資料點)透過邊(連結)連接。節點可以有零個或多個子節點,形成分支,並最終導致底部的葉節點(沒有子節點的節點)。 **2. 節點:**樹的基本建構塊,包含資料和對其子節點的潛在引用。想像一下附近的一棟房子——它保存資訊(資料)並透過道路(邊緣)連接到其他房子(子節點)。 **3.根節點:**樹中最頂層的節點,作為遍歷演算法的起點。將其視為附近的主屋,從這裡開始探索。 **4.葉子節點:**沒有子節點的節點,代表樹中分支的「末端」。想像位於社區邊緣的房屋,沒有進一步的聯繫。 **5.邊:**樹中兩個節點之間的連接,描述它們之間的關係。想想連接附近房屋的道路。 **6. 遍歷:**僅存取樹中每個節點一次的系統過程。這就像探索整個社區,確保您參觀每棟房屋而不會錯過任何一棟或重新參觀同一棟房屋。 **7.廣度優先搜尋(BFS):**一種從根開始向外逐階存取節點的遍歷方法。想像一下,透過造訪第一條街道(層)上的所有房屋,然後再前往下一條街道(層)上的房屋來探索社區。 **8.深度優先搜尋(DFS):**一種遍歷方法,在回溯和探索另一個分支之前,先沿著一個分支(路徑)探索盡可能遠的地方。想像一下,沿著一條路(分支)探索附近區域,直到到達死胡同(葉節點),然後回溯並嘗試另一條路。 DFS 的常見變體包括前序、中序和後序,每種形式都有存取分支內節點的特定順序。 ### 樹遍歷演算法的特點: **1. 只存取每個節點一次:** - 樹遍歷的核心原則是保證樹中的每個節點都被恰好存取一次。這可以防止冗餘處理並確保對樹結構的完整探索。 **2. 探訪順序:** - 雖然每個節點僅被存取一次,但樹遍歷演算法的定義特徵在於它們存取節點的順序。不同的演算法會優先考慮以特定順序探索節點,從而產生不同的遍歷模式。 **3. 遞歸與迭代實作:** - 樹遍歷演算法可以遞歸或迭代地實現。遞歸方法涉及定義在子樹上呼叫自身的函數,模仿樹的層次結構性質。迭代方法利用迴圈和堆疊來管理遍歷過程。 **4.時間與空間複雜度:** - 與任何演算法一樣,樹遍歷方法具有相關的時間和空間複雜度。時間複雜度是指基於樹中節點數 (n) 執行演算法所需的時間量。常見的複雜度包括 O(n)(線性)和 O(n log n)(對數),BFS 和 DFS 變體根據實現的不同具有不同的複雜度。空間複雜度反映了演算法執行所需的額外記憶體量,通常取決於用於遍歷的資料結構(例如堆疊)。 **5.特定於應用的選擇:** - 樹遍歷演算法的選擇在很大程度上取決於手頭上的具體任務。例如,BFS 可能更適合尋找樹中兩個節點之間的最短路徑,而具有變體的 DFS 可用於搜尋特定資料或探索所有可能的路徑。 **6. 不可修改:** - 一般來說,樹遍歷演算法旨在探索現有的樹結構,而不修改樹本身。它們存取節點,對它們包含的資料執行操作,但通常不會更改樹中的連接或資料。 ### 樹遍歷演算法的應用: **1. 檔案系統導航:** - 作業系統使用樹遍歷演算法來導航電腦上的目錄結構。將您的檔案系統想像成一棵樹,其中資料夾作為節點,子資料夾和檔案作為子節點。廣度優先搜尋 (BFS) 可用於列出目錄及其子目錄中的所有文件,而深度優先搜尋 (DFS) 可用於在層次結構中定位特定文件。 **2. 網頁抓取:** - 像 Google 這樣的搜尋引擎利用 BFS 或 DFS 變體來抓取網路。他們從種子 URL(根節點)開始,有系統地探索連結的網頁(子節點)。 BFS 確保在進入更深層次之前探索特定層級(網站)的所有頁面,而 DFS 可能會在回溯和探索其他網站之前更深入地研究特定網站。 **3.人工智慧(AI):** - 人工智慧中的遊戲演算法通常使用樹遍歷來探索可能的動作及其結果。將西洋棋遊戲想像成一棵樹,當前棋盤狀態作為根節點,潛在的移動作為通往新棋盤狀態(子節點)的分支。具有修剪技術的深度優先搜尋可用於評估潛在的移動並確定最有希望的策略。 **4.社會網絡分析:** - 社群媒體平台利用樹遍歷來推薦聯繫或探索朋友網路。將您的個人資料想像為一個節點,將朋友作為子節點。遍歷演算法可用於建議基於共同朋友(樹中的共同祖先)的連接或探索網路以了解資訊流或影響力。 **5.電腦圖形學:** - 光線追蹤是一種用於在 3D 圖形中實現逼真光照效果的技術,通常採用樹遍歷演算法。虛擬場景可以表示為一棵樹,其中物件作為節點,它們的空間關係作為邊緣。遍歷有助於確定光線與哪些物件交互,從而建立逼真的陰影和反射。 **6. 網路路由:** - 電腦網路中的路由協定使用樹遍歷的變體來找到資料包到達目的地的最佳路徑。將網路想像成一棵樹,路由器作為節點,連接作為邊緣。遍歷演算法有助於確定資料在網路中不同點之間傳輸的最有效路徑。 ### 簡單解釋: 想像一下,您是一名送貨員,您有一堆包裹要在附近送貨。附近的房屋透過道路連接起來,形成樹狀結構。 - **房屋是節點:**每個房屋代表樹中的一個節點,包含地址(資料)等資訊以及可能由道路(邊)連接的相鄰房屋(子節點)的地址。 - **您的遞送路線就是遍歷:**樹遍歷演算法定義您存取每個房屋(節點)以遞送包裹(對資料執行操作)的順序。 **您可以透過兩種主要方式進行交付,這對應於兩種常見的樹遍歷方法:** **1. 廣度優先搜尋(BFS):像一個不斷擴大的圓圈一樣提供:** - 您從清單中的第一個房子(根節點)開始。 - 您將包裹運送到該房屋,然後**存取同一條街道(層)上與其直接相連的所有房屋(鄰居/子節點),**然後繼續前進。 - 一旦您運送到第一條街道(層)上的所有房屋,您就會移動到下一條街道(層)並重複該過程,在前往下一層之前存取該層上的所有房屋。 這就像一個不斷擴大的圓圈——您從中心(根部)開始,逐漸向外工作,確保在移動到下一個之前,您可以運送到街道(層)上的所有房屋。如果您想先優先考慮向附近區域的所有房屋送貨,這種方法很有用,也許是因為它們都在同一個街區,並且最大限度地減少行程時間很重要。 **2. 深度優先搜尋(DFS):深入研究一條街道:** - 您從清單中的第一棟房子(根節點)開始。 - 您將包裹運送到該房屋,然後**選擇一條從該房屋引出的相連道路(分支),並沿著它一直走到終點(葉節點)** ,在原路返回之前運送到該路徑(分支)上的所有房屋。 - 一旦到達該路的盡頭(分支),您就回溯到最後一個路口(父節點)並選擇另一條路(分支)進行探索,將物品運送到該新路徑上的所有房屋,直到到達另一個死胡同(葉節點) 。 這就像探索迷宮一樣——你選擇一條路徑(分支)並沿著它一路走下去,運送到沿途的房屋,直到你到達死胡同(葉節點)。然後你原路返回並嘗試另一條路徑(分支),直到你到達所有房屋。如果您正在快速查找特定地址並希望在前往另一條街道(分支)之前探索整條街道(分支),則此方法可能會很有用。 ### 演算法類型: **1.廣度優先搜尋(BFS):** - **概念:** BFS逐級存取節點,從根節點開始,逐層向外進行。想像一下探索一個家譜; BFS 將在向下移動到其子節點(下一層)之前存取所有兄弟節點(同一層級的節點)。 - **怎麼運作的:** ``` 1. Start at the root node and add it to a queue (a data structure that follows a "first-in, first-out" principle). ``` ``` 2. Remove the first node from the queue and visit it (process its data). ``` ``` 3. Add all the unvisited child nodes of the removed node to the back of the queue. ``` ``` 4. Repeat steps 2 and 3 until the queue is empty. ``` - **例子:** 考慮一棵簡單的樹: ``` A / \ B C / \ / \ D E F G ``` BFS 遍歷將依下列順序存取節點:A、B、C、D、E、F、G。 **2.深度優先搜尋(DFS):** - **概念:** DFS 沿著一個分支(路徑)探索盡可能遠的距離,然後回溯並探索另一個分支。 DFS 還有更多變體,但這裡我們將重點放在基本方法上。 - **怎麼運作的:** ``` 1. Start at the root node. ``` ``` 2. Visit the node (process its data). ``` ``` 3. If there are any unvisited child nodes, choose one and repeat steps 2 and 3, essentially following that branch (path) until you reach a leaf node (a node with no children). ``` ``` 4. Once you reach a leaf node, backtrack to the parent node and repeat step 3, exploring another unvisited child node (if any) of the parent. ``` ``` 5. Continue backtracking and exploring until all nodes have been visited. ``` - **例子:** 使用與之前相同的樹: ``` A / \ B C / \ / \ D E F G ``` DFS 遍歷可以以各種順序存取節點,具體取決於每一步選擇的子節點。可能的順序是:A、B、D、E、C、F、G。 **主要區別:** - BFS強調逐級存取節點,確保在深入之前先進行更廣泛的探索。 - DFS 優先考慮完全探索一個分支(路徑),然後再轉向另一個分支(路徑),可能會更快到達特定節點,但不能保證逐級存取。 結論 : ---- 總而言之,這個基本演算法之旅為您理解基本的搜尋、排序和樹遍歷技術奠定了堅實的基礎。這些解釋使用了清晰的語言和相關的類比,使這些抽象概念更加平易近人、直觀。無論您是經驗豐富的程式設計師還是剛開始涉足電腦科學,這種理解都為建立高效且有效的程式奠定了基石。 隨著您對知識的渴望與日俱增,請更深入研究!我的儲存庫充滿了各種演算法和資料結構,等待您的探索([演算法-資料-結構](https://github.com/m-mdy-m/algorithms-data-structures))。這是一個寶庫,您可以在這裡進行實驗、練習並鞏固您對這些基本建置模組的掌握。 **雖然某些部分仍在建設中,**反映了我自己正在進行的學習旅程(這個旅程可能需要 2-3 年才能完成!),但儲存庫正在不斷發展。 冒險不止於探索!我非常重視您的反饋。在文章中遇到障礙?有建設性的批評要分享嗎?還是只是想引發一場關於演算法的對話?我的門(或者更確切地說,我的收件匣)總是開著的。在 Twitter 上聯絡: [@m\_\_mdy\_\_m](https://twitter.com/m__mdy__m)或 Telegram:@m\_mdy\_m。此外,我的 GitHub 帳戶[m-mdy-m](https://github.com/m-mdy-m)歡迎討論和貢獻。讓我們共同建立一個充滿活力的學習社區,在這裡我們分享知識並突破我們的理解界限。 --- 原文出處:https://dev.to/m__mdy__m/basic-algorithms-5bep
### 介紹 每個開發人員都知道編寫測試很重要,但是當涉及 JavaScript 測試時,[有許多框架](https://en.wikipedia.org/wiki/List_of_unit_testing_frameworks#JavaScript)可供選擇。那麼,下次啟動專案時,如何知道該選擇哪個框架呢? 在本文中,我將比較三個流行的框架[——Mocha](https://github.com/mochajs/mocha) 、 [Jest](https://jestjs.io/)和[Jasmine——](https://jasmine.github.io/)以幫助您做出更明智的決定。我將了解這些框架如何處理常見的測試場景,例如模擬函數和非同步呼叫。我將展示如何實施這些測試的範例。我還將討論一些最佳實踐以及為什麼應該使用測試框架。 ### 三個框架 Mocha、Jest 和 Jasmine 都是流行的框架,擁有有用的社群和多年的開發經驗。總體而言,Mocha 和 Jasmine 更適合測試後端,因為它們最初是為 Node 應用程式建立的;因此,他們比 Jest 擁有更多可用的後端工具和文件。對於前端來說,你的測試框架的選擇通常會受到你的前端框架的影響。 Jasmine 更常與 Angular 一起使用,而 Jest 是 Facebook 建立的與 React 一起使用。 無論您選擇哪一個框架,這三個框架都是成熟且有效的選擇。最好的選擇實際上取決於您的專案需求和您的個人喜好。為了幫助您決定哪個框架最適合您,讓我們看看每個框架在一些常見測試場景下的實際應用。 ### 模擬函數 您在應用程式中測試的最常見的事情是函數呼叫。為您的函數編寫可靠的測試非常重要,因為無論測試框架如何,糟糕的測試都可能觸發真實的函數,從而導致記憶體洩漏和瀏覽器中的意外行為。 測試函數呼叫時,您的測試應該: - 專注於函數呼叫的預期結果,而不是函數的實現 - 永遠不要更改應用程式的狀態 - 使用模擬函數,這樣您就不必擔心測試中出現意外的副作用 以下是如何在 Jest、Jasmine 和 Mocha 中模擬前端函數呼叫的範例。 **是** 如果您使用 React,Jest 不需要太多依賴項(如果有的話)。但是,如果您不想深入[研究react-testing-library](https://testing-library.com/docs/react-testing-library/intro) ,Jest也與一些特定於測試的函式庫(例如Enzyme)相容。此範例使用[Enzyme](https://enzymejs.github.io/enzyme/)對元件進行淺層渲染,按一下按鈕,然後查看模式是否已開啟。在這裡,您必須渲染元件並模擬單擊,以查看您的模擬函數呼叫是否按預期開啟模式。 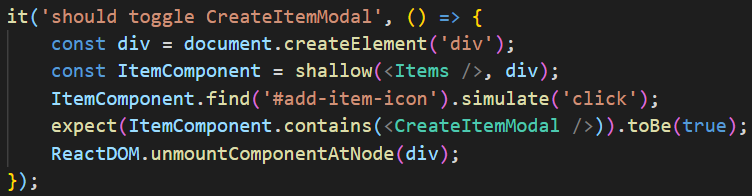 *Jest 中的模擬函數呼叫* **茉莉花** 在所有框架中,Jasmine 更適合 Angular。但是,一旦為 React 設定了所有正確的配置和幫助程式文件,編寫測試就不需要太多程式碼。 在這裡您可以看到使用[ReactTestUtils](https://reactjs.org/docs/test-utils.html)代替 Enzyme 或 React-testing-library(以顯示其他可用工具之一)。 ReactTestUtils 使得在前端使用 Jasmine 變得更加容易,並保持較低的程式碼行數。但是,您需要了解 ReactTestUtils API。  *Jasmine 中的模擬函數呼叫* **摩卡** Mocha 為您提供了更多的靈活性,因為它通常用於前端和後端測試。您必須匯入幾個庫,例如[Chai](https://www.chaijs.com/) ,才能使其與 React 一起使用。雖然 Chai 沒有直接連接到 React,但它是 Mocha 中最常用的斷言函式庫。安裝這些依賴項後,就類似於使用 Jest。此範例結合使用 Enzyme 進行渲染和 Chai 進行斷言。  *Mocha 中的模擬函數呼叫* #### 我的看法 對於模擬函數,這三個函式庫在程式碼行數和複雜性方面都非常相似。我建議只使用最適合您的堆疊的程式庫:Jest for React、Jasmine for Angular 和 Mocha(如果您也在後端使用 Mocha 並且希望保持一致)。 ### 模擬資料 後端測試與前端測試一樣棘手。對於處理資料尤其如此,因為您不希望測試將資料插入真實資料庫中。如果您不小心,這種危險的副作用很容易潛入您的測試套件中。這就是為什麼使用模擬資料設定測試資料庫是最佳實踐的原因。 當您使用模擬資料時,您可以: - 準確地看到錯誤發生的位置,因為您知道期望的值是什麼 - 類型檢查您的後端響應並確保響應不會洩露真實資料 - 更快發現錯誤 您會經常遇到模擬資料以在請求中發送的情況,而這三個框架都支援這種情況。以下是這三個框架如何實現模擬資料的範例。 **是** 在此 Jest 測試中需要注意的最重要的事情是如何檢查資料是否已成功傳遞到 API 或資料庫。最後有幾個 Expect() 匹配器,它們的順序很重要。在發送模擬資料後,您必須準確地告訴 Jest 您希望找到什麼。這裡使用[supertest](https://www.npmjs.com/package/supertest)函式庫來使用假資料發出模擬 post 請求。 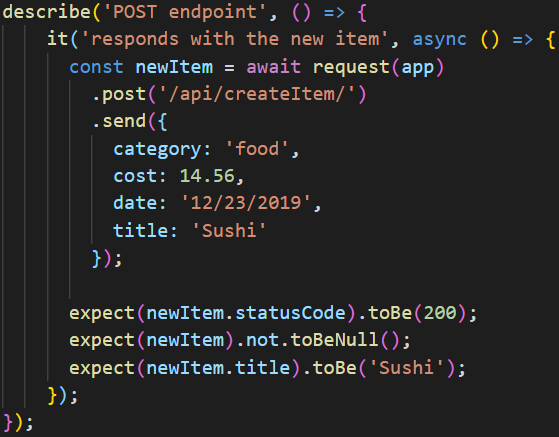 *在 Jest 後端測試中處理資料* **茉莉花** 雖然編寫良好的後端 Jasmine 測試需要更多程式碼,但您可以控制建立和重置資料的方式和時間。 Jasmine 還具有內建工具,用於在測試的其他部分引用模擬資料。此範例使用[請求](https://www.npmjs.com/package/request)庫來處理模擬發布資料請求。 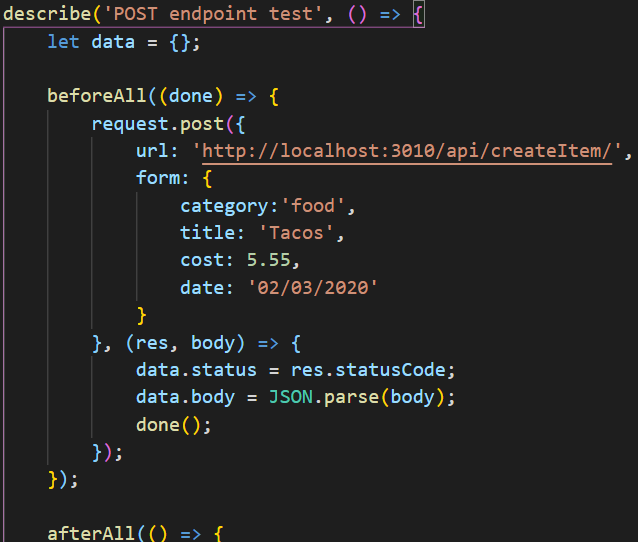 *在 Jasmine 後端測試中處理資料* **摩卡** 在所有框架中,Mocha 需要最多的依賴來處理模擬資料和請求。您可能需要使用[chai-http](https://www.app.devspotlight.com/stories/draft/Comparing-the-top-3-Javascript-testing-frameworks)設定一個模擬伺服器來執行請求,而不是像其他伺服器一樣模擬請求和回應。 Mocha 確實有很好的內建工具,但需要更多時間才能上手。使用 Chai 及其相關函式庫是常見的做法,如下例所示: 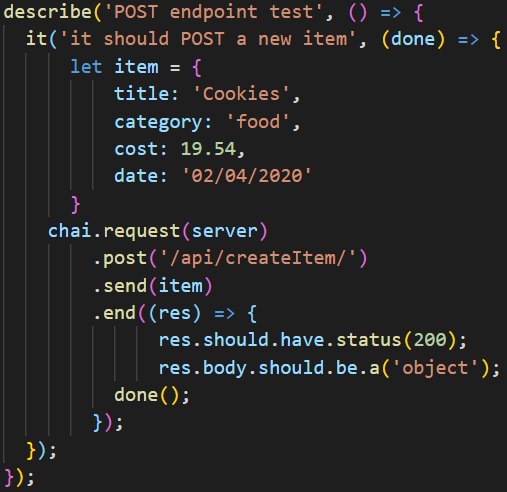 *在 Mocha 後端測試中處理資料* #### 我的看法 後端測試是 Mocha 和 Jasmine 最強的地方。它們是為測試 Node 應用程式而建構的,這一點在它們的工具中得到了體現。它們使您可以透過框架中包含的選項和功能進行更精細的控制。如果您願意花時間加入一些可用的庫,Jest 仍然是一個不錯的選擇。 ### 模擬異步呼叫 眾所周知,非同步程式碼會引起問題,因此此處的測試尤其重要。您不僅必須注意自己程式碼中的非同步為,而且許多進入生產的錯誤可能來自意外的非同步來源,例如第三方服務。當您編寫具有異步行為的測試時,請盡量避免觸發真正的函數呼叫,因為測試的非同步呼叫與實際程式碼的執行重疊。 所有測試框架都為您提供了編寫非同步程式碼的多種選項。如果您的程式碼使用回調,那麼您的測試就可以使用回呼。如果可以選擇,請考慮使用[async/await 模式](https://javascript.info/async-await)。它使您的程式碼更具可讀性,並幫助您快速找到測試的問題所在。 [Promise](https://javascript.info/promise-basics)也是編寫非同步測試的選擇。如果您正在使用不支援非同步/等待的舊程式碼,請考慮使用這些。但是,請確保它們按照您在生產中期望的順序執行。在整個執行過程中檢查您的值可以幫助捕捉奇怪的行為。 以下是 JavaScript 測試框架中的非同步測試範例。 **是** 儘管使用Jest 編寫後端測試很簡單,但由於它最初是為了與React 一起使用而建立的,因此您最終可能會花一些時間閱讀文件並安裝第三方庫,因為它的大多數工具都是特定於前端的。但 Jest 確實可以處理您需要處理的任何格式的非同步呼叫,例如回呼或 Promises。此 async/await 呼叫的工作方式與其他 JavaScript 程式碼中的工作方式相同。  *使用 async/await 開玩笑非同步呼叫* **茉莉花** Jasmine 最初是為 Node.js 建立的,因此它有很多內建功能。但是,在執行測試之前和之後可能需要一些設定。例如,您可以在此處看到,您應該在 beforeAll 方法中處理非同步呼叫,以防止稍後的測試中產生殘留影響。  *使用 async/await 進行 Jasmine 非同步呼叫* **摩卡** 在這裡您可以看到用於處理返回承諾的完成方法。它使用與前面的 Mocha 範例相同的 chai-http 庫。這是在 Mocha 測試中編寫的非同步呼叫的常見方式。您可以在 Mocha 中使用 Promises 或非同步/等待模式。 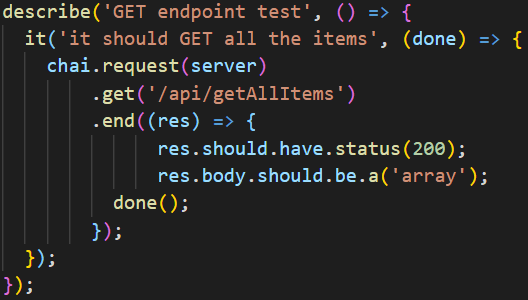 *使用 async/await 進行 Mocha 非同步呼叫* #### 我的看法 對於後端測試,Jasmine 可以輕鬆處理非同步方法並且開箱即用,這將是我的首選。 Mocha 和 Jest 也很有用,儘管它們需要更多地搜尋文件才能找到您需要的內容。 ### 模擬渲染元件 另一個重要且常見的測試是確保渲染的元件在預期時可用。和以前一樣,您通常會看到 Jest 與 React 一起使用,Jasmine 與 Angular 一起使用,但您可以在任何前端程式庫上使用這三個框架中的任何一個。 渲染元件可能是一項昂貴的任務,具體取決於渲染的深度。有些開發人員喜歡使用快照測試,它保存代表 UI 當前狀態的檔案。其他人則喜歡模擬渲染的元件。當您尋找 UI 中的變更時,快照更有用,而當您想要查看元件是否按預期工作時,渲染更有用。兩種方法都很有用。 **是** 正如我之前提到的,Jest 是為 React 建置的,因此您無需導入任何特殊的庫來進行渲染測試。這使得這些測試變得簡單並節省了依賴項的空間。 [ReactDOM](https://reactjs.org/docs/react-dom.html)在許多 React 專案中都很常見,並且附帶了檢查基本渲染所需的方法,如下例所示: 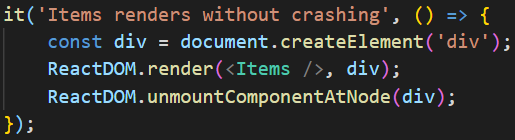 *Jest 框架中的 React 渲染測試* **茉莉花** 設定 Jasmine 來進行 React 渲染測試比看起來更困難;它涉及重要的初始設定。 [Angular 團隊](https://angular.io/guide/testing)使用並推薦[Karma](https://www.npmjs.com/package/karma-jasmine)和 Jasmine 來測試元件。下面的範例用於測試 Angular 元件。您必須匯入要測試的元件,並且可以使用 Angular 隨附的 @angular/core/testing 來設定元件的環境,然後再嘗試渲染該元件並檢查它是否可用。 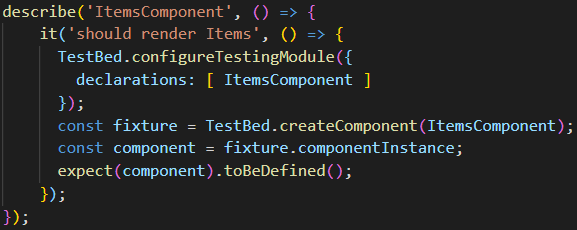 *Jasmine框架中的Angular渲染測試* **摩卡** 您通常會看到[Enzyme](https://enzymejs.github.io/enzyme/)和 Chai 與 Mocha 一起用於前端測試,並且測試 React 渲染沒有任何不同。一旦匯入了所需的特定方法(例如shallow 和expect),您將能夠編寫與其他框架類似的測試。下面的範例利用了 Enzyme 的淺層渲染和 Chai 的斷言。  *Mocha框架中的React渲染測試* #### 我的看法 渲染元件的最佳實踐是僅使用為您的前端庫推薦的測試框架。使用附帶安裝的工具,您無需處理設定錯誤。如果可能,請嘗試使用淺渲染和快照來節省測試時間並專注於渲染元件的核心功能。 ### 結論 希望您現在對這三個流行框架之間的差異有了更好的了解。正如我所提到的,無論您選擇哪種框架,這三個框架都是成熟且有效的選擇,並且可以為您工作,這取決於您的專案的需要和您的偏好。現在您已準備好接受測試! --- 原文出處:https://dev.to/heroku/comparing-the-top-3-javascript-testing-frameworks-2cco
**長話短說** -------- 在本文中,您將學習如何建立人工智慧驅動的電子表格應用程式,該應用程式允許您使用簡單的英語命令執行各種會計功能並輕鬆與資料互動。 我們將介紹如何: - 使用 Next.js 建立 Web 應用程式, - 使用 React Spreadsheet 建立電子表格應用程式,以及 - 使用 CopilotKit 將 AI 整合到軟體應用程式中。 - 讓電子表格更容易使用、更有趣 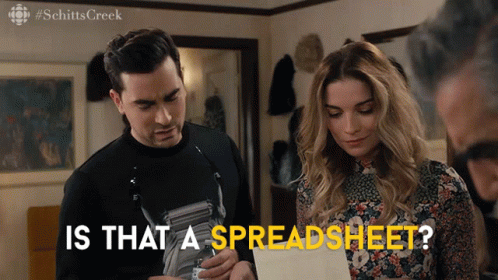 --- CopilotKit:建構應用內人工智慧副駕駛的框架 ========================== CopilotKit是一個[開源的AI副駕駛平台](https://github.com/CopilotKit/CopilotKit)。我們可以輕鬆地將強大的人工智慧整合到您的 React 應用程式中。 建造: - ChatBot:上下文感知的應用內聊天機器人,可以在應用程式內執行操作 💬 - CopilotTextArea:人工智慧驅動的文字字段,具有上下文感知自動完成和插入功能📝 - 聯合代理:應用程式內人工智慧代理,可以與您的應用程式和使用者互動🤖 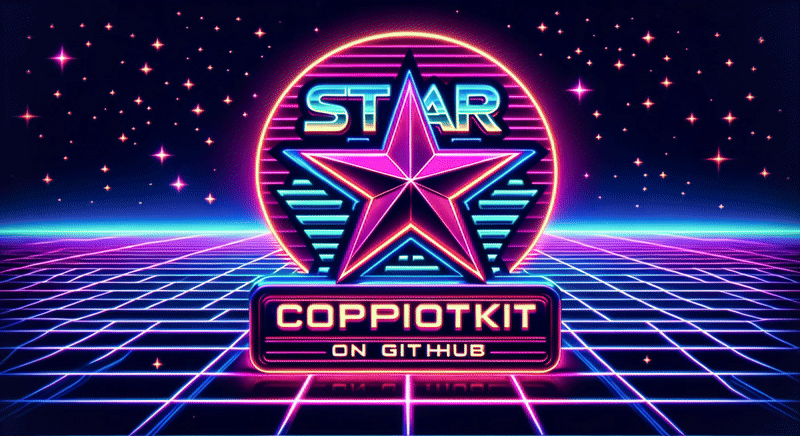 {% cta https://github.com/CopilotKit/CopilotKit %} Star CopilotKit ⭐️ {% endcta %} (請原諒 AI 的拼字錯誤並給 CopilotKit 加上星號:) 現在回到文章! --- 先決條件 ---- 要完全理解本教程,您需要對 React 或 Next.js 有基本的了解。 以下是建立人工智慧驅動的電子表格應用程式所需的工具: - [React Spreadsheet](https://github.com/iddan/react-spreadsheet) - 一個簡單的包,使我們能夠在 React 應用程式中加入電子表格。 - [OpenAI API](https://platform.openai.com/api-keys) - 提供 API 金鑰,使我們能夠使用 ChatGPT 模型執行各種任務。 - [Tavily AI](https://tavily.com/) - 一個搜尋引擎,使人工智慧代理能夠在應用程式中進行研究並存取即時知識。 - [CopilotKit](https://github.com/CopilotKit) - 一個開源副駕駛框架,用於建立自訂 AI 聊天機器人、應用程式內 AI 代理程式和文字區域。 專案設定和套件安裝 --------- 首先,透過在終端機中執行以下程式碼片段來建立 Next.js 應用程式: ``` npx create-next-app spreadsheet-app ``` 選擇您首選的配置設定。在本教學中,我們將使用 TypeScript 和 Next.js App Router。 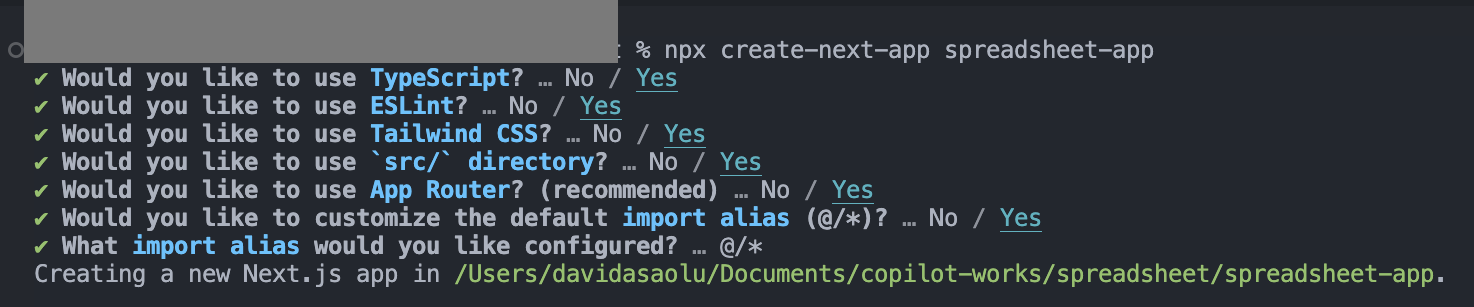 接下來,安裝[OpenAI 函式庫](https://platform.openai.com/docs/introduction)、 [Heroicons](https://www.npmjs.com/package/@heroicons/react)和[React Spreadsheet](https://github.com/iddan/react-spreadsheet)套件及其相依性: ``` npm install openai react-spreadsheet scheduler @heroicons/react ``` 最後,安裝 CopilotKit 軟體套件。這些套件使我們能夠從 React 狀態檢索資料並將 AI copilot 新增至應用程式。 ``` npm install @copilotkit/react-ui @copilotkit/react-textarea @copilotkit/react-core @copilotkit/backend ``` 恭喜!您現在已準備好建立應用程式。 --- 建立電子表格應用程式 ---------- 在本節中,我將引導您使用 React Spreadsheet 建立電子表格應用程式。 該應用程式分為兩個元件: `Sidebar`和`SingleSpreadsheet` 。 要設定這些元件,請導航至 Next.js 應用程式資料夾並建立一個包含以下檔案的`components`資料夾: ``` cd app mkdir components && cd components touch Sidebar.tsx SingleSpreadsheet.tsx ``` 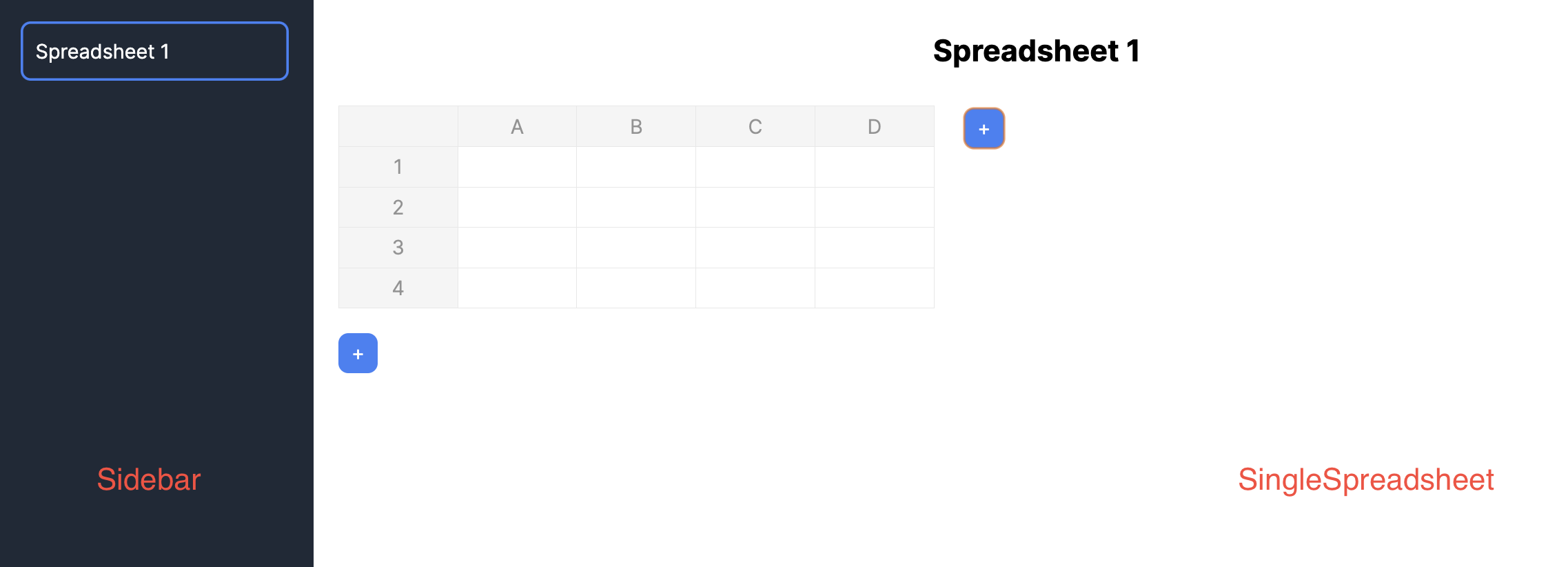 將新建立的元件匯入到**`app/page.tsx`**檔案中。 ``` "use client"; import React, { useState } from "react"; //👇🏻 import the components import { SpreadsheetData } from "./types"; import Sidebar from "./components/Sidebar"; import SingleSpreadsheet from "./components/SingleSpreadsheet"; const Main = () => { return ( <div className='flex'> <p>Hello world</p> </div> ); }; export default Main; ``` 接下來,建立將包含電子表格資料的 React 狀態,並將它們作為 props 傳遞到元件中。 ``` const Main = () => { //👇🏻 holds the title and data within a spreadsheet const [spreadsheets, setSpreadsheets] = React.useState<SpreadsheetData[]>([ { title: "Spreadsheet 1", data: [ [{ value: "" }, { value: "" }, { value: "" }], [{ value: "" }, { value: "" }, { value: "" }], [{ value: "" }, { value: "" }, { value: "" }], ], }, ]); //👇🏻 represents the index of a spreadsheet const [selectedSpreadsheetIndex, setSelectedSpreadsheetIndex] = useState(0); return ( <div className='flex'> <Sidebar spreadsheets={spreadsheets} selectedSpreadsheetIndex={selectedSpreadsheetIndex} setSelectedSpreadsheetIndex={setSelectedSpreadsheetIndex} /> <SingleSpreadsheet spreadsheet={spreadsheets[selectedSpreadsheetIndex]} setSpreadsheet={(spreadsheet) => { setSpreadsheets((prev) => { console.log("setSpreadsheet", spreadsheet); const newSpreadsheets = [...prev]; newSpreadsheets[selectedSpreadsheetIndex] = spreadsheet; return newSpreadsheets; }); }} /> </div> ); }; ``` 此程式碼片段建立了 React 狀態,用於保存電子表格資料及其索引,並將它們作為 props 傳遞到元件中。 `Sidebar`元件接受所有可用的電子表格, `SingleSpreadsheet`元件接收所有電子表格,包括更新電子表格資料的`setSpreadsheet`函數。 將下面的程式碼片段複製到`Sidebar.tsx`檔案中。它顯示應用程式中的所有電子表格,並允許使用者在它們之間進行切換。 ``` import React from "react"; import { SpreadsheetData } from "../types"; interface SidebarProps { spreadsheets: SpreadsheetData[]; selectedSpreadsheetIndex: number; setSelectedSpreadsheetIndex: (index: number) => void; } const Sidebar = ({ spreadsheets, selectedSpreadsheetIndex, setSelectedSpreadsheetIndex, }: SidebarProps) => { return ( <div className='w-64 h-screen bg-gray-800 text-white overflow-auto p-5'> <ul> {spreadsheets.map((spreadsheet, index) => ( <li key={index} className={`mb-4 cursor-pointer ${ index === selectedSpreadsheetIndex ? "ring-2 ring-blue-500 ring-inset p-3 rounded-lg" : "p-3" }`} onClick={() => setSelectedSpreadsheetIndex(index)} > {spreadsheet.title} </li> ))} </ul> </div> ); }; export default Sidebar; ``` 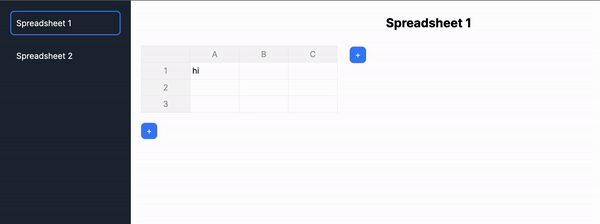 更新`SingleSpreadsheet.tsx`文件,如下所示: ``` import React from "react"; import Spreadsheet from "react-spreadsheet"; import { SpreadsheetData, SpreadsheetRow } from "../types"; interface MainAreaProps { spreadsheet: SpreadsheetData; setSpreadsheet: (spreadsheet: SpreadsheetData) => void; } //👇🏻 adds a new row to the spreadsheet const addRow = () => { const numberOfColumns = spreadsheet.rows[0].length; const newRow: SpreadsheetRow = []; for (let i = 0; i < numberOfColumns; i++) { newRow.push({ value: "" }); } setSpreadsheet({ ...spreadsheet, rows: [...spreadsheet.rows, newRow], }); }; //👇🏻 adds a new column to the spreadsheet const addColumn = () => { const spreadsheetData = [...spreadsheet.data]; for (let i = 0; i < spreadsheet.data.length; i++) { spreadsheet.data[i].push({ value: "" }); } setSpreadsheet({ ...spreadsheet, data: spreadsheetData, }); }; const SingleSpreadsheet = ({ spreadsheet, setSpreadsheet }: MainAreaProps) => { return ( <div className='flex-1 overflow-auto p-5'> {/** -- Spreadsheet title ---*/} <div className='flex items-start'> {/** -- Spreadsheet rows and columns---*/} {/** -- Add column button ---*/} </div> {/** -- Add row button ---*/} </div> ); }; export default SingleSpreadsheet; ``` - 從上面的程式碼片段來看, ``` - The `SingleSpreadsheet.tsx` file includes the addRow and addColumn functions. ``` ``` - The `addRow` function calculates the current number of rows, adds a new row, and updates the spreadsheet accordingly. ``` ``` - Similarly, the `addColumn` function adds a new column to the spreadsheet. ``` ``` - The `SingleSpreadsheet` component renders placeholders for the user interface elements. ``` 更新`SingleSpreadsheet`元件以呈現電子表格標題、其資料以及新增行和列按鈕。 ``` return ( <div className='flex-1 overflow-auto p-5'> {/** -- Spreadsheet title ---*/} <input type='text' value={spreadsheet.title} className='w-full p-2 mb-5 text-center text-2xl font-bold outline-none bg-transparent' onChange={(e) => setSpreadsheet({ ...spreadsheet, title: e.target.value }) } /> {/** -- Spreadsheet rows and columns---*/} <div className='flex items-start'> <Spreadsheet data={spreadsheet.data} onChange={(data) => { console.log("data", data); setSpreadsheet({ ...spreadsheet, data: data as any }); }} /> {/** -- Add column button ---*/} <button className='bg-blue-500 text-white rounded-lg ml-6 w-8 h-8 mt-0.5' onClick={addColumn} > + </button> </div> {/** -- Add row button ---*/} <button className='bg-blue-500 text-white rounded-lg w-8 h-8 mt-5 ' onClick={addRow} > + </button> </div> ); ``` 為了確保一切按預期工作,請在`app`資料夾中建立一個`types.ts`文件,其中包含應用程式中聲明的所有靜態類型。 ``` export interface Cell { value: string; } export type SpreadsheetRow = Cell[]; export interface SpreadsheetData { title: string; rows: SpreadsheetRow[]; } ``` 恭喜! 🎉 您的電子表格應用程式應該可以完美執行。在接下來的部分中,您將了解如何新增 AI 副駕駛,以使用 CopilotKit 自動執行各種任務。 --- 使用 CopilotKit 改進應用程式功能 ---------------------- 在這裡,您將學習如何將 AI 副駕駛加入到電子表格應用程式,以使用 CopilotKit 自動執行複雜的操作。 CopilotKit 提供前端和[後端](https://docs.copilotkit.ai/getting-started/quickstart-backend)套件。它們使您能夠插入 React 狀態並使用 AI 代理在後端處理應用程式資料。 首先,我們將 CopilotKit React 元件新增到應用程式前端。 ### 將 CopilotKit 加入前端 在`app/page.tsx`中,將以下程式碼片段加入`Main`元件的頂部。 ``` import "@copilotkit/react-ui/styles.css"; import { CopilotKit } from "@copilotkit/react-core"; import { CopilotSidebar } from "@copilotkit/react-ui"; import { INSTRUCTIONS } from "./instructions"; const HomePage = () => { return ( <CopilotKit url='/api/copilotkit'> <CopilotSidebar instructions={INSTRUCTIONS} labels={{ initial: "Welcome to the spreadsheet app! How can I help you?", }} defaultOpen={true} clickOutsideToClose={false} > <Main /> </CopilotSidebar> </CopilotKit> ); }; const Main = () => { //--- Main component // }; export default HomePage; ``` - 從上面的程式碼片段來看, ``` - I imported the CopilotKit, its sidebar component, and CSS file to use its frontend components within the application. ``` ``` - The [CopilotKit component](https://docs.copilotkit.ai/reference/CopilotKit) accepts a `url` prop that represents the API server route where CopilotKit will be configured. ``` ``` - The Copilot component also renders the [CopilotSidebar component](https://docs.copilotkit.ai/reference/CopilotSidebar) , allowing users to provide custom instructions to the AI copilot within the application. ``` ``` - Lastly, you can export the `HomePage` component containing the `CopilotSidebar` and the `Main` components. ``` 從上面的程式碼片段中,您會注意到`CopilotSidebar`元件有一個`instructions`屬性。此屬性使您能夠為 CopilotKit 提供額外的上下文或指導。 因此,在`app`資料夾中建立`instructions.ts`檔案並將這些[命令](https://github.com/CopilotKit/spreadsheet-demo/blob/main/src/app/instructions.ts)複製到該檔案中。 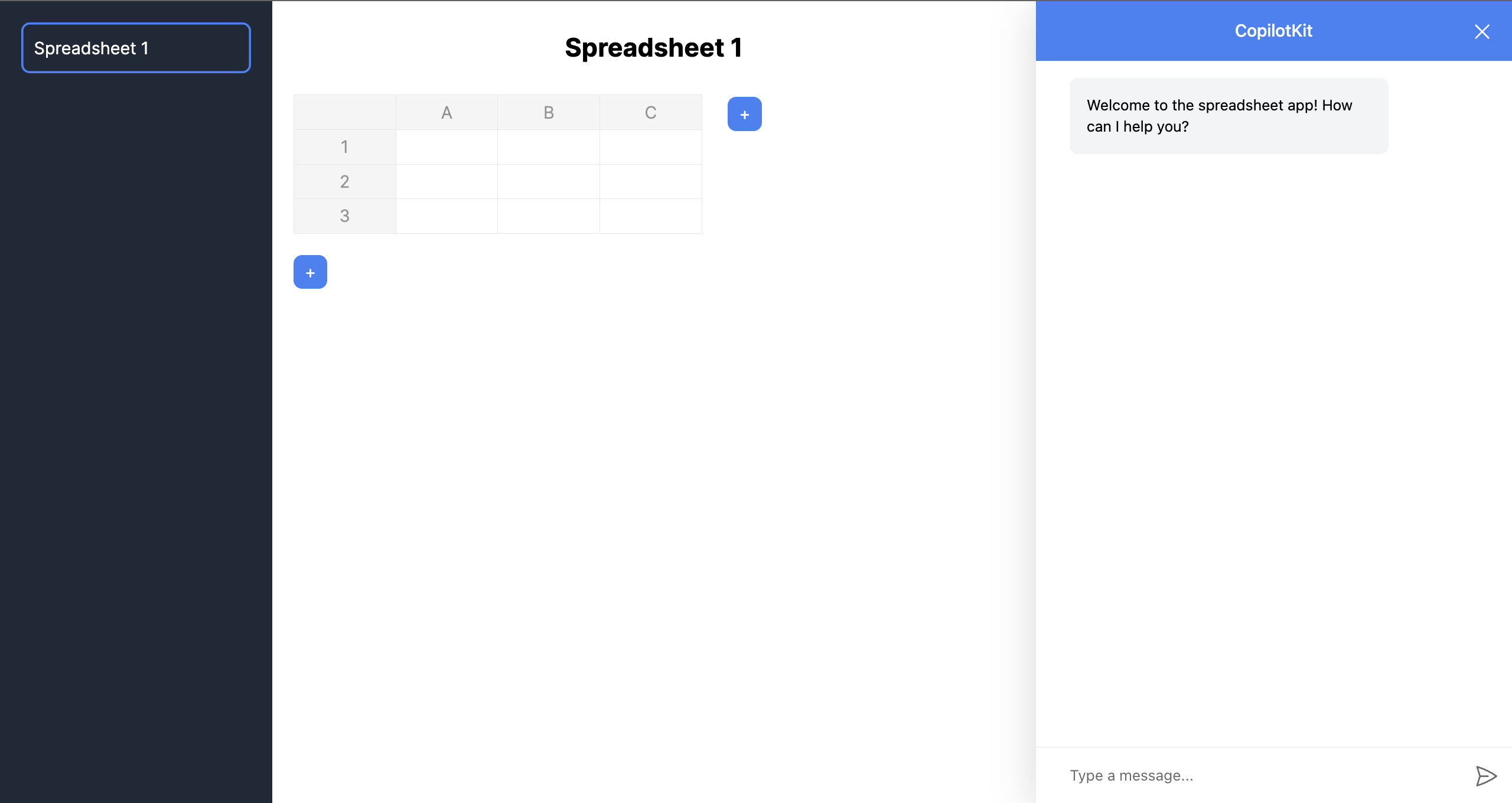 接下來,您需要將 CopilotKit 插入應用程式的狀態以存取應用程式的資料。為了實現這一點,CopilotKit 提供了兩個鉤子: [useCopilotAction](https://docs.copilotkit.ai/reference/useCopilotAction)和[useMakeCopilotReadable](https://docs.copilotkit.ai/reference/useMakeCopilotReadable) 。 [useCopilotAction](https://docs.copilotkit.ai/reference/useCopilotAction)掛鉤可讓您定義 CopilotKit 執行的動作。它接受包含以下參數的物件: - `name` - 操作的名稱。 - `description` - 操作的描述。 - `parameters` - 包含所需參數清單的陣列。 - `render` - 預設的自訂函數或字串。 - `handler` - 由操作觸發的可執行函數。 ``` useCopilotAction({ name: "sayHello", description: "Say hello to someone.", parameters: [ { name: "name", type: "string", description: "name of the person to say greet", }, ], render: "Process greeting message...", handler: async ({ name }) => { alert(`Hello, ${name}!`); }, }); ``` [useMakeCopilotReadable](https://docs.copilotkit.ai/reference/useMakeCopilotReadable)掛鉤向 CopilotKit 提供應用程式狀態。 ``` import { useMakeCopilotReadable } from "@copilotkit/react-core"; const appState = ...; useMakeCopilotReadable(JSON.stringify(appState)); ``` 現在,讓我們回到電子表格應用程式。在`SingleSpreadsheet`元件中,將應用程式狀態傳遞到 CopilotKit 中,如下所示。 ``` import { useCopilotAction, useMakeCopilotReadable, } from "@copilotkit/react-core"; const SingleSpreadsheet = ({ spreadsheet, setSpreadsheet }: MainAreaProps) => { //👇🏻 hook for providing the application state useMakeCopilotReadable( "This is the current spreadsheet: " + JSON.stringify(spreadsheet) ); // --- other lines of code }; ``` 接下來,您需要在`SingleSpreadsheet`元件中新增兩個操作,該元件在使用者更新電子表格資料並使用 CopilotKit 新增資料行時執行。 在繼續之前,請在`app`資料夾中建立一個包含`canonicalSpreadsheetData.ts`檔案的`utils`資料夾。 ``` cd app mkdir utils && cd utils touch canonicalSpreadsheetData.ts ``` 將下面的程式碼片段複製到檔案中。它接受對電子表格所做的更新,並將其轉換為電子表格中資料行所需的格式。 ``` import { SpreadsheetRow } from "../types" export interface RowLike { cells: CellLike[] | undefined; } export interface CellLike { value: string; } export function canonicalSpreadsheetData( rows: RowLike[] | undefined ): SpreadsheetRow[] { const canonicalRows: SpreadsheetRow[] = []; for (const row of rows || []) { const canonicalRow: SpreadsheetRow = []; for (const cell of row.cells || []) { canonicalRow.push({value: cell.value}); } canonicalRows.push(canonicalRow); } return canonicalRows; } ``` 現在,讓我們使用`SingleSpreadsheet`元件中的`useCopilotAction`掛鉤建立操作。複製下面的第一個操作: ``` import { canonicalSpreadsheetData } from "../utils/canonicalSpreadsheetData"; import { PreviewSpreadsheetChanges } from "./PreviewSpreadsheetChanges"; import { SpreadsheetData, SpreadsheetRow } from "../types"; import { useCopilotAction } from "@copilotkit/react-core"; useCopilotAction({ name: "suggestSpreadsheetOverride", description: "Suggest an override of the current spreadsheet", parameters: [ { name: "rows", type: "object[]", description: "The rows of the spreadsheet", attributes: [ { name: "cells", type: "object[]", description: "The cells of the row", attributes: [ { name: "value", type: "string", description: "The value of the cell", }, ], }, ], }, { name: "title", type: "string", description: "The title of the spreadsheet", required: false, }, ], render: (props) => { const { rows } = props.args const newRows = canonicalSpreadsheetData(rows); return ( <PreviewSpreadsheetChanges preCommitTitle="Replace contents" postCommitTitle="Changes committed" newRows={newRows} commit={(rows) => { const updatedSpreadsheet: SpreadsheetData = { title: spreadsheet.title, rows: rows, }; setSpreadsheet(updatedSpreadsheet); }} /> ) }, handler: ({ rows, title }) => { // Do nothing. // The preview component will optionally handle committing the changes. }, }); ``` 上面的程式碼片段執行使用者的任務並使用 CopilotKit 產生 UI 功能顯示結果預覽。 `suggestSpreadsheetOverride`操作傳回一個自訂元件 ( `PreviewSpreadsheetChanges` ),該元件接受以下內容為 props: - 要新增到電子表格的新資料行, - 一些文字 - `preCommitTitle`和`postCommitTitle` ,以及 - 更新電子表格的`commit`函數。 您很快就會學會如何使用它們。 在元件資料夾中建立`PreviewSpreadsheetChanges`元件,並將下列程式碼片段複製到檔案中: ``` import { CheckCircleIcon } from '@heroicons/react/20/solid' import { SpreadsheetRow } from '../types'; import { useState } from 'react'; import Spreadsheet from 'react-spreadsheet'; export interface PreviewSpreadsheetChanges { preCommitTitle: string; postCommitTitle: string; newRows: SpreadsheetRow[]; commit: (rows: SpreadsheetRow[]) => void; } export function PreviewSpreadsheetChanges(props: PreviewSpreadsheetChanges) { const [changesCommitted, setChangesCommitted] = useState(false); const commitChangesButton = () => { return ( <button className="inline-flex items-center gap-x-2 rounded-md bg-indigo-600 px-3.5 py-2.5 text-sm font-semibold text-white shadow-sm hover:bg-indigo-500 focus-visible:outline focus-visible:outline-2 focus-visible:outline-offset-2 focus-visible:outline-indigo-600" onClick={() => { props.commit(props.newRows); setChangesCommitted(true); }} > {props.preCommitTitle} </button> ); } const changesCommittedButtonPlaceholder = () => { return ( <button className=" inline-flex items-center gap-x-2 rounded-md bg-gray-100 px-3.5 py-2.5 text-sm font-semibold text-green-600 shadow-sm cursor-not-allowed" disabled > {props.postCommitTitle} <CheckCircleIcon className="-mr-0.5 h-5 w-5" aria-hidden="true" /> </button> ); } return ( <div className="flex flex-col"> <Spreadsheet data={props.newRows} /> <div className="mt-5"> {changesCommitted ? changesCommittedButtonPlaceholder() : commitChangesButton() } </div> </div> ); } ``` `PreviewSpreadsheetChanges`元件傳回一個電子表格,其中包含從請求產生的資料和一個按鈕(帶有`preCommitTitle`文字),該按鈕允許您將這些變更提交到主電子表格表(透過觸發`commit`函數)。這可確保您在將結果新增至電子表格之前對結果感到滿意。 將下面的第二個操作加入到`SingleSpreadsheet`元件。 ``` useCopilotAction({ name: "appendToSpreadsheet", description: "Append rows to the current spreadsheet", parameters: [ { name: "rows", type: "object[]", description: "The new rows of the spreadsheet", attributes: [ { name: "cells", type: "object[]", description: "The cells of the row", attributes: [ { name: "value", type: "string", description: "The value of the cell", }, ], }, ], }, ], render: (props) => { const status = props.status; const { rows } = props.args const newRows = canonicalSpreadsheetData(rows); return ( <div> <p>Status: {status}</p> <Spreadsheet data={newRows} /> </div> ) }, handler: ({ rows }) => { const canonicalRows = canonicalSpreadsheetData(rows); const updatedSpreadsheet: SpreadsheetData = { title: spreadsheet.title, rows: [...spreadsheet.rows, ...canonicalRows], }; setSpreadsheet(updatedSpreadsheet); }, }); ``` `appendToSpreadsheet`操作透過在電子表格中新增資料行來更新電子表格。 