## 課程目標 認識變數作用域 認識函式的不同寫法與特性 ## 課程內容 來認識一些程式語言觀念與名詞 ``` <button onclick="action1()"> global scope </button> <button onclick="action2()"> local scope </button> ``` ``` var counterA = 0; function action1() { counterA = counterA + 1; alert(counterA); } function action2() { var counterB = 0; counterB = counterB + 1; alert(counterB); } ``` 到 jsfiddle 跑跑看,會發現第一個計數器會不斷 +1 疊加上去;第二個計數器卻永遠顯示 1 這就是變數作用域的區別:變數宣告在很外面的,會在很大的範圍內都可使用這變數;變數宣告在很裡面的,只在裡面的範圍內才可使用這變數 宣告在最外面的稱為全域變數(global),反之則稱為區域變數(local) 目前為止的作業,其實你已經到處在寫 global 與 local 變數了,這觀念還算簡單、直觀 --- 用精確的技術名詞來說明的話 在 ES6 (2015) 之前,JavaScript 中的變數作用域只有 Global Scope 跟 Function Scope 兩種 並且,在使用 `var` 關鍵字時,要留意一種名為 Hoisting 的現象,這是一種會讓人搞錯變數作用域的現象 在 ES6 之後,有了 `const` 與 `let` 兩種新關鍵字,宣告的變數為 Block Scope 使用這兩種關鍵字,就不會出現 Hoisting 的現象 --- 我個人認為,Hoisting 是一個設計失敗的程式語言特性 應該要讓 JavaScript 引擎直接報錯、程式直接壞掉比較好 一般程式語言沒有 Hoisting 這種現象,此為 JavaScript 獨有特性 這是當年 Netscape 瀏覽器公司,為了衝市占率、歡迎大家亂寫 JS 程式碼的產物 我不想細談 Hoisting,反正改天你真的遇到問題,大概知道要往這方向研究就是了 --- 實務上,現在大家都寫 `const` 與 `let`,比較不寫 `var` 了 所以 Function Scope 跟 Block Scope 的差別在哪? 簡單來說,這樣的程式碼,x 正常顯示,y 會報錯 ``` if (true) { var x = 1; const y = 2; } alert(x); alert(y); ``` `var` 會覺得變數作用域,只有 `function 函式` 內、外的差別,內就是同樣 local,外就是 global `const` `let` 會覺得變數作用域,每次遇到 `大括號 {}` 都算一次內、外的差別,大括號裡面就是 local,裡面的裡面就是 local 中的 local 看不太懂沒關係,總之,變數宣告時,遇到 bug,就往前面找大括號,把變數搬來搬去,試試看,會慢慢搞懂的 本課先教你區分 global 與 local 兩種概念就好,這在大多數程式語言都是通用概念 在本系列教材內容以及作業中,`const` `let` `var` 隨便混著用,都可以 大概知道當前變數是 global 還是 local 就好 反正改天你真的遇到問題,大概知道要往這方向研究就是了 --- 接下來談一談 JavaScript 中的函式 之前的課程中,有過這樣的範例 ``` <button id="my-btn">Click me</button> ``` ``` function myFunction() { alert('你點擊了按鈕!'); } var btn = document.getElementById('my-btn'); btn.onclick = myFunction; ``` `myFunction` 被當成變數一樣,被指派給一個物件的屬性了 在很多程式語言中,函式是不能這樣使用的!函式永遠只能單獨加上小括號去執行 `myFunction()` 這個差別有點像是,其他程式語言認為變數是「名詞」,函式是「動詞」。那些語言認為這樣才能溝通、描述世界 而 JavaScript 認為變數是「名詞」,函式是「動詞」也是「動名詞」,也就是認為函式也是一種「名詞」。JavaScript 認為這樣才能溝通、描述世界 中文說「我開車」跟「開車很好玩」,沒有在管「開車」是動詞還是名詞,中文使用者就是習慣這樣溝通 英文說「I drive」跟「Driving is fun」,句子裡面主詞的部份一定要是名詞,如果想放動詞,就先改寫成 +ing 動名詞,英文使用者就是習慣這樣溝通 上面通通看不懂沒關係,反正知道各種程式語言,都是設計者與社群的主觀偏好,然後都能完成任務、各有不同長處短處就好 --- 最後,跟大家談一下函式的不同寫法 ``` function func1() { alert(1); } var func2 = () => { alert(2); } func1(); func2(); ``` ES6 之後有所謂的箭頭函式 他跟傳統寫法的主要差別,在於對於 `this` 關鍵字的認定 在工程師主流推崇 OOP(物件導向程式設計)的年代,`this` 的使用很巧妙、也很讓人困惑 實務上現在寫前端,比較少用 OOP 寫法,稍微偏向 FP(函數式程式設計)多一點,所以 `this` 問題變比較小 我不想細談 `this` 以及兩種函式寫法的差別,在本系列教材內容以及作業中,隨便混著用,都可以 反正改天你真的遇到問題,大概知道要往這方向研究就是了 ## 課後作業 請使用 https://jsfiddle.net 用以下 html 為基礎(你可以稍微修改),id 跟 class 之類的你可以自由決定 ``` simple counter: <button>-</button> <button>+</button> <hr> simple calculator: <input type="text" /> <input type="text" /> <button>加/減/乘/除</button> ``` 這邊有兩個小型應用程式 第一個應用程式,是簡單的計數器 - 第一次點擊 + 號按鈕,會用 alert 跳出 1 - 第二次點擊 + 號按鈕,會用 alert 跳出 2 - 依此類推,每次點 + 都會遞增,每次點 - 都會遞減 - 你會宣告一個全域變數,記錄這個累積的值,才能完成此功能 第二個應用程式,是簡單的計算機 - 有兩個欄位可以輸入數字 - 點擊按鈕,連續跳出四個 alert,分別顯示「加/減/乘/除」的計算結果 - 例如:輸入 6 與 2 -> alert 顯示 8 -> alert 顯示 4 -> alert 顯示 12 -> alert 顯示 3 - 你會宣告兩個區域變數,分別記錄兩個輸入的值,接著用來進行四種計算,才能完成此功能 --- 做出以上功能,你就完成這次的課程目標了!
## 課程目標 認識並且能使用 Local Storage 相關功能 ## 課程內容 Local Storage 提供了前端開發者簡易的儲存功能,能夠把資料儲存在瀏覽器上 跟 cookie 不同的是,local storage 的資料不會挾帶在 http request 中發送,也就是伺服器端無法直接存取 並且 local storage 能儲存的資料大小,比 cookie 大很多 ``` Name: <input type="text"> Age: <input type="text"> <button onclick="save()">Save</button> ``` ``` if (localStorage.getItem("me")) { var me = JSON.parse(localStorage.getItem("me")); alert('hello, ' + me.name + ' (' + me.age + ')'); } function save() { var name = document.querySelectorAll('input')[0].value; var age = document.querySelectorAll('input')[1].value; var me = { name: name, age: age }; localStorage.setItem("me", JSON.stringify(me)); alert('saved'); } ``` 在這邊的範例中,使用 `localStorage.setItem()` 來儲存資料,使用 `localStorage.getItem()` 來取得資料 因為只能在 local storage 中儲存字串,所以使用 `JSON.stringify` 將物件轉換成字串,使用 `JSON.parse` 將字串轉換成物件 在 JavaScript 中,表達物件的字串格式又稱為 JSON 字串,反正就是一種格式而已,資料類型是字串 上述的程式碼,寫起來比 cookie 簡單很多,API 乾淨很多 實務上,如果儲存的資料,不需要在主機端使用,就都用 local storage 即可 --- 關於 local storage 的 debug 除錯,也要知道一下 跟 cookie 差不多 打開瀏覽器開發者工具 -> Application -> Storage -> Local Storage 可以看到網站上有哪些資料,也可以直接在這邊修改、刪除資料 ## 課後作業 請回頭找出你在系列課程二寫的作業 https://codelove.tw/@howtomakeaturn/course/vx8gqZ 當初的待辦事項小工具,少了一個重要功能:儲存按鈕 也就是重新整理之後,待辦事項就通通不見了 請在「匯出」按鈕旁邊,增加一個「儲存」按鈕,讓待辦事項在頁面重新整理之後,還可以繼續使用 - 按下按鈕之後,使用 local storage 儲存待辦事項資料,並跳出 alert 提示「儲存成功!」 - 頁面載入的時候,檢查 local storage,有資料的話,就復原到畫面上 做出以上功能,你就完成這次的課程目標了!
## 課程目標 認識並且能使用 Cookie 相關功能 ## 課程內容 大名鼎鼎的 cookie,很多人在科技新聞上都聽過 某某公司利用網頁 cookie 追蹤個資、某某公司偷偷用 cookie 分享個資 這類新聞提到的 cookie,就是本課在說的 cookie 目前為止的作業,你會發現只要重新整理網頁,畫面上的所有東西就都歸零回預設值了 因為我們說網頁 HTTP 協議是「無狀態」的,也就是每次打開網頁的「狀態」不會被保留 這樣的設計當然有好處以及方便的地方,因為每次打開網頁都是獨立事件、彼此不會互相影響,開發起來單純很多 但是在實務上,經常需要「有狀態」才能完成某些功能,例如「已登入帳號」的狀態,否則網站會變很難用 Cookie 技術就是為了這個原因而誕生 --- Cookie 主要有兩個特性 - cookie 的內容,主機端也能存取 - cookie 的內容,網頁重新整理之後還會保留 Cookie 會出現在 HTTP Request 內,一起送到主機 會員登入功能、各種廣告追蹤功能,就是這樣做到的 HTTP 協議不熟沒關係,反正就是前端工程師設定過 cookie 值之後,後端工程師也能在主機端拿到那些值就對了 --- 注意,由於 cookie 會包含一些網站上的敏感資訊,jsfiddle 等等線上程式碼實驗工具、以及各種免費架站工具,幾乎通通都關閉了 cookie 功能 我目前找到一個可以用 cookie 的架站工具 https://replit.com/ 本課請使用 replit 來跑範例程式碼、寫作業 註冊登入之後,點 Create -> Templates -> 選 HTML, CSS, JS 那個 -> Create Repl 就可以開始寫了,但是右邊的預覽視窗,因為是 iframe,一樣不能用 cookie 請點擊右上方的 Open in a new tab,就可以正常跑 cookie 功能了 --- 來看一些範例程式碼吧 ``` Your Age: <input type="text"> Your Name: <input type="text"> <button onclick="save()">Save</button> <script> if (document.cookie) { alert(document.cookie); var arr = document.cookie.split(';'); var myage = arr[0].split('=')[1]; var myname = arr[1].split('=')[1]; document.querySelectorAll('input')[0].value = myage; document.querySelectorAll('input')[1].value = myname; } function save() { var myage = document.querySelectorAll('input')[0].value; var myname = document.querySelectorAll('input')[1].value; document.cookie = 'myage=' + myage; document.cookie = 'myname=' + myname; alert('saved!'); } </script> ``` 這個範例中,輸入年齡、名字一次,接著重新整理網頁,會發現資料一樣存在欄位裡面,不用重新輸入! 存取值,都是透過 `document.cookie` 這個物件屬性,值的格式是 `name=value` 雖然 `document.cookie=` 寫了兩次,但第二次並不會蓋掉第一次的值,而是兩個都存進 cookie 了 (這個設計,很糟糕,沒錯,讓人看得很混亂。應該是瀏覽器的設計者,有他們的苦衷,我們先不細究原因,先知道怎麼用就好) 然後,讀取的時候,多個值會用分號 `;` 隔開,所以讀取時我用了 `.split()` 這個字串函式 除此之外,cookie 還可以設定「使用期限」以及「限定網域」,但我這邊就先不示範 總之,cookie 的效果大概就是這樣,稍微理解就好。然後這些值,後端工程師在伺服器端,也會收到,就這樣! --- 如上所示,cookie 寫起來,有點麻煩 實務上,每次都這樣手寫,太囉唆,通常會直接找套件來用,我是用這款 https://github.com/js-cookie/js-cookie 用來操作 cookie 的 API,漂亮多了! --- 關於 cookie 的 debug 除錯,也要知道一下 打開瀏覽器開發者工具 -> Application -> Cookies 可以看到網站上有哪些 cookie,也可以直接在這邊修改、刪除 cookie --- cookie 還有一些特性,以及大小限制、使用限制 這邊不細談,稍微知道怎麼用 cookie 就好 有興趣的話,請上網搜尋一下,多研究一些 cookie 的細節 ## 課後作業 請使用 https://replit.com/ 來寫作業 假設你正在幫客戶寫一個「成人限制級網站」 頁面第一次打開會顯示蓋板警告,下方有兩個按鈕 ``` 警告︰您即將進入之網頁內容需滿十八歲方可瀏覽。 根據「兒童及少年福利與權益保障法」規定,本網站已於非闔家皆宜之網頁加以標示。若您尚未年滿十八歲,請點選離開。若您已滿十八歲,亦不可將本站之內容派發、傳閱、出售、出租、交給或借予年齡未滿18歲的人士瀏覽,或將本網站內容向該人士出示、播放或放映。 您年滿十八歲嗎? [離開] [是,我已年滿十八歲] ``` - 點擊離開,把用戶跳轉到 google 首頁 - 點擊確認,就關閉蓋板警告,在網頁上顯示一張美女 or 帥哥圖片:參考圖庫 https://unsplash.com/ - 只要按過確認,重新整理之後,蓋板警告就不會再跳出來 做出以上功能,你就完成這次的課程目標了!
