63 個專案實戰,寫出作品集,讓面試官眼前一亮!
*揭露:這篇文章包含附屬連結;如果您透過本文中提供的不同連結購買產品或服務,我可能會獲得補償。* [ — 這個似乎徹底改變前端開發的工具。 但是,v0真的如同聽起來那麼棒嗎?我花了時間將它與一個新工具 [Webcrumbs Frontend AI](https://www.webcr...
JavaScript 持續演進,即將推出的 ECMAScript 2024 (ES15) 為語言帶來一系列新特性和改進。這些更新旨在提高開發者的生產力、代碼的可讀性和整體性能。讓我們探討 ES15 中一些最值得注意的新增功能。 ## 1. 增強的字串操作 ES15 引入了新的字串操作方法...
Encore.ts 是 TypeScript 的開源後端框架。本指南將引導您了解如何將[Express.js](https://expressjs.com/)應用程式遷移到[Encore.ts,](https://encore.dev)以獲得類型安全的 API 和 9 倍的效能提升。 為什麼要...
資料庫是當今(幾乎)每個軟體的重要組成部分。在這篇文章中,我將告訴您開始與他們合作所需了解的一切。 什麼是資料庫? ======= 如果您管理文件或資料夾中的訊息,您遲早會發現: - 您有多個包含相同資訊的文件 - 您有多個關於相同主題但資訊不同的文件,因此很難理解...
今天,我將向您展示如何建立一個 useDebounce React Hook,它可以非常輕鬆地對 API 呼叫進行反跳操作,以確保它們不會執行得太頻繁。我還製作了一個使用我們的鉤子的演示。它搜尋 Marvel Comic API 並使用 useDebounce 來防止每次按鍵時觸發 API 呼叫。 ...
> 您可以[在此處](https://dev.to/gruberb/web-programming-in-rust-02x-deploy-your-first-app-1k05)找到本系列的第二篇文章(「部署您的第一個 Rust 應用程式」)。 鐵鏽則不同。您可以在周末學習 Pytho...
人工智慧風靡一時,並且有大量的炒作。有人說這將改變我們所知道的世界(以錯誤的方式),而其他人則說這是一種時尚。 然而,正如埃隆馬斯克所說,“最有趣的結果是最有可能的。” 人工智慧不會殺死我們所有人,它也不是一種時尚。相反,它將提高我們的生產力,建立更複雜的系統。 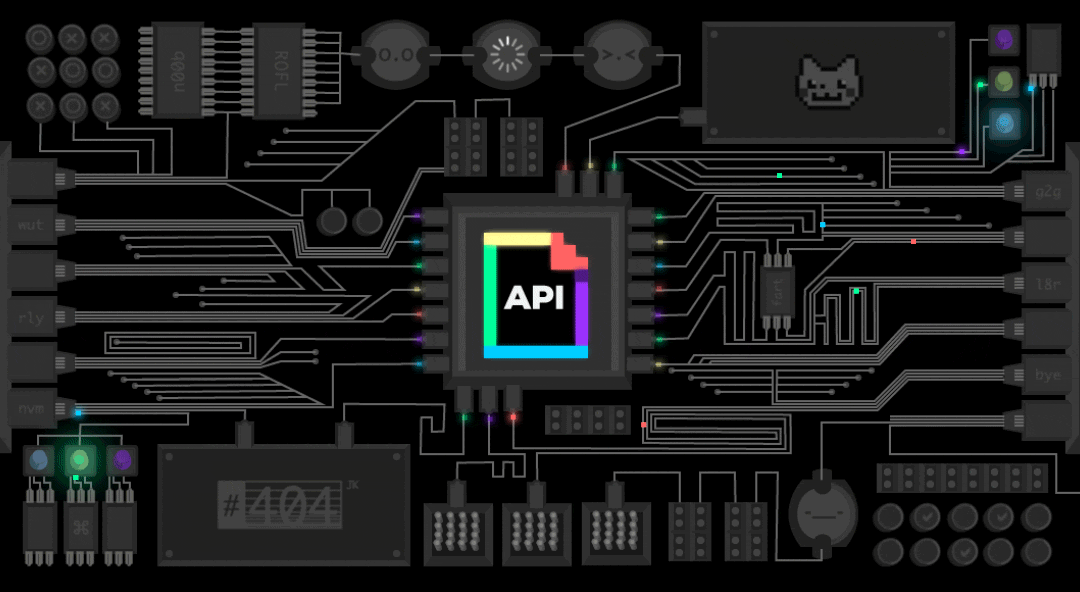 ### 1.Mapbox **API 範例** Mapbox 提供全面的工具和準確的位置資料,您可以使用它們...
介紹 -- 自從新冠疫情以來,我的日曆上充滿了站立會議、團隊會議和客戶電話。 然而,安排活動和邀請客人是無聊的任務。一個星期五,花了太多時間在這些上之後,我想—— 為什麼我要花這麼多時間在這上面? ![嚴重沮喪 GIF](https://media3.giphy.com/m...
簡介✨ ------ 在這個簡單易懂的教學中,您將學習如何使用 cron jobs從頭開始建立自己的 Instagram 自動化工具。 😎 **您將學到什麼:👀** - 了解如何在 Python 專案中設定**日誌記錄**。 - 學習使用**python-crontab**...
隨著 Web 應用程式變得越來越複雜,開發人員需要充分利用現代瀏覽器的全部功能。在本綜合指南中,我們將探索各種尖端的Web API,它們將在2024 年徹底改變Web 開發。使用者友善的Web經驗。 1. 付款請求API:簡化線上交易 ----------------- 付款請求 AP...
本教學將引導您完成使用 ToolJet 和 OpenAI 建立 Grammarly 替代方案的過程。我們將使用 ToolJet 的視覺化應用程式建構器為我們的應用程式設計優雅的使用者介面。然後我們將使用該平台的低程式碼查詢產生器與 OpenAI 連接以執行詳細的文字分析。完成的應用程式將允許您執行四...
所以,你決定要學習 Rust。 好的選擇! Rust 是一種很棒的語言,它將系統程式設計的強大功能與現代語言功能相結合,可用於 Web 開發和區塊鏈。 然而,在學習 Rust 時,阻礙者之一正在熟悉它的語法。 在本文中,我將盡力提供一些範例,讓您對它們感到滿意。 入門:變數和...
我已經使用 Python 和 JavaScript 進行開發,從事人工智慧和非人工智慧專案已經有一段時間了。 我注意到與人工智慧開發相關的生態系統很大程度上偏向 Python。然而,Javascript/Typescript 比 Python 有一些明顯的優點。 - **效能**:Jav...
精選技術文章、免費程式設計資源、以及業界重要新聞!
也歡迎訂閱 YouTube 頻道,觀看每週二晚間的《CodeLove Talk》直播節目,一起討論軟體開發相關的話題!