63 個專案實戰,寫出作品集,讓面試官眼前一亮!
您是否曾經花費數小時調整設計、嘗試不同的風格或瀏覽網站尋找靈感? 無論您是試圖獲得正確外觀的開發人員,還是完善顏色和陰影的設計師,UI 設計過程都感覺永無止境。 即使[GitHub Copilot](https://github.com/features/copilot)可以幫助您更快地...
JavaScript 系列八:第1課 ── 碼表應用程式 https://n-siang.github.io/VuePractice8/ JavaScript 系列八:第2課 ── 筆記應用程式 https://n-siang.github.io/VuePractice8/#/Vue8of...
“你正在建造的東西是開發者的聖杯。到目前為止還沒有人成功過。” 🏆☠️ ----------------------------------- ](https:/...
JavaScript 總是不斷在改變。有些模式持續存在,有些模式會逐漸消失,有些模式會演變成我們從未見過的東西。 以下是 JavaScript 模式的**細分**。 ### 1.**模式匹配(早期提案階段,但很有前景)** 想想`switch`語句——但要更好。模式匹配受到 Has...
JavaScript 系列七:第1課 ── 認識 Vue 基本環境與 render state https://jsfiddle.net/wang_siang/gLcjdph5/2/ JavaScript 系列七:第2課 ── 體驗一下 Reactivity 的效果與便利 https://...
JavaScript 系列六:第1課 ── 認識 data model 與 render function https://jsfiddle.net/wang_siang/vjdrf3uL/ JavaScript 系列六:第2課 ── 認識陣列操作 https://jsfiddle.net...
JavaScript 系列五:第1課 ── 學會 Cookie 相關功能: https://replit.com/@s28688117/js5-1?v=1#index.html JavaScript 系列五:第2課 ── 學會 Local Storage 相關功能: https://jsf...
程式碼審查是建立優秀軟體中最被低估的部分之一。 這一開始可能看起來困難和混亂,但實際上比你想像中的簡單得多。 這篇文章簡要概述了11種實用的方法和策略,以協助開發者進行程式碼審查。 這將幫助你提高程式碼審查的能力。 --- ## 🎯 什麼是程式碼審查? 在深入探討...
您是否曾註意到網頁在執行繁重任務時會凍結?發生這種情況是因為 JavaScript 預設在單執行緒上執行,導致了糟糕的使用者體驗。使用者無法交互,必須等到任務完成。這個問題可以透過使用 Web Worker 來解決。在本文中,我們將透過建立圖像壓縮應用程式,討論什麼是 Web Worker、為什麼它...
編寫乾淨的程式碼是任何開發人員的必備技能。乾淨的程式碼不僅僅是讓你的程式碼可以工作,它還意味著讓它優雅、有效率地工作,並且讓其他開發人員(包括你未來的自己)可以輕鬆理解和維護。在本綜合指南中,我們將探討編寫乾淨 JavaScript 程式碼的原則和最佳實務。 什麼是乾淨程式碼? ------...
JavaScript 系列四:第1課 ── autosize 套件: https://jsfiddle.net/wang_siang/53r61j0p/2/ JavaScript 系列四:第2課 ── vanilla-lazyload 套件 https://jsfiddle.net/wan...
JavaScript 系列三:練習1 ── alert 示警元件: https://jsfiddle.net/wang_siang/vx82au4k/1/ JavaScript 系列三:練習2 ── toast 吐司元件: https://jsfiddle.net/wang_sia...
我在科技社群裡最喜歡的部分就是開源專案的存在。 世界各地的人們為有價值的專案做出貢獻並免費提供這些專案,這真是太酷了。 在這篇部落格中,我們將介紹 11 個您現在應該查看的令人興奮的 GitHub 儲存庫。 讓我們立即開始吧!  ...
歡迎來到高級 JavaScript 的世界!無論您是希望提高技能的經驗豐富的開發人員,還是渴望深入了解 JavaScript 複雜性的愛好者,本部落格旨在啟發和教育您。讓我們探索 20 個高級 JavaScript 技巧,這些技巧不僅可以增強您的編碼能力,還可以在您發現優化程式碼的新穎且令人興奮的方...
