63 個專案實戰,寫出作品集,讓面試官眼前一亮!
使用 prisma 操作 db 會像這樣 ## Create ``` export async function action({ request }) { const formData = await request.formData(); const title = f...
上次撈到了商品資料 這次嘗試把加購的商品,連同主商品一起送出 先不實作動態撈加購 id 就先放一個實際商品 id 做測試 --- 這功能乍聽之下簡單,實際上做起來非常複雜 以預設的 theme Dawn 來說 購物車有三種模式 drawer, page, popu...
上次實作了撈取假資料的互動 現在來嘗試撈真的商品資料 我本以為會很簡單 因為商品資料都是公開的不是嗎? 卻發現其實 shopify 沒有很歡迎外部去撈店家的商品資料 所以 其實需要 app 授權 --- 在 toml 檔案裡面 remix 預設是 ``` ...
寫了 20 年 JavaScript 之後,我見證了許多變化——從回調地獄到 async/await。但即將推出的 JavaScript 特性將徹底改變我們寫程式的方式。 我們已經使用轉譯器和 polyfill 測試了這些提案,結果令人印象深刻:原來需要 30 行的程式碼現在只需 10 行,複...
我有時會想,我們每天所用的東西造就了我們。因此,您的工具越好,您作為專家的價值就越大。 在本文中,我準備了 10 個有趣的服務和模組的列表,它們將幫助您提高工作效率並讓您的生活變得更加輕鬆。 好吧,讓我們開始吧! 🏎️ --- [1.🐜HMPL.js](https://hmp...
成功有了基本檔案 現在試著開發我的推薦模組外掛 首先發現 在 theme editor 加入我的 app block 的話 沒辦法在右側選單 動態選取在 admin panel 建立好的 recommendation set 因為右側選單的內容 是寫死在 liquid 檔案內的 s...
人工智慧代理終於超越了聊天的範疇。他們正在解決多步驟問題、協調工作流程並自主操作。而這些突破的背後,正是MCP。 MCP 正在流行。但如果你對這些術語感到不知所措,那麼你並不孤單。 今天,我們將探討現有 AI 工具為何存在不足之處以及 MCP 如何解決這個問題。我們將介紹核心元件、它們的...
我曾經是將 TypeScript 推向**每個**專案的開發人員。後端? TypeScript。前端? TypeScript。一個五分鐘的腳本來自動重命名檔案?是的,甚至如此。這似乎是正確的舉措——畢竟,靜態類型讓一切變得更好,對吧? 嗯,並非總是如此。 多年來,我一直強迫自己將 Typ...
揭露:本貼文包含附屬連結;如果您透過本文提供的不同連結購買產品或服務,我可能會收到報酬。  、Nextjs 和[CopilotKit](https://go.copilotk...
揭露:本貼文包含附屬連結;如果您透過本文提供的不同連結購買產品或服務,我可能會收到報酬。 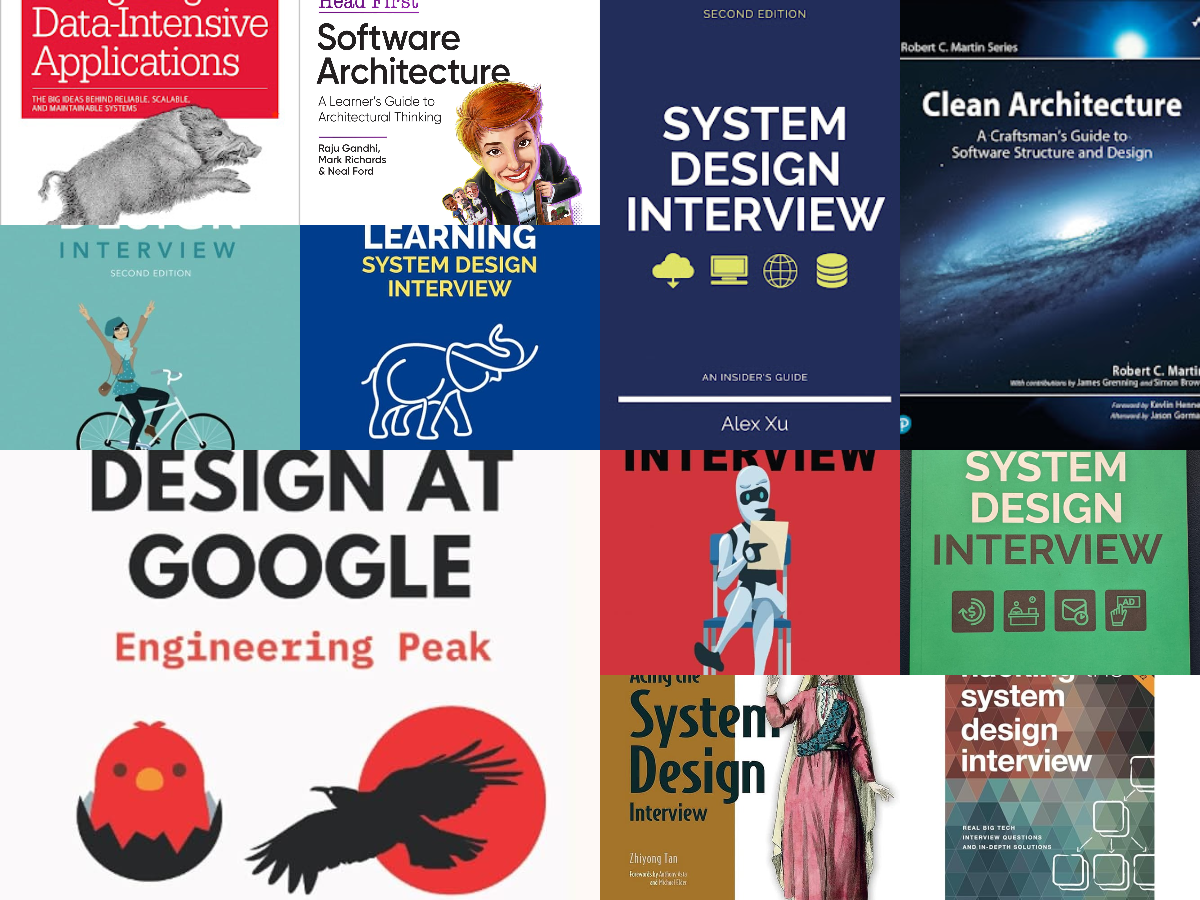 ...