以下是操作的簡短示範: \[https://www.youtube.com/watch?v=kGQ9xl5mSoQ\] 最後,在`Main`元件中新增一個操作,以便在使用者提供指令時建立一個新的電子表格。 ``` useCopilotAction({ name: "createSpreadsheet", description: "Create a new spreadsheet", parameters: [ { name: "rows", type: "object[]", description: "The rows of the spreadsheet", attributes: [ { name: "cells", type: "object[]", description: "The cells of the row", attributes: [ { name: "value", type: "string", description: "The value of the cell", }, ], }, ], }, { name: "title", type: "string", description: "The title of the spreadsheet", }, ], render: (props) => { const { rows, title } = props.args; const newRows = canonicalSpreadsheetData(rows); return ( <PreviewSpreadsheetChanges preCommitTitle="Create spreadsheet" postCommitTitle="Spreadsheet created" newRows={newRows} commit={ (rows) => { const newSpreadsheet: SpreadsheetData = { title: title || "Untitled Spreadsheet", rows: rows, }; setSpreadsheets((prev) => [...prev, newSpreadsheet]); setSelectedSpreadsheetIndex(spreadsheets.length); }} /> ); }, handler: ({ rows, title }) => { // Do nothing. // The preview component will optionally handle committing the changes. }, }); ``` 恭喜!您已成功為此應用程式建立所需的操作。現在,讓我們將應用程式連接到 Copilotkit 後端。 ### 將 Tavily AI 和 OpenAI 加入到 CopilotKit 在本教程的開頭,我向您介紹了[Tavily AI](https://tavily.com/) (一個為 AI 代理提供知識的搜尋引擎)和 OpenAI(一個使我們能夠存取[GPT-4 AI 模型的](https://openai.com/gpt-4)庫)。 在本部分中,您將了解如何取得 Tavily 和 OpenAI API 金鑰並將它們整合到 CopilotKit 中以建立高級智慧應用程式。 造訪[Tavily AI 網站](https://app.tavily.com/sign-in),建立一個帳戶,然後將您的 API 金鑰複製到您專案的`.env.local`檔案中。 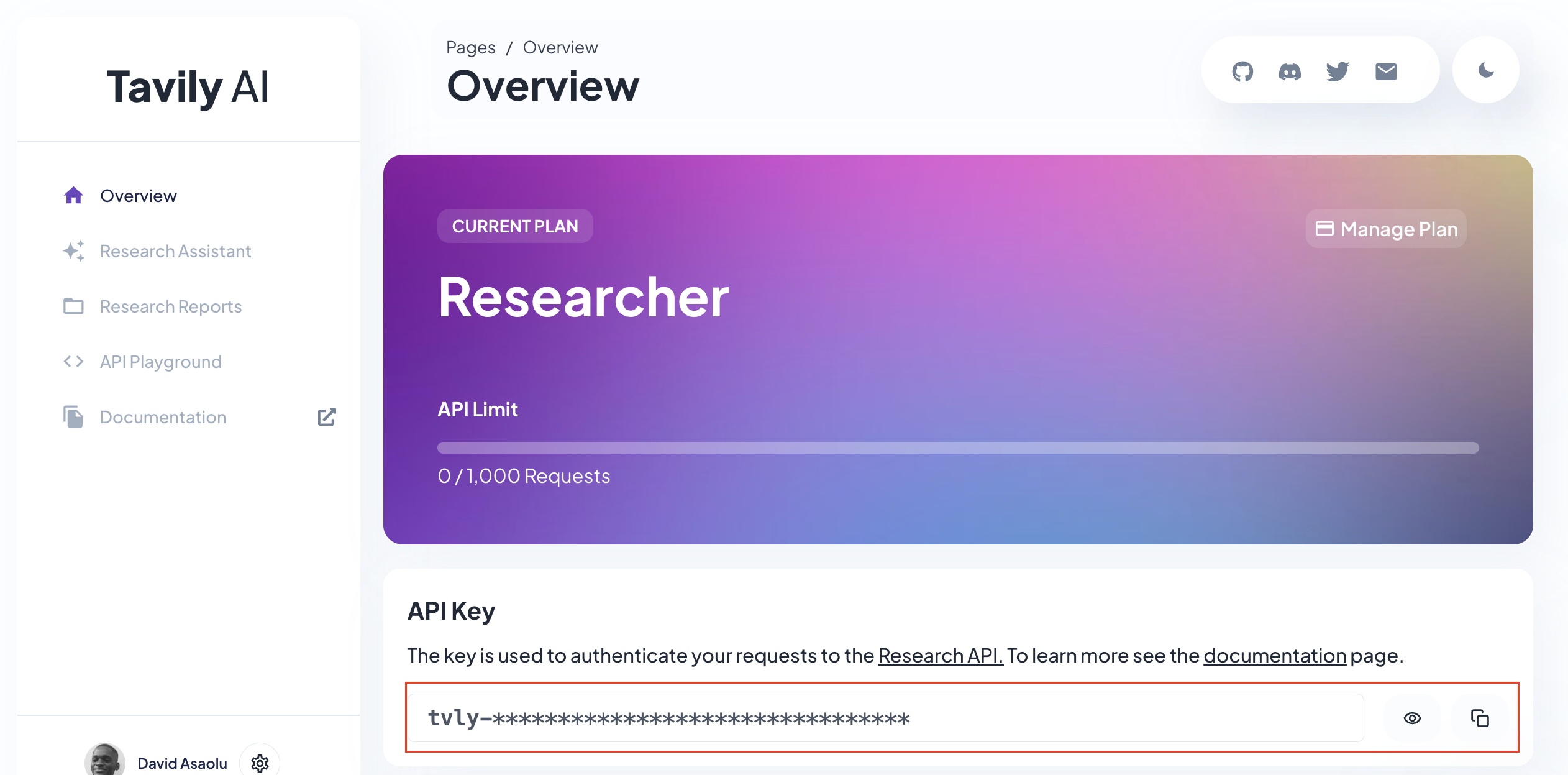 接下來,導覽至[OpenAI 開發者平台](https://platform.openai.com/api-keys),建立 API 金鑰,並將其複製到`.env.local`檔案中。 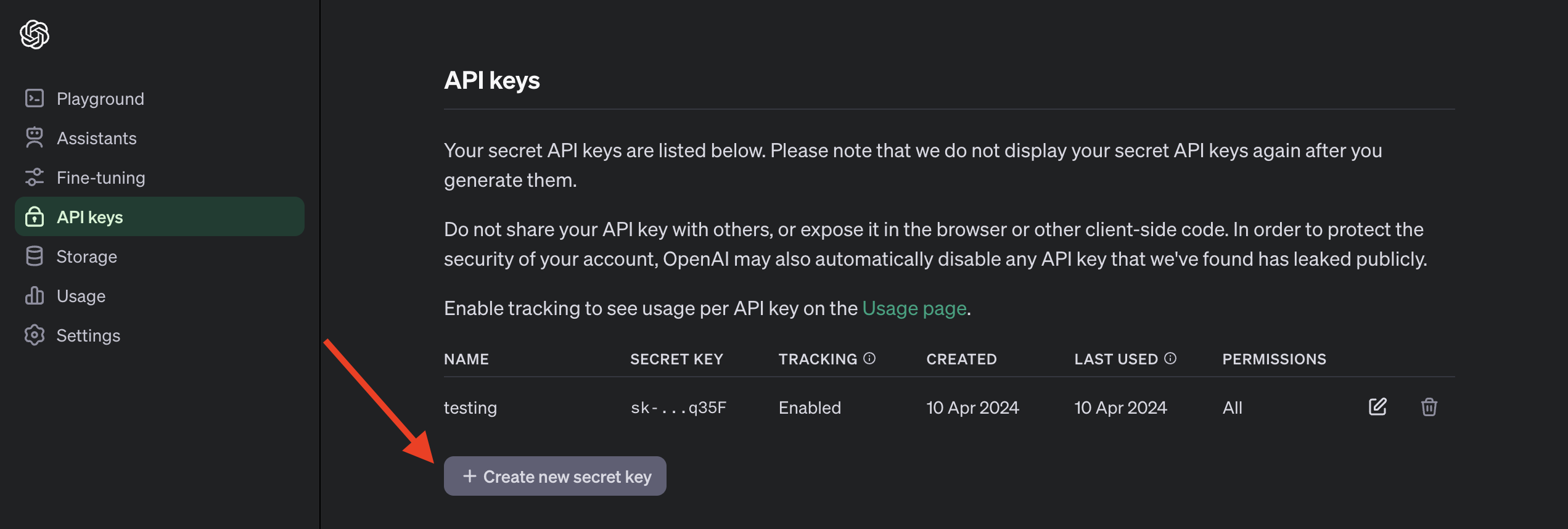 以下是`.env.local`檔案的預覽,其中包括 API 金鑰並指定要使用的 OpenAI 模型。請注意,存取 GPT-4 模型需要[訂閱 ChatGPT Plus](https://openai.com/chatgpt/pricing) 。 ``` TAVILY_API_KEY=<your_API_key> OPENAI_MODEL=gpt-4-1106-preview OPENAI_API_KEY=<your_API_key> ``` 回到我們的應用程式,您需要為 Copilot 建立 API 路由。因此,建立一個包含`route.ts`的`api/copilotkit`資料夾並新增一個`tavily.ts`檔案。 ``` cd app mkdir api && cd api mkdir copilotkit && cd copilotkit touch route.ts tavily.ts ``` 在`tavily.ts`檔案中建立一個函數,該函數接受使用者的查詢,使用 Tavily Search API 對查詢進行研究,並使用[OpenAI GPT-4 模型](https://openai.com/gpt-4)總結結果。 ``` import OpenAI from "openai"; export async function research(query: string) { //👇🏻 sends the request to the Tavily Search API const response = await fetch("https://api.tavily.com/search", { method: "POST", headers: { "Content-Type": "application/json", }, body: JSON.stringify({ api_key: process.env.TAVILY_API_KEY, query, search_depth: "basic", include_answer: true, include_images: false, include_raw_content: false, max_results: 20, }), }); //👇🏻 the response const responseJson = await response.json(); const openai = new OpenAI({ apiKey: process.env.OPENAI_API_KEY! }); //👇🏻 passes the response into the OpenAI GPT-4 model const completion = await openai.chat.completions.create({ messages: [ { role: "system", content: `Summarize the following JSON to answer the research query \`"${query}"\`: ${JSON.stringify( responseJson )} in plain English.`, }, ], model: process.env.OPENAI_MODEL, }); //👇🏻 returns the result return completion.choices[0].message.content; } ``` 最後,您可以透過將使用者的查詢傳遞到函數中並向 CopilotKit 提供其回應來執行`route.ts`檔案中的`research`函數。 ``` import { CopilotBackend, OpenAIAdapter } from "@copilotkit/backend"; import { Action } from "@copilotkit/shared"; import { research } from "./tavily"; //👇🏻 carries out a research on the user's query const researchAction: Action<any> = { name: "research", description: "Call this function to conduct research on a certain query.", parameters: [ { name: "query", type: "string", description: "The query for doing research. 5 characters or longer. Might be multiple words", }, ], handler: async ({ query }) => { console.log("Research query: ", query); const result = await research(query); console.log("Research result: ", result); return result; }, }; export async function POST(req: Request): Promise<Response> { const actions: Action<any>[] = []; if (process.env.TAVILY_API_KEY!) { actions.push(researchAction); } const copilotKit = new CopilotBackend({ actions: actions, }); const openaiModel = process.env.OPENAI_MODEL; return copilotKit.response(req, new OpenAIAdapter({ model: openaiModel })); } ``` 恭喜!您已完成本教學的專案。 結論 -- [CopilotKit](https://copilotkit.ai/)是一款令人難以置信的工具,可讓您在幾分鐘內將 AI Copilot 加入到您的產品中。無論您是對人工智慧聊天機器人和助理感興趣,還是對複雜任務的自動化感興趣,CopilotKit 都能讓您輕鬆實現。 如果您需要建立 AI 產品或將 AI 工具整合到您的軟體應用程式中,您應該考慮 CopilotKit。 您可以在 GitHub 上找到本教學的源程式碼: https://github.com/CopilotKit/spreadsheet-demo 感謝您的閱讀! --- 原文出處:https://dev.to/copilotkit/build-an-ai-powered-spreadsheet-app-nextjs-langchain-copilotkit-109d
🩺 醫生有聽診器。 🔧 機械師有扳手。 👨💻 我們開發人員,有 Git。 您是否注意到 Git 對於程式碼工作來說是如此不可或缺,以至於人們幾乎從未將其包含在他們的技術堆疊或簡歷中?假設你已經知道了,或至少足以應付,但你知道嗎? Git 是一個版本控制系統(VCS)。無所不在的技術使我們能夠儲存、更改程式碼並與他人合作。 > 🚨 作為免責聲明,我想指出 Git 是一個很大的話題。 Git 書籍已經出版,部落格文章也可能被誤認為是學術論文。這不是我來這裡的目的。**我不是 Git 專家**。我的目標是寫一篇我希望在學習 Git 時擁有的 Git 基礎文章。 作為開發人員,我們的日常工作圍繞著閱讀、編寫和審查程式碼。 Git 可以說是我們使用的最重要的工具之一。身為開發人員,掌握 Git 提供的特性和功能是您可以對自己進行的最佳投資之一。 讓我們開始吧 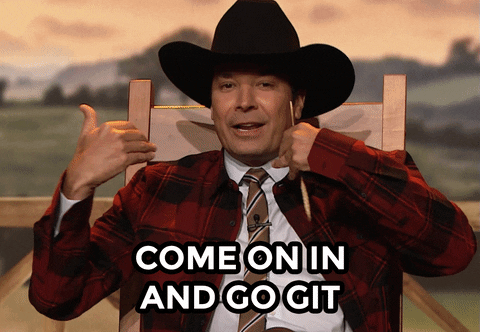 > 如果您覺得我錯過了或應該更詳細地了解特定命令,請在下面的評論中告訴我。我將相應地更新這篇文章。 🙏 --- 當我們談論這個話題時 ---------- 如果您希望將 Git 技能運用到工作中並願意為 Glasskube 做出貢獻,我們於 2 月正式推出,我們的目標是成為 Kubernetes 套件管理的預設預設解決方案。有了您的支持,我們就能實現這一目標。表達您支持的最佳方式是在 GitHub 上為我們加註星標 ⭐ [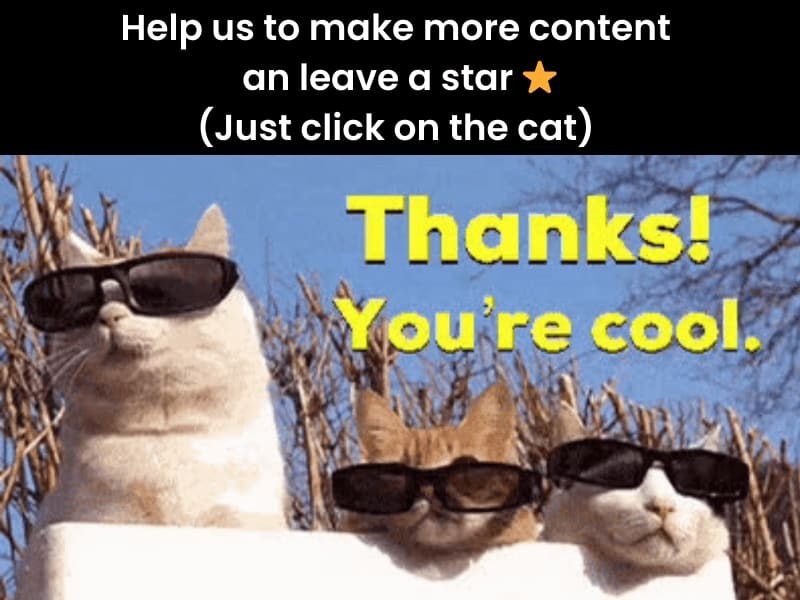](https://github.com/glasskube/glasskube) --- 讓我們打好基礎 ------- Git 有沒有讓你感覺像 Peter Griffin 一樣? 如果您沒有以正確的方式學習 Git,您可能會不斷地摸不著頭腦,陷入同樣的問題,或者後悔有一天在終端中出現另一個合併衝突。讓我們定義一些基本的 Git 概念來確保這種情況不會發生。 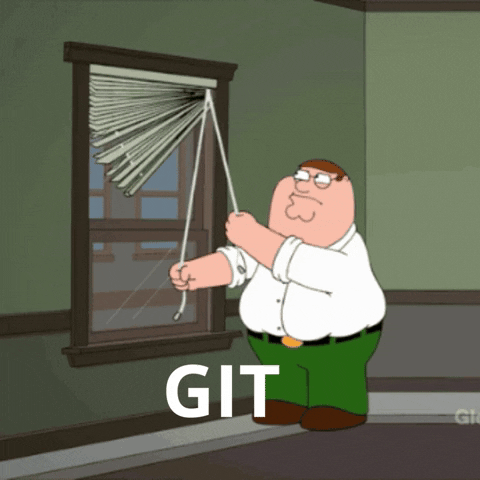 ### 分公司 在 Git 儲存庫中,您會發現一條開發主線,通常名為「main」或「master」( [已棄用](https://github.blog/changelog/2020-10-01-the-default-branch-for-newly-created-repositories-is-now-main/)),其中有幾個[分支](https://git-scm.com/book/en/v2/Git-Branching-Branches-in-a-Nutshell)分支。這些分支代表同時的工作流程,使開發人員能夠在同一專案中同時處理多個功能或修復。 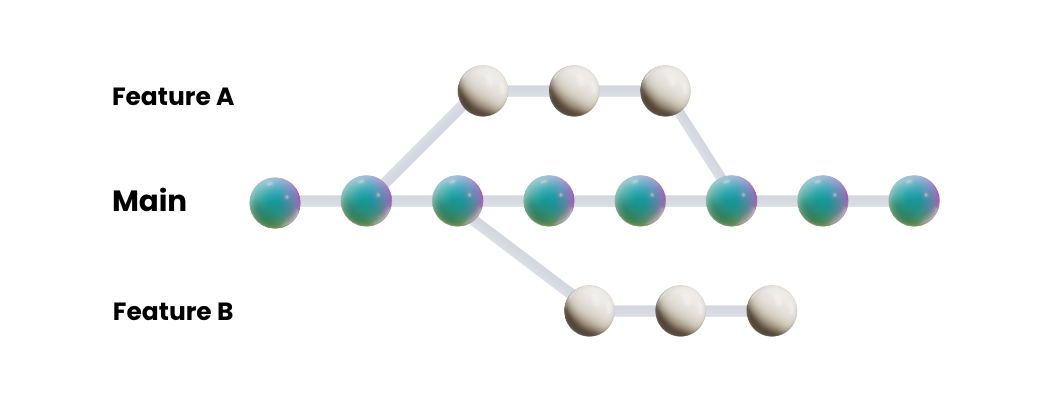 ### 提交 Git 提交作為更新程式碼的捆綁包,捕獲專案程式碼在特定時間點的快照。每次提交都會記錄自上次記錄以來所做的更改,共同建立專案開發旅程的全面歷史。 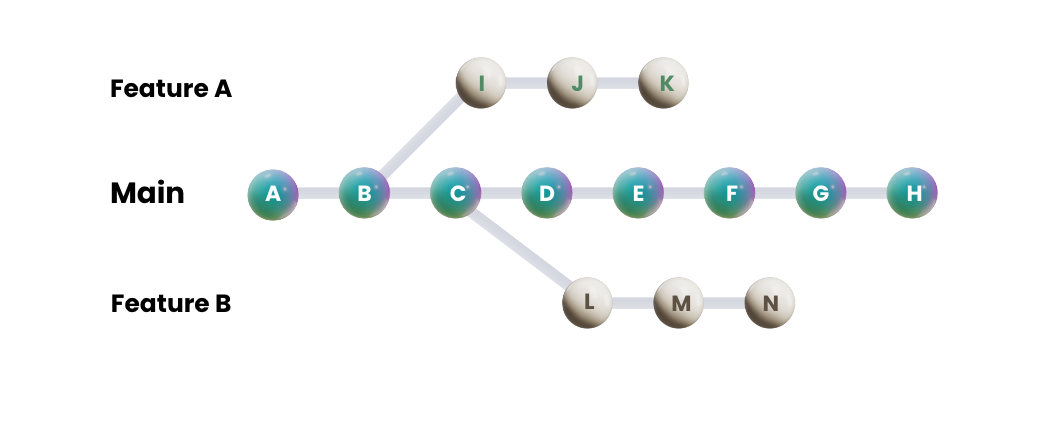 當引用提交時,您通常會使用其唯一標識的加密[雜湊](https://www.mikestreety.co.uk/blog/the-git-commit-hash/)。 例子: ``` git show abc123def456789 ``` 這顯示了有關該哈希提交的詳細資訊。 ### 標籤 Git[標籤](https://git-scm.com/book/en/v2/Git-Basics-Tagging)充當 Git 歷史中的地標,通常標記專案開發中的重要里程碑,例如`releases` 、 `versions`或`standout commits` 。這些標籤對於標記特定時間點非常有價值,通常代表專案旅程中的起點或主要成就。 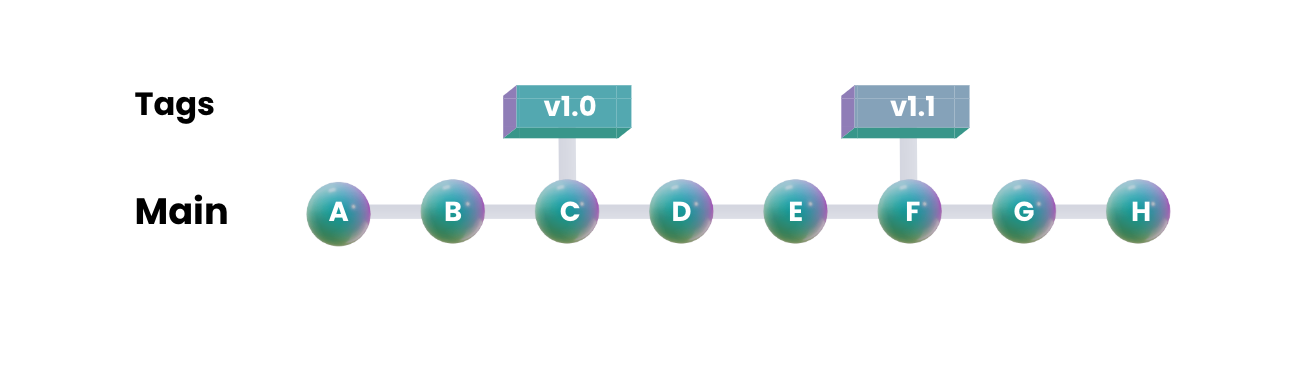 ### 頭 目前簽出分支上的最新提交由`HEAD`指示,充當儲存庫中任何引用的指標。當您位於特定分支時, `HEAD`指向該分支上的最新提交。有時, `HEAD`可以直接指向特定的提交( `detached HEAD`狀態),而不是指向分支的尖端。 ### 階段 了解 Git 階段對於導航 Git 工作流程至關重要。它們代表文件更改在提交到儲存庫之前發生的邏輯轉換。 讓我們深入研究一下 Git 階段的概念: 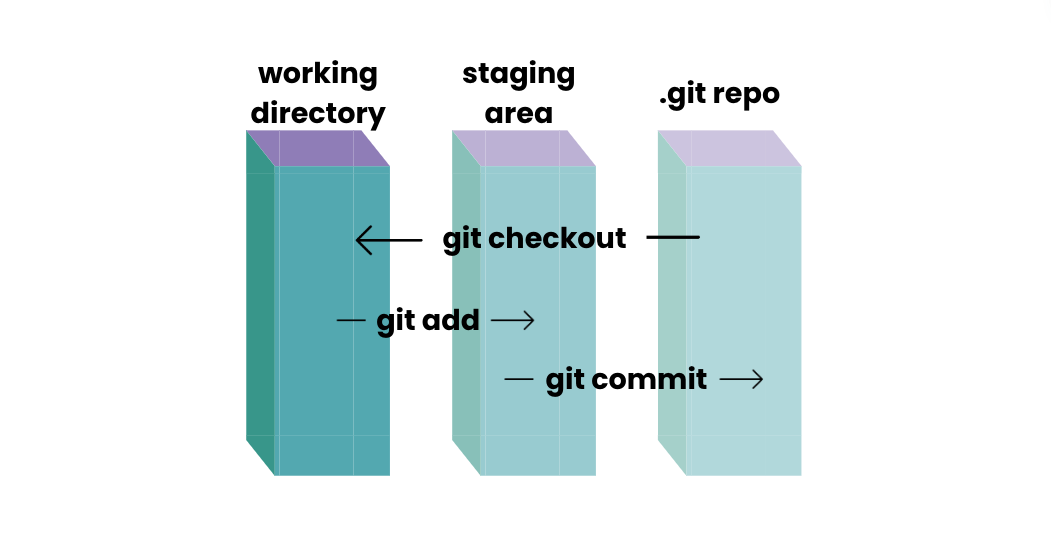 #### 工作目錄👷 `working directory`是您編輯、修改和建立專案文件的位置。表示本機上檔案的目前狀態。 #### 暫存區🚉 `staging`區域就像一個保留區域或預提交區域,您可以在其中準備更改,然後再將更改提交到儲存庫。 > 這裡有用的指令: `git add` > `git rm`也可用於取消暫存更改 #### 本地儲存庫🗄️ 本機儲存庫是 Git 永久儲存已提交變更的位置。它允許您查看專案的歷史記錄,恢復到以前的狀態,並與同一程式碼庫上的其他人進行協作。 > 您可以使用以下指令提交暫存區域中準備好的變更: `git commit` #### 遠端儲存庫🛫 遠端儲存庫是一個集中位置,通常託管在伺服器(例如 GitHub、GitLab 或 Bitbucket)上,您可以在其中與其他人共用專案並進行協作。 > 您可以使用`git push`和`git pull`等命令將提交的變更從本機儲存庫推送/拉取到遠端儲存庫。 Git 入門 ------ 好吧,你必須從某個地方開始,在 Git 中那就是你的`workspace` 。您可以`fork`或`clone`現有儲存庫並擁有該工作區的副本,或者如果您在電腦上的新本機資料夾中全新開始,則必須使用`git init`將其轉換為 git 儲存庫。不容忽視的下一步是設定您的憑證。 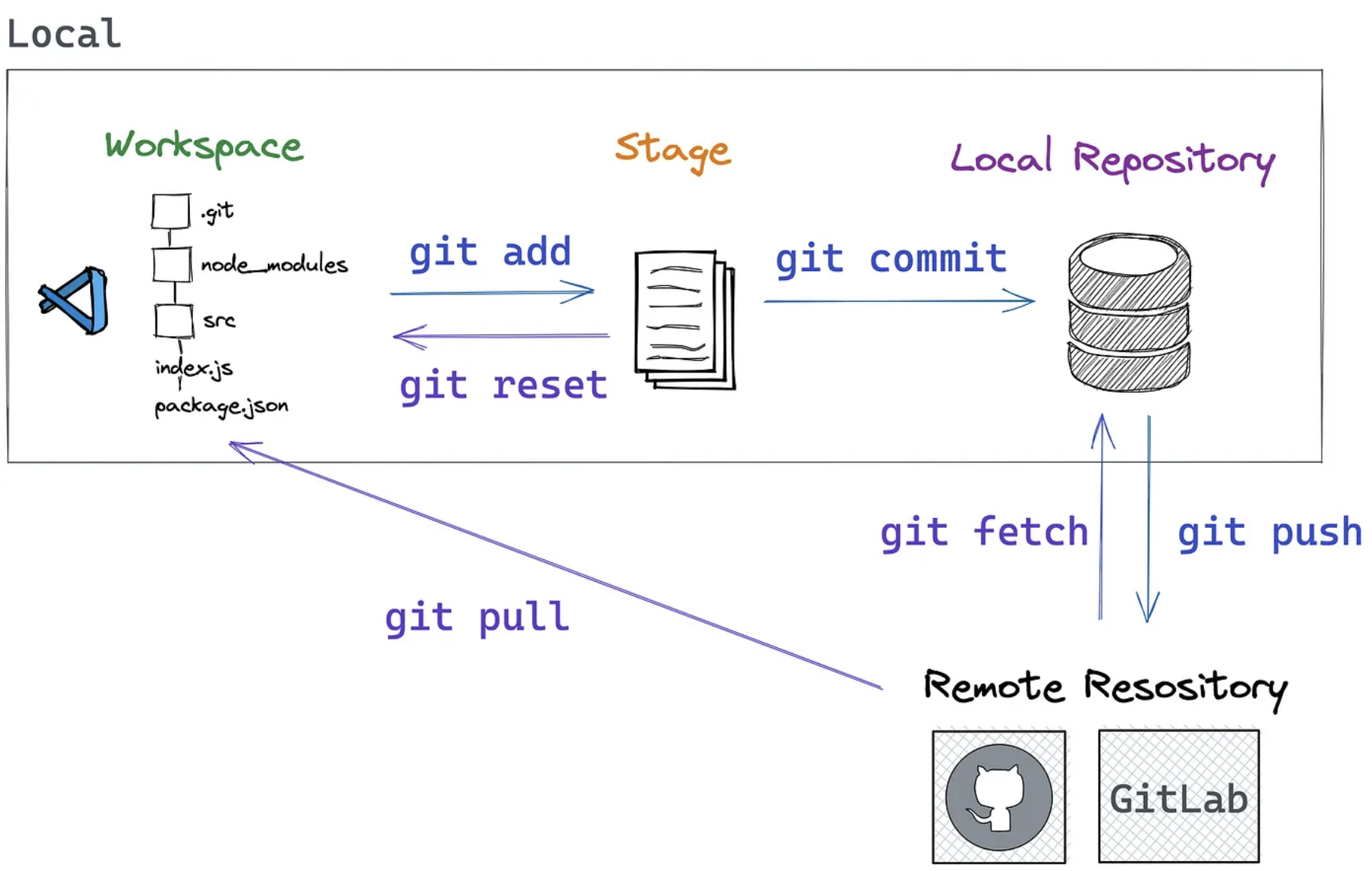 ### 憑證設定 當執行推送和拉取到遠端儲存庫時,您不想每次都輸入使用者名稱和密碼,只需執行以下命令即可避免這種情況: ``` git config --global credential.helper store ``` 第一次與遠端儲存庫互動時,Git 會提示您輸入使用者名稱和密碼。之後,您將不再收到提示 > 請務必注意,憑證以純文字格式儲存在`.git-credentials`檔案中。 若要檢查已配置的憑證,您可以使用下列命令: ``` git config --global credential.helper ``` ### 與分公司合作 在本地工作時,了解您目前所在的分支至關重要。這些命令很有幫助: ``` # Will show the changes in the local repository git branch # Or create a branch directly with git branch feature-branch-name ``` 若要在分支之間轉換,請使用: ``` git switch ``` 除了在它們之間進行轉換之外,您還可以使用: ``` git checkout # A shortcut to switch to a branch that is yet to be created with the -b flag git checkout -b feature-branch-name ``` 若要檢查儲存庫的狀態,請使用: ``` git status ``` 始終清楚了解當前分支的一個好方法是在終端機中查看它。許多終端插件可以幫助解決這個問題。這是[一個](https://gist.github.com/joseluisq/1e96c54fa4e1e5647940)。 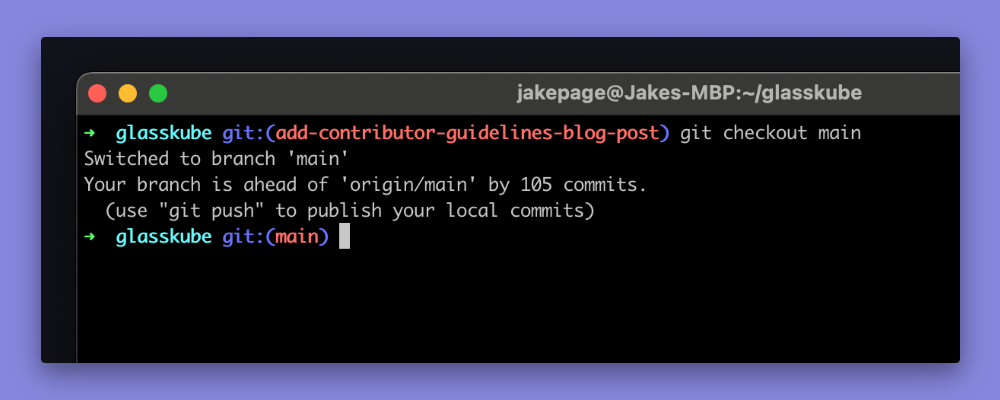 ### 使用提交 在處理提交時,使用 git commit -m 記錄更改,使用 git amend 修改最近的提交,並盡力遵守[提交訊息約定](https://gist.github.com/qoomon/5dfcdf8eec66a051ecd85625518cfd13)。 ``` # Make sure to add a message to each commit git commit -m "meaningful message" ``` 如果您對上次提交進行了更改,則不必完全建立另一個提交,您可以使用 --amend 標誌來使用分階段變更來修改最近的提交 ``` # make your changes git add . git commit --amend # This will open your default text editor to modify the commit message if needed. git push origin your_branch --force ``` > ⚠️ 使用`--force`時要小心,因為它有可能涵蓋目標分支的歷史記錄。通常應避免在 main/master 分支上應用它。 > 根據經驗,最好經常提交,以避免遺失進度或意外重置未暫存的變更。之後可以透過壓縮多個提交或進行互動式變基來重寫歷史記錄。 使用`git log`顯示按時間順序排列的提交列表,從最近的提交開始並按時間倒推 操縱歷史 ---- 操縱歷史涉及一些強大的命令。 `Rebase`重寫提交歷史記錄, `Squashing`將多個提交合併為一個,而`Cherry-picking`選擇特定提交。 ### 變基和合併 將變基與合併進行比較是有意義的,因為它們的目標相同,但實現方式不同。關鍵的差異在於變基重寫了專案的歷史。對於重視清晰且易於理解的專案歷史的專案來說,這是理想的選擇。另一方面,合併透過產生新的合併提交來維護兩個分支歷史記錄。 在變基期間,功能分支的提交歷史記錄在移動到主分支的`HEAD`時會被重組 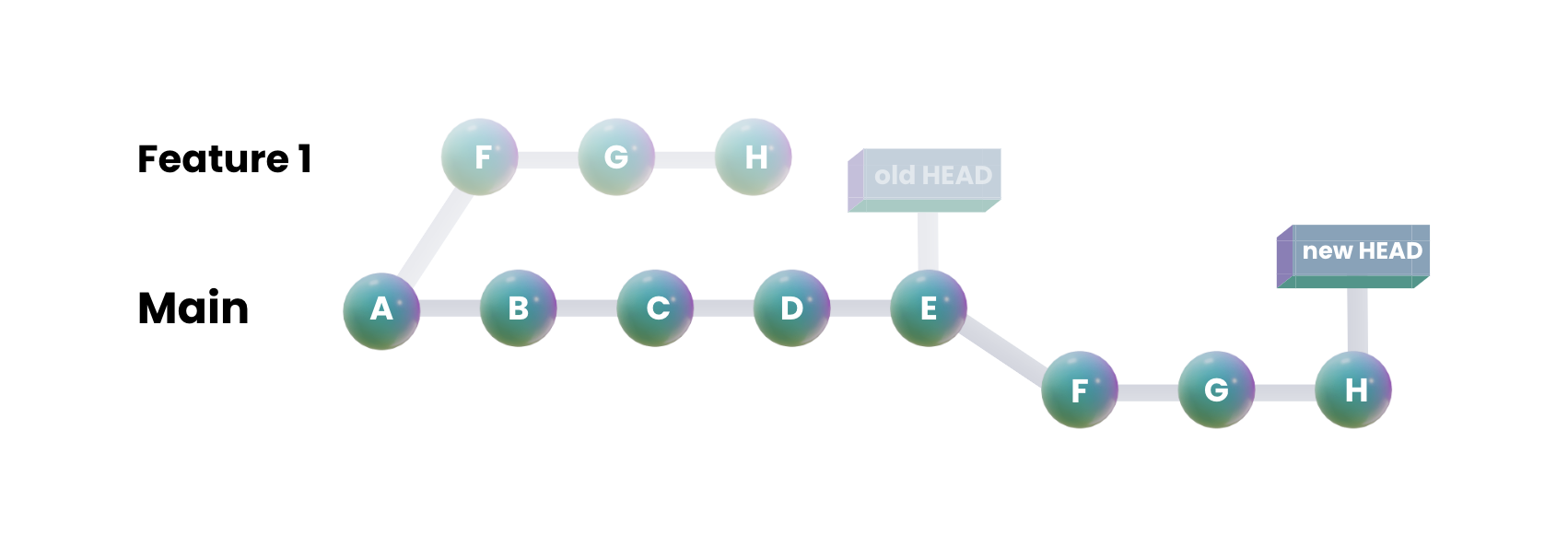 這裡的工作流程非常簡單。 確保您位於要變基的分支上並從遠端儲存庫取得最新變更: ``` git checkout your_branch git fetch ``` 現在選擇您想要變基的分支並執行以下命令: ``` git rebase upstream_branch ``` 變基後,如果分支已推送到遠端儲存庫,您可能需要強制推送變更: ``` git push origin your_branch --force ``` > ⚠️ 使用`--force`時要小心,因為它有可能涵蓋目標分支的歷史記錄。通常應避免在 main/master 分支上應用它。 ### 擠壓 Git 壓縮用於將多個提交壓縮為單一、有凝聚力的提交。 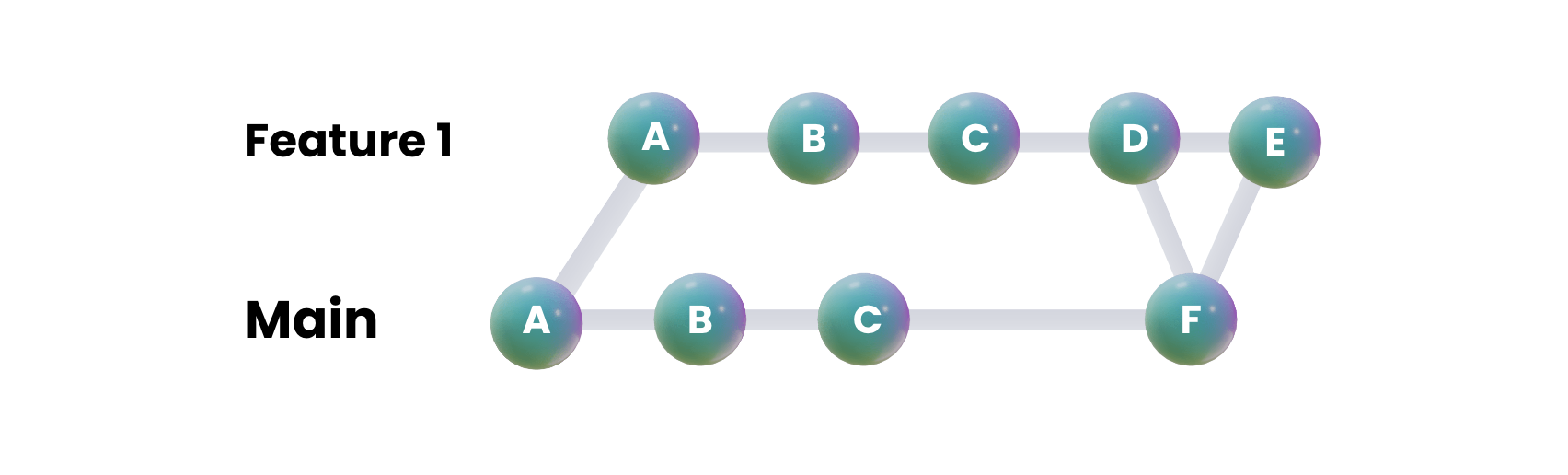 這個概念很容易理解,如果使用的統一程式碼的方法是變基,則特別有用,因為歷史記錄會被改變,所以注意對專案歷史記錄的影響很重要。有時我很難執行擠壓,特別是使用互動式變基,幸運的是我們有一些工具可以幫助我們。這是我首選的壓縮方法,其中涉及將 HEAD 指標向後移動 X 次提交,同時保留分階段的變更。 ``` # Change to the number after HEAD~ depending on the commits you want to squash git reset --soft HEAD~X git commit -m "Your squashed commit message" git push origin your_branch --force ``` > ⚠️ 使用`--force`時要小心,因為它有可能涵蓋目標分支的歷史記錄。通常應避免在 main/master 分支上應用它。 ### 採櫻桃 櫻桃採摘對於選擇性地合併從一個分支到另一個分支的更改非常有用,特別是當合併整個分支不合需要或不可行時。然而,明智地使用櫻桃選擇很重要,因為如果應用不當,可能會導致重複提交和不同的歷史記錄 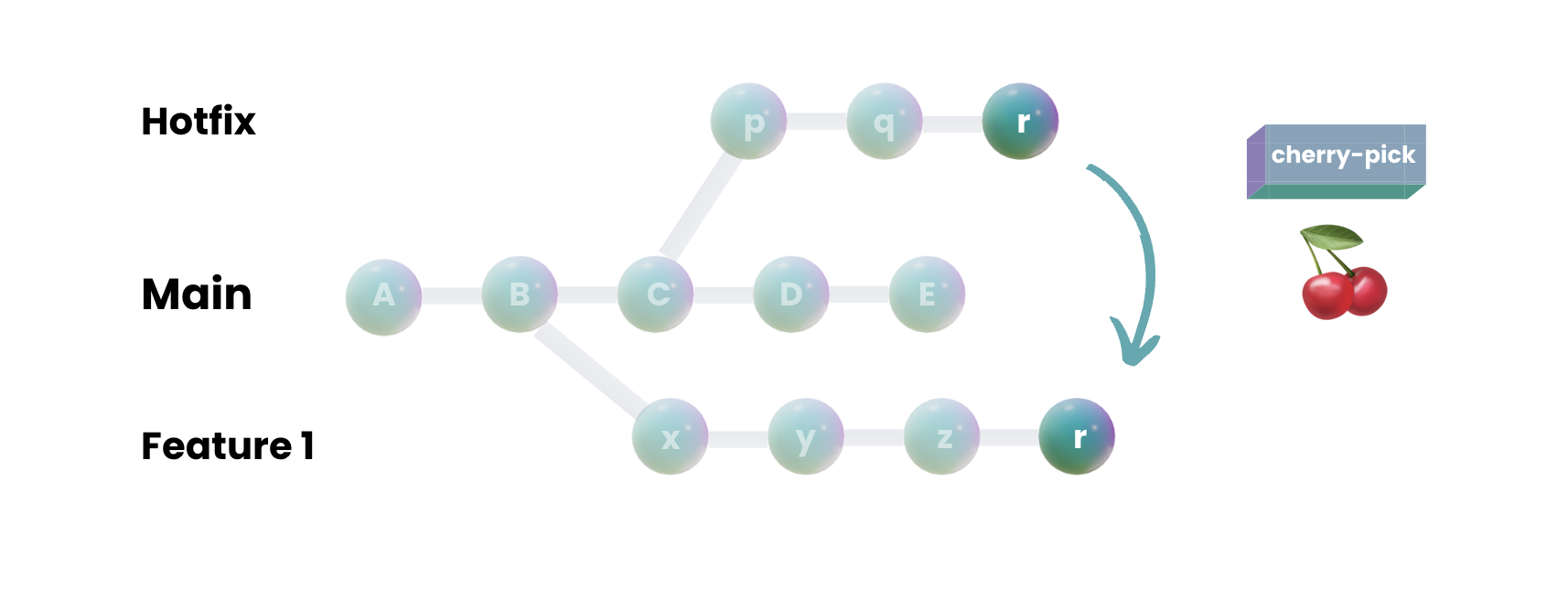 要先執行此操作,您必須確定要選擇的提交的提交哈希,您可以使用`git log`來執行此操作。一旦確定了提交哈希,您就可以執行: ``` git checkout target_branch git cherry-pick <commit-hash> # Do this multiple times if multiple commits are wanted git push origin target_branch ``` 高級 Git 指令 --------- ### 簽署提交 對提交進行簽名是一種驗證 Git 中提交的真實性和完整性的方法。它允許您使用 GPG (GNU Privacy Guard) 金鑰對您的提交進行加密簽名,從而向 Git 保證您確實是該提交的作者。您可以透過建立 GPG 金鑰並將 Git 配置為在提交時使用該金鑰來實現此目的。 步驟如下: ``` # Generate a GPG key gpg --gen-key # Configure Git to Use Your GPG Key git config --global user.signingkey <your-gpg-key-id> # Add the public key to your GitHub account # Signing your commits with the -S flag git commit -S -m "Your commit message" # View signed commits git log --show-signature ``` ### 轉發日誌 我們還沒有探討的一個主題是 Git 引用,它們是指向儲存庫中各種物件的指針,主要是提交,還有標籤和分支。它們可作為 Git 歷史記錄中的命名點,允許使用者瀏覽儲存庫的時間軸並存取專案的特定快照。了解如何導航 git 引用非常有用,他們可以使用 git reflog 來做到這一點。 以下是一些好處: - 恢復遺失的提交或分支 - 除錯和故障排除 - 糾正錯誤 ### 互動式變基 互動式變基是一個強大的 Git 功能,可讓您以互動方式重寫提交歷史記錄。它使您能夠在將提交應用到分支之前對其進行修改、重新排序、組合或刪除。 為了使用它,您必須熟悉可能的操作,例如: - 選擇(“p”) - 改寫(“r”) - 編輯(“e”) - 壁球(“s”) - 刪除(“d”) 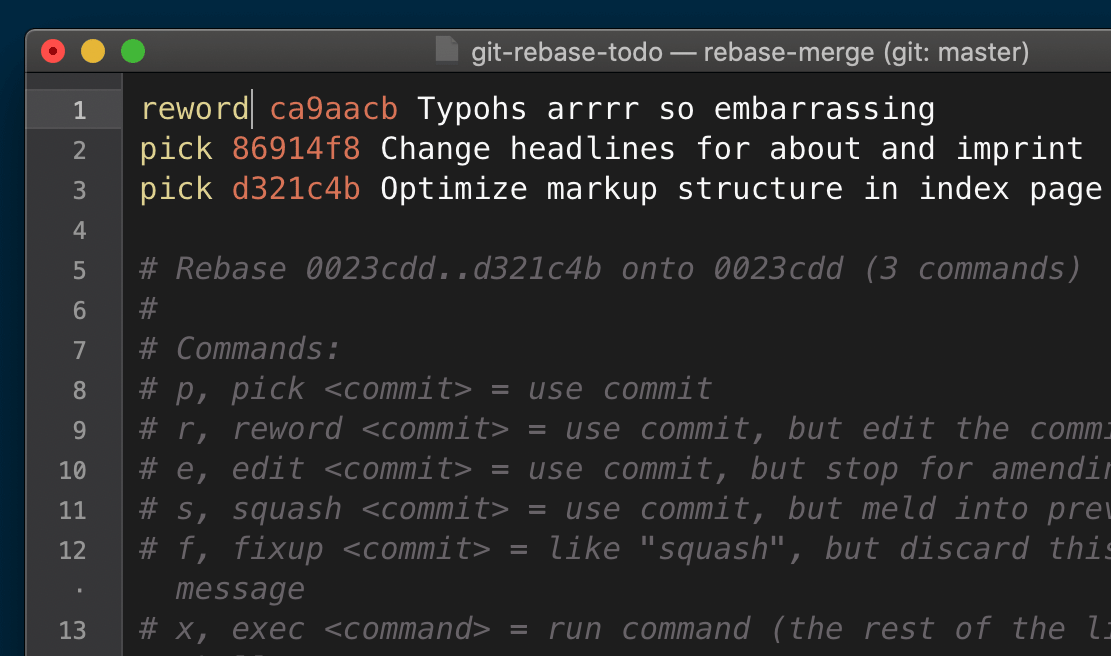 這是一個有用的[影片,](https://www.youtube.com/watch?v=qsTthZi23VE)用於學習如何在終端中執行互動式變基,我還在部落格文章的底部連結了一個有用的工具。 與 Git 協作 -------- ### 起源與上游 **來源**是複製本機 Git 儲存庫時與本機 Git 儲存庫關聯的預設遠端儲存庫。如果您分叉了一個儲存庫,那麼預設情況下該分叉將成為您的「原始」儲存庫。 另一方面,**上游**指的是您的儲存庫分叉的原始儲存庫。 為了讓您的分叉儲存庫與原始專案的最新更改保持同步,您可以從「上游」儲存庫中 git 取得更改,並將它們合併或變基到本機儲存庫中。 若要查看與本機 Git 儲存庫關聯的遠端儲存庫,請執行: ``` git remote -v ``` ### 衝突 不要驚慌,當嘗試合併或變基分支並檢測到衝突時,這只意味著存儲庫中同一文件或文件的不同版本之間存在衝突的更改,並且可以輕鬆解決(大多數情況下)。 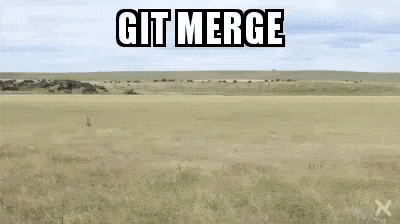 它們通常在受影響的文件中指示,Git 在其中插入衝突標記`<<<<<<<` 、 `=======`和`>>>>>>>`以突出顯示衝突部分。 決定保留、修改或刪除哪些更改,確保產生的程式碼有意義並保留預期的功能。 手動解決衝突檔案中的衝突後,刪除衝突標記`<<<<<<<` 、 `=======`和`>>>>>>>`並根據需要調整程式碼。 對解決方案感到滿意後,請儲存衝突文件中的變更。 > 如果您在解決衝突時遇到問題,該[影片](https://www.youtube.com/watch?v=xNVM5UxlFSA)可以很好地解釋它。 流行的 Git 工作流程 ------------ 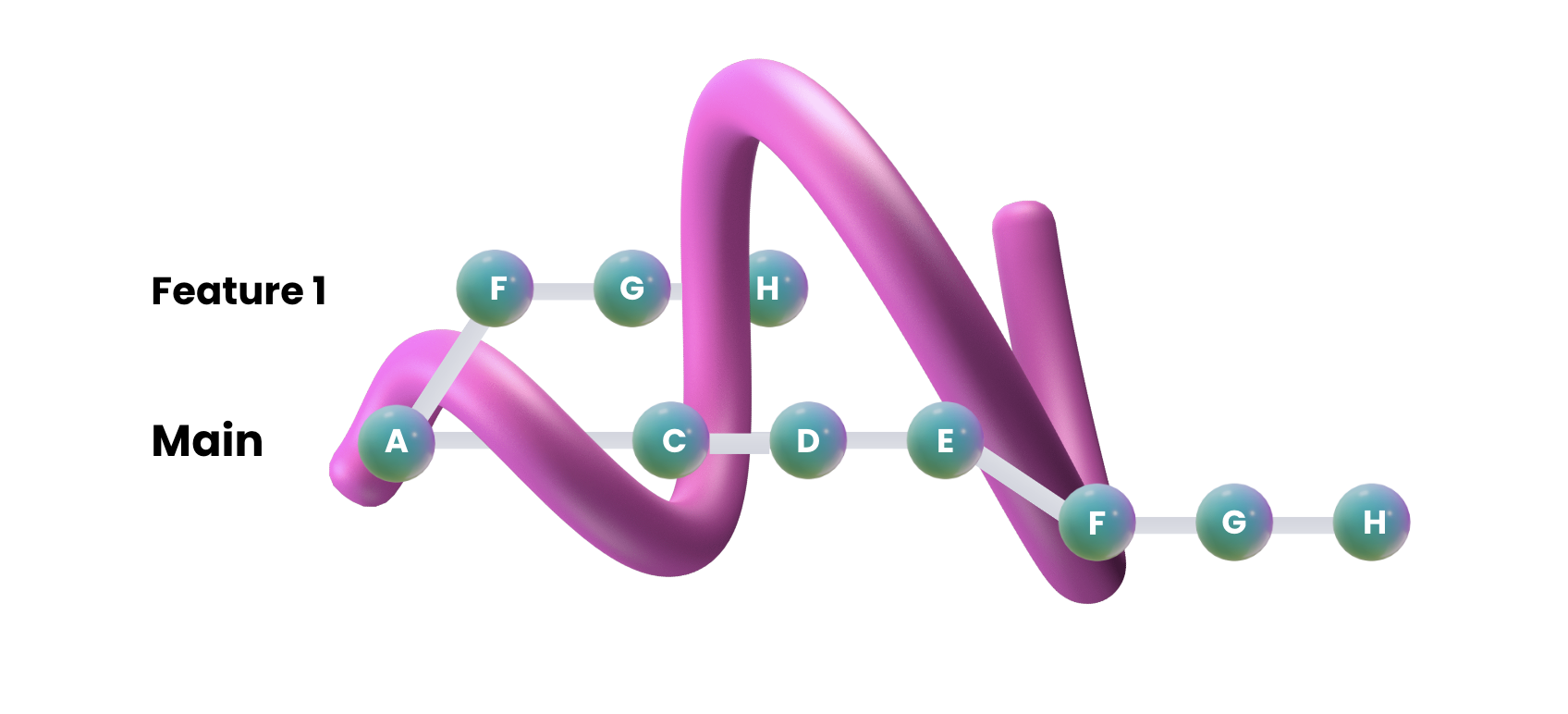 存在各種 Git 工作流程,但需要注意的是,不存在通用的「最佳」Git 工作流程。相反,每種方法都有其自身的優點和缺點。讓我們來探索這些不同的工作流程,以了解它們的優點和缺點。 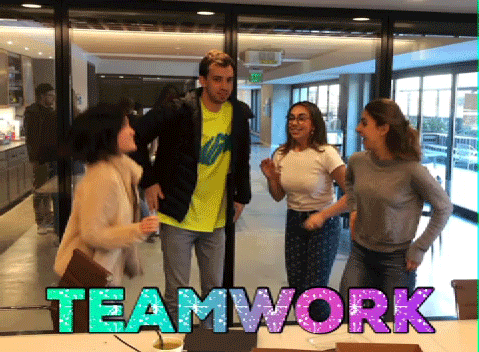 ### 功能分支工作流程🌱 每個新功能或錯誤修復都是在自己的分支中開發的,然後在完成後將其合併回主分支。 > - **優點**:隔離變更並減少衝突。 > - **弱點**:可能變得複雜並且需要勤奮的分行管理。 ### Gitflow 工作流程 🌊 Gitflow 定義了嚴格的分支模型,為不同類型的開發任務提供了預先定義的分支。 它包括長期分支,例如 main、develop、feature 分支、release 分支和 hotfix 分支。 > - **優點**:適合定期發布、長期維護的專案。 > - **缺點**:對於較小的團隊來說可能過於複雜 ### 分岔工作流程🍴 在此工作流程中,每個開發人員都會複製主儲存庫,但他們不會將變更直接推送到主儲存庫,而是將變更推送到自己的儲存庫分支。然後,開發人員建立拉取請求以提出對主儲存庫的更改,從而允許在合併之前進行程式碼審查和協作。 這是我們在開源 Glasskube 儲存庫上進行協作的工作流程。 > - **優點**:鼓勵外部貢獻者進行協作,而無需授予對主儲存庫的直接寫入存取權。 > - **弱點**:維持分支和主儲存庫之間的同步可能具有挑戰性。 ### 拉取請求工作流程 ⏩ 與分叉工作流程類似,但開發人員不是分叉,而是直接在主儲存庫中建立功能分支。 > - **優點**:促進團隊成員之間的程式碼審查、協作和知識共享。 > - **弱點**:對人類程式碼審查員的依賴可能會導致開發過程延遲。 ### 基於主幹的開發🪵 如果您所在的團隊專注於快速迭代和持續交付,您可能會使用基於主幹的開發,開發人員直接在主分支上工作,提交小而頻繁的變更。 > - **優勢**:促進快速迭代、持續集成,並專注於為生產提供小而頻繁的變更。 > - **缺點**:需要強大的自動化測試和部署管道來確保主分支的穩定性,可能不適合發佈時間表嚴格或功能開發複雜的專案。 ### 什麼叉子? 強烈建議在開源專案上進行分叉,因為您可以完全控制自己的儲存庫副本。您可以進行變更、嘗試新功能或修復錯誤,而不會影響原始專案。 > 💡 我花了很長時間才弄清楚,雖然分叉存儲庫作為單獨的實體開始,但它們保留了與原始存儲庫的連接。此連接可讓您追蹤原始專案中的變更並將您的分支與其他人所做的更新同步。 這就是為什麼即使您推送到原始存儲庫也是如此。您的變更也會顯示在遙控器上。 Git 備忘錄 ------- ``` # Clone a Repository git clone <repository_url> # Stage Changes for Commit git add <file(s)> # Commit Changes git commit -m "Commit message" # Push Changes to the Remote Repository git push # Force Push Changes (use with caution) git push --force # Reset Working Directory to Last Commit git reset --hard # Create a New Branch git branch <branch_name> # Switch to a Different Branch git checkout <branch_name> # Merge Changes from Another Branch git merge <branch_name> # Rebase Changes onto Another Branch (use with caution) git rebase <base_branch> # View Status of Working Directory git status # View Commit History git log # Undo Last Commit (use with caution) git reset --soft HEAD^ # Discard Changes in Working Directory git restore <file(s)> # Retrieve Lost Commit References git reflog # Interactive Rebase to Rearrange Commits git rebase --interactive HEAD~3 ``` --- 獎金!一些 Git 工具和資源可以讓您的生活更輕鬆。 -------------------------- - 互動式變基[工具](https://github.com/MitMaro/git-interactive-rebase-tool)。 - [Cdiff](https://github.com/amigrave/cdiff)查看豐富多彩的增量差異。 - 互動式 Git 分支[遊樂場](https://learngitbranching.js.org/?locale=en_US) --- 如果您喜歡這類內容並希望看到更多內容,請考慮在 GitHub 上給我們一個 Star 來支持我們🙏 [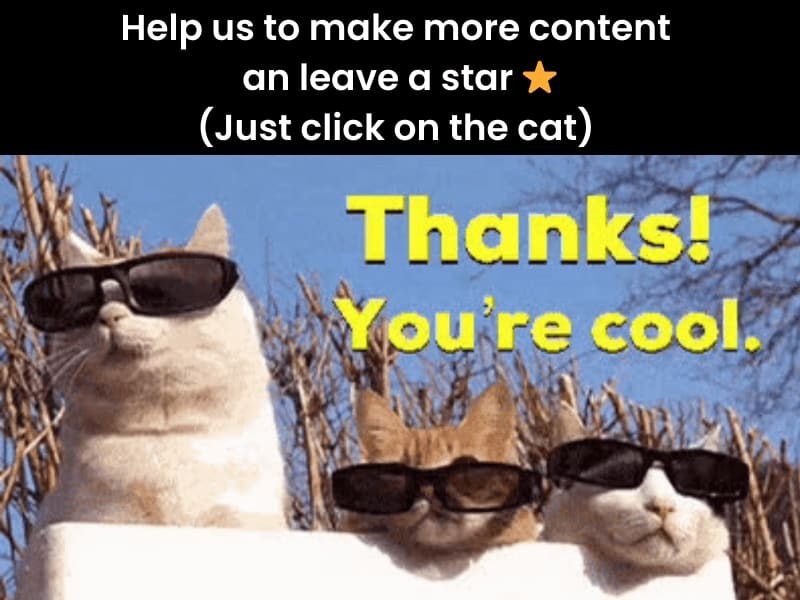](https://github.com/glasskube/glasskube) --- 原文出處:https://dev.to/glasskube/the-guide-to-git-i-never-had-1450
在 React 元件中組織程式碼有時經常被忽視,但在處理高階元件 (HoC)、 `forwardRef`和`memo`時,事情可能會變得複雜。如果處理不當,可能會導致程式碼混亂且難以維護。本文旨在透過提出一種更易於管理的方式來建立程式碼並提高工作效率來解決這些問題。 1️⃣ 在 React 元件中管理 HoC、 `forwardRef`和`memo` ------------------------------------------ ### 問題陳述 身為 React 開發人員,我經常在組織元件方面遇到困難,尤其是在合併`memo`和`forwardRef`時。以下的範例取自[React TypeScript Cheatsheet](https://react-typescript-cheatsheet.netlify.app/docs/basic/getting-started/forward_and_create_ref) ,示範了這個挑戰: ``` import { forwardRef } from 'react'; interface FancyButtonProps { type: 'submit' | 'button'; children?: React.ReactNode; } export const FancyButton = forwardRef<HTMLButtonElement, FancyButtonProps>((props, ref) => ( <button ref={ref} className="MyClassName" type={props.type}> {props.children} </button> )); ``` 根據我自己的經驗,這段程式碼有幾個問題: - 與「裸」元件相比,由於元件包裝在`forwardRef`中,程式碼顯得混亂。 - 類型並不是真正處於最佳位置。與元件中使用的實際參數相比, `forwardRef`的泛型具有相反的順序: `<HTMLButtonElement, FancyButtonProps>`與`(props, ref)` 。 - 重構變得很麻煩,因為它需要仔細注意方括號和圓括號。假設您不再需要`forwardRef` 。即使有了彩虹括號之類的幫助,處理這些括號和圓括號有時也會令人沮喪,尤其是當元件很大時。 - 如果您想要為元件新增`memo`或某些類型的自訂 HoC,以及其中的某些狀態或函數,該怎麼辦?它會變得更加混亂: ``` import { forwardRef, memo } from 'react'; import { useStyles } from '@/modules/core/styles'; interface FancyButtonProps { type: 'submit' | 'button'; children?: React.ReactNode; } export const FancyButton = memo( forwardRef<HTMLButtonElement, FancyButtonProps>(({ type, children }, ref) => { const classes = useStyles(); return <button ref={ref} className={classes.button} type={props.type}> {children} </button> }) ); ``` ### 建議的解決方案 在厭倦了以這種方式組織元件程式碼時遇到的所有麻煩之後,我最終想出了一種方法來解決這些問題。考慮以下方法: ``` import { forwardRef, memo } from 'react'; import { useStyles } from '@/modules/core/styles'; interface FancyButtonProps { type: 'submit' | 'button'; children?: React.ReactNode; } const FancyButtonBase = ( { type, children }: FancyButtonProps, ref: React.ForwardedRef<HTMLButtonElement> ) => { const classes = useStyles(); return ( <button ref={ref} className={classes.button} type={type}> {children} </button> ); }; export const FancyButton = memo(forwardRef(FancyButtonBase)); ``` 該程式碼是不言自明的。元件的實際程式碼位於名稱帶有`Base`後綴的函陣列件中。然後我們匯出下面的元件,以及所有 HoC、 `memo`和`forwardRef` 。 此解決方案具有以下幾個優點: - 實際的元件程式碼與 HoC、 `memo`和`forwardRef`分離,使其類似於「裸」元件。 - 類型位於它們應該在的位置: `(props: FancyButtonProps, ref: React.ForwardedRef<HTMLButtonElement>` 。 - 如果您需要重構此程式碼,例如不再使用`ref` ,您只需刪除第二個參數和`forwardRef`而無需處理方括號和圓括號。 - 如果需要嵌套的 HoC,加入或修改它們很簡單,並且程式碼仍然可讀: ``` import { forwardRef, memo } from 'react'; import { useStyles } from '@/modules/core/styles'; import { withWhatever } from '@/modules/core/hocs'; interface FancyButtonProps { type: 'submit' | 'button'; children?: React.ReactNode; } const FancyButtonBase = ( { type, children }: FancyButtonProps, ref: React.ForwardedRef<HTMLButtonElement> ) => { const classes = useStyles(); return ( <button ref={ref} className={classes.button} type={type}> {children} </button> ); }; export const FancyButton = memo(forwardRef(withWhatever(FancyButtonBase))); ``` 這種方法為元件加入了一行程式碼,但顯著提高了可讀性和可維護性。即使不使用任何 HoC、 `memo`或`forwardRef` ,我仍然對「裸」元件執行此操作: `export const FancyButton = FancyButtonBase` 。 我們可以更進一步,嘗試使用一個非常有用但不太知名的 VSCode 擴充功能來提高我們的工作效率。 2️⃣ 透過「資料夾模板」擴充功能提高工作效率 ----------------------- 下面的 GIF 演示了[VSCode 中「資料夾模板」擴充功能](https://marketplace.visualstudio.com/items?itemName=Huuums.vscode-fast-folder-structure)的強大功能(載入 GIF 可能需要一點時間): 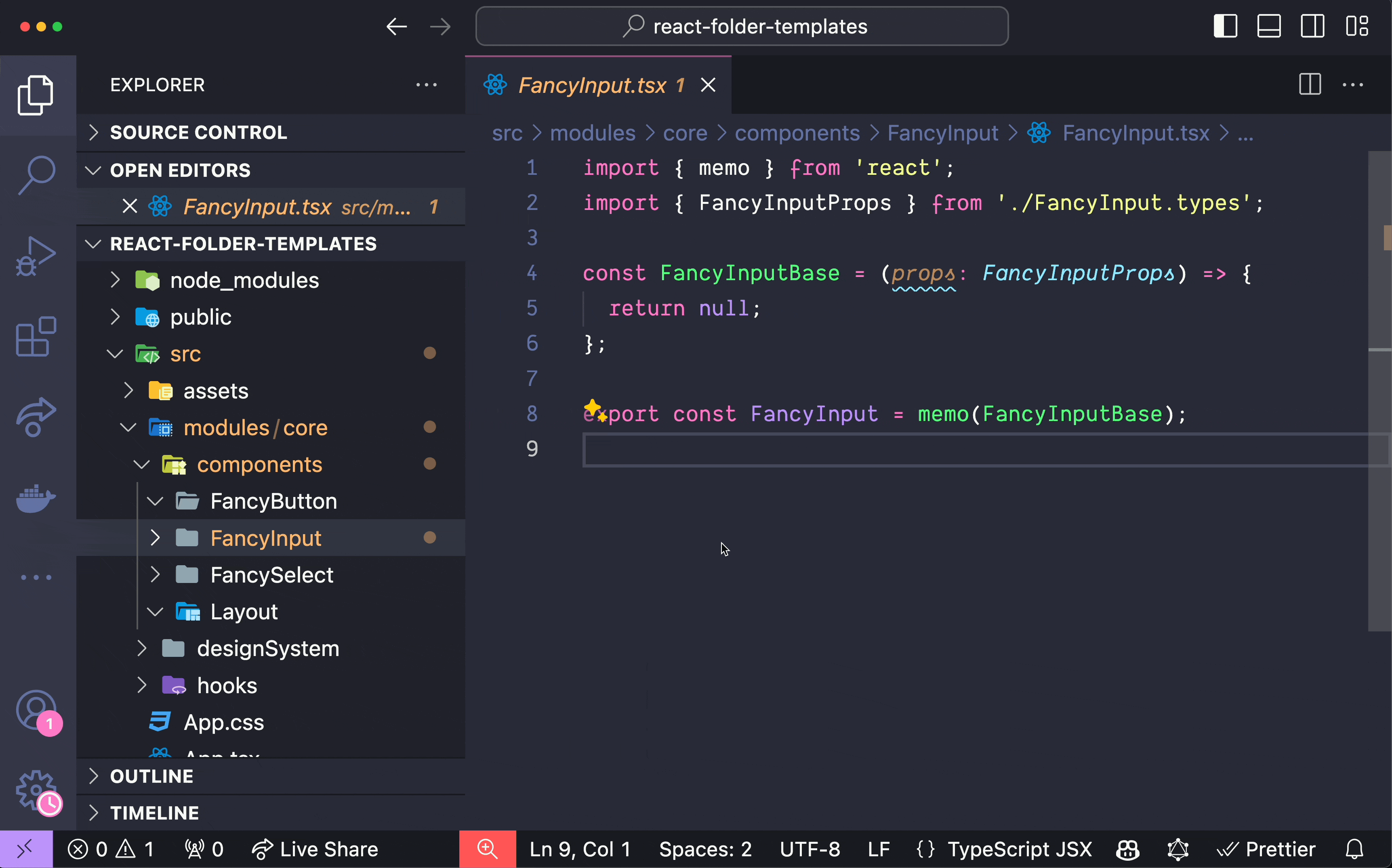 您只需輸入元件的名稱,瞧! 基本上,您也可以告訴 AI 為您編寫此樣板程式碼,但我發現使用此擴充功能要快得多,而且它是高度可自訂的。 如果您需要我分享我預先定義的 React 特定資料夾範本設置,請在評論中告訴我。 🏁 結論 ---- 在 React 元件中組織程式碼,尤其是在使用`memo` 、 `forwardRef`和 HoC 時,可能會是一項艱鉅的任務。然而,透過將實際的元件程式碼與 HoC 分開並確保正確的類型聲明,您可以建立更清晰、更易於維護的程式碼。此外,使用 VSCode 中的「資料夾範本」擴充功能等工具可以幫助簡化您的開發流程並提高工作效率。 如果你認為這是一篇好文章,你可能會發現我之前的文章也很有用: https://dev.to/itswillt/folder-structs-in-react-projects-3dp8 --- 原文出處:https://dev.to/itswillt/organizing-code-in-a-react-component-4coa
背景❔ --- 您是**DevOps**新手還是想學習一些 DevOps 工具?或者您可能已經是**DevOps 工程師,**正在尋找文件和練習技能的空間? 我在**GitHub**上建立了[**devops-basics**](https://github.com/tungbq/devops-basics)儲存庫來幫助您完成這一切! 🥳 簡介:波: ----- [**devops-basics**](https://github.com/tungbq/devops-basics)儲存庫將幫助您增強 DevOps 技能,並作為與 DevOps 相關的文件的書籤。主要特點包括: - **主題廣泛**:探索**20 多個**重要的 DevOps 主題,例如`Docker` 、 `Kubernetes` 、 `Terraform` 、 `Ansible` 、 `Jenkins` 、 `ELK` 、 `Cloud services` 、 `System Architecture` 、 `Monitoring`等。 - **有用的資源**:每個主題都附帶概述、官方文件連結、備忘錄和額外資源,以幫助您了解更多資訊。 - **實踐練習**:取得每個主題的基本範例,以便您可以嘗試所學內容。 - **進階範例**:一旦您掌握了基本概念,探索進階範例將進一步提高您的技能。 在以下部分中,我將引導您了解**devops-basics**儲存庫中的關鍵內容。 開始使用:火箭: -------- ### 什麼是 DevOps? DevOps 將開發 (Dev) 和營運 (Ops) 結合起來,與傳統流程相比,提高軟體開發和交付的效率、速度和安全性。更靈活的軟體開發生命週期可以為企業及其客戶帶來競爭優勢(資料來源:GitLab) ### 入門 - ➡️[入門](https://github.com/tungbq/devops-basics/tree/main/getting-started/) ### 開發營運圖  ### 開發營運工具鏈 - ➡️ [DevOps 工具鏈](https://en.wikipedia.org/wiki/DevOps_toolchain) ### 開發營運路線圖 - ➡️ [roadmap.sh/devops](https://roadmap.sh/devops) DevOps 主題 🔥 ----------- 我們的內容庫涵蓋了廣泛的 DevOps 主題,請在[**主題**](https://github.com/tungbq/devops-basics/tree/main/topics/)下探索它們。您也可以參考[**roadmap.sh/devops**](https://roadmap.sh/devops)查看每個主題在工具鏈中的位置 |專案 |內容 |官方文件 |動手實作 | | :-------------- | :------------------------------------------------- --------------- ----------------------------------- --- | :------------------------------------------------- --------------- ----------------------------------- --------------- -------- | :------------------------------------------------- --------------- ----------------------------------- --------------- ----------------------------------- -------- | |安塞布爾 |[安塞布爾](https://github.com/tungbq/devops-basics/tree/main/topics/ansible/)| 📖 [docs.ansible.com](https://docs.ansible.com/) | ✔️ [ansible-helloworld.sh](https://github.com/tungbq/devops-basics/tree/main/topics/ansible/basic/helloworld/ansible-helloworld.sh) | |碼頭工人 |[碼頭工人](https://github.com/tungbq/devops-basics/tree/main/topics/docker/)| 📖 [docs.docker.com](https://docs.docker.com/) | ✔️ [docker-helloworld.sh](https://github.com/tungbq/devops-basics/tree/main/topics/docker/basic/docker-helloworld.sh) | | Kubernetes (k8s) | [k8s](https://github.com/tungbq/devops-basics/tree/main/topics/k8s/) | 📖 [kubernetes.io/docs](https://kubernetes.io/docs/home/) | ✔️ [k8s-helloworld.sh](https://github.com/tungbq/devops-basics/tree/main/topics/k8s/basic/helloworld/k8s-helloworld.sh) | |伊斯蒂奧 |[伊斯蒂奧](https://github.com/tungbq/devops-basics/tree/main/topics/istio/)| 📖 [istio.io/latest/docs](https://istio.io/latest/docs/) | ✔️[入門](https://istio.io/latest/docs/setup/getting-started/)| |哎呀| [AWS](https://github.com/tungbq/devops-basics/tree/main/topics/aws/) | 📖 [docs.aws.amazon.com](https://docs.aws.amazon.com/) | ✔️ [EC2\_GetStarted](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/EC2_GetStarted.html) | |頭盔|[掌舵](https://github.com/tungbq/devops-basics/tree/main/topics/helm/)| 📖 [helm.sh/docs](https://helm.sh/docs/) | ✔️ [helm-helloworld.sh](https://github.com/tungbq/devops-basics/tree/main/topics/helm/basic/helm-helloworld.sh) | |詹金斯 |[詹金斯](https://github.com/tungbq/devops-basics/tree/main/topics/jenkins/)| 📖 [www.jenkins.io/doc](https://www.jenkins.io/doc/) | ✔️ [Jenkins-Hello-World.md](https://github.com/tungbq/devops-basics/tree/main/topics/jenkins/basic/Jenkins-Hello-World.md) | |地形 |[地形](https://github.com/tungbq/devops-basics/tree/main/topics/terraform/)| 📖 [terraform/文件](https://developer.hashicorp.com/terraform/docs)| ✔️ [terraform-helloworld.sh](https://github.com/tungbq/devops-basics/tree/main/topics/terraform/basic/terraform-helloworld.sh) | |殼牌|[殼](https://github.com/tungbq/devops-basics/tree/main/topics/shell/)| 📖 [devdocs.io/bash](https://devdocs.io/bash/) | ✔️ [basic.sh](https://github.com/tungbq/devops-basics/tree/main/topics/shell/basic/basic.sh) | |去 |[去](https://github.com/tungbq/devops-basics/tree/main/topics/git/)| 📖 [git-scm.com/doc](https://git-scm.com/doc) | ✔️ [git-helloworld.sh](https://github.com/tungbq/devops-basics/tree/main/topics/git/basic/hello-world/git-helloworld.sh) | |每個 |[每個](https://github.com/tungbq/devops-basics/tree/main/topics/elk/)| 📖 [www.elastic.co/guide](https://www.elastic.co/guide/index.html) | ✔️[任何/基本/helloworld](https://github.com/tungbq/devops-basics/tree/main/topics/elk/basic/helloworld/) | |阿爾戈CD |[阿爾古德](https://github.com/tungbq/devops-basics/tree/main/topics/argocd/)| 📖 [argo-cd.readthedocs.io](https://argo-cd.readthedocs.io/en/stable/) | ✔️ [argocd/基本](https://github.com/tungbq/devops-basics/tree/main/topics/argocd/basic/)| | Github-Action | [github 行動](https://github.com/tungbq/devops-basics/tree/main/topics/github-action/)| 📖 [docs.github.com/actions](https://docs.github.com/actions) | ✔️[建立第一個工作流程](https://docs.github.com/en/actions/quickstart#creating-your-first-workflow)| |亞搏體育appGitlab CI | gitlab-ci | [gitlab-ci](https://github.com/tungbq/devops-basics/tree/main/topics/gitlabci/) | 📖 [docs.gitlab.com/ee/ci](https://docs.gitlab.com/ee/ci/) | ✔️[建立第一個管道](https://docs.gitlab.com/ee/ci/quick_start/)| |格羅維 |[絕妙](https://github.com/tungbq/devops-basics/tree/main/topics/groovy/)| 📖 [groovy-lang.org](https://groovy-lang.org/documentation.html) | ✔️[絕妙/基本](https://github.com/tungbq/devops-basics/tree/main/topics/groovy/basic/)| |普羅米修斯|[普羅米修斯](https://github.com/tungbq/devops-basics/tree/main/topics/prometheus/)| 📖 [prometheus.io/docs](https://prometheus.io/docs/) | ✔️ [prometheus-helloworld.sh](https://github.com/tungbq/devops-basics/tree/main/topics/prometheus/basic/prometheus-helloworld.sh) | |蟒蛇 |[蟒蛇](https://github.com/tungbq/devops-basics/tree/main/topics/python/)| 📖 [www.python.org/doc](https://www.python.org/doc/) | ✔️[蟒蛇/基本](https://github.com/tungbq/devops-basics/tree/main/topics/python/basic/)| |開放堆疊 |[開放堆疊](https://github.com/tungbq/devops-basics/tree/main/topics/openstack/)| 📖 [docs.openstack.org](https://docs.openstack.org/2023.2/) | ✔️ [openstack/helloworld](https://github.com/tungbq/devops-basics/tree/main/topics/openstack/basic/) | | Azure-DevOps |[天藍色](https://github.com/tungbq/devops-basics/tree/main/topics/azuredevops/)的📖 [learn.microsoft.com](https://learn.microsoft.com/en-us/azure/devops) | ✔️ [azuredevops/基本](https://github.com/tungbq/devops-basics/tree/main/topics/azuredevops/basic/)| |編碼 |[編碼](https://github.com/tungbq/devops-basics/tree/main/topics/coding/)| 📖[編碼](https://github.com/tungbq/devops-basics/tree/main/topics/coding/)| 🏃 進行中 | |建築|[建築](https://github.com/tungbq/devops-basics/tree/main/topics/architecture/)| 📖[架構/README.md](https://github.com/tungbq/devops-basic/blob/main/topics/architecture/README.md) | ⏩即將推出 | |包裝機|即將推出 | 📖 [www.packer.io](https://www.packer.io/) | ⏩即將推出 | |微服務|[微服務](https://github.com/tungbq/devops-basics/tree/main/topics/microservices/)| 📖 [AWS/微服務](https://aws.amazon.com/microservices/)| ⏩ 即將推出 | | HashiCorp 金庫 |即將推出 | 📖 [hashcorp/vault](https://developer.hashicorp.com/vault/docs) | ⏩ 即將推出 | - 還有**更多即將推出的主題...⏩**您可以關注此存儲庫以獲取更多最新內容 - 有關練習這些工具的其他資源,請造訪: [**devops-project**](https://github.com/tungbq/devops-project) 結論 -- [**devops-basics**](https://github.com/tungbq/devops-basics)儲存庫是您進行 DevOps 學習和實踐的首選資源。無論您是初學者還是經驗豐富的工程師,此儲存庫都可以提供您增強技能並在 DevOps 中取得成功所需的內容。立即探索並升級您的 DevOps 之旅! 如果您發現此存儲庫有幫助,請考慮給它一顆星⭐️以表達您的讚賞。你能給我的任何星星都會幫助我更成長❤️ < 表> ``` <tr> ``` ``` <td> ``` ``` <a href="https://github.com/tungbq/devops-basics" style="text-decoration: none;"><strong>Star devops-basics ⭐️ on GitHub</strong></a> ``` ``` </td> ``` ``` </tr> ``` 謝謝您,編碼愉快! 🔥 --- 原文出處:https://dev.to/tungbq/the-devops-basics-3ecm
