63 個專案實戰,寫出作品集,讓面試官眼前一亮!
如果您的測試可以自行編寫——只需像真實用戶一樣使用您的應用程式,那會怎麼樣? 在這篇文章中,我們探討了代理模式下的 Playwright MCP(模型上下文協議)如何自主導航您的應用程式、發現關鍵功能並產生可執行的測試——無需手動編寫腳本。 我們將透過現場演示來演示如何針對電影應用程式生...
你是否曾經因為等待一個重量級的 IDE 啟動而感到沮喪,或者為了執行一個本該很簡單的命令而不得不點擊 GUI 對話框?*每次我都覺得很煩!* 對許多企業開發者來說,命令列 (shell) 仍然是完成工作最快、最直接的方式。如今,新的工具正在將人工智慧帶入這個熟悉的環境:[基於 shell 的編...
我們需要談談困擾我幾個月的事情。我一直看到獨立駭客和新創公司創始人瘋狂地拼湊各種技術棧,用 Redis 做緩存,用 RabbitMQ 做隊列,用 Elasticsearch 做搜尋,還有用 MongoDB……為什麼? 我也犯過這種錯誤。當我開始建立[UserJot](https://userj...
在目前的市場中,找到適合自己的工作非常困難! 最近,我正在探索 OpenAI Agents SDK 並建立 MCP 代理程式和代理程式工作流程。 為了運用我的學習成果,我想,為什麼不解決一個真正的、常見的問題呢? 因此我建立了這個多代理求職工作流程來找到適合我的工作! 上不尋常的技術。因此,當我在[上一篇文章](https://dev.to/maxprilutskiy/creating-modal-windows-with-pure-cs...
讓我們討論如何建立很酷的新專案。如今,每個人都熱衷於編碼,您需要了解核心產品和 SAAS 基本要素,它們可以幫助您開始進行正確的使用者互動。 我說的是順利的入職、驗證細節以及為計劃存取的人個性化您的網站。這聽起來可能有點令人頭疼,但不該操之過急。你不希望這種事發生在你身上👇 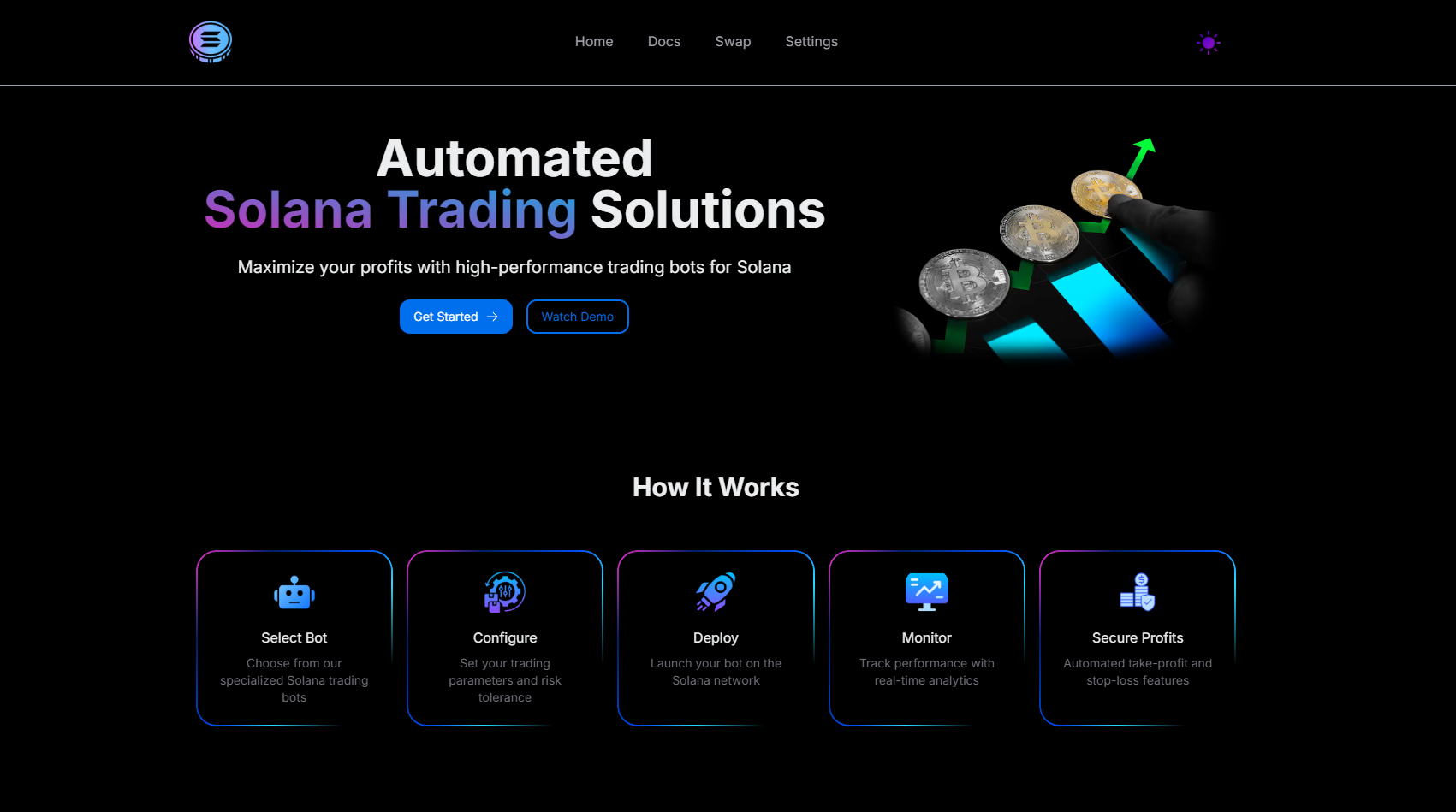 > ☝[整合 Solana 交易機器人平台](https://lucky-crypto-wha...