Hooks 是 React 最強大的功能之一。 它們使我們能夠輕鬆地在應用程式的元件中重用功能。掛鉤的最大優點在於它們的可重用性——您可以跨元件和專案重用掛鉤。 以下是我在每個 React 專案中重複使用的七個最重要的鉤子。今天就試一試,看看它們在建置您自己的 React 應用程式時是否有幫助吧。 原文出處:https://dev.to/webdevhero-com/7-react-hooks-for-every-project-1jdo --- 在我們開始之前,要先澄清一下:並非每個自定義 React 鉤子都需要由您編寫。事實上,我將提到的所有鉤子都來自一個庫“@mantine/hooks”。 Mantine 是一個很棒的第三方庫,其中包含這些鉤子等等。他們將為您的 React 應用程式加入您能想到的幾乎所有重要功能。 您可以在 [mantine.dev](https://mantine.dev) 查看“@mantine/hooks”的文件。 ## `useIntersection` 鉤子 當用戶在您的應用程式中向下滾動頁面時,您可能想知道某個元素何時對他們可見。 例如,您可能希望僅在用戶看到特定元素時才啟動動畫。或者,您可能希望在他們向下滾動頁面一定程度後,顯示或隱藏元素。 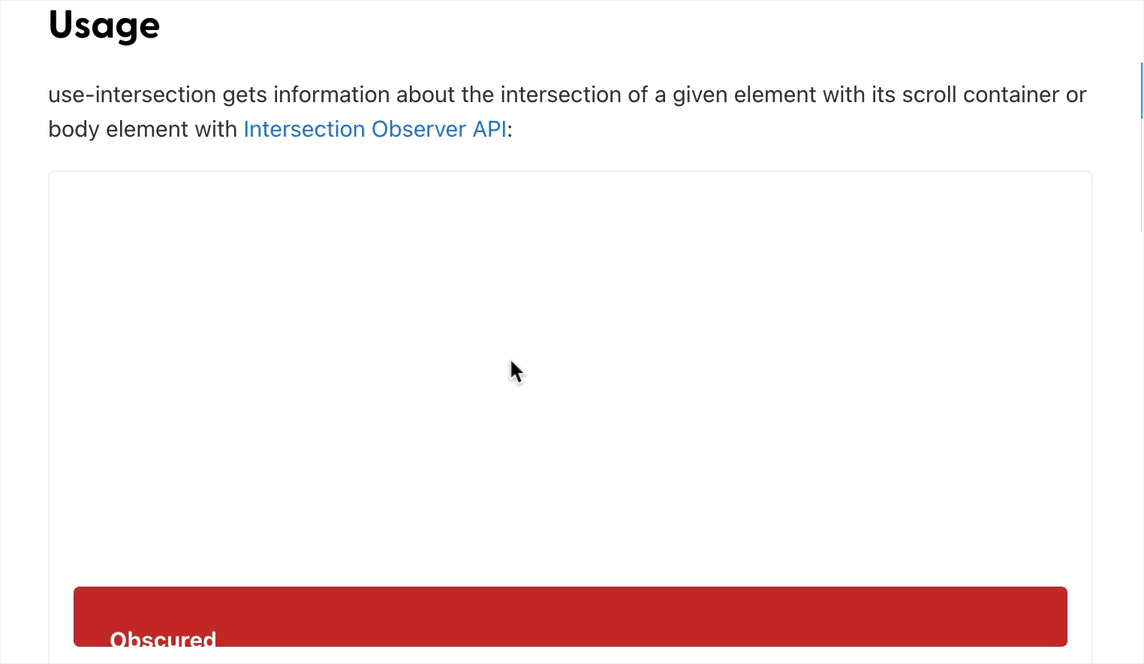 要獲取有關元素是否可見的訊息,我們可以使用 **Intersection Observer API。**這是瀏覽器中內建的 JavaScript API。 我們可以使用純 JavaScript 單獨使用 API,但要知道有關特定元素是否在其滾動容器內,有個好方法是使用 useIntersection 掛鉤。 ``` import { useRef } from 'react'; import { useIntersection } from '@mantine/hooks'; function Demo() { const containerRef = useRef(); const { ref, entry } = useIntersection({ root: containerRef.current, threshold: 1, }); return ( <main ref={containerRef} style={{ overflowY: 'scroll', height: 300 }}> <div ref={ref}> <span> {entry?.isIntersecting ? 'Fully visible' : 'Obscured'} </span> </div> </main> ); } ``` 要使用它,我們需要做的就是在我們的元件中呼叫鉤子,並提供一個根元素。 Root 是滾動容器,可以使用 useRef 掛鉤將其作為 ref 提供。 `useIntersection` 回傳一個我們傳遞給目標元素的 ref,我們想要觀察其在滾動容器中的交集。 一旦我們有了對元素的引用,我們就可以追蹤元素是否相交。在上面的範例中,我們可以根據 entry.isIntersecting 的值查看元素何時被遮擋或何時完全可見。 您可以傳遞其他參數,這些參數允許您配置與目標可見百分比相關的**閾值**。 ## `useScrollLock` 鉤子 另一個與滾動相關的鉤子是 useScrollLock 鉤子。這個鉤子非常簡單:它使您能夠鎖定 body 元素上的任何滾動。 我發現當您想在當前頁面上顯示疊加層或跳出視窗,並且不想讓用戶在後台頁面上上下滾動時,它會很有幫助。這使您可以將注意力集中在視窗上,或允許在其自己的滾動容器內滾動。 ``` import { useScrollLock } from '@mantine/hooks'; import { Button, Group } from '@mantine/core'; import { IconLock, IconLockOpen } from '@tabler/icons'; function Demo() { const [scrollLocked, setScrollLocked] = useScrollLock(); return ( <Group position="center"> <Button onClick={() => setScrollLocked((c) => !c)} variant="outline" leftIcon={scrollLocked ? <IconLock size={16} /> : <IconLockOpen size={16} />} > {scrollLocked ? 'Unlock scroll' : 'Lock scroll'} </Button> </Group> ); } ``` `useScrollLock` 將用戶的滾動鎖定在頁面上的當前位置。該函數回傳一個陣列,它可以被解構,如上面的程式碼所示。 第二個值是一個允許我們鎖定滾動的函數。另一方面,第一個解構值是一個布林值,它告訴我們滾動條是否已被鎖定。 這個值很有用,例如,如果你想在滾動鎖定時顯示某些內容或告訴用戶它已被鎖定。您可以在下面的示例中看到,當滾動條被鎖定或解鎖時,我們會在我們的按鈕中進行指示。 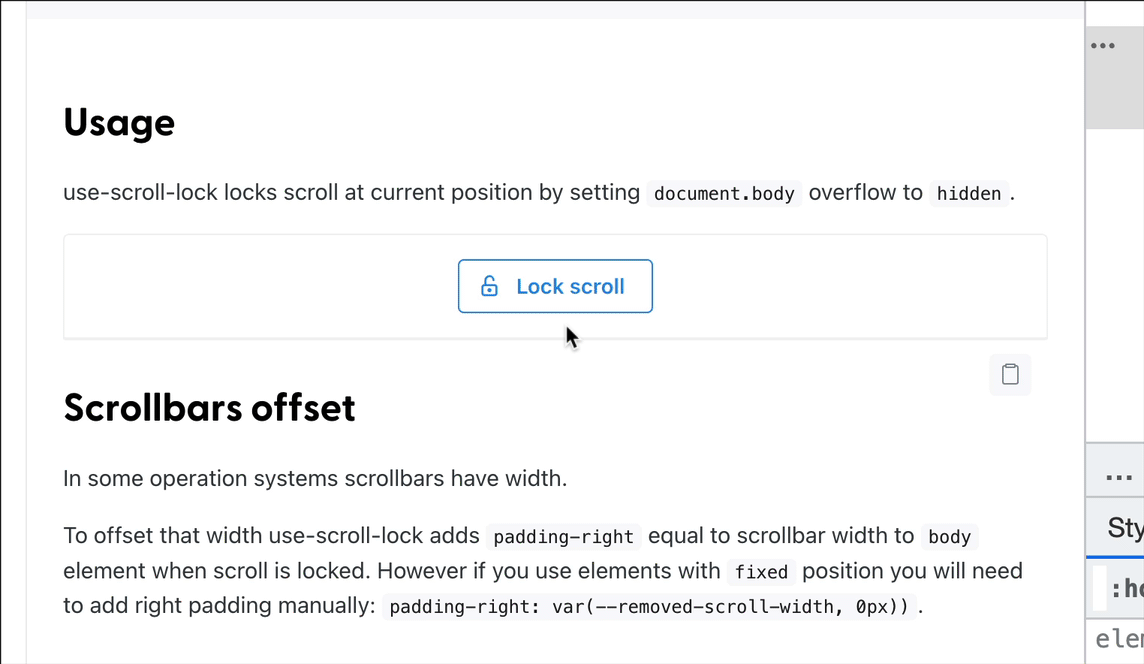 ## `useClipboard` 鉤子 在許多情況下,您希望提供一個按鈕,允許用戶將內容複製到他們的剪貼板,這是存儲複製文本的地方。 一個很好的例子是,如果您的網站上有一個程式碼片段,並且您希望用戶輕鬆複製它。為此,我們可以使用另一個 Web API——**剪貼板 API**。 `@mantine/hooks` 為我們提供了一個方便的 `useClipboard` 鉤子,它回傳幾個屬性:`copied`,它是一個布林值,告訴我們是否已使用鉤子將值複製到剪貼板,以及` copy` 函數,我們可以將我們喜歡的任何字串值傳遞給它以進行複制。 在我們的範例中,我們想複製一個程式碼片段,供我們的用戶粘貼到他們喜歡的地方,如下面的影片所示: 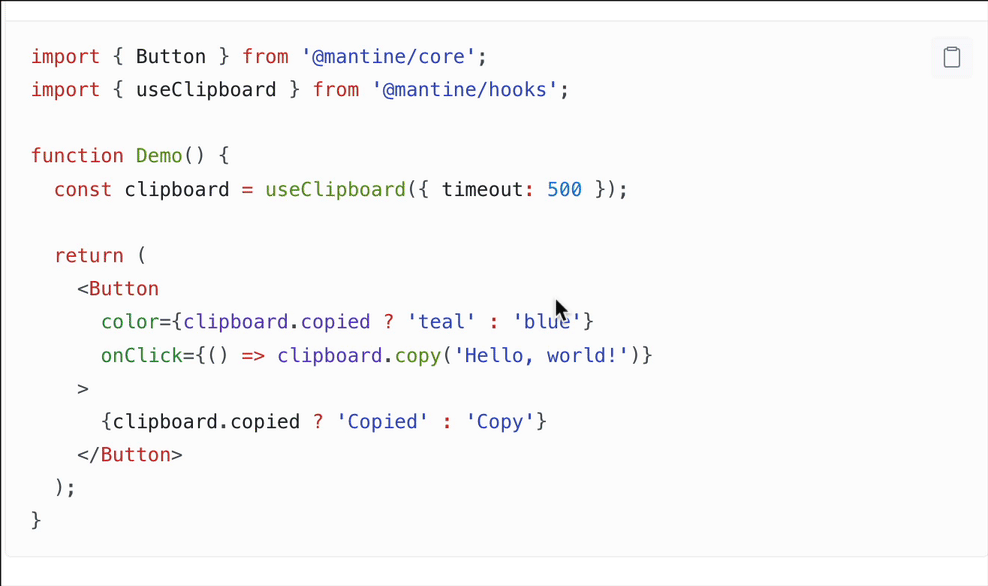 ## useClipboard demo 當他們點擊我們指定的複制按鈕時,我們呼叫我們的 `copy` 函數,將程式碼片段傳遞給它,然後顯示一個小複選標記或向他們表明文本已被複製的東西。 巧妙的是 `useClipboard` 掛鉤帶有 **超時值**。在給定的超時時間(以毫秒為單位)之後,複製的狀態將被重置,向用戶顯示他們可以再次復製文本。 ## `useDebouncedValue` 鉤子 如果您的應用程式中有搜尋輸入,下一個鉤子“useDebouncedValue”是必不可少的。 每當用戶使用輸入執行搜尋時,搜尋操作通常涉及對 API 的 HTTP 請求。 您將遇到的一個典型問題是每次擊鍵都會執行查詢(請求),尤其是如果您希望用戶在鍵入時接收搜尋結果。即使對於一個簡單的搜尋查詢,也不需要在用戶完成輸入他們想要的內容之前執行這麼多請求。 這是 useDebounceValue 掛鉤的一個很好的用例,它對傳遞給它的文本應用「防抖」功能。 ``` import { useState } from 'react'; import { useDebouncedValue } from '@mantine/hooks'; import { getResults } from 'api'; function Demo() { const [value, setValue] = useState(''); const [results, setResults] = useState([]) const [debounced] = useDebouncedValue(value, 200); // wait time of 200 ms useEffect(() => { if (debounced) { handleGetResults() } async function handleGetResults() { const results = await getResults(debounced) setResults(results) } }, [debounced]) return ( <> <input label="Enter search query" value={value} style={{ flex: 1 }} onChange={(event) => setValue(event.currentTarget.value)} /> <ul>{results.map(result => <li>{result}</li>}</ul> </> ); } ``` 您使用 useState 將輸入的文本儲存在一個狀態中,並將狀態變數傳遞給 useDebouncedValue 。 作為該掛鉤的第二個參數,您可以提供一個等待時間,即值被「反抖」的時間段。反抖使我們能夠執行更少的查詢。 您可以在下面的影片中看到結果,用戶在其中鍵入內容,並且僅在 200 毫秒後,我們才能看到去抖值。 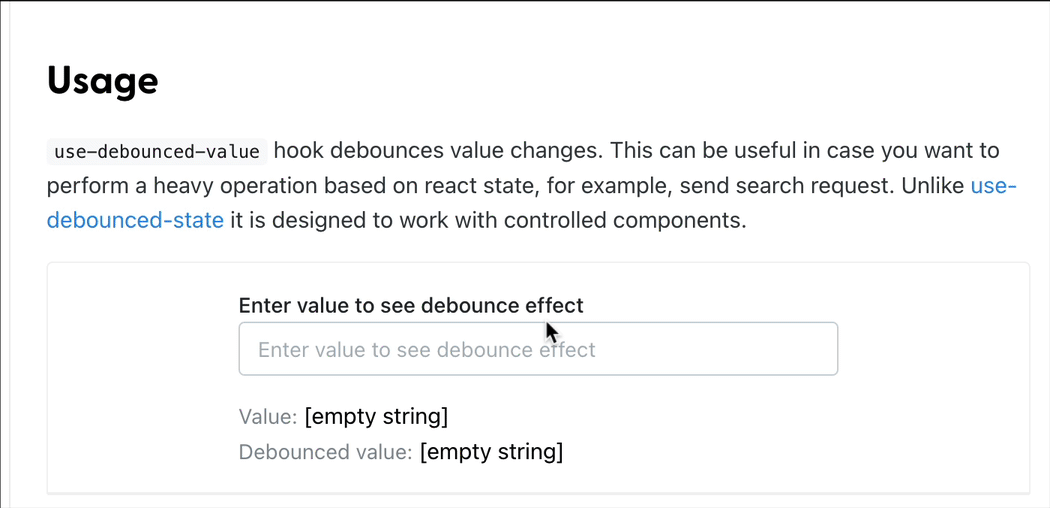 ## `useMediaQuery` 鉤子 我一直使用的另一個非常有用的鉤子是 useMediaQuery 鉤子。 Media Queries 在純 CSS 中使用,`useMediaQuery` 鉤子允許我們訂閱我們傳遞給鉤子的任何媒體查詢。 例如,在我們的元件中,假設我們想要顯示一些文本或根據特定螢幕寬度(例如 900 像素)更改元件的樣式。我們像在 CSS 中一樣提供媒體查詢,並且 useMediaQuery 回傳給我們一個 true 或 false 的 matches 值。 ``` import { useMediaQuery } from '@mantine/hooks'; function Demo() { const matches = useMediaQuery('(min-width: 900px)'); return ( <div style={{ color: matches ? 'teal' : 'red' }}> {matches ? 'I am teal' : 'I am red'} </div> ); } ``` 它用 JavaScript 告訴我們媒體查詢的結果,這在我們想要使用 `style` 屬性在 JSX 中直接更改樣式時特別有用,例如。 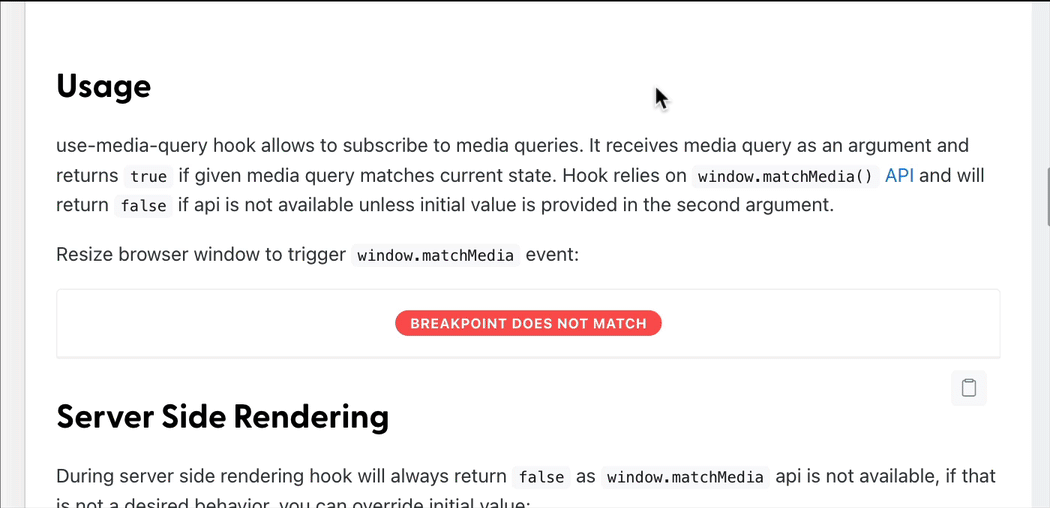 簡而言之,對於少數無法使用 CSS 處理媒體查詢的情況,這是一個必不可少的鉤子。 ## `useClickOutside` 鉤子 下一個掛鉤 - `useClickOutside` - 可能看起來很奇怪,但當您真正需要它時,您會發現它的重要性。 當你開發一個下拉菜單,或者在頁面內容前面彈出,並且之後需要關閉的東西時,這個鉤子是必不可少的。通過單擊按鈕打開這些類型的元件之一非常容易。關閉這些元件有點困難。 為了遵循良好的 UX 實踐,我們希望任何阻礙用戶視圖的東西都可以通過單擊元素外部輕鬆關閉。這正是 useClickOutside 掛鉤讓我們做的。 當我們呼叫 useClickOutside 時,它會返回一個 ref,我們必須將其傳遞給我們想要檢測點擊的外部元素。通常該元素將由一個布林狀態片段控制,例如我們在下面的示例中的狀態(即值“opened”)。 ``` import { useState } from 'react'; import { useClickOutside } from '@mantine/hooks'; function Demo() { const [opened, setOpened] = useState(false); const ref = useClickOutside(() => setOpened(false)); return ( <> <button onClick={() => setOpened(true)}>Open dropdown</button> {opened && ( <div ref={ref} shadow="sm"> <span>Click outside to close</span> </div> )} </> ); } ``` `useClickOutside` 接受一個回調函數,該函數控制當您實際單擊該元素外部時發生的情況。 在大多數情況下,我們想做一些非常簡單的事情,就是關閉它。為此,您可能需要一個狀態設置器(如 `setOpened`)並向其傳遞一個 false 值,然後隱藏您覆蓋的內容。 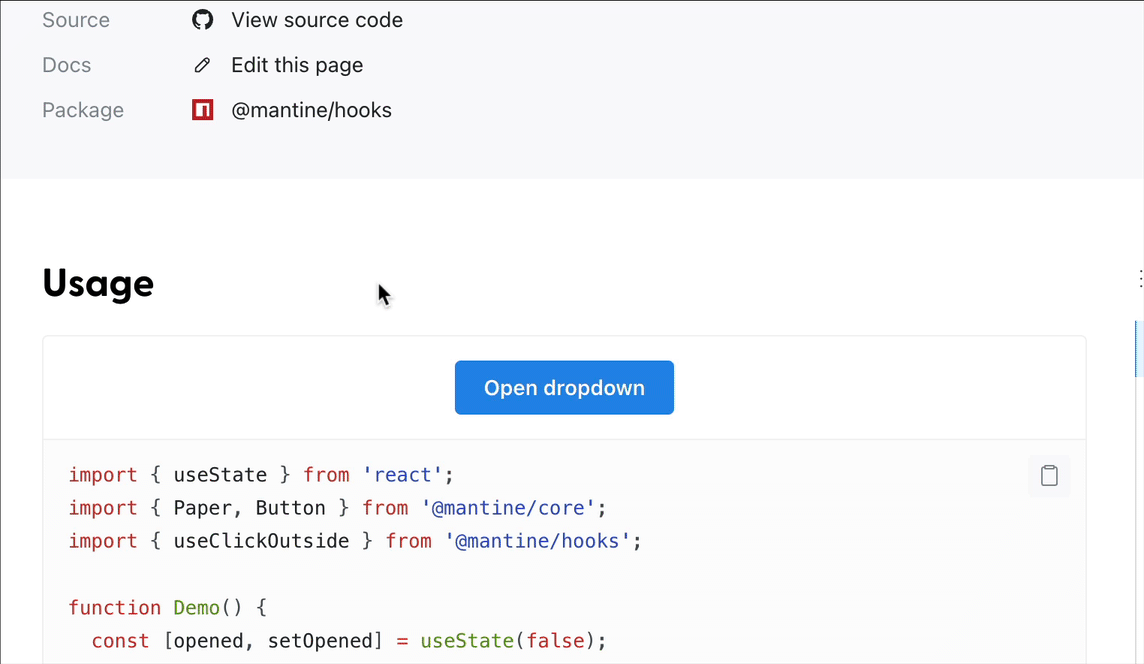 ## `useForm` 鉤子 在這個列表中,我最喜歡和最有用的鉤子是 `useForm` 鉤子。 這個鉤子專門來自 Mantine,涉及從庫中安裝一個特定的包:`@mantine/form`。它會為您提供在 React 中建立表單所需的一切,包括驗證輸入、顯示錯誤訊息以及在提交表單之前確保輸入值正確的能力。 `useForm` 接受一些初始值,這些初始值對應於您在表單中的任何輸入。 ``` import { TextInput, Button } from '@mantine/core'; import { useForm } from '@mantine/form'; function Demo() { const form = useForm({ initialValues: { email: '' }, validate: { email: (value) => (/^\S+@\S+$/.test(value) ? null : 'Invalid email'), }, }); return ( <div> <form onSubmit={form.onSubmit((values) => console.log(values))}> <TextInput withAsterisk label="Email" placeholder="[email protected]" {...form.getInputProps('email')} /> <Button type="submit">Submit</Button> </form> </div> ); } ``` `useForm` 的最大好處是它的助手,例如 `validate` 函數,它接收輸入到每個輸入的值,然後允許您建立驗證規則。 例如,如果您有一個電子郵件輸入,您可能有一個正則表達式來確定它實際上是否是一個有效的電子郵件(如您在上面的程式碼中所見)。如果沒有,那麼您可以顯示一條錯誤訊息並阻止提交表單。 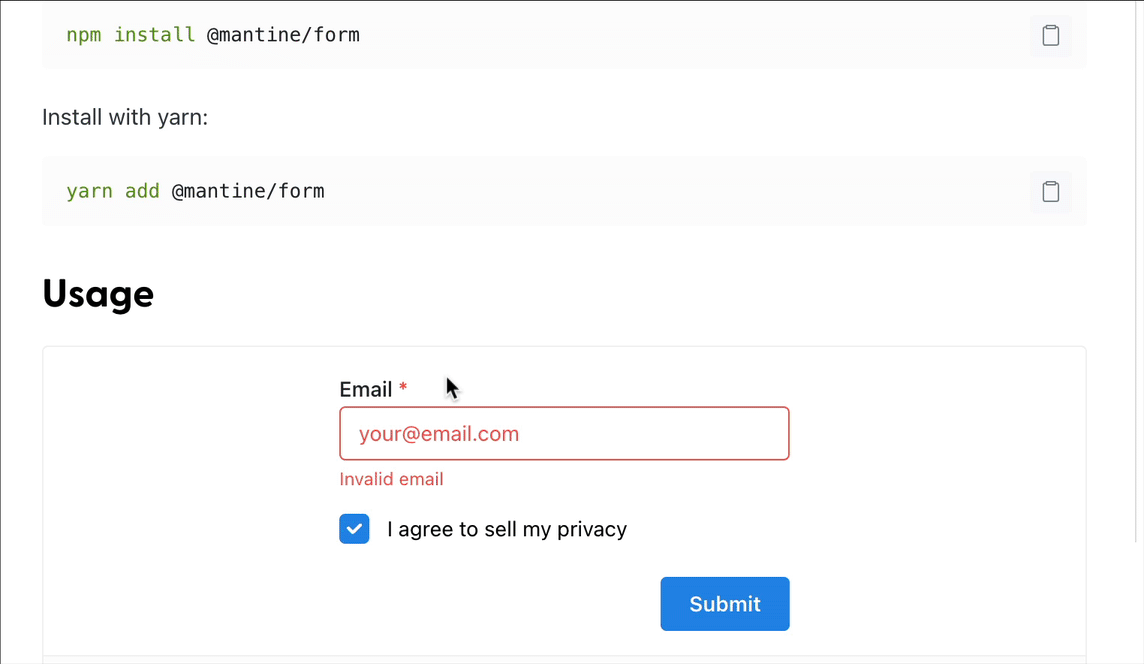 您如何獲取已輸入到表單中的值? Mantine 提供了一個非常方便的助手,叫做“getInputProps”,你只需提供你正在使用的輸入的名稱(比如電子郵件),它就會自動設置一個 onChange 來追蹤你在表單中輸入的值. 此外,為了處理表單提交,並在其值未通過驗證規則時阻止送出,它有一個特殊的 `onSubmit` 函數,您可以將其包裹在常規的 onSubmit 函數中。除了應用驗證規則之外,它還會負責在表單事件上呼叫 `preventDefault()`,這樣您就不必手動執行此操作。 我只用了這個鉤子的基本功能,但我強烈建議您在下一個專案中使用它。傳統上,表單很難正常工作,尤其是需要驗證和可見錯誤訊息的表單。 `useForm` 讓它變得異常簡單! --- 以上,簡單分享,希望對您有幫助。