我必須承認,我很難記住事情。尤其是當涉及到 CSS 時。例如 Flexbox 佈局。 Flex 容器的`justify-content`屬性可以有[超過**12 個**不同的值](https://developer.mozilla.org/en-US/docs/Web/CSS/justify-content),其中許多值可以與關鍵字*safe*或*unsafe*組合。要閱讀[CSS Tricks 上的 Flexbox 完整指南](https://css-tricks.com/snippets/css/a-guide-to-flexbox/),您必須滾動瀏覽高度超過 20k 像素的兩列頁面 - 儘管標題暗示了這一點,但它們並沒有涵蓋所有內容。 我最近偶然發現了一款塔防遊戲教學 Flexbox,這真的... **等等,什麼?** 是的,事實證明,有很多遊戲可以幫助教授 CSS。我收集了一些對我有用的免費 CSS 遊戲,也許可以幫助您再次享受 CSS 的樂趣! 1.Flexbox防禦 ----------- 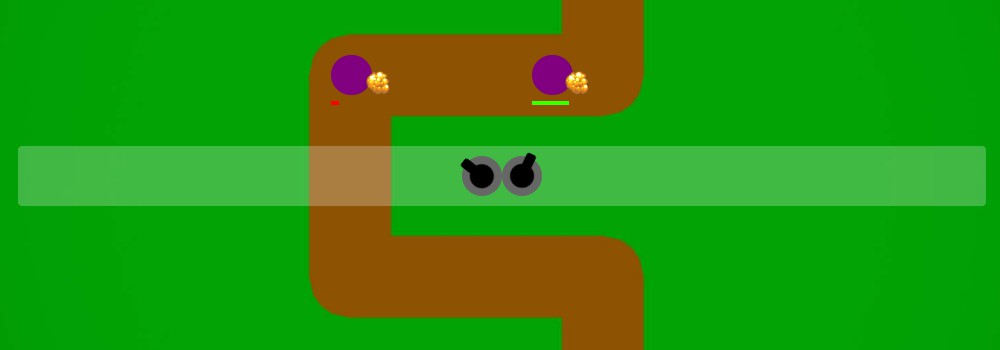 我剛剛提到了這個遊戲。它涵蓋了 12 個不同等級的 Flex 屬性`align-items` 、 `justify-content` 、 `flex-direction` 、 `align-self`和`order` 。尤其是最後 4 個關卡真的很有趣,而且有點棘手。 - 遊戲:http://www.flexboxdefense.com - 貢獻:https://github.com/channingallen/tower-defense - 創作者:[查寧·艾倫](https://github.com/channingallen) 2. Flexbox 青蛙 ------------- 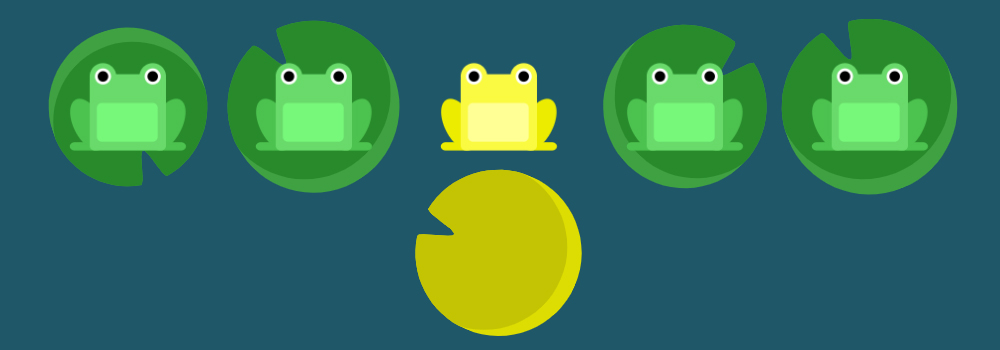 這個遊戲也是關於[Flexbox的](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_Flexible_Box_Layout/Basic_Concepts_of_Flexbox),它涵蓋了更多的flex屬性: `align-items` , `justify-content` , `align-content` , `flex-direction` , `align-self` , `flex-wrap` , `flex-flow`和`order`在24個不同的級別。如果您解決了最後一關,請發表評論。 - 遊戲:https://flexboxfroggy.com - 貢獻:https://github.com/thomaspark/flexboxfroggy - 建立者: [Codepip](https://codepip.com) 3.網格花園 ------ 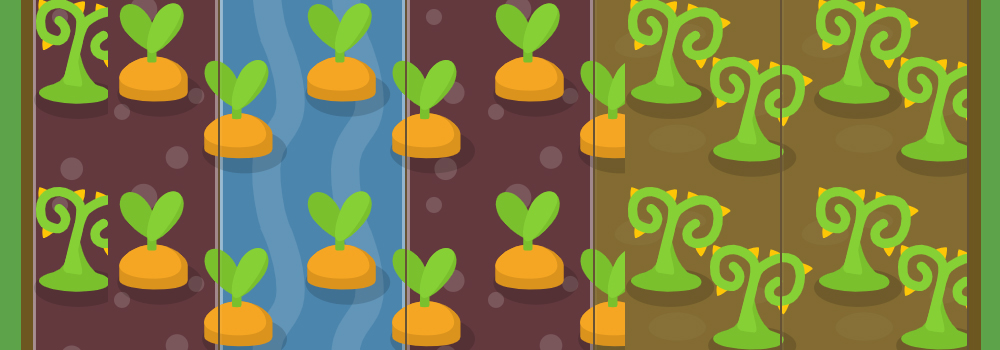 在 28 個不同的層級中,您可以學習[CSS 網格佈局](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_Grid_Layout)。它涵蓋以下網格屬性: `grid-column-start` , `grid-column-end` , `grid-column` , `grid-row-start` , `grid-row-end` , `grid-row` `grid-area` , `order` , `grid-template-columns` 、 `grid-template-rows`和`grid-template` 。 - 遊戲:https://cssgridgarden.com - 貢獻:https://github.com/thomaspark/gridgarden - 建立者: [Codepip](https://codepip.com) 4. CSS 餐廳 --------- 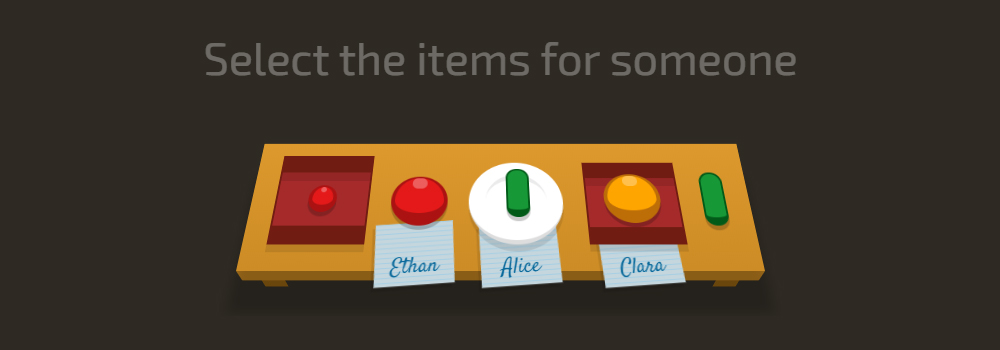 這是一個關於各種[CSS 選擇器](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_Selectors)的小遊戲。嘗試掌握所有 32 個級別,稱自己為 CSS 選擇器專家 - 並且很餓😋。 - 遊戲:http://flukeout.github.io - 貢獻:https://github.com/flukeout/css-diner - 創作者:[路克‧帕科爾斯基](https://github.com/flukeout) 5.展開 ---- 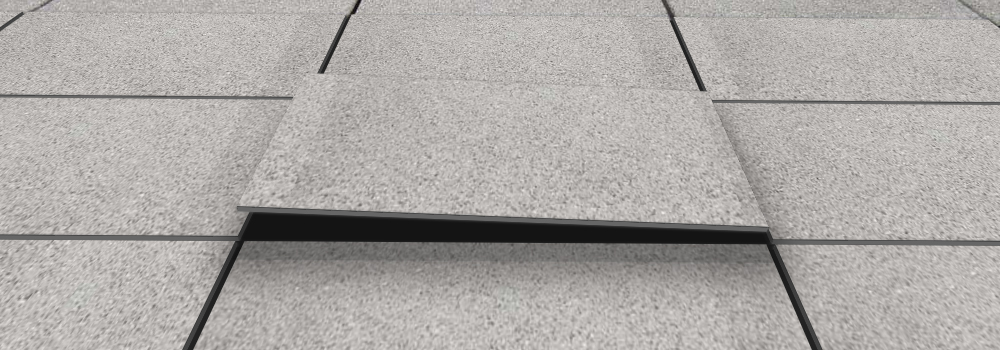 這並不完全是一個遊戲,而是一個關於[CSS 3D 變換的](https://developer.mozilla.org/en-US/docs/Web/CSS/transform)互動式演示。你可能會認為這很無聊,但相信我:動畫很棒,你不會認為這用純 CSS 是不可能的。 - 遊戲:https://rupl.github.io/unfold - 貢獻:https://github.com/rupl/unfold - 創作者:[克里斯魯佩爾](https://chrisruppel.com) 6. 路線圖 ------ 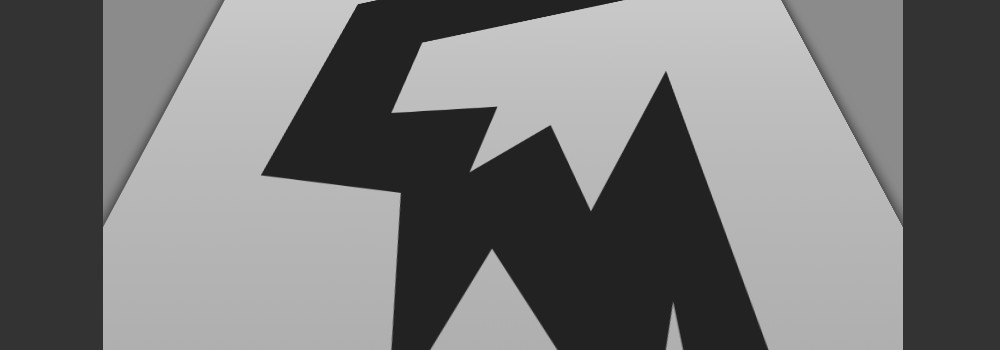 解決這個僅用 CSS 和 HTML 製作的小遊戲需要技巧和速度。它不是直接教CSS,而是透過查看原始碼教授了很多關於`clip-path` 、 `transform`和`animation` with `@keyframes`的內容! 請發表評論,你需要嘗試多少次才能獲勝 - 我需要 8 次! 😅 - 遊戲:http://victordarras.fr/cssgame - 創作者:[維克多·達拉斯](https://twitter.com/victordarras) 7. 嘉年華 ------ 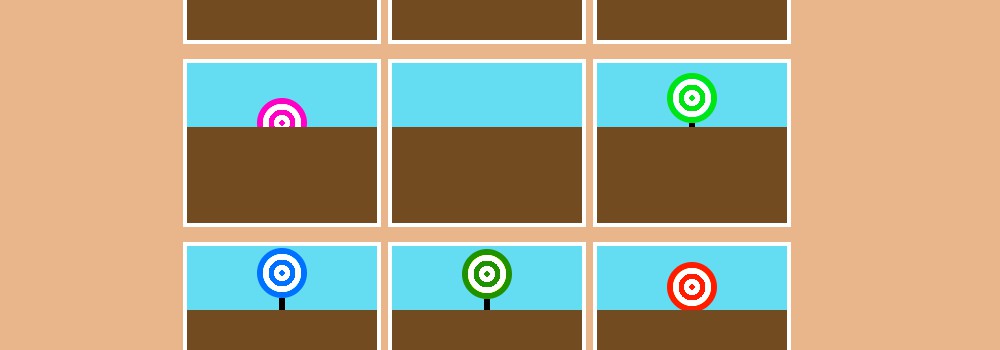 你只有 8 秒的時間來擊中所有目標!一個不錯的 CSS 小遊戲,使用複選框和 CSS 動畫。 - 遊戲:https://codepen.io/una/pen/NxZaNr - 創作者:[烏娜·克拉維茨](https://github.com/una) 8. 井字遊戲 ------- 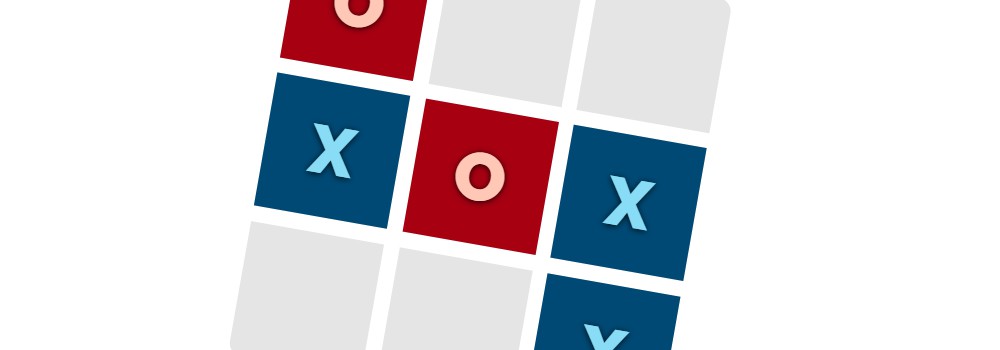 最後成為經典。 Tic-Tac-Toe 是純 CSS 遊戲,有 2 個難度級別,也使用複選框和 CSS 動畫。 - 遊戲:https://codepen.io/alvaromontoro/pen/BexWOw - 創作者:[阿爾瓦羅·蒙托羅](https://github.com/alvaromontoro) 獎金 -- 這是獎勵部分,其中包含評論中的建議: ### 9. Flexbox 殭屍 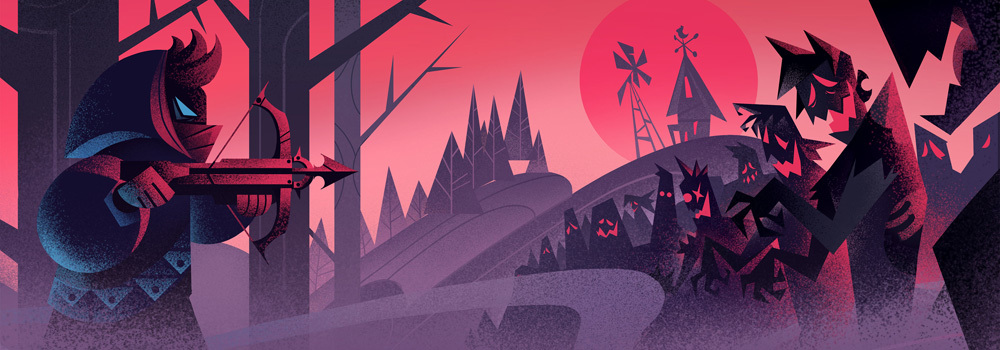 這是一個故事情節驅動的訓練課程,您可以在其中學習使用 Flexbox 和弩來獵殺殭屍。*需要註冊。* - 遊戲:https://mastery.games/flexboxzombies/ - 價格:179 美元(但在撰寫本文時**免費**) - 創作者:[戴夫·格迪斯](https://twitter.com/geddski) ### 10. 服務工程 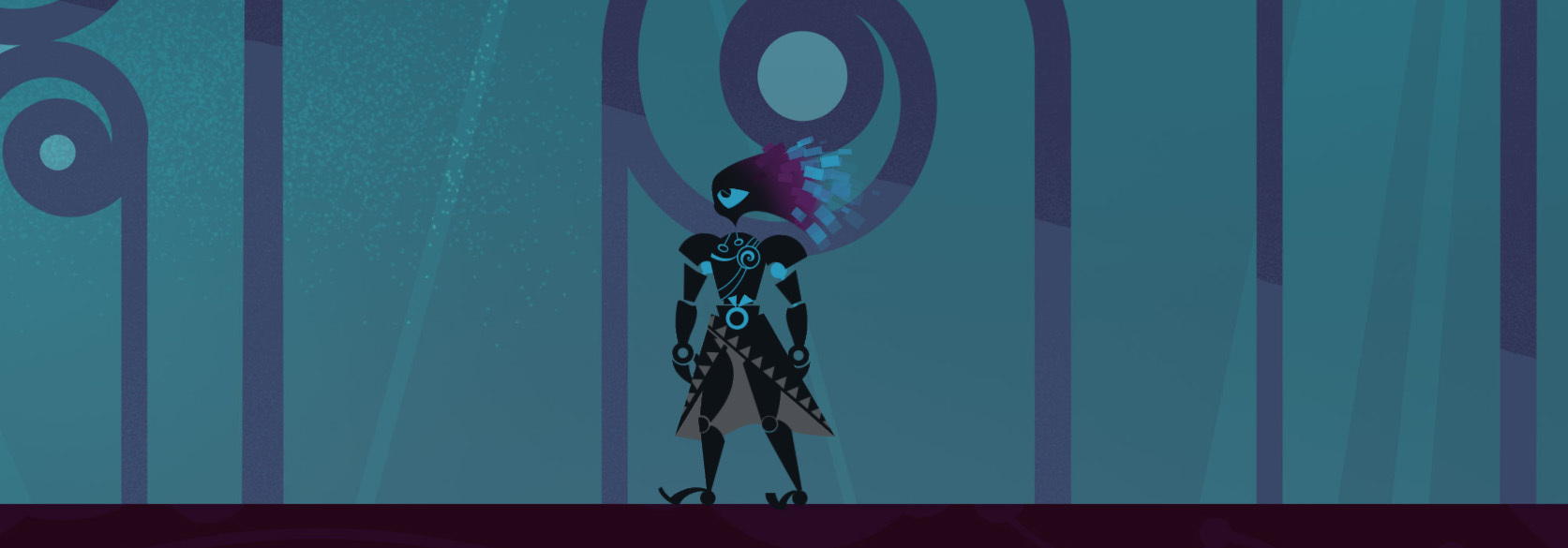 在這次冒險中,您將學習如何避免 PWA 陷阱。您將提升您的技能並與 Service Workers 一起成長。也許甚至可以殺死幾個世紀以來困擾貧窮鄉村工人的野蠻野獸!*需要註冊。* - 遊戲:https://serviceworkies.com - 價格:179 美元(但在撰寫本文時**免費**) - 創作者:[戴夫·格迪斯](https://twitter.com/geddski) ### 11. 網格小動物 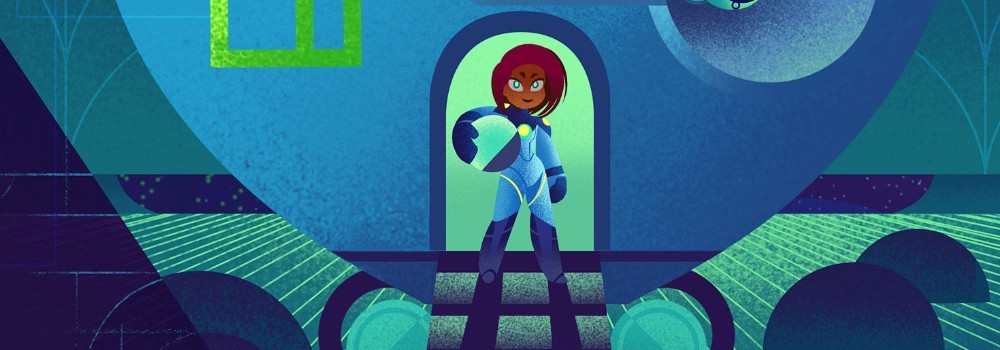 您掌握 CSS Grid 的旅程從神秘的 Grideros 星球開始。你的任務是使用你的飛船強大的網格工具來拯救外星生物免於滅絕。*需要註冊。* - 遊戲:https://gridcritters.com - 價格:179 美元 - 創作者:[戴夫·格迪斯](https://twitter.com/geddski) ### 12.CSS戰鬥 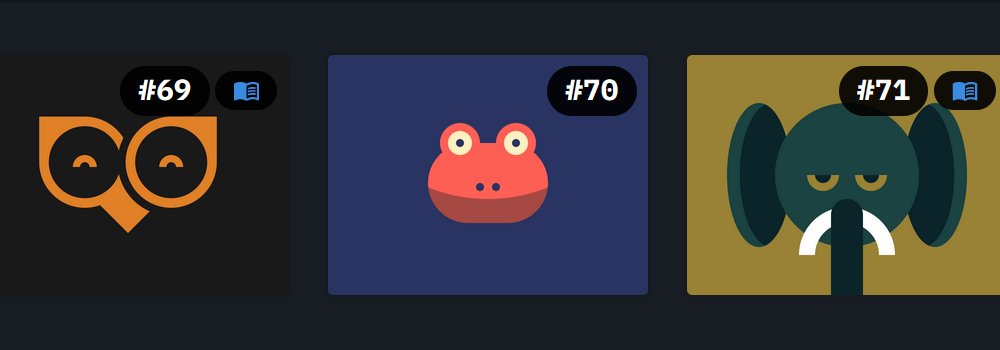 在這款線上 CSS 程式碼高爾夫遊戲中,來自世界各地的玩家嘗試用盡可能小的 CSS 程式碼直觀地複製“目標”,並通過戰鬥力爭登上排行榜的榜首。 - 遊戲:https://cssbattle.dev - 創作者: [Kushagra Gour](https://twitter.com/chinchang457)和[Kushagra Agarwal](https://twitter.com/kushsolitary) 把它包起來 ----- 無論您是初學者還是專家,都沒關係 - 我希望您在玩遊戲時玩得開心,同時學習一些有關 CSS 的知識!特別是在 Codepen 上,您可以找到許多人們僅使用 HTML 和 CSS 建立的精彩遊戲。 如果您知道其他很棒的 CSS 學習遊戲,請在下面的評論中告訴大家。 --- *編輯:2021 年 7 月 2 日*(修復 Flexbox 殭屍連結,加入 cssbattle) *編輯:2019 年 10 月 29 日*(加入 Dave Geddes 在評論中推薦的 3 個獎勵遊戲) *編輯:2019 年 10 月 28 日*(從錯誤的 Flexbox 範例`justify-items`切換到`justify-content` ) *原文發佈於:2019 年 10 月 24 日, [Medium](https://medium.com/@devmount/8-games-to-learn-css-the-fun-way-9b0b8b581d91)* --- 原文出處:https://dev.to/devmount/8-games-to-learn-css-the-fun-way-4e0f
今天,我們將學習如何使用 Wing 作為後端建立全端應用程式。 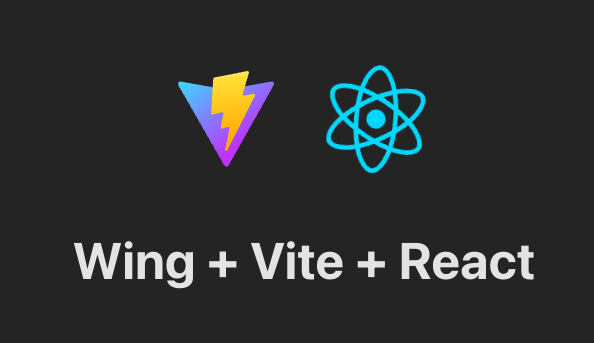 我們將使用 React 和 Vite 作為前端。 我知道還有其他框架,如 Vue、Angular 和 Next,但 React 仍然是最常見的,並且迄今為止有大量值得信賴的新創公司使用它。 如果您不知道, [React](https://github.com/facebook/react)是 Facebook 建立的開源程式庫,用於建立 Web 和本機使用者介面。正如您從儲存庫中看到的,它被超過 2040 萬開發人員使用。所以,這是值得的。 讓我們看看如何使用 Wing 作為後端。 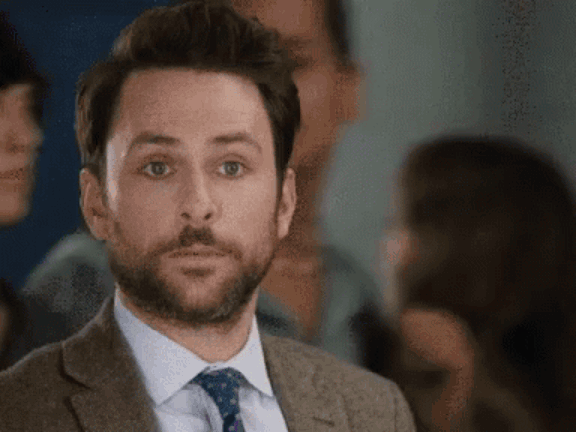 --- [Wing](https://git.new/wing-repo) - 一種雲端程式語言。 --------------------------------------------- 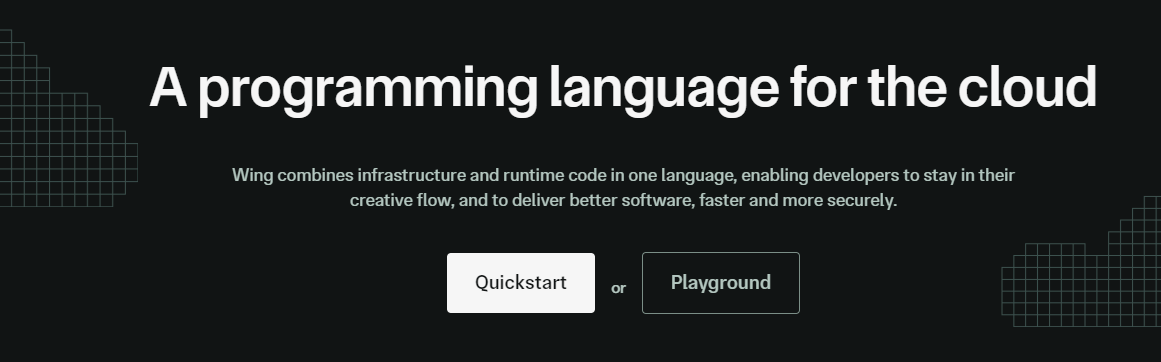 Winglang 是一種專為雲端(又稱「面向雲端」)設計的新型開源程式語言。它允許您在雲端中建立應用程式,並且具有相當簡單的語法。 Wing 程式可以使用功能齊全的模擬器在本地執行(是的,不需要網路),也可以部署到任何雲端供應商。 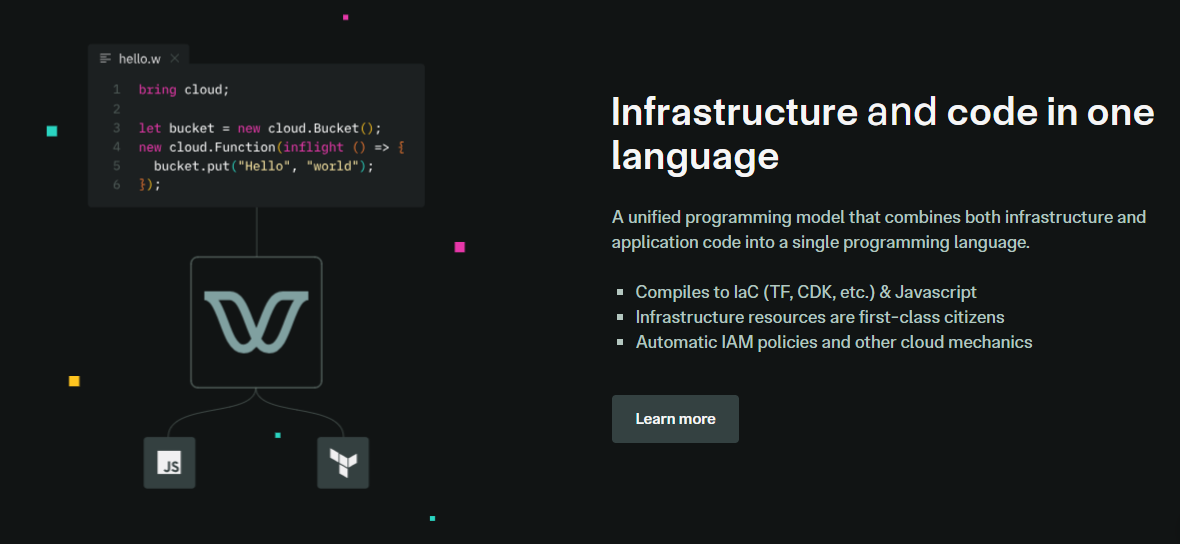 Wing 需要 Node `v20 or higher` 。 建立一個父目錄(我們使用的`shared-counter` )並使用 Vite 使用新的 React 應用程式設定前端。您可以使用這個 npm 指令。 ``` npm create -y vite frontend -- --template react-ts // once installed, you can check if it's running properly. cd frontend npm install npm run dev ``` 您可以使用此 npm 命令安裝 Wing。 ``` npm install -g winglang ``` 您可以使用`wing -V`驗證安裝。 Wing 還提供官方[VSCode 擴充功能](https://marketplace.visualstudio.com/items?itemName=Monada.vscode-wing)和[IntelliJ](https://plugins.jetbrains.com/plugin/22353-wing) ,後者提供語法突出顯示、補全、轉到定義和嵌入式 Wing 控制台支援。您可以在建立應用程式之前安裝它! 建立後端目錄。 ``` mkdir ~/shared-counter/backend cd ~/shared-counter/backend ``` 建立一個新的空 Wing 專案。 ``` wing new empty // This will generate three files: package.json, package-lock.json and main.w file with a simple "hello world" program wing it // to run it in the Wing simulator // The Wing Simulator will be opened in your browser and will show a map of your app with a single function. //You can invoke the function from the interaction panel and check out the result. ``` 使用指令`wing new empty`後的結構如下。 ``` bring cloud; // define a queue, a bucket, and a counter let bucket = new cloud.Bucket(); let counter = new cloud.Counter(initial: 1); let queue = new cloud.Queue(); // When a message is received in the queue -> it should be consumed // by the following closure queue.setConsumer(inflight (message: str) => { // Increment the distributed counter, the index variable will // store the value before the increment let index = counter.inc(); // Once two messages are pushed to the queue, e.g. "Wing" and "Queue". // Two files will be created: // - wing-1.txt with "Hello Wing" // - wing-2.txt with "Hello Queue" bucket.put("wing-{index}.txt", "Hello, {message}"); log("file wing-{index}.txt created"); }); ``` 您可以安裝`@winglibs/vite`來啟動開發伺服器,而不是使用`npm run dev`來啟動本機 Web 伺服器。 ``` // in the backend directory npm i @winglibs/vite ``` 您可以使用`backend/main.w`中提供的 publicEnv 將資料傳送到前端。 讓我們來看一個小例子。 ``` // backend/main.w bring vite; new vite.Vite( root: "../frontend", publicEnv: { TITLE: "Wing + Vite + React" } ); // import it in frontend // frontend/src/App.tsx import "../.winglibs/wing-env.d.ts" //You can access that value like this. <h1>{window.wing.env.TITLE}</h1> ``` 你還可以做更多: - 讀取/更新 API 路線並使用 Wing Simulator 檢查它。 - 使用後端獲取值。 - 使用`@winglibs/websockets`同步瀏覽器,它在後端部署一個 WebSocket 伺服器,您可以連接此 WebSocket 來接收即時通知。 您可以閱讀完整的逐步指南,以了解[如何使用 React 作為前端和 Wing 作為後端建立簡單的 Web 應用程式](https://www.winglang.io/docs/guides/react-vite-websockets)。測試是使用 Wing Simulator 完成的,並使用 Terraform 部署到 AWS。 部署後的AWS架構是這樣的。 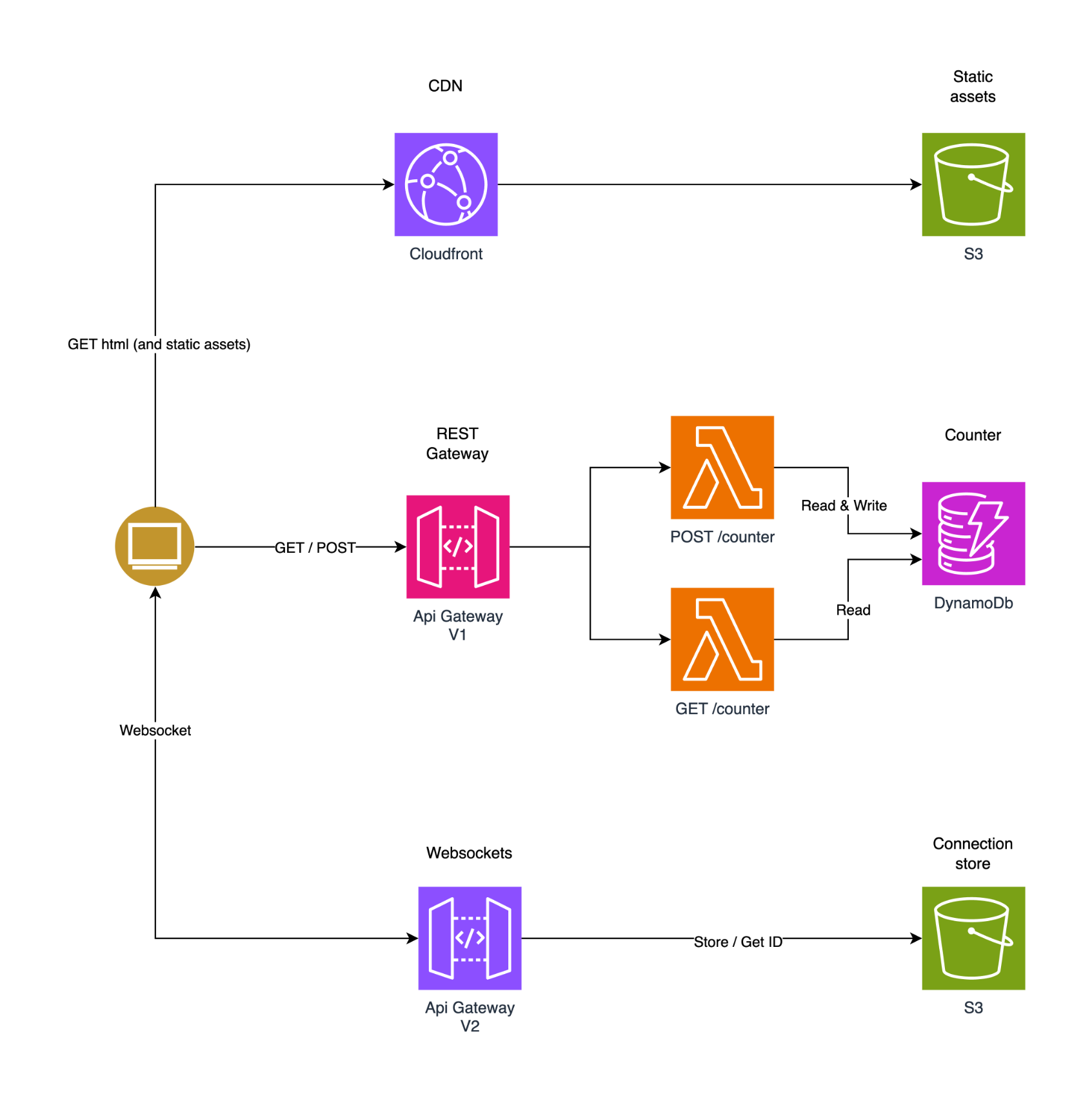 為了提供開發者選擇和更好的體驗,Wing 推出了對[TypeScript (Wing)](https://www.winglang.io/docs/typescript/)等其他語言的全面支援。唯一強制性的事情是您必須安裝 Wing SDK。 這也將使控制台完全可用於本地偵錯和測試,而無需學習 Wing 語言。 Wing 甚至還有其他[指南](https://www.winglang.io/docs/category/guides),因此更容易遵循。 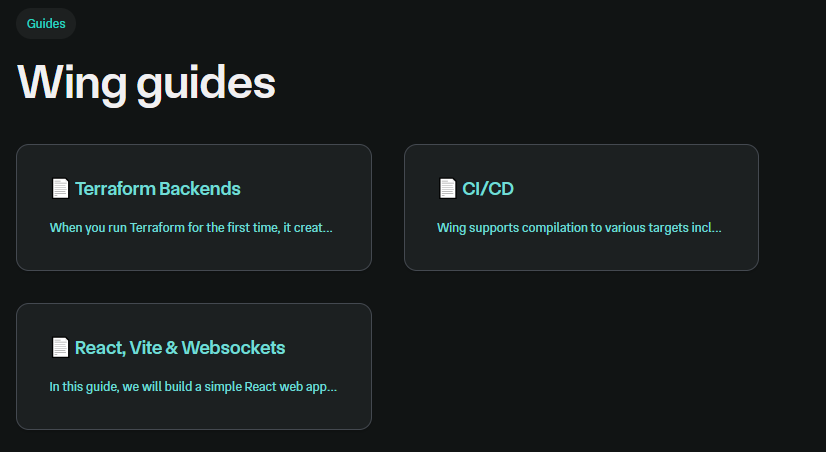 您可以閱讀[文件](https://www.winglang.io/docs)並查看[範例](https://www.winglang.io/docs/category/examples)。 您也可以在[Playground](https://www.winglang.io/play/?code=LwAvACAAVABoAGkAcwAgAGkAcwAgAHQAaABlACAAaQBtAHAAbwByAHQAIABzAHQAYQB0AGUAbQBlAG4AdAAgAGkAbgAgAFcAaQBuAGcALgAKAC8ALwAgAEgAZQByAGUAIAB3AGUAIABiAHIAaQBuAGcAIAB0AGgAZQAgAFcAaQBuAGcAIABzAHQAYQBuAGQAYQByAGQAIABsAGkAYgByAGEAcgB5ACAAdABoAGEAdAAgAAoALwAvACAAYwBvAG4AdABhAGkAbgBzACAAYQBiAHMAdAByAGEAYwB0AGkAbwBuAHMAIABvAGYAIABwAG8AcAB1AGwAYQByACAAYwBsAG8AdQBkACAAcwBlAHIAdgBpAGMAZQBzAC4ACgBiAHIAaQBuAGcAIABjAGwAbwB1AGQAOwAKAAoALwAvACAAVABoAGkAcwAgAGMAbwBkAGUAIABkAGUAZgBpAG4AZQBzACAAYQAgAGIAdQBjAGsAZQB0ACAAYQBzACAAcABhAHIAdAAgAG8AZgAgAHkAbwB1AHIAIABhAHAAcAAuAAoALwAvACAAVwBoAGUAbgAgAGMAbwBtAHAAaQBsAGkAbgBnACAAdABvACAAYQAgAHMAcABlAGMAaQBmAGkAYwAgAGMAbABvAHUAZAAgAHAAcgBvAHYAaQBkAGUAcgAKAC8ALwAgAGkAdAAgAHcAaQBsAGwAIABiAGUAIABzAHUAYgBzAHQAaQB0AHUAdABlAGQAIABiAHkAIABhAG4AIABpAG0AcABsAGUAbQBlAG4AdABhAHQAaQBvAG4AIABmAG8AcgAKAC8ALwAgAHQAaABhAHQAIABjAGwAbwB1AGQALgAgAEkALgBlACwAIABmAG8AcgAgAEEAVwBTACAAaQB0ACAAdwBpAGwAbAAgAGIAZQAgAGEAbgAgAFMAMwAgAEIAdQBjAGsAZQB0AC4ACgBsAGUAdAAgAGIAdQBjAGsAZQB0ACAAPQAgAG4AZQB3ACAAYwBsAG8AdQBkAC4AQgB1AGMAawBlAHQAKAApADsACgAKAC8ALwAgACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAKAC8ALwAgAFkAbwB1ACAAYwBhAG4AIABpAG4AdABlAHIAYQBjAHQAIAB3AGkAdABoACAAdABoAGUAIABhAHAAcAAgAGkAbgAgAHQAaABlACAAYwBvAG4AcwBvAGwAZQAgAC0ALQA%2BAAoALwAvACAACgAvAC8AIABDAGwAaQBjAGsAIABvAG4AIAB0AGgAZQAgAEYAdQBuAGMAdABpAG8AbgAsACAAYQBuAGQAIAB0AGgAZQBuACAAaQBuAHYAbwBrAGUAIABpAHQAIABpAG4AIAB0AGgAZQAKAC8ALwAgAGwAbwB3AGUAcgAgAHIAaQBnAGgAdAAgAHAAYQBuAGUAbAAsACAAbwByACAAYwBsAGkAYwBrACAAbwBuACAAdABoAGUAIABCAHUAYwBrAGUAdAAKAC8ALwAgAHQAbwAgAHMAZQBlACAAaQB0AHMAIABjAG8AbgB0AGUAbgB0AHMAIABpAG4AIAB0AGgAZQAgAHAAYQBuAGUAbAAsACAAZQB0AGMALgAKAC8ALwAgACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAhACEAIQAKAAoALwAvACAAYABpAG4AZgBsAGkAZwBoAHQAcwBgACAAcgBlAHAAcgBlAHMAZQBuAHQAIABjAG8AZABlACAAdABoAGEAdAAgAHIAdQBuAHMAIABsAGEAdABlAHIALAAgAG8AbgAKAC8ALwAgAG8AdABoAGUAcgAgAG0AYQBjAGgAaQBuAGUAcwAsACAAaQBuAHQAZQByAGEAYwB0AGkAbgBnACAAdwBpAHQAaAAgAGMAYQBwAHQAdQByAGUAZAAgAGQAYQB0AGEAIABhAG4AZAAKAC8ALwAgAHIAZQBzAG8AdQByAGMAZQBzACAAZgByAG8AbQAgAHQAaABlACAAcAByAGUALQBmAGwAaQBnAGgAdAAgAHAAaABhAHMAZQAuAAoAbABlAHQAIABoAGUAbABsAG8AXwB3AG8AcgBsAGQAIAA9ACAAaQBuAGYAbABpAGcAaAB0ACAAKAApACAAPQA%2BACAAewAKACAAIABiAHUAYwBrAGUAdAAuAHAAdQB0ACgAIgBoAGUAbABsAG8ALgB0AHgAdAAiACwAIAAiAEgAZQBsAGwAbwAsACAAVwBvAHIAbABkACEAIgApADsACgB9ADsACgAKAC8ALwAgAEkAbgBmAGwAaQBnAGgAdABzACAAYwBhAG4AIABiAGUAIABkAGUAcABsAG8AeQBlAGQAIABhAHMAIABzAGUAcgB2AGUAcgBsAGUAcwBzACAAZgB1AG4AYwB0AGkAbwBuAHMACgBuAGUAdwAgAGMAbABvAHUAZAAuAEYAdQBuAGMAdABpAG8AbgAoAGgAZQBsAGwAbwBfAHcAbwByAGwAZAApADsACgAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAAIAAgACAACgAvAC8AIACRISAAUwB3AGkAdABjAGgAIABmAGkAbABlAHMAIABhAG4AZAAgAHMAZQBlACAAbwB0AGgAZQByACAAZQB4AGEAbQBwAGwAZQBzACAAdwBpAHQAaAAgAG0AbwByAGUACgAvAC8AIABlAHgAcABsAGUAbgBhAHQAaQBvAG4AcwAgAGEAYgBvAHYAZQAuAA%3D%3D)中使用 Wing 查看結構和範例。 如果你比較像輔導員。看這個! https://www.youtube.com/watch?v=wzqCXrsKWbo Wing 在 GitHub 上擁有超過 3500 個 Star,發布了 1500 多個版本,但仍未進入 v1 版本,這意味著意義重大。 去嘗試一下,做一些很酷的事情吧! https://git.new/wing-repo 星翼 ⭐️ --- 開發者生態系統不斷發展,許多開發者圍繞 React 建置了一些獨特的東西。 我不會介紹如何使用 React,因為這是一個非常廣泛的主題,我在最後貼了一些資源來幫助您學習 React。 但為了幫助您建立出色的 React 專案,我們介紹了 25 個開源專案,您可以使用它們來使您的工作更輕鬆。 這將有大量的資源、想法和概念。 我甚至會給你一些學習資源,以及一些產品的專案範例來學習 React。 一切都是免費的,而且只有 React。 讓我們涵蓋這一切! --- 1. [Mantine Hooks](https://www.npmjs.com/package/@mantine/hooks) - 用於狀態和 UI 管理的 React hooks。 -------------------------------------------------------------------------------------------- 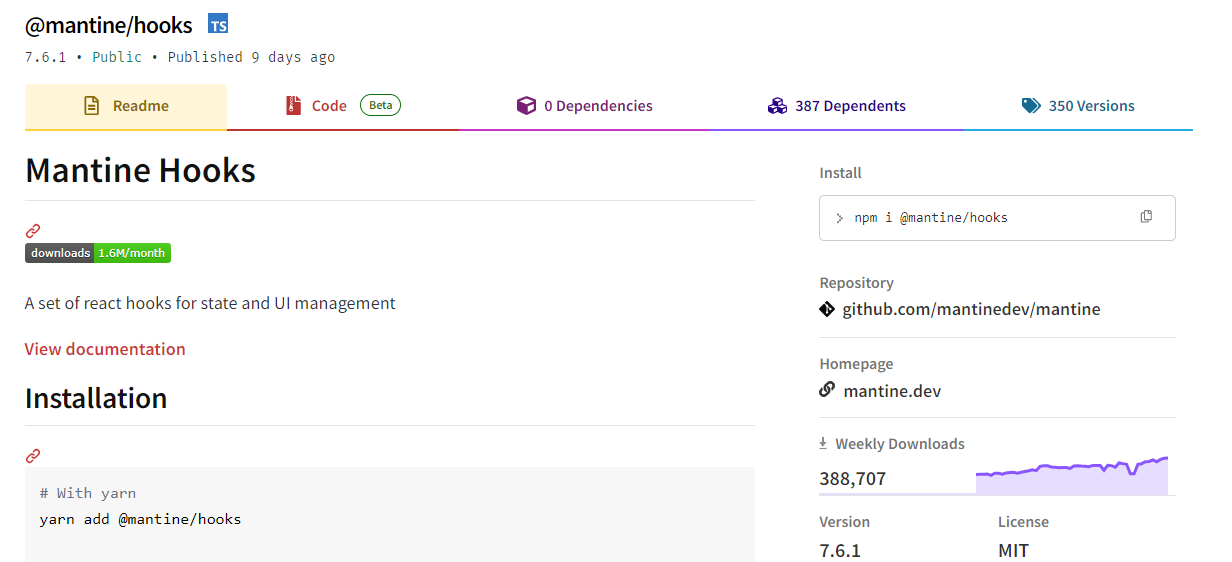 這可能不是專門針對 React 的,但是您可以使用這些鉤子來使您的工作更輕鬆。這些鉤子隨時可用,每個鉤子都有許多選項。 如果我必須評價的話,這將是每個人都可以使用的最有用的專案,而不是從頭開始編寫程式碼。 相信我,獲得 60 多個 Hooks 是一件大事,因為他們有一個簡單的方法讓您可以透過簡單的文件查看每個 Hooks 的演示。 開始使用以下 npm 指令。 ``` npm install @mantine/hooks ``` 這就是如何使用`useScrollIntoView`作為 mantine 掛鉤的一部分。 ``` import { useScrollIntoView } from '@mantine/hooks'; import { Button, Text, Group, Box } from '@mantine/core'; function Demo() { const { scrollIntoView, targetRef } = useScrollIntoView<HTMLDivElement>({ offset: 60, }); return ( <Group justify="center"> <Button onClick={() => scrollIntoView({ alignment: 'center', }) } > Scroll to target </Button> <Box style={{ width: '100%', height: '50vh', backgroundColor: 'var(--mantine-color-blue-light)', }} /> <Text ref={targetRef}>Hello there</Text> </Group> ); } ``` 它們幾乎擁有從本地儲存到分頁、滾動視圖、交叉點,甚至一些非常酷的實用程式(例如滴管和文字選擇)的所有功能。這實在太有幫助了! 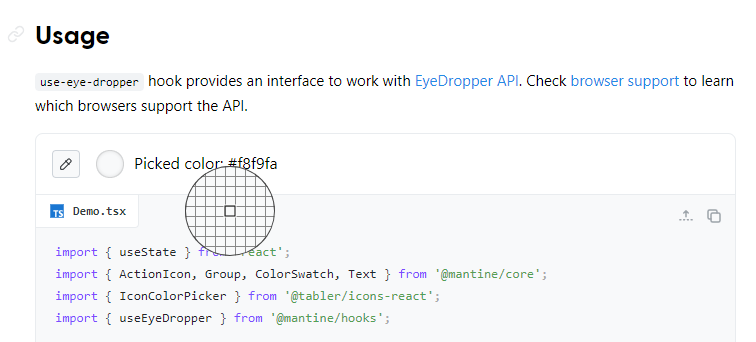 您可以閱讀[文件](https://mantine.dev/hooks/use-click-outside/)。 如果您正在尋找更多選項,您也可以使用[替代庫](https://antonioru.github.io/beautiful-react-hooks/)。 他們在 GitHub 上擁有超過 23k star,但這不僅僅是為了 hooks,因為他們是 React 的元件庫。 隨著`v7`版本的發布,它的每週下載量已超過 38 萬次,這表明它們正在不斷改進且值得信賴。 https://github.com/mantinedev/mantine Star Mantine Hooks ⭐️ --- 2. [React Grid Layout](https://github.com/react-grid-layout/react-grid-layout) - 可拖曳且可調整大小的網格佈局,具有響應式斷點。 -------------------------------------------------------------------------------------------------------- 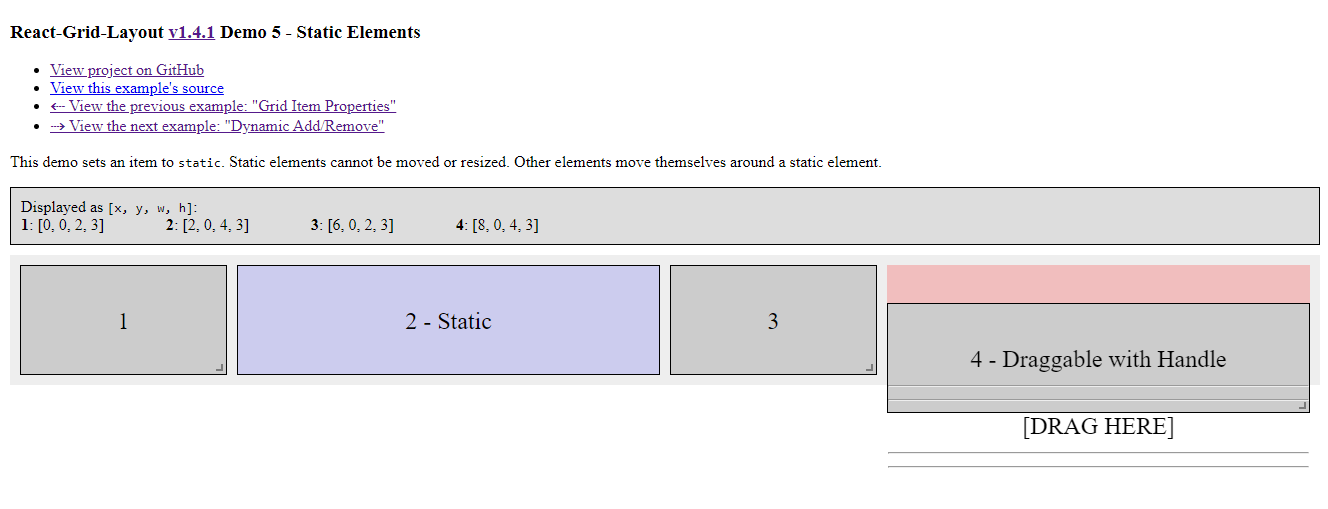 React-Grid-Layout 是專為 React 應用程式建構的響應式網格佈局系統。 透過支援可拖曳、可調整大小和靜態小部件,它提供了使用網格的簡單解決方案。 與 Packery 或 Gridster 等類似系統不同,React-Grid-Layout 不含 jQuery,確保輕量級且高效的實作。 它與伺服器渲染應用程式的無縫整合以及序列化和恢復佈局的能力使其成為開發人員在 React 專案中使用網格佈局的寶貴工具。 開始使用以下 npm 指令。 ``` npm install react-grid-layout ``` 這就是如何使用響應式網格佈局。 ``` import { Responsive as ResponsiveGridLayout } from "react-grid-layout"; class MyResponsiveGrid extends React.Component { render() { // {lg: layout1, md: layout2, ...} const layouts = getLayoutsFromSomewhere(); return ( <ResponsiveGridLayout className="layout" layouts={layouts} breakpoints={{ lg: 1200, md: 996, sm: 768, xs: 480, xxs: 0 }} cols={{ lg: 12, md: 10, sm: 6, xs: 4, xxs: 2 }} > <div key="1">1</div> <div key="2">2</div> <div key="3">3</div> </ResponsiveGridLayout> ); } } ``` 您可以閱讀[文件](https://github.com/react-grid-layout/react-grid-layout?tab=readme-ov-file#installation)並查看[演示](https://react-grid-layout.github.io/react-grid-layout/examples/0-showcase.html)。有一系列[演示](https://github.com/react-grid-layout/react-grid-layout?tab=readme-ov-file#demos),甚至可以透過點擊“查看下一個範例”來獲得。 您也可以嘗試[codesandbox](https://codesandbox.io/p/devbox/github/gilbarbara/react-joyride-demo/tree/main/?embed=1)上的東西。 該專案在 GitHub 上有超過 19k+ 的星星,有超過 16k+ 的開發者使用,並且[npm 套件](https://www.npmjs.com/package/react-grid-layout)的每週下載量超過 600k+。 https://github.com/react-grid-layout/react-grid-layout 明星 React 網格佈局 ⭐️ --- 3. [React Spectrum](https://github.com/adobe/react-spectrum) - 提供出色使用者體驗的程式庫和工具的集合。 ----------------------------------------------------------------------------------- 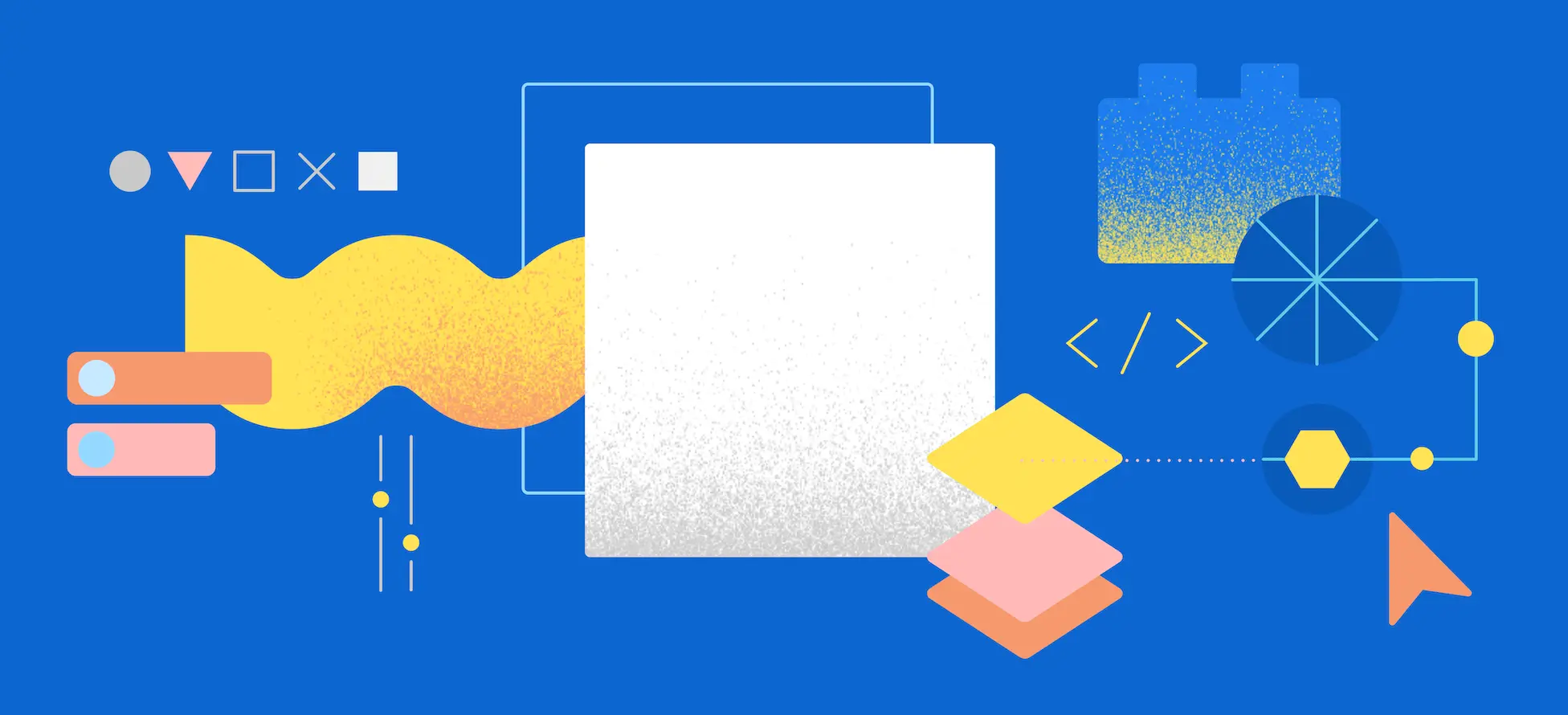 React Spectrum 是一個庫和工具的集合,可幫助您建立自適應、可存取且強大的使用者體驗。 它們提供了太多的東西,以至於很難在一篇文章中涵蓋所有內容。 總的來說,他們提供了這四個庫。 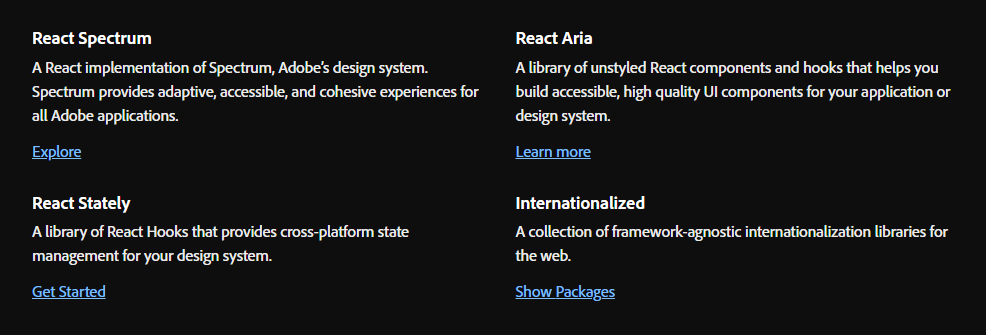 - [反應譜](https://react-spectrum.adobe.com/react-spectrum/index.html) - [React Stately](https://react-spectrum.adobe.com/react-stately/index.html) - 一組龐大的 React Hooks,為您的設計系統提供跨平台狀態管理。 - [反應詠嘆調](https://react-spectrum.adobe.com/react-aria/index.html) - [國際化](https://react-spectrum.adobe.com/internationalized/index.html) 我們將了解一些有關 React Aria 的內容,它是一個無樣式 React 元件和鉤子庫,可幫助您為應用程式建立可存取的、高品質的 UI 元件。 它經過了各種設備、互動方式和輔助技術的精心測試,以確保為所有用戶提供最佳體驗。 開始使用以下 npm 指令。 ``` npm i react-aria-components ``` 這就是建立自訂`select`的方法。 ``` import {Button, Label, ListBox, ListBoxItem, Popover, Select, SelectValue} from 'react-aria-components'; <Select> <Label>Favorite Animal</Label> <Button> <SelectValue /> <span aria-hidden="true">▼</span> </Button> <Popover> <ListBox> <ListBoxItem>Cat</ListBoxItem> <ListBoxItem>Dog</ListBoxItem> <ListBoxItem>Kangaroo</ListBoxItem> </ListBox> </Popover> </Select> ``` 相信我,出於學習目的,這是一座金礦。 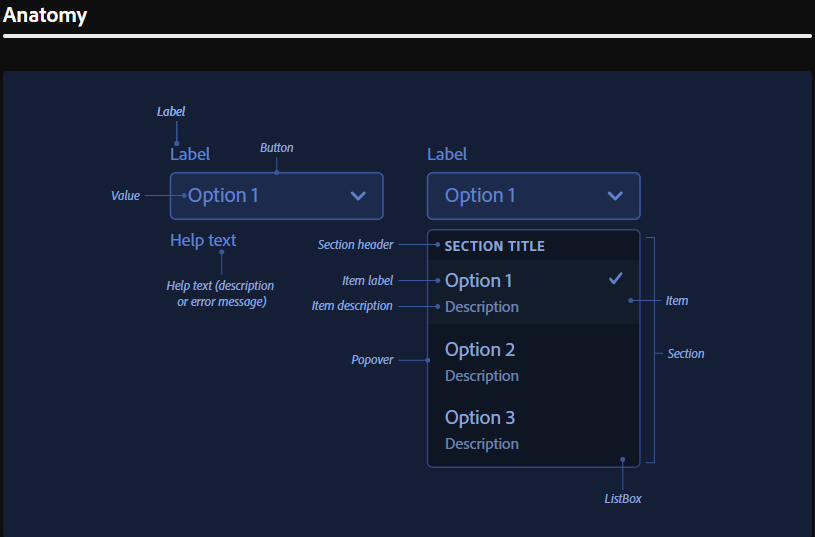 他們使用自己強大的[40 多個樣式元件](https://opensource.adobe.com/spectrum-css/),這比通常提供的要多得多。他們也有自己的一套[設計系統,](https://spectrum.adobe.com/)例如字體、UI、版面、動作等等。 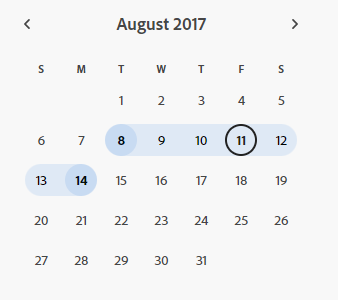 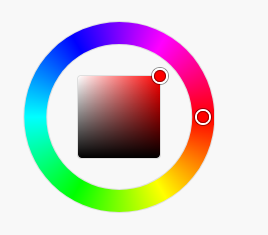 您可以詳細了解[Spectrum](https://react-spectrum.adobe.com/index.html)及其[架構](https://react-spectrum.adobe.com/architecture.html)。 他們在 GitHub 上擁有超過 11,000 顆星,這表明了他們的質量,儘管他們並不廣為人知。研究它們可以為您建立圖書館提供寶貴的見解。 https://github.com/adobe/react-spectrum Star React Spectrum ⭐️ --- 4.[保留 React](https://github.com/StaticMania/keep-react) - Tailwind CSS 和 React.js 的 UI 元件庫。 ------------------------------------------------------------------------------------------- 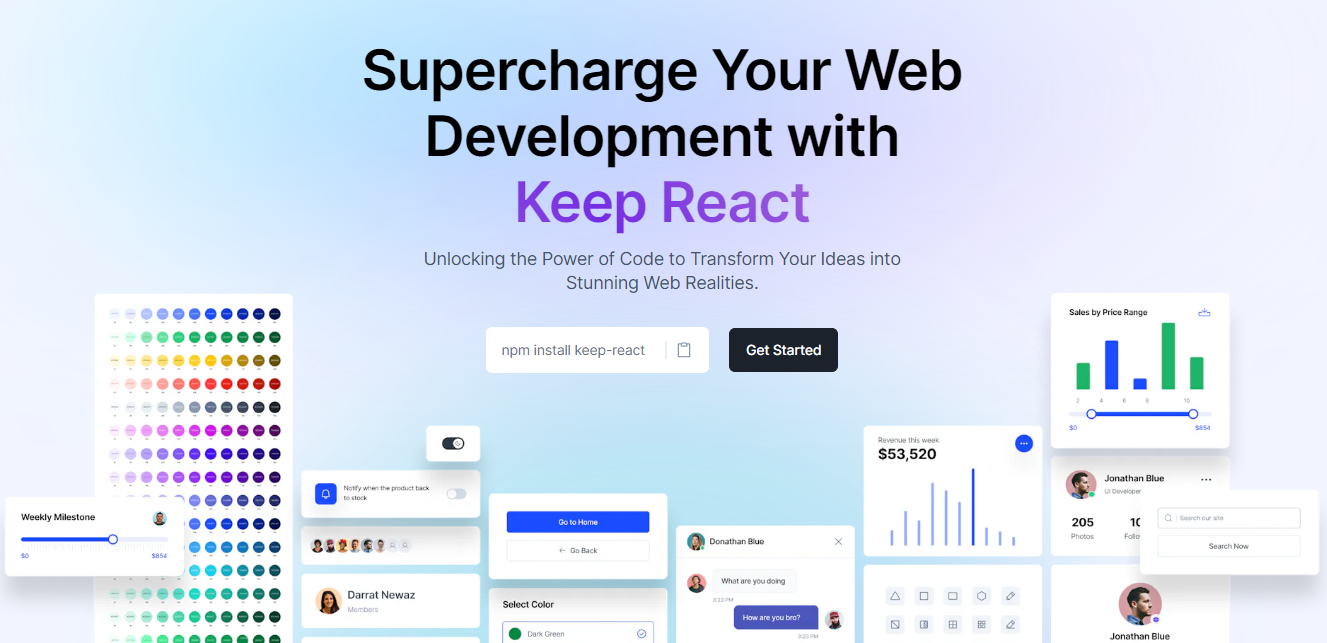 Keep React 是一個基於 Tailwind CSS 和 React.js 建立的開源元件庫。它提供了一組多功能的預先設計的 UI 元件,使開發人員能夠簡化現代、響應式且具有視覺吸引力的 Web 應用程式的建立。 開始使用以下 npm 指令。 ``` npm i keep-react ``` 這就是使用時間軸的方法。 ``` "use client"; import { Timeline } from "keep-react"; import { CalendarBlank } from "phosphor-react"; export const TimelineComponent = () => { return ( <Timeline horizontal={true}> <Timeline.Item> <Timeline.Point icon={<CalendarBlank size={16} />} /> <Timeline.Content> <Timeline.Title>Keep Library v1.0.0</Timeline.Title> <Timeline.Time>Released on December 2, 2021</Timeline.Time> <Timeline.Body> Get started with dozens of web components and interactive elements. </Timeline.Body> </Timeline.Content> </Timeline.Item> <Timeline.Item> <Timeline.Point icon={<CalendarBlank size={16} />} /> <Timeline.Content> <Timeline.Title>Keep Library v1.1.0</Timeline.Title> <Timeline.Time>Released on December 23, 2021</Timeline.Time> <Timeline.Body> Get started with dozens of web components and interactive elements. </Timeline.Body> </Timeline.Content> </Timeline.Item> <Timeline.Item> <Timeline.Point icon={<CalendarBlank size={16} />} /> <Timeline.Content> <Timeline.Title>Keep Library v1.3.0</Timeline.Title> <Timeline.Time>Released on January 5, 2022</Timeline.Time> <Timeline.Body> Get started with dozens of web components and interactive elements. </Timeline.Body> </Timeline.Content> </Timeline.Item> </Timeline> ); } ``` 輸出如下。 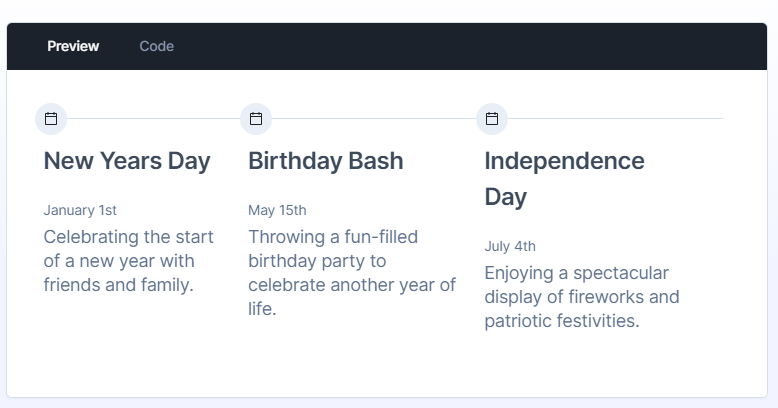 流暢的小動畫讓這一切都是值得的,如果你想快速建立一個 UI,沒有任何麻煩,你可以使用它。 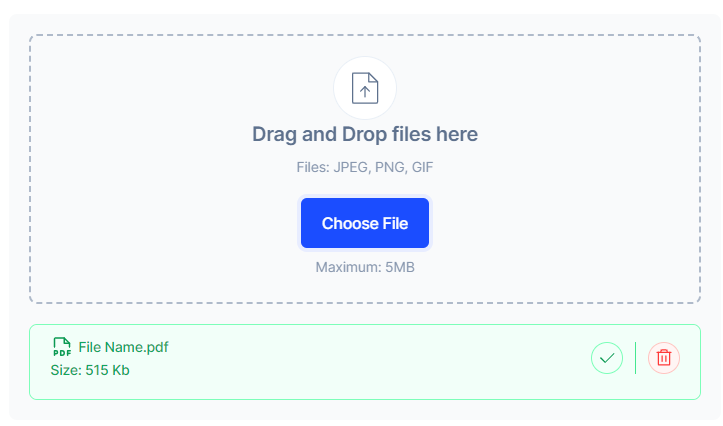 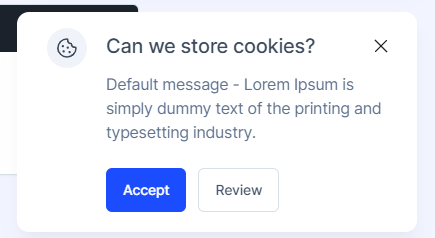 您可以閱讀[文件](https://react.keepdesign.io/docs/getting-started/Introduction)並查看[故事書](https://react-storybook.keepdesign.io/?path=/docs/components-accordion--docs)以進行詳細的使用測驗。 該專案在 GitHub 上有超過 1000 顆星,而且它的一些元件使用起來非常方便。 https://github.com/StaticMania/keep-react Star Keep React ⭐️ --- 5. [React Content Loader](https://github.com/danilowoz/react-content-loader) - SVG 支援的元件,可輕鬆建立骨架載入。 --------------------------------------------------------------------------------------------------- 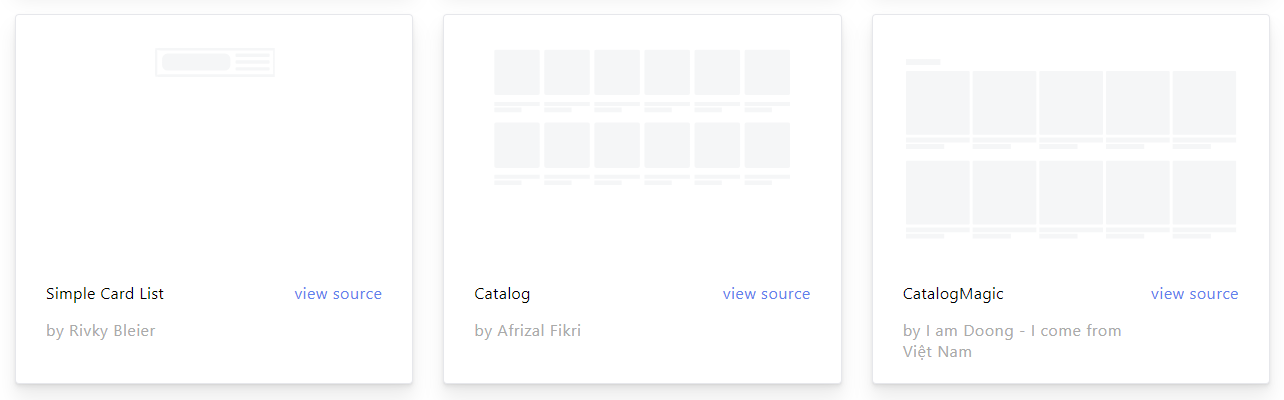 該專案為您提供了一個由 SVG 驅動的元件,可以輕鬆建立佔位符載入(如 Facebook 的卡片載入)。 在載入狀態期間使用骨架來向使用者指示內容仍在載入。 總的來說,這是一個非常方便的專案,可以增強整體使用者體驗。 開始使用以下 npm 指令。 ``` npm i react-content-loader --save ``` 您可以這樣使用它。 ``` import React from "react" import ContentLoader from "react-content-loader" const MyLoader = (props) => ( <ContentLoader speed={2} width={400} height={160} viewBox="0 0 400 160" backgroundColor="#f3f3f3" foregroundColor="#ecebeb" {...props} > <rect x="48" y="8" rx="3" ry="3" width="88" height="6" /> <rect x="48" y="26" rx="3" ry="3" width="52" height="6" /> <rect x="0" y="56" rx="3" ry="3" width="410" height="6" /> <rect x="0" y="72" rx="3" ry="3" width="380" height="6" /> <rect x="0" y="88" rx="3" ry="3" width="178" height="6" /> <circle cx="20" cy="20" r="20" /> </ContentLoader> ) export default MyLoader ``` 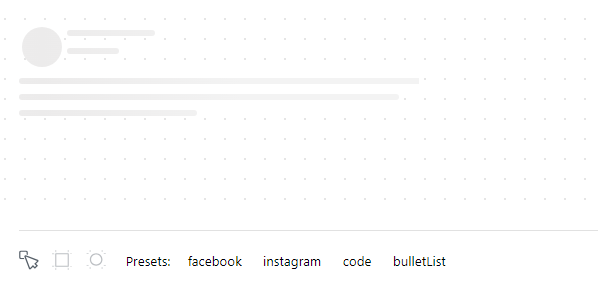 您甚至可以拖曳單一骨架或使用為 Facebook 和 Instagram 等不同社群媒體預先定義的骨架。 您可以閱讀[文件](https://github.com/danilowoz/react-content-loader?tab=readme-ov-file#gettingstarted)並查看[演示](https://skeletonreact.com/)。 該專案在 GitHub 上擁有 13k+ Stars,並在 GitHub 上有 45k+ 開發人員使用。 https://github.com/danilowoz/react-content-loader Star React 內容載入器 ⭐️ --- 6. [React PDF](https://github.com/diegomura/react-pdf) - 使用 React 建立 PDF 檔案。 ---------------------------------------------------------------------------- 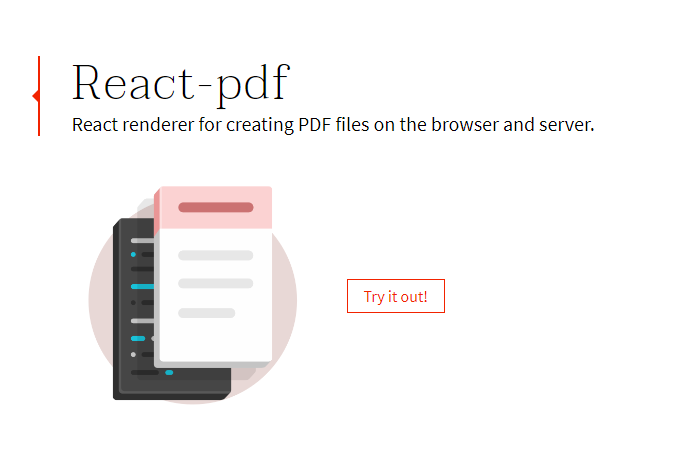 該套件用於使用 React 建立 PDF。 開始使用以下 npm 指令。 ``` npm install @react-pdf/renderer --save ``` 您可以這樣使用它。 ``` import React from 'react'; import { Document, Page, Text, View, StyleSheet } from '@react-pdf/renderer'; // Create styles const styles = StyleSheet.create({ page: { flexDirection: 'row', backgroundColor: '#E4E4E4', }, section: { margin: 10, padding: 10, flexGrow: 1, }, }); // Create Document Component const MyDocument = () => ( <Document> <Page size="A4" style={styles.page}> <View style={styles.section}> <Text>Section #1</Text> </View> <View style={styles.section}> <Text>Section #2</Text> </View> </Page> </Document> ); ``` 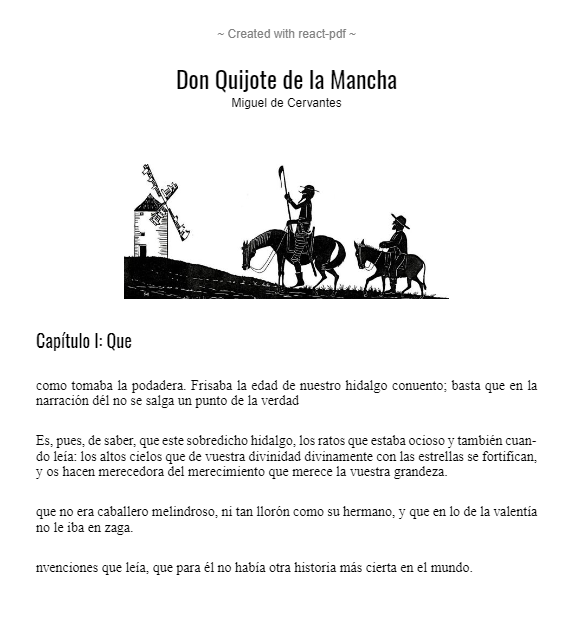 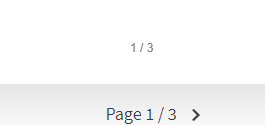 您可以閱讀[文件](https://react-pdf.org/)並查看[演示](https://react-pdf.org/repl)。 React-pdf 現在提供了一個名為`usePDF`的鉤子,可以透過 React hook API 存取所有 PDF 建立功能。如果您需要更多控製文件的呈現方式或更新頻率,這非常有用。 ``` const [instance, update] = usePDF({ document }); ``` 該專案在 GitHub 上有 13k+ Stars,有超過 270 個版本,[每週下載量超過 400k](https://www.npmjs.com/package/@react-pdf/renderer) ,這是一個好兆頭。 https://github.com/diegomura/react-pdf Star React PDF ⭐️ --- 7. [Recharts](https://github.com/recharts/recharts) - 使用 React 和 D3 建立的重新定義的圖表庫。 -------------------------------------------------------------------------------- 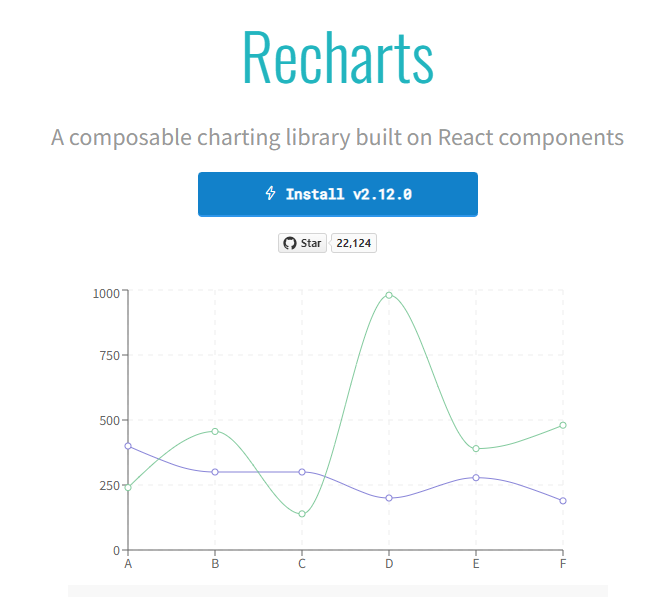 該庫的主要目的是幫助您輕鬆地在 React 應用程式中編寫圖表。 Recharts 的主要原則是。 1. 只需使用 React 元件進行部署即可。 2. 原生 SVG 支持,輕量級,僅依賴一些 D3 子模組。 3. 聲明性元件、圖表元件純粹是表示性的。 開始使用以下 npm 指令。 ``` npm install recharts ``` 您可以這樣使用它。 ``` <LineChart width={500} height={300} data={data} accessibilityLayer> <XAxis dataKey="name"/> <YAxis/> <CartesianGrid stroke="#eee" strokeDasharray="5 5"/> <Line type="monotone" dataKey="uv" stroke="#8884d8" /> <Line type="monotone" dataKey="pv" stroke="#82ca9d" /> <Tooltip/> </LineChart> ``` 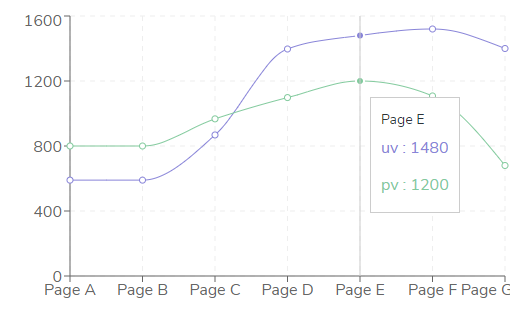 您可以閱讀[文件](https://recharts.org/en-US/guide)並查看有關[Storybook](https://recharts.org/en-US/storybook)的更多資訊。 他們提供了大量的選項來自訂它,這就是開發人員喜歡它的原因。他們也提供一般常見問題的[wiki](https://github.com/recharts/recharts/wiki)頁面。 您也可以在此處的codesandbox 上嘗試。 https://codesandbox.io/embed/kec3v?view=Editor+%2B+Preview&module=%2Fsrc%2Findex.tsx 該專案在 GitHub 上有 22k+ Stars,有 200k+ 開發人員使用。 https://github.com/recharts/recharts 明星 Recharts ⭐️ --- 8. [React Joyride](https://github.com/gilbarbara/react-joyride) - 在您的應用程式中建立導遊。 ------------------------------------------------------------------------------- 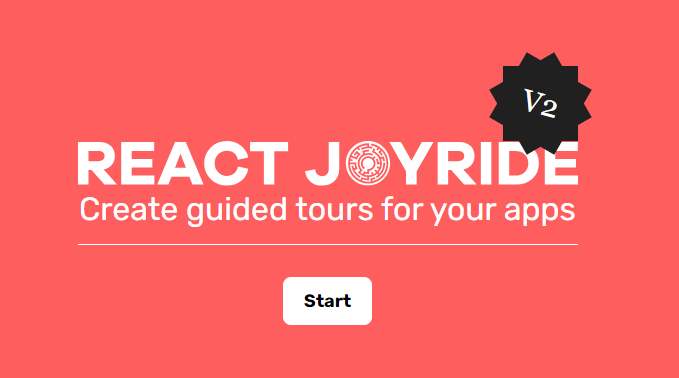 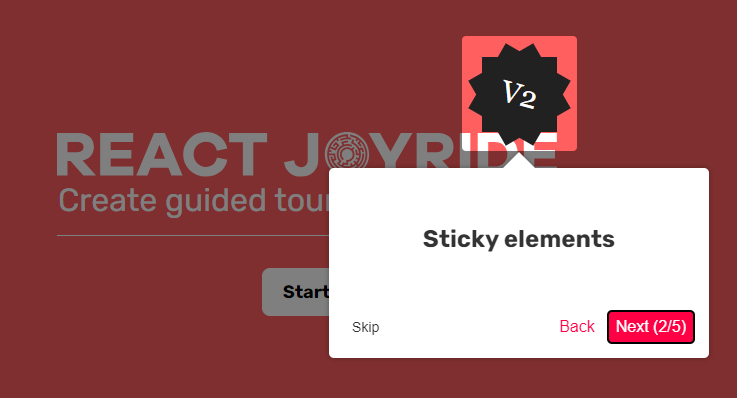 導覽是向新用戶展示您的應用程式或解釋新功能的絕佳方式。它改善了用戶體驗並可以創造個人化的觸感。 開始使用以下 npm 指令。 ``` npm i react-joyride ``` 您可以這樣使用它。 ``` import React, { useState } from 'react'; import Joyride from 'react-joyride'; /* * If your steps are not dynamic you can use a simple array. * Otherwise you can set it as a state inside your component. */ const steps = [ { target: '.my-first-step', content: 'This is my awesome feature!', }, { target: '.my-other-step', content: 'This another awesome feature!', }, ]; export default function App() { // If you want to delay the tour initialization you can use the `run` prop return ( <div> <Joyride steps={steps} /> ... </div> ); } ``` 它們還提供[元件列表](https://docs.react-joyride.com/custom-components)以及自訂預設用戶介面的簡單方法。 您可以閱讀[文件](https://docs.react-joyride.com/)並查看[演示](https://react-joyride.com/)。 您也可以嘗試[codesandbox](https://codesandbox.io/p/devbox/github/gilbarbara/react-joyride-demo/tree/main/?embed=1)上的東西。 他們在 GitHub 上有超過 6k 顆星,npm 套件每週下載量超過 25 萬次。 https://github.com/gilbarbara/react-joyride Star React Joyride ⭐️ --- 9. [SVGR](https://github.com/gregberge/svgr) - 將 SVG 轉換為 React 元件。 ------------------------------------------------------------------ 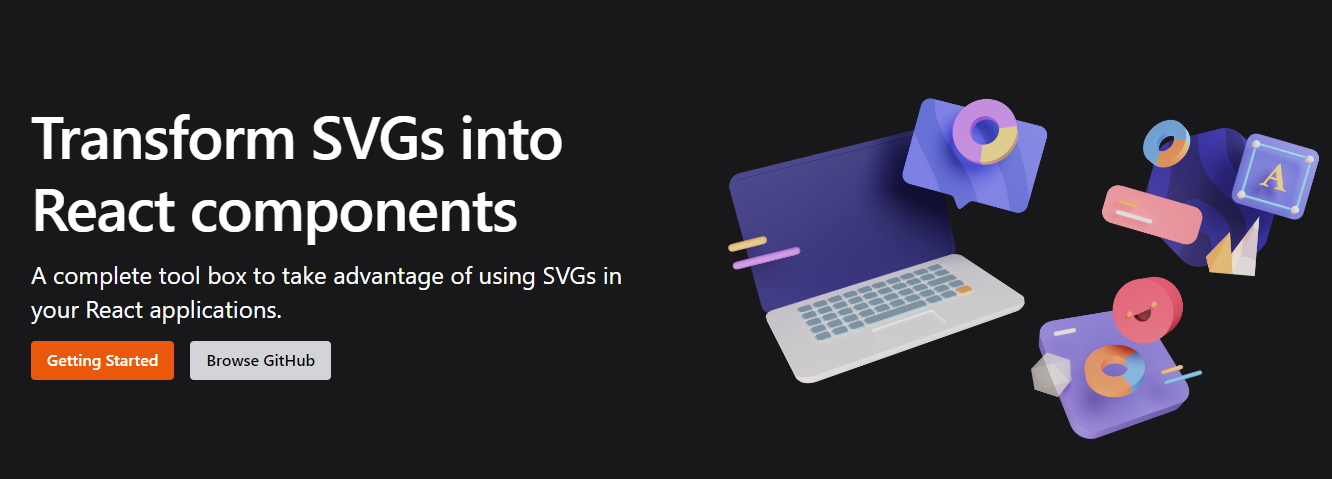 SVGR 是一個將 SVG 轉換為 React 元件的通用工具。 它需要一個原始的 SVG 並將其轉換為隨時可用的 React 元件。 開始使用以下 npm 指令。 ``` npm install @svgr/core ``` 例如,您採用這個 SVG。 ``` <?xml version="1.0" encoding="UTF-8"?> <svg width="48px" height="1px" viewBox="0 0 48 1" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" > <!-- Generator: Sketch 46.2 (44496) - http://www.bohemiancoding.com/sketch --> <title>Rectangle 5</title> <desc>Created with Sketch.</desc> <defs></defs> <g id="Page-1" stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g id="19-Separator" transform="translate(-129.000000, -156.000000)" fill="#063855" > <g id="Controls/Settings" transform="translate(80.000000, 0.000000)"> <g id="Content" transform="translate(0.000000, 64.000000)"> <g id="Group" transform="translate(24.000000, 56.000000)"> <g id="Group-2"> <rect id="Rectangle-5" x="25" y="36" width="48" height="1"></rect> </g> </g> </g> </g> </g> </g> </svg> ``` 執行SVGR後,將轉換為. ``` import * as React from 'react' const SvgComponent = (props) => ( <svg width="1em" height="1em" viewBox="0 0 48 1" {...props}> <path d="M0 0h48v1H0z" fill="currentColor" fillRule="evenodd" /> </svg> ) export default SvgComponent ``` 它使用[SVGO](https://github.com/svg/svgo)優化 SVG,並使用 Prettier 格式化程式碼。 將 HTML 轉換為 JSX 需要幾個步驟: 1. 將 SVG 轉換為 HAST (HTML AST) 2. 將 HAST 轉換為 Babel AST (JSX AST) 3. 使用 Babel 轉換 AST(重新命名屬性、更改屬性值…) 您可以在[Playground](https://react-svgr.com/playground/)閱讀[文件](https://react-svgr.com/docs/getting-started)並檢查內容。 該專案在 GitHub 上擁有 10k+ Stars,有超過 800 萬開發者使用,npm 上每週下載量超過 800k。 https://github.com/gregberge/svgr 明星 SVGR ⭐️ --- 10. [React Sortable Tree](https://github.com/frontend-collective/react-sortable-tree) - 用於巢狀資料和層次結構的拖放可排序元件。 ------------------------------------------------------------------------------------------------------------ 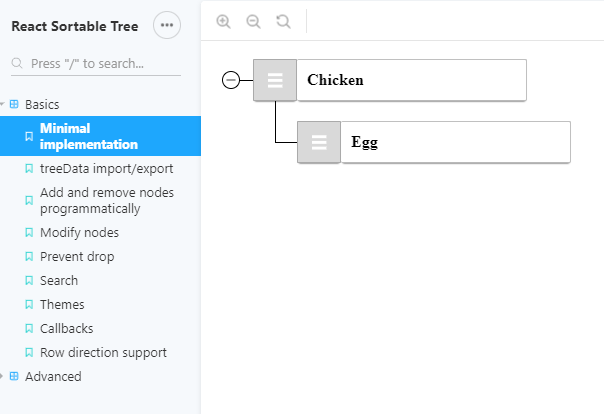 一個 React 元件,支援對分層資料進行拖放排序。 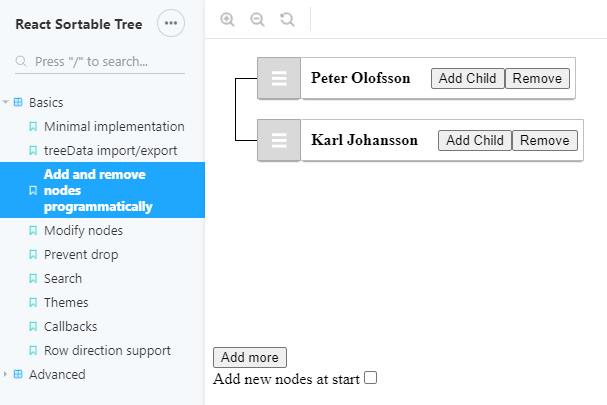 開始使用以下 npm 指令。 ``` npm install react-sortable-tree --save ``` 您可以這樣使用它。 ``` import React, { Component } from 'react'; import SortableTree from 'react-sortable-tree'; import 'react-sortable-tree/style.css'; // This only needs to be imported once in your app export default class Tree extends Component { constructor(props) { super(props); this.state = { treeData: [ { title: 'Chicken', children: [{ title: 'Egg' }] }, { title: 'Fish', children: [{ title: 'fingerline' }] }, ], }; } render() { return ( <div style={{ height: 400 }}> <SortableTree treeData={this.state.treeData} onChange={treeData => this.setState({ treeData })} /> </div> ); } } ``` 檢查由此獲得的各種[道具選項](https://github.com/frontend-collective/react-sortable-tree?tab=readme-ov-file#props)和[主題](https://github.com/frontend-collective/react-sortable-tree?tab=readme-ov-file#featured-themes)。 您可以閱讀[文件](https://github.com/frontend-collective/react-sortable-tree?tab=readme-ov-file#getting-started)並查看[Storybook](https://frontend-collective.github.io/react-sortable-tree/?path=/story/basics--minimal-implementation) ,以獲取一些基本和高級功能的演示。 它可能不會被積極維護(仍然沒有存檔),因此您也可以使用[維護的 fork 版本](https://github.com/nosferatu500/react-sortable-tree)。 該專案在 GitHub 上擁有超過 4,500 個 Star,並被超過 5,000 名開發人員使用。 https://github.com/frontend-collective/react-sortable-tree Star React 可排序樹 ⭐️ --- 11. [React Hot Toast](https://github.com/timolins/react-hot-toast) - 冒煙的 Hot React 通知。 -------------------------------------------------------------------------------------- 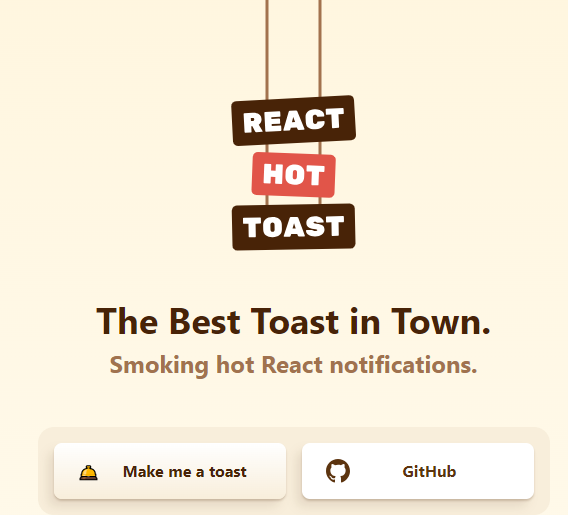 React Hot Toast 透過簡單的自訂選項提供了驚人的 🔥 預設體驗。它利用 Promise API 進行自動加載,確保平穩過渡。 它重量輕,不到 5kb,但仍然可以存取,同時為開發人員提供了像`useToaster()`這樣的無頭鉤子。 首先將 Toaster 加入到您的應用程式中。它將負責渲染發出的所有通知。現在您可以從任何地方觸發 toast() ! 開始使用以下 npm 指令。 ``` npm install react-hot-toast ``` 這就是它的易用性。 ``` import toast, { Toaster } from 'react-hot-toast'; const notify = () => toast('Here is your toast.'); const App = () => { return ( <div> <button onClick={notify}>Make me a toast</button> <Toaster /> </div> ); }; ``` 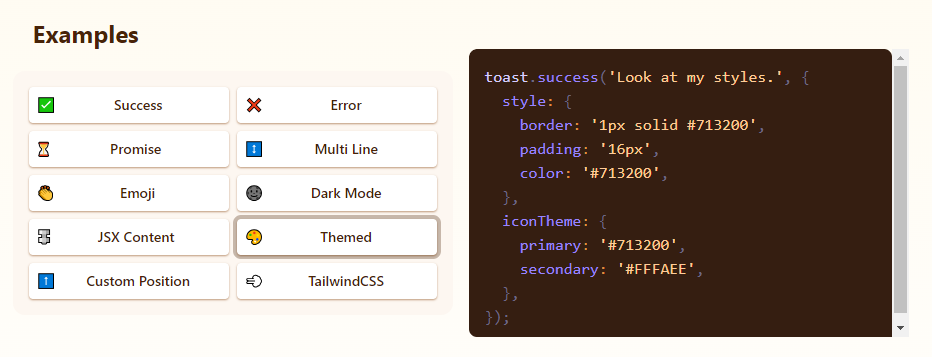 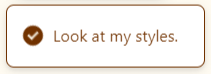 他們有很多自訂選項,但`useToaster()`掛鉤為您提供了一個無頭系統,可以為您管理通知狀態。這使得建立您的通知系統變得更加容易。 您可以閱讀[文件](https://react-hot-toast.com/docs)、[樣式指南](https://react-hot-toast.com/docs/styling)並查看[示範](https://react-hot-toast.com/)。 該專案在 GitHub 上有 8k+ Stars,有 230k+ 開發者使用。 https://github.com/timolins/react-hot-toast Star React Hot Toast ⭐️ --- 12. [Payload](https://github.com/payloadcms/payload) - 建立現代後端+管理 UI 的最佳方式。 -------------------------------------------------------------------------- 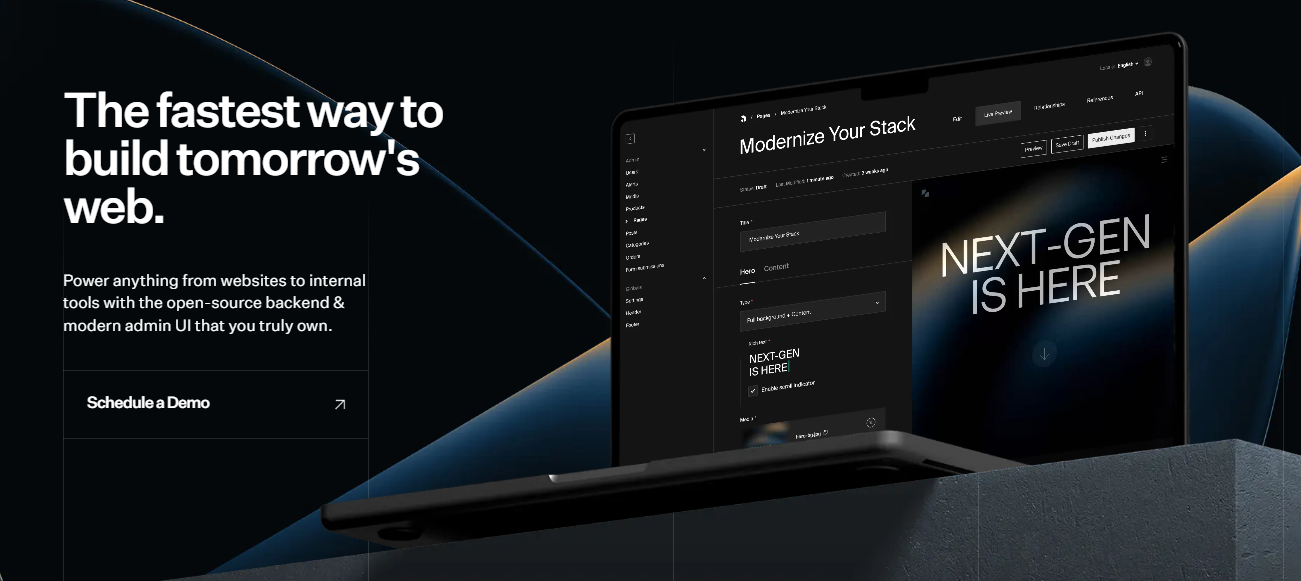 Payload 是一個無頭 CMS 和應用程式框架。它旨在促進您的開發過程,但重要的是,當您的應用程式變得更加複雜時,不要妨礙您。 Payload 沒有黑魔法,完全開源,它既是一個應用程式框架,也是一個無頭 CMS。它確實是適用於 TypeScript 的 Rails,並且您會獲得一個管理面板。您可以使用此[YouTube 影片](https://www.youtube.com/watch?v=In_lFhzmbME)了解有關 Payload 的更多資訊。 https://www.youtube.com/watch?v=In\_lFhzmbME 您可以透過使用Payload來了解[其中涉及的概念](https://payloadcms.com/docs/getting-started/concepts)。 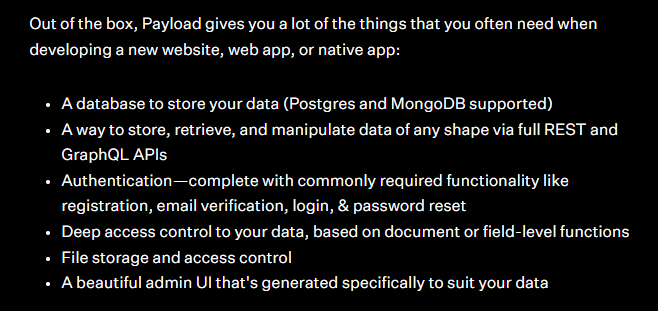 有效負載透過您選擇的資料庫適配器與您的資料庫進行互動。目前,Payload 正式支援兩種資料庫適配器: 1. MongoDB 與 Mongoose 2. Postgres 帶毛毛雨 開始使用以下命令。 ``` npx create-payload-app@latest ``` 您必須產生 Payload 金鑰並更新`server.ts`以初始化 Payload。 ``` import express from 'express' import payload from 'payload' require('dotenv').config() const app = express() const start = async () => { await payload.init({ secret: process.env.PAYLOAD_SECRET, express: app, }) app.listen(3000, async () => { console.log( "Express is now listening for incoming connections on port 3000." ) }) } start() ``` 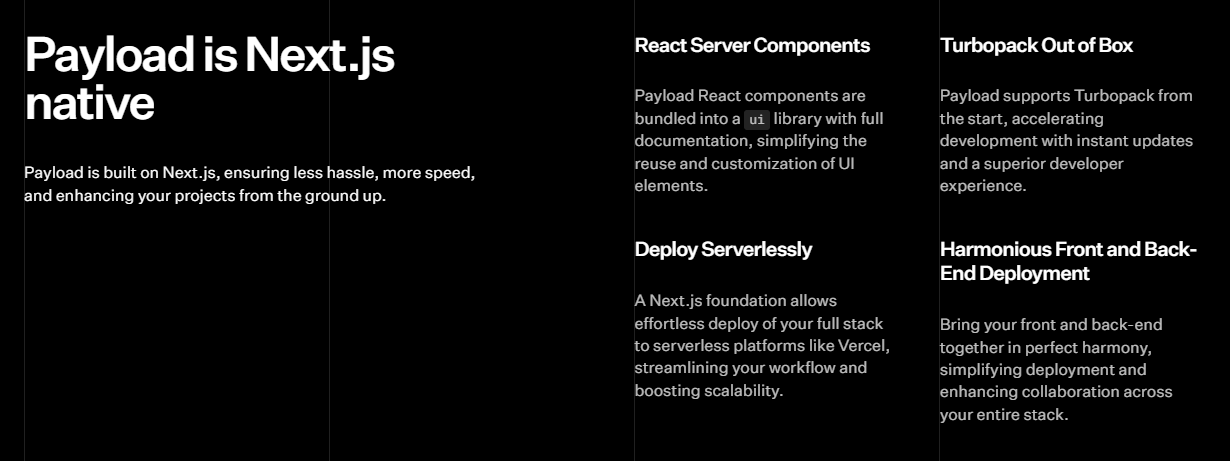 您可以閱讀[文件](https://payloadcms.com/docs/getting-started/what-is-payload)並查看[演示](https://demo.payloadcms.com/?_gl=1*9x0za3*_ga*NzEzMzkwNzIuMTcxMDE2NDk1MA..*_ga_FLQ5THRMZQ*MTcxMDE2NDk1MC4xLjEuMTcxMDE2NDk1MS4wLjAuMA..)。 他們還提供與 Payload + Stripe 無縫整合的[電子商務模板](https://github.com/payloadcms/payload/tree/main/templates/ecommerce)。此範本具有令人驚嘆的、功能齊全的前端,包括購物車、結帳流程、訂單管理等元件。 Payload 在 GitHub 上擁有 18k+ Stars,並且有超過 290 個版本,因此它們不斷改進,尤其是在資料庫支援方面。 https://github.com/payloadcms/payload 明星有效負載 ⭐️ --- 13. [React Player](https://github.com/cookpete/react-player) - 用於播放各種 URL 的 React 元件。 ------------------------------------------------------------------------------------- 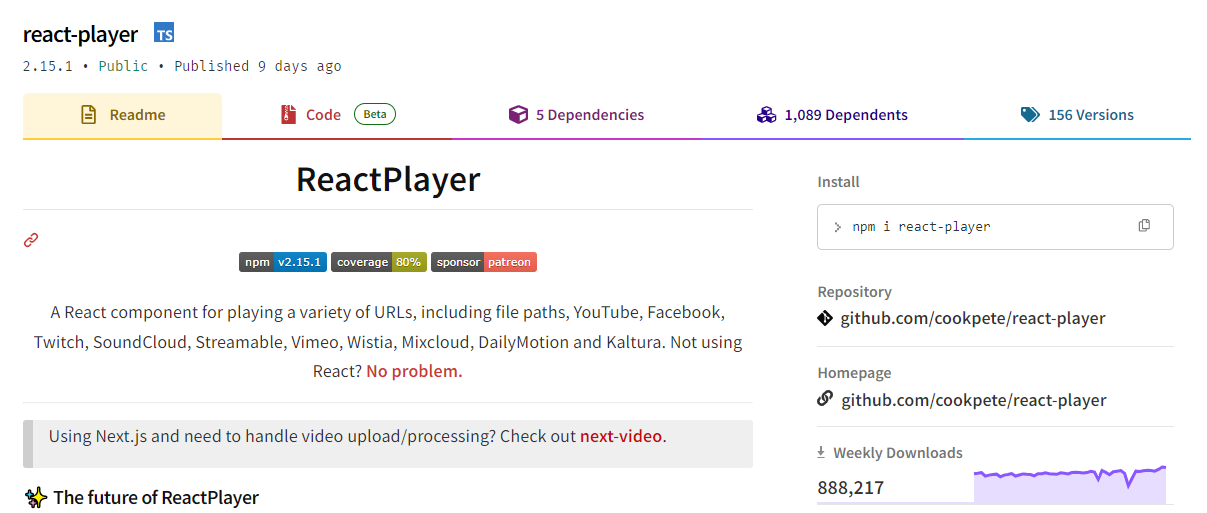 用於播放各種 URL 的 React 元件,包括檔案路徑、YouTube、Facebook、Twitch、SoundCloud、Streamable、Vimeo、Wistia、Mixcloud、DailyMotion 和 Kaltura。您可以看到[支援的媒體](https://github.com/cookpete/react-player?tab=readme-ov-file#supported-media)清單。 ReactPlayer 的維護工作由 Mux 接管,這使它們成為一個不錯的選擇。 開始使用以下 npm 指令。 ``` npm install react-player ``` 您可以這樣使用它。 ``` import React from 'react' import ReactPlayer from 'react-player' // Render a YouTube video player <ReactPlayer url='https://www.youtube.com/watch?v=LXb3EKWsInQ' /> // If you only ever use one type, use imports such as react-player/youtube to reduce your bundle size. // like this: import ReactPlayer from 'react-player/youtube' ``` 您也可以使用`react-player/lazy`為您傳入的URL 延遲載入適當的播放器。這會為您的輸出加入幾個reactPlayer 區塊,但會減少主包的大小。 ``` import React from 'react' import ReactPlayer from 'react-player/lazy' // Lazy load the YouTube player <ReactPlayer url='https://www.youtube.com/watch?v=ysz5S6PUM-U' /> ``` 您可以閱讀[文件](https://github.com/cookpete/react-player?tab=readme-ov-file#props)並查看[演示](https://cookpete.github.io/react-player/)。他們提供了大量的選項,包括加入字幕並以簡單的方式使其響應。 它們在 GitHub 上擁有超過 8000 顆星,被超過 135,000 名開發人員使用,並且 npm 軟體包[每週的下載量超過 800k](https://www.npmjs.com/package/react-player) 。 https://github.com/cookpete/react-player 明星 React 播放器 ⭐️ --- 14. [Victory](https://github.com/FormidableLabs/victory) - 用於建立互動式資料視覺化的 React 元件。 ---------------------------------------------------------------------------------- 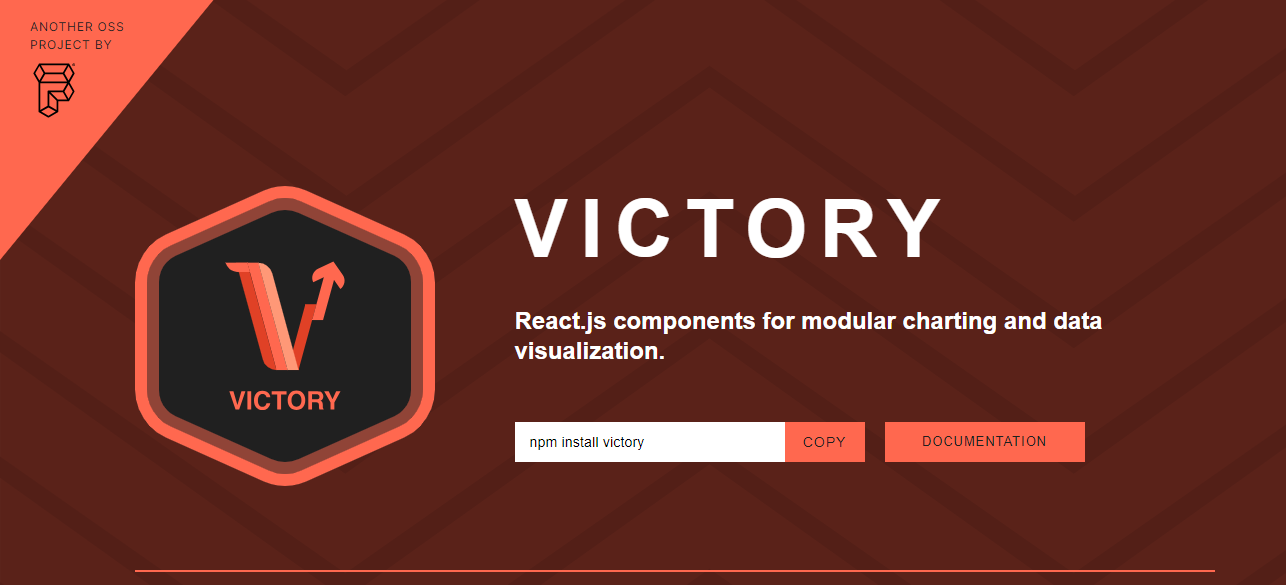 Victory 是一個可組合 React 元件的生態系統,用於建立互動式資料視覺化。 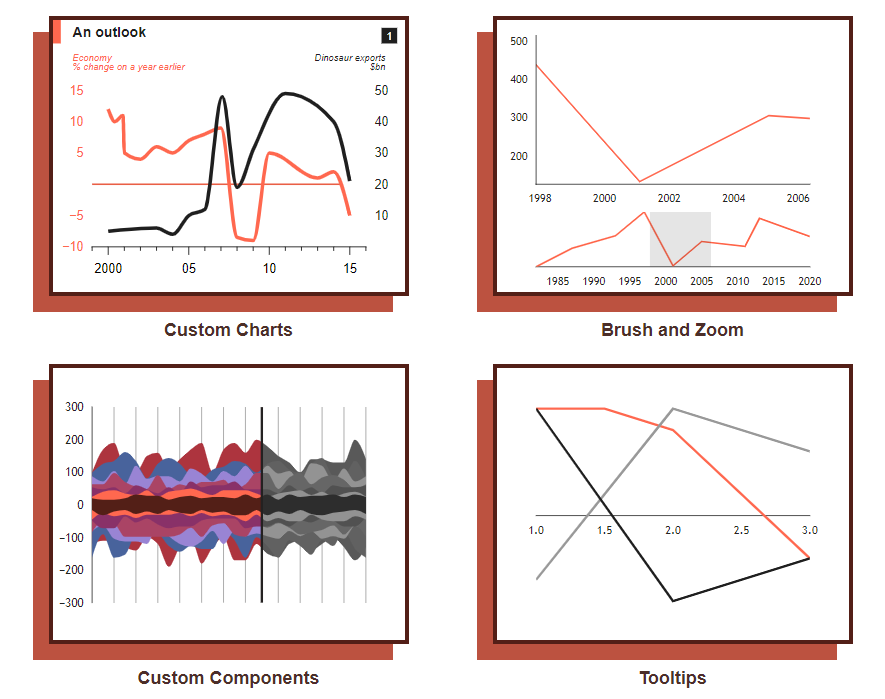 開始使用以下 npm 指令。 ``` npm i --save victory ``` 您可以這樣使用它。 ``` <VictoryChart domainPadding={{ x: 20 }} > <VictoryHistogram style={{ data: { fill: "#c43a31" } }} data={sampleHistogramDateData} bins={[ new Date(2020, 1, 1), new Date(2020, 4, 1), new Date(2020, 8, 1), new Date(2020, 11, 1) ]} /> </VictoryChart> ``` 這就是它的渲染方式。他們還提供通常有用的動畫和主題選項。 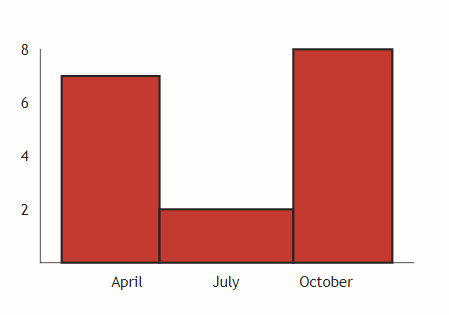 您可以閱讀[文件](https://commerce.nearform.com/open-source/victory/docs)並按照[教學](https://commerce.nearform.com/open-source/victory/docs/native)開始。他們提供大約 15 種不同的圖表選項。 它也可用於[React Native(文件)](https://commerce.nearform.com/open-source/victory/docs/native) ,所以這是一個優點。我還建議您查看他們的常見[問題解答](https://commerce.nearform.com/open-source/victory/docs/faq#frequently-asked-questions-faq),其中描述了常見問題的程式碼解決方案和解釋,例如樣式、註釋(標籤)、處理軸。 該專案在 GitHub 上擁有 10k+ Stars,並在 GitHub 上有 23k+ 開發人員使用。 https://github.com/FormidableLabs/victory 勝利之星 ⭐️ --- 15. [React Slick](https://github.com/akiran/react-slick) - React 輪播元件。 ---------------------------------------------------------------------- 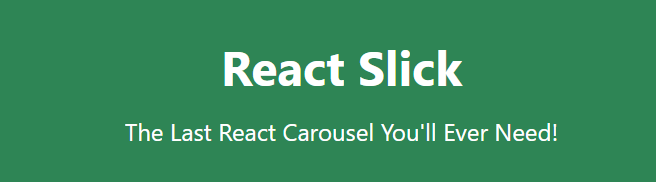 React Slick 是一個使用 React 建構的輪播元件。它是一個光滑的旋轉木馬的反應端口 開始使用以下 npm 指令。 ``` npm install react-slick --save ``` 這是使用自訂分頁的方法。 ``` import React, { Component } from "react"; import Slider from "react-slick"; import { baseUrl } from "./config"; function CustomPaging() { const settings = { customPaging: function(i) { return ( <a> <img src={`${baseUrl}/abstract0${i + 1}.jpg`} /> </a> ); }, dots: true, dotsClass: "slick-dots slick-thumb", infinite: true, speed: 500, slidesToShow: 1, slidesToScroll: 1 }; return ( <div className="slider-container"> <Slider {...settings}> <div> <img src={baseUrl + "/abstract01.jpg"} /> </div> <div> <img src={baseUrl + "/abstract02.jpg"} /> </div> <div> <img src={baseUrl + "/abstract03.jpg"} /> </div> <div> <img src={baseUrl + "/abstract04.jpg"} /> </div> </Slider> </div> ); } export default CustomPaging; ``` 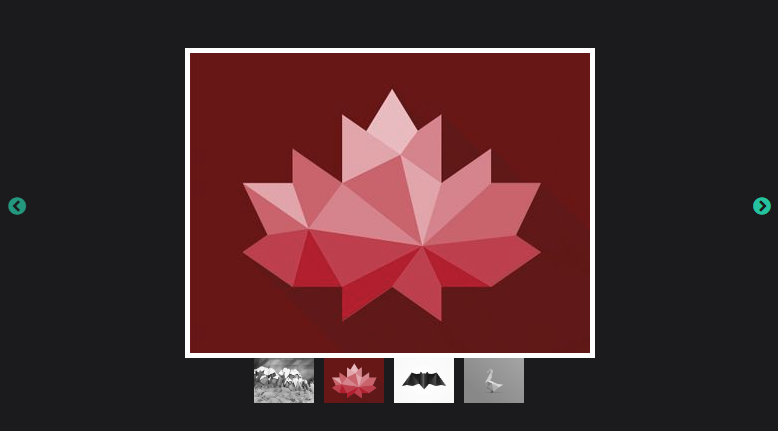 您可以閱讀有關可用的[prop 選項](https://react-slick.neostack.com/docs/api)和[方法](https://react-slick.neostack.com/docs/api#methods)的資訊。 您可以閱讀[文件](https://react-slick.neostack.com/docs/get-started)和所有帶有程式碼和輸出[的範例集](https://react-slick.neostack.com/docs/example/)。 他們在 GitHub 上有超過 11k 顆星,並且有超過 36 萬開發者在 GitHub 上使用它。 https://github.com/akiran/react-slick Star React Slick ⭐️ --- 16. [Medusa](https://github.com/medusajs/medusa) - 數位商務的建構模組。 ------------------------------------------------------------- 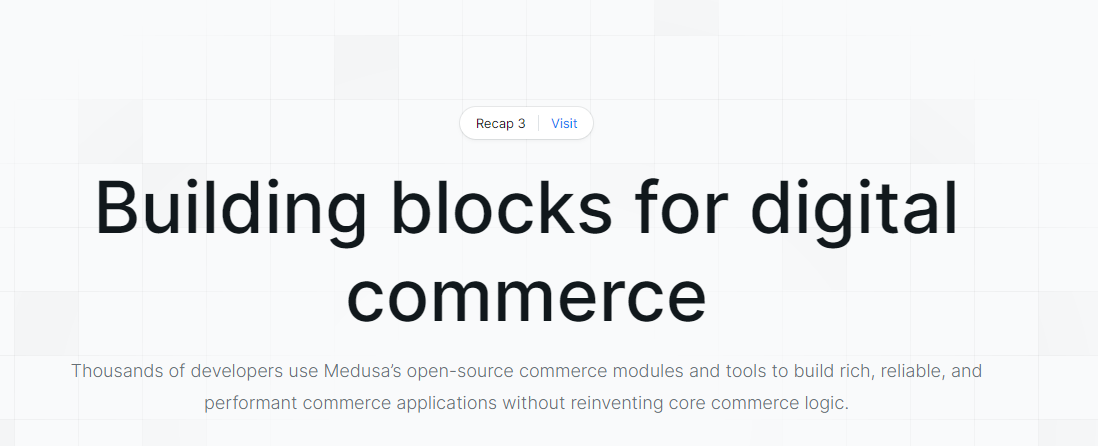 Medusa 是一組商務模組和工具,可讓您建立豐富、可靠且高效能的商務應用程式,而無需重新發明核心商務邏輯。 這些模組可以客製化並用於建立高級電子商務商店、市場或任何需要基礎商務原語的產品。所有模組都是開源的,可以在 npm 上免費取得。 開始使用以下 npm 指令。 ``` npm install medusa-react @tanstack/[email protected] @medusajs/medusa ``` 將其包含在`app.ts`中。 只有 MedusaProvider 的子級才能從其鉤子中受益。因此,Storefront 元件及其子元件現在可以使用 Medusa React 公開的鉤子。 ``` import { MedusaProvider } from "medusa-react" import Storefront from "./Storefront" import { QueryClient } from "@tanstack/react-query" import React from "react" const queryClient = new QueryClient() const App = () => { return ( <MedusaProvider queryClientProviderProps={{ client: queryClient }} baseUrl="http://localhost:9000" > <Storefront /> </MedusaProvider> ) } export default App ``` 例如,這就是您如何使用突變來建立購物車。 ``` import { useCreateCart } from "medusa-react" const Cart = () => { const createCart = useCreateCart() const handleClick = () => { createCart.mutate({}) // create an empty cart } return ( <div> {createCart.isLoading && <div>Loading...</div>} {!createCart.data?.cart && ( <button onClick={handleClick}> Create cart </button> )} {createCart.data?.cart?.id && ( <div>Cart ID: {createCart.data?.cart.id}</div> )} </div> ) } export default Cart ``` 他們提供了一套電子商務模組(大量選項),例如折扣、價目表、禮品卡等。 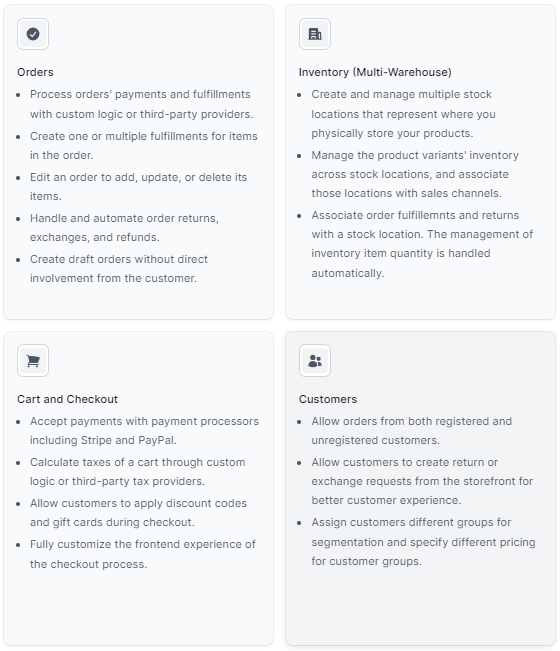 它們還提供了一種簡單的管理員和客戶身份驗證方法,您可以在[文件](https://docs.medusajs.com/)中閱讀。 他們提供了[nextjs 入門模板](https://docs.medusajs.com/starters/nextjs-medusa-starter)和[Medusa React](https://docs.medusajs.com/medusa-react/overview)作為 SDK。 該專案在 GitHub 上有 22k+ Stars,有 4k+ 開發者使用。 https://github.com/medusajs/medusa 明星美杜莎 ⭐️ --- 17. [React Markdown](https://github.com/remarkjs/react-markdown) - React 的 Markdown 元件. --------------------------------------------------------------------------------------- 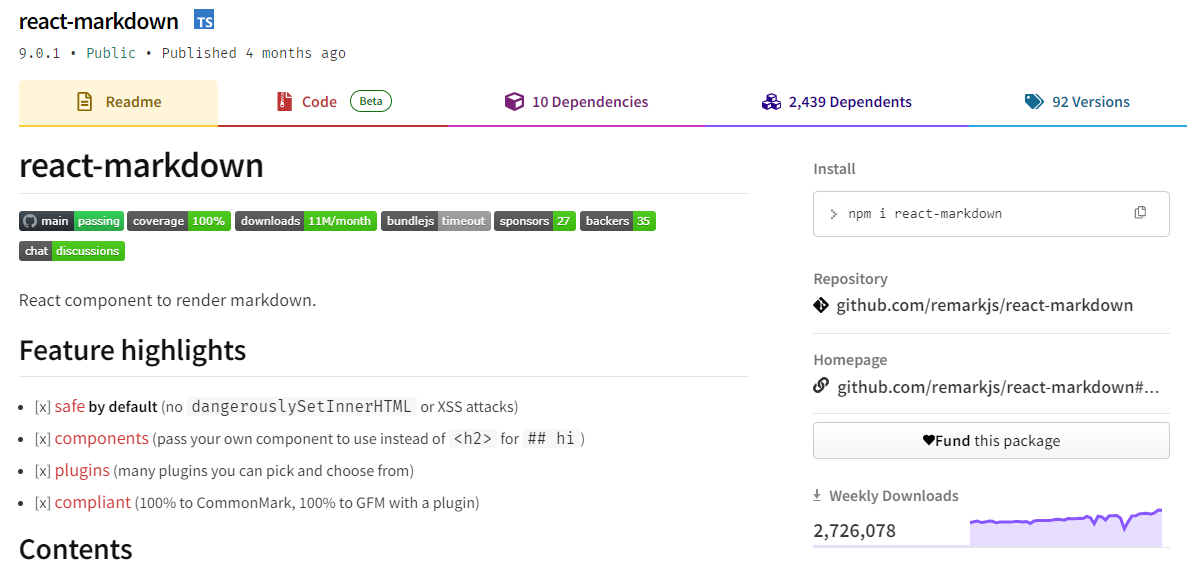 Markdown 至關重要,使用 React 渲染它對於各種場景都非常有用。 它提供了一個 React 元件,能夠安全地將一串 Markdown 渲染到 React 元素中。您可以透過傳遞外掛程式並指定要使用的元件而不是標準 HTML 元素來自訂 Markdown 的轉換。 開始使用以下 npm 指令。 ``` npm i react-markdown ``` 您可以這樣使用它。 ``` import React from 'react' import {createRoot} from 'react-dom/client' import Markdown from 'react-markdown' import remarkGfm from 'remark-gfm' const markdown = `Just a link: www.nasa.gov.` createRoot(document.body).render( <Markdown remarkPlugins={[remarkGfm]}>{markdown}</Markdown> ) ``` 等效的 JSX 是。 ``` <p> Just a link: <a href="http://www.nasa.gov">www.nasa.gov</a>. </p> ``` 他們還提供了一份[備忘錄](https://commonmark.org/help/)和一個十分鐘的逐步[教學](https://commonmark.org/help/tutorial/)。 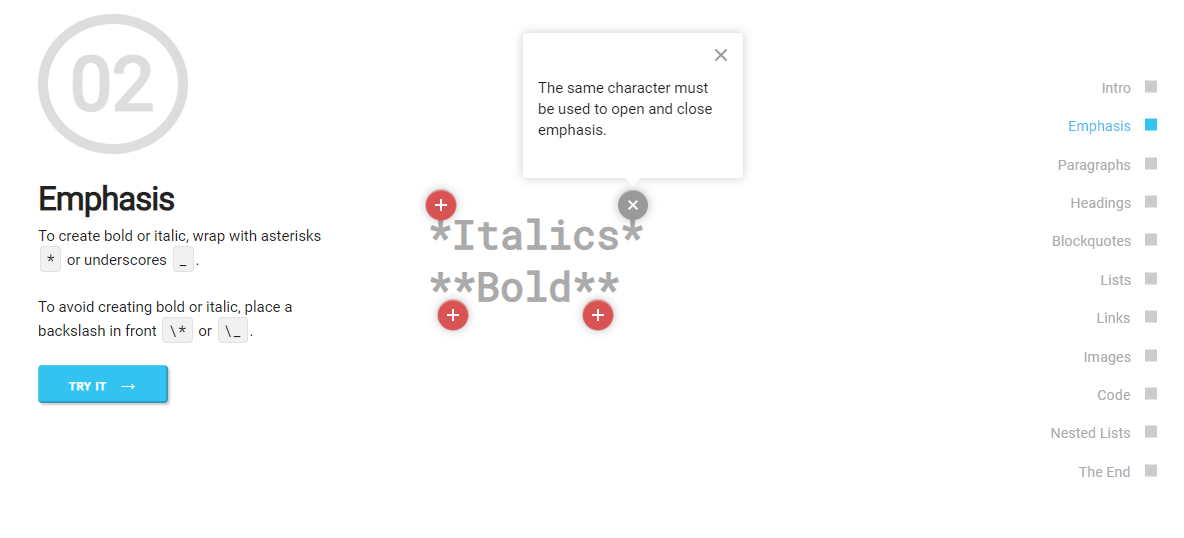 您可以閱讀[文件](https://github.com/remarkjs/react-markdown?tab=readme-ov-file#install)並查看[演示](https://remarkjs.github.io/react-markdown/)。 該專案在 GitHub 上有 12k+ Stars,[每週下載量超過 2700k](https://www.npmjs.com/package/react-markdown) ,並被 200k+ 開發人員使用,證明了它的真正有用性。 https://github.com/remarkjs/react-markdown Star React Markdown ⭐️ --- 18. [React JSONSchema Form](https://github.com/rjsf-team/react-jsonschema-form) - 用於從 JSON Schema 建立 Web 表單。 ------------------------------------------------------------------------------------------------------------ 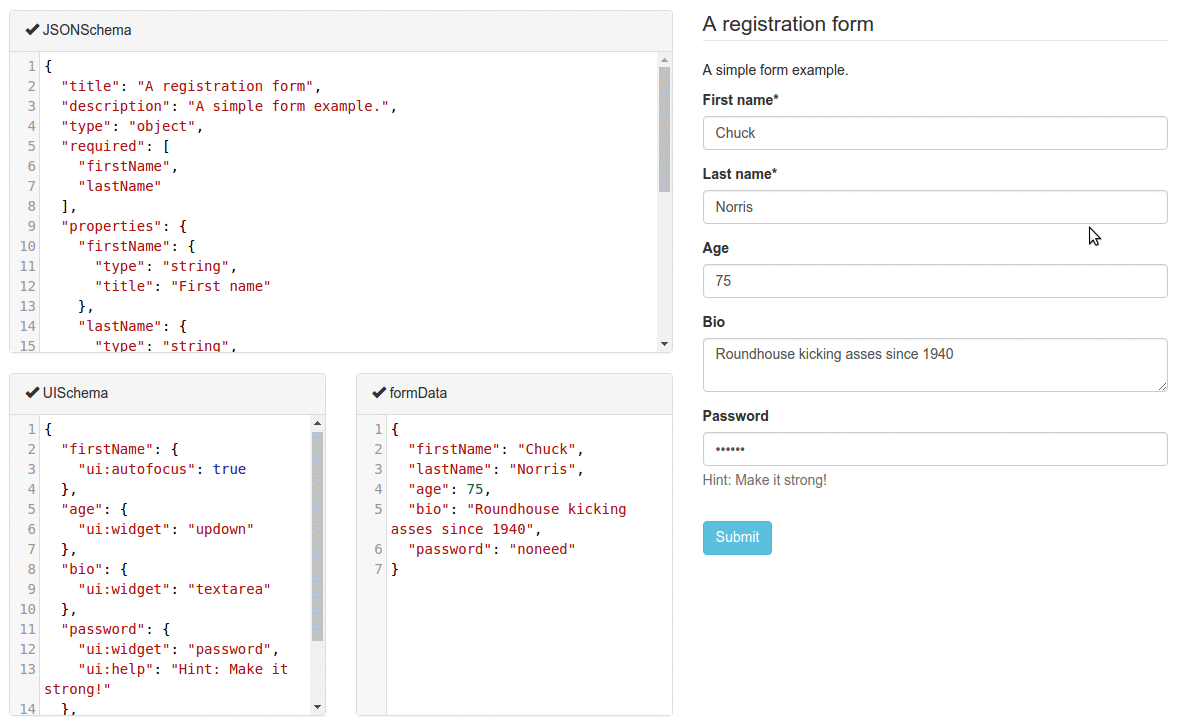 `react-jsonschema-form`會自動從 JSON Schema 產生 React 表單,使其非常適合僅使用 JSON schema 為任何資料產生表單。它提供了像 uiSchema 這樣的自訂選項來自訂預設主題之外的表單外觀。 開始使用以下 npm 指令。 ``` npm install @rjsf/core @rjsf/utils @rjsf/validator-ajv8 --save ``` 您可以這樣使用它。 ``` import { RJSFSchema } from '@rjsf/utils'; import validator from '@rjsf/validator-ajv8'; const schema: RJSFSchema = { title: 'Todo', type: 'object', required: ['title'], properties: { title: { type: 'string', title: 'Title', default: 'A new task' }, done: { type: 'boolean', title: 'Done?', default: false }, }, }; const log = (type) => console.log.bind(console, type); render( <Form schema={schema} validator={validator} onChange={log('changed')} onSubmit={log('submitted')} onError={log('errors')} />, document.getElementById('app') ); ``` 他們提供[高級定制](https://rjsf-team.github.io/react-jsonschema-form/docs/advanced-customization/)選項,包括定制小部件。 您可以閱讀[文件](https://rjsf-team.github.io/react-jsonschema-form/docs/)並查看[即時遊樂場](https://rjsf-team.github.io/react-jsonschema-form/)。 它在 GitHub 上擁有超過 13k 個 Star,並被 5k+ 開發人員使用。他們在`v5`上發布了 190 多個版本,因此他們正在不斷改進。 https://github.com/rjsf-team/react-jsonschema-form Star React JSONSchema 表單 ⭐️ --- 19. [Craft.js](https://github.com/prevwong/craft.js) - 建立可擴充的拖放頁面編輯器。 --------------------------------------------------------------------- 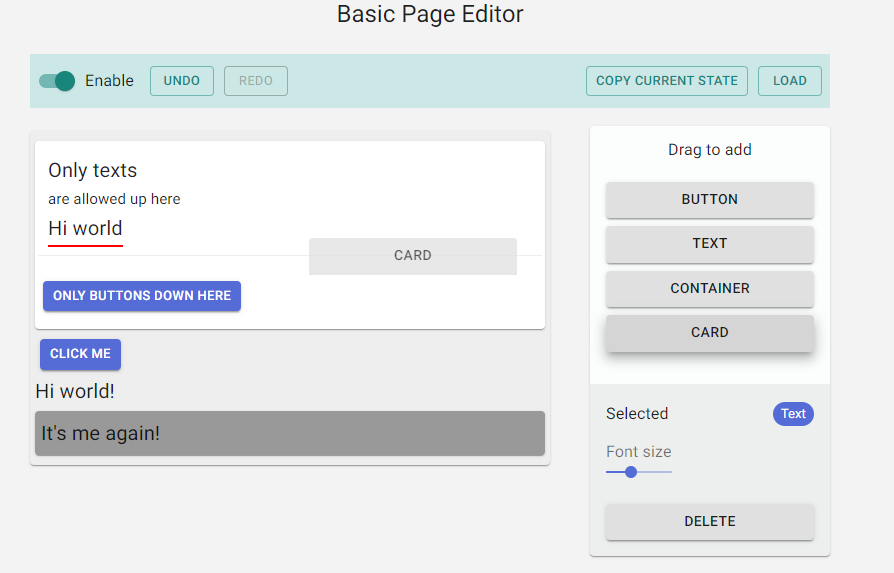 頁面編輯器可以增強使用者體驗,但從頭開始建立頁面編輯器可能會令人望而生畏。現有庫提供具有可編輯元件的預先建置編輯器,但自訂通常需要修改庫本身。 Craft.js 透過模組化頁面編輯器元件、透過拖放功能簡化自訂以及渲染管理來解決這個問題。在 React 中設計你的編輯器,無需複雜的插件系統,專注於你的特定需求和規格。 開始使用以下 npm 指令。 ``` npm install --save @craftjs/core ``` 他們還提供了有關如何入門的[簡短教程](https://craft.js.org/docs/guides/basic-tutorial)。我不會介紹它,因為它非常簡單且詳細。 您可以閱讀[文件](https://craft.js.org/docs/overview)並查看[即時演示](https://craft.js.org/)以及另一個[即時範例](https://craft.js.org/examples/basic)。 它在 GitHub 上有大約 6k+ Stars,但考慮到它們正在改進,仍然很有用。 https://github.com/prevwong/craft.js Star Craft.js ⭐️ --- 20. [Gatsby](https://github.com/gatsbyjs/gatsby) - 最好的基於 React 的框架,具有內建的效能、可擴展性和安全性。 ------------------------------------------------------------------------------------ 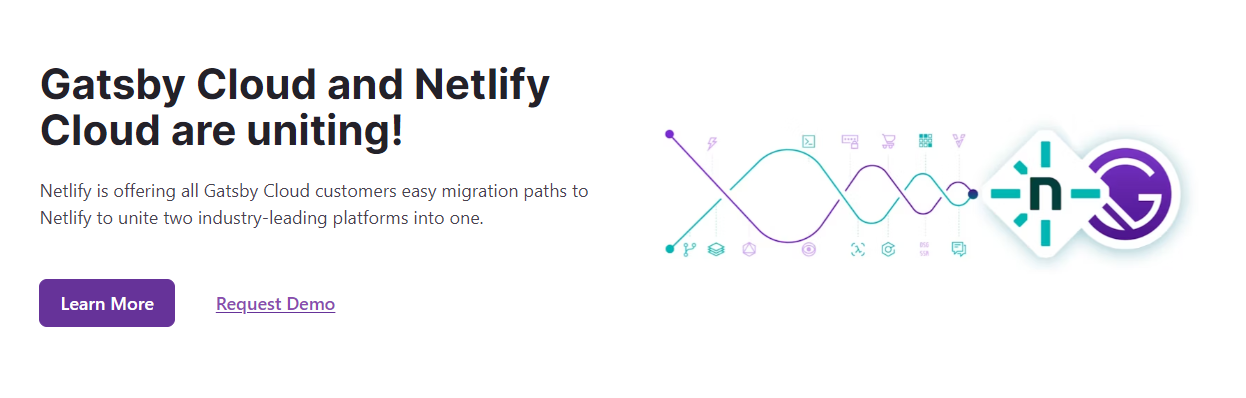 Gatsby 是一個基於 React 的框架,使開發人員能夠建立閃電般快速的網站和應用程式,將動態渲染的靈活性與靜態網站生成的速度融為一體。 憑藉可自訂的 UI 和對各種資料來源的支援等功能,Gatsby 提供了無與倫比的控制和可擴展性。此外,它還可以自動進行效能最佳化,使其成為靜態網站的首選。 開始使用以下 npm 指令。 ``` npm init gatsby ``` 這就是如何在 Gatsby(反應元件)中使用`Link` 。 ``` import React from "react" import { Link } from "gatsby" const Page = () => ( <div> <p> Check out my <Link to="/blog">blog</Link>! </p> <p> {/* Note that external links still use `a` tags. */} Follow me on <a href="https://twitter.com/gatsbyjs">Twitter</a>! </p> </div> ) ``` 他們提供了一組[入門模板,](https://www.gatsbyjs.com/starters/)其中包含如何使用它、涉及的依賴項以及每個模板的演示。 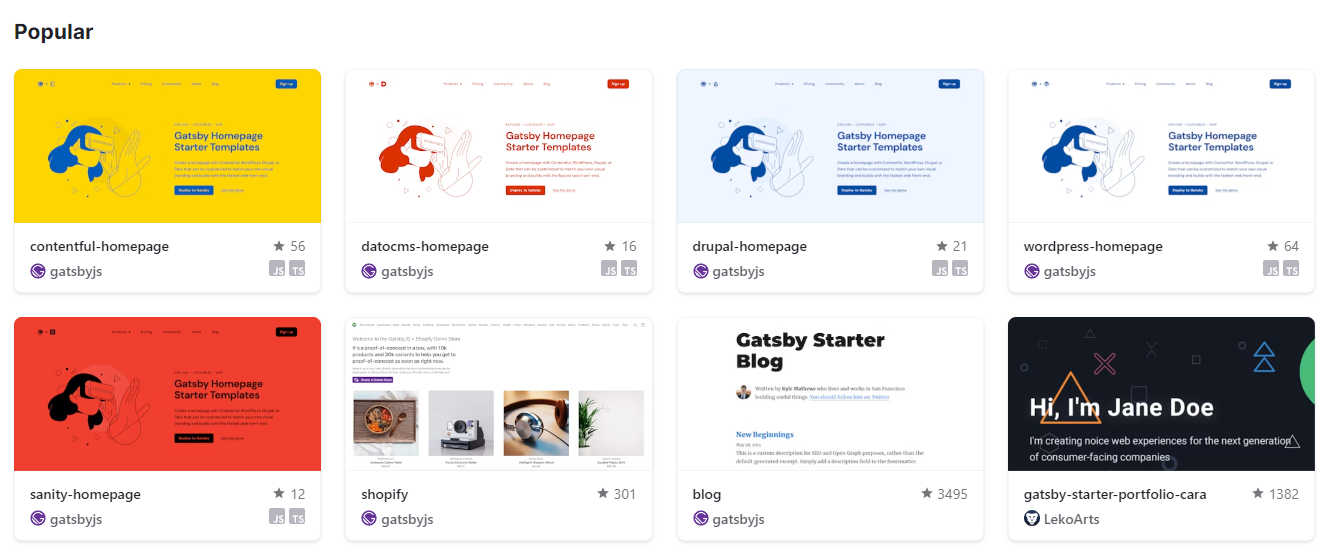 您可以閱讀有關 Gatsby 的一些[常見概念,](https://www.gatsbyjs.com/docs/conceptual/gatsby-concepts/)例如 React Hydration、Gatsby 建置流程等。 您可以閱讀[文件](https://www.gatsbyjs.com/docs/)並查看入門[教學課程](https://www.gatsbyjs.com/docs/tutorial/)。 Gatsby 在 GitHub 上擁有超過 55,000 顆星,並被超過 240,000 名開發者使用 https://github.com/gatsbyjs/gatsby 明星蓋茲比 ⭐️ --- 21. [Chat UI Kit React](https://github.com/chatscope/chat-ui-kit-react) - 在幾分鐘內使用 React 建立您的聊天 UI。 -------------------------------------------------------------------------------------------------- 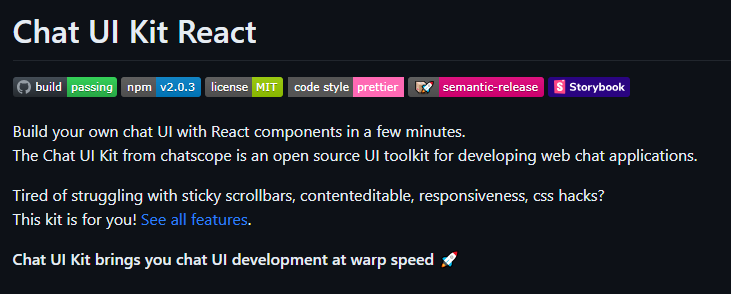 Chatscope 的聊天 UI 工具包是一個用於開發網頁聊天應用程式的開源 UI 工具包。 儘管該專案並未廣泛使用,但這些功能對於剛剛查看該專案的初學者來說還是很有用的。 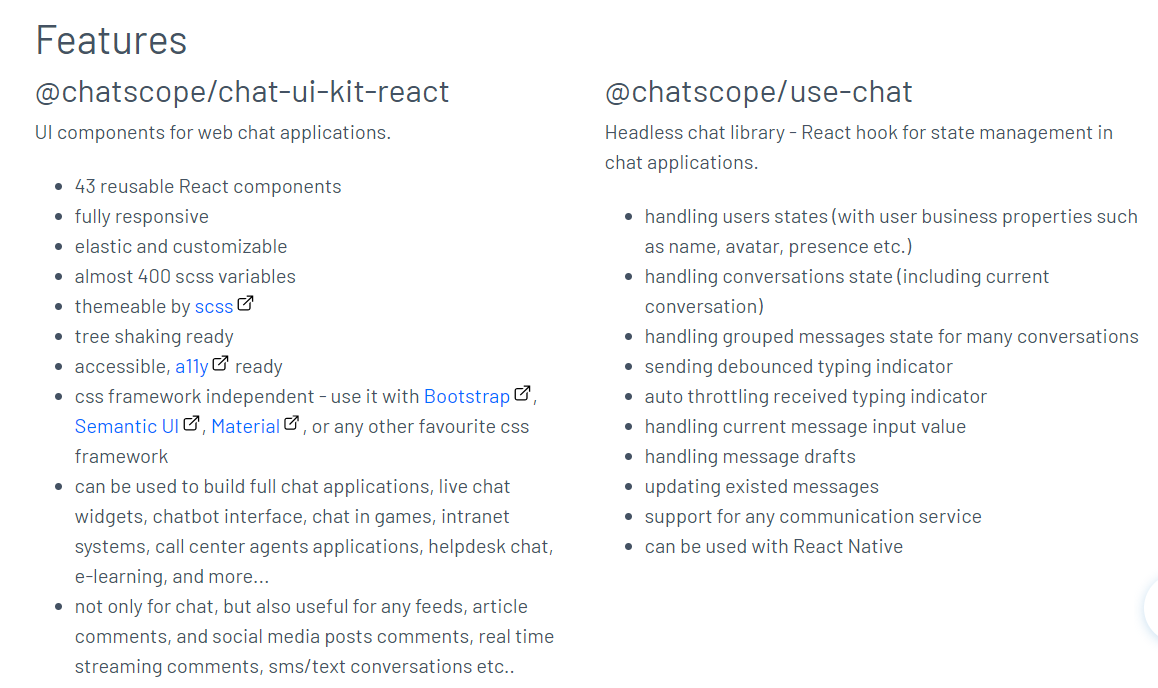 開始使用以下 npm 指令。 ``` npm install @chatscope/chat-ui-kit-react ``` 這就是建立 GUI 的方法。 ``` import styles from '@chatscope/chat-ui-kit-styles/dist/default/styles.min.css'; import { MainContainer, ChatContainer, MessageList, Message, MessageInput } from '@chatscope/chat-ui-kit-react'; <div style={{ position:"relative", height: "500px" }}> <MainContainer> <ChatContainer> <MessageList> <Message model={{ message: "Hello my friend", sentTime: "just now", sender: "Joe" }} /> </MessageList> <MessageInput placeholder="Type message here" /> </ChatContainer> </MainContainer> </div> ``` 您可以閱讀[文件](https://chatscope.io/docs/)。 故事書中有更[詳細的文件](https://chatscope.io/storybook/react/?path=/docs/documentation-introduction--docs)。 它提供了一些方便的元件,例如[`TypingIndicator`](https://chatscope.io/storybook/react/?path=/docs/components-typingindicator--docs) 、 [`Multiline Incoming`](https://chatscope.io/storybook/react/?path=/story/components-message--multiline-incoming)等等。 我知道你們中的一些人更喜歡透過部落格來了解整個結構,因此你可以閱讀使用 Chat UI Kit React 的 Rollbar 的[如何將 ChatGPT 與 React 整合](https://rollbar.com/blog/how-to-integrate-chatgpt-with-react/)。 您可以看到的一些演示: - [聊天機器人使用者介面](https://mars.chatscope.io/) - [與朋友聊天](https://chatscope.io/demo/chat-friends/)- 看看這個! 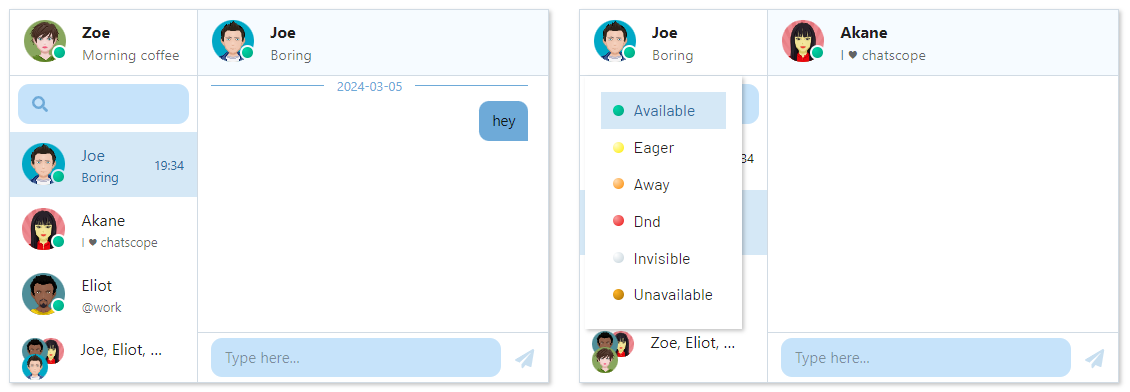 https://github.com/chatscope/chat-ui-kit-react Star Chat UI Kit React ⭐️ --- 22. [Botonic](https://github.com/hubtype/botonic) - 用於建立會話應用程式的 React 框架。 ------------------------------------------------------------------------- 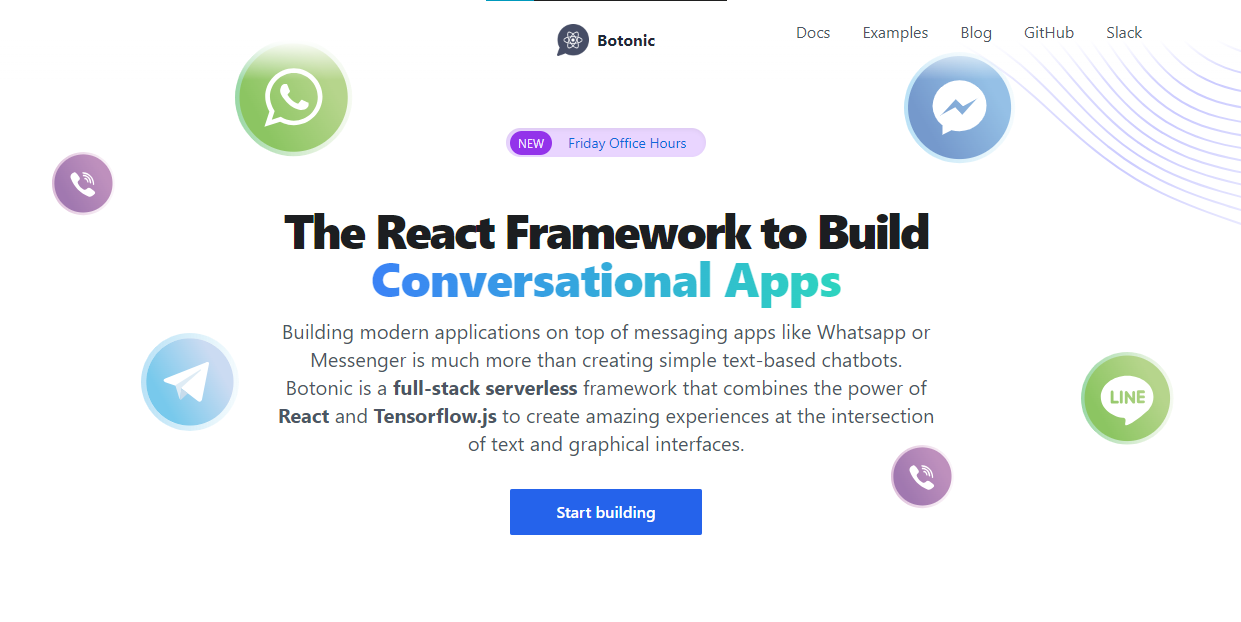 Botonic 是一個全端 Javascript 框架,用於建立在多個平台上執行的聊天機器人和現代對話應用程式:Web、行動和訊息應用程式(Messenger、WhatsApp、Telegram 等)。它建構在 ⚛️ React、Serverless 和 Tensorflow.js 之上。 如果您不了解對話應用程式的概念,可以在[官方部落格](https://www.hubtype.com/blog/what-are-conversational-apps)上閱讀它們。 使用 Botonic,您可以建立包含最佳文字外介面(簡單性、自然語言互動)和圖形介面(多媒體、視覺上下文、豐富互動)的會話應用程式。 這是一個強大的組合,可以提供比僅依賴文字和 NLP 的傳統聊天機器人更好的用戶體驗。 這就是 Botonic 的簡單方式。 ``` export default class extends React.Component { static async botonicInit({ input, session, params, lastRoutePath }) { await humanHandOff(session)) } render() { return ( <Text> Thanks for contacting us! One of our agents will attend you as soon as possible. </Text> ) } } ``` 它們也支援 TypeScript,所以這是一個優點。 您可以看到一些使用 Botonic 建置的[範例](https://botonic.io/examples/)及其原始程式碼。 您可以閱讀[文件](https://botonic.io/docs/welcome)以及如何[從頭開始建立會話應用程式](https://botonic.io/docs/create-convapp)。 https://github.com/hubtype/botonic Star Botonic ⭐️ --- 23. [React Flowbite](https://github.com/themesberg/flowbite-react) - 為 Flowbite 和 Tailwind CSS 建構的 React 元件. ------------------------------------------------------------------------------------------------------------ 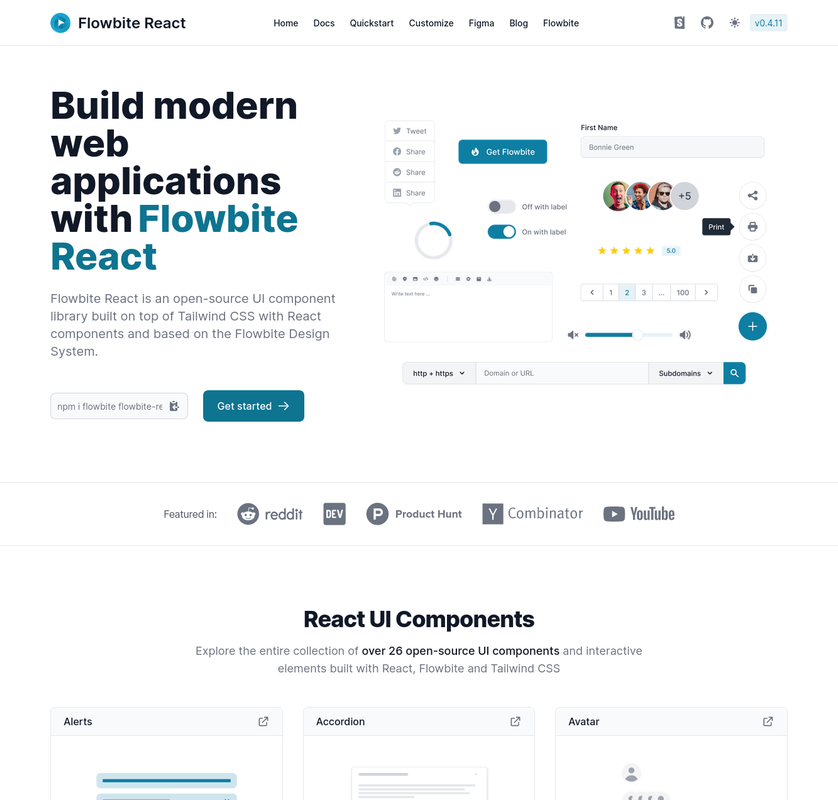 每個人對他們想要用來建立網站的使用者介面都有不同的偏好。 Flowbite React 是 UI 元件的開源集合,在 React 中建置,具有來自 Tailwind CSS 的實用程式類,您可以將其用作使用者介面和網站的起點。 開始使用以下 npm 指令。 ``` npm i flowbite-react ``` 這是一起使用表格和鍵盤元件的方法。 ``` 'use client'; import { Kbd, Table } from 'flowbite-react'; import { MdKeyboardArrowDown, MdKeyboardArrowLeft, MdKeyboardArrowRight, MdKeyboardArrowUp } from 'react-icons/md'; function Component() { return ( <Table> <Table.Head> <Table.HeadCell>Key</Table.HeadCell> <Table.HeadCell>Description</Table.HeadCell> </Table.Head> <Table.Body className="divide-y"> <Table.Row className="bg-white dark:border-gray-700 dark:bg-gray-800"> <Table.Cell className="whitespace-nowrap font-medium text-gray-900 dark:text-white"> <Kbd>Shift</Kbd> <span>or</span> <Kbd>Tab</Kbd> </Table.Cell> <Table.Cell>Navigate to interactive elements</Table.Cell> </Table.Row> <Table.Row className="bg-white dark:border-gray-700 dark:bg-gray-800"> <Table.Cell className="whitespace-nowrap font-medium text-gray-900 dark:text-white"> <Kbd>Enter</Kbd> or <Kbd>Spacebar</Kbd> </Table.Cell> <Table.Cell>Ensure elements with ARIA role="button" can be activated with both key commands.</Table.Cell> </Table.Row> <Table.Row className="bg-white dark:border-gray-700 dark:bg-gray-800"> <Table.Cell className="whitespace-nowrap font-medium text-gray-900 dark:text-white"> <span className="inline-flex gap-1"> <Kbd icon={MdKeyboardArrowUp} /> <Kbd icon={MdKeyboardArrowDown} /> </span> <span> or </span> <span className="inline-flex gap-1"> <Kbd icon={MdKeyboardArrowLeft} /> <Kbd icon={MdKeyboardArrowRight} /> </span> </Table.Cell> <Table.Cell>Choose and activate previous/next tab.</Table.Cell> </Table.Row> </Table.Body> </Table> ); } ``` 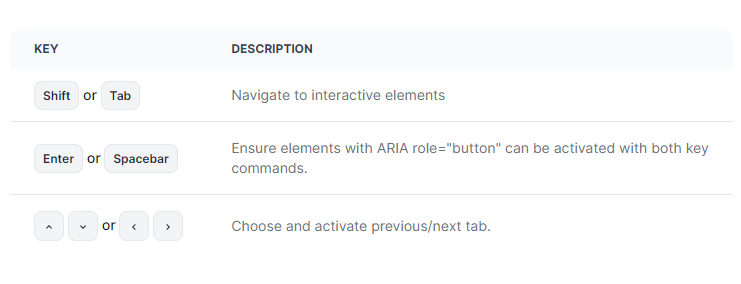 您可以閱讀[文件](https://www.flowbite-react.com/docs/getting-started/introduction)並查看[Storybook](https://storybook.flowbite-react.com/?path=/story/components-accordion--always-open)中的功能。您也可以查看[元件](https://www.flowbite-react.com/docs/components/accordion)清單。 在我看來,如果您想快速設定 UI,但又不想最終為高品質的開源專案使用預先定義的庫元件,那麼這很好。 該專案在 GitHub 上擁有超過 1,500 顆星,擁有超過 37,000 名開發者的用戶群,並受到社群的廣泛認可和信任,使其成為一個可靠的選擇。 https://github.com/themesberg/flowbite-react Star React Flowbite ⭐️ --- 24. [DND 套件](https://github.com/clauderic/dnd-kit)- 輕量級、高效能、可存取且可擴展的拖放功能。 ------------------------------------------------------------------------- 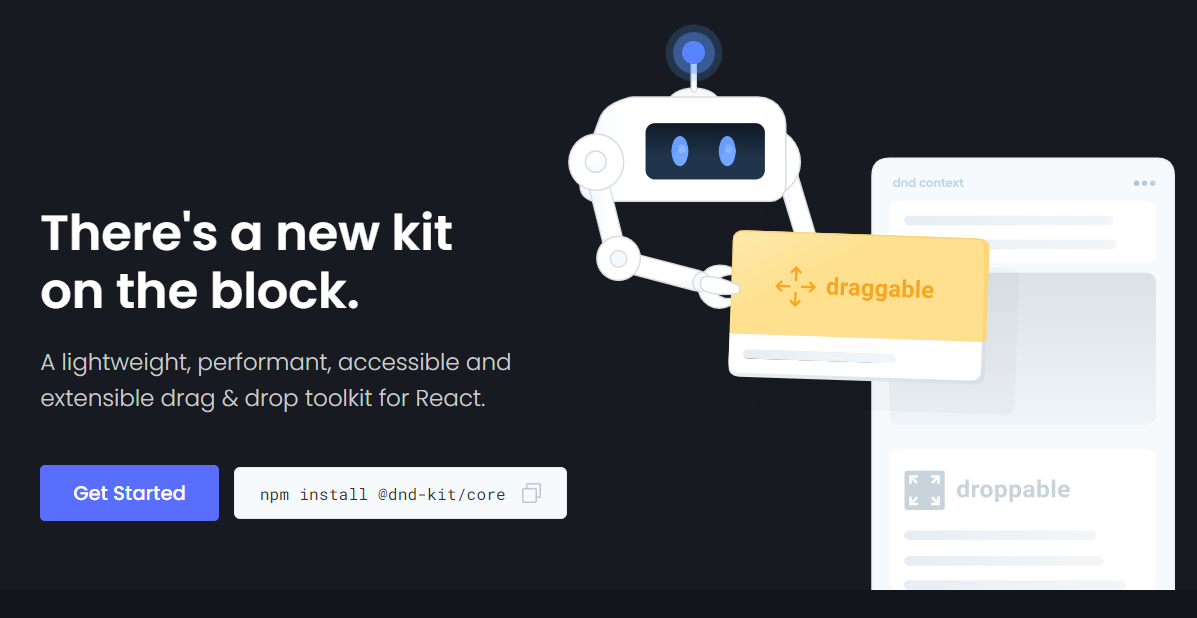 這是一個強大的 React 拖放工具包,擁有可自訂的碰撞檢測、多個啟動器和自動滾動等功能。 它的設計考慮到了 React,提供了方便集成的鉤子,無需進行重大的架構更改。支援從清單到網格和虛擬化清單的各種用例,它既是動態的又是輕量級的,沒有外部相依性。 開始使用以下 npm 指令。 ``` npm install @dnd-kit/core ``` 這就是建立可拖放元件的方法。 `Example.jsx` ``` import React, {useState} from 'react'; import {DndContext} from '@dnd-kit/core'; import {Draggable} from './Draggable'; import {Droppable} from './Droppable'; function Example() { const [parent, setParent] = useState(null); const draggable = ( <Draggable id="draggable"> Go ahead, drag me. </Draggable> ); return ( <DndContext onDragEnd={handleDragEnd}> {!parent ? draggable : null} <Droppable id="droppable"> {parent === "droppable" ? draggable : 'Drop here'} </Droppable> </DndContext> ); function handleDragEnd({over}) { setParent(over ? over.id : null); } } ``` `Droppable.jsx` ``` import React from 'react'; import {useDroppable} from '@dnd-kit/core'; export function Droppable(props) { const {isOver, setNodeRef} = useDroppable({ id: props.id, }); const style = { opacity: isOver ? 1 : 0.5, }; return ( <div ref={setNodeRef} style={style}> {props.children} </div> ); } ``` `Draggable.jsx` ``` import React from 'react'; import {useDraggable} from '@dnd-kit/core'; import {CSS} from '@dnd-kit/utilities'; function Draggable(props) { const {attributes, listeners, setNodeRef, transform} = useDraggable({ id: props.id, }); const style = { // Outputs `translate3d(x, y, 0)` transform: CSS.Translate.toString(transform), }; return ( <button ref={setNodeRef} style={style} {...listeners} {...attributes}> {props.children} </button> ); } ``` 我將可拖曳元件放在可放置元件上。 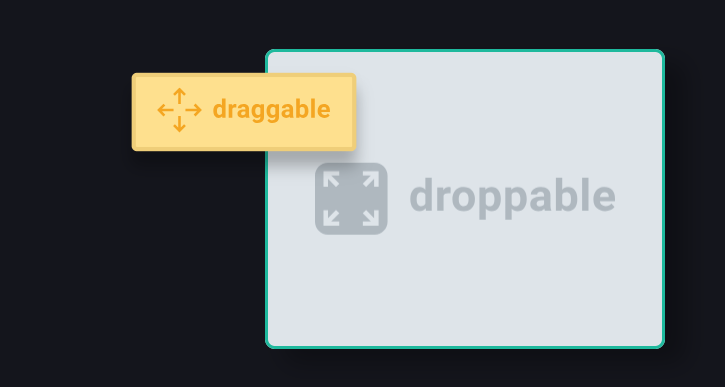 您可以閱讀[文件](https://docs.dndkit.com/)以及滑鼠和指標等[感測器的選項](https://docs.dndkit.com/introduction/installation#core-library)。 它在 GitHub 上擁有 10k+ Stars,並被 GitHub 上 47k+ 開發人員使用。 https://github.com/clauderic/dnd-kit 明星免打擾套件 ⭐️
這篇文章最初發佈在[我的部落格](http://frugencefidel.com/blog/10-javascript-array-methods-you-should-know)上。 在這篇文章中,我將分享 10 個你應該知道的 JavaScript 陣列方法。 如果你對陣列一無所知,可以點選這裡查看[陣列介紹](http://frugencefidel.com/blog/understanding-basics-of-array-in-javascript)。 這裡有 10 個你至少應該知道的 javascript 陣列方法 *1. forEach()* 此方法可以幫助您循環陣列。 ``` const arr = [1, 2, 3, 4, 5, 6]; arr.forEach(item => { console.log(item); // output: 1 2 3 4 5 6 }); ``` *2. includes()* 此方法檢查陣列是否包含在方法中傳遞的專案。 ``` const arr = [1, 2, 3, 4, 5, 6]; arr.includes(2); // output: true arr.includes(7); // output: false ``` *3. filter()* 此方法會建立新陣列,其中僅包含在提供的函數內傳遞條件的元素。 ``` const arr = [1, 2, 3, 4, 5, 6]; // item(s) greater than 3 const filtered = arr.filter(num => num > 3); console.log(filtered); // output: [4, 5, 6] console.log(arr); // output: [1, 2, 3, 4, 5, 6] ``` *4. map()* 此方法透過在每個元素中呼叫提供的函數來建立新陣列。 ``` const arr = [1, 2, 3, 4, 5, 6]; // add one to every element const oneAdded = arr.map(num => num + 1); console.log(oneAdded); // output [2, 3, 4, 5, 6, 7] console.log(arr); // output: [1, 2, 3, 4, 5, 6] ``` *5. reduce()* > reduce() 方法對累加器和陣列中的每個元素(從左到右)套用函數,將其減少為單一值 - MDN ``` const arr = [1, 2, 3, 4, 5, 6]; const sum = arr.reduce((total, value) => total + value, 0); console.log(sum); // 21 ``` *6. some()* 此方法檢查陣列的至少一項是否符合條件。如果通過,則傳回“true”,否則傳回“false”。 ``` const arr = [1, 2, 3, 4, 5, 6]; // at least one element is greater than 4? const largeNum = arr.some(num => num > 4); console.log(largeNum); // output: true // at least one element is less than or equal to 0? const smallNum = arr.some(num => num <= 0); console.log(smallNum); // output: false ``` *7. every()* 此方法檢查所有陣列的專案是否都符合條件。如果通過,則傳回“true”,否則傳回“false”。 ``` const arr = [1, 2, 3, 4, 5, 6]; // all elements are greater than 4 const greaterFour = arr.every(num => num > 4); console.log(greaterFour); // output: false // all elements are less than 10 const lessTen = arr.every(num => num < 10); console.log(lessTen); // output: true ``` *8. sort()* 此方法用於按升序或降序排列/排序陣列的專案。 ``` const arr = [1, 2, 3, 4, 5, 6]; const alpha = ['e', 'a', 'c', 'u', 'y']; // sort in descending order descOrder = arr.sort((a, b) => a > b ? -1 : 1); console.log(descOrder); // output: [6, 5, 4, 3, 2, 1] // sort in ascending order ascOrder = alpha.sort((a, b) => a > b ? 1 : -1); console.log(ascOrder); // output: ['a', 'c', 'e', 'u', 'y'] ``` *9. Array.from()* 這會將所有類似陣列或可迭代的內容變更為真正的陣列,尤其是在使用 DOM 時,這樣您就可以使用其他陣列方法,如reduce、map、filter 等。 ``` const name = 'frugence'; const nameArray = Array.from(name); console.log(name); // output: frugence console.log(nameArray); // output: ['f', 'r', 'u', 'g', 'e', 'n', 'c', 'e'] ``` 使用 DOM ``` // I assume that you have created unorder list of items in our html file. const lis = document.querySelectorAll('li'); const lisArray = Array.from(document.querySelectorAll('li')); // is true array? console.log(Array.isArray(lis)); // output: false console.log(Array.isArray(lisArray)); // output: true ``` *10. Array.of()* 這會根據傳入的每個參數建立陣列。 ``` const nums = Array.of(1, 2, 3, 4, 5, 6); console.log(nums); // output: [1, 2, 3, 4, 5, 6] ``` --- 原文出處:https://dev.to/frugencefidel/10-javascript-array-methods-you-should-know-4lk3