*揭露:本貼文包含附屬連結;如果您透過本文提供的不同連結購買產品或服務,我可能會收到報酬。* 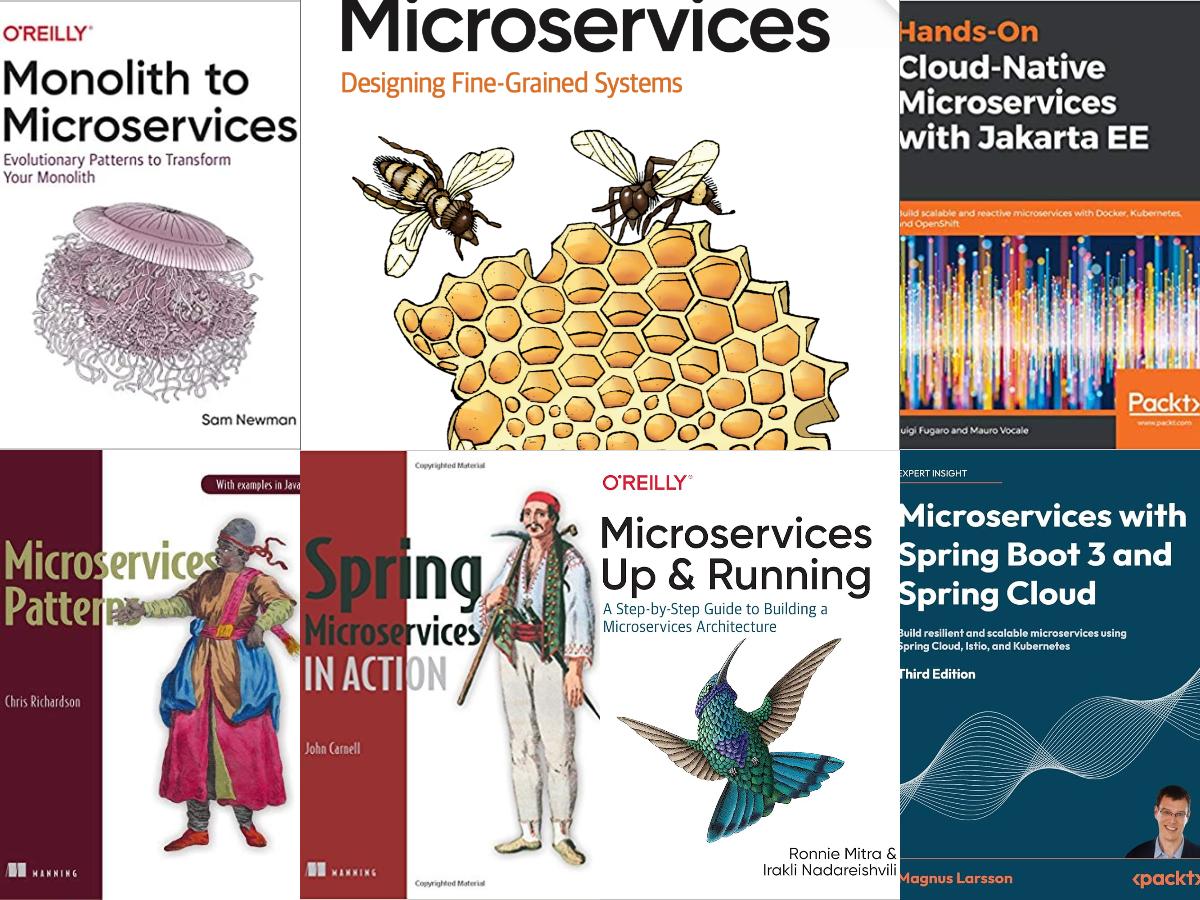 ...
大家好!在本文中我將描述建立 Gallery 應用程式的過程。您可以放心地使用此應用程式並按您的意願進行編輯(您只能在那裡更改圖片,因為有許可證)。雖然功能不多,但是我認為,它非常適合用作工作範例。 💻 該應用程式是什麼樣的,它的功能是什麼? --------------------- ...
JavaScript 系列二:第1課 ── 認識 DOM 樹、新增元素: https://jsfiddle.net/wang_siang/ywvsm36x/3/ JavaScript 系列二:第2課 ── 從 DOM 樹移除元素、動態加上 onclick 事件: https://jsfiddl...
精選技術文章、免費程式設計資源、以及業界重要新聞!
也歡迎訂閱 YouTube 頻道,觀看每週二晚間的《CodeLove Talk》直播節目,一起討論軟體開發相關的話題!