練習1 ── alert 示警元件 https://jsfiddle.net/birdie2019/4ur1c7xf/34/ 練習2 ── toast 吐司元件 https://jsfiddle.net/birdie2019/fmc6b8ht/49/ 研究了一下setTimeout()用法,經過自己的消化吸收寫成筆記記錄下來 分享筆記:https://hackmd.io/@birdie/SJisAlTcs 另外發現display無法做transition的效果,改用opacity 練習3 ── modal 互動視窗元件 https://jsfiddle.net/birdie2019/9p3zsthL/17/ 練習4 ── 表單驗證 https://jsfiddle.net/birdie2019/bdavsur4/91/ 練習5 ── collapse 折疊效果 https://jsfiddle.net/birdie2019/dkncrv03/43/ 認識了scrollHeight屬性,建議可以用圖去搜尋會比較好理解這個屬性 也順便學了clientHeight和offsetHeight 練習6 ── dropdown 下拉式選單 https://jsfiddle.net/birdie2019/xb0ka495/44/ 研究window.onclick怎麼使用並認識了matches() 練習7 ── carousel 輪播元件 https://jsfiddle.net/birdie2019/t6uxb9df/30/ --- 此次教材心得分享: 一開始在腦袋裡會想很複雜,憑空亂想亂打code發現不是再刪掉再亂打,但其實不可能一下就到位,後來拿筆和紙先把需要的功能和自己的邏輯想法簡單的寫和畫下來,釐清自己現在需要怎麼做,方法有幾種,若這個方法不行,還可以用什麼方法可以做,這個方法看似很沒有效率,但真的對我幫助很大且完成此次所有作業。
### 第1課 ── 基本的函數操作、onclick 事件 https://jsfiddle.net/j80d9gao/17/ --- ### 第2課 ── 基本的變數操作、從 html 取得內容 https://jsfiddle.net/09v5oadu/23/ --- ### 第3課 ── 修改 html 的內容 https://jsfiddle.net/2gnuj6wf/47/ --- ### 第4課 ── 基本的陣列操作 https://jsfiddle.net/49qzdj2c/140/ --- ### 第5課 ── 基本的 if/else 條件流程控制 https://jsfiddle.net/8gn7co2v/2/ --- ### 第6課 ── 認識資料型態與轉換 https://jsfiddle.net/Lqjstd6a/ --- ### 第7課 ── 認識巢狀結構 https://jsfiddle.net/cz07a8nu/13/ ---
## 課程目標 認識並且能實做 modal 元件 ## 課程內容 modal 中文叫「互動視窗」元件,也有人叫「燈箱」或者「視窗」 這是幾乎所有網站都會用到的元件 台灣各大媒體網站常見的「蓋板廣告」,就算是一種「視窗」 各大社群網站,逛一逛就跳出「視窗」強迫你登入 點擊照片或者貼文,以前常常會開啟新網頁,現在常常設計成打開「視窗」直接就可以看內容 modal 跳出時,通常會將整個背景變成半透明黑色或白色,來讓視窗內容更顯眼 並且 modal 會有按鈕可以關閉 請上網搜尋 `popup modal ui` `lightbox ui`,四處觀摩一下業界常見的設計 --- 要寫出這種視窗的效果,其實 css 的部份還比 js 的部份難一些 請上網搜尋 `燈箱 css` `popup modal css` `popup modal css` 了解各種不同的寫法與技巧 半透明背景遮罩的英文術語叫 `black overlay` 請上網搜尋 `css 黑色半透明遮罩` `full page black overlay css` 了解各種如何做到此效果。不過,若是做不出來,這個效果也是可以省略不做 ## 課後作業 請使用 https://jsfiddle.net 用以下 html 為基礎(你可以稍微修改),接著寫出 css 與 js 的部份 ``` <button onclick="loginModal()">點我登入帳號</button> <hr> <button onclick="postModal()">點我新增貼文</button> <div class="modal" id="login-modal"> <div>帳號:<input type="text"></div> <div>密碼:<input type="password"></div> <hr> <button class="btn-close">關閉</button> </div> <div class="modal" id="post-modal"> <div>標題:<input type="text"></div> <div>內文:<textarea></textarea></div> <hr> <button class="btn-close">關閉</button> </div> ``` --- 這邊共有兩個按鈕,模擬兩種使用情境 兩個視窗元件,一開始預設是隱藏的,不會在畫面上看到 點擊登入按鈕,會跳出登入視窗。可以點擊視窗內的關閉按鈕,來將視窗關閉 點擊貼文按鈕,會跳出貼文視窗。可以點擊視窗內的關閉按鈕,來將視窗關閉 --- 請稍微加一些 css 屬性,弄得漂亮一點 `<hr>` 只是方便隔開元素,方便別人查看元件用的,醜醜的留在畫面上沒關係 --- 做出以上功能,你就完成這次的課程目標了!
## 課程目標 認識並且能實做 toast 元件 ## 課程內容 toast 中文叫「吐司」元件,就跟烤土司機,烤好吐司會跳出來一樣 用這元件來讓一些操作回饋訊息跳出,可以讓使用者體驗(User Experience,簡稱 UX)更好 很多網站因為沒有做好適當的 toast 元件,使用者在操作時常常覺得「表單到底有沒有送出?」「網站當機了是不是?」 通常要將 toast 做成幾種不同樣式,根據不同使用情境來使用 由於 toast 通常會直接蓋在網站的上方正中央(或者右上角、左下角,不一定) 會蓋到網頁畫面,所以通常會做成「顯示幾秒之後自動關閉」 請上網搜尋 `toast ui` `toast component`,四處觀摩一下業界常見的設計 --- 要在倒數幾秒之後執行任務,你需要 `setTimeout` 這個函式。請自行上網搜尋一下,了解這個函式的用法 ## 課後作業 請使用 https://jsfiddle.net 用以下 html 為基礎(你可以稍微修改),接著寫出 css 與 js 的部份 ``` <button onclick="toastSuccess()">成功訂購商品</button> <hr> <button onclick="toastError()">訂購商品失敗</button> <div class="toast toast-success"> 訂購成功!可以查看 Email 確認訂單細節! </div> <div class="toast toast-error"> 抱歉,訂購失敗!請稍後重新嘗試 </div> ``` --- 這邊共有兩個按鈕,模擬兩種使用情境 兩個吐司元件,一開始預設是隱藏的,不會在畫面上看到 請寫出 `toastSuccess` 函式的內容,在點擊按鈕後,將「成功吐司」顯示在畫面上 5 秒鐘,之後自動消失 請寫出 `toastError` 函式的內容,在點擊按鈕後,將「錯誤吐司」顯示在畫面上 5 秒鐘,之後自動消失 成功吐司請做成綠色 錯誤吐司請做成紅色 這個顏色變化,可以是邊框、背景顏色、字體顏色、影子顏色,都可以,你自由決定 --- 請稍微加一些 css 屬性,弄得漂亮一點 `<hr>` 只是方便隔開元素,方便別人查看元件用的,醜醜的留在畫面上沒關係 --- 做出以上功能,你就完成這次的課程目標了!