**編輯**:大家好!在對本文做出驚人反應後,我建立了一個名為「每週專案俱樂部」的專案。每週您的收件匣都會收到需要解決的問題。你可以努力解決問題,並且你將得到整個俱樂部的幫助,讓你走上正軌。了解更多並[在這裡](https://weeklyproject.club)註冊! 有一天我注意到一個模式。我注意到很多人都在努力 學習編程,但他們心中沒有特定的目標。我已經討論過如何了解您想要學習程式設計的原因可以幫助您選擇要學習的語言[!](https://pickaframework.com/articles/why/) ,以及如何實際做出決定([在這裡!](https://pickaframework.com/feature_fishing/) )但是專案有什麼幫助呢? 當我指導程式設計師時,我發現有一個專案可以幫助排除其他一些幹擾,例如想知道你是否使用了正確的語言。透過專注於一個特定的目標,你就不用那麼費力去擔心*這*是否正是你應該使用的語言。結果是你建立了一些簡潔的東西,並且一路上你學到了一些東西! 2隻鳥,1塊石頭。 這就是為什麼我為初學者程式設計師策劃了這個專案清單。許多人列出了大量的專案來學習編程,但很少按照難度進行組織。我瀏覽了幾個流行的程式設計專案想法清單。如果您想查看完整列表,可以在頁面底部找到來源。 我將其分為教程和想法。教程包含資源連結,而想法只是專案的一般描述。我還列出了我最喜歡的初學者清單。 看看,看看是否有什麼啟發你! 教學 == 我的最愛 ---- - [透過 30 個教學在 30 天內建立 30 個東西](https://javascript30.com) - [在 30 分鐘內建立一個簡單的搜尋機器人](https://medium.freecodecamp.org/how-to-build-a-simple-search-bot-in-30-minutes-eb56fcedcdb1) - [使用 Xamarin 和 Visual Studio 建立 iOS 照片庫應用程式](https://www.raywenderlich.com/134049/building-ios-apps-with-xamarin-and-visual-studio) - [建立 Android 手電筒應用程式](https://www.youtube.com/watch?v=dhWL4DC7Krs)(影片) - [製作聊天應用程式](https://medium.freecodecamp.org/how-to-build-a-chat-application-using-react-redux-redux-saga-and-web-sockets-47423e4bc21a) - [使用 React Native 建立 ToDo 應用程式](https://blog.hasura.io/tutorial-fullstack-react-native-with-graphql-and-authentication-18183d13373a) 簡單的 --- - [使用 C# 和 Xamarin 建立空白應用程式(正在進行中)](https://www.intertech.com/Blog/xamarin-tutorial-part-1-create-a-blank-app/) - [使用 Xamarin 和 Visual Studio 建立 iOS 照片庫應用程式](https://www.raywenderlich.com/134049/building-ios-apps-with-xamarin-and-visual-studio) - [建立加載畫面](https://medium.freecodecamp.org/how-to-build-a-delightful-loading-screen-in-5-minutes-847991da509f) - [使用 JS 建立 HTML 計算器](https://medium.freecodecamp.org/how-to-build-an-html-calculator-app-from-scratch-using-javascript-4454b8714b98) - [建立 React Native Todo 應用程式](https://egghead.io/courses/build-a-react-native-todo-application) - 使用 Node.js 編寫 Twitter 機器人 ``` - [Part 1](https://codeburst.io/build-a-simple-twitter-bot-with-node-js-in-just-38-lines-of-code-ed92db9eb078) ``` ``` - [Part 2](https://codeburst.io/build-a-simple-twitter-bot-with-node-js-part-2-do-more-2ef1e039715d) ``` - [建立一個簡單的 RESTFUL Web 應用程式](https://closebrace.com/tutorials/2017-03-02/creating-a-simple-restful-web-app-with-nodejs-express-and-mongodb) - [在 30 分鐘內建立一個簡單的搜尋機器人](https://medium.freecodecamp.org/how-to-build-a-simple-search-bot-in-30-minutes-eb56fcedcdb1) - [建立一個工作抓取 Web 應用程式](https://medium.freecodecamp.org/how-i-built-a-job-scraping-web-app-using-node-js-and-indreed-7fbba124bbdc) - [使用 Python 挖掘 Twitter 資料](https://marcobonzanini.com/2015/03/02/mining-twitter-data-with-python-part-1/) - [使用 Scrapy 和 MongoDB 抓取網站](https://realpython.com/blog/python/web-scraping-with-scrapy-and-mongodb/) - [如何使用 Python 和 Selenium WebDriver 進行抓取](http://www.byperth.com/2018/04/25/guide-web-scraping-101-what-you-need-to-know-and-how-to-scrape-with-python-selenium-webdriver/) - [我應該使用 BeautifulSoup 觀看哪部電影](https://medium.com/@nishantsahoo.in/which-movie-should-i-watch-5c83a3c0f5b1) - [使用 Flask 建立微博](https://blog.miguelgrinberg.com/post/the-flask-mega-tutorial-part-i-hello-world) - 在 Django 中建立部落格 Web 應用程式 ``` - [Part I : Introduction](https://tutorial.djangogirls.org/en/) ``` ``` - [Part II : Extension To Add More Features](https://legacy.gitbook.com/book/djangogirls/django-girls-tutorial-extensions/details) ``` - [選擇您自己的冒險演示](https://www.twilio.com/blog/2015/03/choose-your-own-adventures-presentations-wizard-mode-part-1-of-3.html) - [使用 Flask 和 RethinkDB 建立待辦事項列表](https://realpython.com/blog/python/rethink-flask-a-simple-todo-list-powered-by-flask-and-rethinkdb/) 中等的 --- - [透過建立簡單的 RPG 遊戲來學習 C#](http://scottlilly.com/learn-c-by-building-a-simple-rpg-index/) - [用 C# 創作 Rogue-like 遊戲](https://roguesharp.wordpress.com/) - [使用 Clojure 建構 Twitter 機器人](http://howistart.org/posts/clojure/1/index.html) - [建立拼字檢查器](https://bernhardwenzel.com/articles/clojure-spellchecker/) - [使用 Java 建立簡單的 HTTP 伺服器](http://javarevisited.blogspot.com/2015/06/how-to-create-http-server-in-java-serversocket-example.html) - [建立 Android 手電筒應用程式](https://www.youtube.com/watch?v=dhWL4DC7Krs)(影片) - [建立具有使用者身份驗證的 Spring Boot 應用程式](https://scotch.io/tutorials/build-a-spring-boot-app-with-user-authentication) - [透過 30 個教學在 30 天內建立 30 個東西](https://javascript30.com) - [使用純 JS 建立應用程式](https://medium.com/codingthesmartway-com-blog/pure-javascript-building-a-real-world-application-from-scratch-5213591cfcd6) - [建立無伺服器 React.js 應用程式](http://serverless-stack.com/) - [建立 Trello 克隆](http://codeloveandboards.com/blog/2016/01/04/trello-tribute-with-phoenix-and-react-pt-1/) - [使用 React、Node、MongoDB 和 SocketIO 建立角色投票應用程式](http://sahatyalkabov.com/create-a-character-voting-app-using-react-nodejs-mongodb-and-socketio/) - [React 教學:克隆 Yelp](https://www.fullstackreact.com/articles/react-tutorial-cloning-yelp/) - [使用 React.js 和 Node.js 建立簡單的中型克隆](https://codeburst.io/build-simple-medium-com-on-node-js-and-react-js-a278c5192f47) - [在 JS 中整合 MailChimp](https://medium.freecodecamp.org/how-to-integrate-mailchimp-in-a-javascript-web-app-2a889fb43f6f) - [使用 React Native 建立 ToDo 應用程式](https://blog.hasura.io/tutorial-fullstack-react-native-with-graphql-and-authentication-18183d13373a) - [製作聊天應用程式](https://medium.freecodecamp.org/how-to-build-a-chat-application-using-react-redux-redux-saga-and-web-sockets-47423e4bc21a) - [使用 React Native 建立新聞應用程式](https://medium.freecodecamp.org/create-a-news-app-using-react-native-ced249263627) - [學習 React 的 Webpack](https://medium.freecodecamp.org/learn-webpack-for-react-a36d4cac5060) - [建立您自己的 React 樣板](https://medium.freecodecamp.org/how-to-build-your-own-react-boilerplate-2f8cbbeb9b3f) - [基本 React+Redux 入門教學](https://hackernoon.com/a-basic-react-redux-introductory-tutorial-adcc681eeb5e) - [建立一個預約安排程序](https://hackernoon.com/build-an-appointment-scheduler-using-react-twilio-and-cosmic-js-95377f6d1040) - 使用 Angular 2+ 建立具有離線功能的 Hacker News 用戶端 ``` - [Part 1](https://houssein.me/angular2-hacker-news) ``` ``` - [Part 2](https://houssein.me/progressive-angular-applications) ``` - 帶有 Angular 5 的 ToDo 應用程式 ``` - [Introduction to Angular](http://www.discoversdk.com/blog/intro-to-angular-and-the-evolution-of-the-web) ``` ``` - [Part 1](http://www.discoversdk.com/blog/angular-5-to-do-list-app-part-1) ``` - 帶有 Angular 5 的 ToDo 應用程式 ``` - [Introduction to Angular](http://www.discoversdk.com/blog/intro-to-angular-and-the-evolution-of-the-web) ``` ``` - [Part 1](http://www.discoversdk.com/blog/angular-5-to-do-list-app-part-1) ``` 難的 -- - [建構一個解釋器](http://www.craftinginterpreters.com/)(第 14 章是用 C 寫的) - [用 C 語言寫一個 Shell](https://brennan.io/2015/01/16/write-a-shell-in-c/) - [編寫 FUSE 文件系統](https://www.cs.nmsu.edu/~pfeiffer/fuse-tutorial/) - [建立您自己的文字編輯器](http://viewsourcecode.org/snaptoken/kilo/) - [建立自己的 Lisp](http://www.buildyourownlisp.com/) - [建構 CoreWiki](https://www.youtube.com/playlist?list=PLVMqA0_8O85yC78I4Xj7z48ES48IQBa7p)這是一個 Wiki 風格的內容管理系統,完全用 C# 使用 ASP.NET Core 和 Razor Pages 編寫。您可以[在這裡](https://github.com/csharpfritz/CoreWiki)找到原始程式碼。 - [建構 JIRA 與 Clojure 和 Atlassian Connect 的集成](https://hackernoon.com/building-a-jira-integration-with-clojure-atlassian-connect-506ebd112807) - [建構一個解釋器](http://www.craftinginterpreters.com/)(第 4-13 章是用 Java 寫的) - [使用 Mocha、React、Redux 和 Immutable 透過測試優先開發來建立全端電影投票應用程式](https://teropa.info/blog/2015/09/10/full-stack-redux-tutorial.html) - [使用 React 和 Node 建立 Twitter Stream](https://scotch.io/tutorials/build-a-real-time-twitter-stream-with-node-and-react-js) - 使用 Webtask.io 建立無伺服器 MERN Story 應用程式 ``` - [Part 1](https://scotch.io/tutorials/build-a-serverless-mern-story-app-with-webtask-io-zero-to-deploy-1) ``` ``` - [Part 2](https://scotch.io/tutorials/build-a-serverless-mern-story-app-with-webtask-io-zero-to-deploy-2) ``` - [使用 React + Parcel 建立 Chrome 擴充功能](https://medium.freecodecamp.org/building-chrome-extensions-in-react-parcel-79d0240dd58f) ``` [Testing React App With Pupepeteer and Jest](https://blog.bitsrc.io/testing-your-react-app-with-puppeteer-and-jest-c72b3dfcde59) ``` - [用 React 編寫生命遊戲](https://medium.freecodecamp.org/create-gameoflife-with-react-in-one-hour-8e686a410174) - [建立帶有情感分析的聊天應用程式](https://codeburst.io/build-a-chat-app-with-sentiment-analysis-using-next-js-c43ebf3ea643) - [建立全端 Web 應用程式設置](https://hackernoon.com/full-stack-web-application-using-react-node-js-express-and-webpack-97dbd5b9d708) - 建立隨機報價機 ``` - [Part 1](https://www.youtube.com/watch?v=3QngsWA9IEE) ``` ``` - [Part 2](https://www.youtube.com/watch?v=XnoTmO06OYo) ``` ``` - [Part 3](https://www.youtube.com/watch?v=us51Jne67_I) ``` ``` - [Part 4](https://www.youtube.com/watch?v=iZx7hqHb5MU) ``` ``` - [Part 5](https://www.youtube.com/watch?v=lpba9vBqXl0) ``` ``` - [Part 6](https://www.youtube.com/watch?v=Jvp8j6zrFHE) ``` ``` - [Part 7](https://www.youtube.com/watch?v=M_hFfrN8_PQ) ``` - 使用 Angular 6 建立美麗的現實世界應用程式: ``` - [Part I](https://medium.com/@hamedbaatour/build-a-real-world-beautiful-web-app-with-angular-6-a-to-z-ultimate-guide-2018-part-i-e121dd1d55e) ``` - [使用 BootStrap 4 和 Angular 6 建立響應式佈局](https://medium.com/@tomastrajan/how-to-build-responsive-layouts-with-bootstrap-4-and-angular-6-cfbb108d797b) - [使用 Django 和測試驅動開發建立待辦事項列表](http://www.obeythetestinggoat.com/) - [使用 Python 建立 RESTful 微服務](http://www.skybert.net/python/developing-a-restful-micro-service-in-python/) - [使用 Docker、Flask 和 React 的微服務](https://testdriven.io/) - [使用 Flask 建立簡單的 Web 應用程式](https://pythonspot.com/flask-web-app-with-python/) - [使用 Flask 建立 RESTful API – TDD 方式](https://scotch.io/tutorials/build-a-restful-api-with-flask-the-tdd-way) - [在 20 分鐘內建立 Django API](https://codeburst.io/create-a-django-api-in-under-20-minutes-2a082a60f6f3) 想法 == 簡單的 --- ### 99 瓶 - 建立一個程序,列印歌曲“牆上的 99 瓶啤酒”的每一行。 - 不要使用所有數字的列表,也不要手動輸入所有數字。請改用內建函數。 - 除了短語“取下一個”之外,您不得直接在歌詞中輸入任何數字/數字名稱。 - 請記住,當您還剩下 1 瓶時,「瓶子」一詞將變為單數。 ### 魔術8球 - 模擬神奇的 8 球。 - 允許使用者輸入他們的問題。 - 顯示正在進行的訊息(即“思考”)。 - 建立 20 個回應,並顯示隨機回應。 - 允許用戶提出另一個問題或退出。 - 獎金: ``` - Add a gui. ``` ``` - It must have a box for users to enter the question. ``` ``` - It must have at least 4 buttons: ``` ``` - ask ``` ``` - clear (the text box) ``` ``` - play again ``` ``` - quit (this must close the window) ``` ### 石頭剪刀布遊戲 - 建立一個石頭剪刀布遊戲。 - 讓玩家選擇石頭、剪刀或布。 - 讓計算機選擇它的移動方式。 - 比較選擇並決定誰獲勝。 - 列印結果。 - 子目標: ``` - Give the player the option to play again. ``` ``` - Keep a record of the score (e.g. Player: 3 / Computer: 6). ``` ### 倒數時鐘 - 建立一個程序,允許使用者選擇時間和日期,然後以給定的時間間隔(例如每秒)列印一條訊息,告訴使用者距離所選時間還有多長時間。 - 子目標: ``` - If the selected time has already passed, have the program tell the user to start over. ``` ``` - If your program asks for the year, month, day, hour, etc. separately, allow the user to be able to type in either the month name or its number. ``` ``` - TIP: Making use of built in modules such as time and datetime can change this project from a nightmare into a much simpler task. ``` 中等的 --- ### 番茄計時器 建立一個番茄計時器。 番茄計時器是一種時間管理方法。該技術使用計時器將工作分解為多個時間間隔,通常長度為 25 分鐘,中間間隔短暫的休息。這些間隔被命名為“pomodoros”,是意大利語單字“pomodoro”(番茄)的英文複數形式,以西里洛在大學時使用的番茄形狀的廚房計時器命名。 原始技巧有六個步驟: 決定要完成的任務。 設定番茄計時器(傳統上為 25 分鐘)。 完成任務。 當計時器響起時結束工作並在一張紙上畫上複選標記。 如果您的複選標記少於四個,請短暫休息(3-5 分鐘),然後轉到步驟 2。 四個番茄鐘後,休息較長時間(15-30 分鐘),將複選標記計數重設為零,然後轉到步驟 1。 要了解有關番茄計時器的更多訊息[,請單擊此處](https://en.wikipedia.org/wiki/Pomodoro_Technique) ### 谷歌案例 - 這是一個可以讓你玩英文句子的遊戲。 - 使用者將以任何格式輸入一個句子。(大寫或小寫或兩者的混合) - 程式必須將給定的句子轉換為Google大小寫。什麼是Google大小寫句子風格?\[know\_about\_it\_here:\](這是一種寫作風格,我們將所有小寫字母替換為大寫字母,留下所有單字的首字母)。 - 子目標: ``` - Program must then convert the given sentence in camel case.To know more about camel case ``` ``` [click_here](https://en.wikipedia.org/wiki/Camel_case) ``` ``` - Sentence can be entered with any number of spaces. ``` ### 擲骰子模擬器 - 允許使用者輸入骰子的面數以及應擲骰子的次數。 - 您的程式應該模擬擲骰子並追蹤每個數字出現的次數(這不必顯示)。 - 最後,列印出每個數字出現的次數。 - 子目標: ``` - Adjust your program so that if the user does not type in a number when they need to, the program will keep prompting them to type in a real number until they do so. ``` ``` - Put the program into a loop so that the user can continue to simulate dice rolls without having to restart the entire program. ``` ``` - In addition to printing out how many times each side appeared, also print out the percentage it appeared. If you can, round the percentage to 4 digits total OR two decimal places. ``` - 獎金: ``` - You are about to play a board game, but you realize you don't have any dice. Fortunately you have this program. ``` ``` - 1. Create a program that opens a new window and draws 2 six-sided dice ``` ``` - 2. Allow the user to quit, or roll again ``` ``` - Allow the user to select the number of dice to be drawn on screen(1-4) 2. Add up the total of the dice and display it ``` ### 計算並修復綠雞蛋和火腿 你們有些人可能還記得蘇博士的故事「綠雞蛋和火腿」。對於那些不記得或從未聽說過的人,[這](http://pastebin.com/XMY48CnN)是這個故事。然而,我給你的故事有一個問題——每次使用「我」這個詞時,它都是小寫的。 由於此問題,您的工作是執行以下操作: - 將我給您的故事複製到常規文字檔案中。 - 建立一個程式來通讀故事並在任何時候將字母 i 變為大寫。 (當它也用在 sam-I-am 的名字中時,請務必更改它。) - 讓你的程式建立一個新文件,並讓它正確地寫出故事。 - 印出有多少錯誤被修正。 - 完成後,您應該已經糾正了[這麼多](https://i.imgur.com/GRkj3yz.jpg)錯誤。 難的 -- ### 隨機維基百科文章 如果您曾造訪維基百科,您可能已經注意到螢幕左側有一個指向隨機文章的連結。雖然看到您被帶到哪篇文章可能很有趣,但有時看到文章的名稱會很好,這樣您就可以在聽起來很無聊時跳過它。幸運的是,維基百科有一個 API,允許我們這樣做[點擊這裡](https://en.wikipedia.org/w/api.php?action=query&list=random&rnnamespace=0&rnlimit=10&format=json)。 然而,有一個困境。由於維基百科擁有有關世界各地主題的文章,其中一些文章的標題中包含特殊字元。例如,關於西班牙畫家[埃拉斯托·科爾特斯·華雷斯 (Erasto Cortés Juárez)](https://en.wikipedia.org/wiki/Erasto_Cort%C3%A9s_Ju%C3%A1rez)的文章中就有 é 和 á。如果您查看這篇特定文章的[API](https://en.wikipedia.org/w/api.php?action=query&prop=info&pageids=39608394&inprop=url&format=json) ,您將看到標題是“Erasto Cort\\u00e9s Ju\\u00e1rez”,並且 \\u00e9 和 \\u00e1 正在替換前面提到的兩個字母。 (有關這是什麼的訊息,請首先查看文件中[本頁](https://docs.python.org/2/howto/unicode.html)的前半部分)。為了讓你的程式正常運作,你必須以某種方式處理這個問題。 - 建立一個程序,從官方維基百科 API 中提取標題,然後一一詢問用戶是否願意閱讀該文章。 - 例子: ``` - If the first title is Reddit, then the program should ask something along the lines of "Would you like to read about Reddit?" If the user says yes, then the program should open up the article for the user to read. ``` ``` - HINT: Click [here](https://en.wikipedia.org/wiki?curid=39608394) to see how the article's ID can be used to access the actual article. ``` - 子目標: ``` - As mentioned before, do something about the possibility of unicode appearing in the title. ``` ``` - Whether you want your program to simply filter out these articles or you want to actually turn the codes into readable characters, that's up to you. ``` ``` - Make the program pause once the user has selected an article to read, and allow him or her to continue browsing different article titles once finished reading. ``` ``` - Allow the user to simply press ENTER to be asked about a new article. ``` ### 天氣如何? 如果您想了解 API 的基礎知識,請查看 iamapizza 的[這篇](http://www.reddit.com/r/explainlikeimfive/comments/qowts/eli5_what_is_api/c3z9kok)文章。 - 建立一個程序,從 OpenWeatherMap.org 提取資料並列印有關當前天氣的訊息,例如您居住的地方的最高氣溫、最低氣溫和雨量。 - 子目標: ``` - Print out data for the next 5-7 days so you have a 5 day/week long forecast. ``` ``` - Print the data to another file that you can open up and view at, instead of viewing the information in the command line. ``` ``` - If you know html, write a file that you can print information to so that your project is more interesting. ``` - 尖端: ``` - APIs that are in Json are essentially lists and dictionaries. Remember that to reference something in a list, you must refer to it by what number element it is in the list, and to reference a key in a dictionary, you must refer to it by its name. ``` ``` - Don't like Celsius? Add &units=imperial to the end of the URL of the API to receive your data in Fahrenheit. ``` ### 來源 - https://github.com/tuvtran/project-based-learning - https://github.com/jorgegonzalez/beginner-projects - https://github.com/MunGell/awesome-for-beginners/blob/master/README.md - https://github.com/sarahbohr/AbsoluteBeginnerProjects --- 你怎麼認為?您喜歡透過特定專案進行學習還是不喜歡透過特定專案進行學習? --- 原文出處:https://dev.to/samborick/100-project-ideas-oda
> **2019 年 9 月 25 日更新:**感謝[ラナ・kuaru](https://twitter.com/rana_kualu)的辛勤工作,本文現已提供日文版。請點擊下面的連結查看他們的工作。如果您知道本文被翻譯成其他語言,請告訴我,我會將其發佈在這裡。 [🇯🇵 閱讀日語](https://qiita.com/rana_kualu/items/7b62898d373901466f5c) > **2019 年 7 月 8 日更新:**我最近發現大約兩年前發佈在法語留言板上的[這篇非常相似的文章](https://bookmarks.ecyseo.net/?EAWvDw)。如果您有興趣學習一些 shell 命令——並且您*會說 français* ,那麼它是對我下面的文章的一個很好的補充。 直到大約一年前,我幾乎只在 macOS 和 Ubuntu 作業系統中工作。在這兩個作業系統上, `bash`是我的預設 shell。在過去的六、七年裡,我對`bash`工作原理有了大致的了解,並想為那些剛入門的人概述一些更常見/有用的命令。如果您認為您了解有關`bash`所有訊息,請無論如何看看下面的內容 - 我已經提供了一些提示和您可能忘記的標誌的提醒,這可以讓您的工作更輕鬆一些。 下面的命令或多或少以敘述風格排列,因此如果您剛開始使用`bash` ,您可以從頭到尾完成操作。事情到最後通常會變得不那麼常見並且變得更加困難。 <a name="toc"></a> 目錄 -- - [基礎](#the-basics) ``` - [First Commands, Navigating the Filesystem](#first-commands) ``` ``` - [`pwd / ls / cd`](#pwd-ls-cd) ``` ``` - [`; / && / &`](#semicolon-andand-and) ``` ``` - [Getting Help](#getting-help) ``` ``` - [`-h`](#minus-h) ``` ``` - [`man`](#man) ``` ``` - [Viewing and Editing Files](#viewing-and-editing-files) ``` ``` - [`head / tail / cat / less`](#head-tail-cat-less) ``` ``` - [`nano / nedit`](#nano-nedit) ``` ``` - [Creating and Deleting Files and Directories](#creating-and-deleting-files) ``` ``` - [`touch`](#touch) ``` ``` - [`mkdir / rm / rmdir`](#mkdir-rm-rmdir) ``` ``` - [Moving and Copying Files, Making Links, Command History](#moving-and-copying-files) ``` ``` - [`mv / cp / ln`](#mv-cp-ln) ``` ``` - [Command History](#command-history) ``` ``` - [Directory Trees, Disk Usage, and Processes](#directory-trees-disk-usage-processes) ``` ``` - [`mkdir –p / tree`](#mkdir--p-tree) ``` ``` - [`df / du / ps`](#df-du-ps) ``` ``` - [Miscellaneous](#basic-misc) ``` ``` - [`passwd / logout / exit`](#passwd-logout-exit) ``` ``` - [`clear / *`](#clear-glob) ``` - [中間的](#intermediate) ``` - [Disk, Memory, and Processor Usage](#disk-memory-processor) ``` ``` - [`ncdu`](#ncdu) ``` ``` - [`top / htop`](#top-htop) ``` ``` - [REPLs and Software Versions](#REPLs-software-versions) ``` ``` - [REPLs](#REPLs) ``` ``` - [`-version / --version / -v`](#version) ``` ``` - [Environment Variables and Aliases](#env-vars-aliases) ``` ``` - [Environment Variables](#env-vars) ``` ``` - [Aliases](#aliases) ``` ``` - [Basic `bash` Scripting](#basic-bash-scripting) ``` ``` - [`bash` Scripts](#bash-scripts) ``` ``` - [Custom Prompt and `ls`](#custom-prompt-ls) ``` ``` - [Config Files](#config-files) ``` ``` - [Config Files / `.bashrc`](#config-bashrc) ``` ``` - [Types of Shells](#types-of-shells) ``` ``` - [Finding Things](#finding-things) ``` ``` - [`whereis / which / whatis`](#whereis-which-whatis) ``` ``` - [`locate / find`](#locate-find) ``` ``` - [Downloading Things](#downloading-things) ``` ``` - [`ping / wget / curl`](#ping-wget-curl) ``` ``` - [`apt / gunzip / tar / gzip`](#apt-gunzip-tar-gzip) ``` ``` - [Redirecting Input and Output](#redirecting-io) ``` ``` - [`| / > / < / echo / printf`](#pipe-gt-lt-echo-printf) ``` ``` - [`0 / 1 / 2 / tee`](#std-tee) ``` - [先進的](#advanced) ``` - [Superuser](#superuser) ``` ``` - [`sudo / su`](#sudo-su) ``` ``` - [`!!`](#click-click) ``` ``` - [File Permissions](#file-permissions) ``` ``` - [File Permissions](#file-permissions-sub) ``` ``` - [`chmod / chown`](#chmod-chown) ``` ``` - [User and Group Management](#users-groups) ``` ``` - [Users](#users) ``` ``` - [Groups](#groups) ``` ``` - [Text Processing](#text-processing) ``` ``` - [`uniq / sort / diff / cmp`](#uniq-sort-diff-cmp) ``` ``` - [`cut / sed`](#cut-sed) ``` ``` - [Pattern Matching](#pattern-matching) ``` ``` - [`grep`](#grep) ``` ``` - [`awk`](#awk) ``` ``` - [Copying Files Over `ssh`](#ssh) ``` ``` - [`ssh / scp`](#ssh-scp) ``` ``` - [`rsync`](#rsync) ``` ``` - [Long-Running Processes](#long-running-processes) ``` ``` - [`yes / nohup / ps / kill`](#yes-nohup-ps-kill) ``` ``` - [`cron / crontab / >>`](#cron) ``` ``` - [Miscellaneous](#advanced-misc) ``` ``` - [`pushd / popd`](#pushd-popd) ``` ``` - [`xdg-open`](#xdg-open) ``` ``` - [`xargs`](#xargs) ``` - [獎勵:有趣但大多無用的東西](#bonus) ``` - [`w / write / wall / lynx`](#w-write-wall-lynx) ``` ``` - [`nautilus / date / cal / bc`](#nautilus-date-cal-bc) ``` --- <a name="the-basics"></a> 基礎 == <a name="first-commands"></a> 第一個指令,瀏覽檔案系統 ------------ 現代檔案系統具有目錄(資料夾)樹,其中目錄要么是*根目錄*(沒有父目錄),要么是*子目錄*(包含在單一其他目錄中,我們稱之為“父目錄”)。向後遍歷檔案樹(從子目錄到父目錄)將始終到達根目錄。有些檔案系統有多個根目錄(如 Windows 的磁碟機: `C:\` 、 `A:\`等),但 Unix 和類別 Unix 系統只有一個名為`\`的根目錄。 <a name="pwd-ls-cd"></a> ### `pwd / ls / cd` [\[ 返回目錄 \]](#toc) 在檔案系統中工作時,使用者始終*在*某個目錄中工作,我們稱之為當前目錄或*工作目錄*。使用`pwd`列印使用者的工作目錄: ``` [ andrew@pc01 ~ ]$ pwd /home/andrew ``` 使用`ls`列出該目錄的內容(檔案和/或子目錄等): ``` [ andrew@pc01 ~ ]$ ls Git TEST jdoc test test.file ``` > **獎金:** > > 使用`ls -a`顯示隱藏(“點”)文件 > > 使用`ls -l`顯示文件詳細訊息 > > 組合多個標誌,如`ls -l -a` > > 有時您可以連結諸如`ls -la`之類的標誌,而不是`ls -l -a` 使用`cd`更改到不同的目錄(更改目錄): ``` [ andrew@pc01 ~ ]$ cd TEST/ [ andrew@pc01 TEST ]$ pwd /home/andrew/TEST [ andrew@pc01 TEST ]$ cd A [ andrew@pc01 A ]$ pwd /home/andrew/TEST/A ``` `cd ..`是「 `cd`到父目錄」的簡寫: ``` [ andrew@pc01 A ]$ cd .. [ andrew@pc01 TEST ]$ pwd /home/andrew/TEST ``` `cd ~`或只是`cd`是「 `cd`到我的主目錄」的簡寫(通常`/home/username`或類似的東西): ``` [ andrew@pc01 TEST ]$ cd [ andrew@pc01 ~ ]$ pwd /home/andrew ``` > **獎金:** > > `cd ~user`表示「 `cd`到`user`的主目錄 > > 您可以使用`cd ../..`等跳轉多個目錄等級。 > > 使用`cd -`返回到最近的目錄 > > `.`是「此目錄」的簡寫,因此`cd .`不會做太多事情 <a name="semicolon-andand-and"></a> ### `; / && / &` [\[ 返回目錄 \]](#toc) 我們在命令列中輸入的內容稱為*命令*,它們總是執行儲存在電腦上某處的一些機器碼。有時這個機器碼是一個內建的Linux命令,有時它是一個應用程式,有時它是你自己寫的一些程式碼。有時,我們會想依序執行一個指令。為此,我們可以使用`;` (分號): ``` [ andrew@pc01 ~ ]$ ls; pwd Git TEST jdoc test test.file /home/andrew ``` 上面的分號表示我首先 ( `ls` ) 列出工作目錄的內容,然後 ( `pwd` ) 列印其位置。連結命令的另一個有用工具是`&&` 。使用`&&`時,如果左側命令失敗,則右側命令將不會執行。 `;`和`&&`都可以在同一行中多次使用: ``` # whoops! I made a typo here! [ andrew@pc01 ~ ]$ cd /Giit/Parser && pwd && ls && cd -bash: cd: /Giit/Parser: No such file or directory # the first command passes now, so the following commands are run [ andrew@pc01 ~ ]$ cd Git/Parser/ && pwd && ls && cd /home/andrew/Git/Parser README.md doc.sh pom.xml resource run.sh shell.sh source src target ``` ....但是與`;` ,即使第一個命令失敗,第二個命令也會執行: ``` # pwd and ls still run, even though the cd command failed [ andrew@pc01 ~ ]$ cd /Giit/Parser ; pwd ; ls -bash: cd: /Giit/Parser: No such file or directory /home/andrew Git TEST jdoc test test.file ``` `&`看起來與`&&`類似,但實際上實現了完全不同的功能。通常,當您執行長時間執行的命令時,命令列將等待該命令完成,然後才允許您輸入另一個命令。在命令後面加上`&`可以防止這種情況發生,並允許您在舊命令仍在執行時執行新命令: ``` [ andrew@pc01 ~ ]$ cd Git/Parser && mvn package & cd [1] 9263 ``` > **額外的好處:**當我們在命令後使用`&`來「隱藏」它時,我們說該作業(或「進程」;這些術語或多或少可以互換)是「後台的」。若要查看目前正在執行的背景作業,請使用`jobs`指令: > ````bash \[ andrew@pc01 ~ \]$ 職位 \[1\]+ 執行 cd Git/Parser/ && mvn package & ``` <a name="getting-help"></a> ## Getting Help <a name="minus-h"></a> ### `-h` [[ Back to Table of Contents ]](#toc) Type `-h` or `--help` after almost any command to bring up a help menu for that command: ``` \[ andrew@pc01 ~ \]$ du --help 用法:你\[選項\]...\[檔案\]... 或: du \[選項\]... --files0-from=F 對目錄遞歸地總結文件集的磁碟使用情況。 長期權的強制性參數對於短期權也是強制性的。 -0, --null 以 NUL 結束每個輸出行,而不是換行符 -a, --all 計算所有檔案的寫入計數,而不僅僅是目錄 ``` --apparent-size print apparent sizes, rather than disk usage; although ``` ``` the apparent size is usually smaller, it may be ``` ``` larger due to holes in ('sparse') files, internal ``` ``` fragmentation, indirect blocks, and the like ``` -B, --block-size=SIZE 在列印前按 SIZE 縮放大小;例如, ``` '-BM' prints sizes in units of 1,048,576 bytes; ``` ``` see SIZE format below ``` … ``` <a name="man"></a> ### `man` [[ Back to Table of Contents ]](#toc) Type `man` before almost any command to bring up a manual for that command (quit `man` with `q`): ``` LS(1) 使用者指令 LS(1) 姓名 ``` ls - list directory contents ``` 概要 ``` ls [OPTION]... [FILE]... ``` 描述 ``` List information about the FILEs (the current directory by default). ``` ``` Sort entries alphabetically if none of -cftuvSUX nor --sort is speci- ``` ``` fied. ``` ``` Mandatory arguments to long options are mandatory for short options ``` ``` too. ``` … ``` <a name="viewing-and-editing-files"></a> ## Viewing and Editing Files <a name="head-tail-cat-less"></a> ### `head / tail / cat / less` [[ Back to Table of Contents ]](#toc) `head` outputs the first few lines of a file. The `-n` flag specifies the number of lines to show (the default is 10): ``` 列印前三行 ===== \[ andrew@pc01 ~ \]$ 頭 -n 3 c 這 文件 有 ``` `tail` outputs the last few lines of a file. You can get the last `n` lines (like above), or you can get the end of the file beginning from the `N`-th line with `tail -n +N`: ``` 從第 4 行開始列印文件末尾 ============== \[ andrew@pc01 ~ \]$ tail -n +4 c 確切地 六 線 ``` `cat` concatenates a list of files and sends them to the standard output stream (usually the terminal). `cat` can be used with just a single file, or multiple files, and is often used to quickly view them. (**Be warned**: if you use `cat` in this way, you may be accused of a [_Useless Use of Cat_ (UUOC)](http://bit.ly/2SPHE4V), but it's not that big of a deal, so don't worry too much about it.) ``` \[ andrew@pc01 ~ \]$ 貓 a 歸檔一個 \[ andrew@pc01 ~ \]$ 貓 ab 歸檔一個 文件b ``` `less` is another tool for quickly viewing a file -- it opens up a `vim`-like read-only window. (Yes, there is a command called `more`, but `less` -- unintuitively -- offers a superset of the functionality of `more` and is recommended over it.) Learn more (or less?) about [less](http://man7.org/linux/man-pages/man1/less.1.html) and [more](http://man7.org/linux/man-pages/man1/more.1.html) at their `man` pages. <a name="nano-nedit"></a> ### `nano / nedit` [[ Back to Table of Contents ]](#toc) `nano` is a minimalistic command-line text editor. It's a great editor for beginners or people who don't want to learn a million shortcuts. It was more than sufficient for me for the first few years of my coding career (I'm only now starting to look into more powerful editors, mainly because defining your own syntax highlighting in `nano` can be a bit of a pain.) `nedit` is a small graphical editor, it opens up an X Window and allows point-and-click editing, drag-and-drop, syntax highlighting and more. I use `nedit` sometimes when I want to make small changes to a script and re-run it over and over. Other common CLI (command-line interface) / GUI (graphical user interface) editors include `emacs`, `vi`, `vim`, `gedit`, Notepad++, Atom, and lots more. Some cool ones that I've played around with (and can endorse) include Micro, Light Table, and VS Code. All modern editors offer basic conveniences like search and replace, syntax highlighting, and so on. `vi(m)` and `emacs` have more features than `nano` and `nedit`, but they have a much steeper learning curve. Try a few different editors out and find one that works for you! <a name="creating-and-deleting-files"></a> ## Creating and Deleting Files and Directories <a name="touch"></a> ### `touch` [[ Back to Table of Contents ]](#toc) `touch` was created to modify file timestamps, but it can also be used to quickly create an empty file. You can create a new file by opening it with a text editor, like `nano`: ``` \[ andrew@pc01 前 \]$ ls \[ andrew@pc01 ex \]$ 奈米 a ``` _...editing file..._ ``` \[ andrew@pc01 前 \]$ ls A ``` ...or by simply using `touch`: ``` \[ andrew@pc01 ex \]$ touch b && ls ab ``` > **Bonus**: > > Background a process with \^z (Ctrl+z) > > ```bash > [ andrew@pc01 ex ]$ nano a > ``` > > _...editing file, then hit \^z..._ > > ```bash > Use fg to return to nano > > [1]+ Stopped nano a > [ andrew@pc01 ex ]$ fg > ``` > > _...editing file again..._ --- > **Double Bonus:** > > Kill the current (foreground) process by pressing \^c (Ctrl+c) while it’s running > > Kill a background process with `kill %N` where `N` is the job index shown by the `jobs` command <a name="mkdir-rm-rmdir"></a> ### `mkdir / rm / rmdir` [[ Back to Table of Contents ]](#toc) `mkdir` is used to create new, empty directories: ``` \[ andrew@pc01 ex \]$ ls && mkdir c && ls ab ABC ``` You can remove any file with `rm` -- but be careful, this is non-recoverable! ``` \[ andrew@pc01 ex \]$ rm a && ls 西元前 ``` You can add an _"are you sure?"