歡迎來到高級 JavaScript 的世界!無論您是希望提高技能的經驗豐富的開發人員,還是渴望深入了解 JavaScript 複雜性的愛好者,本部落格旨在啟發和教育您。讓我們探索 20 個高級 JavaScript 技巧,這些技巧不僅可以增強您的編碼能力,還可以在您發現優化程式碼的新穎且令人興奮的方...
[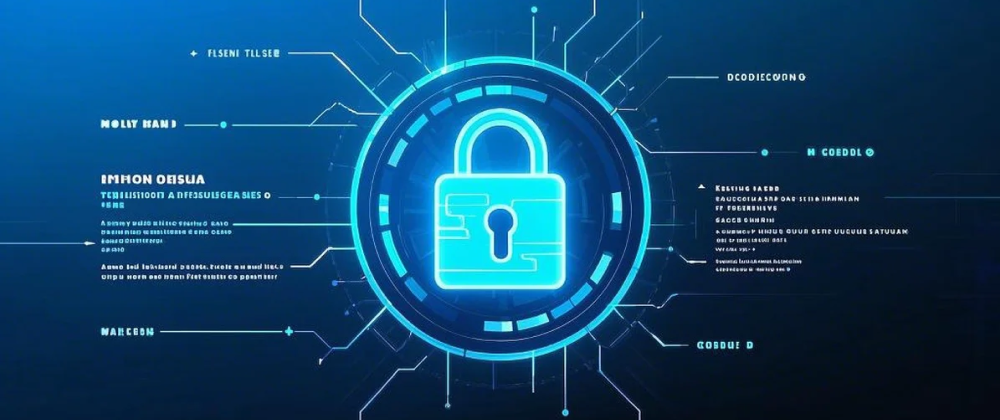](https://leapcell.io/?lc_t=d_jsauth) 在前端專案開發中,使用者認證主要有四種方...
DeepSeek-R1 在人工智慧界引起了不小的轟動。該模型由中國人工智慧公司[DeepSeek](https://www.deepseek.com/)開發,正在與 OpenAI 的頂級模型進行比較。 DeepSeek-R1 之所以令人興奮,不僅是因為它的功能,還因為它是開源的,任何人都可以下載並在...
總結 -- 在本教學中,我們將指導您使用[Anthropic AI API](https://www.anthropic.com/api) 、 [Pinecone API](https://www.pinecone.io/)和[CopilotKit](https://go.copilotki...
自訂鉤子不僅僅是 React 中的一個便利性——它們也是模組化和可維護程式碼的遊戲規則改變者。它們允許開發人員以以前不可能的方式封裝邏輯、管理狀態並簡化複雜的功能。 ** 。這種強大的不變性不僅僅是另一種方法 - 它是您編寫更安全、更可預測的程式碼的秘密武器✨。 老實說,當我第一次發現 Object.freeze() 時,我幾乎忽略了它。 「只是不要...
對於科技來說,這將是激動人心的一年,我們可能會看到跨多個領域、人工智慧工具、框架、資料庫等的突破性成就, 因此,我策劃了一些您必須用來建立下一個大型專案的開源工具。 ![新年卡通gif](https://dev-to-uploads.s3.amazonaws.com/uploads/ar...
嘿,開發人員! 因此,我最近一直致力於一些以 SEO 為重點的專案,我想我應該向 Next.js 開發人員分享一些我在這一過程中學到的最佳實踐和策略。 --- Next.js 2025 年 SEO 檢查表 ---------------------- **目錄** -...
精選技術文章、免費程式設計資源、以及業界重要新聞!
也歡迎訂閱 YouTube 頻道,觀看每週二晚間的《CodeLove Talk》直播節目,一起討論軟體開發相關的話題!