簡單複習一下,在這份教材中,我們學會了 - DOM 樹基本觀念 - DOM 樹新增、刪除 - DOM 樹找到元素的方法 - 基本的除錯技巧 - 動態加上 onclick 事件 - 操作 onchange 事件 - 基本的 for 迴圈用法 - 在 DOM 樹存取、修改元素的 class - 在 DOM 樹存取、修改元素的 style - 學會 `.querySelector()` `.querySelectorAll()` 的用法(Selector API) - 學會 `.closest()` 的用法 - 初步認識「重構」的觀念 利用本課程學到的技巧,你已能夠對 DOM 樹進行很多操作,也能夠處理工作上很多功能需求! DOM 的操作函式有非常多,本課只教了最基本的幾個,實務上你會看到超多尋找 DOM 元素的函式 你就隨便找、隨便用,都可以,能順利找到、處理元素就好! --- 觀察本課內容,你會發現完全沒有用到第三方套件,通通是寫原生 JavaScript 本課所學內容,其實就是所有前端框架的基礎。不管你用了任何複雜的工具或框架,它們的背後,其實就只是這樣操作 DOM 而已,並沒有很難 本課內容所教的開發方法,大概是西元 2000 - 2010 年代的開發方式,很傳統,現在比較少這樣寫 實務上,現代前端工程師,工作時主要在使用「框架」來開發,例如 Vue 或者 React 因此,坊間補習班,大多是直接從「現代前端框架」開始教 然而,我觀察到,大多數畢業學生,因此對於 DOM 基本觀念一知半解、覺得框架很黑箱,並且基本功非常不好 所以我課程設計先從老方法開始教,讓大家基本功比較好,接著再學工具與框架,會更有踏實的感覺 --- 消化、研究完本課程之後,關於 JavaScript 更多必學的基本觀念 請接著前往「自學網頁の嬰兒教材:JavaScript(三)」開始學習吧! https://codelove.tw/@howtomakeaturn/course/gqveaW
## 課程目標 能動態在 DOM 樹修改元素的 style 屬性 ## 課程內容 實務上有個功能很常見,就是元素開關(toggle)的效果 點一下讓某元素出現,再點一下讓某元素消失 例如:跳出一張照片顯示、再關閉這張照片;或者顯示提示訊息文字,點擊後關閉文字(不是 alert 那種喔,是更漂亮、用 html/css 實做的那種漂亮小框框訊息) 讓元素開開關關出現的情境有很多,該怎麼做到呢?其實,修改元素的 css 屬性 `display` 就可以了 ``` <button onclick="myFunction()">點我開開關關</button> <div id="myDIV"> 我會一下出現一下消失 </div> ``` ``` function myFunction() { var x = document.getElementById("myDIV"); if (x.style.display === "none") { x.style.display = "block"; } else { x.style.display = "none"; } } ``` 如果你不知道 css 的 display 是什麼,以及有哪些值可用,請去查詢一下 最簡單、常用的基本上是 ``` display: none; display: inline; display: block; display: inline-block; ``` 修改這個值,就能做到開關顯示元素的效果了 --- 目前為止,你應該發現,能用 JavaScript 動態修改 class,也能動態修改 style 感覺好像網頁上任何東西、html、css,都可以用 JavaScript 動態修改? 你猜得沒錯!在前端工程領域,就是幾乎什麼東西都能用 JavaScript 來操作,所以現代的各種網頁,才會那麼強大、那麼多功能! 如果有個東西你不知道怎麼改,但感覺應該能用 JavaScript 做到,那就上網搜尋一下,一定會找到屬性或者函式可以用的! ## 課後作業 接續前一課的作業,現在要進一步加強清單功能 你發現有些事項,完成之後,你不想要刪除掉,你想要留著,比較有成就感 但又需要知道哪些已完成,所以要有能備註完成與否的方法 --- 請在每個事項後面,加上 `(已完成)` 的文字,但是 display 為 none 所以先不顯示 然後在這文字後面,再加上「標示為已完成」的按鈕,點下去才顯示 `(已完成)` 文字 舉例來說,建立了三則待辦事項之後,看起來像這樣 ``` - 倒垃圾 [標示為已完成][刪除] - 繳電話費 [標示為已完成][刪除] - 採買本週食材 [標示為已完成][刪除] ``` 如果倒完垃圾了,就可以點擊按鈕,來備註已完成,這時才顯示 `(已完成)` 文字 ``` - 倒垃圾(已完成)[標示為未完成][刪除] - 繳電話費 [標示為已完成][刪除] - 採買本週食材 [標示為已完成][刪除] ``` 這時後面的按鈕就變成「標示為未完成」了 所以這個完成與否按鈕,就是一個開關(toggle),可以開開關關的 做出這個功能,你就完成這次的課程目標了!
## 課程目標 學會 onchange 事件的用法 ## 課程內容 目前為止,應該已經熟悉 `onclick` 事件的用法 接下來,學一個很常用、很好用的新事件 `onchange` ``` <select onchange="show1()"> <option value="apple">蘋果</option> <option value="banana">香蕉</option> </select> <label> <input type="radio" name="gender" value="male" onchange="show2()"> 男生 </label> <label> <input type="radio" name="gender" value="female" onchange="show2()"> 女生 </label> ``` ``` function show1() { alert(event.target.options[event.target.selectedIndex].value) } function show2() { alert(event.target.value) } ``` 到 jsfiddle 試試看,就會清楚實際效果囉! --- 不同的 html 元素,對於觸發 `onchange` 的定義有點不同,取得「變化後的新值」的方式也有點不同 上面簡單示範兩種。實務上,如果一直觸發不了,或是抓不到值的話,就多上網查查範例 然後一直嘗試 alert 或者 console.log 看看到底抓到什麼變數、到底變數內容是什麼 多實驗、多練習,習慣之後就會上手了 ## 課後作業 接續前一課的作業,現在要讓清單看起來更「花俏一點」 你希望選擇任務急迫性之後,輸入文字的框框,能跟著有樣式變化 --- 目前的 select 選單 選單內有「一般」、「重要」、「緊急」三種選項 請在選單加上 onchange 事件,讓選項改變的時候,輸入文字的框框,能跟著有樣式變化 請讓文字輸入框框,在選單為「重要」時,顯示為「橘色」;在選單為「緊急」時,顯示為「紅色」 這個顏色變化,可以是邊框、背景顏色、字體顏色、影子顏色,都可以,你自由決定 目的只是讓用戶有更多「視覺回饋」,讓用戶可以預期,新事項在清單內會變色而已 請在 `<input>` 或者在它父元素,加上不同的 class,用不同 class 顯示的不同顏色,來做到這點 做出這個功能,你就完成這次的課程目標了!
## 課程目標 能動態加上 onclick 事件屬性 能選取到父元素、子元素 能夠在 DOM 樹進行「移除」 能存取到 onclick 事件發生的元素 ## 課程內容 現在已經會在 DOM 樹增加元素、加入文字了 記得之前寫過的「事件」嗎? ``` <button onclick="myFunction()">Click me</button> ``` ``` function myFunction() { alert('你點擊了按鈕!'); } ``` 這當然也能用 JavaScript 動態加上去 ``` <button id="my-btn">Click me</button> ``` ``` function myFunction() { alert('你點擊了按鈕!'); } var btn = document.getElementById('my-btn'); btn.onclick = myFunction; ``` 在 JavaScript 裡面,像這樣定義的「函式」,它的「名稱」其實也會是一個「變數」 所以可以直接指派給 `.onclick` 屬性 覺得有點怪,沒關係,先知道可以這樣設定「事件屬性」就好 這種「把函式設定到事件屬性」的行為,我們叫「綁定」 因為在事件發生的時候,要固定執行任務,就像是綁定任務給你,固定由你來負責的感覺 --- 接著多談一下元素的父子關係 ``` <div id="app"> 這是簡介 <h1>這是標題</h1> <p id="para">這是段落文字</p> </div> ``` ``` var app = document.getElementById('app'); alert(app.children[0].textContent) alert(app.children[1].textContent) ``` 到 jsfiddle 執行看看,有看懂了嗎? 元素的 `.children` 屬性可以存取到子元素,以陣列的形式出現 別忘記陣列的索引是從 0 開始喔 接著試試這樣 ``` var para = document.getElementById('para'); alert(para.parentElement.textContent) ``` 元素的 `.parentElement` 屬性可以存取到父元素 這邊把父元素的整個內容跳出來看,像這樣跳一堆字,實務上沒什麼用 重點是,可以存取到父元素、子元素,就可以在整個 DOM 上上下下跑來跑去,用上一課教的 `.append()` 來到處新增元素啦! --- 接著,來學學如何刪除元素吧 繼續上面的範例,請在 jsfiddle 試試這段 ``` var para = document.getElementById('para'); para.remove(); ``` 然後試試這樣 ``` var app = document.getElementById('app'); app.remove(); ``` 很簡單、直觀吧! --- 最後,來學習怎麼抓到事件發生當下的元素 記得之前有學過一個 `document` 物件嗎? 這是一個 JavaScript 在瀏覽器中內建的「物件」,這個物件代表網頁「當下的狀態」,有很多資料、函數可以呼叫使用 現在來學另一個 `event` 物件,這個物件代表網頁「當下正在發生的事件」,也有很多資料、函數可以呼叫使用 ``` <button onclick="hello()">蘋果</button> <button onclick="hello()">香蕉</button> <button onclick="hello()">西瓜</button> ``` ``` function hello() { alert(event.target.textContent) } ``` 綁定函式到事件屬性之後,可以利用 `event.target` 來找到當前發生事件的元素 找到元素之後,就可以自由的存取元素屬性、或者對元素做任何你想做的事情囉! ## 課後作業 接續前一課的作業,這次要來實作「刪除事項」的功能 --- 請在事項的旁邊,加上刪除按鈕,請使用這樣的結構 ``` <ul> <li> <span>倒垃圾</span> <button>刪除</button> </li> <li> <span>繳電話費</span> <button>刪除</button> </li> <li> <span>採買本週食材</span> <button>刪除</button> </li> </ul> ``` 點擊「刪除」按鈕,會將事項刪除不見 如此一來,你的清單小程式,就可以新增事項、也可以刪除事項囉 請稍微替這個「刪除」按鈕加一點 css 屬性,弄得漂亮一點,看起來才專業 做出這個功能,你就完成這次的課程目標了!
簡單複習一下,在這份教材中,我們學會了 - 宣告變數 - 宣告函式 - 操作 onclick 事件 - 字串操作、數字操作 - 能從網頁元素取得資料 - 能更新網頁元素的內容 - if/else 條件流程控制 - 認識資料型態的差異與轉換 在任何學校、補習班,學習程式設計,不論程式語言,不論領域,有很多通用的基本觀念要學 本課程的內容,已讓你學會了其中的一大部份 我鼓勵你針對其中有興趣的部份,搜尋關鍵字,自行研究一下,有更多好用、有趣的東西可以學 --- 在程式設計中,一樣的任務,有多種寫法可以完成 在網頁開發中,更是如此。上網研究時,你會發現,在 JavaScript 中有很多函式、屬性,看起來根本目的重複了 為何會有這麼多種寫法?哪種是對的? 有人會說A才是正確做法;有人會說B才是正確做法 我個人建議是:通通都可以!隨便寫,你高興就好,能完成任務就好 各種做法的優劣之處,更有經驗之後,才能分得出差別 很多時候,根本就只是主觀偏好而已,沒有優劣之分 更多時候,都只是出於歷史因素: 「當年為了XXX的苦衷,設計成這樣。後來大環境改變,有了新的設計。但是要兼具新舊相容性,於是並存,導致各種用法看起來有點亂」 所以,你就隨便寫吧!能搞定、東西能跑,就對了! --- 消化、研究完本課程之後,關於 JavaScript 更多必學的基本觀念 請接著前往「自學網頁の嬰兒教材:JavaScript(二)」開始學習吧! https://codelove.tw/@howtomakeaturn/course/vx8gqZ
## 課程目標 學會基本的陣列取值 能從 html 元素中,得到用戶選取的內容 ## 課程內容 這課先來學一點陣列觀念 ``` var colors = ['red', 'orange', 'yellow', 'green']; ``` 中括號 `[]` 包起來就是宣告一個陣列,並記錄到 `colors` 變數中。此陣列內容是四個字串。 陣列索引從 0~3,因為在程式設計中,索引通常是從 0 開始而不是從 1 開始。 在陣列後方,使用中括號加上索引,就可取得陣列內容的值 ``` alert(colors[0]); alert(colors[1]); ``` 這兩個 alert 會顯示出陣列中第一個元素、第二個元素,馬上到 jsfiddle 試試看就會清楚了! --- 在前幾課,我們學會了取得 `<input type="text" />` 這種元素內容的方法 文字輸入框是最常用到的網頁功能,除此之外,下拉式選單也很常用 ``` <select id="my-colors"> <option value="red">鮮豔的紅色</option> <option value="orange">美麗的橘色</option> <option value="yellow">亮眼的黃色</option> </select> <button onclick="showColor()">Click me</button> ``` 像這樣的選單,如何取得用戶選取的值呢? ``` function showColor() { var menu = document.getElementById("my-colors"); var index = menu.selectedIndex; alert(index); var value = menu.options[index].value; var text = menu.options[index].text; alert(value); alert(text); } ``` 首先一樣用 `document.getElementById` 找到我們的選單元素 選單元素這種物件,會有 `.selectedIndex` 屬性來代表目前選中的索引 我們用 alert 先把索引跳出來看一下 同時,選單元素這種物件,會有 `.options` 屬性來代表其中的 `<option>` 元素,並且會是一個陣列 這個陣列裡面,通通都是物件,一個物件代表一個 `<option>` 元素 每個 `option` 物件,又有 `.value` 屬性可供存取,以及 `.text` 屬性可供存取 工程師可以根據需要選擇 `.value` 或 `.text` 來使用 我們在寫 html select 元素時,通常會在 value 放英文單字,然後 text 放清楚的中文說明 請在 jsfiddle 試試看上面的範例,實驗一下、玩玩看,就會清楚了! ## 課後作業 接續前一課的作業,你的「線上下單」頁面,目前會顯示訂單資訊,方便顧客確認 除了顧客名稱之外,這課的作業要加強下單功能、顯示更多訂單資訊 --- 除了輸入客戶名字的欄位之外,請用 `select` 元素加上一個選單,讓用戶可以選擇服裝的分類:男裝、女裝 接著再用 `select` 元素多做一個選單,讓用戶可以選擇服裝的類型:外套、上衣、下身 這樣顧客就知道這間「成衣批發工廠」,有提供哪些商品批發了! 接著要將顧客選擇的內容,顯示在訂單資訊裡面 類似這樣: ``` ---------- |您的訂單 | |顧客姓名:XXX| |服裝分類:XXX| |服裝類型:XXX| ---------- ``` XXX的地方預設是空白,在點擊訂購按鈕之後,跳出招呼訊息之後,就把XXX改為顧客輸入、選擇的內容 請替這個訂單資訊區塊加一些 css 屬性,弄得漂亮一點 做出以上功能,你就完成這次的課程目標了!