_ prompt with the `-i` flag: ``` \[ andrew@pc01 前 \]$ rm -ib rm:刪除常規空文件“b”? y ``` Remove an empty directory with `rmdir`. If you `ls -a` in an empty directory, you should only see a reference to the directory itself (`.`) and a reference to its parent directory (`..`): ``` \[ andrew@pc01 ex \]$ rmdir c && ls -a 。 .. ``` `rmdir` removes empty directories only: ``` \[ andrew@pc01 ex \]$ cd .. && ls 測試/ \*.txt 0.txt 1.txt a a.txt bc \[ andrew@pc01 ~ \]$ rmdir 測試/ rmdir:無法刪除“test/”:目錄不為空 ``` ...but you can remove a directory -- and all of its contents -- with `rm -rf` (`-r` = recursive, `-f` = force): ``` \[ andrew@pc01 ~ \]$ rm –rf 測試 ``` <a name="moving-and-copying-files"></a> ## Moving and Copying Files, Making Links, Command History <a name="mv-cp-ln"></a> ### `mv / cp / ln` [[ Back to Table of Contents ]](#toc) `mv` moves / renames a file. You can `mv` a file to a new directory and keep the same file name or `mv` a file to a "new file" (rename it): ``` \[ andrew@pc01 ex \]$ ls && mv ae && ls A B C D BCDE ``` `cp` copies a file: ``` \[ andrew@pc01 ex \]$ cp e e2 && ls BCDE E2 ``` `ln` creates a hard link to a file: ``` ln 的第一個參數是 TARGET,第二個參數是 NEW LINK ================================= \[ andrew@pc01 ex \]$ ln bf && ls bcde e2 f ``` `ln -s` creates a soft link to a file: ``` \[ andrew@pc01 ex \]$ ln -sbg && ls BCDE E2 FG ``` Hard links reference the same actual bytes in memory which contain a file, while soft links refer to the original file name, which itself points to those bytes. [You can read more about soft vs. hard links here.](http://bit.ly/2D0W8cN) <a name="command-history"></a> ### Command History [[ Back to Table of Contents ]](#toc) `bash` has two big features to help you complete and re-run commands, the first is _tab completion_. Simply type the first part of a command, hit the \<tab\> key, and let the terminal guess what you're trying to do: ``` \[ andrew@pc01 目錄 \]$ ls 另一個長檔名 這是一個長檔名 一個新檔名 \[ andrew@pc01 目錄 \]$ ls t ``` _...hit the TAB key after typing `ls t` and the command is completed..._ ``` \[ andrew@pc01 dir \]$ ls 這是檔名 這是長檔名 ``` You may have to hit \<TAB\> multiple times if there's an ambiguity: ``` \[ andrew@pc01 目錄 \]$ ls a \[ andrew@pc01 目錄 \]$ ls an 一個新檔名另一個長檔名 ``` `bash` keeps a short history of the commands you've typed previously and lets you search through those commands by typing \^r (Ctrl+r): ``` \[ andrew@pc01 目錄 \] ``` _...hit \^r (Ctrl+r) to search the command history..._ ``` (反向搜尋)``: ``` _...type 'anew' and the last command containing this is found..._ ``` (reverse-i-search)`anew': 觸碰新檔名 ``` <a name="directory-trees-disk-usage-processes"></a> ## Directory Trees, Disk Usage, and Processes <a name="mkdir--p-tree"></a> ### `mkdir –p / tree` [[ Back to Table of Contents ]](#toc) `mkdir`, by default, only makes a single directory. This means that if, for instance, directory `d/e` doesn't exist, then `d/e/f` can't be made with `mkdir` by itself: ``` \[ andrew@pc01 ex \]$ ls && mkdir d/e/f ABC mkdir:無法建立目錄「d/e/f」:沒有這樣的檔案或目錄 ``` But if we pass the `-p` flag to `mkdir`, it will make all directories in the path if they don't already exist: ``` \[ andrew@pc01 ex \]$ mkdir -pd/e/f && ls A B C D ``` `tree` can help you better visualise a directory's structure by printing a nicely-formatted directory tree. By default, it prints the entire tree structure (beginning with the specified directory), but you can restrict it to a certain number of levels with the `-L` flag: ``` \[ andrew@pc01 前 \]$ 樹 -L 2 。 |-- 一個 |-- b |-- c `--d ``` `--e ``` 3個目錄,2個文件 ``` You can hide empty directories in `tree`'s output with `--prune`. Note that this also removes "recursively empty" directories, or directories which aren't empty _per se_, but which contain only other empty directories, or other recursively empty directories: ``` \[ andrew@pc01 ex \]$ 樹 --prune 。 |-- 一個 `--b ``` <a name="df-du-ps"></a> ### `df / du / ps` [[ Back to Table of Contents ]](#toc) `df` is used to show how much space is taken up by files for the disks or your system (hard drives, etc.). ``` \[ andrew@pc01 前 \]$ df -h 已使用的檔案系統大小 可用 使用% 安裝於 udev 126G 0 126G 0% /dev tmpfs 26G 2.0G 24G 8% /執行 /dev/mapper/ubuntu--vg-root 1.6T 1.3T 252G 84% / … ``` In the above command, `-h` doesn't mean "help", but "human-readable". Some commands use this convention to display file / disk sizes with `K` for kilobytes, `G` for gigabytes, and so on, instead of writing out a gigantic integer number of bytes. `du` shows file space usage for a particular directory and its subdirectories. If you want to know how much space is free on a given hard drive, use `df`; if you want to know how much space a directory is taking up, use `du`: ``` \[ andrew@pc01 ex \]$ 你 4 ./d/e/f 8./d/e 12 ./天 4./c 20 . ``` `du` takes a `--max-depth=N` flag, which only shows directories `N` levels down (or fewer) from the specified directory: ``` \[ andrew@pc01 ex \]$ du -h --max-深度=1 12K./天 4.0K./c 20K。 ``` `ps` shows all of the user's currently-running processes (aka. jobs): ``` \[ andrew@pc01 前 \]$ ps PID TTY 時間 CMD 16642 分/15 00:00:00 ps 25409 點/15 00:00:00 重擊 ``` <a name="basic-misc"></a> ## Miscellaneous <a name="passwd-logout-exit"></a> ### `passwd / logout / exit` [[ Back to Table of Contents ]](#toc) Change your account password with `passwd`. It will ask for your current password for verification, then ask you to enter the new password twice, so you don't make any typos: ``` \[ andrew@pc01 目錄 \]$ 密碼 更改安德魯的密碼。 (目前)UNIX 密碼: 輸入新的 UNIX 密碼: 重新輸入新的 UNIX 密碼: passwd:密碼更新成功 ``` `logout` exits a shell you’ve logged in to (where you have a user account): ``` \[ andrew@pc01 目錄 \]$ 註銷 ────────────────────────────────────────────────── ── ────────────────────────────── 會話已停止 ``` - Press <return> to exit tab ``` ``` - Press R to restart session ``` ``` - Press S to save terminal output to file ``` ``` `exit` exits any kind of shell: ``` \[ andrew@pc01 ~ \]$ 退出 登出 ────────────────────────────────────────────────── ── ────────────────────────────── 會話已停止 ``` - Press <return> to exit tab ``` ``` - Press R to restart session ``` ``` - Press S to save terminal output to file ``` ``` <a name="clear-glob"></a> ### `clear / *` [[ Back to Table of Contents ]](#toc) Run `clear` to move the current terminal line to the top of the screen. This command just adds blank lines below the current prompt line. It's good for clearing your workspace. Use the glob (`*`, aka. Kleene Star, aka. wildcard) when looking for files. Notice the difference between the following two commands: ``` \[ andrew@pc01 ~ \]$ ls Git/Parser/source/ PArrayUtils.java PFile.java PSQLFile.java PWatchman.java PDateTimeUtils.java PFixedWidthFile.java PStringUtils.java PXSVFile.java PDelimitedFile.java PNode.java PTextFile.java Parser.java \[ andrew@pc01 ~ \]$ ls Git/Parser/source/PD\* Git/Parser/source/PDateTimeUtils.java Git/Parser/source/PDelimitedFile.java ``` The glob can be used multiple times in a command and matches zero or more characers: ``` \[ andrew@pc01 ~ \]$ ls Git/Parser/source/P *D* m\* Git/Parser/source/PDateTimeUtils.java Git/Parser/source/PDelimitedFile.java ``` <a name="intermediate"></a> # Intermediate <a name="disk-memory-processor"></a> ## Disk, Memory, and Processor Usage <a name="ncdu"></a> ### `ncdu` [[ Back to Table of Contents ]](#toc) `ncdu` (NCurses Disk Usage) provides a navigable overview of file space usage, like an improved `du`. It opens a read-only `vim`-like window (press `q` to quit): ``` \[ andrew@pc01 ~ \]$ ncdu ncdu 1.11 ~ 使用箭頭鍵導航,按 ?求助 \--- /home/安德魯 ------------------------------------------- ------------------ 148.2 MiB \[##########\] /.m2 91.5 MiB \[######\] /.sbt 79.8 MiB \[######\] /.cache 64.9 MiB \[####\] /.ivy2 40.6 MiB \[##\] /.sdkman 30.2 MiB \[##\] /.local 27.4 MiB \[#\] /.mozilla 24.4 MiB \[#\] /.nanobackups 10.2 MiB \[ \] .confout3.txt ``` 8.4 MiB [ ] /.config ``` ``` 5.9 MiB [ ] /.nbi ``` ``` 5.8 MiB [ ] /.oh-my-zsh ``` ``` 4.3 MiB [ ] /Git ``` ``` 3.7 MiB [ ] /.myshell ``` ``` 1.7 MiB [ ] /jdoc ``` ``` 1.5 MiB [ ] .confout2.txt ``` ``` 1.5 MiB [ ] /.netbeans ``` ``` 1.1 MiB [ ] /.jenv ``` 564.0 KiB \[ \] /.rstudio-desktop 磁碟使用總量:552.7 MiB 表觀大小:523.6 MiB 專案:14618 ``` <a name="top-htop"></a> ### `top / htop` [[ Back to Table of Contents ]](#toc) `top` displays all currently-running processes and their owners, memory usage, and more. `htop` is an improved, interactive `top`. (Note: you can pass the `-u username` flag to restrict the displayed processes to only those owner by `username`.) ``` \[ andrew@pc01 ~ \]$ htop 1 \[ 0.0%\] 9 \[ 0.0%\] 17 \[ 0.0%\] 25 \[ 0.0%\] 2 \[ 0.0%\] 10 \[ 0.0%\] 18 \[ 0.0%\] 26 \[ 0.0%\] 3 \[ 0.0%\] 11 \[ 0.0%\] 19 \[ 0.0%\] 27 \[ 0.0%\] 4 \[ 0.0%\] 12 \[ 0.0%\] 20 \[ 0.0%\] 28 \[ 0.0%\] 5 \[ 0.0%\] 13 \[ 0.0%\] 21 \[| 1.3%\] 29 \[ 0.0%\] 6 \[ 0.0%\] 14 \[ 0.0%\] 22 \[ 0.0%\] 30 \[| 0.6%\] 7 \[ 0.0%\] 15 \[ 0.0%\] 23 \[ 0.0%\] 31 \[ 0.0%\] 8 \[ 0.0%\] 16 \[ 0.0%\] 24 \[ 0.0%\] 32 \[ 0.0%\] Mem\[|||||||||||||||||||1.42G/252G\] 任務:188、366 個; 1 執行 交換電壓\[| 2.47G/256G\]平均負載:0.00 0.00 0.00 ``` Uptime: 432 days(!), 00:03:55 ``` PID USER PRI NI VIRT RES SHR S CPU% MEM% TIME+ 指令 9389 安德魯 20 0 23344 3848 2848 R 1.3 0.0 0:00.10 htop 10103 根 20 0 3216M 17896 2444 S 0.7 0.0 5h48:56 /usr/bin/dockerd ``` 1 root 20 0 181M 4604 2972 S 0.0 0.0 15:29.66 /lib/systemd/syst ``` 533 根 20 0 44676 6908 6716 S 0.0 0.0 11:19.77 /lib/systemd/syst 546 根 20 0 244M 0 0 S 0.0 0.0 0:01.39 /sbin/lvmetad -f 1526 根 20 0 329M 2252 1916 S 0.0 0.0 0:00.00 /usr/sbin/ModemMa 1544 根 20 0 329M 2252 1916 S 0.0 0.0 0:00.06 /usr/sbin/ModemMa F1Help F2Setup F3SearchF4FilterF5Tree F6SortByF7Nice -F8Nice +F9Kill F10Quit ``` <a name="REPLs-software-versions"></a> ## REPLs and Software Versions <a name="REPLs"></a> ### REPLs [[ Back to Table of Contents ]](#toc) A **REPL** is a Read-Evaluate-Print Loop, similar to the command line, but usually used for particular programming languages. You can open the Python REPL with the `python` command (and quit with the `quit()` function): ``` \[ andrew@pc01 ~ \]$ python Python 3.5.2(默認,2018 年 11 月 12 日,13:43:14)... > > > 辭職() ``` Open the R REPL with the `R` command (and quit with the `q()` function): ``` \[ andrew@pc01 ~ \]$ R R版3.5.2(2018-12-20)--「蛋殼冰屋」... > q() 儲存工作區影像? \[是/否/c\]: 否 ``` Open the Scala REPL with the `scala` command (and quit with the `:quit` command): ``` \[ andrew@pc01 ~ \]$ scala 歡迎使用 Scala 2.11.12 ... 斯卡拉>:退出 ``` Open the Java REPL with the `jshell` command (and quit with the `/exit` command): ``` \[ andrew@pc01 ~ \]$ jshell |歡迎使用 JShell——版本 11.0.1 ... jshell> /退出 ``` Alternatively, you can exit any of these REPLs with \^d (Ctrl+d). \^d is the EOF (end of file) marker on Unix and signifies the end of input. <a name="version"></a> ### `-version / --version / -v` [[ Back to Table of Contents ]](#toc) Most commands and programs have a `-version` or `--version` flag which gives the software version of that command or program. Most applications make this information easily available: ``` \[ andrew@pc01 ~ \]$ ls --version ls (GNU coreutils) 8.25 ... \[ andrew@pc01 ~ \]$ ncdu -版本 NCDU 1.11 \[ andrew@pc01 ~ \]$ python --version Python 3.5.2 ``` ...but some are less intuitive: ``` \[ andrew@pc01 ~ \]$ sbt scalaVersion … \[資訊\]2.12.4 ``` Note that some programs use `-v` as a version flag, while others use `-v` to mean "verbose", which will run the application while printing lots of diagnostic or debugging information: ``` SCP(1) BSD 通用指令手冊 SCP(1) 姓名 ``` scp -- secure copy (remote file copy program) ``` … -v 詳細模式。導致 scp 和 ssh(1) 列印偵錯訊息 ``` about their progress. This is helpful in debugging connection, ``` ``` authentication, and configuration problems. ``` … ``` <a name="env-vars-aliases"></a> ## Environment Variables and Aliases <a name="env-vars"></a> ### Environment Variables [[ Back to Table of Contents ]](#toc) **Environment variables** (sometimes shortened to "env vars") are persistent variables that can be created and used within your `bash` shell. They are defined with an equals sign (`=`) and used with a dollar sign (`$`). You can see all currently-defined env vars with `printenv`: ``` \[ andrew@pc01 ~ \]$ printenv SPARK\_HOME=/usr/local/spark 術語=xterm … ``` Set a new environment variable with an `=` sign (don't put any spaces before or after the `=`, though!): ``` \[ andrew@pc01 ~ \]$ myvar=你好 ``` Print a specific env var to the terminal with `echo` and a preceding `$` sign: ``` \[ andrew@pc01 ~ \]$ echo $myvar 你好 ``` Environment variables which contain spaces or other whitespace should be surrounded by quotes (`"..."`). Note that reassigning a value to an env var overwrites it without warning: ``` \[ andrew@pc01 ~ \]$ myvar="你好,世界!" && 回顯 $myvar 你好世界! ``` Env vars can also be defined using the `export` command. When defined this way, they will also be available to sub-processes (commands called from this shell): ``` \[ andrew@pc01 ~ \]$ export myvar="另一" && echo $myvar 另一個 ``` You can unset an environment variable by leaving the right-hand side of the `=` blank or by using the `unset` command: ``` \[ andrew@pc01 ~ \]$ 取消設定 mynewvar \[ andrew@pc01 ~ \]$ echo $mynewvar ``` <a name="aliases"></a> ### Aliases [[ Back to Table of Contents ]](#toc) **Aliases** are similar to environment variables but are usually used in a different way -- to replace long commands with shorter ones: ``` \[ andrew@pc01 apidocs \]$ ls -l -a -h -t 總計 220K drwxr-xr-x 5 安德魯 安德魯 4.0K 12 月 21 日 12:37 。 -rw-r--r-- 1 安德魯 安德魯 9.9K 十二月 21 12:37 help-doc.html -rw-r--r-- 1 安德魯 安德魯 4.5K 12 月 21 日 12:37 script.js … \[ andrew@pc01 apidocs \]$ 別名 lc="ls -l -a -h -t" \[ andrew@pc01 apidocs \]$ lc 總計 220K drwxr-xr-x 5 安德魯 安德魯 4.0K 12 月 21 日 12:37 。 -rw-r--r-- 1 安德魯 安德魯 9.9K 十二月 21 12:37 help-doc.html -rw-r--r-- 1 安德魯 安德魯 4.5K 12 月 21 日 12:37 script.js … ``` You can remove an alias with `unalias`: ``` \[ andrew@pc01 apidocs \]$ unalias lc \[ andrew@pc01 apidocs \]$ lc 目前未安裝程式“lc”。 … ``` > **Bonus:** > > [Read about the subtle differences between environment variables and aliases here.](http://bit.ly/2TDG8Tx) > > [Some programs, like **git**, allow you to define aliases specifically for that software.](http://bit.ly/2TG8X1A) <a name="basic-bash-scripting"></a> ## Basic `bash` Scripting <a name="bash-scripts"></a> ### `bash` Scripts [[ Back to Table of Contents ]](#toc) `bash` scripts (usually ending in `.sh`) allow you to automate complicated processes, packaging them into reusable functions. A `bash` script can contain any number of normal shell commands: ``` \[ andrew@pc01 ~ \]$ echo "ls && touch file && ls" > ex.sh ``` A shell script can be executed with the `source` command or the `sh` command: ``` \[ andrew@pc01 ~ \]$ 源 ex.sh 桌面 Git TEST c ex.sh 專案測試 桌面 Git TEST c ex.sh 檔案專案測試 ``` Shell scripts can be made executable with the `chmod` command (more on this later): ``` \[ andrew@pc01 ~ \]$ echo "ls && touch file2 && ls" > ex2.sh \[ andrew@pc01 ~ \]$ chmod +x ex2.sh ``` An executable shell script can be run by preceding it with `./`: ``` \[ andrew@pc01 ~ \]$ ./ex2.sh 桌面 Git TEST c ex.sh ex2.sh 檔案專案測試 桌面 Git TEST c ex.sh ex2.sh 檔案 file2 專案測試 ``` Long lines of code can be split by ending a command with `\`: ``` \[ andrew@pc01 ~ \]$ echo "for i in {1..3}; do echo \\ > \\"歡迎\\$i次\\";完成” > ex3.sh ``` Bash scripts can contain loops, functions, and more! ``` \[ andrew@pc01 ~ \]$ 源 ex3.sh 歡迎1次 歡迎2次 歡迎3次 ``` <a name="custom-prompt-ls"></a> ### Custom Prompt and `ls` [[ Back to Table of Contents ]](#toc) Bash scripting can make your life a whole lot easier and more colourful. [Check out this great bash scripting cheat sheet.](https://devhints.io/bash) `$PS1` (Prompt String 1) is the environment variable that defines your main shell prompt ([learn about the other prompts here](http://bit.ly/2SPgsmT)): ``` \[ andrew@pc01 ~ \]$ printf "%q" $PS1 $'\\n\\\[\\E\[1m\\\]\\\[\\E\[30m\\\]\\A'$'\\\[\\E\[37m\\\]|\\\[\\E\[36m\\\]\\u\\\[\\E\[37m \\\]@\\\[\\E\[34m\\\]\\h'$'\\\[\\E\[32m\\\]\\W\\\[\\E\[37m\\\]|'$'\\\[\\E(B\\E\[m\\\] ' ``` You can change your default prompt with the `export` command: ``` \[ andrew@pc01 ~ \]$ export PS1="\\n此處指令> " 此處指令> echo $PS1 \\n此處指令> ``` ...[you can add colours, too!](http://bit.ly/2TMbEit): ``` 此處指令> export PS1="\\e\[1;31m\\n程式碼: \\e\[39m" (這應該是紅色的,但在 Markdown 中可能不會這樣顯示) =============================== 程式碼:回顯$PS1 \\e\[1;31m\\n程式碼: \\e\[39m ``` You can also change the colours shown by `ls` by editing the `$LS_COLORS` environment variable: ``` (同樣,這些顏色可能不會出現在 Markdown 中) =========================== 程式碼:ls 桌面 Git TEST c ex.sh ex2.sh ex3.sh 檔案 file2 專案測試 程式碼:匯出 LS\_COLORS='di=31:fi=0:ln=96:or=31:mi=31:ex=92' 程式碼:ls 桌面 Git TEST c ex.sh ex2.sh ex3.sh 檔案 file2 專案測試 ``` <a name="config-files"></a> ## Config Files <a name="config-bashrc"></a> ### Config Files / `.bashrc` [[ Back to Table of Contents ]](#toc) If you tried the commands in the last section and logged out and back in, you may have noticed that your changes disappeared. _config_ (configuration) files let you maintain settings for your shell or for a particular program every time you log in (or run that program). The main configuration file for a `bash` shell is the `~/.bashrc` file. Aliases, environment variables, and functions added to `~/.bashrc` will be available every time you log in. Commands in `~/.bashrc` will be run every time you log in. If you edit your `~/.bashrc` file, you can reload it without logging out by using the `source` command: ``` \[ andrew@pc01 ~ \]$ nano ~/.bashrc ``` _...add the line `echo “~/.bashrc loaded!”` to the top of the file_... ``` \[ andrew@pc01 ~ \]$ 源 ~/.bashrc ~/.bashrc 已載入! ``` _...log out and log back in..._ ``` 最後登入:2019 年 1 月 11 日星期五 10:29:07 從 111.11.11.111 ~/.bashrc 已加載! \[ 安德魯@pc01 ~ \] ``` <a name="types-of-shells"></a> ### Types of Shells [[ Back to Table of Contents ]](#toc) _Login_ shells are shells you log in to (where you have a username). _Interactive_ shells are shells which accept commands. Shells can be login and interactive, non-login and non-interactive, or any other combination. In addition to `~/.bashrc`, there are a few other scripts which are `sourced` by the shell automatically when you log in or log out. These are: - `/etc/profile` - `~/.bash_profile` - `~/.bash_login` - `~/.profile` - `~/.bash_logout` - `/etc/bash.bash_logout` Which of these scripts are sourced, and the order in which they're sourced, depend on the type of shell opened. See [the bash man page](https://linux.die.net/man/1/bash) and [these](http://bit.ly/2TGCwA8) Stack Overflow [posts](http://bit.ly/2TFHFsf) for more information. Note that `bash` scripts can `source` other scripts. For instance, in your `~/.bashrc`, you could include the line: ``` 來源~/.bashrc\_addl ``` ...which would also `source` that `.bashrc_addl` script. This file can contain its own aliases, functions, environment variables, and so on. It could, in turn, `source` other scripts, as well. (Be careful to avoid infinite loops of script-sourcing!) It may be helpful to split commands into different shell scripts based on functionality or machine type (Ubuntu vs. Red Hat vs. macOS), for example: - `~/.bash_ubuntu` -- configuration specific to Ubuntu-based machines - `~/.bashrc_styles` -- aesthetic settings, like `PS1` and `LS_COLORS` - `~/.bash_java` -- configuration specific to the Java language I try to keep separate `bash` files for aesthetic configurations and OS- or machine-specific code, and then I have one big `bash` file containing shortcuts, etc. that I use on every machine and every OS. Note that there are also _different shells_. `bash` is just one kind of shell (the "Bourne Again Shell"). Other common ones include `zsh`, `csh`, `fish`, and more. Play around with different shells and find one that's right for you, but be aware that this tutorial contains `bash` shell commands only and not everything listed here (maybe none of it) will be applicable to shells other than `bash`. <a name="finding-things"></a> ## Finding Things <a name="whereis-which-whatis"></a> ### `whereis / which / whatis` [[ Back to Table of Contents ]](#toc) `whereis` searches for "possibly useful" files related to a particular command. It will attempt to return the location of the binary (executable machine code), source (code source files), and `man` page for that command: ``` \[ andrew@pc01 ~ \]$ whereis ls ls: /bin/ls /usr/share/man/man1/ls.1.gz ``` `which` will only return the location of the binary (the command itself): ``` \[ andrew@pc01 ~ \]$ 其中 ls /bin/ls ``` `whatis` prints out the one-line description of a command from its `man` page: ``` \[ andrew@pc01 ~ \]$ 什麼是哪裡是哪個什麼是 whereis (1) - 尋找指令的二進位、原始檔和手冊頁文件 which (1) - 定位指令 Whatis (1) - 顯示一行手冊頁描述 ``` `which` is useful for finding the "original version" of a command which may be hidden by an alias: ``` \[ andrew@pc01 ~ \]$ 別名 ls="ls -l" “original” ls 已被上面定義的別名“隱藏” =========================== \[ andrew@pc01 ~ \]$ ls 總計 36 drwxr-xr-x 2 安德魯 andrew 4096 Jan 9 14:47 桌面 drwxr-xr-x 4 安德魯 安德魯 4096 十二月 6 10:43 Git … 但我們仍然可以使用返回的位置來呼叫「原始」ls ======================= \[ andrew@pc01 ~ \]$ /bin/ls 桌面 Git TEST c ex.sh ex2.sh ex3.sh 檔案 file2 專案測試 ``` <a name="locate-find"></a> ### `locate / find` [[ Back to Table of Contents ]](#toc) `locate` finds a file anywhere on the system by referring to a semi-regularly-updated cached list of files: ``` \[ andrew@pc01 ~ \]$ 找到 README.md /home/andrew/.config/micro/plugins/gotham-colors/README.md /home/andrew/.jenv/README.md /home/andrew/.myshell/README.md … ``` Because it's just searching a list, `locate` is usually faster than the alternative, `find`. `find` iterates through the file system to find the file you're looking for. Because it's actually looking at the files which _currently_ exist on the system, though, it will always return an up-to-date list of files, which is not necessarily true with `locate`. ``` \[ andrew@pc01 ~ \]$ find ~/ -iname "README.md" /home/andrew/.jenv/README.md /home/andrew/.config/micro/plugins/gotham-colors/README.md /home/andrew/.oh-my-zsh/plugins/ant/README.md … ``` `find` was written for the very first version of Unix in 1971, and is therefore much more widely available than `locate`, which was added to GNU in 1994. `find` has many more features than `locate`, and can search by file age, size, ownership, type, timestamp, permissions, depth within the file system; `find` can search using regular expressions, execute commands on files it finds, and more. When you need a fast (but possibly outdated) list of files, or you’re not sure what directory a particular file is in, use `locate`. When you need an accurate file list, maybe based on something other than the files’ names, and you need to do something with those files, use `find`. <a name="downloading-things"></a> ## Downloading Things <a name="ping-wget-curl"></a> ### `ping / wget / curl` [[ Back to Table of Contents ]](#toc) `ping` attempts to open a line of communication with a network host. Mainly, it's used to check whether or not your Internet connection is down: ``` \[ andrew@pc01 ~ \]$ ping google.com PING google.com (74.125.193.100) 56(84) 位元組資料。 