MCP 正在流行。人工智慧代理現在可以與真實的工具和應用程式對話並真正完成任務。 這解鎖了許多強大的用例。開發人員開始建立狂野的 MCP 伺服器。 今天,我們將了解 MCP,並探索 30 多個具有完整原始碼的 MCP 伺服器。 您將在幾乎每個範例中找到一個演示,並在最後找到一些有用...
模型上下文協定(MCP)是2025年的最新趨勢。 它使擴展您的工作流程變得更容易,並開啟許多強大的用例。 今天,我們將學習如何將 Cursor 與 100 多個 MCP 伺服器連接,最後提供一些很棒的範例。 讓我們開始吧。 --- ### 涵蓋哪些內容? 簡而言之...
**總結** 在本文中,您將學習如何使用 Langraph、CopilotKit 和 Tavily 建立結合人機互動功能的代理原生研究畫布應用程式。 在開始之前,我們將介紹以下內容: - 什麼是 AI 代理? - 使用 LangGraph Studio 建置和視覺化 Lang...
在我擔任[Vance](https://www.linkedin.com/posts/skysingh04_newintern-devops-aws-activity-7281995442278572033-zMkh)的 DevOps 工程師期間,我們在**AWS Glue**上執行了大約 80 個...
歡迎來到高級 JavaScript 的世界!無論您是希望提高技能的經驗豐富的開發人員,還是渴望深入了解 JavaScript 複雜性的愛好者,本部落格旨在啟發和教育您。讓我們探索 20 個高級 JavaScript 技巧,這些技巧不僅可以增強您的編碼能力,還可以在您發現優化程式碼的新穎且令人興奮的方...
隨著我們進入 2025 年,後端開發的格局正在發生根本性的轉變。當今的開發人員面臨著更複雜的決策,需要平衡傳統的穩定性和現代的效能需求。 ### 為什麼 2025 年框架選擇至關重要 選擇正確的後端框架的風險從未如此高。隨著人工智慧應用、即時處理要求和微服務架構的爆炸性增長,您的框架選擇...
#### **🤖 Ollama** Ollama 是一個 **在本地運行大型語言模型 (LLMs) 的框架**。它讓你可以 **下載、運行並與 AI 模型互動**,而無需依賴雲端 API。 🔹 **範例:** `ollama run deepseek-r1:1.5b` – 本地運行 De...
DeepSeek-R1 在人工智慧界引起了不小的轟動。該模型由中國人工智慧公司[DeepSeek](https://www.deepseek.com/)開發,正在與 OpenAI 的頂級模型進行比較。 DeepSeek-R1 之所以令人興奮,不僅是因為它的功能,還因為它是開源的,任何人都可以下載並在...
自訂鉤子不僅僅是 React 中的一個便利性——它們也是模組化和可維護程式碼的遊戲規則改變者。它們允許開發人員以以前不可能的方式封裝邏輯、管理狀態並簡化複雜的功能。  ,一個超級方便的自動 API 文件產生工具。 LiveAPI的後端是Golang,我正在發現Golang獨特而酷的功能。 對於那些不知道的人來說, [Golang(Go...
對於科技來說,這將是激動人心的一年,我們可能會看到跨多個領域、人工智慧工具、框架、資料庫等的突破性成就, 因此,我策劃了一些您必須用來建立下一個大型專案的開源工具。 ](https://leapcell.io/?lc_t=d_js) Express 是 Node.js 中極為常...
精選技術文章、免費程式設計資源、以及業界重要新聞!
也歡迎訂閱 YouTube 頻道,觀看每週二晚間的《CodeLove Talk》直播節目,一起討論軟體開發相關的話題!