## 課程目標 學會基本的變數賦值 學會基本的字串連接 能從 html 元素中,得到用戶輸入的內容 ## 課程內容 在上一課的內容中,我們這樣來顯示訊息視窗 ``` alert('你點擊了按鈕!'); ``` 像這樣單引號包起來的內容 `'你點擊了按鈕!'` 我們叫「字串」,是一種資料類型 除了直接把字串當參數傳給函數之外,還可以先把字串紀錄在「變數」之中,方便重複使用 ``` var message = '你點擊了按鈕!'; alert(message); ``` 就跟函數名稱一樣,變數名稱是隨便命名的,取一個自己看得懂的名稱就行了 記錄到變數之後,就可以到處重複使用,不用每次手寫整段資料內容 不論是字串或者變數,都可以連接起來使用 ``` var text1 = '恭喜'; var text2 = '發財'; alert(text1 + text2); alert('萬事' + '如意'); ``` 除了「字串」這種資料類型之外,還有「數字」等等其他類型,之後課程會提到 --- 不論是購物網站、社群網站、各種網站,一定會經常需要用戶輸入的內容 有一個簡單的方法可以取得用戶輸入內容 ``` <input id="keyword" type="text" /> <button onclick="myFunction()">Click me</button> ``` ``` function myFunction() { var str = document.getElementById('keyword').value; alert(str); } ``` 函數中的 `document` 是一個 JavaScript 在瀏覽器中內建的「物件」,這個物件代表網頁當下的狀態,有很多資料、函數可以呼叫使用 後面的 `.` 代表去呼叫這個「物件」的「函數」,或者去存取這個「物件」的「屬性」 這邊的 `document.getElementById('keyword')` 代表以 `'keyword'` 為參數去呼叫 `getElementById`函數 這函數會去找出網頁中 id 為 `keyword` 的元素,元素在 JavaScript 中也是一種物件 後面的 `.value` 就是去存取元素的 value 屬性。各種輸入相關的元素,都會把用戶的輸入內容存在 `value` 這屬性之中 使用以上的幾段程式碼,可以輕鬆地取得用戶輸入的內容 請在 jsfiddle 試試看上面的範例,把輸入欄位與按鈕寫在 html 區塊,把函數定義寫在 JavaScript 區塊,實驗一下、玩玩看! ## 課後作業 接續第一課的作業,你的「線上下單」頁面,目前有個訂購按鈕 你對按下按鈕的導購訊息,不太滿意,你希望能顯示客戶的名字,看起來更專業 這課的作業要來改善這個「線上下單」頁面的功能 --- 請使用 jsfiddle,多加一個文字輸入欄位,讓客戶能輸入名字 在客戶點擊訂購按鈕之後,跳出的訊息會顯示: 「XXX您好!謝謝您對我們的衣服有興趣!請致電 0987-654-321,會有專人提供您報價!」 請稍微替這個輸入客戶名字的欄位加一些 css 屬性,弄得漂亮一點才專業 做出以上功能,你就完成這次的課程目標了!
## 課程目標 學會定義函數的基本方法 學會呼叫函數的基本方法 學會 onclick 事件的用法 ## 課程內容 請打開這個網站,用這個網站來練習&寫作業: https://jsfiddle.net 在學 html/css 時,我們會給元素一些屬性,例如 id 與 class 屬性,連結的 href 屬性,圖片的 src 屬性 同樣的語法,還可以用來做一些互動式效果、功能 例如,可以這樣在按鈕上做出「點擊後」觸發的效果 ``` <button onclick="myFunction()">Click me</button> ``` onclick 我們叫「事件屬性」。也是屬性的一種,語法跟一般屬性相同 屬性內容是去執行 `myFunction`,後面的小括號 `()` 代表執行的意思 這邊的 `myFunction` 是所謂的「函數」。名稱是隨便命名的。根據互動的內容,取一個自己看得懂的名稱就行了 小括號裡面可以傳參數,這邊我們沒用到參數,所以直接小括號包起來 `()` 就可以 如何定義 `myFunction` 函數的內容呢?這樣即可 ``` function myFunction() { alert('你點擊了按鈕!'); alert('這是很好的開始!'); } ``` 這個函數中,使用了 JavaScript 在瀏覽器中內建的 `alert` 函數。這函數會根據參數,跳出小視窗,把參數顯示在畫面上 在 JavaScript 中,一段動作的結尾都會加上分號 `;` 用來代表這段結束 分號其實可以省略不寫,程式一樣會正常執行,但加上分號,看起來更清楚一點點 有點像寫文章時結尾的「句點」,有人習慣寫,有人經常不寫,但加了比較清楚 請在 jsfiddle 試試看上面的範例,把按鈕寫在 html 區塊,把函數定義寫在 JavaScript 區塊,實驗一下、玩玩看! ## 課後作業 假設你家裡是開成衣批發工廠的,你用 html/css 寫了一個工廠的介紹網頁 在「線上下單」頁面,你希望引導客戶聯絡你,你親口介紹、聊過才報價、提高成交率 你不想把商品報價、聯絡方式直接顯示在畫面上,你希望是點擊按鈕之後,才顯示聯絡資訊 這次作業,需要你實作這個功能。 --- 請使用 jsfiddle,做一個「我要訂購」按鈕 點擊這個按鈕,會跳出 alert 訊息顯示「謝謝您對我們的衣服有興趣!請致電 0987-654-321,會有專人提供您報價!」 接著做一個「認識工廠」按鈕 點擊這個按鈕,會跳出 alert 訊息顯示「我們工廠位於新北市,通過國際 ISO9001 認證,品質讓您放心!」 請稍微替這兩個按鈕加一點 css 屬性,弄得漂亮一點,漂亮的按鈕,會讓客戶更想點 做出這兩個按鈕,你就完成這次的課程目標了!
React 作為一個強大的前端工具,有很多需要熟悉的基本觀念&語法。 這篇文章做一個快速的全面整理,方便工作時,可以隨時翻閱,希望您喜歡。 - 原文出處:https://dev.to/reedbarger/the-react-cheatsheet-for-2020-real-world-examples-4hgg ## 核心概念 ### 元素和 JSX - React 元素的基本語法 ``` // In a nutshell, JSX allows us to write HTML in our JS // JSX can use any valid html tags (i.e. div/span, h1-h6, form/input, etc) <div>Hello React</div> ``` - JSX 元素就是一段表達式 ``` // as an expression, JSX can be assigned to variables... const greeting = <div>Hello React</div>; const isNewToReact = true; // ... or can be displayed conditionally function sayGreeting() { if (isNewToReact) { // ... or returned from functions, etc. return greeting; // displays: Hello React } else { return <div>Hi again, React</div>; } } ``` - JSX 允許我們嵌套表達式 ``` const year = 2020; // we can insert primitive JS values in curly braces: {} const greeting = <div>Hello React in {year}</div>; // trying to insert objects will result in an error ``` - JSX 允許我們嵌套元素 ``` // to write JSX on multiple lines, wrap in parentheses: () const greeting = ( // div is the parent element <div> {/* h1 and p are child elements */} <h1>Hello!</h1> <p>Welcome to React</p> </div> ); // 'parents' and 'children' are how we describe JSX elements in relation // to one another, like we would talk about HTML elements ``` - HTML 和 JSX 的語法略有不同 ``` // Empty div is not <div></div> (HTML), but <div/> (JSX) <div/> // A single tag element like input is not <input> (HTML), but <input/> (JSX) <input name="email" /> // Attributes are written in camelcase for JSX (like JS variables <button className="submit-button">Submit</button> // not 'class' (HTML) ``` - 最基本的 React 應用程式需要三樣東西: - 1. ReactDOM.render() 渲染我們的應用程序 - 2. 一個 JSX 元素(在此情況下,稱為根節點) - 3. 一個用於掛載應用程式的 DOM 元素(通常是 index.html 文件中 id 為 root 的 div) ``` // imports needed if using NPM package; not if from CDN links import React from "react"; import ReactDOM from "react-dom"; const greeting = <h1>Hello React</h1>; // ReactDOM.render(root node, mounting point) ReactDOM.render(greeting, document.getElementById("root")); ``` ### 元件和 Props - 基本 React 元件的語法 ``` import React from "react"; // 1st component type: function component function Header() { // function components must be capitalized unlike normal JS functions // note the capitalized name here: 'Header' return <h1>Hello React</h1>; } // function components with arrow functions are also valid const Header = () => <h1>Hello React</h1>; // 2nd component type: class component // (classes are another type of function) class Header extends React.Component { // class components have more boilerplate (with extends and render method) render() { return <h1>Hello React</h1>; } } ``` - 如何使用元件 ``` // do we call these function components like normal functions? // No, to execute them and display the JSX they return... const Header = () => <h1>Hello React</h1>; // ...we use them as 'custom' JSX elements ReactDOM.render(<Header />, document.getElementById("root")); // renders: <h1>Hello React</h1> ``` - 元件可以在我們的應用程式中重複使用 ``` // for example, this Header component can be reused in any app page // this component shown for the '/' route function IndexPage() { return ( <div> <Header /> <Hero /> <Footer /> </div> ); } // shown for the '/about' route function AboutPage() { return ( <div> <Header /> <About /> <Testimonials /> <Footer /> </div> ); } ``` - 資料可以通過 props 動態傳遞給元件 ``` // What if we want to pass data to our component from a parent? // I.e. to pass a user's name to display in our Header? const username = "John"; // we add custom 'attributes' called props ReactDOM.render( <Header username={username} />, document.getElementById("root") ); // we called this prop 'username', but can use any valid JS identifier // props is the object that every component receives as an argument function Header(props) { // the props we make on the component (i.e. username) // become properties on the props object return <h1>Hello {props.username}</h1>; } ``` - Props 不可直接改變(mutate) ``` // Components must ideally be 'pure' functions. // That is, for every input, we be able to expect the same output // we cannot do the following with props: function Header(props) { // we cannot mutate the props object, we can only read from it props.username = "Doug"; return <h1>Hello {props.username}</h1>; } // But what if we want to modify a prop value that comes in? // That's where we would use state (see the useState section) ``` - 如果我們想將元素/元件作為 props 傳遞給其它元件,可以用 children props ``` // Can we accept React elements (or components) as props? // Yes, through a special property on the props object called 'children' function Layout(props) { return <div className="container">{props.children}</div>; } // The children prop is very useful for when you want the same // component (such as a Layout component) to wrap all other components: function IndexPage() { return ( <Layout> <Header /> <Hero /> <Footer /> </Layout> ); } // different page, but uses same Layout component (thanks to children prop) function AboutPage() { return ( <Layout> <About /> <Footer /> </Layout> ); } ``` - 可以用三元運算子來條件顯示元件 ``` // if-statements are fine to conditionally show , however... // ...only ternaries (seen below) allow us to insert these conditionals // in JSX, however function Header() { const isAuthenticated = checkAuth(); return ( <nav> <Logo /> {/* if isAuth is true, show AuthLinks. If false, Login */} {isAuthenticated ? <AuthLinks /> : <Login />} {/* if isAuth is true, show Greeting. If false, nothing. */} {isAuthenticated && <Greeting />} </nav> ); } ``` - Fragments 是用來顯示多個元件的特殊元件,無需向 DOM 添加額外的元素 - Fragments 適合用在條件邏輯 ``` // we can improve the logic in the previous example // if isAuthenticated is true, how do we display both AuthLinks and Greeting? function Header() { const isAuthenticated = checkAuth(); return ( <nav> <Logo /> {/* we can render both components with a fragment */} {/* fragments are very concise: <> </> */} {isAuthenticated ? ( <> <AuthLinks /> <Greeting /> </> ) : ( <Login /> )} </nav> ); } ``` ### 列表和 keys - 使用 .map() 將資料列表(陣列)轉換為元素列表 ``` const people = ["John", "Bob", "Fred"]; const peopleList = people.map(person => <p>{person}</p>); ``` - .map() 也可用來轉換為元件列表 ``` function App() { const people = ['John', 'Bob', 'Fred']; // can interpolate returned list of elements in {} return ( <ul> {/* we're passing each array element as props */} {people.map(person => <Person name={person} />} </ul> ); } function Person({ name }) { // gets 'name' prop using object destructuring return <p>this person's name is: {name}</p>; } ``` - 迭代的每個 React 元素都需要一個特殊的 `key` prop - key 對於 React 來說是必須的,以便能夠追蹤每個正在用 map 迭代的元素 - 沒有 key,當資料改變時,React 較難弄清楚元素應該如何更新 - key 應該是唯一值,才能讓 React 知道如何分辨 ``` function App() { const people = ['John', 'Bob', 'Fred']; return ( <ul> {/* keys need to be primitive values, ideally a generated id */} {people.map(person => <Person key={person} name={person} />)} </ul> ); } // If you don't have ids with your set of data or unique primitive values, // you can use the second parameter of .map() to get each elements index function App() { const people = ['John', 'Bob', 'Fred']; return ( <ul> {/* use array element index for key */} {people.map((person, i) => <Person key={i} name={person} />)} </ul> ); } ``` ### 事件和事件處理器 - React 和 HTML 中的事件略有不同 ``` // Note: most event handler functions start with 'handle' function handleToggleTheme() { // code to toggle app theme } // in html, onclick is all lowercase <button onclick="handleToggleTheme()"> Submit </button> // in JSX, onClick is camelcase, like attributes / props // we also pass a reference to the function with curly braces <button onClick={handleToggleTheme}> Submit </button> ``` - 最該先學的 React 事件是 onClick 和 onChange - onClick 處理 JSX 元素上的點擊事件(也就是按鈕動作) - onChange 處理鍵盤事件(也就是打字動作) ``` function App() { function