使用 32 位元組資料 Ping 74.125.193.100: 來自 74.125.193.100 的回覆:位元組=32 時間<1ms TTL=64 … ``` `wget` is used to easily download a file from the Internet: ``` \[ andrew@pc01 ~ \]$ wget \\ > http://releases.ubuntu.com/18.10/ubuntu-18.10-desktop-amd64.iso ``` `curl` can be used just like `wget` (don’t forget the `--output` flag): ``` \[ andrew@pc01 ~ \]$ 捲曲 \\ > http://releases.ubuntu.com/18.10/ubuntu-18.10-desktop-amd64.iso \\ > \--輸出ubuntu.iso ``` `curl` and `wget` have their own strengths and weaknesses. `curl` supports many more protocols and is more widely available than `wget`; `curl` can also send data, while `wget` can only receive data. `wget` can download files recursively, while `curl` cannot. In general, I use `wget` when I need to download things from the Internet. I don’t often need to send data using `curl`, but it’s good to be aware of it for the rare occasion that you do. <a name="apt-gunzip-tar-gzip"></a> ### `apt / gunzip / tar / gzip` [[ Back to Table of Contents ]](#toc) Debian-descended Linux distributions have a fantastic package management tool called `apt`. It can be used to install, upgrade, or delete software on your machine. To search `apt` for a particular piece of software, use `apt search`, and install it with `apt install`: ``` \[ andrew@pc01 ~ \]$ apt 搜尋漂白位 ...bleachbit/bionic、bionic 2.0-2 全部 從系統中刪除不需要的文件 您需要“sudo”來安裝軟體 ============== \[ andrew@pc01 ~ \]$ sudo apt installbleachbit ``` Linux software often comes packaged in `.tar.gz` ("tarball") files: ``` \[ andrew@pc01 ~ \]$ wget \\ > https://github.com/atom/atom/releases/download/v1.35.0-beta0/atom-amd64.tar.gz ``` ...these types of files can be unzipped with `gunzip`: ``` \[ andrew@pc01 ~ \]$gunzipatom-amd64.tar.gz && ls 原子 amd64.tar ``` A `.tar.gz` file will be `gunzip`-ped to a `.tar` file, which can be extracted to a directory of files using `tar -xf` (`-x` for "extract", `-f` to specify the file to "untar"): ``` \[ andrew@pc01 ~ \]$ tar -xfatom-amd64.tar && mv \\ 原子-beta-1.35.0-beta0-amd64 原子 && ls 原子atom-amd64.tar ``` To go in the reverse direction, you can create (`-c`) a tar file from a directory and zip it (or unzip it, as appropriate) with `-z`: ``` \[ andrew@pc01 ~ \]$ tar -zcf 壓縮.tar.gz 原子 && ls 原子atom-amd64.tar壓縮.tar.gz ``` `.tar` files can also be zipped with `gzip`: ``` \[ andrew@pc01 ~ \]$ gzipatom-amd64.tar && ls 原子 原子-amd64.tar.gz 壓縮.tar.gz ``` <a name="redirecting-io"></a> ## Redirecting Input and Output <a name="pipe-gt-lt-echo-printf"></a> ### `| / > / < / echo / printf` [[ Back to Table of Contents ]](#toc) By default, shell commands read their input from the standard input stream (aka. stdin or 0) and write to the standard output stream (aka. stdout or 1), unless there’s an error, which is written to the standard error stream (aka. stderr or 2). `echo` writes text to stdout by default, which in most cases will simply print it to the terminal: ``` \[ andrew@pc01 ~ \]$ 回顯“你好” 你好 ``` The pipe operator, `|`, redirects the output of the first command to the input of the second command: ``` 'wc'(字數)傳回檔案中的行數、字數、位元組數 ======================== \[ andrew@pc01 ~ \]$ echo "範例文件" |廁所 ``` 1 2 17 ``` ``` `>` redirects output from stdout to a particular location ``` \[ andrew@pc01 ~ \]$ echo "test" > 文件 && 頭文件 測試 ``` `printf` is an improved `echo`, allowing formatting and escape sequences: ``` \[ andrew@pc01 ~ \]$ printf "1\\n3\\n2" 1 3 2 ``` `<` gets input from a particular location, rather than stdin: ``` 'sort' 依字母/數字順序對檔案的行進行排序 ======================== \[ andrew@pc01 ~ \]$ sort <(printf "1\\n3\\n2") 1 2 3 ``` Rather than a [UUOC](#viewing-and-editing-files), the recommended way to send the contents of a file to a command is to use `<`. Note that this causes data to "flow" right-to-left on the command line, rather than (the perhaps more natural, for English-speakers) left-to-right: ``` \[ andrew@pc01 ~ \]$ printf "1\\n3\\n2" > 文件 && 排序 < 文件 1 2 3 ``` <a name="std-tee"></a> ### `0 / 1 / 2 / tee` [[ Back to Table of Contents ]](#toc) 0, 1, and 2 are the standard input, output, and error streams, respectively. Input and output streams can be redirected with the `|`, `>`, and `<` operators mentioned previously, but stdin, stdout, and stderr can also be manipulated directly using their numeric identifiers: Write to stdout or stderr with `>&1` or `>&2`: ``` \[ andrew@pc01 ~ \]$ 貓測試 回顯“標準輸出”>&1 回顯“標準錯誤”>&2 ``` By default, stdout and stderr both print output to the terminal: ``` \[ andrew@pc01 ~ \]$ ./測試 標準錯誤 標準輸出 ``` Redirect stdout to `/dev/null` (only print output sent to stderr): ``` \[ andrew@pc01 ~ \]$ ./test 1>/dev/null 標準錯誤 ``` Redirect stderr to `/dev/null` (only print output sent to stdout): ``` \[ andrew@pc01 ~ \]$ ./test 2>/dev/null 標準輸出 ``` Redirect all output to `/dev/null` (print nothing): ``` \[ andrew@pc01 ~ \]$ ./test &>/dev/null ``` Send output to stdout and any number of additional locations with `tee`: ``` \[ andrew@pc01 ~ \]$ ls && echo "測試" | tee 文件1 文件2 文件3 && ls 文件0 測試 文件0 文件1 文件2 文件3 ``` <a name="advanced"></a> # Advanced <a name="superuser"></a> ## Superuser <a name="sudo-su"></a> ### `sudo / su` [[ Back to Table of Contents ]](#toc) You can check what your username is with `whoami`: ``` \[ andrew@pc01 abc \]$ whoami 安德魯 ``` ...and run a command as another user with `sudo -u username` (you will need that user's password): ``` \[ andrew@pc01 abc \]$ sudo -u 測試觸摸 def && ls -l 總計 0 -rw-r--r-- 1 次測試 0 Jan 11 20:05 def ``` If `–u` is not provided, the default user is the superuser (usually called "root"), with unlimited permissions: ``` \[ andrew@pc01 abc \]$ sudo touch ghi && ls -l 總計 0 -rw-r--r-- 1 次測試 0 Jan 11 20:05 def -rw-r--r-- 1 root root 0 Jan 11 20:14 ghi ``` Use `su` to become another user temporarily (and `exit` to switch back): ``` \[ andrew@pc01 abc \]$ su 測試 密碼: test@pc01:/home/andrew/abc$ whoami 測試 test@pc01:/home/andrew/abc$ 退出 出口 \[ andrew@pc01 abc \]$ whoami 安德魯 ``` [Learn more about the differences between `sudo` and `su` here.](http://bit.ly/2SKQH77) <a name="click-click"></a> ### `!!` [[ Back to Table of Contents ]](#toc) The superuser (usually "root") is the only person who can install software, create users, and so on. Sometimes it's easy to forget that, and you may get an error: ``` \[ andrew@pc01 ~ \]$ apt 安裝 ruby E:無法開啟鎖定檔案 /var/lib/dpkg/lock-frontend - 開啟(13:權限被拒絕) E: 無法取得 dpkg 前端鎖定 (/var/lib/dpkg/lock-frontend),您是 root 嗎? ``` You could retype the command and add `sudo` at the front of it (run it as the superuser): ``` \[ andrew@pc01 ~ \]$ sudo apt install ruby 正在閱讀包裝清單... ``` Or, you could use the `!!` shortcut, which retains the previous command: ``` \[ andrew@pc01 ~ \]$ apt 安裝 ruby E:無法開啟鎖定檔案 /var/lib/dpkg/lock-frontend - 開啟(13:權限被拒絕) E: 無法取得 dpkg 前端鎖定 (/var/lib/dpkg/lock-frontend),您是 root 嗎? \[ andrew@pc01 ~ \]$ sudo !! sudo apt 安裝 ruby 正在閱讀包裝清單... ``` By default, running a command with `sudo` (and correctly entering the password) allows the user to run superuser commands for the next 15 minutes. Once those 15 minutes are up, the user will again be prompted to enter the superuser password if they try to run a restricted command. <a name="file-permissions"></a> ## File Permissions <a name="file-permissions-sub"></a> ### File Permissions [[ Back to Table of Contents ]](#toc) Files may be able to be read (`r`), written to (`w`), and/or executed (`x`) by different users or groups of users, or not at all. File permissions can be seen with the `ls -l` command and are represented by 10 characters: ``` \[ andrew@pc01 ~ \]$ ls -lh 總計 8 drwxr-xr-x 4 安德魯 安德魯 4.0K 1 月 4 日 19:37 品嚐 -rwxr-xr-x 1 安德魯 安德魯 40 Jan 11 16:16 測試 -rw-r--r-- 1 安德魯 安德魯 0 一月 11 16:34 tist ``` The first character of each line represents the type of file, (`d` = directory, `l` = link, `-` = regular file, and so on); then there are three groups of three characters which represent the permissions held by the user (u) who owns the file, the permissions held by the group (g) which owns the file, and the permissions held any other (o) users. (The number which follows this string of characters is the number of links in the file system to that file (4 or 1 above).) `r` means that person / those people have read permission, `w` is write permission, `x` is execute permission. If a directory is “executable”, that means it can be opened and its contents can be listed. These three permissions are often represented with a single three-digit number, where, if `x` is enabled, the number is incremented by 1, if `w` is enabled, the number is incremented by 2, and if `r` is enabled, the number is incremented by 4. Note that these are equivalent to binary digits (`r-x` -> `101` -> `5`, for example). So the above three files have permissions of 755, 755, and 644, respectively. The next two strings in each list are the name of the owner (`andrew`, in this case) and the group of the owner (also `andrew`, in this case). Then comes the size of the file, its most recent modification time, and its name. The `–h` flag makes the output human readable (i.e. printing `4.0K` instead of `4096` bytes). <a name="chmod-chown"></a> ### `chmod / chown` [[ Back to Table of Contents ]](#toc) File permissions can be modified with `chmod` by setting the access bits: ``` \[ andrew@pc01 ~ \]$ chmod 777 測試 && chmod 000 tit && ls -lh 總計 8.0K drwxr-xr-x 4 安德魯 安德魯 4.0K 1 月 4 日 19:37 品嚐 -rwxrwxrwx 1 安德魯 安德魯 40 Jan 11 16:16 測試 \---------- 1 安德魯 安德魯 0 一月 11 16:34 tist ``` ...or by adding (`+`) or removing (`-`) `r`, `w`, and `x` permissions with flags: ``` \[ andrew@pc01 ~ \]$ chmod +rwx Tist && chmod -w 測試 && ls -lh chmod:測試:新權限是 r-xrwxrwx,而不是 r-xr-xr-x 總計 8.0K drwxr-xr-x 4 安德魯 安德魯 4.0K 1 月 4 日 19:37 品嚐 -r-xrwxrwx 1 安德魯 安德魯 40 Jan 11 16:16 測試 -rwxr-xr-x 1 安德魯 安德魯 0 一月 11 16:34 tist ``` The user who owns a file can be changed with `chown`: ``` \[ andrew@pc01 ~ \]$ sudo chown 碼頭測試 ``` The group which owns a file can be changed with `chgrp`: ``` \[ andrew@pc01 ~ \]$ sudo chgrp hadoop tit && ls -lh 總計 8.0K drwxr-xr-x 4 安德魯 安德魯 4.0K 1 月 4 日 19:37 品嚐 \-----w--w- 1 瑪麗娜·安德魯 2011 年 1 月 40 日 16:16 測試 -rwxr-xr-x 1 安德魯 hadoop 0 一月 11 16:34 tist ``` <a name="users-groups"></a> ## User and Group Management <a name="users"></a> ### Users [[ Back to Table of Contents ]](#toc) `users` shows all users currently logged in. Note that a user can be logged in multiple times if -- for instance -- they're connected via multiple `ssh` sessions. ``` \[ andrew@pc01 ~ \]$ 用戶 安德魯·科林·科林·科林·科林·科林·克里希納·克里希納 ``` To see all users (even those not logged in), check `/etc/passwd`. (**WARNING**: do not modify this file! You can corrupt your user accounts and make it impossible to log in to your system.) ``` \[ andrew@pc01 ~ \]$ alias au="cut -d: -f1 /etc/passwd \\ > |排序| uniq”&& au \_易於 一個廣告 安德魯... ``` Add a user with `useradd`: ``` \[ andrew@pc01 ~ \]$ sudo useradd aardvark && au \_易於 土豚 一個廣告... ``` Delete a user with `userdel`: ``` \[ andrew@pc01 ~ \]$ sudo userdel aardvark && au \_易於 一個廣告 安德魯... ``` [Change a user’s default shell, username, password, or group membership with `usermod`.](http://bit.ly/2D4upIg) <a name="groups"></a> ### Groups [[ Back to Table of Contents ]](#toc) `groups` shows all of the groups of which the current user is a member: ``` \[ andrew@pc01 ~ \]$ 組 andrew adm cdrom sudo dial plugdev lpadmin sambashare hadoop ``` To see all groups on the system, check `/etc/group`. (**DO NOT MODIFY** this file unless you know what you are doing.) ``` \[ andrew@pc01 ~ \]$ alias ag=“cut -d: -f1 /etc/group \\ > |排序”&& ag 管理員 一個廣告 安德魯... ``` Add a group with `groupadd`: ``` \[ andrew@pc01 ~ \]$ sudo groupadd aardvark && ag 土豚 管理員 一個廣告... ``` Delete a group with `groupdel`: ``` \[ andrew@pc01 ~ \]$ sudo groupdel aardvark && ag 管理員 一個廣告 安德魯... ``` [Change a group’s name, ID number, or password with `groupmod`.](https://linux.die.net/man/8/groupmod) <a name="text-processing"></a> ## Text Processing <a name="uniq-sort-diff-cmp"></a> ### `uniq / sort / diff / cmp` [[ Back to Table of Contents ]](#toc) `uniq` can print unique lines (default) or repeated lines: ``` \[ andrew@pc01 man \]$ printf "1\\n2\\n2" > a && \\ > printf "1\\n3\\n2" > b \[ andrew@pc01 人 \]$ uniq a 1 2 ``` `sort` will sort lines alphabetically / numerically: ``` \[ andrew@pc01 man \]$ 排序 b 1 2 3 ``` `diff` will report which lines differ between two files: ``` \[ andrew@pc01 人 \]$ diff ab 2c2 < 2 --- > 3 ``` `cmp` reports which bytes differ between two files: ``` \[andrew@pc01 人\]$ cmp ab ab 不同:字元 3,第 2 行 ``` <a name="cut-sed"></a> ### `cut / sed` [[ Back to Table of Contents ]](#toc) `cut` is usually used to cut a line into sections on some delimiter (good for CSV processing). `-d` specifies the delimiter and `-f` specifies the field index to print (starting with 1 for the first field): ``` \[ andrew@pc01 人 \]$ printf "137.99.234.23" > c \[ andrew@pc01 man \]$ cut -d'.' c-f1 137 ``` `sed` is commonly used to replace a string with another string in a file: ``` \[ andrew@pc01 man \]$ echo "舊" | sed s/舊/新/ 新的 ``` ...but `sed` is an extremely powerful utility, and cannot be properly summarised here. It’s actually Turing-complete, so it can do anything that any other programming language can do. `sed` can find and replace based on regular expressions, selectively print lines of a file which match or contain a certain pattern, edit text files in-place and non-interactively, and much more. A few good tutorials on `sed` include: - [https://www.tutorialspoint.com/sed/](https://www.tutorialspoint.com/sed/) - [http://www.grymoire.com/Unix/Sed.html](http://www.grymoire.com/Unix/Sed.html) - [https://www.computerhope.com/unix/used.htm](https://www.computerhope.com/unix/used.htm) <a name="pattern-matching"></a> ## Pattern Matching <a name="grep"></a> ### `grep` [[ Back to Table of Contents ]](#toc) The name `grep` comes from `g`/`re`/`p` (search `g`lobally for a `r`egular `e`xpression and `p`rint it); it’s used for finding text in files. `grep` is used to find lines of a file which match some pattern: ``` \[ andrew@pc01 ~ \]$ grep -e " *.fi.* " /etc/profile /etc/profile:Bourne shell 的系統範圍 .profile 檔案 (sh(1)) =================================================== ``` # The file bash.bashrc already sets the default PS1. ``` ``` fi ``` ``` fi ``` … ``` ...or contain some word: ``` \[ andrew@pc01 ~ \]$ grep "andrew" /etc/passwd 安德魯:x:1000:1000:安德魯,,,:/home/andrew:/bin/bash ``` `grep` is usually the go-to choice for simply finding matching lines in a file, if you’re planning on allowing some other program to handle those lines (or if you just want to view them). `grep` allows for (`-E`) use of extended regular expressions, (`-F`) matching any one of multiple strings at once, and (`-r`) recursively searching files within a directory. These flags used to be implemented as separate commands (`egrep`, `fgrep`, and `rgrep`, respectively), but those commands are now deprecated. > **Bonus**: [see the origins of the names of a few famous `bash` commands](https://kb.iu.edu/d/abnd) <a name="awk"></a> ### `awk` [[ Back to Table of Contents ]](#toc) `awk` is a pattern-matching language built around reading and manipulating delimited data files, like CSV files. As a rule of thumb, `grep` is good for finding strings and patterns in files, `sed` is good for one-to-one replacement of strings in files, and `awk` is good for extracting strings and patterns from files and analysing them. As an example of what `awk` can do, here’s a file containing two columns of data: ``` \[ andrew@pc01 ~ \]$ printf "A 10\\nB 20\\nC 60" > 文件 ``` Loop over the lines, add the number to sum, increment count, print the average: ``` \[ andrew@pc01 ~ \]$ awk 'BEGIN {sum=0;計數=0; OFS=“”} {sum+=$2; count++} END {print "平均值:", sum/count}' 文件 平均:30 ``` `sed` and `awk` are both Turing-complete languages. There have been multiple books written about each of them. They can be extremely useful with pattern matching and text processing. I really don’t have enough space here to do either of them justice. Go read more about them! > **Bonus**: [learn about some of the differences between `sed`, `grep`, and `awk`](http://bit.ly/2AI3IaN) <a name="ssh"></a> ## Copying Files Over `ssh` <a name="ssh-scp"></a> ### `ssh / scp` [[ Back to Table of Contents ]](#toc) `ssh` is how Unix-based machines connect to each other over a network: ``` \[ andrew@pc01 ~ \]$ ssh –p安德魯@137.xxx.xxx.89 上次登入:2019 年 1 月 11 日星期五 12:30:52,來自 137.xxx.xxx.199 ``` Notice that my prompt has changed as I’m now on a different machine: ``` \[ andrew@pc02 ~ \]$ 退出 登出 與 137.xxx.xxx.89 的連線已關閉。 ``` Create a file on machine 1: ``` \[ andrew@pc01 ~ \]$ echo "你好" > 你好 ``` Copy it to machine 2 using `scp` (secure copy; note that `scp` uses `–P` for a port #, `ssh` uses `–p`) ``` \[ andrew@pc01 ~ \]$ scp –P你好安德魯@137.xxx.xxx.89:~ 你好 100% 0 0.0KB/秒 00:00 ``` `ssh` into machine 2: ``` \[ andrew@pc02 ~ \]$ ssh –p安德魯@137.xxx.xxx.89 上次登入:2019 年 1 月 11 日星期五 22:47:37,來自 137.xxx.xxx.79 ``` The file’s there! ``` \[ andrew@pc02 ~ \]$ ls 你好多xargs \[ andrew@pc02 ~ \]$ 貓你好 你好 ``` <a name="rsync"></a> ### `rsync` [[ Back to Table of Contents ]](#toc) `rsync` is a file-copying tool which minimises the amount of data copied by looking for deltas (changes) between files. Suppose we have two directories: `d`, with one file, and `s`, with two files: ``` \[ andrew@pc01 d \]$ ls && ls ../s f0 f0 f1 ``` Sync the directories (copying only missing data) with `rsync`: ``` \[ andrew@pc01 d \]$ rsync -off ../s/\* . 正在發送增量文件列表... ``` `d` now contains all files that `s` contains: ``` \[ andrew@pc01 d \]$ ls f0 f1 ``` `rsync` can be performed over `ssh` as well: ``` \[ andrew@pc02 r \]$ ls \[ andrew@pc02 r \]$ rsync -avz -e "ssh -p “ [email protected]:~/s/\* 。 接收增量檔案列表 f0 f1 發送 62 位元組 接收 150 位元組 141.33 位元組/秒 總大小為 0 加速率為 0.00 \[ andrew@pc02 r \]$ ls f0 f1 ``` <a name="long-running-processes"></a> ## Long-Running Processes <a name="yes-nohup-ps-kill"></a> ### `yes / nohup / ps / kill` [[ Back to Table of Contents ]](#toc) Sometimes, `ssh` connections can disconnect due to network or hardware problems. Any processes initialized through that connection will be “hung up” and terminate. Running a command with `nohup` insures that the command will not be hung up if the shell is closed or if the network connection fails. Run `yes` (continually outputs "y" until it’s killed) with `nohup`: ``` \[ andrew@pc01 ~ \]$ nohup 是 & \[1\]13173 ``` `ps` shows a list of the current user’s processes (note PID number 13173): ``` \[ andrew@pc01 ~ \]$ ps | sed -n '/是/p' 13173 分/10 00:00:12 是 ``` _...log out and log back into this shell..._ The process has disappeared from `ps`! ``` \[ andrew@pc01 ~ \]$ ps | sed -n '/是/p' ``` But it still appears in `top` and `htop` output: ``` \[ andrew@pc01 ~ \]$ 頂部 -bn 1 | sed -n '/是/p' 13173 安德魯 20 0 4372 704 636 D 25.0 0.0 0:35.99 是 ``` Kill this process with `-9` followed by its process ID (PID) number: ``` \[ andrew@pc01 ~ \]$ 殺死 -9 13173 ``` It no longer appears in `top`, because it’s been killed: ``` \[ andrew@pc01 ~ \]$ 頂部 -bn 1 | sed -n '/是/p' ``` <a name="cron"></a> ### `cron / crontab / >>` [[ Back to Table of Contents ]](#toc) `cron` provides an easy way of automating regular, scheduled tasks. You can edit your `cron` jobs with `crontab –e` (opens a text editor). Append the line: ``` - - - - - 日期 >> ~/datefile.txt ``` This will run the `date` command every minute, appending (with the `>>` operator) the output to a file: ``` \[ andrew@pc02 ~ \]$ head ~/datefile.txt 2019 年 1 月 12 日星期六 14:37:01 GMT 2019 年 1 月 12 日星期六
可以說,對於任何開源專案來說,最重要的文件就是自述文件。一個好的自述文件不僅告訴人們該專案的用途和用途,還告訴人們他們如何使用該專案並為其做出貢獻。 如果您在撰寫自述文件時沒有充分解釋您的專案的用途或人們如何使用它,那麼它幾乎違背了開源的目的,因為其他開發人員不太可能參與或為其做出貢獻。 TL;DR - 太長?跳到最後並使用我的[模板](#template)。 什麼是自述文件? -------- 本質上,自述文件是一個單一的文字檔案( `.txt`或`.md` ),可作為專案或目錄的一站式文件。對於大多數開源專案來說,它通常是最明顯的文件和登陸頁面。即使自述文件的名稱全部大寫,也是為了吸引讀者的注意力並確保這是他們首先閱讀的內容。 有證據顯示自述文件的歷史可以追溯到 20 世紀 70 年代。我能找到的最古老的例子是 DEC PDP-10 計算機的[自述文件](http://pdp-10.trailing-edge.com/decus_20tap3_198111/01/decus/20-0079/readme.txt.html),日期為 1974 年 11 月 27 日。儘管自述文件名稱的起源存在爭議,但最流行的兩種理論是: 1. 帶有打孔卡的原始大型計算機的程式設計師會留下一疊帶有“READ ME!”的紙質指令。寫在前面。 2. 這個名字是向劉易斯·卡羅爾的《愛麗絲夢遊仙境》致敬,其中主角愛麗絲發現了一瓶標有“喝我”的藥水和標有“吃我”的蛋糕,這使她的體型發生了變化。 自述文件中應包含哪些內容? ------------- 好的,那麼一個很棒的自述文件該包含什麼內容呢?作為起點,我建議您包括以下關鍵內容: **1. 命名事物** 不要忘記為您的專案或功能命名。 GitHub 上有數量驚人的沒有名稱的專案。 **2. 介紹或總結** 寫一篇簡短的兩三行簡介,解釋你的專案的用途和用途。還要省略“簡介”、“摘要”或“概述”等標題 - 很明顯這是一個簡介。 **3. 先決條件** 在介紹之後立即加入一個部分,標題為列出任何想要使用該專案的人在開始之前可能需要的任何先決知識或工具。例如,如果它在最新版本的 Python 上執行,請告訴他們安裝 Python。這是一個例子: ``` Prerequisites Before you continue, ensure you have met the following requirements: * You have installed the latest version of Ruby. * You are using a Linux or Mac OS machine. Windows is not currently supported. * You have a basic understanding of graph theory. ``` **4. 如何安裝** 提供安裝步驟!令人驚訝的是,我遇到的許多專案只提供基本的使用說明,並期望您神奇地知道如何安裝它。如果需要多個步驟,請確保將安裝分解為編號的步驟。 **5. 如何使用該東西** 新增使用者安裝專案後如何使用該專案的步驟。如果您認為有用,請確保包含使用範例和解釋命令選項或標誌的參考。如果您在單獨的文件或網站中有更高級的文件,請從此處連結到該文件。 **6. 如何為事情做出貢獻** 提供為專案做出貢獻的步驟。或者,如果您希望人們在向您的專案貢獻拉取請求之前閱讀它,您可能希望在單獨的文件中建立貢獻者指南,並從自述文件連結到該指南。 **7. 加入貢獻者** 在作者部分感謝為該專案提供幫助的任何貢獻者。這是一種很好的方式,讓開源感覺像是團隊的努力,並感謝每個花時間做出貢獻的人。 **8. 加入致謝** 同樣,如果您使用了其他人的作品(程式碼、設計、圖像等),並且該作品具有需要確認的版權,您可能需要在此處加入。您還可以感謝為該專案提供幫助的任何其他開發商或機構。 **九、聯絡方式** 您可能不想這樣做,因為您是一個非常注重隱私的人,但如果有人有疑問、想要與您合作或對您的專案印象深刻並為您提供工作機會,那麼將您的聯絡方式放在前面是有意義的中心。 **10.新增許可證訊息** 如果適用,您肯定希望包含許可證資訊。除非您提供此軟體,否則依賴第三方軟體的新創公司和其他公司不太可能能夠使用您的專案。請造訪[Choosealicense.com](https://choosealicense.com/)或[opensource.org](https://opensource.org/licenses) ,以取得您可以使用的授權清單。 在您的自述文件中加入耀斑 🔥 -------------- 如果您確實想讓您的自述文件脫穎而出並且看起來具有視覺吸引力,您可以執行以下操作: - **新增徽標**:如果您的專案有徽標,請將其新增至自述文件的頂部。品牌使專案看起來更專業並幫助人們記住它。 - **新增徽章或盾牌**:您可以新增徽章和盾牌以反映專案的當前狀態、它使用的許可證以及它使用的任何依賴項是否是最新的。而且它們看起來很酷!您可以在[Shields.io](https://shields.io/)找到徽章清單或自行設計。 - **新增螢幕截圖**:有時一個簡單的螢幕截圖或一組螢幕截圖可以表達的內容遠遠超過一千個單字。但請注意,如果您確實使用螢幕截圖,則每次更新專案時都需要更新它們。 - **使用表情符號?** :現在很多專案似乎都使用表情符號,儘管是否使用它們取決於您。它們是為你的自述文件注入一點色彩和幽默的好方法,讓專案感覺更人性化。 如果您使用[所有貢獻者](https://allcontributors.org/)來感謝貢獻,您可以使用他們的[表情符號鍵](https://allcontributors.org/docs/en/emoji-key)來表示不同的貢獻類型: ``` * 🐛 for raising a bug ``` ``` * 💻 for submitting code ``` ``` * 📖 for docs contributions etc. ``` 這是 GitHub 徽章或盾牌的樣子,供參考(毫無疑問您以前見過它們!):  我的模板<a name="template"></a> --------------------------- 我建立了一個模板,涵蓋了本文中提出的大部分建議。請隨意分叉存儲庫,提出改進建議或根據自己的目的自訂它!您可以在 GitHub[上](https://github.com/scottydocs/README-template.md)找到我的模板。 資源 -- 如果您需要更多有關自述文件的建議,我還推薦以下資源: - Daniel Beck 在 2016 年 Write the Docs 的演講[「Write the Readable」](https://www.youtube.com/watch?v=2dAK42B7qtw) 。 - Lorna Jane Mitchell 在 API 文件 2019 中的演講[「Github 作為登陸頁面」](https://youtu.be/fXMN4X9B8Rg) 。 - 查看 Franck Abgrall 的[README 產生器](https://github.com/kefranabg/readme-md-generator)。 --- 原文出處:https://dev.to/scottydocs/how-to-write-a-kickass-readme-5af9
介紹 -- 過去一年,生成式人工智慧一直是科技領域的熱門話題。每個人都在使用它來建造很酷的專案。谷歌有自己的生成人工智慧,稱為 Gemini。 最近,Google 為 Gemini 開發者推出了 API。它附帶了幾個庫和框架,開發人員可以使用它們將其合併到他們的應用程式中。 在本文中,我們將建立一個簡單的 Node.js 應用程式並將 Google Gemini 整合到其中。我們將使用[**Google Gemini SDK**](https://www.npmjs.com/package/@google/generative-ai) 。 那麼,事不宜遲,讓我們開始吧! 什麼是雙子座? ------- 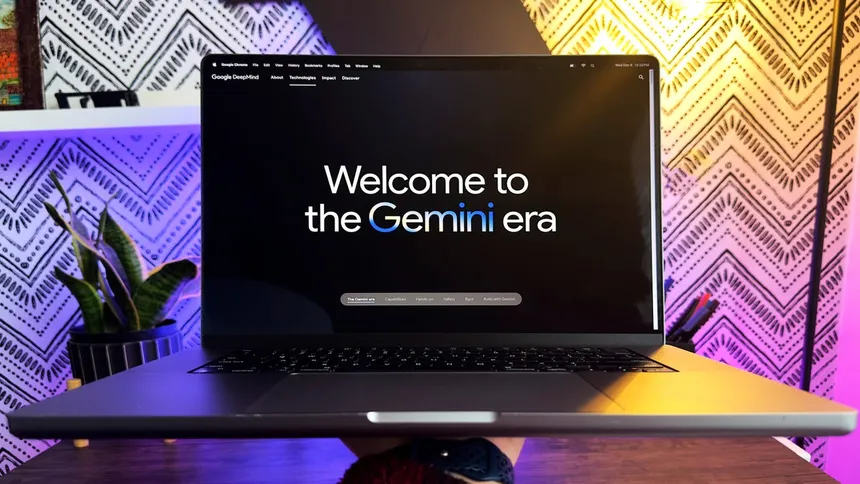 Google Gemini 是由 Google AI 開發的強大且多方面的 AI 模型。 Gemini 不僅處理文字;也處理文字。它可以理解和操作各種格式,如程式碼、音訊、圖像和視訊。這為您的 Node.js 專案帶來了令人興奮的可能性。 專案設定: ----- ### **1.建立Node.js專案:** 要啟動我們的專案,我們需要設定 Node.js 環境。那麼,讓我們建立一個節點專案。在終端機中執行以下命令。 ``` npm init ``` 這將初始化一個新的 Node.js 專案。 ### 2.安裝依賴項: 現在,我們將安裝專案所需的依賴項。 ``` npm install express body-parser @google/generative-ai dotenv ``` 這將安裝以下軟體包: - express:流行的 Node.js Web 框架 - body-parser:用來解析請求體的中介軟體 - @google/generative-ai:用於存取 Gemini 模型的套件 - dotenv:從 .env 檔案載入環境變數 ### 3.**設定環境變數:** 接下來,我們將建立一個`.env`資料夾來安全地儲存 API 憑證等敏感資訊。 ``` //.env API_KEY=YOUR_API_KEY PORT=3000 ``` ### 4.**取得API金鑰:** 在使用 Gemini 之前,我們需要從 Google Developers Console 設定 API 憑證。為此,我們需要註冊 Google 帳戶並建立 API 金鑰。 登入後,前往<https://makersuite.google.com/app/apikey> 。我們會得到這樣的結果: 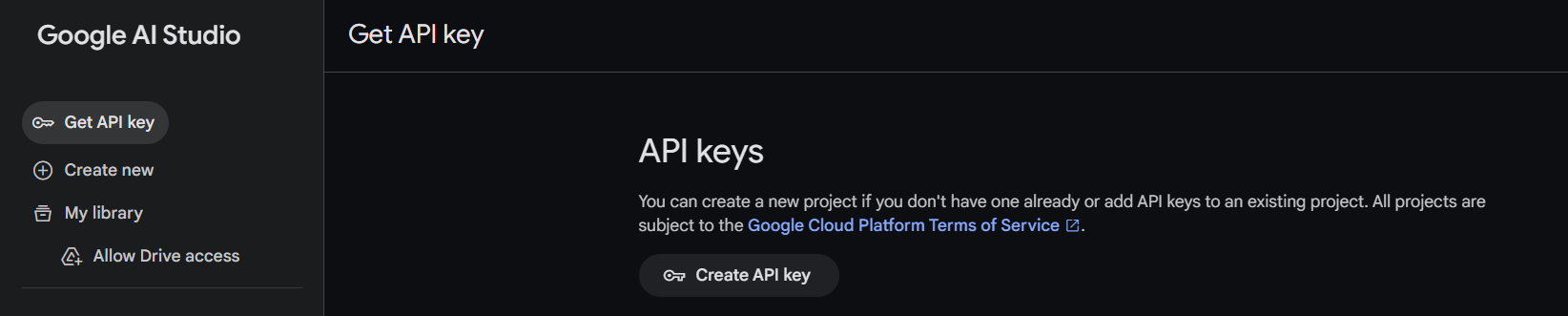 然後我們將點擊“建立 API 金鑰”按鈕。這將產生一個唯一的 API 金鑰,我們將使用它來驗證對 Google Generative AI API 的請求。 > 要測試您的 API,您可以執行以下 Curl 命令: > > ```javascript > 捲曲\\ > -H '內容類型:application/json' \\ > -d '{"contents":\[{"parts":\[{"text":"寫一個關於魔法背包的故事"}\]}\]}' \\ > -X POST https://generativelanguage.googleapis.com/v1beta/models/gemini-pro:generateContent?key=YOUR\_API\_KEY > ```` > > 將`YOUR_API_KEY`替換為我們先前獲得的實際 API 金鑰。 取得 API 金鑰後,我們將使用 API 金鑰更新`.env`檔。 ### 5. 建立 Express 伺服器: 現在,我們將在根目錄中建立一個`index.js`檔案並設定一個基本的express 伺服器。請看下面的程式碼: ``` const express = require("express"); const dotenv = require("dotenv"); dotenv.config(); const app = express(); const port = process.env.PORT; app.get("/", (req, res) => { res.send("Hello World"); }); app.listen(port, () => { console.log(`Server running on port ${port}`); }); ``` 在這裡,我們使用“dotenv”套件從`.env`檔案存取連接埠號碼。 在專案的頂部,我們使用`dotenv.config()`載入環境變數,使其可以在整個檔案中存取。 ### 6. 執行專案: 在此步驟中,我們將向`package.json`檔案新增一個啟動腳本,以輕鬆執行我們的專案。 因此,將以下腳本新增至 package.json 檔案中。 ``` "scripts": { "start": "node index.js" } ``` package.json 檔案應如下所示: 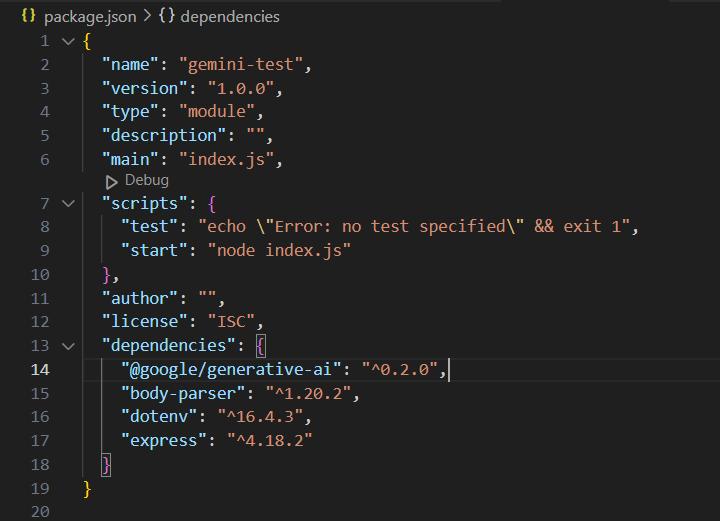 要檢查一切是否正常,讓我們使用以下命令執行該專案: ``` npm run start ``` 這將啟動 Express 伺服器。現在如果我們造訪這個 URL <http://localhost:3000/>我們會得到: 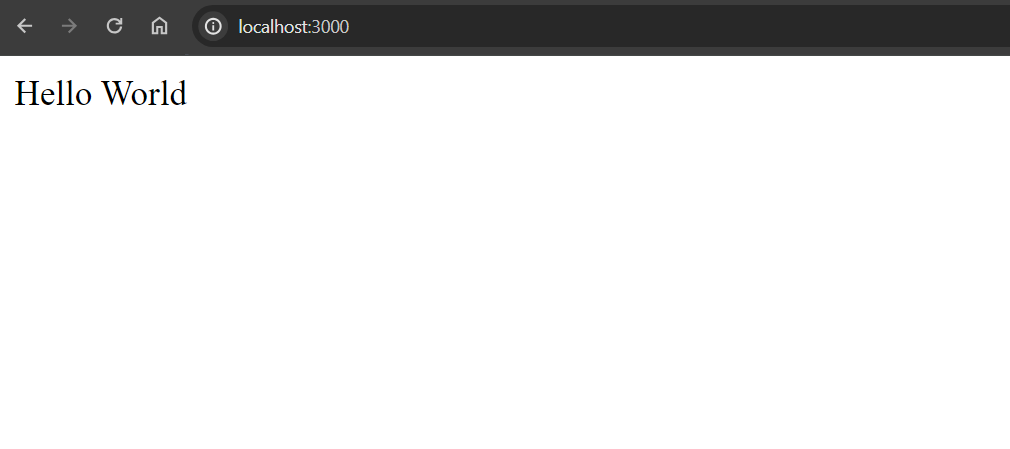 驚人的!專案設定已完成並且執行完美。接下來,我們將在下一節中將 Gemini 加入我們的專案中 新增Google雙子座: ------------ ### 1. 設定路由和中介軟體: 要將 Gemini 新增至我們的專案中,我們將建立一個`/generate`路由,以便與 Gemini AI 進行通訊。 為此,將以下程式碼新增至`index.js`檔案。 ``` const bodyParser = require("body-parser"); const { generateResponse } = require("./controllers/index.js"); //middleware to parse the body content to JSON app.use(bodyParser.json()); app.post("/generate", generateResponse); ``` 在這裡,我們使用`body-parser`中間件將內容解析為 JSON 格式。 ### 2.設定Google Generative AI: 現在,我們將建立一個控制器資料夾,並在其中建立一個`index.js`檔案。在這裡,我們將建立一個新的控制器函數來處理上面程式碼中聲明的生成路由。 ``` const { GoogleGenerativeAI } = require("@google/generative-ai"); const dotenv = require("dotenv"); dotenv.config(); // GoogleGenerativeAI required config const configuration = new GoogleGenerativeAI(process.env.API_KEY); // Model initialization const modelId = "gemini-pro"; const model = configuration.getGenerativeModel({ model: modelId }); ``` 在這裡,我們透過傳遞環境變數中的 API 金鑰來為 Google Generative AI API 建立一個配置物件。 然後,我們透過向配置物件的`getGenerativeModel`方法提供模型 ID(“gemini-pro”)來初始化模型。 > #### **型號配置:** > > 我們也可以依照自己的方便配置模型參數 > > 這些參數值控制模型如何產生回應。 > > 例子: > > ```javascript > 常量產生配置 = { > 停止序列:\[“紅色”\], > 最大輸出令牌:200, > 溫度:0.9, > 頂部P:0.1, > 頂級K:16, > }; > > const model = configuration.getGenerativeModel({ model: modelId, GenerationConfig }); > ```` > #### **安全設定:** > > 我們可以使用安全設定來防止有害的反應。預設情況下,安全性設定配置為阻止在各個維度上具有中等到高可能性不安全的內容。 > > 這是一個例子: > > ```javascript > const { HarmBlockThreshold, HarmCategory } = require("@google/generative-ai"); > > 常量安全設定 = \[ > { > ``` > category: HarmCategory.HARM_CATEGORY_HARASSMENT, > > ``` > ``` > threshold: HarmBlockThreshold.BLOCK_ONLY_HIGH, > > ``` > }, > { > ``` > category: HarmCategory.HARM_CATEGORY_HATE_SPEECH, > > ``` > ``` > threshold: HarmBlockThreshold.BLOCK_MEDIUM_AND_ABOVE, > > ``` > }, > \]; > > const model = genAI.getGenerativeModel({ model: "MODEL\_NAME", safetySettings }); > ```` > > 透過這些安全設置,我們可以透過最大限度地減少有害內容生成的可能性來增強安全性。 ### 3. 管理對話歷史記錄: 為了追蹤對話歷史記錄,我們建立了一個陣列`history`並將其從控制器檔案中匯出: ``` export const history = []; ``` ### 4.**實現控制器功能:** 現在,我們將編寫一個控制器函數`generateResponse`來處理產生路由(/generate)並產生對使用者請求的回應。 ``` /** * Generates a response based on the given prompt. * @param {Object} req - The request object. * @param {Object} res - The response object. * @returns {Promise} - A promise that resolves when the response is sent. */ export const generateResponse = async (req, res) => { try { const { prompt } = req.body; const result = await model.generateContent(prompt); const response = await result.response; const text = response.text(); console.log(text); history.push(text); console.log(history); res.send({ response: text }); } catch (err) { console.error(err); res.status(500).json({ message: "Internal server error" }); } }; ``` 在這裡,我們從請求正文中獲取提示,並使用`model.generateContent`方法根據提示產生回應。 為了追蹤響應,我們將響應推送到歷史陣列。 ### 5. 查看回覆紀錄: 現在,我們將建立一條路線來檢查我們的回應歷史記錄。該端點傳回`history`陣列。 將簡單程式碼加入`./index.js`資料夾中。 ``` app.get("/generate", (req, res) => { res.send(history); }); ``` 我們就完成了! ### 6.執行專案: 現在,我們必須檢查我們的應用程式是否正常運作! 讓我們使用以下命令來執行我們的專案: ``` npm run start ``` 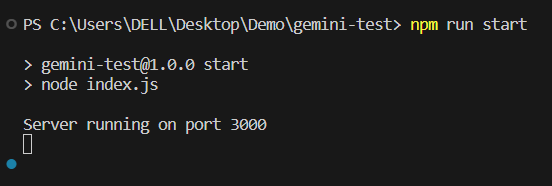 沒有錯誤!感謝上帝! :) 它運作正常。 ### 7. 檢查功能 接下來,我們將使用 Postman 發出 Post 請求來驗證我們的控制器功能。 我們將使用以下 JSON 負載向<http://localhost:3000/generate>發送 POST 請求: ``` { "prompt": "Write 3 Javascript Tips for Beginners" } ``` 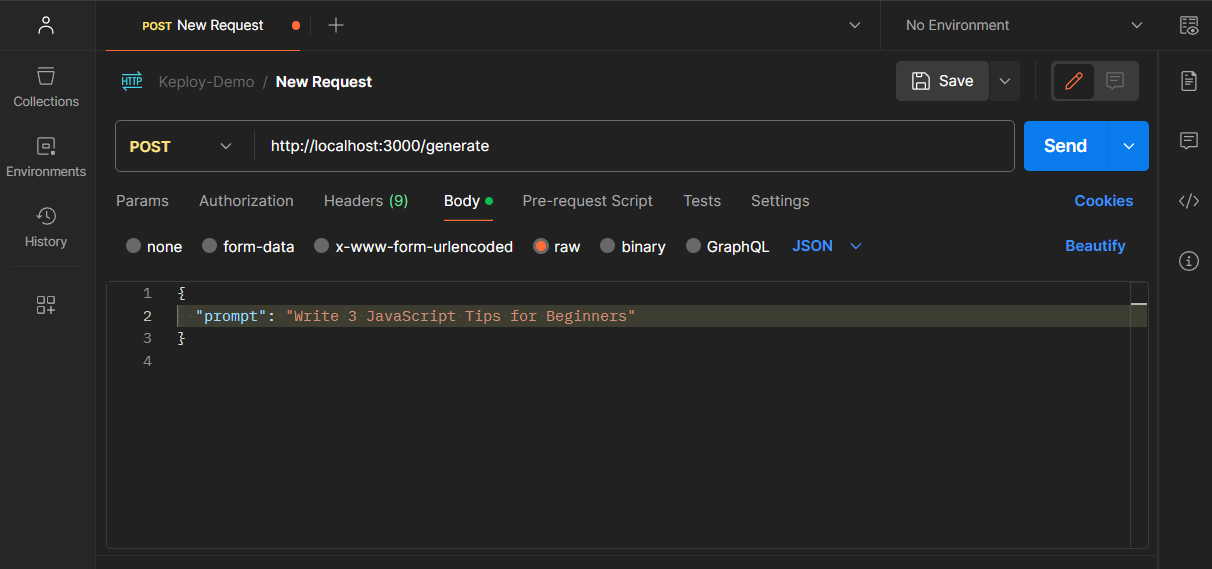 我們得到了回應: ``` { "response": "1. **Use console.log() for Debugging:**\n - console.log() is a useful tool for debugging your JavaScript code. It allows you to inspect the values of variables and expressions, and to see how your code is executing. This can be especially helpful when you encounter errors or unexpected behavior in your program.\n\n2. **Learn the Basics of Data Types:**\n - JavaScript has several built-in data types, including strings, numbers, booleans, and objects. Understanding the properties and behaviors of each data type is crucial for writing effective code. For instance, strings can be manipulated using string methods, while numbers can be used in mathematical operations.\n\n3. **Use Strict Mode:**\n - Strict mode is a way to opt-in to a restricted and secure subset of JavaScript. It helps you to write more secure and reliable code, as it throws errors for common mistakes that would otherwise go unnoticed in regular JavaScript mode. To enable strict mode, simply add \"use strict;\" at the beginning of your JavaScript file or module." } ``` 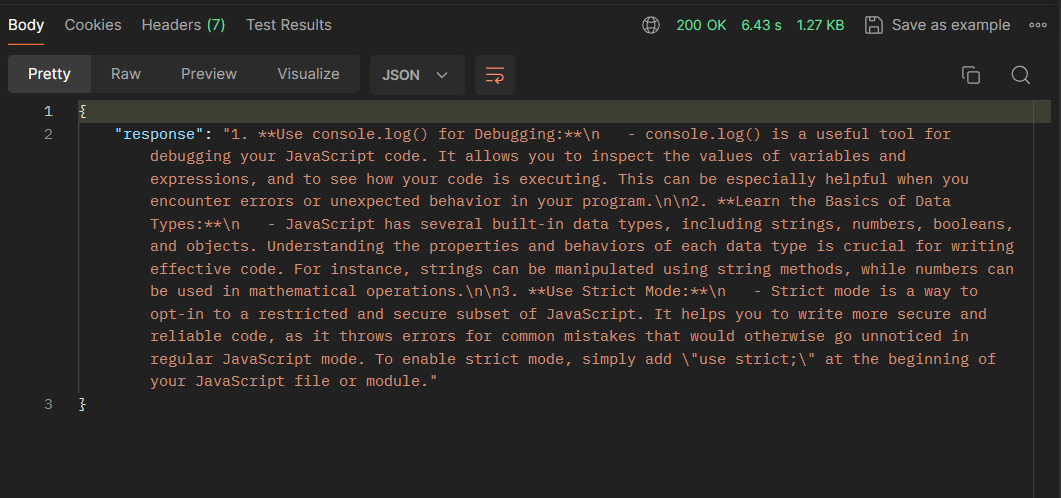 偉大的!我們的 Gemini AI 整合正在按預期工作! 此外,我們可以造訪[http://localhost:3000/generate 的](http://localhost:3000/generate)歷史記錄端點來查看對話歷史記錄。 這樣,我們就將 Gemini AI 整合到了 Node.js 應用程式中。在接下來的文章中,我們將探索 Gemini AI 的更多用例。 到那時,請繼續關注! 結論 -- 如果您發現這篇部落格文章有幫助,請考慮與可能受益的其他人分享。您也可以關注我,以了解更多有關 Javascript、React 和其他 Web 開發主題的內容。 要贊助我的工作,請存取: [Arindam 的贊助頁面](https://arindam1729.hashnode.dev/sponsor)並探索各種贊助選項。 在[Twitter](https://twitter.com/intent/follow?screen_name=Arindam_1729) 、 [LinkedIn](https://www.linkedin.com/in/arindam2004/) 、 [Youtube](https://www.youtube.com/channel/@Arindam_1729)和[GitHub](https://github.com/Arindam200)上與我聯絡。 感謝您的閱讀:) 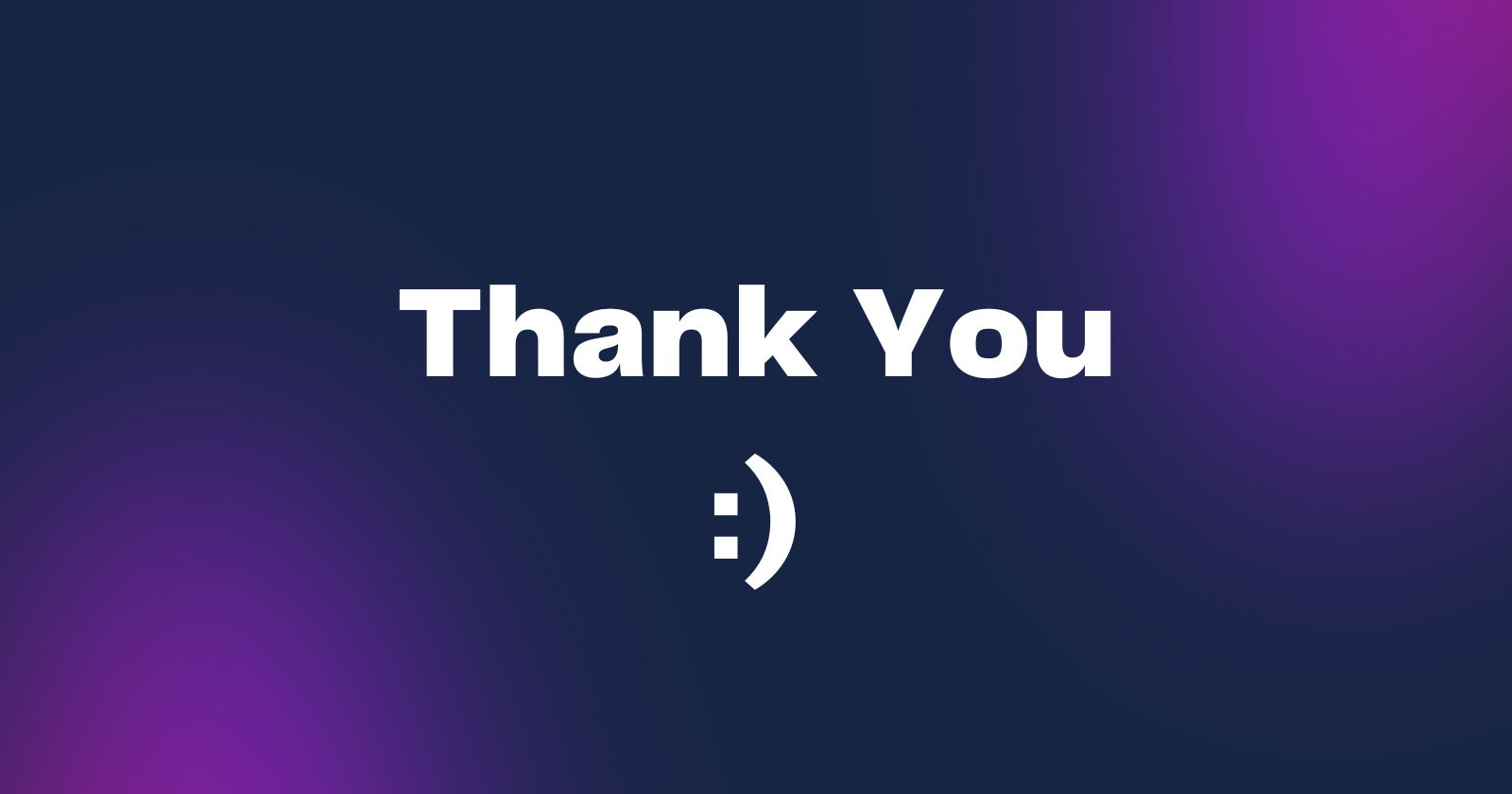 --- 原文出處:https://dev.to/arindam_1729/how-to-use-google-gemini-with-nodejs-2d39