handleChange(event) { // when passing the function to an event handler, like onChange // we get access to data about the event (an object) const inputText = event.target.value; const inputName = event.target.name; // myInput // we get the text typed in and other data from event.target } function handleSubmit() { // on click doesn't usually need event data } return ( <div> <input type="text" name="myInput" onChange={handleChange} /> <button onClick={handleSubmit}>Submit</button> </div> ); } ``` ## React Hooks ### State and useState - useState 為我們提供 function component 中的本地狀態 ``` import React from 'react'; // create state variable // syntax: const [stateVariable] = React.useState(defaultValue); function App() { const [language] = React.useState('javascript'); // we use array destructuring to declare state variable return <div>I am learning {language}</div>; } ``` - 注意:此段文章中的任何 hook 都來自 React 套件,且可以單獨導入 ``` import React, { useState } from "react"; function App() { const [language] = useState("javascript"); return <div>I am learning {language}</div>; } ``` - useState 還為我們提供了一個 `setter` 函數來更新它的狀態 ``` function App() { // the setter function is always the second destructured value const [language, setLanguage] = React.useState("python"); // the convention for the setter name is 'setStateVariable' return ( <div> {/* why use an arrow function here instead onClick={setterFn()} ? */} <button onClick={() => setLanguage("javascript")}> Change language to JS </button> {/* if not, setLanguage would be called immediately and not on click */} <p>I am now learning {language}</p> </div> ); } // note that whenever the setter function is called, the state updates, // and the App component re-renders to display the new state ``` - useState 可以在單個元件中使用一次或多次 ``` function App() { const [language, setLanguage] = React.useState("python"); const [yearsExperience, setYearsExperience] = React.useState(0); return ( <div> <button onClick={() => setLanguage("javascript")}> Change language to JS </button> <input type="number" value={yearsExperience} onChange={event => setYearsExperience(event.target.value)} /> <p>I am now learning {language}</p> <p>I have {yearsExperience} years of experience</p> </div> ); } ``` - useState 可以接受 primitive value 或物件 ``` // we have the option to organize state using whatever is the // most appropriate data type, according to the data we're tracking function App() { const [developer, setDeveloper] = React.useState({ language: "", yearsExperience: 0 }); function handleChangeYearsExperience(event) { const years = event.target.value; // we must pass in the previous state object we had with the spread operator setDeveloper({ ...developer, yearsExperience: years }); } return ( <div> {/* no need to get prev state here; we are replacing the entire object */} <button onClick={() => setDeveloper({ language: "javascript", yearsExperience: 0 }) } > Change language to JS </button> {/* we can also pass a reference to the function */} <input type="number" value={developer.yearsExperience} onChange={handleChangeYearsExperience} /> <p>I am now learning {developer.language}</p> <p>I have {developer.yearsExperience} years of experience</p> </div> ); } ``` - 如果新狀態依賴於之前的狀態,我們可以在 setter 函數中使用一個函數來為我們提供之前狀態的值 ``` function App() { const [developer, setDeveloper] = React.useState({ language: "", yearsExperience: 0, isEmployed: false }); function handleToggleEmployment(event) { // we get the previous state variable's value in the parameters // we can name 'prevState' however we like setDeveloper(prevState => { return { ...prevState, isEmployed: !prevState.isEmployed }; // it is essential to return the new state from this function }); } return ( <button onClick={handleToggleEmployment}>Toggle Employment Status</button> ); } ``` ### side effects 和 useEffect - useEffect 讓我們在 function component 中執行副作用。什麼是副作用? - 副作用是我們需要接觸外部世界的地方。例如,從 API 獲取資料或操作 DOM。 - 副作用是可能以不可預測的方式,改變我們元件狀態的操作(導致「副作用」)。 - useEffect 接受 callback function(稱為 effect 函數),預設情況下,每次重新渲染時都會運行 - useEffect 在我們的元件載入後運行,可以準確在元件的各個生命週期觸發 ``` // what does our code do? Picks a color from the colors array // and makes it the background color function App() { const [colorIndex, setColorIndex] = React.useState(0); const colors = ["blue", "green", "red", "orange"]; // we are performing a 'side effect' since we are working with an API // we are working with the DOM, a browser API outside of React useEffect(() => { document.body.style.backgroundColor = colors[colorIndex]; }); // whenever state is updated, App re-renders and useEffect runs function handleChangeIndex() { const next = colorIndex + 1 === colors.length ? 0 : colorIndex + 1; setColorIndex(next); } return <button onClick={handleChangeIndex}>Change background color</button>; } ``` - 如果不想在每次渲染後都執行 callback function,可以在第二個參數傳一個空陣列 ``` function App() { ... // now our button doesn't work no matter how many times we click it... useEffect(() => { document.body.style.backgroundColor = colors[colorIndex]; }, []); // the background color is only set once, upon mount // how do we not have the effect function run for every state update... // but still have it work whenever the button is clicked? return ( <button onClick={handleChangeIndex}> Change background color </button> ); } ``` - useEffect 讓我們能夠在 dependencies 陣列的內容改變時,才執行 - dependencies 陣列是第二個參數,如果陣列中的任何一個值發生變化,effect function 將再次運行 ``` function App() { const [colorIndex, setColorIndex] = React.useState(0); const colors = ["blue", "green", "red", "orange"]; // we add colorIndex to our dependencies array // when colorIndex changes, useEffect will execute the effect fn again useEffect(() => { document.body.style.backgroundColor = colors[colorIndex]; // when we use useEffect, we must think about what state values // we want our side effect to sync with }, [colorIndex]); function handleChangeIndex() { const next = colorIndex + 1 === colors.length ? 0 : colorIndex + 1; setColorIndex(next); } return <button onClick={handleChangeIndex}>Change background color</button>; } ``` - 可以在 useEffect 最後回傳一個函數,來取消訂閱某些效果 ``` function MouseTracker() { const [mousePosition, setMousePosition] = useState({ x: 0, y: 0 }); React.useEffect(() => { // .addEventListener() sets up an active listener... window.addEventListener("mousemove", handleMouseMove); // ...so when we navigate away from this page, it needs to be // removed to stop listening. Otherwise, it will try to set // state in a component that doesn't exist (causing an error) // We unsubscribe any subscriptions / listeners w/ this 'cleanup function' return () => { window.removeEventListener("mousemove", handleMouseMove); }; }, []); function handleMouseMove(event) { setMousePosition({ x: event.pageX, y: event.pageY }); } return ( <div> <h1>The current mouse position is:</h1> <p> X: {mousePosition.x}, Y: {mousePosition.y} </p> </div> ); } // Note: we could extract the reused logic in the callbacks to // their own function, but I believe this is more readable ``` - 使用 useEffect 撈取資料 - 請注意,如果要使用更簡潔的 async/awit 語法處理 promise,則需要建立一個單獨的函數(因為 effect callback function 不能是 async) ``` const endpoint = "https://api.github.com/users/codeartistryio"; // with promises: function App() { const [user, setUser] = React.useState(null); React.useEffect(() => { // promises work in callback fetch(endpoint) .then(response => response.json()) .then(data => setUser(data)); }, []); } // with async / await syntax for promise: function App() { const [user, setUser] = React.useState(null); // cannot make useEffect callback function async React.useEffect(() => { getUser(); }, []); // instead, use async / await in separate function, then call // function back in useEffect async function getUser() { const response = await fetch("https://api.github.com/codeartistryio"); const data = await response.json(); setUser(data); } } ``` ### 效能和 useCallback - useCallback 是一個用來提高元件性能的 hook - 如果你有一個經常重新渲染的元件,useCallback 可以防止元件內的 callback function 在每次元件重新渲染時都重新創建(導致元件重新運行) - useCallback 僅在其依賴項之一改變時重新運行 ``` // in Timer, we are calculating the date and putting it in state a lot // this results in a re-render for every state update // we had a function handleIncrementCount to increment the state 'count'... function Timer() { const [time, setTime] = React.useState(); const [count, setCount] = React.useState(0); // ... but unless we wrap it in useCallback, the function is // recreated for every single re-render (bad performance hit) // useCallback hook returns a callback that isn't recreated every time const inc = React.useCallback( function handleIncrementCount() { setCount(prevCount => prevCount + 1); }, // useCallback accepts a second arg of a dependencies array like useEffect // useCallback will only run if any dependency changes (here it's 'setCount') [setCount] ); React.useEffect(() => { const timeout = setTimeout(() => { const currentTime = JSON.stringify(new Date(Date.now())); setTime(currentTime); }, 300); return () => { clearTimeout(timeout); }; }, [time]); return ( <div> <p>The current time is: {time}</p> <p>Count: {count}</p> <button onClick={inc}>+</button> </div> ); } ``` ### Memoization 和 useMemo - useMemo 和 useCallback 非常相似,都是為了提高效能。但就不是為了暫存 callback function,而是為了暫存耗費效能的運算結果 - useMemo 允許我們「記憶」已經為某些輸入進行了昂貴計算的結果(之後就不用重新計算了) - useMemo 從計算中回傳一個值,而不是 callback 函數(但可以是一個函數) ``` // useMemo is useful when we need a lot of computing resources // to perform an operation, but don't want to repeat it on each re-render function App() { // state to select a word in 'words' array below const [wordIndex, setWordIndex] = useState(0); // state for counter const [count, setCount] = useState(0); // words we'll use to calculate letter count const words = ["i", "am", "learning", "react"]; const word = words[wordIndex]; function getLetterCount(word) { // we mimic expensive calculation with a very long (unnecessary) loop let i = 0; while (i < 1000000) i++; return word.length; } // Memoize expensive function to return previous value if input was the same // only perform calculation if new word without a cached value const letterCount = React.useMemo(() => getLetterCount(word), [word]); // if calculation was done without useMemo, like so: // const letterCount = getLetterCount(word); // there would be a delay in updating the counter // we would have to wait for the expensive function to finish function handleChangeIndex() { // flip from one word in the array to the next const next = wordIndex + 1 === words.length ? 