長話短說 ---- 俗話說,給貓剝皮有多種方法…在科技界,給 Lambda 函數剝皮有 5 種方法 🤩 我們將比較 5 個開發工具 - ✅[翼](#1-wing) - ✅[刷子](#2-pulumi) - ✅ [AWS-CDK](#3-awscdk) - ✅ [Terraform 的 CDK](#4-cdk-for-terraform) - ✅[地形](#5-terraform) 介紹 -- 當開發人員試圖彌合開發和 DevOps 之間的差距時,我認為比較程式語言和 DevTools 會很有幫助。 讓我們從一個簡單函數的想法開始,該函數將文字檔案上傳到我們的雲端應用程式中的儲存桶。 下一步是展示實現這一目標的幾種方法。 **注意:**在雲端開發中,管理權限和儲存桶身分、打包執行時程式碼以及處理基礎架構和執行時的多個檔案會增加開發過程的複雜性。 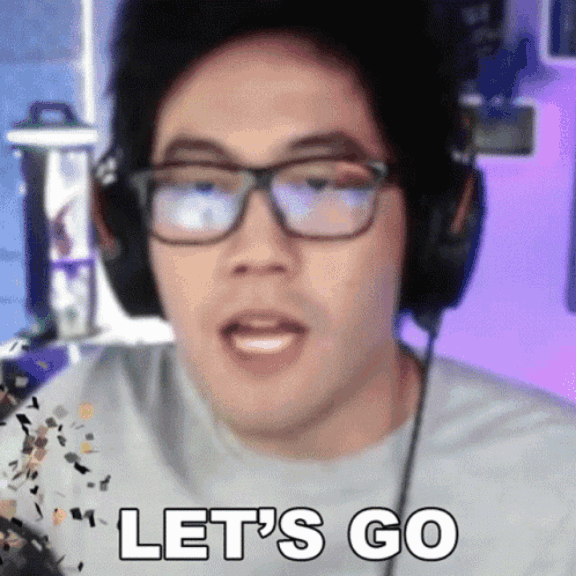 讓我們深入研究一些程式碼! --- 1.[翼](https://github.com/winglang/wing) --------------------------------------- > [安裝 Wing](https://www.winglang.io/docs)後,讓我們建立一個檔案: `main.w` > 如果您不熟悉 Wing 程式語言,請查看[此處的](https://github.com/winglang/wing)**開源儲存庫** ``` bring cloud; let bucket = new cloud.Bucket(); new cloud.Function(inflight () => { bucket.put("hello.txt", "world!"); }); ``` **讓我們詳細分析一下上面程式碼中發生的情況。** > `bring cloud`是 Wing 的導入語法 > **建立一個雲端儲存桶:** `let bucket = new cloud.Bucket();`初始化一個新的雲端儲存桶實例。 > 在後端,Wing 平台在您的雲端供應商環境中配置一個新儲存桶。此桶用於儲存和檢索資料。 > **建立雲端函數:** `new cloud.Function(inflight () => { ... });`語句定義了一個新的雲函數。 > 該函數被觸發後,將執行其主體內定義的操作。 > `bucket.put("hello.txt", "world!");`上傳一個名為 hello.txt 的文件,其中包含內容世界!到之前建立的雲端儲存桶。 編譯並部署到 AWS ---------- - `wing compile --platform tf-aws main.w` - `terraform apply` 就是這樣,Wing 負責處理複雜性(權限、在執行時程式碼中獲取存儲桶身份、將執行時程式碼打包到存儲桶中、必須編寫多個文件 - 用於基礎設施和執行時)等。 更不用說它會產生 IAC(TF 或 CF),以及可以使用現有工具部署的 Javascript。 但在開發時,您可以使用本機模擬器獲得即時回饋並縮短迭代周期 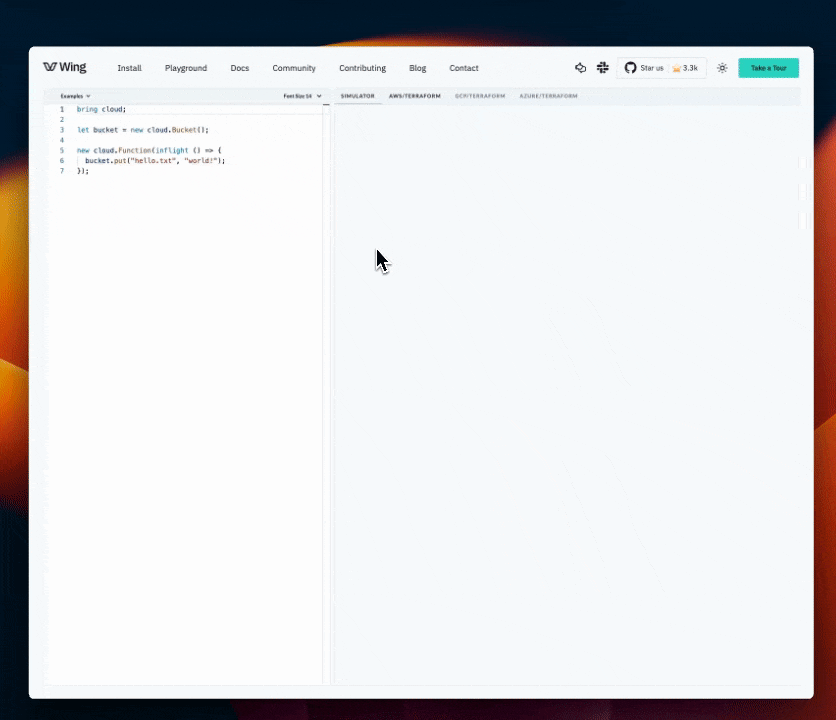 Wing 甚至還有一個[遊樂場](https://www.winglang.io/play/?code=YgByAGkAbgBnACAAYwBsAG8AdQBkADsACgAKAGwAZQB0ACAAYgB1AGMAawBlAHQAIAA9ACAAbgBlAHcAIABjAGwAbwB1AGQALgBCAHUAYwBrAGUAdAAoACkAOwAKAAoAbgBlAHcAIABjAGwAbwB1AGQALgBGAHUAbgBjAHQAaQBvAG4AKABpAG4AZgBsAGkAZwBoAHQAIAAoACkAIAA9AD4AIAB7AAoAIAAgAGIAdQBjAGsAZQB0AC4AcAB1AHQAKAAiAGgAZQBsAGwAbwAuAHQAeAB0ACIALAAgACIAdwBvAHIAbABkACEAIgApADsACgB9ACkAOwA%3D),您可以在瀏覽器中試用! 2.[刷子](https://www.pulumi.com) ------------------------------ > 步驟1:初始化一個新的Pulumi專案 ``` mkdir pulumi-s3-lambda-ts cd pulumi-s3-lambda-ts pulumi new aws-typescript ``` > 步驟 2. 編寫程式碼以將文字檔案上傳到 S3。 這將是您的專案結構。 ``` pulumi-s3-lambda-ts/ ├─ src/ │ ├─ index.ts # Pulumi infrastructure code │ └─ lambda/ │ └─ index.ts # Lambda function code to upload a file to S3 ├─ tsconfig.json # TypeScript configuration └─ package.json # Node.js project file with dependencies ``` 讓我們將此程式碼加入**index.ts** ``` import * as pulumi from "@pulumi/pulumi"; import * as aws from "@pulumi/aws"; // Create an AWS S3 bucket const bucket = new aws.s3.Bucket("myBucket", { acl: "private", }); // IAM role for the Lambda function const lambdaRole = new aws.iam.Role("lambdaRole", { assumeRolePolicy: JSON.stringify({ Version: "2023-10-17", Statement: [{ Action: "sts:AssumeRole", Principal: { Service: "lambda.amazonaws.com", }, Effect: "Allow", Sid: "", }], }), }); // Attach the AWSLambdaBasicExecutionRole policy new aws.iam.RolePolicyAttachment("lambdaExecutionRole", { role: lambdaRole, policyArn: aws.iam.ManagedPolicy.AWSLambdaBasicExecutionRole, }); // Policy to allow Lambda function to access the S3 bucket const lambdaS3Policy = new aws.iam.Policy("lambdaS3Policy", { policy: bucket.arn.apply(arn => JSON.stringify({ Version: "2023-10-17", Statement: [{ Action: ["s3:PutObject", "s3:GetObject"], Resource: `${arn}/*`, Effect: "Allow", }], })), }); // Attach policy to Lambda role new aws.iam.RolePolicyAttachment("lambdaS3PolicyAttachment", { role: lambdaRole, policyArn: lambdaS3Policy.arn, }); // Lambda function const lambda = new aws.lambda.Function("myLambda", { code: new pulumi.asset.AssetArchive({ ".": new pulumi.asset.FileArchive("./src/lambda"), }), runtime: aws.lambda.Runtime.NodeJS12dX, role: lambdaRole.arn, handler: "index.handler", environment: { variables: { BUCKET_NAME: bucket.bucket, }, }, }); export const bucketName = bucket.id; export const lambdaArn = lambda.arn; ``` 接下來,為 Lambda 函數程式碼建立**lambda/index.ts**目錄: ``` import { S3 } from "aws-sdk"; const s3 = new S3(); export const handler = async (): Promise<void> => { const bucketName = process.env.BUCKET_NAME || ""; const fileName = "example.txt"; const content = "Hello, Pulumi!"; const params = { Bucket: bucketName, Key: fileName, Body: content, }; try { await s3.putObject(params).promise(); console.log(`File uploaded successfully at https://${bucketName}.s3.amazonaws.com/${fileName}`); } catch (err) { console.log(err); } }; ``` > 步驟 3:TypeScript 設定 (tsconfig.json) ``` { "compilerOptions": { "target": "ES2018", "module": "CommonJS", "strict": true, "esModuleInterop": true, "skipLibCheck": true, "forceConsistentCasingInFileNames": true }, "include": ["src/**/*.ts"], "exclude": ["node_modules", "**/*.spec.ts"] } ``` **建立Pulumi專案後,會自動產生yaml檔案。** **pulumi.yaml** ``` name: s3-lambda-pulumi runtime: nodejs description: A simple example that uploads a file to an S3 bucket using a Lambda function template: config: aws:region: description: The AWS region to deploy into default: us-west-2 ``` 與 Pulumi 一起部署 ------------- 確保正確設定包含`index.js`檔案的`lambda`目錄。然後,執行以下命令來部署您的基礎架構: `pulumi up` --- 3. [AWS-CDK](https://aws.amazon.com/cdk) ---------------------------------------- > 步驟1:初始化一個新的CDK專案 ``` mkdir cdk-s3-lambda cd cdk-s3-lambda cdk init app --language=typescript ``` > 第 2 步:新增依賴項 ``` npm install @aws-cdk/aws-lambda @aws-cdk/aws-s3 ``` > 步驟 3:在 CDK 中定義 AWS 資源 文件: **index.js** ``` import * as cdk from '@aws-cdk/core'; import * as lambda from '@aws-cdk/aws-lambda'; import * as s3 from '@aws-cdk/aws-s3'; export class CdkS3LambdaStack extends cdk.Stack { constructor(scope: cdk.Construct, id: string, props?: cdk.StackProps) { super(scope, id, props); // Create the S3 bucket const bucket = new s3.Bucket(this, 'MyBucket', { removalPolicy: cdk.RemovalPolicy.DESTROY, // NOT recommended for production code }); // Define the Lambda function const lambdaFunction = new lambda.Function(this, 'MyLambda', { runtime: lambda.Runtime.NODEJS_14_X, // Define the runtime handler: 'index.handler', // Specifies the entry point code: lambda.Code.fromAsset('lambda'), // Directory containing your Lambda code environment: { BUCKET_NAME: bucket.bucketName, }, }); // Grant the Lambda function permissions to write to the S3 bucket bucket.grantWrite(lambdaFunction); } } ``` > 步驟 4:Lambda 函數程式碼 在 pulumi 目錄中建立與上面相同的檔案結構: **index.ts** ``` import { S3 } from 'aws-sdk'; const s3 = new S3(); exports.handler = async (event: any) => { const bucketName = process.env.BUCKET_NAME; const fileName = 'uploaded_file.txt'; const content = 'Hello, CDK! This file was uploaded by a Lambda function!'; try { const result = await s3.putObject({ Bucket: bucketName!, Key: fileName, Body: content, }).promise(); console.log(`File uploaded successfully: ${result}`); return { statusCode: 200, body: `File uploaded successfully: ${fileName}`, }; } catch (error) { console.log(error); return { statusCode: 500, body: `Failed to upload file: ${error}`, }; } }; ``` 部署 CDK 堆疊 --------- 首先,編譯 TypeScript 程式碼: `npm run build` ,然後 將您的 CDK 部署到 AWS: `cdk deploy` --- [4.Terraform 的 CDK](https://developer.hashicorp.com/terraform/cdktf) -------------------------------------------------------------------- > 步驟1:初始化一個新的CDKTF專案 ``` mkdir cdktf-s3-lambda-ts cd cdktf-s3-lambda-ts ``` 然後,使用 TypeScript 初始化一個新的 CDKTF 專案: ``` cdktf init --template="typescript" --local ``` > 步驟 2:安裝 AWS Provider 並新增相依性 ``` npm install @cdktf/provider-aws ``` > 第 3 步:定義基礎設施 編輯 main.ts 以定義 S3 儲存桶和 Lambda 函數: ``` import { Construct } from 'constructs'; import { App, TerraformStack } from 'cdktf'; import { AwsProvider, s3, lambdafunction, iam } from '@cdktf/provider-aws'; class MyStack extends TerraformStack { constructor(scope: Construct, id: string) { super(scope, id); new AwsProvider(this, 'aws', { region: 'us-west-2' }); // S3 bucket const bucket = new s3.S3Bucket(this, 'lambdaBucket', { bucketPrefix: 'cdktf-lambda-' }); // IAM role for Lambda const role = new iam.IamRole(this, 'lambdaRole', { name: 'lambda_execution_role', assumeRolePolicy: JSON.stringify({ Version: '2023-10-17', Statement: [{ Action: 'sts:AssumeRole', Principal: { Service: 'lambda.amazonaws.com' }, Effect: 'Allow', }], }), }); new iam.IamRolePolicyAttachment(this, 'lambdaPolicy', { role: role.name, policyArn: 'arn:aws:iam::aws:policy/service-role/AWSLambdaBasicExecutionRole', }); const lambdaFunction = new lambdafunction.LambdaFunction(this, 'MyLambda', { functionName: 'myLambdaFunction', handler: 'index.handler', role: role.arn, runtime: 'nodejs14.x', s3Bucket: bucket.bucket, // Assuming the Lambda code is uploaded to this bucket s3Key: 'lambda.zip', // Assuming the Lambda code zip file is named lambda.zip environment: { variables: { BUCKET_NAME: bucket.bucket, }, }, }); // Grant the Lambda function permissions to write to the S3 bucket new s3.S3BucketPolicy(this, 'BucketPolicy', { bucket: bucket.bucket, policy: bucket.bucket.apply(name => JSON.stringify({ Version: '2023-10-17', Statement: [{ Action: 's3:*', Resource: `arn:aws:s3:::${name}/*`, Effect: 'Allow', Principal: { AWS: role.arn, }, }], })), }); } } const app = new App(); new MyStack(app, 'cdktf-s3-lambda-ts'); app.synth(); ``` > 步驟 4:Lambda 函數程式碼 Lambda 函數程式碼應使用 TypeScript 編寫並編譯為 JavaScript,因為 AWS Lambda 本機執行 JavaScript。以下是您需要編譯和壓縮的 Lambda 函數的範例**index.ts** : ``` import { S3 } from 'aws-sdk'; const s3 = new S3(); exports.handler = async () => { const bucketName = process.env.BUCKET_NAME || ''; const content = 'Hello, CDKTF!'; const params = { Bucket: bucketName, Key: `upload-${Date.now()}.txt`, Body: content, }; try { await s3.putObject(params).promise(); return { statusCode: 200, body: 'File uploaded successfully' }; } catch (err) { console.error(err); return { statusCode: 500, body: 'Failed to upload file' }; } }; ``` 您需要將此 TypeScript 程式碼編譯為 JavaScript,對其進行壓縮,然後手動或使用腳本將其上傳到 S3 儲存桶。 確保 LambdaFunction 資源中的 s3Key 指向儲存桶中正確的 zip 檔案。 編譯和部署您的 CDKTF 專案 ---------------- 使用`npm run build`編譯專案 **生成 Terraform 配置文件** 執行`cdktf synth`指令。此命令執行您的 CDKTF 應用程式,該應用程式在`cdktf.out`目錄中產生 Terraform 設定檔( `*.tf.json`檔案): **部署您的基礎設施** `cdktf deploy` 5.[地形](https://developer.hashicorp.com/terraform) ------------------------------------------------- > 第 1 步:Terraform 設定 定義您的 AWS 供應商和 S3 儲存桶 使用以下內容建立名為**main.tf**的檔案: ``` provider "aws" { region = "us-west-2" # Choose your AWS region } resource "aws_s3_bucket" "lambda_bucket" { bucket_prefix = "lambda-upload-bucket-" acl = "private" } resource "aws_iam_role" "lambda_execution_role" { name = "lambda_execution_role" assume_role_policy = jsonencode({ Version = "2023-10-17" Statement = [ { Action = "sts:AssumeRole" Effect = "Allow" Principal = { Service = "lambda.amazonaws.com" } }, ] }) } resource "aws_iam_policy" "lambda_s3_policy" { name = "lambda_s3_policy" description = "IAM policy for Lambda to access S3" policy = jsonencode({ Version = "2023-10-17" Statement = [ { Action = ["s3:PutObject", "s3:GetObject"], Effect = "Allow", Resource = "${aws_s3_bucket.lambda_bucket.arn}/*" }, ] }) } resource "aws_iam_role_policy_attachment" "lambda_s3_access" { role = aws_iam_role.lambda_execution_role.name policy_arn = aws_iam_policy.lambda_s3_policy.arn } resource "aws_lambda_function" "uploader_lambda" { function_name = "S3Uploader" s3_bucket = "YOUR_DEPLOYMENT_BUCKET_NAME" # Set your deployment bucket name here s3_key = "lambda.zip" # Upload your ZIP file to S3 and set its key here handler = "index.handler" role = aws_iam_role.lambda_execution_role.arn runtime = "nodejs14.x" environment { variables = { BUCKET_NAME = aws_s3_bucket.lambda_bucket.bucket } } } ``` > 步驟 2:Lambda 函數程式碼 (TypeScript) 為 Lambda 函數建立 TypeScript 檔案**index.ts** : ``` import { S3 } from 'aws-sdk'; const s3 = new S3(); exports.handler = async (event: any) => { const bucketName = process.env.BUCKET_NAME; const fileName = `uploaded-${Date.now()}.txt`; const content = 'Hello, Terraform and AWS Lambda!'; try { await s3.putObject({ Bucket: bucketName!, Key: fileName, Body: content, }).promise(); console.log('Upload successful'); return { statusCode: 200, body: JSON.stringify({ message: 'Upload successful' }), }; } catch (error) { console.error('Upload failed:', error); return { statusCode: 500, body: JSON.stringify({ message: 'Upload failed' }), }; } }; ``` 最後,將 Lambda 函數程式碼上傳到指定的 S3 儲存桶後,執行`terraform apply` 。 --- 我希望您喜歡在我們的雲端應用程式中編寫將文字檔案上傳到儲存桶的函數的五種簡單方法的比較。 正如您所看到的,除了一段程式碼之外,大多數程式碼都變得非常複雜。 結論! --- [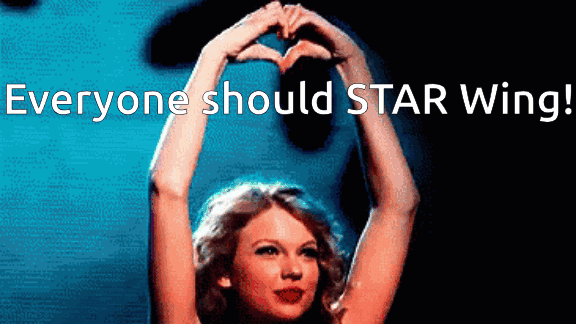](https://github.com/winglang/wing) 點擊圖片⬆️ 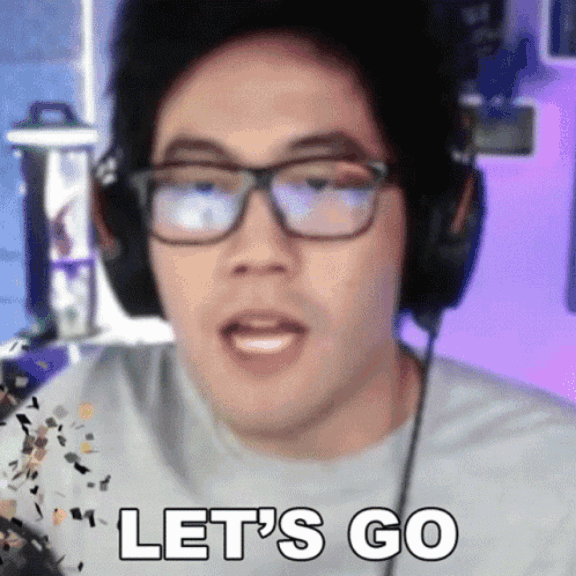 > 如果您對 Wing 感興趣並且喜歡我們如何簡化雲端開發流程,請給我們一顆 ⭐ 顆星。 {% cta https://github.com/winglang/wing %} 請star ⭐ Wing {% endcta %} --- 原文出處:https://dev.to/winglang/5-ways-to-write-a-simple-function-in-your-cloud-app-1jgl
在我最新的文章中,我談到了 Vagrant 以及它如何幫助我們在幾分鐘內建立虛擬機,但如果可以做得更快、更好、更可自訂呢?讓我們學習如何使用 Docker 輕鬆開發、部署和執行應用程式! **目錄**[介紹](#intro)[Docker 與虛擬機](#docker-vs-vm)[安裝](#installation)[基本指令](#basic-commands)[範例:Jenkins 容器](#example-jenkins)[資料持久化](#data-persistence)[資料持久化-卷](#data-persistence-volumes)[最後的想法](#final-thoughts)[資源](#resources) 介紹 -- 如果你谷歌一下*Docker* ,你會發現 Docker 是一個使用作業系統級虛擬化來建立自包含容器的軟體平台。 幸運的是,我會用簡單的英語向您解釋這意味著什麼。 您可能已經使用 Oracle VM 或[Vagrant](https://letslearnabout.net/devops/vagrant-tutorial-beginners/)建立了多個虛擬機器。 Docker 就是類似的東西(但更好,稍後會詳細介紹)。 使用 Docker,我們選擇一個映像(將 Docker 映像視為配方)並下載它。然後,我們建立該映像或容器的實例,與虛擬機器非常相似。 #### 影像: 用於建立一個或多個容器的套件或模板 #### 容器: 影像的實例彼此隔離,有自己的環境。 但讓我們看看它的實際效果。這是一個docker映像程式碼: ``免費:23.04 執行 apt-get update 執行 apt-get install -y curl nginx` 還記得我說過 Docker 鏡像是一個食譜嗎?在此映像或*配方*中,Docker 取得 Ubuntu 23.04 版本,更新 SO,然後安裝*curl*和*nginx* 。 誠然,這是一個簡短的 Docker 映像版本,但它幫助我們直觀地了解了 Docker 的含義。 現在,使用這個鏡像,我們可以建立一個容器(想像一個虛擬機),它將建立一個類似 Linux Ubuntu 的虛擬機,已經更新,帶有curl和nginx。 我們公司的所有開發人員都可以使用相同的映像來安裝相同的程式、軟體包和版本。不再有「但是…但它可以在我的電腦上執行!」;現在每台計算機都有相同的規格。 Docker 與虛擬機 ----------- 但是…如果 Docker 建立了一個類似 VM 的容器,為什麼我們不只使用虛擬機器呢? 我可以從低層次的角度解釋Docker 容器如何比虛擬機器更好,甚至可以從另一個網站(如本網站)獲取一些很酷的資訊圖表,並解釋Docker 對每個容器使用相同的內核,使其輕量且快速,只需幾秒鐘即可旋轉一個容器: 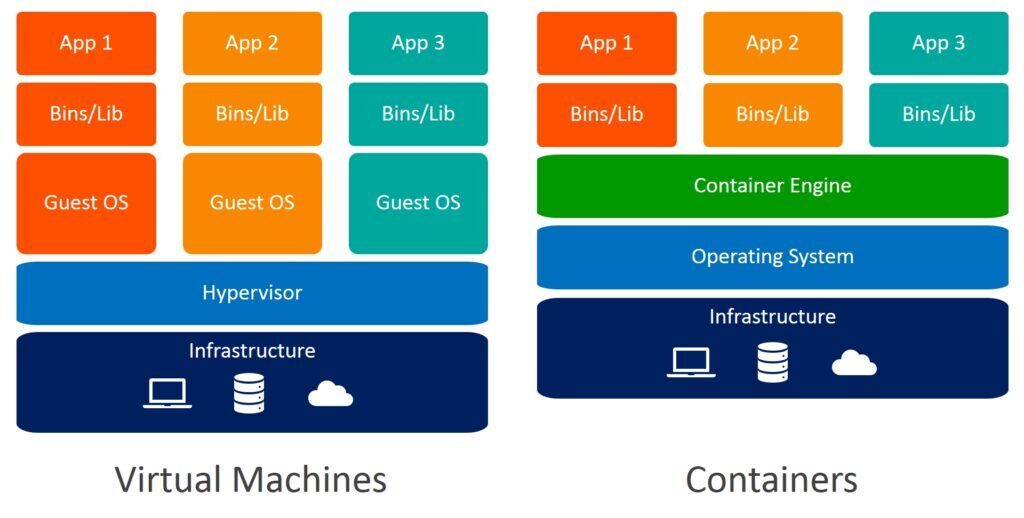 但使用 Docker 和 Docker 容器有一個很大的優點: 想像一下,您想要開發一個 21.1 NodeJS:您建立自己的 Docker 映像,在其中取得 Ubuntu 映像,更新它,安裝所有 NodeJS 相關的東西,然後將該映像分發給開發團隊。 在正常設定中,您必須上傳 NodeJS 應用程式,將其部署到您的伺服器上,並且您必須確保伺服器具有所有依賴項並且其 NodeJS 與您的伺服器相容。 而且你不想為此打賭。 使用 Docker,我們可以建立 Docker 映像,將其上傳到 Docker 相容的伺服器,僅此而已。 Docker 伺服器不在乎你使用什麼 Linux、安裝什麼軟體包或你的應用程式的語言是什麼:它只需要執行映像。就是這樣。 讓我強調這一點:我們不關心伺服器安裝了什麼。我們上傳並執行 Docker 映像。這就是我們所要做的。 安裝 -- 您可以安裝 Docker Desktop,這是一個 GUI Docker 應用程式,但我們這些強大的開發人員使用適當的終端工具,因此您將安裝 Docker Engine,即 Docker 的終端版本。 拋開笑話不談,您可以安裝任何您想要的內容: [Docker Desktop](https://docs.docker.com/desktop/)或[Docker Engine](https://docs.docker.com/engine/install/) ,只需確保遵循作業系統的說明即可。例如,對於基於 Debian 的發行版(例如 Ubuntu): 解除安裝先前的 Docker 版本 `sudo apt-get purge docker-ce docker-ce-cli containerd.io docker-buildx-plugin docker-compose-plugin docker-ce-rootless-extras sudo rm -rf /var/lib/docker sudo rm -rf /var/lib/containerd` 安裝 Docker `curl -fsSL https://get.docker.com -o get-docker.sh sudo sh ./get-docker.sh` 檢查 Docker 是否已安裝 `sudo docker version` 讓我們進行一個測試。在終端機中執行以下命令: `sudo docker run docker/whalesay cowsay boo` 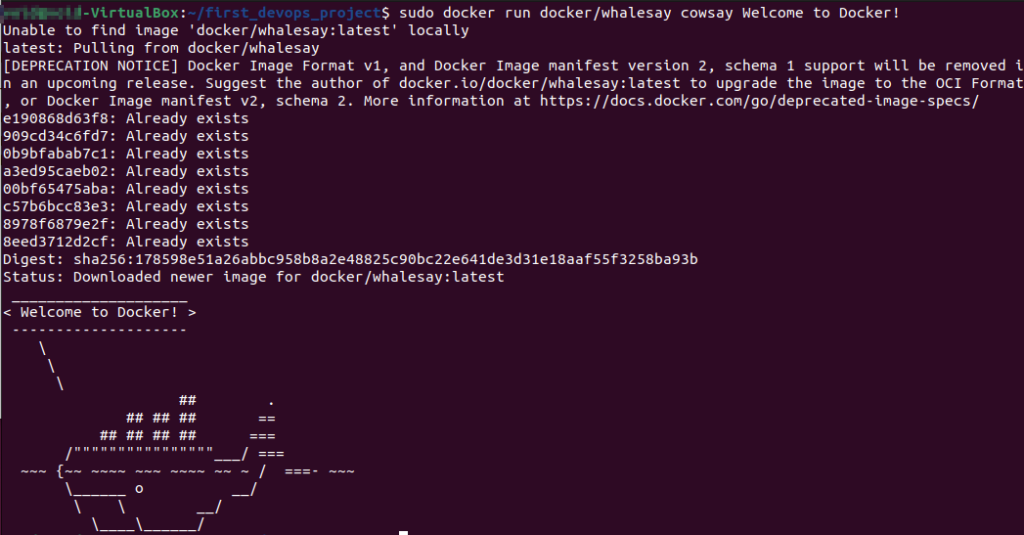 **重要提示:**每個 Docker 指令都需要 sudo 權限。您可以將使用者新增至*docker*群組,但儘管如此,它仍然不斷要求 sudo 權限。我發現透過執行命令`sudo chmod 666 /var/run/docker.sock` ,您不再被要求提供 sudo 權限(您可以使用類似的命令,例如*chmod +x* )。 基本指令 ---- 我們已經啟動並執行了 Docker。讓我們看看一些基本指令。如果你想要的話,你的麵包和黃油: 列出所有圖像 `docker images` 從鏡像下載或執行容器 `docker run <IMAGE_NAME>` 下載特定版本 `docker run <IMAGE_NAME>:<VERSION>` 在背景執行容器 `docker run -d <IMAGE_NAME>` 將容器從後台帶到前台 `docker run attach <ID>` 執行命令 `docker run ubuntu cat /etc/ *release* docker執行ubuntu睡眠15` 下載鏡像以便稍後執行 `docker pull <IMAGE_NAME>` 在docker容器內執行指令 `docker exec <COMMAND>` 連接到容器的 bash `docker run -it <IMAGE_NAME> bash` 列出所有正在執行的容器 `docker ps` 列出所有容器,無論是否執行 `docker ps -a` 執行一個帶有其他容器連結的容器: `docker 執行 -p : - 關聯: docker run -p 5000:80 --link redis:redis 投票應用` 從 JSON 格式的圖像或容器中獲取詳細訊息 `docker inspect <NAME_OR_ID>` 從背景執行的容器取得日誌 `docker logs <NAME_OR_ID>` 取得影像的所有圖層 `docker history <IMAGE_NAME>` 停止容器 `docker stop <IMAGE_NAME_OR_ID>` 永久刪除容器 `docker rm <IMAGE_NAME_OR_ID>` 永久刪除未使用的影像 `docker rmi <IMAGE_NAME>` 從 Dockerfile 建置映像 `docker build . -t <NAME>` 環境變數 `docker 執行 -e = docker run -e APP\_COLOR=blue simple-webapp-color` 範例:Jenkins 容器 ------------- 讓我們使用一個現實生活中的範例:使用 Jenkins 容器。 在以後的文章中,我將更深入地討論 Jenkins 及其功能,但 Jenkins 是一個很棒的 DevOps CI/CD 工具。讓我們下載 Jenkins 並在我們的電腦上執行它: `docker run jenkins/jenkins # 這會下載並執行 jenkins docker ps # 取得容器ID和端口 碼頭工人檢查\# 取得容器IP` 使用以下命令在虛擬機器中開啟瀏覽器: `docker run -p 8080:8080 jenkins/jenkins # Map the port` 使用以下命令在主機中開啟瀏覽器: 在這裡,我們在 Ubuntu 虛擬機器中安裝並下載 Docker 映像並執行它。我們可以透過開啟瀏覽器並使用 Docker 容器的 IP 和連接埠來查看虛擬機器中的 Jenkins,但透過映射端口,我們可以在主機中開啟 Jenkins。 結構是: 使用 Windows 主機 -> Linux VM -> 在 Linux 中執行的 Docker 容器 現在,Linux 正在執行一個輕量級 Docker 容器,我們可以從 Windows 電腦存取它。那不是很好嗎? 資料持久化 ----- 我們停止 Jenkins 容器,第二天我們恢復它以繼續工作。但我們已經失去了一切。發生了什麼事???? 僅 Docker 不具備資料持久性。 容器使用自己的資料夾(Jenkins 上的*/var/jenkins\_home* 、MySQL 上的*/var/lib/mysql*等),但是當您停止容器並再次執行映像時,您將從頭開始建立容器。我們對於它可以做些什麼呢? 我們可以透過連結執行Docker的作業系統中的資料夾和容器的資料夾來實現*資料持久化*。 `mkdir my\_jenkins\_data docker run -p 8080:8080 -v /home/ /my\_jenkins\_data:/var/jenkins\_home jenkins/jenkins` 在這裡,我們建立了一個名為*my\_jenkins\_data*的資料夾,並將其與 Jenkins 資料夾*/var/jenkins\_home*連結,Docker 在其中儲存所有變更。 因此,如果我們再次執行該命令,我們將建立一個新容器,連結儲存的訊息,就像我們正在恢復容器一樣。 資料容量的持久性 -------- 我們可以簡化這個過程。我們可以讓 Docker 透過在*/var/lib/docker/volumes/\**中建立磁碟區來管理磁碟區,而不是為我們的資料夾提供長字串。 建立卷 `docker volume create test_volume` 這會在 /var/lib/docker/volumes/test\_volume 中建立一個磁碟區 `docker run -v test_volume:var/lib/mysql mysql` 我們也可以使用現代的方式,它更長但更聲明性和冗長: `docker run / --mount type=bind, source=/data/mysql, target=/var/lib/mysql mysql` 最後的想法 ----- 正如我們剛剛看到的,Docker 之所以出色,有以下幾個原因: 1. **隔離性**:Docker允許應用程式與底層系統隔離,確保不同環境下的一致性。 3. **效率**:透過容器化優化資源利用率,更有效率地利用系統資源。 5. **可移植性**:Docker容器可以在任何安裝了Docker的機器上執行,從而可以輕鬆地在不同環境中部署應用程式。 7. **可擴展性**:使用 Docker,可以根據需求透過增加或減少容器數量來輕鬆擴展應用程式。 9. **一致性**:Docker確保開發、測試和生產環境的一致性,減少「它在我的機器上執行」的問題。 11. **生態系統**:Docker 擁有豐富的生態系統,提供廣泛的工具和服務來補充容器化,使其成為應用程式部署和管理的多功能平台。 13. **部署**:Docker 讓部署變得更容易、更安全。我們不是管理套件及其版本,而是將 Docker 映像上傳到伺服器。 資源 -- [原帖](https://letslearnabout.net/devops/docker-tutorial-beginners/) [Vagrant 初學者教程](https://letslearnabout.net/devops/vagrant-tutorial-beginners/) [Docker 桌面](https://docs.docker.com/desktop/) [Docker引擎](https://docs.docker.com/engine/install/) [Docker 在 DevOps 中的作用](https://kodekloud.com/blog/role-of-docker-in-devops/) [碼頭工人中心](https://hub.docker.com/) --- 原文出處:https://dev.to/davidmm1707/docker-basics-for-beginners-49l9
是的,你沒聽錯,我的 GitHub 自述文件有淺色和深色模式,甚至是響應式的。在這篇文章中,我將簡要介紹我用來實現這一目標的技巧(以及使它變得困難的事情!) 但首先,讓我們看看我的個人資料在不同的螢幕尺寸和顏色偏好下是什麼樣子(或者親自嘗試一下 [GrahamTheDevRel 在 GitHub 上的個人資料](https://github.com/GrahamTheDevRel)! ## 深色模式 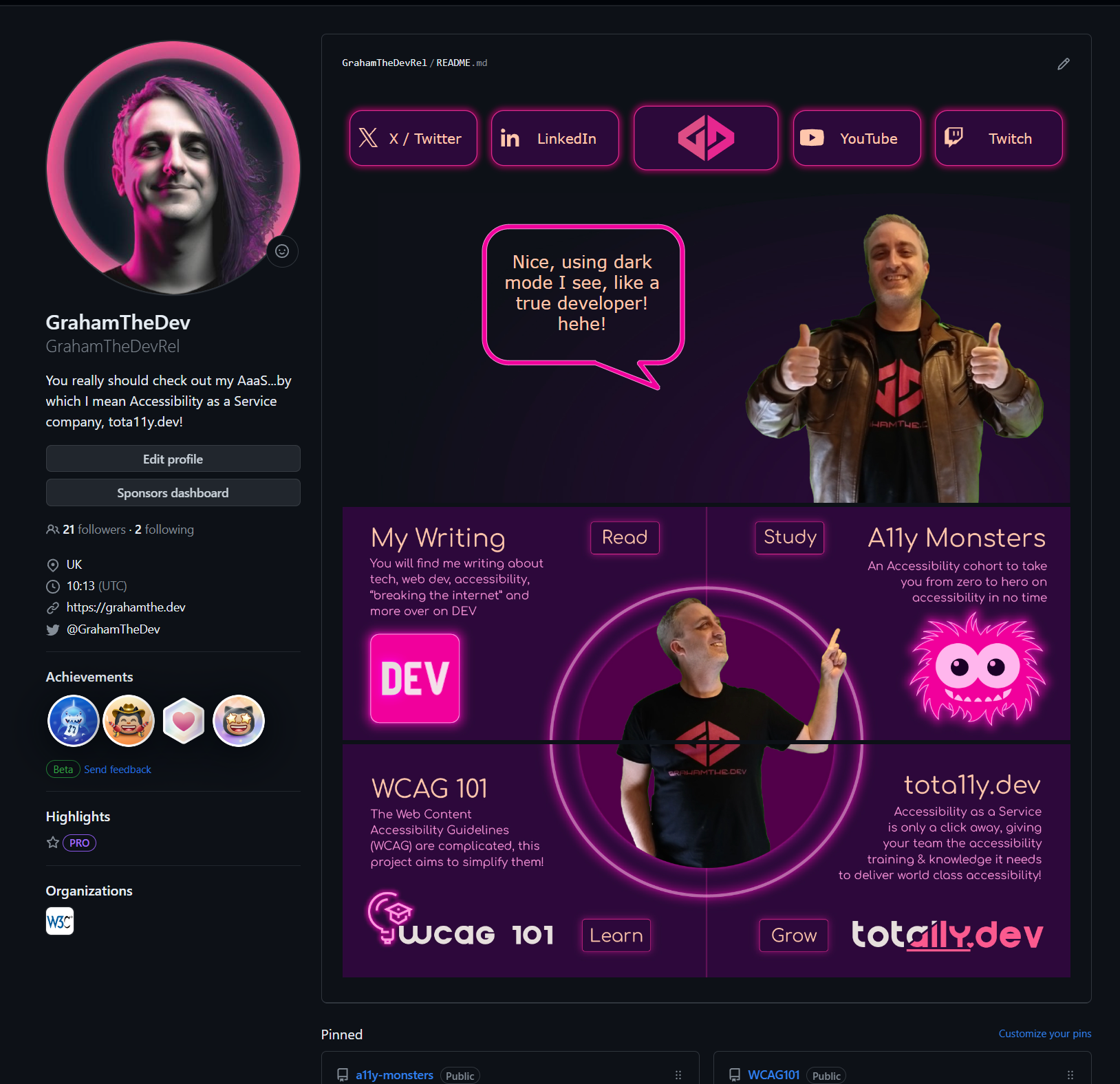 ## 移動的 請注意以下部分的不同按鈕設計和佈局。 建立這些按鈕比您想像的要困難得多! 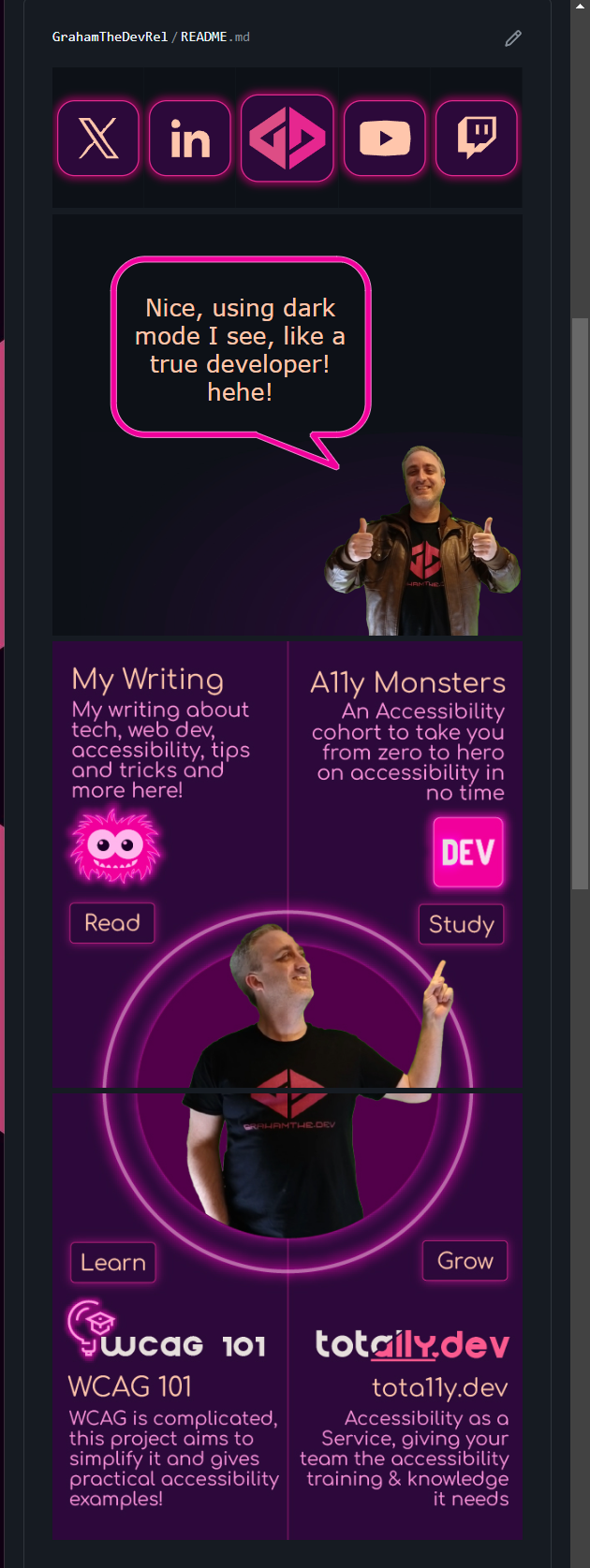 ## 燈光模式 我忍不住在英雄部分錶達了不同的訊息,當然我只是在開玩笑! 🤣💗 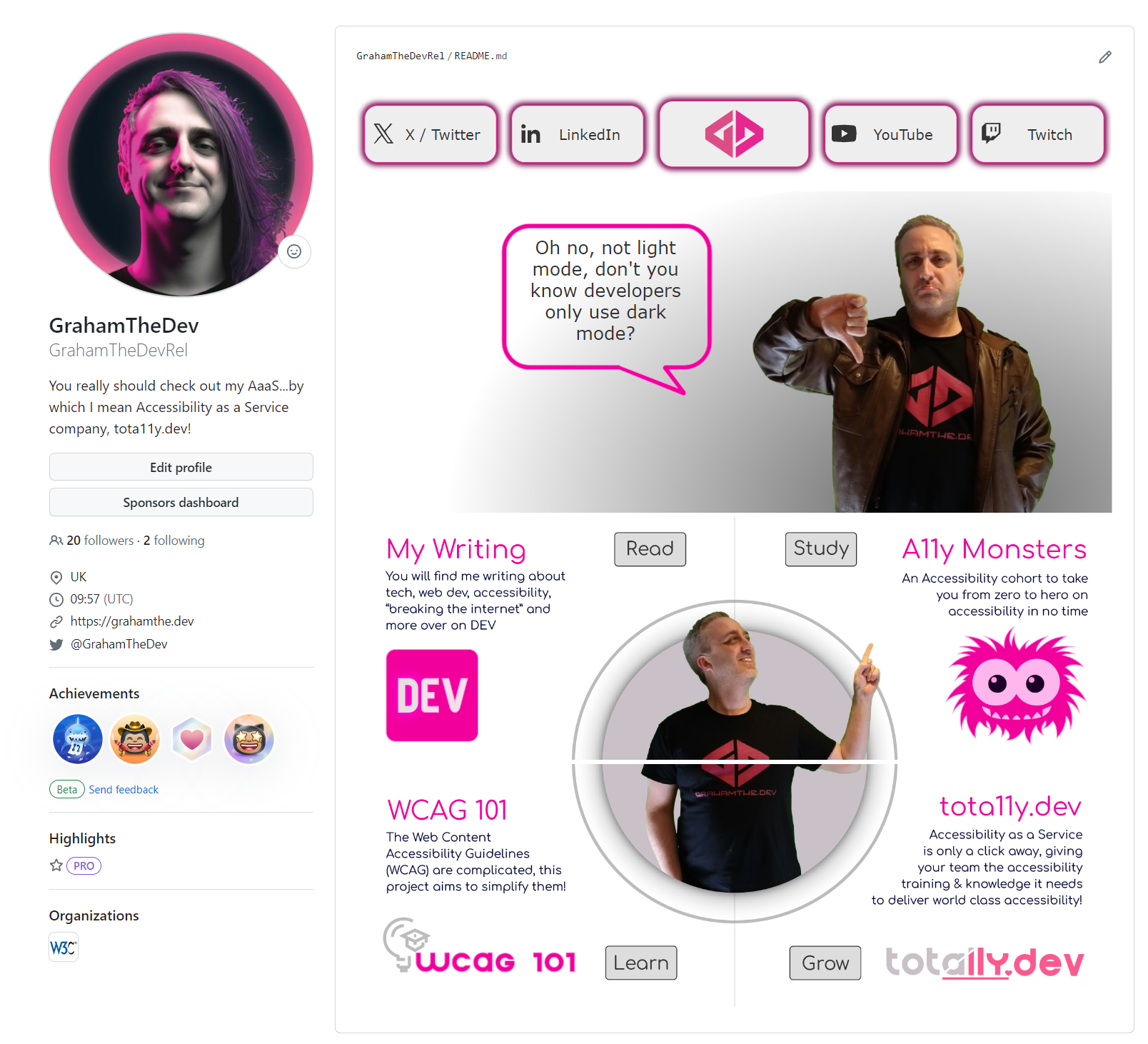 ## 喔...我有沒有提到它是動畫的? 請記住,前 5 個按鈕都是單獨的圖像!花了一點功夫才把它弄好! ## 使用了一些技巧! 好吧,所以你來這裡不僅僅是為了看我的個人資料! (如果你這樣做了,也愛你!🤣💗) 不,你是來學一些技巧的,這樣你就可以自己做對吧? 嗯,有一個“技巧”,然後只需一個 HTML 功能,您就可以自己完成此操作。 讓我們從最有趣的開始: ## 製作響應式按鈕和圖像的技巧! 網站上的按鈕和英雄圖像是有趣的部分。 為了讓它們發揮作用,我們使用了許多人沒有聽說過的 SVG 功能「<foreignObject>」。 ## `<foreignObject>` 和 SVG 獲勝! 這是使事物響應的最大技巧。 你看,在 markdown 文件中我們能做的事情非常有限(這就是為什麼我們看到人們使用 `<table>` 等進行佈局......ewww)。 但 SVG 有一個獨特的功能,[`<foreignObject>`](https://developer.mozilla.org/en-US/docs/Web/SVG/Element/foreignObject)。 這允許您在 SVG 中包含許多內容,**包括 HTML 和 CSS。** 有了它,我們就有了更多的力量! 您可以在 CodePen 的演示中看到(單擊按鈕可以更改外部容器的大小,它代表頁面上圖像的可用空間): ### SVG 中 CSS 的 CodePen 演示 **一定要查看 html 面板**,所有技巧都在那裡! https://codepen.io/GrahamTheDev/pen/mdomxyy 關鍵部分在這裡: ``` <svg xmlns="http://www.w3.org/2000/svg" fill="none"> <foreignObject width="100%" height="100%"> <div xmlns="http://www.w3.org/1999/xhtml"> <!--we can include <style> elements, html elements etc. here now, with a few restrictions! Note it must be in xHTML style (so <img src="" /> not <img src="" > to be valid --> </div> </foreignObject> </svg> ``` 從那裡我們可以使用內聯 `<style>` 元素和標準 HTML 元素來建立響應式映像。 但您可能會注意到該演示中使用的標記的另一件事。 ## 圖片很吸引人! 我將圖像(SVG 氣泡和我的圖像)作為 [`data` URL](https://developer.mozilla.org/en-US/docs/Web/HTTP/Basics_of_HTTP/Data_URLs )。 這是因為所謂的[內容安全策略 (CSP)](https://developer.mozilla.org/en-US/docs/Web/HTTP/CSP) 及其在 GitHub 上的實作方式。 現在我不會解釋 CSP,但本質上它們有一條規則:「嘿,除了當前上下文之外,不能從任何地方加載圖像」。 對於普通圖像來說這不是問題,但這是圖像中的圖像,並且該圖像的“上下文”就是其本身。 因此,如果我們嘗試在 SVG 中包含另一個圖像,它將來自不同的位置並破壞我們的 SVG。 幸運的是,「資料」 URI 被視為相同的上下文/來源。 這就是為什麼它們在我們的範例中使用的原因,如果您想自己實現的話,還需要考慮另一件事! ## 最後一個技巧,「<picture>」元素且沒有空格。 我的意思是,這甚至不是一個技巧! 我的自述文件中的最後 4 個框是響應式的(並尊重顏色偏好),但它們使用標準媒體查詢來工作。 這裡唯一的考慮是嘗試找到一個有效的斷點,恰好是 GitHub 上的 768px。 然後我建立了 4 組圖像: - 深色模式和桌面 - 黑暗模式和移動設備 - 燈光模式和桌面 - 燈光模式和移動。 ### 大或小圖像 為了獲得正確的圖像,我們在每個來源上對桌面(大)圖像使用“media =”(min-width:769px)`,對於移動(小)圖像使用“media =“max-width:768px)”放入我們的“<picture>”元素。 ### 淺色和深色模式 若要取得淺色或深色模式,我們使用[`prefers-color-scheme`](https://developer.mozilla.org/en-US/docs/Web/CSS/@media/prefers-color-scheme)媒體查詢。 ### 結合我們的查詢和來源 然後我們只需設定「<picture>」元素來使用「(**寬度查詢**)和(顏色首選項)」的組合來選擇我們想要的來源: ``` <picture> <source media="(min-width: 769px) and (prefers-color-scheme: light)" srcset="readme/[email protected]"> <source media="(max-width: 768px) and (prefers-color-scheme: light)" srcset="readme/[email protected]"> <source media="(max-width: 768px) and (prefers-color-scheme: dark)" srcset="readme/[email protected]"> <img src="readme/[email protected]" alt="You will find me writing about tech, web dev, accessibility, breaking the internet and more over on DEV! Purple and neon pink design with Graham pointing at the next section" width="50%" title="My writing on DEV"> </picture> ``` 本身並不困難,但建立 4 種圖像變體非常耗時。 ### 沒有空格 我遇到了最後一個問題。 底部實際上由 4 個不同的圖像組成(是的,我必須為其建立 16 個不同的圖像...)。 這樣做的原因是每個部分都是一個可點擊的連結。 並不複雜,但有一個小問題要注意。 如果您想要讓兩個影像直接並排接觸(因此兩個影像的寬度均為 50%),則必須刪除錨點、圖片元素甚至這些圖片元素內的來源之間的所有空白。 否則 GitHub 會為你的元素加入一些邊距,並且它們將不會在同一行。 另外,儘管我刪除了所有空白,但我遇到了另一個限制,但第一行和第二行之間仍然有 8px 的間隙,您無法遺憾地刪除它(因此之間的線!)。 ## 包起來! 我可能會在未來對內容安全策略、「<picture>」元素技巧,當然還有「<foreignObject>」做一些更深入的解釋。 這更多的是對概念的介紹,以便您可以自己使用它們,而不是教程。 但現在你已經了解我使用的技巧,我希望看到你建立一個比我的更漂亮的 GitHub 自述文件了! 如果您這樣做,請在評論中分享! 💪🏼🙏🏼💗 大家編碼愉快! 💗 --- 原文出處:https://dev.to/grahamthedev/take-your-github-readme-to-the-next-level-responsive-and-light-and-dark-modes--3kpc
你的轉職路上,還缺少一份自學作業包!寫完這幾包,直接拿作品去面試上班!
本論壇另有附設一個 LINE 新手發問&交流群組!歡迎加入討論!