0 : wordIndex + 1; setWordIndex(next); } return ( <div> <p> {word} has {letterCount} letters </p> <button onClick={handleChangeIndex}>Next word</button> <p>Counter: {count}</p> <button onClick={() => setCount(count + 1)}>+</button> </div> ); } ``` ### Refs 和 useRef - Refs 是所有 React 組件都可用的特殊屬性。允許我們在元件安裝時,創建針對特定元素/元件的引用 - useRef 允許我們輕鬆使用 React refs - 我們在元件開頭呼叫 useRef,並將回傳值附加到元素的 ref 屬性來引用它 - 一旦我們創建了一個引用,就可以用它來改變元素的屬性,或者可以呼叫該元素上的任何可用方法(比如用 .focus() 來聚焦) ``` function App() { const [query, setQuery] = React.useState("react hooks"); // we can pass useRef a default value // we don't need it here, so we pass in null to ref an empty object const searchInput = useRef(null); function handleClearSearch() { // current references the text input once App mounts searchInput.current.value = ""; // useRef can store basically any value in its .current property searchInput.current.focus(); } return ( <form> <input type="text" onChange={event => setQuery(event.target.value)} ref={searchInput} /> <button type="submit">Search</button> <button type="button" onClick={handleClearSearch}> Clear </button> </form> ); } ``` ## 進階 Hook ### Context 和 useContext - 在 React 中,盡量避免創建多個 props 來將資料從父元件向下傳遞兩個或多個層級 ``` // Context helps us avoid creating multiple duplicate props // This pattern is also called props drilling: function App() { // we want to pass user data down to Header const [user] = React.useState({ name: "Fred" }); return ( // first 'user' prop <Main user={user} /> ); } const Main = ({ user }) => ( <> {/* second 'user' prop */} <Header user={user} /> <div>Main app content...</div> </> ); const Header = ({ user }) => <header>Welcome, {user.name}!</header>; ``` - Context 有助於將 props 從父組件向下傳遞到多個層級的子元件 ``` // Here is the previous example rewritten with Context // First we create context, where we can pass in default values const UserContext = React.createContext(); // we call this 'UserContext' because that's what data we're passing down function App() { // we want to pass user data down to Header const [user] = React.useState({ name: "Fred" }); return ( {/* we wrap the parent component with the provider property */} {/* we pass data down the computer tree w/ value prop */} <UserContext.Provider value={user}> <Main /> </UserContext.Provider> ); } const Main = () => ( <> <Header /> <div>Main app content...</div> </> ); // we can remove the two 'user' props, we can just use consumer // to consume the data where we need it const Header = () => ( {/* we use this pattern called render props to get access to the data*/} <UserContext.Consumer> {user => <header>Welcome, {user.name}!</header>} </UserContext.Consumer> ); ``` - useContext hook 可以移除這種看起來很怪的 props 渲染模式,同時又能在各種 function component 中隨意使用 ``` const Header = () => { // we pass in the entire context object to consume it const user = React.useContext(UserContext); // and we can remove the Consumer tags return <header>Welcome, {user.name}!</header>; }; ``` ### Reducers 和 useReducer - Reducers 是簡單、可預測的(純)函數,它接受一個先前的狀態物件和一個動作物件,並回傳一個新的狀態物件。例如: ``` // let's say this reducer manages user state in our app: function reducer(state, action) { // reducers often use a switch statement to update state // in one way or another based on the action's type property switch (action.type) { // if action.type has the string 'LOGIN' on it case "LOGIN": // we get data from the payload object on action return { username: action.payload.username, isAuth: true }; case "SIGNOUT": return { username: "", isAuth: false }; default: // if no case matches, return previous state return state; } } ``` - Reducers 是一種強大的狀態管理模式,用於流行的 Redux 狀態管理套件(這套很常與 React 一起使用) - 與 useState(用於本地元件狀態)相比,Reducers 可以通過 useReducer hook 在 React 中使用,以便管理我們應用程序的狀態 - useReducer 可以與 useContext 配對來管理資料並輕鬆地將資料傳遞給元件 - useReducer + useContext 可以當成應用程式的整套狀態管理系統 ``` const initialState = { username: "", isAuth: false }; function reducer(state, action) { switch (action.type) { case "LOGIN": return { username: action.payload.username, isAuth: true }; case "SIGNOUT": // could also spread in initialState here return { username: "", isAuth: false }; default: return state; } } function App() { // useReducer requires a reducer function to use and an initialState const [state, dispatch] = useReducer(reducer, initialState); // we get the current result of the reducer on 'state' // we use dispatch to 'dispatch' actions, to run our reducer // with the data it needs (the action object) function handleLogin() { dispatch({ type: "LOGIN", payload: { username: "Ted" } }); } function handleSignout() { dispatch({ type: "SIGNOUT" }); } return ( <> Current user: {state.username}, isAuthenticated: {state.isAuth} <button onClick={handleLogin}>Login</button> <button onClick={handleSignout}>Signout</button> </> ); } ``` ### 編寫 custom hooks - 創建 hook 就能輕鬆在元件之間重用某些行為 - hook 是一種比以前的 class component 更容易理解的模式,例如 higher-order components 或者 render props - 根據需要,隨時可以自創一些 hook ``` // here's a custom hook that is used to fetch data from an API function useAPI(endpoint) { const [value, setValue] = React.useState([]); React.useEffect(() => { getData(); }, []); async function getData() { const response = await fetch(endpoint); const data = await response.json(); setValue(data); }; return value; }; // this is a working example! try it yourself (i.e. in codesandbox.io) function App() { const todos = useAPI("https://todos-dsequjaojf.now.sh/todos"); return ( <ul> {todos.map(todo => <li key={todo.id}>{todo.text}</li>)} </ul> ); } ``` ### Hooks 規則 - 使用 React hooks 有兩個核心規則,要遵守才能正常運作: - 1. Hooks 只能在元件開頭呼叫 - Hooks 不能放在條件、迴圈或嵌套函數中 - 2. Hooks只能在 function component 內部使用 - Hooks 不能放在普通的 JavaScript 函數或 class component 中 ``` function checkAuth() { // Rule 2 Violated! Hooks cannot be used in normal functions, only components React.useEffect(() => { getUser(); }, []); } function App() { // this is the only validly executed hook in this component const [user, setUser] = React.useState(null); // Rule 1 violated! Hooks cannot be used within conditionals (or loops) if (!user) { React.useEffect(() => { setUser({ isAuth: false }); // if you want to conditionally execute an effect, use the // dependencies array for useEffect }, []); } checkAuth(); // Rule 1 violated! Hooks cannot be used in nested functions return <div onClick={() => React.useMemo(() => doStuff(), [])}>Our app</div>; } ``` --- 以上是快速整理,希望對您有幫助!
面試的時候,常常會被問到這題。看似簡單,其實有一些進階注意事項,此篇與您分享。 - 原文出處:https://dev.to/andyrewlee/stand-out-in-a-react-interview-by-rendering-a-list-like-a-pro-1cn5 # 基本做法 以下是最基本做法。根據陣列內容,直接渲染成一個列表。 ``` import { useState, useEffect } from "react"; const App = () => { const [posts, setPosts] = useState([]); const [currentPost, setCurrentPost] = useState(undefined); useEffect(() => { const initialize = async () => { const res = await fetch("https://jsonplaceholder.typicode.com/posts"); const json = await res.json(); setPosts(json); }; initialize(); }, []); const onPostClick = (post) => { setCurrentPost(post); }; return ( <div> {currentPost && <h1>{currentPost.title}</h1>} <PostList posts={posts} onPostClick={onPostClick} /> </div> ); }; const PostList = ({ posts, onPostClick }) => { return ( <div> {posts.map((post) => ( <Post post={post} onPostClick={onPostClick} /> ))} </div> ); }; const Post = ({ post, onPostClick }) => { const onClick = () => { onPostClick(post); }; return <div onClick={onClick}>{post.title}</div>; }; export default App; ``` # 改進方法 以下是四個可以改進的方向,以及背後的原因。 ## 1. 指定 key 替每個元件提供一個 key 屬性,可以幫助後續 React 渲染時改善效能。要注意 key 屬性要是唯一值,不要直接用陣列索引當成 key。 ``` {posts.map((post) => ( <Post key={post.id} post={post} onPostClick={onPostClick} /> ))} ``` ## 2. 優化渲染 每次點擊列表項目時,都會重新渲染 `PostList` 和每個 `Post`。 ``` const Post = ({ post, onPostClick }) => { console.log("post rendered"); const onClick = () => { onPostClick(post); }; return <div onClick={onClick}>{post}</div>; }; ``` 可以使用 React 提供的 `memo` 功能來優化 `PostList` 元件。用 `memo` 包裝元件時,等於告訴 React:除非 props 改變,否則不要重新渲染這個元件。 ``` import { useState, useEffect, memo } from "react"; const PostList = memo(({ posts, onPostClick }) => { return ( <div> {posts.map((post) => ( <Post post={post} onPostClick={onPostClick} /> ))} </div> ); }); ``` 不過,實際跑下去,會發現還是重新渲染了。因為每次 currentPost 改變時,App 都會重新渲染。每次重新渲染都會重新建立 onPostClick 函數。當一個函數被重新建立時(即使函式內容一樣),都算是新的實體。所以技術上來說,props 確實發生了變化,所以 `PostList` 會重新渲染。 ``` const fn1 = () => {}; const fn2 = () => {}; fn1 === fn2; // => false ``` 這種狀況,就可以使用 useCallback hook 來告訴 React 不要重新建立函數。 ``` const onPostClick = useCallback((post) => { setCurrentPost(post); }, []); ``` 上面的例子中,使用 useCallback 沒問題,可以避免全部貼文被重新渲染。但要知道的是,並非什麼函式都適合包在 useCallback 裡面。 ``` const Post = ({ post, onPostClick }) => { const useCalllback(onClick = () => { onPostClick(post); }, []); return <div onClick={onClick}>{post.title}</div>; }; ``` 比方說,在 Post 元件使用 useCallback 就不太有意義。因為這個元件是輕量級的。應該只在有意義的情況下使用 useCallback(可以用 profiling 工具分析效能確認)。`useCallback` 有缺點:它增加了程式碼的複雜性。使用 useCallback 會在每次渲染時運行的額外程式碼。 ## 3. 元件卸載時清理乾淨 目前的元件沒有在卸載時進行清理。例如,如果在收到 URL 回應結果之前就離開頁面怎麼辦?這時應該要取消請求。 ``` useEffect(() => { const initialize = async () => { const res = await fetch("https://jsonplaceholder.typicode.com/posts"); const json = await res.json(); setPosts(json); }; initialize(); }, []); ``` `useEffect` 可以分為兩部分:掛載時運行的程式碼、卸載時運行的程式碼: ``` useEffect(() => { // When component mounts what code should I run? return () => { // When component unmounts what code should I run (clean up)? }; }, []); ``` 我們可以使用 AbortController ,並在清理時呼叫 controller.abort() 來取消請求。 ``` useEffect(() => { const controller = new AbortController(); const signal = controller.signal; const initialize = async () => { try { const res = await fetch("https://jsonplaceholder.typicode.com/posts", { signal }); const json = await res.json(); setPosts(json); } catch (err) { console.log(err); } }; initialize(); return () => { controller.abort(); }; }, []); ``` ## 4. 增加無障礙輔助功能 以上範例太過簡單,沒有太多無障礙功能可以加入。一旦應用程式變複雜,絕對應該要開始考慮這點。 有個簡單的無障礙測試:可以單獨只使用鍵盤就操作一個應用程式嗎? 關於這點,快速解法是:把每個項目都轉成按鈕,用鍵盤時就可以在元件之間切換。 ``` const Post = ({ post, onPostClick }) => { const onClick = () => { onPostClick(post); }; return <button onClick={onClick}>{post}</button>; }; ``` # 結論 在 React 中渲染列表,乍看之下,只是個簡單的面試問題。但其實可深可淺。下次面試時遇到這問題,可以思考一下此篇文章談到的各個面向。
你的轉職路上,還缺少一份自學作業包!寫完這幾包,直接拿作品去面試上班!
本論壇另有附設一個 LINE 新手發問&交流群組!歡迎加入討論!